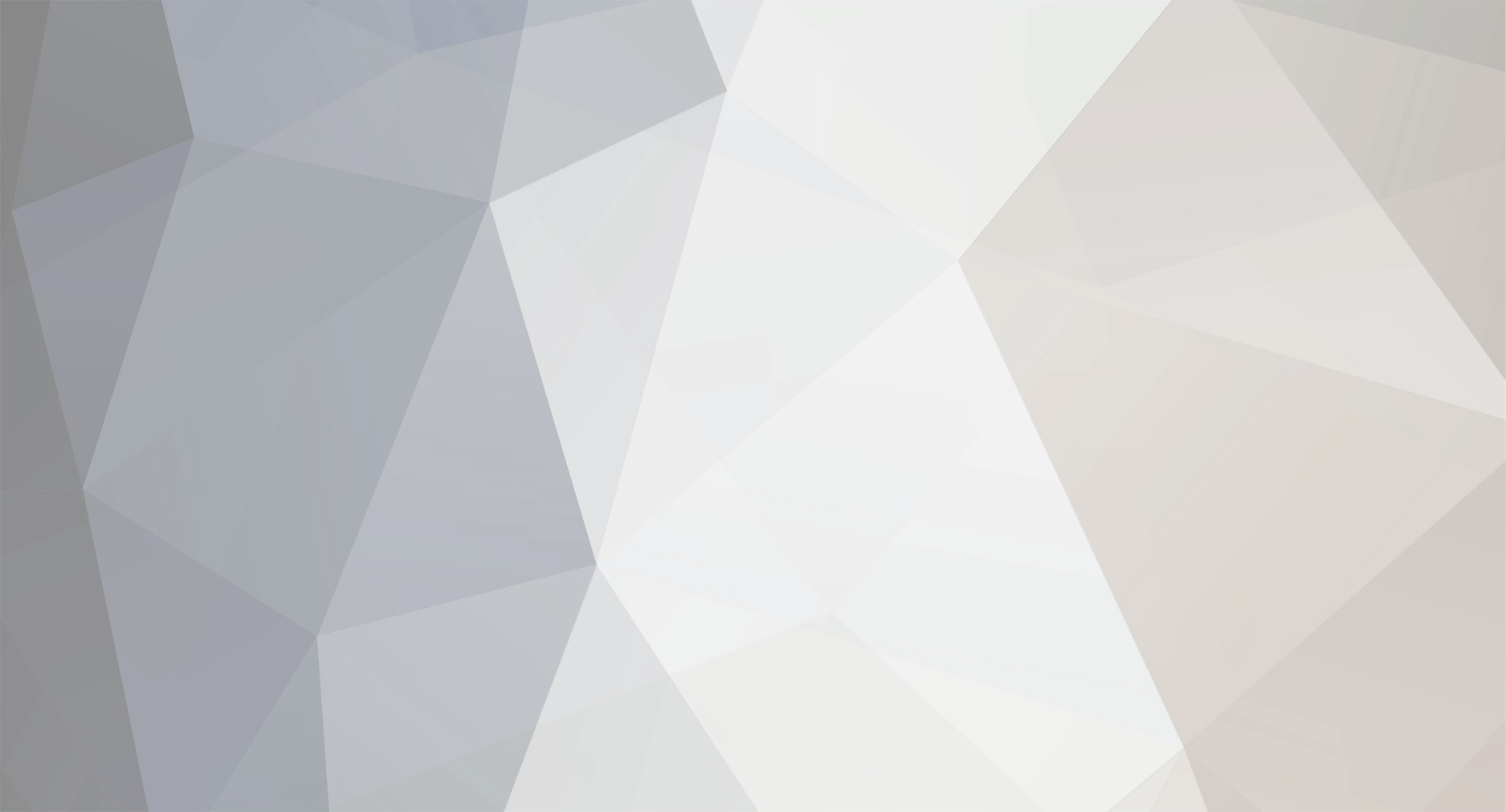
adamAndMath
Members-
Posts
24 -
Joined
-
Last visited
Everything posted by adamAndMath
-
Then use this: @Override public int damageDropped(int meta) { return meta % 4; }
-
Put this into your block: @Override public int damageDropped(int meta) { return meta / 4; }
-
Sorry. Here is the code: package uwe.core.world; import static net.minecraftforge.event.terraingen.InitMapGenEvent.EventType.CAVE; import static net.minecraftforge.event.terraingen.PopulateChunkEvent.Populate.EventType.LAVA; import java.util.List; import java.util.Random; import uwe.core.world.gen.feature.WorldGenNightStone; import net.minecraft.block.Block; import net.minecraft.block.BlockSand; import net.minecraft.entity.EnumCreatureType; import net.minecraft.util.IProgressUpdate; import net.minecraft.util.MathHelper; import net.minecraft.world.ChunkPosition; import net.minecraft.world.SpawnerAnimals; import net.minecraft.world.World; import net.minecraft.world.biome.BiomeGenBase; import net.minecraft.world.chunk.Chunk; import net.minecraft.world.chunk.IChunkProvider; import net.minecraft.world.gen.MapGenBase; import net.minecraft.world.gen.MapGenCaves; import net.minecraft.world.gen.NoiseGeneratorOctaves; import net.minecraft.world.gen.feature.WorldGenLakes; import net.minecraftforge.common.MinecraftForge; import net.minecraftforge.event.Event.Result; import net.minecraftforge.event.terraingen.ChunkProviderEvent; import net.minecraftforge.event.terraingen.PopulateChunkEvent; import net.minecraftforge.event.terraingen.TerrainGen; public class ChunkProviderL1 implements IChunkProvider { float[] parabolicField; private final byte stoneByte = (byte) Block.stone.blockID; private final byte waterByte = (byte) Block.waterStill.blockID; private final int blockMain = Block.stone.blockID; private final int liquidStill = Block.waterStill.blockID; private Random rand; /** A NoiseGeneratorOctaves used in generating nether terrain */ private NoiseGeneratorOctaves noiseGen1; private NoiseGeneratorOctaves noiseGen2; private NoiseGeneratorOctaves noiseGen3; private NoiseGeneratorOctaves noiseGen4; private NoiseGeneratorOctaves noiseGen5; private NoiseGeneratorOctaves noiseGen6; /** Is the world that the nether is getting generated. */ private World worldObj; private double[] noiseField; /** The biomes that are used to generate the chunk */ private BiomeGenBase[] biomesForGeneration; private MapGenBase caveGenerator = new MapGenCaves(); protected double[] noiseData1; protected double[] noiseData2; protected double[] noiseData3; protected double[] noiseData5; protected double[] noiseData6; { caveGenerator = TerrainGen.getModdedMapGen(caveGenerator, CAVE); } public ChunkProviderL1(World par1World, long par2) { this.worldObj = par1World; this.rand = new Random(par2); this.noiseGen1 = new NoiseGeneratorOctaves(this.rand, 16); this.noiseGen2 = new NoiseGeneratorOctaves(this.rand, 16); this.noiseGen3 = new NoiseGeneratorOctaves(this.rand, ; this.noiseGen4 = new NoiseGeneratorOctaves(this.rand, 4); this.noiseGen5 = new NoiseGeneratorOctaves(this.rand, 16); this.noiseGen6 = new NoiseGeneratorOctaves(this.rand, 16); NoiseGeneratorOctaves[] noiseGens = {noiseGen1, noiseGen2, noiseGen3, noiseGen4, noiseGen5, noiseGen6}; noiseGens = TerrainGen.getModdedNoiseGenerators(par1World, this.rand, noiseGens); this.noiseGen1 = noiseGens[0]; this.noiseGen2 = noiseGens[1]; this.noiseGen3 = noiseGens[2]; this.noiseGen4 = noiseGens[3]; this.noiseGen5 = noiseGens[4]; this.noiseGen6 = noiseGens[5]; } /** * Generates the shape of the terrain. */ public void generateTerrain(int chunkX, int chunkZ, byte[] blockArray) { byte chunkSize = 4; byte height = (byte) (64 / chunkSize); byte oceanHeight = 32; int sizeX = chunkSize + 1; byte sizeY = (byte) (height + 1); int sizeZ = chunkSize + 1; this.biomesForGeneration = this.worldObj.getWorldChunkManager().getBiomesForGeneration(this.biomesForGeneration, chunkX * 4 - 2, chunkZ * 4 - 2, sizeX + 5, sizeY + 5); this.noiseField = this.initializeNoiseField(this.noiseField, chunkX * chunkSize, 0, chunkZ * chunkSize, sizeX, sizeY, sizeZ); for(int x = 0; x < chunkSize; ++x) { for(int z = 0; z < chunkSize; ++z) { for(int y = 0; y < height; ++y) { double terrainType = 1.0D; double nf1 = this.noiseField[((x + 0) * sizeZ + z + 0) * sizeY + y + 0]; double nf2 = this.noiseField[((x + 0) * sizeZ + z + 1) * sizeY + y + 0]; double nf3 = this.noiseField[((x + 1) * sizeZ + z + 0) * sizeY + y + 0]; double nf4 = this.noiseField[((x + 1) * sizeZ + z + 1) * sizeY + y + 0]; double nf1plus = (this.noiseField[((x + 0) * sizeZ + z + 0) * sizeY + y + 1] - nf1) * terrainType; double nf2plus = (this.noiseField[((x + 0) * sizeZ + z + 1) * sizeY + y + 1] - nf2) * terrainType; double nf3plus = (this.noiseField[((x + 1) * sizeZ + z + 0) * sizeY + y + 1] - nf3) * terrainType; double nf4plus = (this.noiseField[((x + 1) * sizeZ + z + 1) * sizeY + y + 1] - nf4) * terrainType; int test0 = 2 * chunkSize; int test1 = 16 / chunkSize; for(int exY = 0; exY < test0; ++exY) { double d9 = 0.25D; double mainNoise = nf1; double secondNoise = nf2; double d12 = (nf3 - nf1) * d9; double d13 = (nf4 - nf2) * d9; for(int exX = 0; exX < test1; ++exX) { int place = exX + x * test1 << 11 | 0 + z * test1 << 7 | y * test0 + exY; short worldHeight = 128; double d14 = 0.25D; double d15 = (secondNoise - mainNoise) * d14; double d16 = mainNoise - d15; for(int exZ = 0; exZ < test1; ++exZ) { int placedBlock = 0; if ((d16 += d15) > 0.0D) { placedBlock = stoneByte; } else if (y * 8 + exY < oceanHeight) { placedBlock = waterByte; } blockArray[place] = (byte) placedBlock; place += worldHeight; } mainNoise += d12; secondNoise += d13; } nf1 += nf1plus; nf2 += nf2plus; nf3 += nf3plus; nf4 += nf4plus; } } } } } /** * name based on ChunkProviderGenerate */ public void replaceBlocksForBiome(int par1, int par2, byte[] blockArray, BiomeGenBase[] biomes) { ChunkProviderEvent.ReplaceBiomeBlocks event = new ChunkProviderEvent.ReplaceBiomeBlocks(this, par1, par2, blockArray, biomes); MinecraftForge.EVENT_BUS.post(event); if (event.getResult() == Result.DENY) { return; } byte waterHeight = 63; for (int posX = 0; posX < 16; ++posX) { for (int posZ = 0; posZ < 16; ++posZ) { byte air = (byte) blockMain; byte ground = (byte) blockMain; for (int posY = 127; posY >= 0; --posY) { int pos = (posZ * 16 + posX) * 128 + posY; if (posY <= 0 + this.rand.nextInt(5)) { blockArray[pos] = (byte) Block.bedrock.blockID; } else if (posY >= 127 - this.rand.nextInt(5)) { blockArray[pos] = (byte) Block.bedrock.blockID; } else { if (blockArray[pos] == stoneByte) { if (posY >= waterHeight - 4 && posY <= waterHeight + 1) { air = (byte) blockMain; ground = (byte) blockMain; } if (posY < waterHeight && air == 0) { air = (byte) liquidStill; } if (posY >= waterHeight - 1) { blockArray[pos] = air; } else { blockArray[pos] = ground; } } } } } } } @Override public Chunk loadChunk(int chunkX, int chunkZ) { return this.provideChunk(chunkX, chunkZ); } @Override public Chunk provideChunk(int chunkX, int chunkZ) { this.rand.setSeed((long) chunkX * 341873128712L + (long) chunkZ * 132897987541L); byte[] blockArray = new byte[32768]; this.generateTerrain(chunkX, chunkZ, blockArray); this.biomesForGeneration = this.worldObj.getWorldChunkManager().loadBlockGeneratorData(this.biomesForGeneration, chunkX * 16, chunkZ * 16, 16, 16); this.replaceBlocksForBiome(chunkX, chunkZ, blockArray, biomesForGeneration); this.caveGenerator.generate(this, this.worldObj, chunkX, chunkZ, blockArray); Chunk chunk = new Chunk(this.worldObj, blockArray, chunkX, chunkZ); byte[] biomeIds = chunk.getBiomeArray(); for(int i = 0; i < biomeIds.length; ++i) { biomeIds[i] = (byte) biomesForGeneration[i].biomeID; } chunk.resetRelightChecks(); return chunk; } private double[] initializeNoiseField(double[] par1ArrayOfDouble, int par2, int par3, int par4, int par5, int par6, int par7) { ChunkProviderEvent.InitNoiseField event = new ChunkProviderEvent.InitNoiseField(this, par1ArrayOfDouble, par2, par3, par4, par5, par6, par7); MinecraftForge.EVENT_BUS.post(event); if (event.getResult() == Result.DENY) return event.noisefield; if (par1ArrayOfDouble == null) { par1ArrayOfDouble = new double[par5 * par6 * par7]; } if (this.parabolicField == null) { this.parabolicField = new float[25]; for (int k1 = -2; k1 <= 2; ++k1) { for (int l1 = -2; l1 <= 2; ++l1) { float f = 10.0F / MathHelper.sqrt_float((float)(k1 * k1 + l1 * l1) + 0.2F); this.parabolicField[k1 + 2 + (l1 + 2) * 5] = f; } } } double d0 = 684.412D; double d1 = 684.412D; this.noiseData5 = this.noiseGen5.generateNoiseOctaves(this.noiseData5, par2, par4, par5, par7, 1.121D, 1.121D, 0.5D); this.noiseData6 = this.noiseGen6.generateNoiseOctaves(this.noiseData6, par2, par4, par5, par7, 200.0D, 200.0D, 0.5D); this.noiseData3 = this.noiseGen3.generateNoiseOctaves(this.noiseData3, par2, par3, par4, par5, par6, par7, d0 / 80.0D, d1 / 160.0D, d0 / 80.0D); this.noiseData1 = this.noiseGen1.generateNoiseOctaves(this.noiseData1, par2, par3, par4, par5, par6, par7, d0, d1, d0); this.noiseData2 = this.noiseGen2.generateNoiseOctaves(this.noiseData2, par2, par3, par4, par5, par6, par7, d0, d1, d0); int i2 = 0; int j2 = 0; for (int k2 = 0; k2 < par5; ++k2) { for (int l2 = 0; l2 < par7; ++l2) { float f1 = 0.0F; float f2 = 0.0F; float f3 = 0.0F; byte b0 = 2; BiomeGenBase biomegenbase = this.biomesForGeneration[k2 + 2 + (l2 + 2) * (par5 + 5)]; for (int i3 = -b0; i3 <= b0; ++i3) { for (int j3 = -b0; j3 <= b0; ++j3) { BiomeGenBase biomegenbase1 = this.biomesForGeneration[k2 + i3 + 2 + (l2 + j3 + 2) * (par5 + 5)]; float f4 = this.parabolicField[i3 + 2 + (j3 + 2) * 5] / (biomegenbase1.minHeight + 2.0F); if (biomegenbase1.minHeight > biomegenbase.minHeight) { f4 /= 2.0F; } f1 += biomegenbase1.maxHeight * f4; f2 += biomegenbase1.minHeight * f4; f3 += f4; } } f1 /= f3; f2 /= f3; f1 = f1 * 0.9F + 0.1F; f2 = (f2 * 4.0F - 1.0F) / 8.0F; double d2 = this.noiseData6[j2] / 8000.0D; if (d2 < 0.0D) { d2 = -d2 * 0.3D; } d2 = d2 * 3.0D - 2.0D; if (d2 < 0.0D) { d2 /= 2.0D; if (d2 < -1.0D) { d2 = -1.0D; } d2 /= 1.4D; d2 /= 2.0D; } else { if (d2 > 1.0D) { d2 = 1.0D; } d2 /= 8.0D; } ++j2; for (int k3 = 0; k3 < par6; ++k3) { double d3 = (double)f2; double d4 = (double)f1; d3 += d2 * 0.2D; d3 = d3 * (double)par6 / 16.0D; double d5 = (double)par6 / 2.0D + d3 * 4.0D; double d6 = 0.0D; double d7 = ((double)k3 - d5) * 12.0D * 128.0D / 128.0D / d4; if (d7 < 0.0D) { d7 *= 4.0D; } double d8 = this.noiseData1[i2] / 512.0D; double d9 = this.noiseData2[i2] / 512.0D; double d10 = (this.noiseData3[i2] / 10.0D + 1.0D) / 2.0D; if (d10 < 0.0D) { d6 = d8; } else if (d10 > 1.0D) { d6 = d9; } else { d6 = d8 + (d9 - d8) * d10; } d6 -= d7; if (k3 > par6 - 4) { double d11 = (double)((float)(k3 - (par6 - 4)) / 3.0F); d6 = d6 * (1.0D - d11) + -10.0D * d11; } par1ArrayOfDouble[i2] = d6; ++i2; } } } return par1ArrayOfDouble; } @Override public boolean chunkExists(int par1, int par2) { return true; } @Override public void populate(IChunkProvider chunkProvider, int chunkX, int chunkZ) { BlockSand.fallInstantly = true; int posX = chunkX * 16; int posZ = chunkZ * 16; BiomeGenBase biomegenbase = worldObj.getBiomeGenForCoords(posX + 16, posZ + 16); rand.setSeed(worldObj.getSeed()); long seedX = rand.nextLong() / 2L * 2L + 1L; long seedZ = rand.nextLong() / 2L * 2L + 1L; rand.setSeed((long)chunkX * seedX + (long)chunkZ * seedZ ^ worldObj.getSeed()); boolean flag = false; MinecraftForge.EVENT_BUS.post(new PopulateChunkEvent.Pre(chunkProvider, worldObj, rand, chunkX, chunkZ, flag)); int x; int y; int z; int generates = rand.nextInt(rand.nextInt(10) + 1); for(int i = 0; i < generates; ++i) { x = posX + rand.nextInt(16) + 8; y = rand.nextInt(128 + 4); z = posZ + rand.nextInt(16) + 8; (new WorldGenNightStone()).generate(worldObj, rand, x, y, z); } for(int i = 0; i < 10; ++i) { x = posX + rand.nextInt(16) + 8; y = rand.nextInt(128); z = posZ + rand.nextInt(16) + 8; (new WorldGenNightStone()).generate(worldObj, rand, x, y, z); } if (TerrainGen.populate(chunkProvider, worldObj, rand, chunkX, chunkZ, flag, LAVA) && !flag && this.rand.nextInt( == 0) { x = posX + this.rand.nextInt(16) + 8; y = this.rand.nextInt(this.rand.nextInt(120) + ; z = posZ + this.rand.nextInt(16) + 8; if (y < 63 || this.rand.nextInt(10) == 0) { (new WorldGenLakes(Block.lavaStill.blockID)).generate(this.worldObj, this.rand, x, y, z); } } biomegenbase.decorate(this.worldObj, this.rand, posX, posZ); SpawnerAnimals.performWorldGenSpawning(this.worldObj, biomegenbase, posX + 8, posZ + 8, 16, 16, this.rand); MinecraftForge.EVENT_BUS.post(new PopulateChunkEvent.Post(chunkProvider, worldObj, rand, chunkX, chunkZ, flag)); BlockSand.fallInstantly = false; } @Override public boolean saveChunks(boolean par1, IProgressUpdate par2IProgressUpdate) { return true; } @Override public void func_104112_b() { } @Override public boolean unloadQueuedChunks() { return false; } @Override public boolean canSave() { return true; } @Override public String makeString() { return "Layer1RandomLevelSource"; } @Override @SuppressWarnings("rawtypes") public List getPossibleCreatures(EnumCreatureType par1EnumCreatureType, int par2, int par3, int par4) { BiomeGenBase var5 = this.worldObj.getBiomeGenForCoords(par2, par4); return var5 == null? null : var5.getSpawnableList(par1EnumCreatureType); } @Override public ChunkPosition findClosestStructure(World par1World, String par2Str, int par3, int par4, int par5) { return null; } @Override public int getLoadedChunkCount() { return 0; } @Override public void recreateStructures(int par1, int par2) { } }
-
This is my world right now: [/img] As you can see there a lots of layers missing, and i don't know why. If someone could tell me how to fix it, it would be awesome.
-
SideOnly means that the thing is only used on a specific side. And When you then say Side.Client, you say, that it is only used on the client side.
-
Hey guys. I'm doing the same as you, and it seems to me, that it will log in after some time. though I might be wrong.
-
I would try to reinstall mcp
-
is that CallableLvl1 class yours, course if it isn't, I would say you change some existing code.
-
Thanks again, but the only way i can see for making roof is to higher d0, but when doing that the terrain becomes nethery.
-
Sorry, I must be blind, because I cant see any differences between the different generateTerrain.
-
Ok, and I have spend so much time renaming variables. Well I would like to generate a dimension with a roof that sortof follows the grounds height. Can you tell me have to use the output of "initializeNoiseField".
-
Thanks, but I mostly asked because I dont now the functionality of the function "initializeNoiseField".
-
wacth this vidio it helped me alot
-
This is the only thing I need done before releasing my mod
-
I'm making a mod, which include new dimensions, and I was wondering, if someone could point mi to an in depth tutorial on terrain generation?
-
Dedicated server keeps saying NoClassDefFoundError guiScreen.java
adamAndMath replied to WilliamLe's topic in Modder Support
Instead of this @NetworkMod I think you should use this @NetworkMod(clientSideRequired = true, serverSideRequired = true) -
Thanks that worked
-
You need to return the container on the server side
-
Can we see your IGuiHandler, it would make it a lot easier to help you.
-
I have already made it generate, but i need to know were it is generated before it is, so that i can make a device, that points towards the closest structure.
-
I don't know how to add a StructureStart to a already existing dimension.
-
thanks, but i have already made the base structure. I just want it to generate like when using StructureStart. I know it would work extending it and generating it in a knew dimension but i don't know how to do it in already existing ones.
-
I want to generate a structure like Strongholds and Mineshafts. I need it to generate a exact amount of times and to be randomly shaped. If somebody could please give me an example or an explanation on how to do this, it would help me so much.