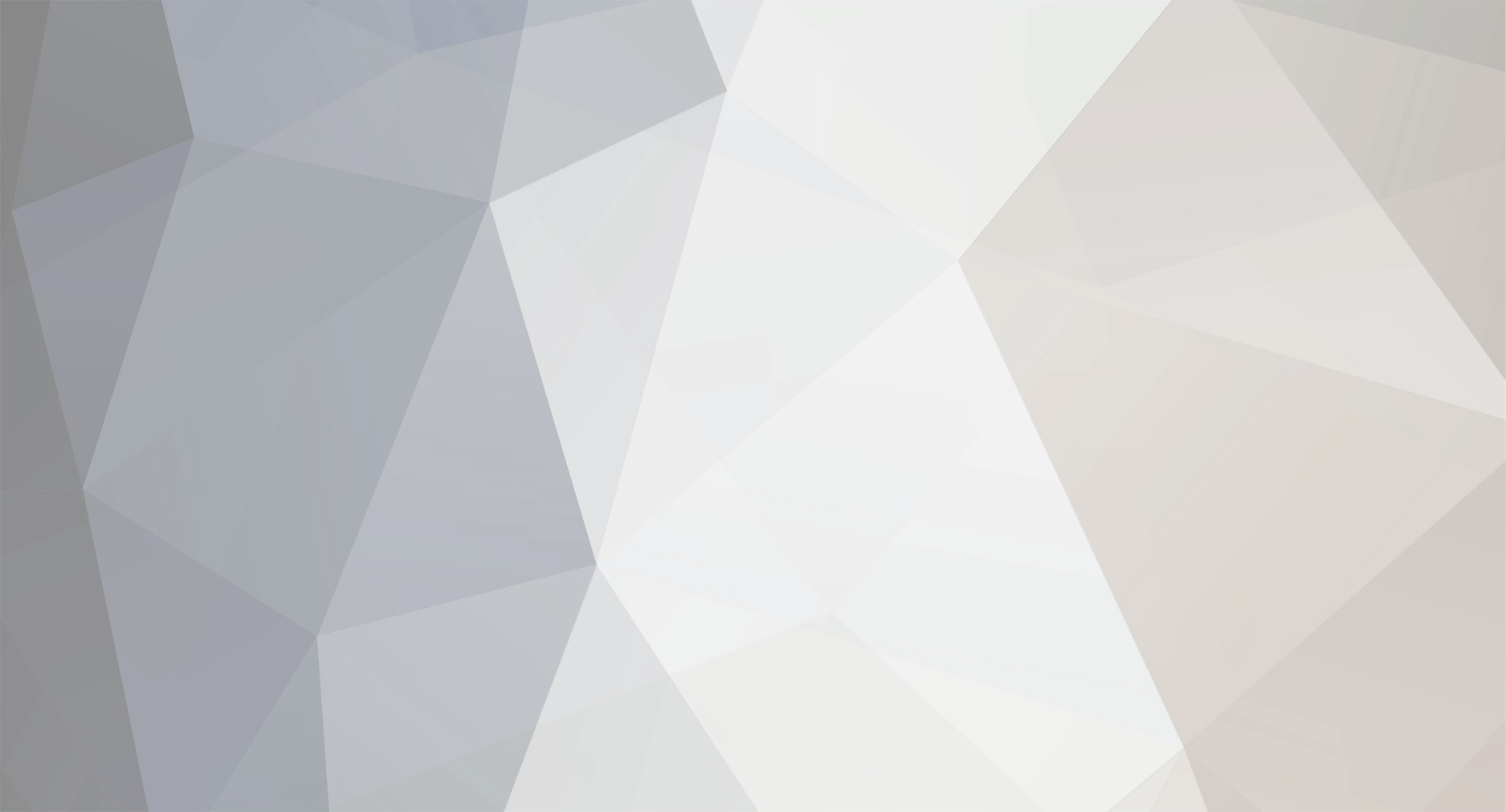
TuxCraft
Members-
Posts
92 -
Joined
-
Last visited
Everything posted by TuxCraft
-
So if I had a setup like this... modName > modDeveloper > folderA > folderB And folderA contained fileA and folderB contained fileB And fileA was testing for fileB I'd do this... File f = new File("/modDeveloper/folderA/fileB.class");
-
I want the mod I'm making to be very customizable by the user so when it loads I want it to check and if a file is present it will do something. I know I could use a config file to do something similar but I'd like to do something on a larger scale. What would the correct file path be to find the files I want to check? If I knew that I could then use something like this... File f = new File("minecraft/forge/where/do/I/go"); if(f.exists()) { } Does it start at the .minecraft folder and then go up? Is it different between the forge eclipse environment and actual game running environment? Also could I make it relative to the minecraft forge mods folder, that would be the easiest way because I can easily find my way from there. And one more thing, will it have to go through all the steps to get to the %appdata% folder because I know that varies from computer to computer and if your using a launcher or not.
-
Works like a charm, thanks
-
I'd rather not post the whole core file, but I put what I think you'll need. @Mod(modid = TuxWeaponsCore.modid, name = "Tux's Weapons Mod", version = "0.1") @NetworkMod(clientSideRequired=true, serverSideRequired=false, channels={"TuxWeaponsC"}) ... @Instance("TuxWeapons") public static TuxWeaponsCore instance; @SidedProxy(clientSide="mods.TuxWeapons.ClientProxy", serverSide="mods.TuxWeapons.CommonProxy") public static CommonProxy proxy; public static final String modid = "TuxWeaponsCore"; ... proxy.registerRenderers(); proxy.registerServerTickHandler(); Did I reference it wrong in my proxy?
-
Maybe this will help? I'd prefer to not use modloader classes...
-
I did have my proxy registers before my weapons and items, I moved them to the end and now it works great. However when I did your mod loader to forge method quick fix I ran into some problems. It crashes again, but when I comment out the new methods its fine. Here's my proxy again, I want to make sure that I get the little things like this too so that I'm up to standards =D
-
My mod works fine until I try to register a model in the client proxy. Here's the crash report... And the client proxy code It works fine without this line (MinecraftForgeClient.registerItemRenderer(TuxWeaponsCore.fireChargeCannon.itemID, (IItemRenderer) new FireChargeCannonRenderer())). I am following Ichun's 3d item tutorial which can be found .
-
I'm making a harpoon for my mod and the mechanics work fine, what doesn't work is the damage, the most important part. I modeled it after the arrow code so I throw the harpoon at a mob and it bounces off but it doesn't do any damage. Here's my code, please tell me what I've done wrong. itemHarpoon Entity Harpoon
-
I'm making a weapon mod and its pretty important, at least to me, that the weapons can be enchanted. When I put the weapon in the enchantment table it shows the list of enchantments I can select from, but when I click one nothing happens. I can however enchant through book and anvil. For my weapons I'm not using the default enumtoolmaterial, might that be why I'm encountering problems?
-
Google it. http://www.minecraftforge.net/wiki/Icons_and_Textures
-
Thank you, I did that and so far so good, the only thing I'm not sure about enchantments. There is one function that in a comment says that it holds enchantments, but it returns an nbttagcompound. Here's what I have so far. public static ItemStack getItemStack(Item item, int i, String s) { ItemStack par1itemstack = new ItemStack (item, 1); par1itemstack.setItemDamage(i); if(s != null) { par1itemstack.setItemName(s); } return par1itemstack; } And this to add the item par1EntityPlayer.inventory.addItemStackToInventory(itemSpear.getItemStack(itemTool.item, damage, null));
-
So for example to get damage would I do something like this? short damage = itemstack.stackTagCompound.getShort("Damage"); If it is, that isn't working for me.
-
Editing Player Class (Custom Player Class?)
TuxCraft replied to FoxxCommand's topic in Modder Support
When eclipse says you have an uninitialized variable that means nowhere in your code there is something that says variable x equals something. To solve it either have your uninitialized variable as a parameter or just set it equal to 0, or null and then have another method change it later on. Hoped that made sense. -
Thanks for responding but I should probably add that I want to convert it to an itemstack so that it will keep its name, damage etc. So that means your first method wouldn't work. As for your second one par3EntityPlayer.inventory.getCurrentItem() returns an itemstack so you wouldn't be able to put it in the new itemstack annotation. If you wanted to do that you'd have to add .getItem() to the end. However that returns the item not the itemstack. Would I have to just get all the features I want the item to keep in different variables and store it in nbt or something? The main ones I'd like it to keep are the name, damage, and enchantment. For the name I found this method... itemToDropName = par3EntityPlayer.inventory.getCurrentItem().getDisplayName() For the rest I'm not sure what to do, please help.
-
How do I make an item reference itself as an itemstack? I've tried this itemToDrop = par3EntityPlayer.inventory.getCurrentItem(); But when I add it back into the inventory (par3EntityPlayer.inventory.addItemStackToInventory(itemToDrop) in another method the item disappears whenever I try to use it (onItemRightClick). I think it might be a server/client error but I'm very inexperienced with packets and such. I tried following the tutorial on the wiki but I really didn't understand it.
-
Yes, I was thinking something along those lines, but I'm not sure how to get the default 2d renderer code in there and working.
-
Is this what your looking for? I know these work for tooltips, just put it in the string like this "§4Wassup".
-
Ok, I get the concept, but that's it Using the tutorial you linked to I was able to set up the random numbers being displayed when the player collides with the spear entity, and I noticed as I was messing around that if you put the addItemstack line in the spear entity's onCollide method try section it did the same thing as if it were just in the onCollide method. Do you put the addItemstack line in the packet handler file? When I tried putting that next to the system.out.print line it through a fit because it didn't like the EntityPlayer variable.
-
Thanks Maze, that was a good tip. I read the article oracle posted on instances and that does clear a lot up for me. I couldn't use "this" in another class because it wouldn't know what instance "this" was referring to. So I tried putting this in the on release function... EntitySpear.spearToDrop = par3EntityPlayer.inventory.getCurrentItem(); And it works... kind of. It gives me back the exact spear I threw, damage, custom name, enchantment everything, but when I throw it again it just bounces off the entity I threw it at and does no damage. And when I try to attack with it it immediately deletes itself from my inventory.
-
Thanks Maze, that was a good tip. I read the article oracle posted on instances and that does clear a lot up for me. I couldn't use "this" in another class because it wouldn't know what instance "this" was referring to. So I tried putting this in the on release function... EntitySpear.spearToDrop = par3EntityPlayer.inventory.getCurrentItem(); And it works... kind of. It gives me back the exact spear I threw, damage, custom name, enchantment everything, but when I throw it again it just bounces off the entity I threw it at and does no damage. And when I try to attack with it it immediately deletes itself from my inventory.
-
Yup, exactly.
-
Yup, exactly.
-
Rendering hates me, please help.
-
Rendering hates me, please help.
-
So how would I have my code call the Item an itemstack? When I do this... public static ItemStack thisSpear = new ItemStack(this, 1); It says this can't be used with a static modifier but adding the static modifier is the only way to get the entity to read it. I'll look at the entityItem code, that might have some insight on my dilemma.