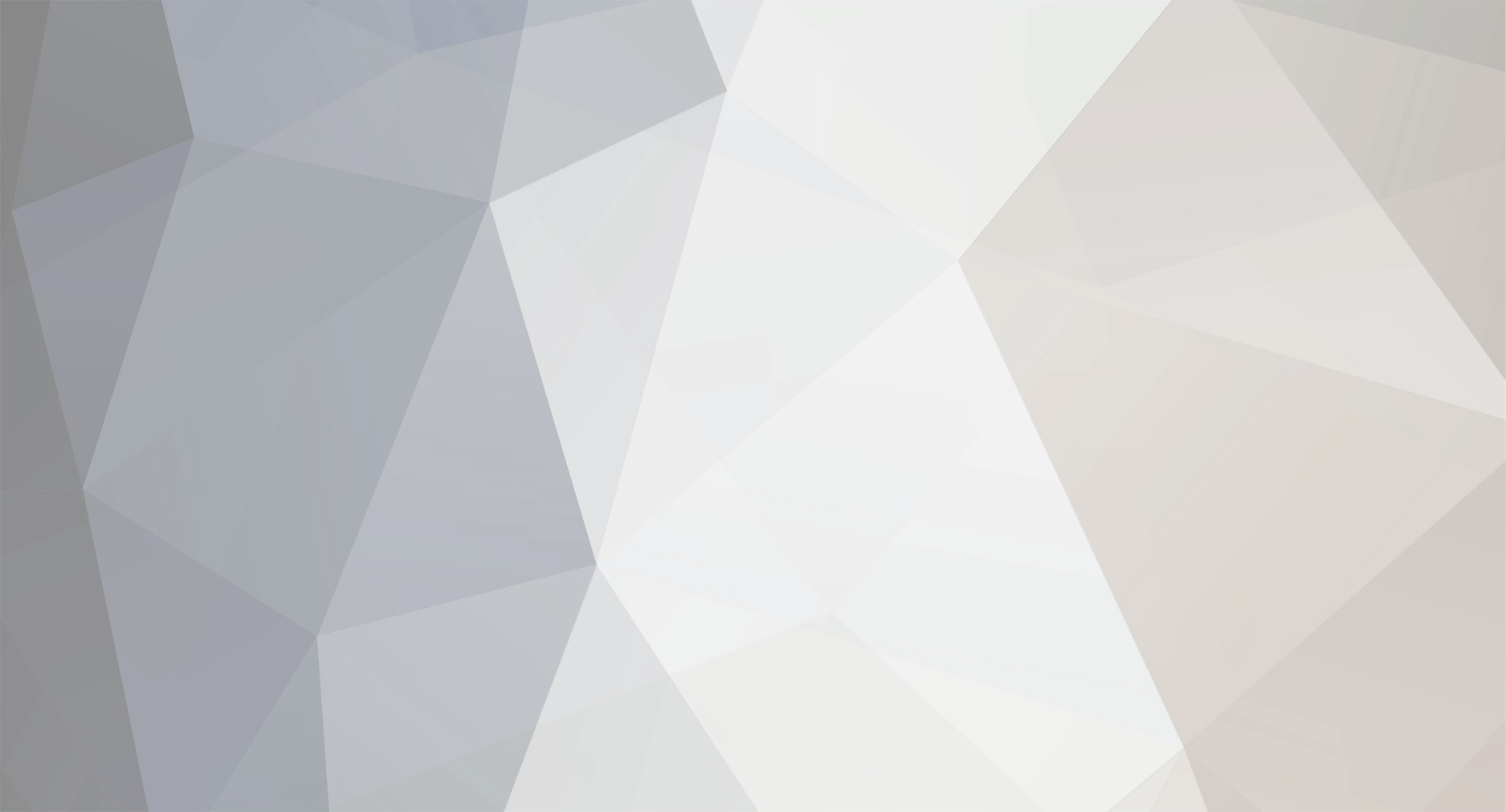
tal124
Members-
Posts
87 -
Joined
-
Last visited
Everything posted by tal124
-
Fluid Blocks/Fluids not placing with my BucketItem
tal124 replied to tal124's topic in Modder Support
I believe my problem is with my registration of the fluids and not with the fluids themselves public class ModFluids extends Fluids{ //Deferred register calls the block public static final DeferredRegister<Fluid> FLUIDS = DeferredRegister.create(ForgeRegistries.FLUIDS, chocolate.MODID); //Attaches the deferred register to the event bus public static void init() { FLUIDS.register(FMLJavaModLoadingContext.get().getModEventBus()); } public static final FlowingFluid FLOWINGCHOCOLATEFLUID = null; public static final FlowingFluid CHOCOLATEFLUID = null; public static final FlowingFluid FLOWINGMILKCHOCOLATEFLUID = null; public static final FlowingFluid MILKCHOCOLATEFLUID = null; I thought doing this might be helpful, and I've looked into the code for Water fluid registration but it uses deprecated code that I'm not sure what would be used in place for forge -
Fluid Blocks/Fluids not placing with my BucketItem
tal124 replied to tal124's topic in Modder Support
Oops I showed the wrong code, I have two fluids public static RegistryObject<BucketItem> CHOCOLATE_BUCKET = ITEMS.register("chocolate_bucket", () -> new BucketItem( () -> ModFluids.chocolatefluid, new Item.Properties().group(ChocolateItemGroup.CHOCOLATE_ITEM_GROUP) .maxStackSize(1).containerItem(BUCKET) ) ); public static RegistryObject<BucketItem> MILK_CHOCOLATE_BUCKET = ITEMS.register("milk_chocolate_bucket", () -> new BucketItem( () -> ModFluids.chocolatefluid, new Item.Properties().group(ChocolateItemGroup.CHOCOLATE_ITEM_GROUP) .maxStackSize(1).containerItem(BUCKET) ) ); And this is the code for my fluid blocks public static final RegistryObject<FlowingFluidBlock> CHOCOLATE_FLUID_BLOCK = BLOCKS.register("chocolate_fluid", () -> new FlowingFluidBlock(() -> ModFluids.chocolatefluid, AbstractBlock.Properties.create(Material.WATER).noDrops().doesNotBlockMovement()) ); public static final RegistryObject<FlowingFluidBlock> MILK_CHOCOLATE_FLUID_BLOCK = BLOCKS.register("milk_chocolate_fluid", () -> new FlowingFluidBlock(() -> ModFluids.milkchocolatefluid, AbstractBlock.Properties.create(Material.WATER).noDrops().doesNotBlockMovement()) ); I'll comment out my current code and give ForgeFlowingFluid a try Edit: The code used to register my fluids public class ModFluids { //Deferred register calls the block public static final DeferredRegister<Fluid> FLUIDS = DeferredRegister.create(ForgeRegistries.FLUIDS, chocolate.MODID); //Attaches the deferred register to the event bus public static void init() { FLUIDS.register(FMLJavaModLoadingContext.get().getModEventBus()); } public static final ChocolateFluid.Source chocolatefluid = null; public static final ChocolateFluid.Flowing flowingchocolatefluid = null; public static final MilkChocolateFluid.Source milkchocolatefluid = null; public static final MilkChocolateFluid.Flowing flowingmilkchocolate = null; } Also, I've tried using forgeflowingfluid and got the same result. I'm genuinely perplexed by this. -
I have been trying to figure out why my fluids won't place down from my bucket items that I have created public abstract class MilkChocolateFluid extends FlowingFluid { @Override public Fluid getFlowingFluid() { return ModFluids.flowingmilkchocolate; } @Override public Fluid getStillFluid() { return ModFluids.milkchocolatefluid; } @Override protected boolean canSourcesMultiply() { return false; } @Override protected void beforeReplacingBlock(IWorld worldIn, BlockPos pos, BlockState state) { } @Override protected int getSlopeFindDistance(IWorldReader worldIn) { return 4; } @Override protected int getLevelDecreasePerBlock(IWorldReader worldIn) { return 3; } @Override public BucketItem getFilledBucket() { return ModItems.MILK_CHOCOLATE_BUCKET.get(); } @Override protected boolean canDisplace(FluidState fluidState, IBlockReader blockReader, BlockPos pos, Fluid fluid, Direction direction) { return direction == Direction.DOWN && !fluid.isIn((ITag<Fluid>) ModFluids.milkchocolatefluid); } @Override public int getTickRate(IWorldReader p_205569_1_) { return 20; } @Override protected float getExplosionResistance() { return 100.0F; } @Override protected BlockState getBlockState(FluidState state) { return ModBlocks.CHOCOLATE_FLUID_BLOCK.get().getDefaultState().with(FlowingFluidBlock.LEVEL, Integer.valueOf(getLevelFromState(state))); } @Override public boolean isEquivalentTo(Fluid fluidIn) { return fluidIn == ModFluids.milkchocolatefluid || fluidIn == ModFluids.flowingmilkchocolate; } public static class Flowing extends ChocolateFluid { @Override protected void fillStateContainer(StateContainer.Builder<Fluid, FluidState> builder) { super.fillStateContainer(builder); builder.add(LEVEL_1_8); } @Override public BucketItem getFilledBucket() { return null; } @Override public boolean isSource(FluidState state) { return false; } @Override public int getLevel(FluidState state) { return state.get(ChocolateFluid.LEVEL_1_8); } } public static class Source extends ChocolateFluid { @Override public BucketItem getFilledBucket() { return ModItems.MILK_CHOCOLATE_BUCKET.get(); } @Override public boolean isSource(FluidState state) { return true; } @Override public int getLevel(FluidState state) { return 8; } } } Just in case I'll also put down my Bucket Item code public static RegistryObject<BucketItem> CHOCOLATE_BUCKET = ITEMS.register("chocolate_bucket", () -> new BucketItem( () -> ModFluids.chocolatefluid, new Item.Properties().group(ChocolateItemGroup.CHOCOLATE_ITEM_GROUP) .maxStackSize(1).containerItem(BUCKET) ) ); It registers into the game, is there something I'm missing to connect the two?
-
I was making a new mod and implementing its ore generation, but forge recently updated from .46 to .48 and I'm wondering if that's what's causing this error, but I don't know what else I could put in its place. public class OreGeneration { public static void generateOres(final BiomeLoadingEvent event) { if(!(event.getCategory().equals(Biome.Category.NETHER) || event.getCategory().equals(Biome.Category.THEEND))) { generateOre(event.getGeneration(), OreFeatureConfig.FillerBlockType.BASE_STONE_OVERWORLD, ModBlock.COPPER_ORE.get() .getDefaultState(), 9, 30, 80, 30 ); } } private static void generateOre(BiomeGenerationSettingsBuilder settings, RuleTest fillerType, BlockState state, int veinSize, int minHeight, int maxHeight, int perChunk) { settings.withFeature(GenerationStage.Decoration.UNDERGROUND_ORES, Feature.ORE.withConfiguration(new OreFeatureConfig(fillerType, state, veinSize)) .withPlacement(Placement.RANGE.configure(new TopSolidRangeConfig(minHeight, 0, maxHeight))) .square().func_242731_b(perChunk) ); } } This code works perfectly fine, and generates the ore into the world when I go back to the project public class OreGeneration { public static void generateOres(final BiomeLoadingEvent event) { if(!(event.getCategory().equals(Biome.Category.NETHER) || event.getCategory().equals(Biome.Category.THEEND))) { generateOre(event.getGeneration(), OreFeatureConfig.FillerBlockType.BASE_STONE_OVERWORLD, ModBlocks.CHOCOLATE_ORE.get() .getDefaultState(), 9, 30, 80, 30 ); } } private static void generateOre(BiomeGenerationSettingsBuilder settings, RuleTest fillerType, BlockState state, int veinSize, int minHeight, int maxHeight, int perChunk) { settings.withFeature(GenerationStage.Decoration.UNDERGROUND_ORES, Feature.ORE.withConfiguration(new OreFeatureConfig(fillerType, state, veinSize)) .withPlacement(Placement.RANGE.configure(new TopSolidRangeConfig(minHeight, 0, maxHeight))) .square().func_242731_b(perChunk) ); } } but this virtually the same code won't recognize .func_242731_b which is what I need to make it work because without it the game runs but it won't generate the ore.
-
While block is in world change it to another after x amount of time
tal124 replied to tal124's topic in Modder Support
I've found it and I've looked it over but I'm not too sure which section is referring to a change in block type back to dirt, and I see the "turnToDirt" parameter and I don't fully understand it [removed Mojang code - diesieben07] I've been putting some of the code into my own, but I'm not sure how this will actively switch my own block -
Hello, I've seen mods like blood alchemy turn liquid blood into blocks and AE2 turn Certus seeds into full formed pure Certus quartz, I was wondering how I would go about setting it so that once my block (Copper ore) is set down in the world for long enough that it will turn into oxidized copper ore (just as a way to see if I can) I have no idea where to start with this kind of code and it may come in handy later if I wish to start an actual project of my own and would it also be possible to speed up the process when next to another block or liquid like water?
-
I wasn't expecting such a quick response, Thank you.
-
Hello, I've just started learning how to mod following a few guides, And I've searched around google for a fix for my problem. But, I simply can not find one. The client with the mod worked fine, until I added in a few of the resources so I could have block/item textures, however it isn't the .json's that crashes the launch its the sounds.json file which I don't even have Sorry if this seems like an easy fix and I'm missing it, thank you for any help. I'll put my crash log down under ---- Minecraft Crash Report ---- // I let you down. Sorry :( Time: 3/9/21 4:59 AM Description: Rendering overlay net.minecraft.util.ResourceLocationException: Non [a-z0-9_.-] character in namespace of location: TestMod:sounds.json at net.minecraft.util.ResourceLocation.<init>(ResourceLocation.java:31) ~[forge:?] {re:classloading} at net.minecraft.util.ResourceLocation.<init>(ResourceLocation.java:42) ~[forge:?] {re:classloading} at net.minecraft.client.audio.SoundHandler.prepare(SoundHandler.java:59) ~[forge:?] {re:classloading,pl:runtimedistcleaner:A} at net.minecraft.client.audio.SoundHandler.prepare(SoundHandler.java:34) ~[forge:?] {re:classloading,pl:runtimedistcleaner:A} at net.minecraft.client.resources.ReloadListener.lambda$reload$0(ReloadListener.java:12) ~[forge:?] {re:classloading} at java.util.concurrent.CompletableFuture$AsyncSupply.run(CompletableFuture.java:1604) ~[?:1.8.0_282] {} at java.util.concurrent.CompletableFuture$AsyncSupply.exec(CompletableFuture.java:1596) ~[?:1.8.0_282] {} at java.util.concurrent.ForkJoinTask.doExec(ForkJoinTask.java:289) ~[?:1.8.0_282] {} at java.util.concurrent.ForkJoinPool$WorkQueue.runTask(ForkJoinPool.java:1056) ~[?:1.8.0_282] {} at java.util.concurrent.ForkJoinPool.runWorker(ForkJoinPool.java:1692) ~[?:1.8.0_282] {} at java.util.concurrent.ForkJoinWorkerThread.run(ForkJoinWorkerThread.java:175) ~[?:1.8.0_282] {} A detailed walkthrough of the error, its code path and all known details is as follows: --------------------------------------------------------------------------------------- -- Head -- Thread: Render thread Stacktrace: at net.minecraft.client.renderer.GameRenderer.updateCameraAndRender(GameRenderer.java:496) ~[forge:?] {re:classloading,pl:accesstransformer:B,pl:runtimedistcleaner:A} -- Overlay render details -- Details: Overlay name: net.minecraft.client.gui.ResourceLoadProgressGui Stacktrace: at net.minecraft.client.renderer.GameRenderer.updateCameraAndRender(GameRenderer.java:496) ~[forge-1.16.5-36.0.46_mapped_snapshot_20201028-1.16.3-recomp.jar:?] {re:classloading,pl:accesstransformer:B,pl:runtimedistcleaner:A} at net.minecraft.client.Minecraft.runGameLoop(Minecraft.java:1002) ~[forge-1.16.5-36.0.46_mapped_snapshot_20201028-1.16.3-recomp.jar:?] {re:classloading,pl:accesstransformer:B,pl:runtimedistcleaner:A} at net.minecraft.client.Minecraft.run(Minecraft.java:612) ~[forge-1.16.5-36.0.46_mapped_snapshot_20201028-1.16.3-recomp.jar:?] {re:classloading,pl:accesstransformer:B,pl:runtimedistcleaner:A} at net.minecraft.client.main.Main.main(Main.java:184) ~[forge-1.16.5-36.0.46_mapped_snapshot_20201028-1.16.3-recomp.jar:?] {re:classloading,pl:runtimedistcleaner:A} at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_282] {} at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_282] {} at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_282] {} at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_282] {} at net.minecraftforge.userdev.FMLUserdevClientLaunchProvider.lambda$launchService$0(FMLUserdevClientLaunchProvider.java:52) ~[forge-1.16.5-36.0.46_mapped_snapshot_20201028-1.16.3-recomp.jar:?] {} at cpw.mods.modlauncher.LaunchServiceHandlerDecorator.launch(LaunchServiceHandlerDecorator.java:37) [modlauncher-8.0.9.jar:?] {} at cpw.mods.modlauncher.LaunchServiceHandler.launch(LaunchServiceHandler.java:54) [modlauncher-8.0.9.jar:?] {} at cpw.mods.modlauncher.LaunchServiceHandler.launch(LaunchServiceHandler.java:72) [modlauncher-8.0.9.jar:?] {} at cpw.mods.modlauncher.Launcher.run(Launcher.java:82) [modlauncher-8.0.9.jar:?] {} at cpw.mods.modlauncher.Launcher.main(Launcher.java:66) [modlauncher-8.0.9.jar:?] {} at net.minecraftforge.userdev.LaunchTesting.main(LaunchTesting.java:105) [forge-1.16.5-36.0.46_mapped_snapshot_20201028-1.16.3-recomp.jar:?] {} -- System Details -- Details: Minecraft Version: 1.16.5 Minecraft Version ID: 1.16.5 Operating System: Windows 10 (amd64) version 10.0 Java Version: 1.8.0_282, Amazon.com Inc. Java VM Version: OpenJDK 64-Bit Server VM (mixed mode), Amazon.com Inc. Memory: 655926072 bytes (625 MB) / 3182428160 bytes (3035 MB) up to 7618953216 bytes (7266 MB) CPUs: 24 JVM Flags: 1 total; -XX:HeapDumpPath=MojangTricksIntelDriversForPerformance_javaw.exe_minecraft.exe.heapdump ModLauncher: 8.0.9+86+master.3cf110c ModLauncher launch target: fmluserdevclient ModLauncher naming: mcp ModLauncher services: /mixin-0.8.2.jar mixin PLUGINSERVICE /eventbus-4.0.0.jar eventbus PLUGINSERVICE /forge-1.16.5-36.0.46_mapped_snapshot_20201028-1.16.3-launcher.jar object_holder_definalize PLUGINSERVICE /forge-1.16.5-36.0.46_mapped_snapshot_20201028-1.16.3-launcher.jar runtime_enum_extender PLUGINSERVICE /accesstransformers-3.0.1.jar accesstransformer PLUGINSERVICE /forge-1.16.5-36.0.46_mapped_snapshot_20201028-1.16.3-launcher.jar capability_inject_definalize PLUGINSERVICE /forge-1.16.5-36.0.46_mapped_snapshot_20201028-1.16.3-launcher.jar runtimedistcleaner PLUGINSERVICE /mixin-0.8.2.jar mixin TRANSFORMATIONSERVICE /forge-1.16.5-36.0.46_mapped_snapshot_20201028-1.16.3-launcher.jar fml TRANSFORMATIONSERVICE FML: 36.0 Forge: net.minecraftforge:36.0.46 FML Language Providers: [email protected] minecraft@1 Mod List: client-extra.jar |Minecraft |minecraft |1.16.5 |SIDED_SETU|a1:d4:5e:04:4f:d3:d6:e0:7b:37:97:cf:77:b0:de:ad:4a:47:ce:8c:96:49:5f:0a:cf:8c:ae:b2:6d:4b:8a:3f forge-1.16.5-36.0.46_mapped_snapshot_20201028-1.16|Forge |forge |36.0.46 |SIDED_SETU|NOSIGNATURE main |Test Mod |testmod |NONE |SIDED_SETU|NOSIGNATURE Crash Report UUID: eb1005cb-f6ae-440e-9c07-b7a0c6c219c1 Launched Version: MOD_DEV Backend library: LWJGL version 3.2.2 build 10 Backend API: GeForce GTX 1080/PCIe/SSE2 GL version 4.6.0 NVIDIA 461.40, NVIDIA Corporation GL Caps: Using framebuffer using OpenGL 3.0 Using VBOs: Yes Is Modded: Definitely; Client brand changed to 'forge' Type: Client (map_client.txt) Graphics mode: fancy Resource Packs: Current Language: English (US) CPU: 24x AMD Ryzen 9 3900X 12-Core Processor
-
NVM got it to work i guess they didn't fix it for magiclauncher yet
-
it still doesn't work this time it does
-
i can't seem to get on the game here is the ForgeModLoader-client-0 file