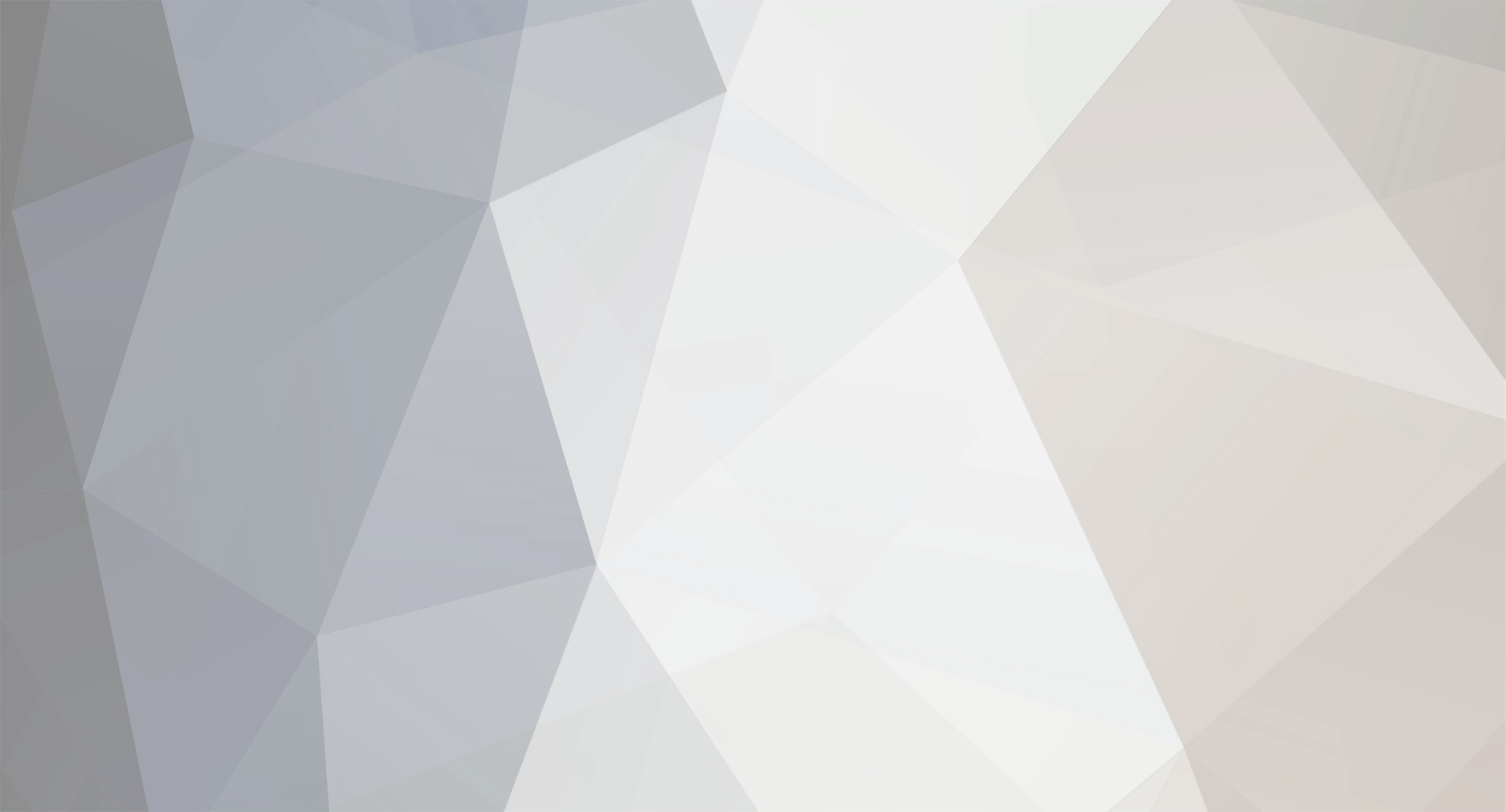
Novality
Members-
Posts
36 -
Joined
-
Last visited
Everything posted by Novality
-
[1.16.5] Executing a command as server console [SOLVED]
Novality replied to Novality's topic in Modder Support
One that executes commands as if it was entered in the server console. -
I've looked far and wide across the internet for some sort of help, but I can't find any methods that allow me to send commands through console. Not much more needs to be said, this is quite a simple post. If someone could give me something, anything, that would be nice.
-
[SOLVED] Sending a message to all current players [1.16.5]
Novality replied to Novality's topic in Modder Support
Thanks! -
[SOLVED] Sending a message to all current players [1.16.5]
Novality posted a topic in Modder Support
Here is my code: if (entity instanceof PlayerEntity) { Collection<NetworkPlayerInfo> users = Minecraft.getInstance().getConnection().getOnlinePlayers(); PlayerEntity player2 = (PlayerEntity) entity; specificPlayer.sendMessage(new StringTextComponent(player.getDisplayName().getString() + "evaporated " + player2.getDisplayName().getString() + " using the power of the void."), player.getUUID()); } I have a method of sending a message to a specific player. And a method of grabbing all online players. I'm not the smartest with java and I can't really figure out how to put the two together. I want to be able to send this message to all players, not just one as is currently. If someone could give me an example and explain what they did that would be great. As I said, I'm not the greatest with java (actually a beginner (blah blah I know, shouldn't start Java off with mc modding but here I am)) but coming from other languages like Python I'm a fast learner 😏 -
[1.16.5] Creating custom damage source to allow custom death messages
Novality replied to Novality's topic in Modder Support
Thank you. Disappointed though that this won't deal OUT_OF_WORLD damage but it's still pog. -
[1.16.5] Creating custom damage source to allow custom death messages
Novality replied to Novality's topic in Modder Support
I want to deal void damage to an entity (which I've already done). If it's a player that is killed, I want to override the "Player fell out of the world." death message and implement my own. -
[1.16.5] Creating custom damage source to allow custom death messages
Novality replied to Novality's topic in Modder Support
If I create my own damagesource i no longer will need to damage it with "entity.hurt(DamageSource.OUT_OF_WORLD, health);" right? Otherwise that'll kill the entity and I wont get the death message I want. Using "new DamageSource(name)" how can I specify that it should deal OUT_OF_WORLD damage? -
@Override public boolean onLeftClickEntity(ItemStack stack, PlayerEntity player, Entity entity) { float health = ((LivingEntity) entity).getMaxHealth(); return entity.hurt(DamageSource.OUT_OF_WORLD, health); } I have this current code above ^ I know there is a way to create my own damage source, but I couldn't find a way to have it deal void damage. Also, how would I go about specifying in the lang file to add a new death message? If anyone could point me in the right direction that would be great.
-
Before I start this post, I would like to inform anyone reading that I am new to coding in Java, especially with modding. I am using eclipse IDE to create somewhat of a test mod. When loading debug on eclipse to preview my mod, mod loading gets stuck on 1/5, and the last message sent in the console is "[10:00:11] [Client thread/DEBUG] [FML]: Attempting to inject @SidedProxy classes into voidgear" So I suspect it is something to do with the proxy classes. Here is my code, I've followed a few tutorials to get up to this point: In Main.java: package xyz.novality.voidgear; import xyz.novality.voidgear.proxy.CommonProxy; import xyz.novality.voidgear.util.Reference; import net.minecraftforge.fml.common.Mod; import net.minecraftforge.fml.common.Mod.EventHandler; import net.minecraftforge.fml.common.Mod.Instance; import net.minecraftforge.fml.common.SidedProxy; import net.minecraftforge.fml.common.event.FMLInitializationEvent; import net.minecraftforge.fml.common.event.FMLPostInitializationEvent; import net.minecraftforge.fml.common.event.FMLPreInitializationEvent; import org.apache.logging.log4j.Logger; @Mod(modid = Main.MODID, name = Main.NAME, version = Main.VERSION) public class Main { public static final String MODID = "voidgear"; public static final String NAME = "Void Gear"; public static final String VERSION = "1.0.0"; private static Logger logger; @Instance public static Main instance; @SidedProxy(clientSide = Reference.CLIENT, serverSide = Reference.COMMON) public static CommonProxy proxy; @EventHandler public static void preInit(FMLPreInitializationEvent event) { logger = event.getModLog(); } @EventHandler public static void init(FMLInitializationEvent event) { logger.info("Mod successfully initialized!"); } @EventHandler public static void postInit(FMLPostInitializationEvent event) {} } In ItemInit.java: package xyz.novality.voidgear.init; import java.util.ArrayList; import java.util.List; import net.minecraft.item.Item; import xyz.novality.voidgear.objects.items.ItemBase; public class ItemInit { public static final List<Item> ITEMS = new ArrayList<Item>(); public static final Item VOID_SWORD = new ItemBase("sword_void"); } In ItemBase.java: package xyz.novality.voidgear.objects.items; import net.minecraft.creativetab.CreativeTabs; import net.minecraft.item.Item; import xyz.novality.voidgear.Main; import xyz.novality.voidgear.init.ItemInit; import xyz.novality.voidgear.util.IHasModel; public class ItemBase extends Item implements IHasModel { public ItemBase(String name) { setUnlocalizedName(name); setRegistryName(name); setCreativeTab(CreativeTabs.COMBAT); ItemInit.ITEMS.add(this); } @Override public void registerModels() { Main.proxy.registerItemRenderer(this, 0, "inventory"); } } In ClientProxy.java: package xyz.novality.voidgear.proxy; import net.minecraft.client.renderer.block.model.ModelResourceLocation; import net.minecraft.item.Item; import net.minecraftforge.client.model.ModelLoader; public class ClientProxy extends CommonProxy { @Override public void registerItemRenderer(Item item, int meta, String id) { ModelLoader.setCustomModelResourceLocation(item, meta, new ModelResourceLocation(item.getRegistryName(), id)); } } In CommonProxy.java: package xyz.novality.voidgear.proxy; import net.minecraft.item.Item; public class CommonProxy { public void registerItemRenderer(Item item, int meta, String id) {} } In IHasModel.java: package xyz.novality.voidgear.util; public interface IHasModel { public void registerModels(); } In Reference.java: package xyz.novality.voidgear.util; public class Reference { public static final String MODID = "voidgear"; public static final String NAME = "Void Gear"; public static final String VERSION = "1.0.0"; public static final String CLIENT = "xyz.novality.voidgear.proxy.ClientProxy"; public static final String COMMON = "xyz.novality.voidgear.proxy.CommonProxy"; } In RegistryHandler.java: package xyz.novality.voidgear.util.handlers; import net.minecraft.item.Item; import net.minecraftforge.client.event.ModelRegistryEvent; import net.minecraftforge.event.RegistryEvent; import net.minecraftforge.fml.common.Mod.EventBusSubscriber; import net.minecraftforge.fml.common.eventhandler.SubscribeEvent; import xyz.novality.voidgear.init.ItemInit; import xyz.novality.voidgear.util.IHasModel; @EventBusSubscriber public class RegistryHandler { @SubscribeEvent public static void onItemRegister(RegistryEvent.Register<Item> event) { event.getRegistry().registerAll(ItemInit.ITEMS.toArray(new Item[0])); } @SubscribeEvent public static void onModelRegister(ModelRegistryEvent event) { for(Item item : ItemInit.ITEMS) if (item instanceof IHasModel) { ((IHasModel)item).registerModels(); } } } In en_us.lang: item.sword_void.name=Void Sword In sword_void.json: { "parent": "item/generated", "textures": { "layer0": "voidgear:items/sword_void" } } In mcmod.info: [ { "modid": "voidgear", "name": "Void Gear", "description": "Void Gear mod lol", "version": "1.0.0", "mcversion": "1.12.2", "url": "novality.xyz/voidgear", "updateUrl": "", "authorList": ["Savage O-Press#0001"], "credits": "Savage O-Press#0001", "logoFile": "", "screenshots": [], "dependencies": [] } ] In pack.mcmeta: { "pack": { "description": "voidgear resources", "pack_format": 3, "_comment": "A pack_format of 3 should be used starting with Minecraft 1.11. All resources, including language files, should be lowercase (eg: en_us.lang). A pack_format of 2 will load your mod resources with LegacyV2Adapter, which requires language files to have uppercase letters (eg: en_US.lang)." } } In build.gradle: buildscript { repositories { maven { url = 'https://files.minecraftforge.net/maven' } jcenter() mavenCentral() } dependencies { classpath 'net.minecraftforge.gradle:ForgeGradle:3.+' } } apply plugin: 'net.minecraftforge.gradle' // Only edit below this line, the above code adds and enables the necessary things for Forge to be setup. apply plugin: 'eclipse' apply plugin: 'maven-publish' version = '1.12.2-1.0.0' group = 'xyz.novality.voidgear' // http://maven.apache.org/guides/mini/guide-naming-conventions.html archivesBaseName = 'voidgear' sourceCompatibility = targetCompatibility = compileJava.sourceCompatibility = compileJava.targetCompatibility = '1.8' // Need this here so eclipse task generates correctly. minecraft { // The mappings can be changed at any time, and must be in the following format. // snapshot_YYYYMMDD Snapshot are built nightly. // stable_# Stables are built at the discretion of the MCP team. // Use non-default mappings at your own risk. they may not always work. // Simply re-run your setup task after changing the mappings to update your workspace. //mappings channel: 'snapshot', version: '20171003-1.12' mappings channel: 'snapshot', version: '20171003-1.12' // makeObfSourceJar = false // an Srg named sources jar is made by default. uncomment this to disable. // accessTransformer = file('src/main/resources/META-INF/accesstransformer.cfg') // Default run configurations. // These can be tweaked, removed, or duplicated as needed. runs { client { workingDirectory project.file('run') // Recommended logging data for a userdev environment property 'forge.logging.markers', 'SCAN,REGISTRIES,REGISTRYDUMP' // Recommended logging level for the console property 'forge.logging.console.level', 'debug' } server { // Recommended logging data for a userdev environment property 'forge.logging.markers', 'SCAN,REGISTRIES,REGISTRYDUMP' // Recommended logging level for the console property 'forge.logging.console.level', 'debug' } } } dependencies { // Specify the version of Minecraft to use, If this is any group other then 'net.minecraft' it is assumed // that the dep is a ForgeGradle 'patcher' dependency. And it's patches will be applied. // The userdev artifact is a special name and will get all sorts of transformations applied to it. minecraft 'net.minecraftforge:forge:1.12.2-14.23.5.2855' // You may put jars on which you depend on in ./libs or you may define them like so.. // compile "some.group:artifact:version:classifier" // compile "some.group:artifact:version" // Real examples // compile 'com.mod-buildcraft:buildcraft:6.0.8:dev' // adds buildcraft to the dev env // compile 'com.googlecode.efficient-java-matrix-library:ejml:0.24' // adds ejml to the dev env // The 'provided' configuration is for optional dependencies that exist at compile-time but might not at runtime. // provided 'com.mod-buildcraft:buildcraft:6.0.8:dev' // These dependencies get remapped to your current MCP mappings // deobf 'com.mod-buildcraft:buildcraft:6.0.8:dev' // For more info... // http://www.gradle.org/docs/current/userguide/artifact_dependencies_tutorial.html // http://www.gradle.org/docs/current/userguide/dependency_management.html } // Example for how to get properties into the manifest for reading by the runtime.. jar { manifest { attributes([ "Specification-Title": "examplemod", "Specification-Vendor": "examplemodsareus", "Specification-Version": "1", // We are version 1 of ourselves "Implementation-Title": project.name, "Implementation-Version": "${version}", "Implementation-Vendor" :"examplemodsareus", "Implementation-Timestamp": new Date().format("yyyy-MM-dd'T'HH:mm:ssZ") ]) } } // Example configuration to allow publishing using the maven-publish task // This is the preferred method to reobfuscate your jar file jar.finalizedBy('reobfJar') // However if you are in a multi-project build, dev time needs unobfed jar files, so you can delay the obfuscation until publishing by doing //publish.dependsOn('reobfJar') publishing { publications { mavenJava(MavenPublication) { artifact jar } } repositories { maven { url "file:///${project.projectDir}/mcmodsrepo" } } } If any other files are needed, let me know ^-^ If anyone notices any code errors or anything that I have done wrong that could be causing this issue, please say so! Reminder that this is a 1.12.2 mod for forge. Any help would be greatly appreciated! :)