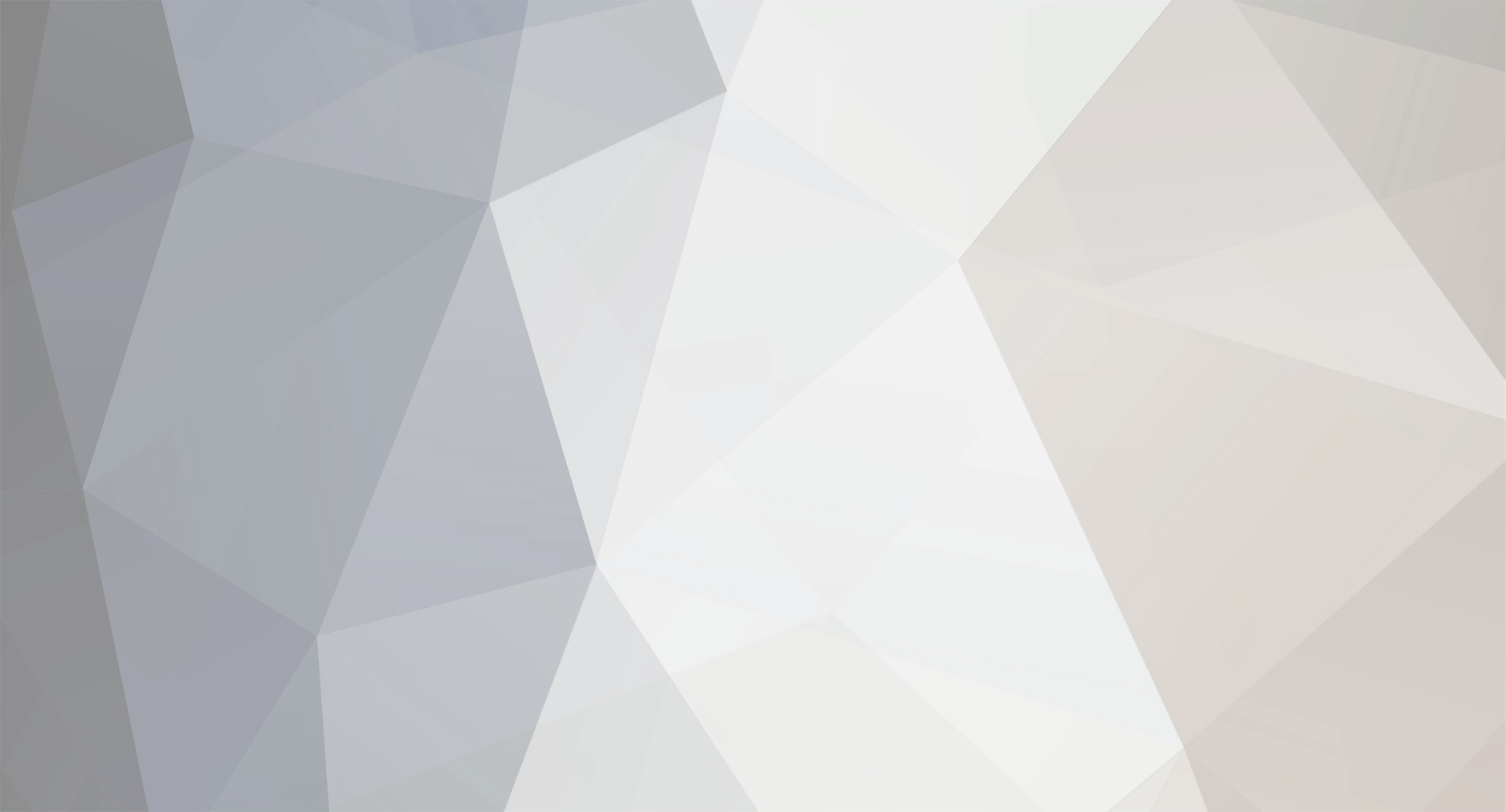
TheGreyGhost
Members-
Posts
3280 -
Joined
-
Last visited
-
Days Won
8
Everything posted by TheGreyGhost
-
(unsolved)(1.7.2) Manually Creating Mob Models
TheGreyGhost replied to Sanocon's topic in Modder Support
Hi I'd suggest you use Techne to make something simple, then look at the code and customise it to your needs. I reckon that will answer all your questions. Collision with the ground is not at pixel level, it is done by putting a rectangular bounding box around the entire mob. (AxisAlignedBoundingBox). -TGG -
Hi if (helmet.getItem() == FireArmorBaseFile.FireHelmet) { player.addPotionEffect((new PotionEffect(Potion.nightVision.getId(), 400, 0))); } So you say that this line is being executed if (helmet.getItem() == FireArmorBaseFile.FireHelmet but it is never true, even when you think the helmet is being worn Depending on how you've coded FireHelmet, this might work instead if (helmet.getItem().itemID == FireArmorBaseFile.FireHelmet.itemID) BTW that should probably be FireArmorBaseFile.fireHelmet ? If that doesn't work, show us your FireArmorBaseFile.java? -TGG
-
Hi The vanilla FurnaceRecipes is a pretty straightforward example of how you might do it. You would just need to modify the data structures and methods to have two inputs instead of just one. -TGG
-
How much java should i know before starting serious modding
TheGreyGhost replied to ClarmonkGaming's topic in Modder Support
Hi I think a good general rule is that when you don't know enough Java to understand how the vanilla code for some feature works after studying it for a while, then you probably need more practice before you implement something similar. If I were going to recommend a checklist I'd say you need to be comfortable with the following concepts to do anything "serious" in Minecraft - know the basic syntax (keywords like for, switch, if, while, do, final, static, public etc) - understand the different types of variables (int, byte, float, etc) - understand what inheritance is and how it works (extends), instanceof and casting (MyBaseObject)derivedObject; - have a good working knowledge of the container classes (ArrayLists, HashMaps, etc) - understand the difference between MyObject a = b; and MyObject a = b.clone(); or MyObject a = new Object(b); - know the difference between a == b and a.equals(b) Knowing a bit about OpenGL would help too http://www.glprogramming.com/red/index.html - know how to use your integrated debugger properly (breakpoints, watches, etc) Once you're comfortable with that lot, then you've got a decent chance of understanding the vanilla code and learning how to create your own stuff. Reading up on good coding style and code design would be a bit help (eg "Effective Java" textbook) My only other advice would be to create small pieces and test them one by one. So if you're making a mod with fifteen types of new weapons that fire everything from bullets to small nukes, start with just a bullet- (1) copy a class from vanilla (say - snowball) into your own classes and see if you can spawn it. (2) Once that works, test whether you can spawn it and it flies through the air straight until it hits something. (3) Once that works, add a damage effect for when it hits a mob. (4) etc etc There's nothing worse than coding up a storm for 3 days and then when you try to test it nothing works, it takes hours to find any bug because you don't know which parts of the code are reliable and which aren't, every time you try to fix something it breaks something else, you realise you made a couple of incorrect assumptions so half of the code you wrote is useless and has to be scrapped, etc etc Apart from anything else, coding & testing in small chunks is much more rewarding because you get a better sense of progress and it's good to change between coding & debugging fairly often, keeps you from getting bored. -TGG -
Hi Well that depends on how flexible you want to be. If you've only got a few recipes you can just hard code it. Something like /** * Used to get the resulting ItemStack from two source ItemStacks */ public ItemStack getSmeltingResult(ItemStack item1, ItemStack item2) { if (item1 == null || item2 == null) { return null; } if ( (item1.isItemStackEqual(myRecipeInput1) && item2.isItemStackEqual(myRecipeInput2)) || (item1.isItemStackEqual(myRecipeInput2) && item2.isItemStackEqual(myRecipeInput1)) ) { return myRecipeOutput.copy(); } else { return null; } } Is that what you meant? -TGG
-
Hi The problem is caused by this line on the server FreshPower.getBlock("fp_steelifierActive") For some reason your getBlock is returning null. -TGG
-
Hi I'm not sure I understand your question? In your addSmelting function you just need to check the values of input1 and input2, and if they match your recipe, return the appropriate ItemStack -TGG
-
Hi I reckon I remember somebody asking a question in this forum about exactly the same thing a few months ago. Ah yeah... http://www.minecraftforge.net/forum/index.php/topic,8218.0.html Might be of some help to you.... -TGG
-
Hi I think it might be a bug, try raising it in the support forum. It the meantime you might be able to work around it by calling Minecraft.getMinecraft().gameSettings.loadOptions(); after your ClientRegistry.registerKeyBinding(...) I haven't tried it, but it looks like it might work. -TGG
-
Are you sure you have set KeyBinding.getKeyDescription properly? It might help debugging to put a breakpoint in GameSettings::saveOptions and ::loadOptions for (j = 0; j < i; ++j) { KeyBinding keybinding = akeybinding[j]; printwriter.println("key_" + keybinding.getKeyDescription() + ":" + keybinding.getKeyCode()); } Then you should be able to see whether it is being saved and loaded (and if not, why not) -TGG
-
Hi I would suggest you take a step back and first of all try to implement your dynamic lighting just on the client. (And actually I think there's a good chance you don't need any custom packets at all, because the vanilla automatically synchronises the position and look of other players as well as the item they're holding. If you want items eg in the hotbar to also be effective, you can just send a packet every time the player equips/unequips, you don't need to send a packet every time they move as well) i.e. In your client tickhandler, just read the player position and update the dynamic lighting. Once you've got that working you can progress to the more complicated stuff. -TGG
-
Hi EntityLivingBase.getPosition(partialTick) can be useful to get the position during rendering. I don't really understand the symptoms of your problem, but I think a couple of breakpoints in your debugger should be able to solve it very quickly. When you say "it is random", is that really true? I.e. if you stop moving, does it flick around between -1 and +1? If you move a small amount, does it jump to +1, move a bit more and jump to +0, move a bit more and jump to -1, or similar? I gather that you want an integer ("block") position instead of the player's double position? if so, beware that casting to int discards the fraction, so negative numbers will go up but positive numbers will go down, which I don't think is what you want. You need Math.floor(). -TGG
-
Hi A little bit more info here http://greyminecraftcoder.blogspot.com/2013/11/how-forge-starts-up-your-code.html Like DieSieben7 says, the @EventHandler in @Mod.java has a couple of lines of info on each one. -TGG
-
[SOLVED][1.7.2] Rendering icon in inventory turns completely grey
TheGreyGhost replied to coolAlias's topic in Modder Support
nice work! -
Hi If I understand your code right, this fragment here for(int a = 0; a < 6; ++a){ for(int b = 0; b < 6; ++b){ for(int c = 0; c < 6; ++c){ for(int d = 0; d < 6; ++d){ for(int e = 0; e < 6; ++e){ for(int f = 0; f < 6; ++f){ will iterate a total of 6*6*6*6*6*6 = 46656 times and you are adding a whole heap of recipes on every single iteration You could perhaps change the code to just create each recipe once instead of thousands of times, but I think GotoLink's suggestion is a much better idea. -TGG
-
[SOLVED][1.7.2] Rendering icon in inventory turns completely grey
TheGreyGhost replied to coolAlias's topic in Modder Support
Hi Man I hate this type of bug. This class might perhaps be of some help https://github.com/TheGreyGhost/SpeedyTools/blob/master/src/speedytools/clientonly/OpenGLdebugging.java It's something I put together to help figure out which OpenGL settings vanilla has modified before calling my rendering code. If you run it for the "good" vs "bad" renders you might be able to spot the difference. dumpAllIsEnabled() currently works. The rest isn't fully functional yet but I reckon you've got the skills to tweak it so it gives you useful information :-) Was based on this very useful link http://www.glprogramming.com/red/index.html -TGG -
Hi Just a general suggestion, I find with new code that it's very helpful to try it in small pieces and get it working by itself before I try to integrate it into a bigger project. I keep a separate project called "TestFramework" full of simple classes to test out my new classes and make sure I understand how they work - Item Renderers, Tick Handlers, Tile Entities, etc. Otherwise it just gets too hard to figure out where the bugs are. -TGG
-
[SOLVED][1.7.2] Rendering icon in inventory turns completely grey
TheGreyGhost replied to coolAlias's topic in Modder Support
Hi I reckon this is almost certainly a problem with OpenGL or the Tessellator settings carrying over from previous renders - but I'm not sure what. Does the grey background still appear even if you do nothing in your render? If not, perhaps your texture hasn't been bound properly. You might try rendering a vanilla icon on the slot (by calling the vanilla code) and then rendering your icon over the top of the vanilla icon. If that fixes it, then it shouldn't be too hard to track down the setting that's the problem. Strange huh -TGG -
Hi This link might be of interest to you http://greyminecraftcoder.blogspot.com.au/2013/08/lighting.html -TGG
-
There are four different buses, two of main interest: Forge events goes on the MinecraftForge.EVENT_BUS FML events go on the FMLCommonHandler bus If you register your event on the wrong bus it won't be called. Easiest way: look to see which package it is in. TickEvent is in package cpw.mods.fml, so use FMLCommonHandler and eg ClientChatReceivedEvent is in net.minecraftforge, so use MinecraftForge -TGG
-
http://www.minecraftforge.net/forum/index.php/topic,16506.msg83670.html#msg83670 should get you started
-
Event registration checks for correct bus
TheGreyGhost replied to TheGreyGhost's topic in Suggestions
Perhaps something like public class MinecraftForge { /** * The core Forge EventBusses, all events for Forge will be fired on these, * you should use this to register all your listeners. * This replaces every register*Handler() function in the old version of Forge. * TERRAIN_GEN_BUS for terrain gen events * ORE_GEN_BUS for ore gen events * EVENT_BUS for everything else */ public static final EventBus EVENT_BUS = new EventBus("EVENT_BUS"); public static final EventBus TERRAIN_GEN_BUS = new EventBus("TERRAIN_GEN_BUS"); public static final EventBus ORE_GEN_BUS = new EventBus("ORE_GEN_BUS"); and public class EventBus { private static int maxID = 0; private ConcurrentHashMap<Object, ArrayList<IEventListener>> listeners = new ConcurrentHashMap<Object, ArrayList<IEventListener>>(); private final int busID = maxID++; private String busName; public EventBus(String init_busName) { busName = init_busName; ListenerList.resize(busID + 1); } // ... private void register(Class<?> eventType, Object target, Method method) { try { Constructor<?> ctr = eventType.getConstructor(); ctr.setAccessible(true); Event event = (Event)ctr.newInstance(); if (! event.whichBus().equals(busName)) { throw new IllegalArgumentException( "Method " + method + " has @ForgeSubscribe annotation, but is being registered on the wrong bus " + "(" + busName + " instead of " + event.whichBus() + ")" ); } // ... etc ... } catch (Exception e) { e.printStackTrace(); } } and public class Event { //... private static ListenerList listeners = new ListenerList(); public String whichBus() {return "";}; // ... } public class BlockEvent extends Event { //... @Override public String whichBus() {return "EVENT_BUS";}; //... } -
Hi Perhaps it would be helpful to new modders if the Event handler registration would check that you have registered your event handler to the correct bus. It is rather easy to get them confused (or be unaware that eg the FML has a different bus) and all you can tell is that your event is not called. -TGG
-
Hi I was curious, so I tried it. It works fine for me with the following code (dingdingdingdingdign..): MyEventHandler:: package com.example.examplemod; public class MyEventHandler { @SubscribeEvent public void onTick(TickEvent.ClientTickEvent event){ System.out.print("ding"); } } ExampleMod:: package com.example.examplemod; import cpw.mods.fml.common.FMLCommonHandler; import net.minecraft.init.Blocks; import cpw.mods.fml.common.Mod; import cpw.mods.fml.common.Mod.EventHandler; import cpw.mods.fml.common.event.FMLInitializationEvent; import net.minecraftforge.common.MinecraftForge; @Mod(modid = ExampleMod.MODID, version = ExampleMod.VERSION) public class ExampleMod { public static final String MODID = "examplemod"; public static final String VERSION = "1.0"; @EventHandler public void init(FMLInitializationEvent event) { // some example code System.out.println("DIRT BLOCK >> "+Blocks.dirt.getUnlocalizedName()); FMLCommonHandler.instance().bus().register(new MyEventHandler()); } } -TGG
-
[1.6.4] Get the Resource/Resource location of any item.
TheGreyGhost replied to lexwebb's topic in Modder Support
Hi There is some discussion around textures and paths in here. It was fairly complicated to figure out. You might be able to find a way for your code to call makeFullPath and get the full path. http://www.minecraftforge.net/forum/index.php?topic=11963.0 -TGG