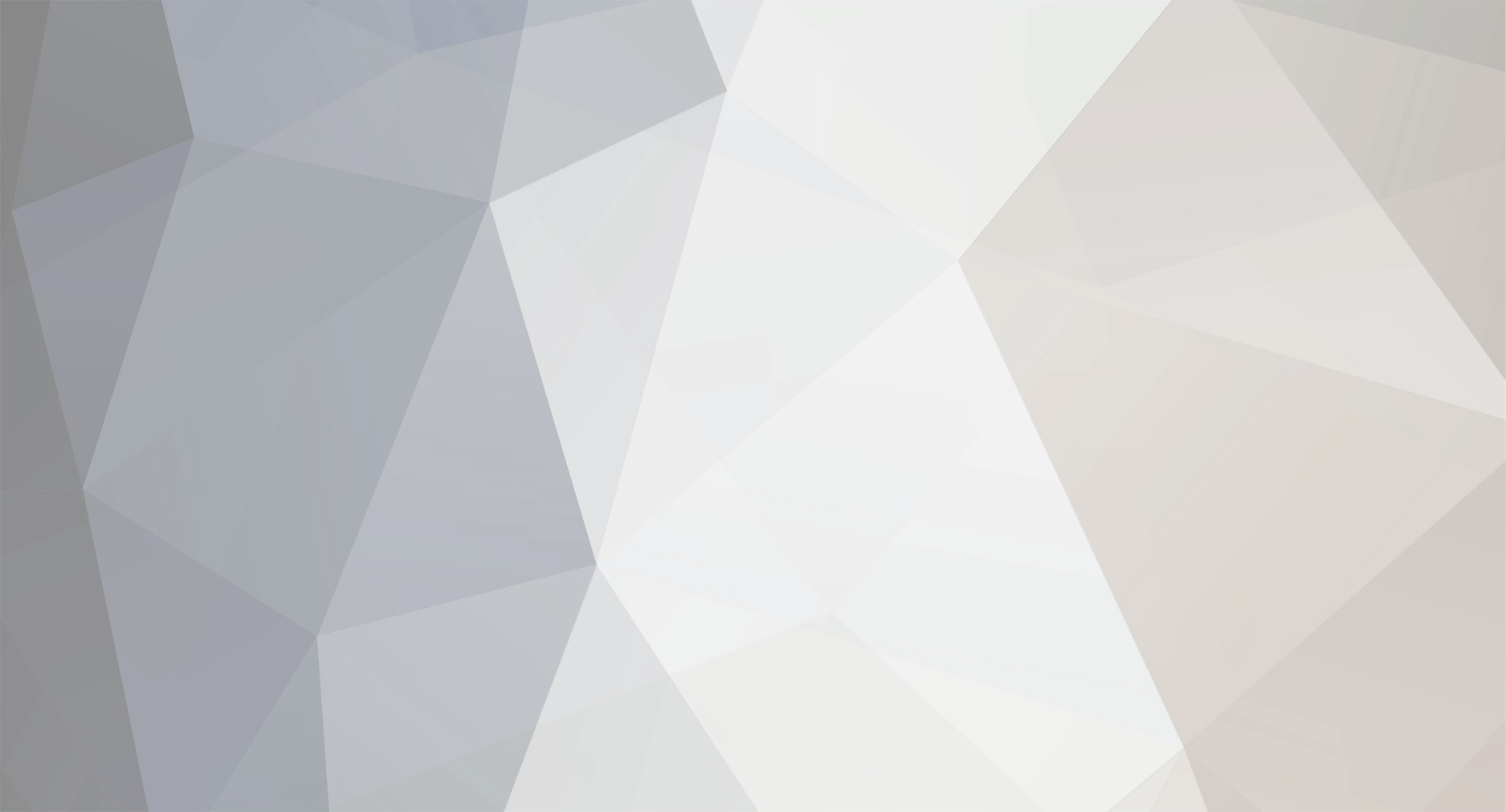
TheGreyGhost
Members-
Posts
3280 -
Joined
-
Last visited
-
Days Won
8
Everything posted by TheGreyGhost
-
Hi It's for sure possible but you will probably need to dig deep into the code and pull a number of not-very-robust tricks. Unless you're an advanced modder I wouldn't recommend it unless you're up for a frustrating challenge. -TGG
-
In that case, it might mean that the texture file wasn't found; due to a bug in Forge it fails silently when that happens. Where is your item texture stored? should be in assets/bmm/textures/item/iron_rivet_item -TGG
-
Hi The DrawHighlightEvent might help you, if you only want to do this on the client side. It is the event called when Minecraft draws the box around the block or entity you're currently looking at. If you need it on the server side, you could perhaps perform a raytrace yourself on the server, say in a PlayerTick event. I'm not 100% sure that will work because I'm not certain that the server knows which way the player is facing. If not, and your server needs to know, you could use the DrawHighlightEvent and send a custom packet to the Server every time a new block is highlighted. Easier: if you don't care whether the player is looking at the block, just whether the player is near to the block, then you could use the PlayerTick event and check within a radius of the player to see if there are any of your block nearby. -TGG
-
Hi THis page has a very detailed description https://gist.github.com/williewillus/353c872bcf1a6ace9921189f6100d09a -TGG
-
[1.14.4] Z fighting issues with semi-transparent entity layers
TheGreyGhost replied to JayZX535's topic in Modder Support
Hi It would help to have a self-contained project which I can compile and run eg if you have a test harness for the class. Helps me understand it better. -TGG -
Hmm ok I suggest you put a breakpoint at that line and see which ResourceLocation exactly is causing the problem -TGG
-
Hi public <E extends Entity> boolean shouldRender(E entityIn, ClippingHelperImpl frustumIn, double camX, double camY, double camZ) { EntityRenderer<? super E> entityrenderer = this.getRenderer(entityIn); <--- crashes here? return entityrenderer.shouldRender(entityIn, frustumIn, camX, camY, camZ); <-- crashes here? } I don't see an obvious cause of a crash at that line but from the context I think it's almost 100% certain you have registered a null EntityRenderer for that Entity You could put a breakpoint at that line and see what happens directly, but I suspect your entity renderer registration is screwed up, possibly your object instances are being created in the wrong order. -TGG
-
Hi Would you please also paste the console error log; it probably has something saying "missing texture" or "missing model". -TGG
-
Howdy This part here at java.util.Objects.requireNonNull(Objects.java:203) at java.util.Optional.<init>(Optional.java:96) at java.util.Optional.of(Optional.java:108) at net.minecraft.client.renderer.RenderState$TextureState.<init>(SourceFile:192) tells me that you are trying to initialise your Renderer with a texture ResourceLocation that is null, i.e. hasn't been set properly. Looking at your code, I suspect this: p_209263_0_.put(SkullBlock.Types.PLAYER, DefaultPlayerSkin.getDefaultSkinLegacy()); I would suggest you put a breakpoint in a suitable location and have a look at what the ResourceLocation has been set to. eg in the put statement above, or in SkullEntityTileRenderer::func_228879_a_ Cheers TGG
-
[1.15.2] Join the minecraft thread from another thread
TheGreyGhost replied to uiytt's topic in Modder Support
Hi You could consider using the packet receiving system to do that. Any time a packet is received, it is in a network thread. The network thread creates a Runnable lambda which is then executed in either the client thread or the server thread. Some more info here: http://greyminecraftcoder.blogspot.com/2020/03/thread-safety-with-network-messages-1144.html There's probably a way for your second thread to add your own Runnable lambda to those queues without using packets. Alternatively, you could perhaps subscribe to a Tick event on the server or the client, and every tick check your second thread to see whether it has finished executing or not. -TGG -
[1.14.4] Z fighting issues with semi-transparent entity layers
TheGreyGhost replied to JayZX535's topic in Modder Support
Awesome When you've got it working, would you mind posting it on GitHub? I would like to use some parts of your code to add to an example/tutorial project I'm working on, if that's ok? Cheers TGG -
Hi I'd advise you swap to the latest forge version (not recommended), because the mcp mappings are much better. It sounds like you're doing things the right way. You might find this example project useful (see MBE80 and MBE21), it has some working examples of models (rendered using a TER not an entity but the ModelRenderer is the same) https://github.com/TheGreyGhost/MinecraftByExample Don't use the cubeList.add(). addBox() should work fine instead. -TGG
-
How to get current world so i can use .setBlockState() [1.15.2]
TheGreyGhost replied to Spirfire972's topic in Modder Support
Hi That's way too early to be writing to the world, it won't have been loaded yet. Also, there is more than one world (eg the nether). When do you want to do this writing of blocks into the world? i.e. at what time, in response to what? Cheers TGG -
[1.14.4] Z fighting issues with semi-transparent entity layers
TheGreyGhost replied to JayZX535's topic in Modder Support
Hi Cool sounds like you've nearly cracked it. I think there are really only a couple of ways your brown dog could turn blue... either he's being rendered with a coloured tint (I see something suspicious in vanilla... TintedAgeableModel which appears to be used for the wolf when it is wet), or your texture image isn't right - perhaps the setRGB isn't working quite right. Lighting can sometimes give things a blue tint but I don't think that's the likely problem in this case. You could test the difference by making your texture deliberately white (or pure red, pure blue, or pure green) and seeing what happens to the rendered colour. You could also set a breakpoint in the WolfRenderer class and trace into the render method to see what it's doing with the quads (i.e. if it's rendering with a tint) -TGG -
How to get current world so i can use .setBlockState() [1.15.2]
TheGreyGhost replied to Spirfire972's topic in Modder Support
Hi Where is this code located? i.e. in which method, or which event? -TGG -
Hi You may need to set a different rendertype eg public class StartupClientOnly { /** * Tell the renderer this is a solid block (default is translucent) * @param event */ @SubscribeEvent public static void onClientSetupEvent(FMLClientSetupEvent event) { RenderTypeLookup.setRenderLayer(yourBlockInstance, RenderType.getTranslucent()); } } where your main mod class has done this: MOD_EVENT_BUS.register(StartupClientOnly.class); although the default is translucent so that seems less likely. Are you sure that your block texture has an alpha channel with the proper values for transparency? -TGG
-
[1.15.2]Using mods in development workspace
TheGreyGhost replied to undertrox's topic in Modder Support
Hi That used to work in older versions but I haven't tried it since 1.12.2. If no-one here has an answer, you might try raising it as an issue on the Forge github. It does sound like a bug. https://github.com/MinecraftForge/MinecraftForge/issues -TGG -
Howdy Please include the crash report? That will provide vital clues... -TGG
-
[1.14.4] Z fighting issues with semi-transparent entity layers
TheGreyGhost replied to JayZX535's topic in Modder Support
Hi If your entity is rendering as transparent, it sounds like there is something wrong with your texture. Perhaps your texture has an alpha channel which you have set to zero? Or perhaps it is not binding properly. I would suggest using a breakpoint to inspect your tinted image after doing graphics.drawImage(). It contains an array of int which you can inspect directly, that will show you which part of your code is causing the problem. I'd also suggest manually generating a test BufferedImage (eg fill it manually with 0xffffffff = opaque white) and seeing whether you get a white wolf when you render with it. I also think that the XOR mode is very unlikely to give you the effect you want; you probably need setComposite() instead. I doubt that the OpenGL method would be much more efficient than your Graphics code approach, and it's a lot more complicated. Maybe leave that as a last resort... -TGG -
[1.14.4] Z fighting issues with semi-transparent entity layers
TheGreyGhost replied to JayZX535's topic in Modder Support
Hi I think you could do it both ways; I've previously used OpenGL to render to a bitmap which then gets used as the texture for a second render. I've also manually iterated through the pixels & calculated manually. First way is faster, second is easier to code. Because the texture is so small (64 x 32 pixels = 2048 pixels), it really shouldn't take very long to manually iterate through them and calculate each pixel. Java can do millions or even hundreds of millions of calculations per second. Perhaps there is a logic flaw in your code? Could you paste it so we can have a look? Or a github link? The basic alpha blending equation for an overlay layer is (for each red, blue, green pixel value) pixel value = base * (1-alpha) + overlay * (alpha) That gives you the base layer with the first overlay ("base1"). Then you repeat the calculation again for the second layer with its own alpha pixel value = base1 * (1-alpha2) + overlay2 * (alpha2) etc There are lots of other equations possible... it all depends what effect you're trying to achieve. -TGG -
Hi I guess you're talking about json models for blocks or items? If this is just a once-off thing, I would personally use Excel (with formulas to copy the right words into the right places in the file) and then export as text. I would also get my mod working perfectly with just one item/block first. And then when I had ironed out all the bugs, I would add all the remaining items/blocks. -TGG
-
You can register either the class or an instance, but it affects which methods get checked for events! If you register the class: static methods are checked for events If you register an instance of the class, non-static methods are checked for events. In this case I think you've registered it correctly. I suggest you troubleshoot it by putting a breakpoint in the forge code, at the point that it posts the DifficultyChangeEvent. That will tell you if your registration has failed. (Search project and library for usages of DifficultyChangeEvent) -TGG
-
Hi The reason you should use @Override (it's optional) is so that, when Forge or minecraft updates and the signature for a method changes, your compiler tells you that there is a problem. eg public class ItemVariants extends Item { // what animation to use when the player holds the "use" button @Override public UseAction getUseAction(ItemStack stack) { return UseAction.DRINK; } } When minecraft is updated, and getUseAction(ItemStack stack) changes to getUseActionAnimationType(ItemStack stack), or changes to getUseAction(ItemStack stack, Hand whichHandUsing), then your compiler will give you an error. Otherwise your code will silently stop working because your item will now use the Item base method which is public UseAction getUseAction(ItemStack stack) { return stack.getItem().isFood() ? UseAction.EAT : UseAction.NONE; } This happens a lot and from bitter experience I can tell you that @Override can save you hours of pain and frustration. -TGG
-
[Solved][1.14.4] Get all files in specific folder
TheGreyGhost replied to Croa's topic in Modder Support
Howdy This might be promising? https://stackoverflow.com/questions/17421590/iterate-over-folders-in-jar-file-directly Java also has a stack of Reflection methods that would probably be suitable to iterate through a jar. You might also get some clues from the Forge mod loading code, which (from a very quick look) appears to put a wrapper around a jar so that it appears to be a filesystem see AbstractJarFileLocator -TGG -
[1.15.2] Flag problem with cauldron-like block
TheGreyGhost replied to SW Raki's topic in Modder Support
Howdy The problem is that there is only one instance of your DungInfuserBlock block, not one for each placed block. If you try and store information about each world block in your DungInfuserBlock, it can only store one at a time. (The DungInfuserBlock is a "flyweight" object - google Design Pattern Flyweight for more info). You should use a TileEntity instead, to store information about each placed block. The vanilla CampfireBlock (with CampfireTileEntity) does this, for example. There is also an example of this in the following tutorial project: https://github.com/TheGreyGhost/MinecraftByExample/tree/master see mbe20. -TGG