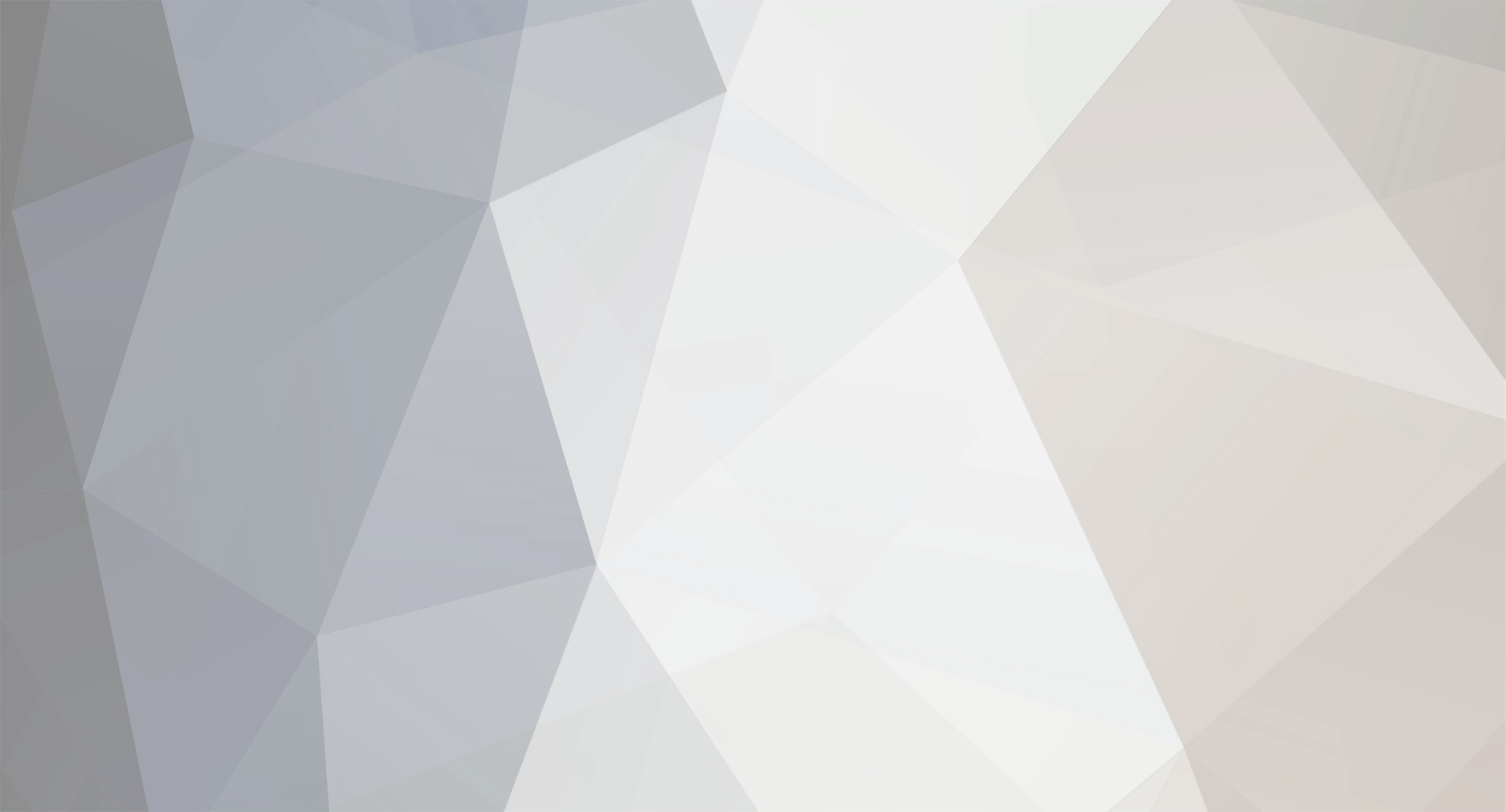
ashtonr12
-
Posts
479 -
Joined
-
Last visited
Posts posted by ashtonr12
-
-
ok so now the item loses health until its got 1 left then just stays in the inventory, ie doesnt break?
public void onUpdate(ItemStack stack, World world, Entity entity, int par4, boolean par5) { if(stack.getItemDamage() == 1) { stack.itemID = 522; }else if(stack.getItemDamage() > 1 ){ stack.setItemDamage(stack.getItemDamage() +1); }else{ stack.setItemDamage(20); } }
-
ignore i just figured out hte answe to my own question.
-
how would i make it so that when entityplayer holds itemPike the players reach extends by say 3 blocks?
-
i found this but i doesnt seem to work and im not good enough to manipulate it, this currently as soon as its gotten dmg value goes straight to one the all the way up to its max the crashes the game, what i would like it to do it when held go down from the highest value until it breaks. if you could explain to me how to do this that would be great.
public void onUpdate(ItemStack stack, World world, Entity entity, int par4, boolean par5) { if(stack.getItemDamage() == 1) { stack.itemID = 1; }else if(stack.getItemDamage() > 1 ){ stack.setItemDamage(stack.getItemDamage() - 1); }else{ stack.setItemDamage(20); } }
-
Someone who understands my point of view! MARRY ME!
kiddding but hey thankyou!
-
if i wanted to make an item decay upon being held how would i do this?
i have already tried a few methods to no avail and i cant find any good/ uptodate tutorials for it.
i was wondeing if anyone could help me with this, i am assuming i will have to use the servertick somehow.
-
i cant figure it out, i have tried so many numbers... i will just make the recipie not use it
-
so its not 8226 for swiftness 2?
-
ItemStack swiftness2 = new ItemStack(Item.potion, 1, 8226);
ItemStack diamond = new ItemStack(Item.diamond);
GameRegistry.addRecipe(new ItemStack(LSboots), "DDD","SPS","LPL",'D', diamond,'L', ashtonsmod.SuppleObsidian,'S', ashtonsmod.LightLeather,'P', swiftness2);
-
so can someone help me make the ore that spawns in sand spawn higher? like on surface sand in deserts and stuff? not just lower down?
i need to do this without raising the other two ores up and the y coord specifications are written before the ores are set.
package ashtonsmod.common; import java.util.Random; import net.minecraft.world.World; import net.minecraft.world.chunk.IChunkProvider; import net.minecraft.world.gen.feature.WorldGenMinable; import cpw.mods.fml.common.IWorldGenerator; public class WorldGen implements IWorldGenerator{ private static final int BlockX = 0; private int BlockZ; @Override public void generate(Random random, int chunkX, int chunkZ, World world,IChunkProvider chunkGenerator, IChunkProvider chunkProvider){ switch(world.provider.dimensionId){ case 1: generateNether(world, random, chunkX * 16, chunkZ * 16); case 0: generateSurface(world, random, chunkX * 16, chunkZ * 16); case -1: generateEnd(world, random, chunkX * 16, chunkZ * 16); } } private void generateNether(World world, Random random, int chunkX, int chunkZ) { } private void generateEnd(World world, Random random, int chunkX, int chunkZ){ } private void generateSurface(World world, Random random, int chunkX,int chunkZ){ int xCoord = chunkX; int yCoord = random.nextInt(64); int zCoord = chunkZ; (new WorldGenMinable(ashtonsmod.LightSteelOre.blockID, 7)).generate(world, random, (xCoord + random.nextInt(60)), yCoord, (zCoord + random.nextInt(60))); (new WorldGenMinable(ashtonsmod.AmethystOre.blockID, 5)).generate(world, random, (xCoord + random.nextInt(60)), yCoord, (zCoord + random.nextInt(80))); (new WorldGenMinableSand(ashtonsmod.AmethystOre.blockID, 15)).generate(world, random, (xCoord + random.nextInt(40)), yCoord, (zCoord + random.nextInt(40))); } }
-
yes thankyou i didnt realise it was a metadata item stupidly and couldnt figure out how to do that
thankyou
-
how do you us a potion in a crafting recipe?
-
so can someone help me make the ore that spawns in sand spawn higher? like on surface sand in deserts and stuff? not just lower down?
i need to do this without raising the other two ores up and the y coord specifications are written before the ores are set.
package ashtonsmod.common; import java.util.Random; import net.minecraft.world.World; import net.minecraft.world.chunk.IChunkProvider; import net.minecraft.world.gen.feature.WorldGenMinable; import cpw.mods.fml.common.IWorldGenerator; public class WorldGen implements IWorldGenerator{ private static final int BlockX = 0; private int BlockZ; @Override public void generate(Random random, int chunkX, int chunkZ, World world,IChunkProvider chunkGenerator, IChunkProvider chunkProvider){ switch(world.provider.dimensionId){ case 1: generateNether(world, random, chunkX * 16, chunkZ * 16); case 0: generateSurface(world, random, chunkX * 16, chunkZ * 16); case -1: generateEnd(world, random, chunkX * 16, chunkZ * 16); } } private void generateNether(World world, Random random, int chunkX, int chunkZ) { } private void generateEnd(World world, Random random, int chunkX, int chunkZ){ } private void generateSurface(World world, Random random, int chunkX,int chunkZ){ int xCoord = chunkX; int yCoord = random.nextInt(64); int zCoord = chunkZ; (new WorldGenMinable(ashtonsmod.LightSteelOre.blockID, 7)).generate(world, random, (xCoord + random.nextInt(60)), yCoord, (zCoord + random.nextInt(60))); (new WorldGenMinable(ashtonsmod.AmethystOre.blockID, 5)).generate(world, random, (xCoord + random.nextInt(60)), yCoord, (zCoord + random.nextInt(80))); (new WorldGenMinableSand(ashtonsmod.AmethystOre.blockID, 15)).generate(world, random, (xCoord + random.nextInt(40)), yCoord, (zCoord + random.nextInt(40))); } }
-
try and follow this,
1. assume i have a complete and utter perfect understanding of java.
2. therefore with a little experimentation i could code anything i liked into minecraft with no need from this forum.
3.therefore this forum is for people who do NOT have a complete understanding of java and cannot code anything they like into minecraft with no help.
4. therefore you should stop telling me to go learn more java because i came here because i didnt know enough and am seeking help.
-
he has update, he is using setUnlocalised name instead of setblockName
-
ok so this now throws no errors but im not sure if it works or not as i cannot find any ore in the sand.
minable sand class;
package ashtonsmod.common; import java.util.Random; import net.minecraft.block.Block; import net.minecraft.util.MathHelper; import net.minecraft.world.World; import net.minecraft.world.gen.feature.WorldGenerator; public class WorldGenMinableSand extends WorldGenerator { /** The block ID of the ore to be placed using this generator. */ private int minableBlockId; private int minableBlockMeta = 0; /** The number of blocks to generate. */ private int numberOfBlocks; public WorldGenMinableSand(int par1, int par2) { this.minableBlockId = par1; this.numberOfBlocks = par2; } public WorldGenMinableSand(int id, int meta, int number) { this(id, number); minableBlockMeta = meta; } public boolean generate(World par1World, Random par2Random, int par3, int par4, int par5) { float var6 = par2Random.nextFloat() * (float)Math.PI; double var7 = (double)((float)(par3 + + MathHelper.sin(var6) * (float)this.numberOfBlocks / 8.0F); double var9 = (double)((float)(par3 + - MathHelper.sin(var6) * (float)this.numberOfBlocks / 8.0F); double var11 = (double)((float)(par5 + + MathHelper.cos(var6) * (float)this.numberOfBlocks / 8.0F); double var13 = (double)((float)(par5 + - MathHelper.cos(var6) * (float)this.numberOfBlocks / 8.0F); double var15 = (double)(par4 + par2Random.nextInt(3) - 2); double var17 = (double)(par4 + par2Random.nextInt(3) - 2); for (int var19 = 0; var19 <= this.numberOfBlocks; ++var19) { double var20 = var7 + (var9 - var7) * (double)var19 / (double)this.numberOfBlocks; double var22 = var15 + (var17 - var15) * (double)var19 / (double)this.numberOfBlocks; double var24 = var11 + (var13 - var11) * (double)var19 / (double)this.numberOfBlocks; double var26 = par2Random.nextDouble() * (double)this.numberOfBlocks / 16.0D; double var28 = (double)(MathHelper.sin((float)var19 * (float)Math.PI / (float)this.numberOfBlocks) + 1.0F) * var26 + 1.0D; double var30 = (double)(MathHelper.sin((float)var19 * (float)Math.PI / (float)this.numberOfBlocks) + 1.0F) * var26 + 1.0D; int var32 = MathHelper.floor_double(var20 - var28 / 2.0D); int var33 = MathHelper.floor_double(var22 - var30 / 2.0D); int var34 = MathHelper.floor_double(var24 - var28 / 2.0D); int var35 = MathHelper.floor_double(var20 + var28 / 2.0D); int var36 = MathHelper.floor_double(var22 + var30 / 2.0D); int var37 = MathHelper.floor_double(var24 + var28 / 2.0D); for (int var38 = var32; var38 <= var35; ++var38) { double var39 = ((double)var38 + 0.5D - var20) / (var28 / 2.0D); if (var39 * var39 < 1.0D) { for (int var41 = var33; var41 <= var36; ++var41) { double var42 = ((double)var41 + 0.5D - var22) / (var30 / 2.0D); if (var39 * var39 + var42 * var42 < 1.0D) { for (int var44 = var34; var44 <= var37; ++var44) { double var45 = ((double)var44 + 0.5D - var24) / (var28 / 2.0D); Block block = Block.blocksList[par1World.getBlockId(var38, var41, var44)]; if (var39 * var39 + var42 * var42 + var45 * var45 < 1.0D && par1World.getBlockId(var38, var41, var44) == Block.sand.blockID) { par1World.setBlockAndMetadata(var38, var41, var44, this.minableBlockId, minableBlockMeta); } } } } } } } return true; } }
worldgen class;
package ashtonsmod.common; import java.util.Random; import net.minecraft.world.World; import net.minecraft.world.chunk.IChunkProvider; import net.minecraft.world.gen.feature.WorldGenMinable; import cpw.mods.fml.common.IWorldGenerator; public class WorldGen implements IWorldGenerator{ private static final int BlockX = 0; private int BlockZ; @Override public void generate(Random random, int chunkX, int chunkZ, World world,IChunkProvider chunkGenerator, IChunkProvider chunkProvider){ switch(world.provider.dimensionId){ case 1: generateNether(world, random, chunkX * 16, chunkZ * 16); case 0: generateSurface(world, random, chunkX * 16, chunkZ * 16); case -1: generateEnd(world, random, chunkX * 16, chunkZ * 16); } } private void generateNether(World world, Random random, int chunkX, int chunkZ) { } private void generateEnd(World world, Random random, int chunkX, int chunkZ){ } private void generateSurface(World world, Random random, int chunkX,int chunkZ){ int xCoord = chunkX; int yCoord = random.nextInt(64); int zCoord = chunkZ; (new WorldGenMinable(ashtonsmod.LightSteelOre.blockID, 7)).generate(world, random, (xCoord + random.nextInt(60)), yCoord, (zCoord + random.nextInt(60))); (new WorldGenMinable(ashtonsmod.AmethystOre.blockID, 5)).generate(world, random, (xCoord + random.nextInt(60)), yCoord, (zCoord + random.nextInt(80))); (new WorldGenMinableSand(ashtonsmod.AmethystOre.blockID, 5)).generate(world, random, (xCoord + random.nextInt(60)), yCoord, (zCoord + random.nextInt(10))); } }
just had a thought, i would like it to spawn in surface sand and this is where i am looking for it, did i get the y coord wrong for this?
-
so the fix would be to change the main class name?
-
ashtonsmod.common cannot be resolved to a type the fix you told me doesnt work.
(new ashtonsmod.common.WorldGenMinableSand(ashtonsmod.AmethystOre.blockID, 5)).generate(world, random, xCoord, yCoord, zCoord);
-
thats a bit gay...
if so many people want it and it makes sense alongside livingentityevent.
jeez thats really annoying.
-
ashtonsmod.common cannot be resolved to a type
-
so why arent there?
-
thankyou that seems to have worked, might you know how to generate blocks that will only spawn in sand because on my other thread i got told to go learn java angain and that is getting a bit tiresome...
-
youd think there was a way
-
really
dammit
Newbie needs help
in Modder Support
Posted
ok so i am assuming you already have our proxies set up for step sounds you simply need to type
.setStepSound(Block.s
and a lot of possible sounds should pop up
ie
.setStepSound(Block.soundClothFootstep)
this line goes on the end of your block init
(exampleblock = new blockexampleblock(block id, index texture).setUnlocalisedName("Example Block").setStepSound(Block.soundClothFootstep).setHardness(0.8F).setCreativeTab(tabBlocks);
and the block properties comes in the super constructor for that block ie
public BlockExampleBlock(int id, int texture)
{
super(id, texture, Material.rock);
}
the material in this block is set to "rock"
if you need more examples or any mroe help just ask