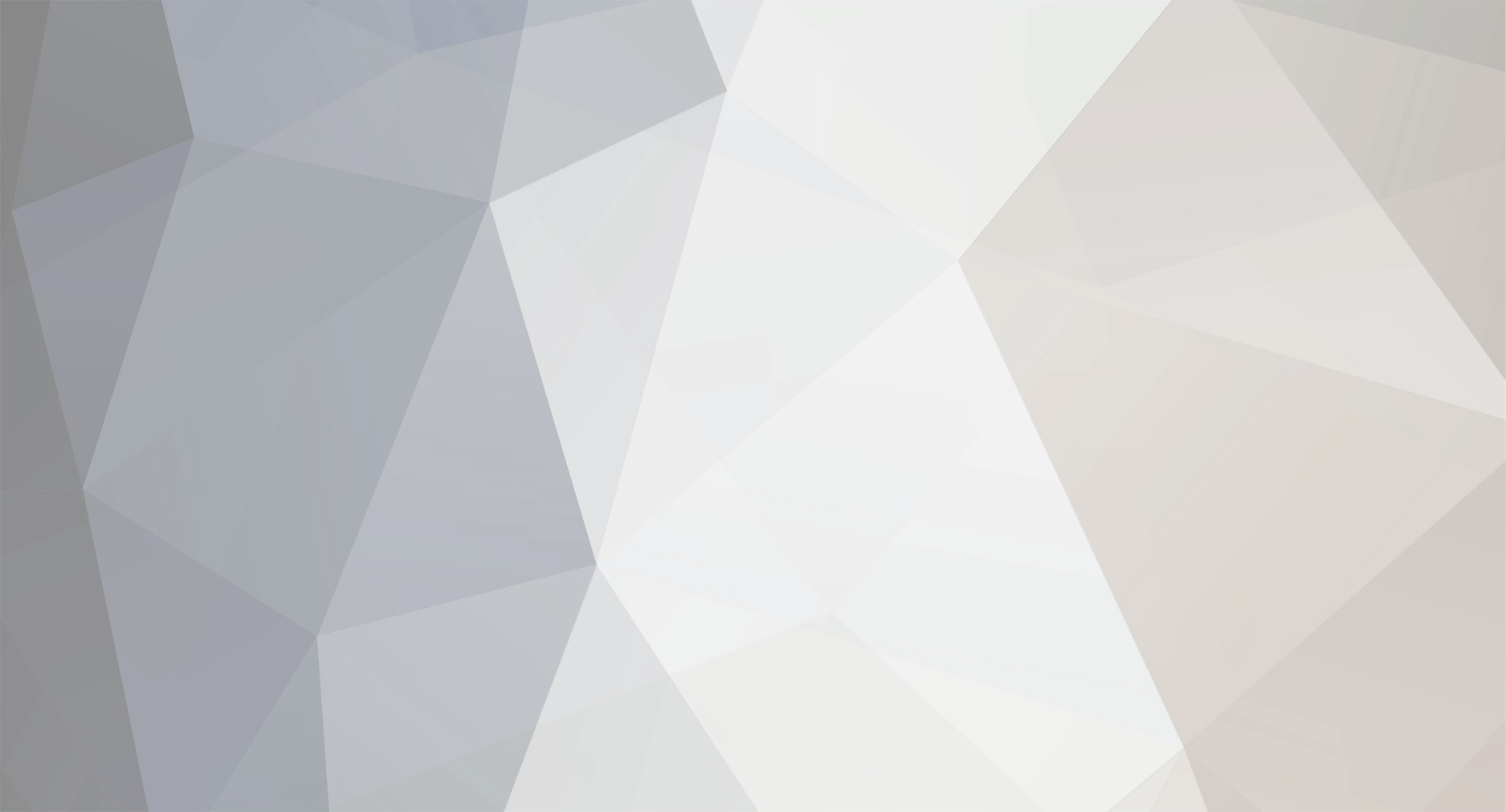
LightningFlash
Members-
Posts
7 -
Joined
-
Last visited
-
Days Won
1
Everything posted by LightningFlash
-
I have a tool that harvests multiple crops at once, that either replants or harvests the crops depending on an enchant on the tool. The harvesting and loot dropping works fine, but when it comes to updating what block is rendering, only the clicked block is updated properly, while the others drop the loot but appear to say as the fully grown crop, until right clicked again. The normal path for the tool is to just break the crop, as if the player harvested the crop. The other path is to reset the growth of the crop and drop the crop's loot, minus one seed. I have already tried all combinations of method parameters for setBlock() and destroyBlock(), and the updateing of the crop blocks still does not happen. The code thus far: public class ScytheItem extends HoeItem { public ScytheItem(Tier tier, int atkBase, float atkSpeed) { super(tier, atkBase, atkSpeed, new Item.Properties().stacksTo(1)); } @Override public @NotNull InteractionResult useOn(@NotNull UseOnContext context) { Level level = context.getLevel(); Player player = context.getPlayer(); InteractionHand hand = context.getHand(); ItemStack stack = context.getItemInHand(); if (!level.isClientSide() && player != null) { BlockPos pos = context.getClickedPos(); Block block = level.getBlockState(pos).getBlock(); if(block instanceof CropBlock) { level.playSound(null, pos, block.getSoundType(level.getBlockState(pos), level, pos, player).getBreakSound(), SoundSource.BLOCKS, 1.0F, AOTMain.RANDOM.nextFloat()); Map<Enchantment, Integer> enchantments = stack.getAllEnchantments(); int harvestSize = 1 + enchantments.getOrDefault(EnchantmentInit.HARVESTING_SIZE.get(), 0); for (int xOff = -harvestSize; xOff <= harvestSize; xOff++) { for (int zOff = -harvestSize; zOff <= harvestSize; zOff++) { BlockPos newPos = pos.offset(xOff, 0, zOff); Block blockAt = level.getBlockState(newPos).getBlock(); if (blockAt instanceof CropBlock crop) { if (crop.isMaxAge(level.getBlockState(newPos))) { if (enchantments.containsKey(EnchantmentInit.AUTO_PLANTER.get())) { dropLoot(level, newPos, level.getBlockState(newPos), block); level.setBlock(newPos, crop.getStateForAge(0), Block.UPDATE_CLIENTS); // the broken bit } else level.destroyBlock(newPos, true); // the broken bit } } } } stack.hurtAndBreak(1, player, (p) -> p.broadcastBreakEvent(hand)); } } return super.useOn(context); } private void dropLoot(@NotNull Level level, @NotNull BlockPos pos, @NotNull BlockState state, @NotNull Block block) { List<ItemStack> drops = getDrops(level, pos, state); findSeeds(block, drops).ifPresent(seed -> seed.shrink(1)); drops.forEach((drop) -> Block.popResource(level, pos, drop)); } private List<ItemStack> getDrops(@NotNull Level level, @NotNull BlockPos pos, @NotNull BlockState state) { return Block.getDrops(state, (ServerLevel) level, pos, null); } private Optional<ItemStack> findSeeds(@NotNull Block block, final Collection<ItemStack> stacks) { Item seedsItem = block.asItem(); return stacks.stream().filter((stack) -> stack.getItem() == seedsItem).findAny(); } }
-
I am trying to override or disable some vanilla recipes, as part of my mod. All of the methods that I have seen online, so far, do not work. So far, I have tried: Creating a JSON file in the "data/minecraft/recipes" folder, that has air as the ingredient and a barrier as the result This only works for crafting table recipes adding a smelting recipe this way works as intended Editing the ordering to AFTER in the forge dependency inside the "mods.toml" file Found an older post about using the FurnaceRecipe class, that no longer exists From: here So, what is required to disable a vanilla smelting recipe? I know some may say to not do this, but part of the progression of my mod kind of requires disabling some of the furnace recipes. Also, it seems that smelting recipes don't technically have to have the same filename as the block used, r as the result. When overriding crafting recipes, just adding the edited/removed recipe file in the minecraft data folder, under the same name as the original recipe. Edit: the crafting recipes seem to be cached between worlds? I created a new world and the edited crafting recipes works, but it doesn't work in an already made world?
-
As the title says, is there any way to define an Enum or List, to use as an argument in a command? Currently, if I am copying a section of my command for each subsequent argument. This is what I am taking about: .then(Commands.literal("-B") .then(Commands.literal("-m") .then(Commands.literal("-b") .executes(context -> execute(context, "mine","blocks","book")) ) .then(Commands.literal("-i") .executes(context -> execute(context, "mine","items","book")) ) ) .then(Commands.literal("-a") .then(Commands.literal("-b") .executes(context -> execute(context, "aot","blocks","book")) ) .then(Commands.literal("-i") .executes(context -> execute(context, "aot","items","book")) ) ) ) The code above will repeat the -i and -b section for each argument before them. For example, the first argument has the possible values of: B, M, S, T, and A. The above section of code has to be copied for the list of values for the first argument. It would be much cleaner and more maintainable, if I could use the values of a List or Enum instead, so I can use just one section of code and not five or more. Simply, I would like to accomplish something like the give command, where you have a list of entities you can use as an argument (@e, @a, @p, @s, @r). The code of the give command uses the EntityArgument, however, I just need an Enum or List of Strings to use as the argument.
-
[1.19] Have custom command give player a written book?
LightningFlash replied to LightningFlash's topic in Modder Support
Ok adding this does finally make it work. I now just have to add a loop that fits the strings to the max number of characters allowed per page, which should not be to hard. Thanks for the help. -
[1.19] Have custom command give player a written book?
LightningFlash replied to LightningFlash's topic in Modder Support
Ok, I followed the code from that class, and it does add text to the pages. However, it only adds the first string to each page. My current code: // create the itemstack ItemStack stack = new ItemStack(Items.WRITTEN_BOOK); // create the main NBT tag stack.setTag(new CompoundTag()); // set the title and author stack.getTag().putString("author", "AOT Command"); stack.getTag().putString("title", "Registry Out"); // list of pages ListTag book_pages = new ListTag(); // list of strings (page text) List<String> pages = Lists.newArrayList(); // fill the page strings with some basic text for (int i = 0; i < 10; i++) pages.add("i = " + i); // add the page string to the list tag pages.stream().map(StringTag::valueOf).forEach(book_pages::add); // add the list tag to the NBT to the itemstack stack.addTagElement("pages",book_pages); // drop finalized book to player player.drop(stack, false, false); -
As the title says, I would like to have a custom command give the player a signed book with some filled pages. I have the book being given to the player, with a title and author; however, I am having issues with how to add the pages. When I try to add a page, all I get is red text saying "* Invalid book tag *". Below is the code I have so far: ItemStack stack = new ItemStack(Items.WRITTEN_BOOK); stack.setTag(new CompoundTag()); stack.getTag().putString("author", "AOT Command"); stack.getTag().putString("title", "Registry Out"); stack.getTag().putString("pages", "testing"); player.drop(stack, false, false);
-
I am currently adding a bunch of tools, using the appropriate classes, i.e. AxeItem, PickaxeItem, etc. The issue I am having is that my axes can break stone. I set up the tool material enum just like how the vanilla game does it, referencing the vanilla ItemTier class. I added the items just like how the game does it, referencing the vanilla Items class. My question is why are my custom axes able to break and drop stone?