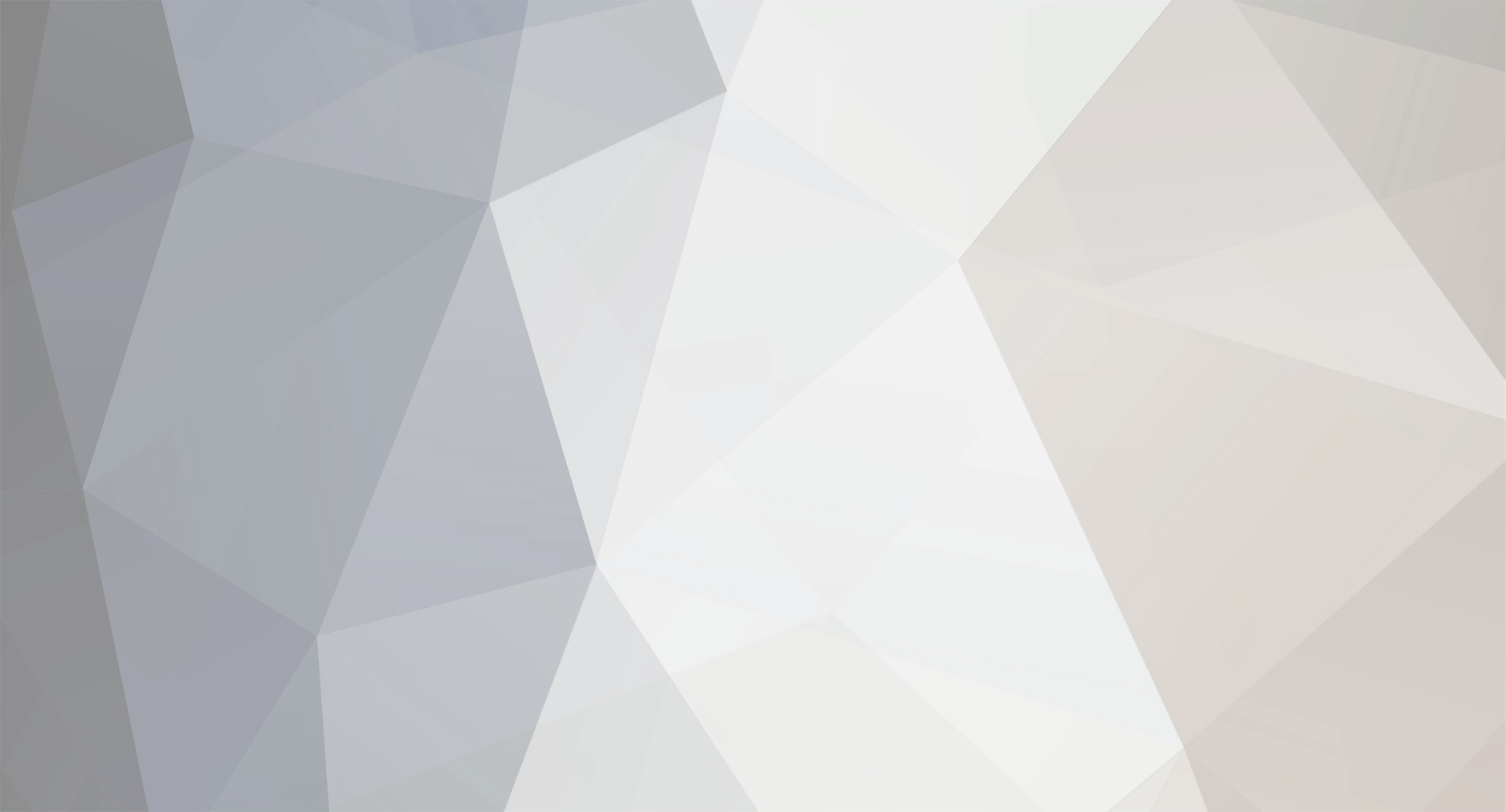
TheGreyGhost
Members-
Posts
3280 -
Joined
-
Last visited
-
Days Won
8
Everything posted by TheGreyGhost
-
Hi There's a working example of TileEntities in this project, it might give you some clues. https://github.com/TheGreyGhost/MinecraftByExample (see mbe20, mbe21) Cheers TGG PS @Deprecated //Forge: Do not use, use BlockState.hasTileEntity/Block.createTileEntity instead public interface ITileEntityProvider { @Nullable TileEntity createNewTileEntity(IBlockReader worldIn); }
-
1.15.2 entity body parts are in wrong places
TheGreyGhost replied to treblotmail's topic in Modder Support
Hi You can probably manually fix your code using these as a guide: http://greyminecraftcoder.blogspot.com/2020/03/minecraft-model-basics-rotation-1144.html https://github.com/TheGreyGhost/MinecraftByExample (mbe80 for example of model adjustable in real time) -TGG PS cute panda by the way...! -
Register custom particle in the vanilla ParticleManager
TheGreyGhost replied to Shelk's topic in Modder Support
Hi This example project has a working example of custom particles. https://github.com/TheGreyGhost/MinecraftByExample (see mbe50) -TGG -
IInventory vs ItemStackHandler for Containers with GUI
TheGreyGhost posted a topic in Modder Support
Howdy Folks. I'm creating a container with GUI similar to vanilla chest. Previously I've used IInventory to do that but I hear phrases like "that's deprecated" and "use IItemHandler instead". ItemStackHandler I understand fine, but it doesn't seem to be directly compatible with GUI. What's the "Forge way" to code a chest similar to Vanilla? i.e. currently I'm doing composition to adapt the ItemStackHandler to an IInventory, sometimes in a pretty clumsy way public class TileEntityInventoryBasic extends TileEntity implements IInventory { // Create and initialize the item variable that will store store the item private final int NUMBER_OF_SLOTS = 9; private final ItemStackHandler itemStackHandler = new ItemStackHandler(NUMBER_OF_SLOTS); public TileEntityInventoryBasic() { super(StartupCommon.tileEntityInventoryBasic); clear(); } /* The following are some IInventory methods you are required to override */ // Gets the number of slots in the inventory @Override public int getSizeInventory() { return itemStackHandler.getSlots(); } // returns true if all of the slots in the inventory are empty @Override public boolean isEmpty() { for (int i = 0; i < itemStackHandler.getSlots(); ++i) { if (!itemStackHandler.getStackInSlot(i).isEmpty()) { // isEmpty() return false; } } return true; } // Gets the stack in the given slot @Override public ItemStack getStackInSlot(int slotIndex) { return itemStackHandler.getStackInSlot(slotIndex); } /** * Removes some of the units from itemstack in the given slot, and returns as a separate itemstack * @param slotIndex the slot number to remove the item from * @param count the number of units to remove * @return a new itemstack containing the units removed from the slot */ @Override public ItemStack decrStackSize(int slotIndex, int count) { ItemStack extractedItemStack = itemStackHandler.extractItem(slotIndex, count, false); if (!extractedItemStack.isEmpty()) { markDirty(); } } (etc) and the reason I think I need to do this is because Slot needs an IInventory public class ContainerBasic extends Container { // Stores a reference to the tile entity instance for later use private TileEntityInventoryBasic tileEntityInventoryBasic; for (int x = 0; x < TE_INVENTORY_SLOT_COUNT; x++) { int slotNumber = x; addSlot(new Slot(tileEntityInventoryBasic, slotNumber, TILE_INVENTORY_XPOS + SLOT_X_SPACING * x, TILE_INVENTORY_YPOS)); /// slot requires an IInventory. } } Am I missing something? -TGG -
[1.14.4] Using JSON file for configuration
TheGreyGhost replied to superhize's topic in Modder Support
Hi I did something similar with another mod I was working on, you might find some inspiration in there. https://github.com/TheGreyGhost/dragonmounts2-1.12.2/tree/configurable-checkpoint2 See the classes: DragonVariantTag DragonVariants DragonVariantsReader Typical usage is a config file like : dragon1.json { "breedinternalname": "fire", "lifestage": { "size5adult": 5, // size of the adult in metres "healthbase": 50.0, // base health of the adult "armorbase": 10.0 // base armour of the adult }, "egg": { "size": 1.0, //the size (width and height) of the egg in metres "particlesname": "townaura" //what particle effect does the egg produce while incubating? as per the /particle command } } with code that looks like this: private static final DragonVariantTag EGG_SIZE_METRES = DragonVariantTag.addTag("size", 0.5, 0.05, 2.0, "the size (width and height) of the egg in metres").categories(Category.EGG); private static final DragonVariantTag EGG_INCUBATION_DAYS = DragonVariantTag.addTag("incubationduration", 1.0, 0.1, 10.0, "the number of days that the egg will incubate until it hatches").categories(Category.EGG); private static final DragonVariantTag EGG_WIGGLE = DragonVariantTag.addTag("wiggle", true, "should the egg wiggle when it gets near to hatching?").categories(Category.EGG); private static final DragonVariantTag EGG_GLOW = DragonVariantTag.addTag("glow", false, "should the egg glow when it gets near to hatching?").categories(Category.EGG); and final String TEST_FOLDER = "testdata/testdvr1"; DragonVariantsReader dragonVariantsReader = new DragonVariantsReader( Minecraft.getMinecraft().getResourceManager(), TEST_FOLDER); Map<String, DragonVariants> allVariants = dragonVariantsReader.readAllVariants(); DragonVariants dragonVariantsFire = allVariants.get("fire"); double eggSizeMetres = (double)dragonVariantsFire.getValueOrDefault(modifiedCategory, EGG_SIZE_METRES); I don't have a standalone test harness at the moment unfortunately, but the classes are reasonably self-contained and (hopefully) fairly intuitive to figure out. The code also includes functionality for "modifiers" and "ariantTagValidator" to check for config tag problems and/or initialise resources based on the tags, but those are optional. Cheers TGG- 1 reply
-
- 1
-
-
[1.14.4] Syncing rendered held item movement with head?
TheGreyGhost replied to JayZX535's topic in Modder Support
Hi Jay You might find this link useful, although it sounds like you've got a good grasp of the concepts already http://greyminecraftcoder.blogspot.com/2020/03/minecraft-model-basics-rotation-1144.html Something I've found extremely useful while debugging model rotations, translations, and scales is to add in-game tweakable parameters, i.e. typing a command /mbedebug setheadrotation 2 53 6 and seeing what effect it has on the model without having to recompile. See mbe80 in here for an example https://github.com/TheGreyGhost/MinecraftByExample There are lots of traps when rotating and translating and I can never figure it out on paper, I find it easier to get 80% of the way there based on the theory and then get the rest of the way by trial and error. Things that have tripped me up: * order of transformation (translation, rotation) is extremely important and (at least for me) not intuitive. Even when I try to follow the theoretical rules I still usually stuff it up * rotation around an arbitrary point (as you already said) is translate, rotate, translate, but not necessarily in the order/direction you expect * model space vs world space -> your rotations and translations may be around a different axis to what you expect, or around a different origin, and translation may be in the opposite or even in an oblique direction Generally the answer has been: keep model-space and world space transformations separate if vanilla forces you to apply one at a time that you don't want to, you need to transform back from world to model, do your desired transformation, then transform from model back to world again. take baby steps use interactive in-game parameter tweaking of some kind (eg the mbe80 I mentioned above) -TGG -
[1.15.2] Why are there two seperate event busses?
TheGreyGhost replied to Budschie's topic in Modder Support
Hi In addition to what Draco said: I think the difference arises when more than one mod is installed. Each mod gets its own MOD bus to deal with its own lifecycle events during startup+initialisation All mods share the same FORGE bus for gameplay events I guess the reason is to better control the order that the events are called in for different mods. No idea why that would be important. -TGG -
Assigning custom model location to item and block in 1.15
TheGreyGhost replied to AntonBespoiasov's topic in Modder Support
Hi You could just make a simple json for each item registry; eg { "parent": "block/bedrock" } Or is there a particular reason you don't want a .json for each item? -TGG -
Hi I always find the order of translation-scaling-rotation-etc operations to be confusing so this might be off base, but I've usually found the trick to be either 1) put the operation in a different order (eg rotate then translate instead of translate then rotate); or 2) undo the transformation from model space to world space, perform the operation, then reapply the original transformation Not sure if that's possible (or desirable) inside your new rendertype, but it might be worth a try. -TGG
-
Custom OBJ, vanilla texture - loads, but is not scaled correctly
TheGreyGhost replied to briclabs's topic in Modder Support
Hi Ah yeah that makes sense. BTW these is a flag available in the loader to flip the V texture coordinate https://github.com/TheGreyGhost/MinecraftByExample/blob/master/src/main/resources/assets/minecraftbyexample/models/block/mbe21_ter_wavefront_model.json You can also override the path to the mtl file, so at least you would only need one obj file. There is a more-compact way to use the same obj with different texture each time, but you will need to write your own OBJmodel loader by customising the Forge one to add a couple of custom flags for your own use, or to automatically choose a texture based on the block that's being loaded. The Forge code (OBJloader and OBJmodel) is not too difficult to understand once you know what it's doing. -TGG -
[1.15.2] What render event should I use?
TheGreyGhost replied to Fabillotic's topic in Modder Support
Ah, ok. In that case RenderWorldLastEvent probably is the best one - I've never seen that disabling clouds problem with it. Might be worth tracing into updateCameraAndRender() to see why. It might be a forge bug perhaps... Or perhaps your drawing code is wrong (relying on the renderer to be in a particular state, and this state changes if you turn the clouds off). I think in any case you should convert your direct calls into the proper RenderType and IVertexBuffer calls. -TGG PS That mod is dead and buried a long time ago now Too many projects, too little time... -
Custom OBJ, vanilla texture - loads, but is not scaled correctly
TheGreyGhost replied to briclabs's topic in Modder Support
Hi That is a strange one. I've just implemented obj model in this example project (for a TER, not a block admittedly), you might have a look for clues in there (https://github.com/TheGreyGhost/MinecraftByExample see RenderWavefrontObj) My guess: Forge is assuming that your texture is for a single face but your texture sheet is actually for all six faces, i.e. it is using u,v = 0,0 to 0.25, 0.25 or similar. Might be a forge bug, or might be something you've configured incorrectly I'd suggest either trial and error to get the texture right, or digging into the model baking process (set a breakpoint inside OBJloader and/or OBJmodel to see what happens to your vertices and textures after your model loads). It may be a bit tricky because minecraft stitches block textures together into a single texture sheet, so the u,v are harder to interpret. -TGG -
[1.14.4] Z fighting issues with semi-transparent entity layers
TheGreyGhost replied to JayZX535's topic in Modder Support
Keen, thanks dude I'll grab it and probably work it into my tutorial project in the next month or so with any luck. And will give credit to you of course -
Can somone help me update a 1.7.10 mod to 1.12?
TheGreyGhost replied to Myrosb's topic in Modder Support
Hi Looks like someone else might already have done it https://www.curseforge.com/minecraft/mc-mods/mario-mod-2 Just so you know - what you're asking for is literally months of work... -TGG -
Urgent: Desperately Need Help With Update
TheGreyGhost replied to insane_gravy's topic in Modder Support
In that case I hope you cope with frustration well Might be better to say "sorry bud, too hard" and abort the mission now, unless you're really really invested in resurrecting a zombie... that "one weekend code update" will be six months before you know it... -
Replacement of the deprecated GlStateManager.color4f [1.15.2]
TheGreyGhost replied to Budschie's topic in Modder Support
Hi Rendering is very different now. Check out this link: https://gist.github.com/williewillus/30d7e3f775fe93c503bddf054ef3f93e -TGG -
assets Not Working but it's in the right section
TheGreyGhost replied to TheThorneCorporation's topic in Modder Support
Hi Look at mbe10 in this example project, this is the resource structure you need to follow. https://github.com/TheGreyGhost/MinecraftByExample/tree/master At Ugdhar said, often the console log will give you an good clue (typically "file not found: xxxx" which tells you exactly where forge expected to find your file -TGG -
Urgent: Desperately Need Help With Update
TheGreyGhost replied to insane_gravy's topic in Modder Support
Hi Updating from 1.5 to 1.12 can be done and it's not as hard as you might think because there was a lot less functionality back then. But most of the code will need to be refactored by hand, search and replace is not going to work for you. You're also going to have to relearn a bunch of stuff because as the other folks said, many parts of the vanilla code and forge have completely changed the way they do things. If the mod was simple (new block types, item types) then it won't be too difficult. If it did complicated new functionality or buried deep into vanilla it's going to be a real chore. At least you will be able to re-use the artwork! This example project might help get you started (available for 1.12.2) https://github.com/TheGreyGhost/MinecraftByExample/tree/1-12-2-final Unfortunately 1.12 is "no longer supported on this forum" so most folks here will refuse to help you, you will need to get advice from other forums or direct messaging. -TGG -
[1.15.2] What render event should I use?
TheGreyGhost replied to Fabillotic's topic in Modder Support
Hi In that case the DrawHighlightEvent is definitely the one you want. Funnily enough one of my first mods was the same thing, great minds think alike obviously Abandoned it in 1.8 because it was too much work to keep up to date... -TGG -
[1.15.2][Solved] World#getLightValue always return 0
TheGreyGhost replied to elias54's topic in Modder Support
Hi I would suggest you look at the DaylightDetectorBlock for inspiration. getLightFor seems to be the key. -TGG -
Hi You might find this working example useful; see mbe01 https://github.com/TheGreyGhost/MinecraftByExample -TGG
-
[1.15.2] What render event should I use?
TheGreyGhost replied to Fabillotic's topic in Modder Support
Hi I've got an example mod that has two examples of rendering 3D lines https://github.com/TheGreyGhost/MinecraftByExample see mbe21-RenderLines and DebugBlockVoxelShapeHighlighter There is some further advice in this link about rendering and using RenderWorldLastEvent: https://gist.github.com/williewillus/353c872bcf1a6ace9921189f6100d09a The appropriate event for rendering depends on when you want to render them; is it related to a block or item, or related to the player, or does it occur all the time? Perhaps describe for us in more detail what the gameplay effect you're trying to achieve is? -TGG -
Hi You'll probably find this link useful https://gist.github.com/williewillus/353c872bcf1a6ace9921189f6100d09a This example project might help if you decide to go down the path of sending a custom packet to spawn particles (mbe60 uses this with the TargetEffectMessageToClient example, i.e. the server sends a network message to the client to say "spawn some particles at this [x,y,z] location"). https://github.com/TheGreyGhost/MinecraftByExample -TGG
-
Hi I think it's probably a client vs server thing. The method you're calling does nothing on the client, and I'm guessing that ItemTossEvent is only ever called on the Server. You could try checking that the world is a ServerWorld, and if it is then casting it to ServerWorld and calling spawnParticle(). That sends a packet to the client to spawn the particle you want. -TGG