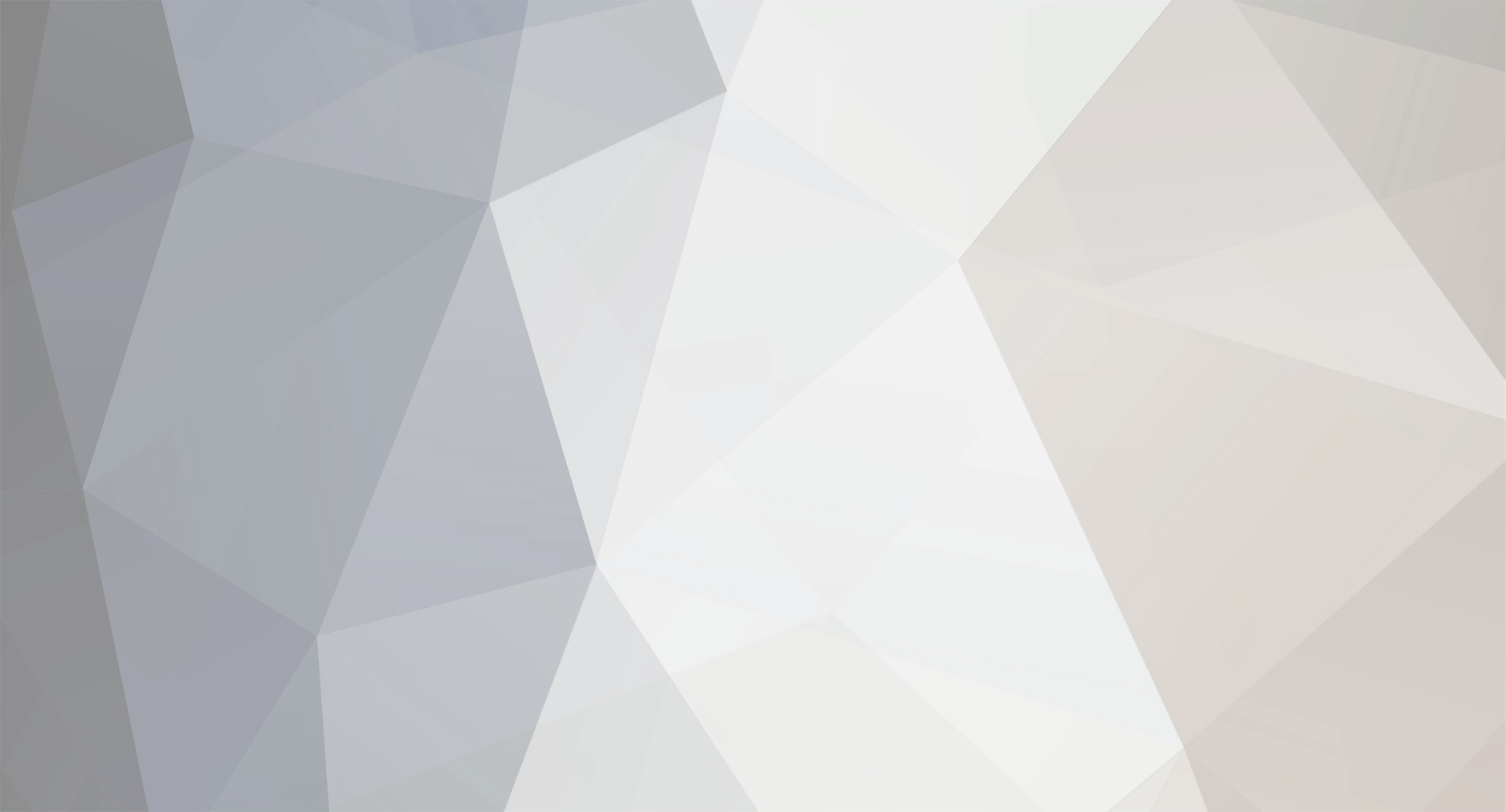
Tekner
Members-
Posts
43 -
Joined
-
Last visited
Everything posted by Tekner
-
You'll want to override the following functions: //This disables the ability to place torches/redstone/etc on this block. Use it on non-solid blocks or blocks with custom renderers. public boolean isOpaqueCube() { return false; } //This removes the collision box from the block, making things pass through it. public AxisAlignedBB getCollisionBoundingBoxFromPool(World par1World, int par2, int par3, int par4) { return null; }
-
The api for computercraft is included in the normal mod download in a folder called, appropriately, "api".
-
The reason it works in eclipse is because it runs the game off of the source files, as you'd expect. Forge is a mod loader and does not edit base class files except where it needs to for its own purposes. That said, it will never compile base class changes. Those stay in eclipse and for good reason. If you have to change something in the vanilla code, you use reflection. If you don't know what reflection is, well you have more homework to do (Quite a bit. Reflection is an advanced topic, but there are tutorials you can copy/paste and modify to get it to work.) The short explanation is that reflection is a computer program going over its own code and changing its behavior run-time. This lets you change things that are otherwise inaccessible (private/protected classes, methods, and variables.) Reflection is very slow and should only be used to change/check things sparingly, so be careful. For more advanced/heavy reflection, use the ASM provided by Minecraft Forge. For lighter things like single variable edits/checks, you can use the ObfuscationReflectionHelper class, also provided in Minecraft Forge. An example of using one of its static methods in one of my mod's code is ObfuscationReflectionHelper.setPrivateValue(EntityRenderer.class, Minecraft.getMinecraft().entityRenderer, 1f, "fovModifierHand", "field_78507_R"); Note that I haven't checked if that exact code is still valid since I ported my mod from 1.5.2 to 1.6.2, but the way you use it should still be, which is all that matters here.
-
You can create your own redstone block class by copying the code from the vanilla redstone block (inheriting may or may not be enough depending on if the methods/fields you need to access are set to private or protected.) Then change your custom class to always be on. Then, when right clicking vanilla redstone, change it to be your custom version of redstone and vice versa to turn it off. This is essentially replacing the redstone with a redstone torch, but makes it more discrete visually. I haven't looked at any of that code, but if it uses tile entities in any way, make sure you clean them up when switching blocks or you'll encounter weird behavior.
-
This doesn't answer your question directly, but maybe this will still be helpful. What launcher are you planning on using to go along with your modpack (assuming you meant modpack and not just a single mod)? Most modpacks use a specific, or even proprietary (like the Technic Pack), launcher to make sure all of its files are correct. The advantage of this is that it can update your modpack for your users automatically and it can update any file, not just mods. This lets you add things like Optifine or other minecraft mods that have to be added directly to the Minecraft.jar like your modified mojang.png. If you don't know where to begin on using a launcher for your modpack, I'd personally recommend looking at sk89q's launcher. You can create your own modpacks and it can be used for vanilla minecraft as well, so your users can still use only one launcher.
-
I was wondering if there's already an external or forge-implemented API for adding tabs, specifically to the player's inventory screen (<E> Key.) The effect I'm looking for is something like what Tinkers' Construct has for its extra armor/accessories tab and its knapsack tab (Direwolf20 shows it off in his mod spotlight found in that link at 25:00 minutes.) Now, I know I can fake it by drawing on tabs in the tick handler when the current gui screen is the player inventory, but handling that and disabling/enabling inventories to only draw when a certain tab is active is going to get very tricky and/or messy, which is why I'm wondering if there's a better, probably more streamlined and compatible (E.g. so my tabs won't draw on top or under Tinkers' tabs) way to do it. Thanks in advance. P.S. I haven't yet updated my mod from 1.5.2 to 1.6.2. If the method to do this is 1.6.2 only, that'd be good to know
-
Mod works fine in Eclipse, but not in Minecraft?
Tekner replied to dstars5's topic in Modder Support
I had a feeling it had to do with the mods folder being part of your package hierarchy. -
Mod works fine in Eclipse, but not in Minecraft?
Tekner replied to dstars5's topic in Modder Support
So you're saying that your root folder, "mods, is in "MCP\src\minecraft"? And the full path is "MCP\src\minecraft\mods\SpawnEggsPlus\common\SpawnEggsPlus"? Also, why are one of your folders called "mods"? I'm guessing that's so that you can put your textures and things in there? You should be putting your "mods" data folder directly into the "MCP\jars\bin\minecraft.jar". That may be part of your problem. Lastly, the folder that you're right clicking on to zip up is the "mods" folder in "MCP\reobf", right? And that's after running both "recompile.bat" AND "reobfuscate.bat"? (or .sh if you're using Unix.) -
You should look up how the vanilla beacon does it. The best way to do this is to use math (Sin and Cosine) and offset your x, y, and z positions accordingly. If you don't know basic geometry to be able to do this, now's a better time than ever to learn.
-
In your mcp folder (the one with all the batch and shell files), first run recompile.bat on Windows, or recompile.sh if you're using Unix. After that's done, run reobfuscate (bat or sh.) Your mod will be in its own folder in the mcp/reobf/ directory. Using an archiving tool to zip the folder into a .zip or .jar (jar files are zip files with a different extension.)
-
Your Gui can extend GuiScreen and your block can implement ITileEntityProvider if you don't want a container. This also means that you don't need to pass a Container to the server in your GuiHandler. I know this works because my mod currently already does this. As for closing the currently opened Gui, just use: mc.displayGuiScreen((GuiScreen)null); if calling this from a gui window, or: Minecraft.getMinecraft().displayGuiScreen((GuiScreen)null); from anywhere else in the client code. If you want to close it with the E key, then you'll have you use a KeyHandler. There are plenty of tutorials about them. Be aware that if you register a KeyBinding using the same key as one that Minecraft already uses, it'll override it and make it not function normally anymore. This means that if you bind the above code to the E key, opening your inventory won't work anymore ...unless you use this little trick that I figured out myself: Minecraft.getMinecraft().gameSettings.keyBindJump = KeysHandler.jump; In this case, I have my own KeyBinding (KeysHandler.jump) using the space key. By binding Minecraft's normal keybind to mine, both it and my mod can use the key however they needs to.
-
Your Gui can extend GuiScreen and your block can implement ITileEntityProvider if you don't want a container. This also means that you don't need to pass a Container to the server in your GuiHandler. I know this works because my mod currently already does this. As for closing the currently opened Gui, just use: mc.displayGuiScreen((GuiScreen)null); if calling this from a gui window, or: Minecraft.getMinecraft().displayGuiScreen((GuiScreen)null); from anywhere else in the client code. If you want to close it with the E key, then you'll have you use a KeyHandler. There are plenty of tutorials about them. Be aware that if you register a KeyBinding using the same key as one that Minecraft already uses, it'll override it and make it not function normally anymore. This means that if you bind the above code to the E key, opening your inventory won't work anymore ...unless you use this little trick that I figured out myself: Minecraft.getMinecraft().gameSettings.keyBindJump = KeysHandler.jump; In this case, I have my own KeyBinding (KeysHandler.jump) using the space key. By binding Minecraft's normal keybind to mine, both it and my mod can use the key however they needs to.
-
The first two can easily be done without editing base classes (which you should avoid at any cost unless it is 100% necessary.) All you have to do is make new tools and armor extending the vanilla tools and armor, and then change the vanilla recipes to output the new tools and armor. By harvest levels, I'm assuming you mean hardness. You can easily change hardness by using the Block class. It has a list of all the blocks in the game and you can use setHardness() on any of them. Example: Block.obsidian.setHardness(25); 25 is half the vanilla hardness of obsidian, so it'll take half the time to harvest it. I'm not 100% sure about changing block drops, but there's probably an event or hook somewhere you can look into. Don't forget to check the source code in Eclipse. The stuff in there is more useful than you may realize. Specifically, look in the package hierarchy net.minecraftforge.event.
-
The first two can easily be done without editing base classes (which you should avoid at any cost unless it is 100% necessary.) All you have to do is make new tools and armor extending the vanilla tools and armor, and then change the vanilla recipes to output the new tools and armor. By harvest levels, I'm assuming you mean hardness. You can easily change hardness by using the Block class. It has a list of all the blocks in the game and you can use setHardness() on any of them. Example: Block.obsidian.setHardness(25); 25 is half the vanilla hardness of obsidian, so it'll take half the time to harvest it. I'm not 100% sure about changing block drops, but there's probably an event or hook somewhere you can look into. Don't forget to check the source code in Eclipse. The stuff in there is more useful than you may realize. Specifically, look in the package hierarchy net.minecraftforge.event.
-
Any time I need to draw an icon, or anything really, I just draw it off of a sprite sheet. I bind the texture like you do and use drawTexturedModalRect(). You just specify where to draw it, and where on the sprite sheet the part you want to draw is and how big it is. Make sure the file is 256x256 or a multiple of that (E.g. 512x512.) The position and size is also assuming that your sheet is 256x256, so position (128, 0) is always the top middle. If you don't want to do it that way (I'd recommend you do because you don't have to put your icons in the mods folder and you can put everything in one file.), you are probably just not getting the path right. I haven't done icons the way you tried, as I said, but I don't think you're supposed to put icon files in your mods folder. I'd recommend you make a gui folder right next to your code and reference it in there. A path like "/legendz/gui/npcdialog.png" should work fine.
-
Any time I need to draw an icon, or anything really, I just draw it off of a sprite sheet. I bind the texture like you do and use drawTexturedModalRect(). You just specify where to draw it, and where on the sprite sheet the part you want to draw is and how big it is. Make sure the file is 256x256 or a multiple of that (E.g. 512x512.) The position and size is also assuming that your sheet is 256x256, so position (128, 0) is always the top middle. If you don't want to do it that way (I'd recommend you do because you don't have to put your icons in the mods folder and you can put everything in one file.), you are probably just not getting the path right. I haven't done icons the way you tried, as I said, but I don't think you're supposed to put icon files in your mods folder. I'd recommend you make a gui folder right next to your code and reference it in there. A path like "/legendz/gui/npcdialog.png" should work fine.
-
I don't have any actual experience with this, but I do have a few ideas. First, when dealing with something from Minecraft itself like this, look at the source code. The second thing is that I know the way pistons work is they basically "deconstruct" the block, turn it into an entity so that it can move, and then "reconstructs" it where it ends up. I have a suspicion that either the block's rendering is turned off or it is actually destroyed before the movement is done. Try adding println() statements to figure out what events are being triggered or if the block may be getting recreated (which feels excessive and isn't that likely.)
-
I don't have any actual experience with this, but I do have a few ideas. First, when dealing with something from Minecraft itself like this, look at the source code. The second thing is that I know the way pistons work is they basically "deconstruct" the block, turn it into an entity so that it can move, and then "reconstructs" it where it ends up. I have a suspicion that either the block's rendering is turned off or it is actually destroyed before the movement is done. Try adding println() statements to figure out what events are being triggered or if the block may be getting recreated (which feels excessive and isn't that likely.)
-
I have a feeling the bug you're experiencing is because of the way the rendering is done. It doesn't render them absolutely, but relatively to the camera. So in order for anything to appear in a fixed position, its render position must be subtracted by the camera position. If you don't do this subtraction, it'll render in whatever position you specified relative to the camera, always. In this case, you probably specified something like (0, 1, 0) if it's above your head. Check the position of (x, y, z) in the render class and you'll see that they constantly change based on where the player (camera) is, which is why you can use them as if they're absolute coordinates like most everything else using xyz.
-
I have a feeling the bug you're experiencing is because of the way the rendering is done. It doesn't render them absolutely, but relatively to the camera. So in order for anything to appear in a fixed position, its render position must be subtracted by the camera position. If you don't do this subtraction, it'll render in whatever position you specified relative to the camera, always. In this case, you probably specified something like (0, 1, 0) if it's above your head. Check the position of (x, y, z) in the render class and you'll see that they constantly change based on where the player (camera) is, which is why you can use them as if they're absolute coordinates like most everything else using xyz.
-
There is a hook for interacting with blocks and with buckets. You can't detect breaking and placing blocks directly, however, you can cancel the PlayerInteractEvent to prevent breaking and placing. One of the features of the mod I'm working on can define a region to prevent players from doing anything in it. For placing blocks, since the block you clicked on and where the block actually gets placed vary, I'd suggest you do this: @ForgeSubscribe public void onBlock(PlayerInteractEvent event) { int x = event.x, z = event.z; if (event.action == PlayerInteractEvent.Action.RIGHT_CLICK_BLOCK) { switch(event.face) { case 2: z --; break; case 3: z ++; break; case 4: x --; break; case 5: x ++; break; } } } Using that, you can then use the new x and z (and y if you want to add that in) positions to check for permissions. You can go even further and check if the item in the player's hand is an ItemBlock (which means it'll probably be placed shortly after this event triggers). That's probably about the closest you can get to checking for a player placing a block aside from checking if there is a block somewhere there wasn't one before, but then you'd have to handle removing the block yourself. As for the buckets: @ForgeSubscribe public void onBucket(FillBucketEvent event) { int x = event.target.blockX, z = event.target.blockZ; if (event.target.typeOfHit.ordinal() == 0) { switch(event.target.sideHit) { case 2: z --; break; case 3: z ++; break; case 4: x --; break; case 5: x ++; break; } } } Is the synonymous code I use for catching bucket events. This event should trigger for both filling and emptying buckets.
-
There is a hook for interacting with blocks and with buckets. You can't detect breaking and placing blocks directly, however, you can cancel the PlayerInteractEvent to prevent breaking and placing. One of the features of the mod I'm working on can define a region to prevent players from doing anything in it. For placing blocks, since the block you clicked on and where the block actually gets placed vary, I'd suggest you do this: @ForgeSubscribe public void onBlock(PlayerInteractEvent event) { int x = event.x, z = event.z; if (event.action == PlayerInteractEvent.Action.RIGHT_CLICK_BLOCK) { switch(event.face) { case 2: z --; break; case 3: z ++; break; case 4: x --; break; case 5: x ++; break; } } } Using that, you can then use the new x and z (and y if you want to add that in) positions to check for permissions. You can go even further and check if the item in the player's hand is an ItemBlock (which means it'll probably be placed shortly after this event triggers). That's probably about the closest you can get to checking for a player placing a block aside from checking if there is a block somewhere there wasn't one before, but then you'd have to handle removing the block yourself. As for the buckets: @ForgeSubscribe public void onBucket(FillBucketEvent event) { int x = event.target.blockX, z = event.target.blockZ; if (event.target.typeOfHit.ordinal() == 0) { switch(event.target.sideHit) { case 2: z --; break; case 3: z ++; break; case 4: x --; break; case 5: x ++; break; } } } Is the synonymous code I use for catching bucket events. This event should trigger for both filling and emptying buckets.
-
Do I have to anything special to make a mod multiplayer?
Tekner replied to Zeretul4's topic in Modder Support
It makes no sense why the initialization of a Block should cause a gui error. I have a feeling you set your block up wrong. Blocks should only extend Block or BlockContainer, not any gui classes. The only "gui" code a block should really have is player.openGui(). Guis are handled by a gui handler, not the block. I'll need to see your class code. From the looks of it, your frigerator extends ModBlocks? If that's the case, I'll need to see that too. -
Do I have to anything special to make a mod multiplayer?
Tekner replied to Zeretul4's topic in Modder Support
It makes no sense why the initialization of a Block should cause a gui error. I have a feeling you set your block up wrong. Blocks should only extend Block or BlockContainer, not any gui classes. The only "gui" code a block should really have is player.openGui(). Guis are handled by a gui handler, not the block. I'll need to see your class code. From the looks of it, your frigerator extends ModBlocks? If that's the case, I'll need to see that too. -
Try using GameRegistry.findBlock(string modId, String name); You can then just access its blockId through that.