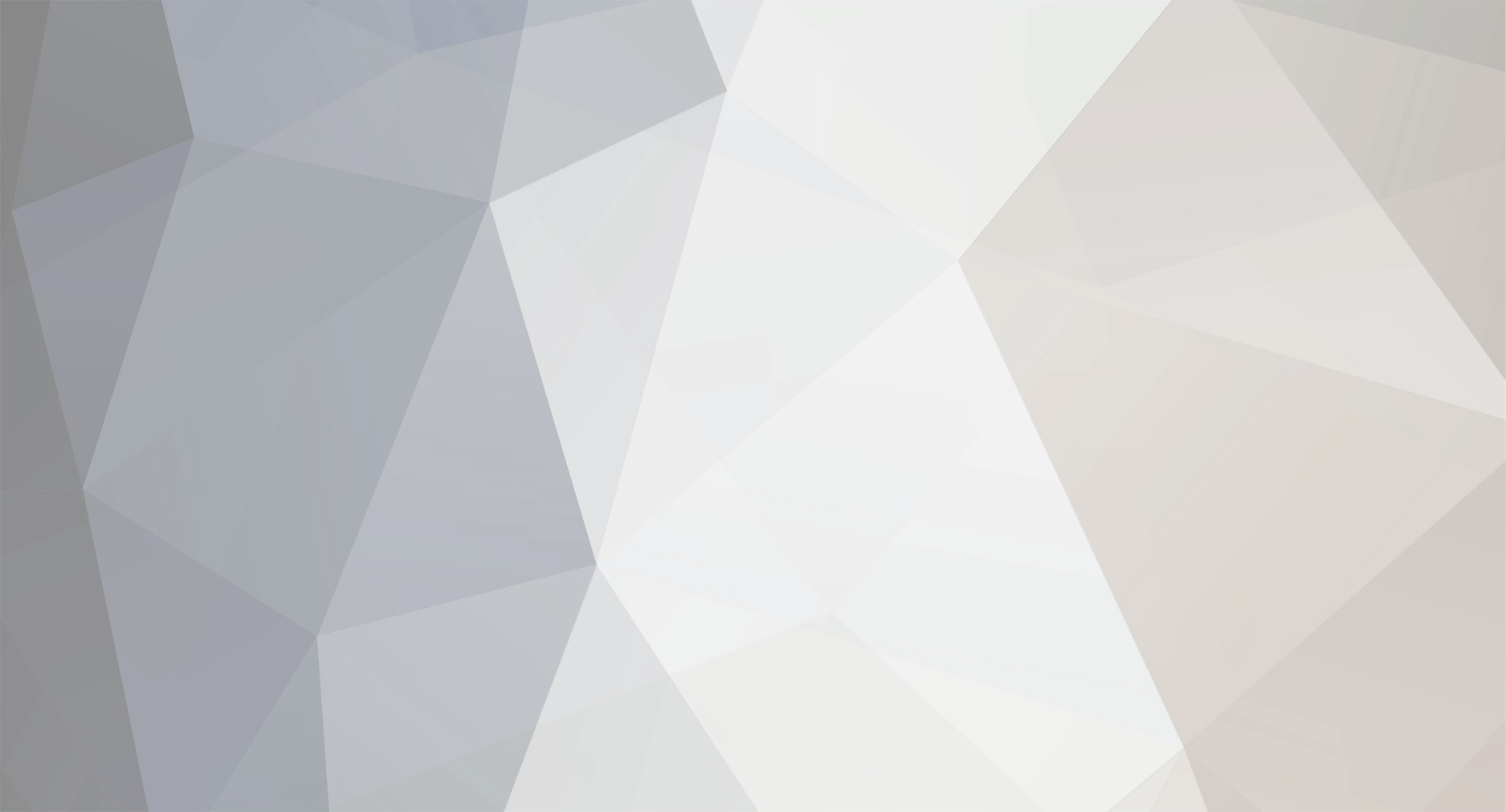
TuxCraft
Members-
Posts
92 -
Joined
-
Last visited
Everything posted by TuxCraft
-
Ok, I created a common and client proxy and put this in the client proxy [embed=425,349]@Override public void load() { RenderingRegistry.registerEntityRenderingHandler(EntityFireB.class, new RenderFireball(0.5F)); }[/embed] But it doesn't work, it doesn't give me any errors but my throwable item is still a white cube (I'll be using my Fireball as an example for now because the code is much skinnier)
-
I also tried making a throwable item and that too is a white box when it's thrown.
-
Originally I was going to change the weapon damage through that but I found a better way and I haven't taken out the repetitive mats yet.
-
I'm making a mod with spears in it and when I try to render the spear as just an arrow it decides it wants to be a white box (kinda fun for pixel-arting villager houses though). How would I get it to render even just as a normal arrow. My main mod file public class mainMod_XXX { static EnumToolMaterial toolIRON = EnumHelper.addToolMaterial("IRON", 2, 250, 6.0F, 2, 14); static EnumToolMaterial toolGOLD = EnumHelper.addToolMaterial("GOLD", 0, 32, 12.0F, 0, 22); static EnumToolMaterial toolDIAMOND = EnumHelper.addToolMaterial("DIAMOND", 3, 1561, 8.0F, 3, 10); static EnumToolMaterial toolSTONE = EnumHelper.addToolMaterial("STONE", 1, 131, 4.0F, 1, 5); static EnumToolMaterial toolWOOD = EnumHelper.addToolMaterial("WOOD", 0, 59, 2.0F, 0, 15); //Spears public static final Item ironSpear = new ItemSpear(9104, toolIRON, 1).setIconCoord(2, 0).setItemName("ironSpear"); public static final Item goldSpear = new ItemSpear(9106, toolGOLD, 2).setIconCoord(4, 0).setItemName("goldSpear"); public static final Item diamondSpear = new ItemSpear(9105, toolDIAMOND, 3).setIconCoord(3, 0).setItemName("diamondSpear"); public static final Item stoneSpear = new ItemSpear(9103, toolSTONE, 4).setIconCoord(1, 0).setItemName("stoneSpear"); public static final Item woodSpear = new ItemSpear(9102, toolWOOD, 5).setIconCoord(0, 0).setItemName("woodSpear"); // The instance of your mod that Forge uses. @Instance("weapons") public static mainMod_XXX instance; @PreInit public void preInit(FMLPreInitializationEvent event) { // Stub Method } public void init(FMLInitializationEvent preEvent) { } @Init public void load(FMLInitializationEvent event) { LanguageRegistry.addName(ironSpear, "Iron Spear"); LanguageRegistry.addName(diamondSpear, "Diamond Spear"); LanguageRegistry.addName(goldSpear, "Gold Spear"); LanguageRegistry.addName(woodSpear, "Wood Spear"); LanguageRegistry.addName(stoneSpear, "Stone Spear"); } @PostInit public void postInit(FMLPostInitializationEvent event) { // Stub Method } public void load() { MinecraftForgeClient.preloadTexture("/Mod_XXX/textures.png"); } public void AddRenderer(Map map) { map.put(EntitySpear.class, new RenderArrow()); } } Item Spear is pretty much sword code mixed with bow code. EntitySpear is an exact duplicate of EntityArrow except with a bunch of renames to make it work. RenderSpear @SideOnly(Side.CLIENT) public class RenderSpear extends Render { public void renderSpear(EntitySpear par1EntitySpear, double par2, double par4, double par6, float par8, float par9) { this.loadTexture("/Mod_XXX/spear.png"); GL11.glPushMatrix(); GL11.glTranslatef((float)par2, (float)par4, (float)par6); GL11.glRotatef(par1EntitySpear.prevRotationYaw + (par1EntitySpear.rotationYaw - par1EntitySpear.prevRotationYaw) * par9 - 90.0F, 0.0F, 1.0F, 0.0F); GL11.glRotatef(par1EntitySpear.prevRotationPitch + (par1EntitySpear.rotationPitch - par1EntitySpear.prevRotationPitch) * par9, 0.0F, 0.0F, 1.0F); Tessellator var10 = Tessellator.instance; byte var11 = 0; float var12 = 0.0F; float var13 = 0.5F; float var14 = (float)(0 + var11 * 10) / 32.0F; float var15 = (float)(5 + var11 * 10) / 32.0F; float var16 = 0.0F; float var17 = 0.15625F; float var18 = (float)(5 + var11 * 10) / 32.0F; float var19 = (float)(10 + var11 * 10) / 32.0F; float var20 = 0.05625F; GL11.glEnable(GL12.GL_RESCALE_NORMAL); float var21 = (float)par1EntitySpear.spearShake - par9; if (var21 > 0.0F) { float var22 = -MathHelper.sin(var21 * 3.0F) * var21; GL11.glRotatef(var22, 0.0F, 0.0F, 1.0F); } GL11.glRotatef(45.0F, 1.0F, 0.0F, 0.0F); GL11.glScalef(var20, var20, var20); GL11.glTranslatef(-4.0F, 0.0F, 0.0F); GL11.glNormal3f(var20, 0.0F, 0.0F); var10.startDrawingQuads(); var10.addVertexWithUV(-7.0D, -2.0D, -2.0D, (double)var16, (double)var18); var10.addVertexWithUV(-7.0D, -2.0D, 2.0D, (double)var17, (double)var18); var10.addVertexWithUV(-7.0D, 2.0D, 2.0D, (double)var17, (double)var19); var10.addVertexWithUV(-7.0D, 2.0D, -2.0D, (double)var16, (double)var19); var10.draw(); GL11.glNormal3f(-var20, 0.0F, 0.0F); var10.startDrawingQuads(); var10.addVertexWithUV(-7.0D, 2.0D, -2.0D, (double)var16, (double)var18); var10.addVertexWithUV(-7.0D, 2.0D, 2.0D, (double)var17, (double)var18); var10.addVertexWithUV(-7.0D, -2.0D, 2.0D, (double)var17, (double)var19); var10.addVertexWithUV(-7.0D, -2.0D, -2.0D, (double)var16, (double)var19); var10.draw(); for (int var23 = 0; var23 < 4; ++var23) { GL11.glRotatef(90.0F, 1.0F, 0.0F, 0.0F); GL11.glNormal3f(0.0F, 0.0F, var20); var10.startDrawingQuads(); var10.addVertexWithUV(-8.0D, -2.0D, 0.0D, (double)var12, (double)var14); var10.addVertexWithUV(8.0D, -2.0D, 0.0D, (double)var13, (double)var14); var10.addVertexWithUV(8.0D, 2.0D, 0.0D, (double)var13, (double)var15); var10.addVertexWithUV(-8.0D, 2.0D, 0.0D, (double)var12, (double)var15); var10.draw(); } GL11.glDisable(GL12.GL_RESCALE_NORMAL); GL11.glPopMatrix(); } public void doRender(Entity par1Entity, double par2, double par4, double par6, float par8, float par9) { this.renderSpear((EntitySpear)par1Entity, par2, par4, par6, par8, par9); } } Also if you could point me towards the bit in the render spear code where I could elongate the arrow/spear that would be great
-
[SOLVED] Minecraft SRC instillation error
TuxCraft replied to TuxCraft's topic in Support & Bug Reports
Okay, when you said java you meant java thought you were using java as shorthand for jdk. Updated java and it works! Thank you -
[SOLVED] Minecraft SRC instillation error
TuxCraft replied to TuxCraft's topic in Support & Bug Reports
JDK or the Apple release? I'll try both though. -
[SOLVED] Minecraft SRC instillation error
TuxCraft replied to TuxCraft's topic in Support & Bug Reports
I am using mac 10.8.2, please help, I want to continue the development of my mod. -
I tried updating my Forge MCP package today and it decompiled fine, but when I tried to run it through eclipse it didn't open and threw me these two lines in the console. _NSJVMLoadLibrary: NSAddLibrary failed for /libjawt.dylib JavaVM FATAL: lookup of function JAWT_GetAWT failed. Exit I deleted that version and tried again with various other releases but none of them worked, even the ones I was previously using. I have tried both 1.4.7 and 1.5 builds. Here's the forge log running it from a fresh build through eclipse. [embed=425,349]2013-03-13 07:03:26 [iNFO] [ForgeModLoader] Forge Mod Loader version 5.0.21.575 for Minecraft 1.5 loading 2013-03-13 07:03:26 [iNFO] [ForgeModLoader] Managed to load a deobfuscated Minecraft name- we are in a deobfuscated environment. Skipping runtime deobfuscation 2013-03-13 07:03:26 [FINEST] [ForgeModLoader] All core mods are successfully located 2013-03-13 07:03:26 [FINEST] [ForgeModLoader] Discovering coremods 2013-03-13 07:03:26 [FINEST] [ForgeModLoader] Downloading file http://files.minecraftforge.net/fmllibs/argo-small-3.2.jar 2013-03-13 07:03:26 [iNFO] [ForgeModLoader] Downloading file http://files.minecraftforge.net/fmllibs/argo-small-3.2.jar 2013-03-13 07:03:26 [FINEST] [ForgeModLoader] Download complete 2013-03-13 07:03:26 [iNFO] [ForgeModLoader] Download complete 2013-03-13 07:03:26 [FINEST] [ForgeModLoader] Library file argo-small-3.2.jar was downloaded and verified successfully 2013-03-13 07:03:26 [FINEST] [ForgeModLoader] Downloading file http://files.minecraftforge.net/fmllibs/guava-14.0-rc3.jar 2013-03-13 07:03:26 [iNFO] [ForgeModLoader] Downloading file http://files.minecraftforge.net/fmllibs/guava-14.0-rc3.jar 2013-03-13 07:03:28 [FINEST] [ForgeModLoader] Download complete 2013-03-13 07:03:28 [iNFO] [ForgeModLoader] Download complete 2013-03-13 07:03:28 [FINEST] [ForgeModLoader] Library file guava-14.0-rc3.jar was downloaded and verified successfully 2013-03-13 07:03:28 [FINEST] [ForgeModLoader] Downloading file http://files.minecraftforge.net/fmllibs/asm-all-4.1.jar 2013-03-13 07:03:28 [iNFO] [ForgeModLoader] Downloading file http://files.minecraftforge.net/fmllibs/asm-all-4.1.jar 2013-03-13 07:03:28 [FINEST] [ForgeModLoader] Download complete 2013-03-13 07:03:28 [iNFO] [ForgeModLoader] Download complete 2013-03-13 07:03:28 [FINEST] [ForgeModLoader] Library file asm-all-4.1.jar was downloaded and verified successfully 2013-03-13 07:03:28 [FINEST] [ForgeModLoader] Downloading file http://files.minecraftforge.net/fmllibs/bcprov-jdk15on-148.jar 2013-03-13 07:03:28 [iNFO] [ForgeModLoader] Downloading file http://files.minecraftforge.net/fmllibs/bcprov-jdk15on-148.jar 2013-03-13 07:03:30 [FINEST] [ForgeModLoader] Download complete 2013-03-13 07:03:30 [iNFO] [ForgeModLoader] Download complete 2013-03-13 07:03:30 [FINEST] [ForgeModLoader] Library file bcprov-jdk15on-148.jar was downloaded and verified successfully 2013-03-13 07:03:30 [FINEST] [ForgeModLoader] Downloading file http://files.minecraftforge.net/fmllibs/deobfuscation_data_1.5.zip 2013-03-13 07:03:30 [iNFO] [ForgeModLoader] Downloading file http://files.minecraftforge.net/fmllibs/deobfuscation_data_1.5.zip 2013-03-13 07:03:30 [FINEST] [ForgeModLoader] Download complete 2013-03-13 07:03:30 [iNFO] [ForgeModLoader] Download complete 2013-03-13 07:03:30 [FINEST] [ForgeModLoader] Library file deobfuscation_data_1.5.zip was downloaded and verified successfully 2013-03-13 07:03:30 [FINEST] [ForgeModLoader] Downloading file http://files.minecraftforge.net/fmllibs/scala-library.jar 2013-03-13 07:03:30 [iNFO] [ForgeModLoader] Downloading file http://files.minecraftforge.net/fmllibs/scala-library.jar 2013-03-13 07:03:34 [FINEST] [ForgeModLoader] Download complete 2013-03-13 07:03:34 [iNFO] [ForgeModLoader] Download complete 2013-03-13 07:03:34 [FINEST] [ForgeModLoader] Library file scala-library.jar was downloaded and verified successfully 2013-03-13 07:03:34 [FINEST] [ForgeModLoader] Running coremod plugins 2013-03-13 07:03:34 [FINEST] [ForgeModLoader] Running coremod plugin FMLCorePlugin 2013-03-13 07:03:34 [FINEST] [ForgeModLoader] Coremod plugin FMLCorePlugin run successfully 2013-03-13 07:03:34 [FINEST] [ForgeModLoader] Running coremod plugin FMLForgePlugin 2013-03-13 07:03:34 [FINEST] [ForgeModLoader] Coremod plugin FMLForgePlugin run successfully 2013-03-13 07:03:34 [FINEST] [ForgeModLoader] Validating minecraft 2013-03-13 07:03:34 [FINEST] [ForgeModLoader] Minecraft validated, launching... 2013-03-13 07:03:36 [iNFO] [sTDOUT] 229 recipes 2013-03-13 07:03:36 [iNFO] [sTDOUT] 27 achievements 2013-03-13 07:03:36 [iNFO] [Minecraft-Client] Setting user: Player446 2013-03-13 07:03:36 [iNFO] [sTDOUT] (Session ID is -) 2013-03-13 07:03:36 [iNFO] [sTDERR] Client asked for parameter: server 2013-03-13 07:03:36 [iNFO] [Minecraft-Client] LWJGL Version: 2.4.2[/embed]