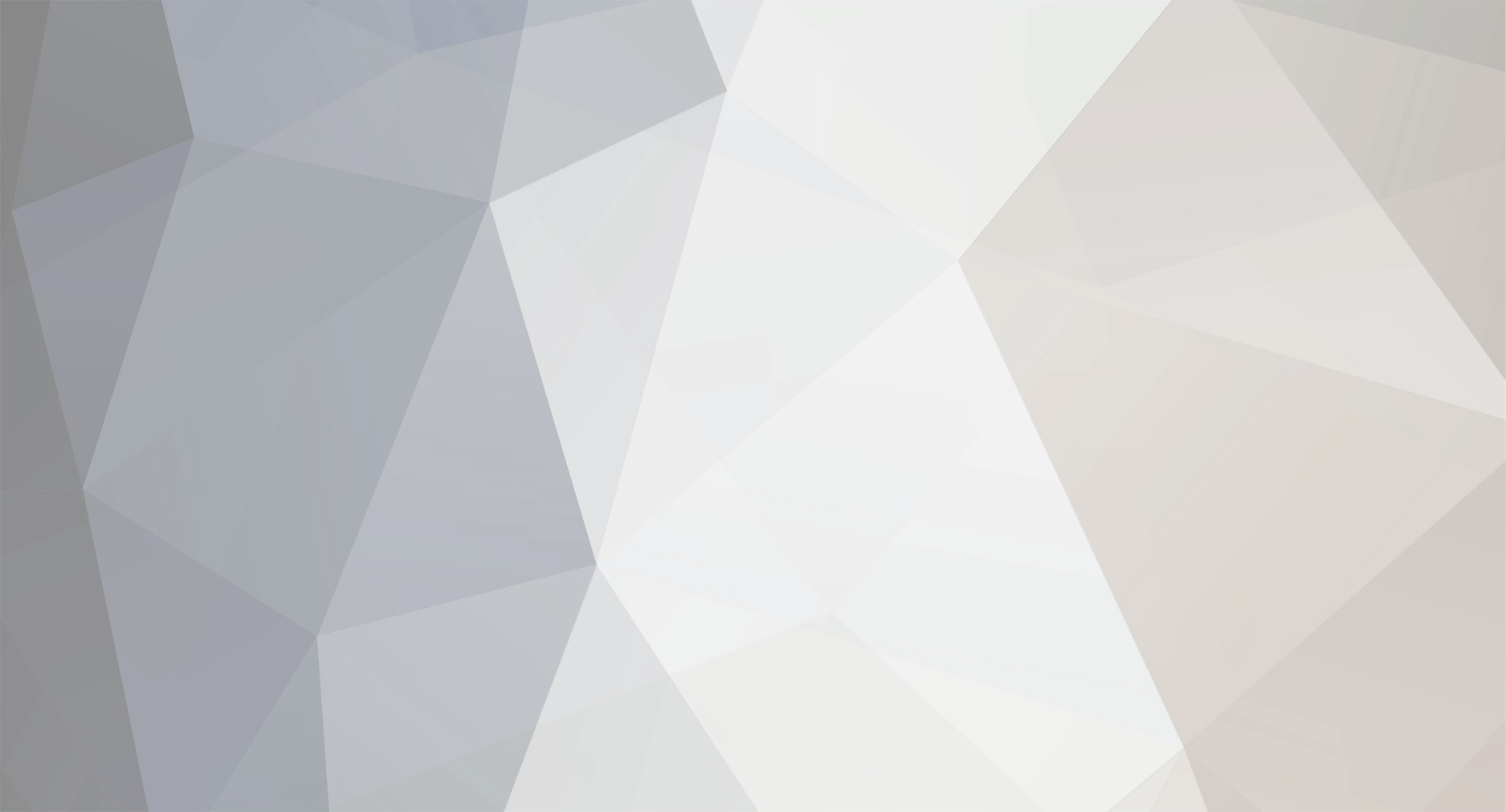
dev909
-
Posts
45 -
Joined
-
Last visited
Posts posted by dev909
-
-
Proxy Class:
Item blockStainedStoneBricks = GameRegistry.findItem(ColoramaMod.MODID, ColoramaReg.blockStainedStoneBricks.getUnlocalizedName().substring(5)); registerItemRenders(blockStainedStoneBricks, 4); public void registerItemRenders(Item item, int meta) { for (int i = 0; i < meta; i++) { ModelResourceLocation itemModelResourceLocation = new ModelResourceLocation(getFullName(item) + "_" + String.valueOf(i), "inventory"); ModelLoader.setCustomModelResourceLocation(item, i, itemModelResourceLocation); } }
Registration class
private static String NAME_BLOCK_STAINED_STONE_BRICKS = "stainedStoneBricks"; GameRegistry.registerBlock(blockStainedStoneBricks = new BlockStainedStoneBrick(Material.rock, NAME_BLOCK_STAINED_STONE_BRICKS), IBStainedStoneBricks.class, NAME_BLOCK_STAINED_STONE_BRICKS);
ItemBlock
@Override public String getUnlocalizedName(ItemStack stack) { BlockStainedStoneBrick.EnumType enumType = BlockStainedStoneBrick.EnumType.byMetadata(stack.getMetadata()); return super.getUnlocalizedName() + "." + enumType.toString(); }
Thats the snippet of which blocks arn't working. From the crash file/testing in game, the models themselves are fine. Its just having problems with the inventory rendering.
-
I normally don't post random files, but almost every other thread that I found related to this someone asked to see their jar file. Just trying to cut down on time.
What exact code do you need to see? The customModelResourceLocations? The block declarations?
-
Hey all,
So after building my mod I'm missing just some of the models from the mod. I have searched around and it seems like the number one cause is mismatched filenames. I checked it and no avail. I even copy and pasted the entire assets folder into the jar and it still didn't work. Weirdly enough I'm only missing models from my stone brick blocks.
I've posted the errors I got here: http://pastebin.com/zvhZWTPf
I've also posted the jar here: https://drive.google.com/file/d/0B8m1DG4ghVbcZmZnZGN4Qy1kbzg/view?usp=sharing
-
How about the first place you use a World reference?
Also, why are you writing the block metadata to your TE's description packet? You don't DO anything with it and the client should already know.
Sorry for the wait, I've been pretty busy over the last few weeks and kinda forgot about Minecraft. Anyways, I saw a tutorial somewhere online that included the metadata.
-
I don't really understand where I should put World#isRemote. Should it be placed just after where I check the block instance? After I get the TE instance? After I check the NBT compound? Only around the code where I handle the TE? I just tried after getting the NBT tag, and I got the same result I had earlier where the changes would not occur right away for both players, but they do update at the same time. I'll post my TE class (sigh
):
TE.class
@Override public Packet getDescriptionPacket() { NBTTagCompound nbtTagCompound = new NBTTagCompound(); this.writeToNBT(nbtTagCompound); int metadata = getBlockMetadata(); return new S35PacketUpdateTileEntity(this.pos, metadata, nbtTagCompound); } @Override public void onDataPacket(NetworkManager net, S35PacketUpdateTileEntity pkt) { readFromNBT(pkt.getNbtCompound()); } @Override public void writeToNBT(NBTTagCompound parentNBTTagCompound) { super.writeToNBT(parentNBTTagCompound); parentNBTTagCompound.setIntArray("rgb", RGB); } @Override public void readFromNBT(NBTTagCompound parentNBTTagCompound) { super.readFromNBT(parentNBTTagCompound); int[] rgbIn = DEFAULT_RGB; if (parentNBTTagCompound.hasKey("rgb")) { rgbIn = parentNBTTagCompound.getIntArray("rgb"); } RGB = rgbIn; }
-
Ok so I now got:
Block block = worldIn.getBlockState(pos).getBlock(); //Check block against target blocks if (block instanceof CBlock) { TileEntity te = worldIn.getTileEntity(pos); if (te instanceof CBlock_TE) { CBlock_TE tile = (CBlock_TE) te; NBTTagCompound nbt = stack.getTagCompound(); if (nbt != null && nbt.hasKey("rgb")) { int[] rgb = nbt.getIntArray("rgb"); tile.setColor(rgb[0], rgb[1], rgb[2]); worldIn.markAndNotifyBlock(pos, worldIn.getChunkFromBlockCoords(pos), block.getDefaultState(), block.getDefaultState(), 3); stack.damageItem(1, playerIn); return true; } else { return false; } } }
Same thing as the original problem. Updates instantly for one player, but the other is delayed...
-
I didn't know what the flags were, so I just put a 0 there. Is there a list of flags somewhere?
-
And World#markAndNotifyBlock does not work. I have used in by itself and with markBlockForUpdate/markDirty.
-
So would something like this do?
Block block = worldIn.getBlockState(pos).getBlock(); //Check block against target blocks if (block instanceof CBlock) { TileEntity te = worldIn.getTileEntity(pos); if (te instanceof CBlock_TE) { CBlock_TE tile = (CBlock_TE) te; NBTTagCompound nbt = stack.getTagCompound(); if (nbt != null && nbt.hasKey("rgb")) { if (!worldIn.isRemote) { int[] rgb = nbt.getIntArray("rgb"); tile.setColor(rgb[0], rgb[1], rgb[2]); tile.getWorld().markBlockForUpdate(pos); tile.markDirty(); stack.damageItem(1, playerIn); return true; } } else { return false; } } }
and for World#markAndNotifyblock....the last param is int flags. What are the flags I could use?
EDIT: Nevermind it doesn't. I tried a couple different ways to place world.isremote in the code and every way doesn't work. The closest thing I got was that both test clients updated at the same time...but there was still a huge delay before the update occured. Also from reading into world.isremote, if isremote is false, then it means dedicated server right? If its true then its a 'combined client'? To get it to work on both sides, wouldn't I have to make two clauses, one for dedicated and one for combined? But they would both have to be the same code in order to work correctly; as in in the combined clause, in order for it to work the same in single player and lan, wouldn't the code have to be the same as the dedicated clause? Because it works fine for single player, but when opened to lan the game world should still be combined (isremote true)? And when in lan (combined) the update delay still occurs so I need to use the dedicated server clause code to fix it? Does anyone get what I mean? Or did I just completely overthink and confuse myself (and possibly others)? lol
-
I have a TE that, when in single player, works perfectly. Such as that I click the block, logic happens and the appearance changes instantly. I thought that was it for the TE. When I complied a test mod for my brother to try with me, we noticed that whenever one of us click on the TE block, the changes occur some seconds later. The change occurs instantly after the other player (the one who did not click the block) updates the chunk by destroying/placing another block nearby. Now I know to force TE updates that I need to use TE.getWorld().markBlockforUpdate() wheverever I want the update to occur. For good measures I place markDirty() beneath that too. In my TE class, I have both getDescriptionPacket() and onDataPacket() defined. As far as I read, those two methods would allow for updates across the server and all the clients. I have only one field in the NBT (intArray) and I need the entire field, so again from reading about, it seems that getDescriptionPacket()/ondataPacket() is what I need.
Relevant code:
ItemPaintbrush.class
@Override
public boolean onItemUse(ItemStack stack, EntityPlayer playerIn, World worldIn, BlockPos pos, EnumFacing side, float hitX, float hitY, float hitZ) {
//Get block
Block block = worldIn.getBlockState(pos).getBlock();
//Check block against target blocks
if (block instanceof CBlock) {
TileEntity te = worldIn.getTileEntity(pos);
if (te instanceof CBlock_TE) {
CBlock_TE tile = (CBlock_TE) te;
NBTTagCompound nbt = stack.getTagCompound();
if (nbt != null && nbt.hasKey("rgb")) {
int[] rgb = nbt.getIntArray("rgb");
tile.setColor(rgb[0], rgb[1], rgb[2]);
tile.getWorld().markBlockForUpdate(pos);
tile.markDirty();
stack.damageItem(1, playerIn);
return true;
}
else {
return false;
}
}
}
..
..
TE.class
@Override
public Packet getDescriptionPacket() {
NBTTagCompound nbtTagCompound = new NBTTagCompound();
this.writeToNBT(nbtTagCompound);
int metadata = getBlockMetadata();
return new S35PacketUpdateTileEntity(this.pos, metadata, nbtTagCompound);
}
@Override
public void onDataPacket(NetworkManager net, S35PacketUpdateTileEntity pkt) {
readFromNBT(pkt.getNbtCompound());
}
@Override
public void writeToNBT(NBTTagCompound parentNBTTagCompound) {
super.writeToNBT(parentNBTTagCompound);
parentNBTTagCompound.setIntArray("rgb", RGB);
}
@Override
public void readFromNBT(NBTTagCompound parentNBTTagCompound) {
super.readFromNBT(parentNBTTagCompound);
int[] rgbIn = DEFAULT_RGB;
if (parentNBTTagCompound.hasKey("rgb")) {
rgbIn = parentNBTTagCompound.getIntArray("rgb");
}
RGB = rgbIn;
}
..
..
I mean shouldn't this work? Item forces an update, which calls getDescriptionPacket() which sends out to clients that receive it through onDataPacket()?
-
Ah. I did. In a few classes actually. Weird, I don't remember actually importing it. Oh well, I got it fixed now. Thanks alot!
-
scala/actors/threadpool/Arrays
- Why are you using this class (hint: you shouldn't be using it).
I thought it was weird too. But as far as I remember.....I didn't use it. I thought it was just some random underlaying forge class
-
Well if you clicked on this thread then most likely you know what the error is about. I do not. I have googled around and it almost seems like for every thread I found, they was a different unique-ish solution. Here's the crash report:
---- Minecraft Crash Report ----
// Shall we play a game?
Time: 6/3/16 8:09 PM
Description: There was a severe problem during mod loading that has caused the game to fail
net.minecraftforge.fml.common.LoaderException: java.lang.NoClassDefFoundError: scala/actors/threadpool/Arrays
at net.minecraftforge.fml.common.LoadController.transition(LoadController.java:162)
at net.minecraftforge.fml.common.Loader.preinitializeMods(Loader.java:559)
at net.minecraftforge.fml.client.FMLClientHandler.beginMinecraftLoading(FMLClientHandler.java:243)
at net.minecraft.client.Minecraft.func_71384_a(Minecraft.java:417)
at net.minecraft.client.Minecraft.func_99999_d(Minecraft.java:329)
at net.minecraft.client.main.Main.main(SourceFile:124)
at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62)
at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43)
at java.lang.reflect.Method.invoke(Method.java:483)
at net.minecraft.launchwrapper.Launch.launch(Launch.java:135)
at net.minecraft.launchwrapper.Launch.main(Launch.java:28)
Caused by: java.lang.NoClassDefFoundError: scala/actors/threadpool/Arrays
at net.dev909.colorama.data.blocks.BlockPaintcan.<init>(BlockPaintcan.java:49)
at net.dev909.colorama.data.ColoramaReg.init(ColoramaReg.java:115)
at net.dev909.colorama.data.ColoramaMod.preInit(ColoramaMod.java:44)
at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62)
at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43)
at java.lang.reflect.Method.invoke(Method.java:483)
at net.minecraftforge.fml.common.FMLModContainer.handleModStateEvent(FMLModContainer.java:560)
at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62)
at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43)
at java.lang.reflect.Method.invoke(Method.java:483)
at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74)
at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47)
at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322)
at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304)
at com.google.common.eventbus.EventBus.post(EventBus.java:275)
at net.minecraftforge.fml.common.LoadController.sendEventToModContainer(LoadController.java:211)
at net.minecraftforge.fml.common.LoadController.propogateStateMessage(LoadController.java:189)
at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62)
at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43)
at java.lang.reflect.Method.invoke(Method.java:483)
at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74)
at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47)
at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322)
at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304)
at com.google.common.eventbus.EventBus.post(EventBus.java:275)
at net.minecraftforge.fml.common.LoadController.distributeStateMessage(LoadController.java:118)
at net.minecraftforge.fml.common.Loader.preinitializeMods(Loader.java:556)
... 10 more
Caused by: java.lang.ClassNotFoundException: scala.actors.threadpool.Arrays
at net.minecraft.launchwrapper.LaunchClassLoader.findClass(LaunchClassLoader.java:191)
at java.lang.ClassLoader.loadClass(ClassLoader.java:424)
at java.lang.ClassLoader.loadClass(ClassLoader.java:357)
... 40 more
Caused by: java.lang.NullPointerException
at net.minecraft.launchwrapper.LaunchClassLoader.findClass(LaunchClassLoader.java:182)
... 42 more
A detailed walkthrough of the error, its code path and all known details is as follows:
---------------------------------------------------------------------------------------
-- System Details --
Details:
Minecraft Version: 1.8.9
Operating System: Windows 10 (amd64) version 10.0
Java Version: 1.8.0_25, Oracle Corporation
Java VM Version: Java HotSpot 64-Bit Server VM (mixed mode), Oracle Corporation
Memory: 108441080 bytes (103 MB) / 262320128 bytes (250 MB) up to 1060372480 bytes (1011 MB)
JVM Flags: 6 total; -XX:HeapDumpPath=MojangTricksIntelDriversForPerformance_javaw.exe_minecraft.exe.heapdump -Xmx1G -XX:+UseConcMarkSweepGC -XX:+CMSIncrementalMode -XX:-UseAdaptiveSizePolicy -Xmn128M
IntCache: cache: 0, tcache: 0, allocated: 0, tallocated: 0
FML: MCP 9.19 Powered by Forge 11.15.1.1847 4 mods loaded, 4 mods active
States: 'U' = Unloaded 'L' = Loaded 'C' = Constructed 'H' = Pre-initialized 'I' = Initialized 'J' = Post-initialized 'A' = Available 'D' = Disabled 'E' = Errored
UCH mcp{9.19} [Minecraft Coder Pack] (minecraft.jar)
UCH FML{8.0.99.99} [Forge Mod Loader] (forge-1.8.9-11.15.1.1847.jar)
UCH Forge{11.15.1.1847} [Minecraft Forge] (forge-1.8.9-11.15.1.1847.jar)
UCE colorama{BETA_1.0} [colorAMA!] (colorama-BETA_1.0.jar)
Loaded coremods (and transformers):
GL info: ' Vendor: 'NVIDIA Corporation' Version: '4.5.0 NVIDIA 364.72' Renderer: 'GeForce GTX 770/PCIe/SSE2'
File structure within the jar file:
jar/net/dev909/colorama/proxy
jar/net/dev909/colorama/data
jar/net/dev909/colorama/data/utils
jar/net/dev909/colorama/data/tileentities
jar/net/dev909/colorama/data/items
jar/net/dev909/colorama/data/blocks
jar/assets/colorama/textures
jar/assets/colorama/models
jar/assets/colorama/lang
jar/assets/colorama/blockstates
I don't understand what's happening...
-
Ahhhhhhhhhhhhhhhhhhhhhhhhhhhh I forgot to look into the itemblock...oops heheh
Yeah I got it fixed now. Didn't realize that for some reason my ItemBlock#getUnlocalizedName(ItemStack) was returning only the integer value of the blockstate, and not calling the block's actual getUnlocalizedName(). Thanks alot!
-
So I have a couple blocks in my mod that have different properties. One block for example, uses PropertyEnum. In my lang file, tile.blockname.enum_name.name works. Another block though uses PropertyInteger. When I try to set a name like tile.blockname.integer_value.name it does not work. I checked through vanilla code at blocks like anvil, cactus, cake, etc, and most of them just uses the format tile.blockname.name, without specifying the integer value. This doesn't work for me either. So how do blocks with PropertyIntegers work for lang files?
One thing I noticed is that in game, the PropertyInteger block's name is not in the format of tile.blockname.name. It's in the format of modID:blockname[PropertyInteger_name=integer_value].name. I think this is slightly weird. Also I tried using modID:blockname[PropertyInteger_name=integer_value].name and a few slight variations to see if they worked but nothing did work.
-
I have a custom item which i want to apply damage to when i click a custom block. The whole point to it is that after clicking a block, it takes one more damage until its damage is at 0 and must click another block for it to "refill" the damage before being used again. In the items constructor i set the max damage to 16. In the items use method, after all other logic is done, i call the items set damage method and set it as 1 lower than what it currently is. Also when i click on another certain block im calling the set damage method to the items max damage, as in the item gets fully repaired (without using anvils). In game this doesnt work, and no damage bar is shown on the item. I cannot post my code as im not home on my computer. So basically the question is how do i allow an item to lower its damage when clicked on a certain block, and how can the items damage "refill" after clicking another block. Or in better terms, how does minecraft handle item damage to items that are not considered tools?
-
Method is onItemUse(), and it works perfectly, thanks!
As a side query, is there any benefit of having the original code in the blocks use method and the sneaking code in the items use method, compared to having all the code in the items use method? Besides for having cleaner looking code?
-
I have an item and block that interact with each other just fine. I want to add a little bit more functionality by giving the item another 'mode' aka change behavior if the player is sneaking. So I added an if else block into my code to see if player is sneaking, and then do stuff. However in game my debug code will not fire if I'm sneaking and clicking on the block with the item. When I switch to an empty hand, the debug code fires and then it crashes (because the clause with the logic for sneaking is supposed to be using a certain item, not an empty hand). So this is making me wonder if it is possible to right click a block, while sneaking, with a specific item?
All code is done within the block's onBlockActivated() method.
-
I read about this not too long ago. I believe its not technically fired twice, its only being printed teice; once for client and once for server. If you read the full line of the console it will have a client or server tag in front. Shouldnt really be a big deal but if you want it to print once you have to add @sideOnly before the method I think...
-
Ah, works now. Thank you so much! Out of curiosity the missing closing tag you edited about...is that something I should know for nbt, or is it just something that was missing like /code] in your post?
-
Post your new code.
Right...forgot about that..
Here's the relevant classes. The approach is to use an int array. Code flow is paintcan: set paintbrush color -> block: get color from paintbrush -> tileentity: get color from block, update -> block: render new color.
COBlock.java
@Override public boolean onBlockActivated(World worldIn, BlockPos pos, IBlockState state, EntityPlayer playerIn, EnumFacing side, float hitX, float hitY, float hitZ) { System.out.println("> onblockactivated"); if (ItemStack.areItemsEqual(playerIn.getCurrentEquippedItem(), new ItemStack(ColoramaReg.COPaintbrush))) { System.out.println("> objects are equal"); TileEntity te = worldIn.getTileEntity(pos); System.out.println("> got tileentity"); if (te instanceof COBlock_TE) { COBlock_TE tile = (COBlock_TE)te; NBTTagCompound nbtTagCompound = playerIn.getCurrentEquippedItem().getTagCompound(); if (nbtTagCompound != null && nbtTagCompound.hasKey("rgb")) { int[] rgb = nbtTagCompound.getIntArray("rgb"); tile.setColor(rgb[0], rgb[1], rgb[2]); tile.getWorld().markBlockForUpdate(pos); System.out.println("> color changed"); return true; } else { return false; } } } return false; }
COBlock_TE.java
public class COBlock_TE extends TileEntity { private static final int[] DEFAULT_RGB = {255,255,255}; private int[] RGB = DEFAULT_RGB; public int getColor() {return convertToInt(RGB[0],RGB[1],RGB[2]);} public void setColor(int r, int g, int b) { this.RGB[0] = r; this.RGB[1] = g; this.RGB[2] = b; } public static int convertToInt(int r, int g, int b) {return (r*65536)+(g*256)+b;} @Override public Packet getDescriptionPacket() { NBTTagCompound nbtTagCompound = new NBTTagCompound(); writeToNBT(nbtTagCompound); int metadata = getBlockMetadata(); return new S35PacketUpdateTileEntity(this.pos, metadata, nbtTagCompound); } @Override public void onDataPacket(NetworkManager net, S35PacketUpdateTileEntity pkt) { readFromNBT(pkt.getNbtCompound()); } @Override public void writeToNBT(NBTTagCompound parentNBTTagCompound) { super.writeToNBT(parentNBTTagCompound); parentNBTTagCompound.setIntArray("rgb", RGB); } @Override public void readFromNBT(NBTTagCompound parentNBTTagCompound) { super.readFromNBT(parentNBTTagCompound); int[] rgbIn = DEFAULT_RGB; if (parentNBTTagCompound.hasKey("rgb")) { rgbIn = parentNBTTagCompound.getIntArray("rgb"); } RGB = rgbIn; } }
COBlockPaintcan.java
@Override public boolean onBlockActivated(World worldIn, BlockPos pos, IBlockState state, EntityPlayer playerIn, EnumFacing side, float hitX, float hitY, float hitZ) { System.out.println("> onblockactivated"); if (ItemStack.areItemStacksEqual(playerIn.getCurrentEquippedItem(), new ItemStack(ColoramaReg.COPaintbrush))) { System.out.println("> objects are equal"); NBTTagCompound nbtTagCompound = playerIn.getCurrentEquippedItem().getTagCompound(); System.out.println("> nbt tag...."); if (nbtTagCompound == null) { nbtTagCompound = new NBTTagCompound(); playerIn.getCurrentEquippedItem().setTagCompound(nbtTagCompound); System.out.println("> nbt tag created"); } nbtTagCompound.setIntArray("rgb", new int[]{255,0,0}); System.out.println("> nbt tag COLOR set to 255,0,0"); } return false; }
-
Sorry for dragging up an old thread, but I haven't really had much time to code within the last couple months or so.
Anyways I got a tile entity working for what I need, such as that I can right click a block and change its color in real time. Yay! I'm using just one int variable in the TE (color) and for the nbt compound and it works great. Blocks get updated right away with the right color, and only blocks that are clicked. However if I want to expand functionality from just one color, to three values (as in red, green, and blue variables) I get problems. I have tried two different methods that are not really that different from each other. I tried created an int array in the TE, and use int array for the nbt data, and I have tried storing three ints in the nbt data. I have updated the relevant classes for both approaches. No matter which one I choose, whenever I'm in game, if I place multiple blocks down, and right click just ONE, all the blocks get updated. Almost as if the block is acting as a singleton again and not having their own separate entities. Any clues?
-
Hello possible saviors, I've been looking all over the forum, google, and src code for an answer but now I'm here. Ok here's what I'm trying to do: I want to be able to right click a block and render a new color on the block. So far I found two ways to possibly do this. The first way I found the method Block.recolorBlock(), which I think is the best way to go as I'm hoping that it will allow me to color per side (as its arg suggests) and that I can hopefully do it on any block. The second way was to create a custom block, override the color methods (getBlockColor(), getRenderColor(), colorMultiplier()), create a custom model that allows tinting (much like grass), and then when right click, of course change color. Preferably I would rather be able to set a custom color instead of using an enum for the recolorBlock() arg. I tried both ways and nothing has worked so far. Here's the codes:
Method 1 (recolorBlock()):
Item (foo):
public class foo { public boolean onItemUse(ItemStack stack, EntityPlayer playerIn, World worldIn, BlockPos pos, EnumFacing side, float hitX, float hitY, float hitZ) { worldIn.getBlockState(pos).getBlock().recolorBlock(worldIn, pos, EnumFacing.UP, EnumDyeColor.RED); return true; } }
Also tried the opposite way, using onBlockActivated() instead of onItemUse()
Block (foobarBlock):
public class foobarBlock extends Block { public foobarBlock() { super(Material.wood); // TODO Auto-generated constructor stub } public void onBlockActivated(World par1World, int par2, int par3, int par4, EntityPlayer player) { BlockPos pos = new BlockPos(par2,par3,par4); this.recolorBlock(par1World, pos, EnumFacing.UP, EnumDyeColor.RED); } }
Method 2 (Custom blocks):
Block (foorbarBlock):
public class foobarBlock extends Block { private int blockColor; public foobarBlock() { super(Material.wood); //set blockColor to a sample color (teal, cyanish) blockColor = 65535; // TODO Auto-generated constructor stub } public void setBlockColor(int color) { this.blockColor = color; } @SideOnly(Side.CLIENT) public int getBlockColor() { return this.blockColor; } @SideOnly(Side.CLIENT) public int getRenderColor(IBlockState state) { return this.getBlockColor(); } @SideOnly(Side.CLIENT) public int colorMultiplier(IBlockAccess worldIn, BlockPos pos, int renderPass) { return this.getBlockColor(); } }
Item (foo):
public class foo { public boolean onItemUse(ItemStack stack, EntityPlayer playerIn, World worldIn, BlockPos pos, EnumFacing side, float hitX, float hitY, float hitZ) { if (worldIn.getBlockState(pos).getBlock() == Blocks.foobar) { foobarBlock block = (foobarBlock) worldIn.getBlockState(pos).getBlock(); //set block to a red block.setBlockColor(16711680); return true; } else { return false; } } }
-
Where would the config folder be for testing in eclipse? Somewhere in the eclipse folder?
[1.8.9] [Solved] Missing some models after building
in ForgeGradle
Posted
Fixed it. To anyone else with similar problems....seriously check your capitals. My problem was that I was giving my unlocalized name as "stainedStoneBricks". My Item models for the block was "stainedStonebricks". As larsgerrits said,