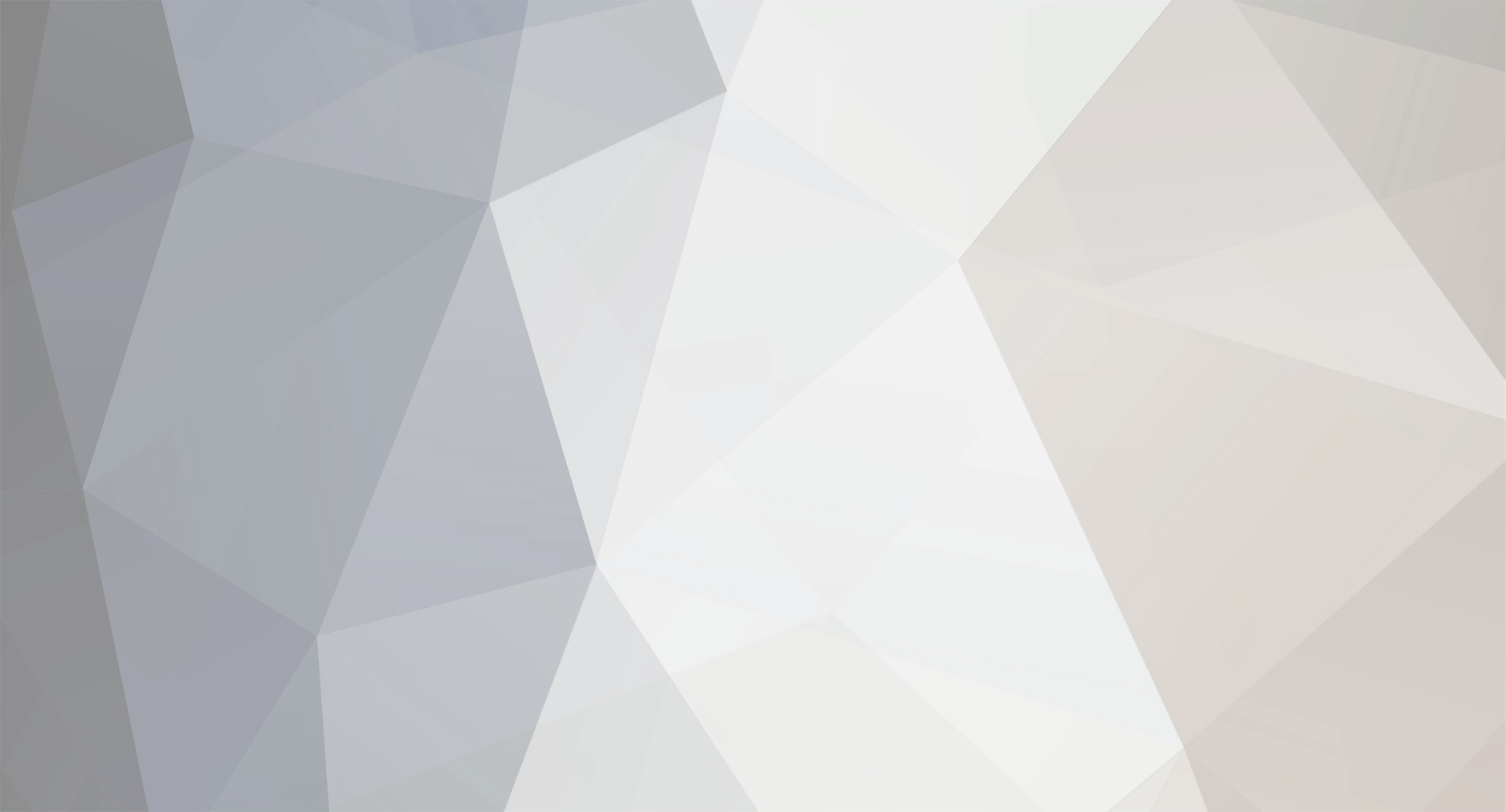
TLHPoE
Members-
Posts
638 -
Joined
-
Last visited
Everything posted by TLHPoE
-
Is there anyway to replicate its function then?
-
I found a PR on the forge GitHub that adds a player enchant item event, but it is not in the recommended build of Forge for 1.9.4 (12.17.0.1976). Is it under another name in this build? Or do I have to switch to another build?
-
I'm stuck on increasing the jump height of the player. Here's my code: @SubscribeEvent public void onJump(LivingEvent.LivingJumpEvent e) { if(!e.getEntityLiving().worldObj.isRemote && e.getEntityLiving() instanceof EntityPlayer) { EntityPlayer p = (EntityPlayer) e.getEntityLiving(); PlayerStats stats = ScalaeCapabilities.getStats(p); if(stats.hasPerk("jump_height_1") || stats.hasPerk("jump_height_2")) { p.posY += 10D; System.out.println("HELLO"); } } } I've tried using the *= operator and nothing happens. The message "HELLO" is being printed.
-
I have this event method registered: @SubscribeEvent public void onUpdate(LivingEvent.LivingUpdateEvent e) { if(e.getEntityLiving() instanceof EntityPlayer) { EntityPlayer p = (EntityPlayer) e.getEntityLiving(); if(!p.worldObj.isRemote) { PlayerStats stats = ScalaeCapabilities.getStats(p); ... if(p.getActiveItemStack() != null && p.getActiveItemStack().getItem() == Items.BOW && (stats.hasPerk(31) || stats.hasPerk(30))) { Field f = null; int useCount = -1; try { f = EntityLivingBase.class.getDeclaredField("activeItemStackUseCount"); f.setAccessible(true); useCount = f.getInt(p); if(stats.hasPerk(31)) { f.set(p, useCount + 1); System.out.println("HELLO"); } } catch(NoSuchFieldException e1) { e1.printStackTrace(); } catch(SecurityException e1) { e1.printStackTrace(); } catch(IllegalArgumentException e1) { e1.printStackTrace(); } catch(IllegalAccessException e1) { e1.printStackTrace(); } } } } } I thought this code would decrease the amount of time needed to fully draw the bow, but instead it did nothing to the length of time (visually at least), made the arrows travel a meager 1 block away after drawing for a couple of seconds, and not being able to produce the "critical hit" that is usually seen when you fully draw the bow and release the arrow. The message "HELLO" is being printed.
-
The line where I sync the code (in the 1st code excerpt below) it returns a null error. Attach Event: @SubscribeEvent public void attachCapabilities(AttachCapabilitiesEvent.Entity e) { net.minecraft.entity.Entity et = e.getEntity(); if(!et.worldObj.isRemote && et instanceof EntityPlayer) { e.addCapability(new ResourceLocation(Scalae.MODID, "IScalaeCapability"), new ICapabilitySerializable<NBTTagCompound>() { IScalaeCapability instance = Scalae.CAPABILITY.getDefaultInstance(); @Override public boolean hasCapability(Capability<?> capability, EnumFacing facing) { return capability == Scalae.CAPABILITY; } @Override public <T> T getCapability(Capability<T> capability, EnumFacing facing) { return capability == Scalae.CAPABILITY ? Scalae.CAPABILITY.<T> cast(instance) : null; } @Override public NBTTagCompound serializeNBT() { return (NBTTagCompound) Scalae.CAPABILITY.getStorage().writeNBT(Scalae.CAPABILITY, instance, null); } @Override public void deserializeNBT(NBTTagCompound nbt) { Scalae.CAPABILITY.getStorage().readNBT(Scalae.CAPABILITY, instance, null, nbt); } }); ScalaeCapabilities.getStats((EntityPlayerMP) et).sync(); } } Here's the get method: public static PlayerStats getStats(EntityPlayer p) { if(!p.worldObj.isRemote) { final IScalaeCapability cap = p.getCapability(Scalae.CAPABILITY, null); if(cap != null) { return cap.getStats().setPlayer(p); } } return null; } And the sync method: public void sync() { //Located in PlayerStats.class, has a player field if(player != null) { Scalae.network.sendTo(new MessageSync(this), (EntityPlayerMP) player); } } I have a message class that can be sent from the client to the server for a request to sync data, but I can't find a good event to place it in client-side.
-
Whenever I render my overlay, the background when opening the inventory or going to the menu is pitch black. Render Code: package tlhpoe.scalae.handler; import org.lwjgl.opengl.*; import net.minecraft.client.*; import net.minecraft.client.gui.*; import net.minecraft.client.renderer.*; import net.minecraft.util.*; import net.minecraftforge.client.event.*; import net.minecraftforge.client.event.RenderGameOverlayEvent.*; import net.minecraftforge.fml.common.eventhandler.*; import net.minecraftforge.fml.common.gameevent.*; import net.minecraftforge.fml.common.gameevent.TickEvent.*; import net.minecraftforge.fml.relauncher.*; import tlhpoe.scalae.*; import tlhpoe.scalae.capabilities.*; @SideOnly(Side.CLIENT) public class ScalaeClientEvents extends Gui { private static final ResourceLocation TEXTURE = new ResourceLocation(Scalae.MODID, "textures/gui/xp_bar.png"); private Minecraft mc; public ScalaeClientEvents() { this.mc = Minecraft.getMinecraft(); } @SubscribeEvent public void drawXPBarPost(RenderGameOverlayEvent.Pre e) { if(e.getType() == ElementType.EXPERIENCE) { e.setCanceled(true); ScaledResolution sRes = new ScaledResolution(mc); int xPos = (sRes.getScaledWidth() - 182) / 2, yPos = sRes.getScaledHeight() - 32 + 3; mc.getTextureManager().bindTexture(TEXTURE); GlStateManager.pushAttrib(); GlStateManager.color(1F, 1F, 1F); GlStateManager.enableAlpha(); GlStateManager.enableBlend(); drawTexturedModalRect(xPos, yPos, 0, 0, 182, 5); int width = (int) (182 * ((float) ClientProxy.PLAYER_XP / (float) ScalaeCapabilities.getXPRequired(ClientProxy.PLAYER_LEVEL))); drawTexturedModalRect(xPos, yPos, 0, 5, width, 5); String s = "" + ClientProxy.PLAYER_LEVEL; xPos = (sRes.getScaledWidth() - mc.fontRendererObj.getStringWidth(s)) / 2; yPos -= 6; mc.fontRendererObj.drawString(s, xPos + 1, yPos, 0); mc.fontRendererObj.drawString(s, xPos - 1, yPos, 0); mc.fontRendererObj.drawString(s, xPos, yPos + 1, 0); mc.fontRendererObj.drawString(s, xPos, yPos - 1, 0); mc.fontRendererObj.drawString(s, xPos, yPos, 0xFFF600); GlStateManager.popAttrib(); } } } I restarted the game and now the chat is black, but not the backgrounds. When the event isn't canceled, everything renders fine.
-
I'm having some trouble with the item model and texture setting. There's nothing in the console that indicates that the texture wasn't found or that the JSON model file loaded. Mod File: package tlhpoe.scalae; import net.minecraftforge.common.capabilities.*; import net.minecraftforge.fml.common.*; import net.minecraftforge.fml.common.Mod.*; import net.minecraftforge.fml.common.event.*; import tlhpoe.scalae.capabilities.ScalaeCapabilities.*; @Mod(modid = Scalae.MODID, version = Scalae.VERSION) public class Scalae { public static final String MODID = "scalae"; public static final String VERSION = "1.0"; @Instance(MODID) public static Scalae instance; @SidedProxy(serverSide = "tlhpoe.scalae.CommonProxy", clientSide = "tlhpoe.scalae.ClientProxy") public static CommonProxy proxy; @CapabilityInject(IScalaeCapability.class) public static final Capability<IScalaeCapability> CAPABILITY = null; @EventHandler public void preInit(FMLPreInitializationEvent event) { proxy.initServer(event); proxy.initClient(event); } @EventHandler public void init(FMLInitializationEvent event) { proxy.initServer(event); proxy.initClient(event); } @EventHandler public void postInit(FMLPostInitializationEvent event) { proxy.initServer(event); proxy.initClient(event); } } Common Proxy: package tlhpoe.scalae; import net.minecraftforge.common.*; import net.minecraftforge.common.capabilities.*; import net.minecraftforge.fml.common.event.*; import net.minecraftforge.fml.common.registry.*; import tlhpoe.scalae.capabilities.ScalaeCapabilities.*; import tlhpoe.scalae.handler.*; import tlhpoe.scalae.item.*; public class CommonProxy { public void initServer(FMLStateEvent state) { if(state instanceof FMLPreInitializationEvent) { GameRegistry.register(ItemScalaeXP.INSTANCE); CapabilityManager.INSTANCE.register(IScalaeCapability.class, new Storage(), DefaultImpl.class); MinecraftForge.EVENT_BUS.register(new ScalaeEvents()); } } public void initClient(FMLStateEvent state) { } } ClientProxy: package tlhpoe.scalae; import net.minecraft.client.*; import net.minecraft.client.renderer.block.model.*; import net.minecraft.item.*; import net.minecraftforge.client.model.*; import net.minecraftforge.fml.common.event.*; import tlhpoe.scalae.item.*; public class ClientProxy extends CommonProxy { @Override public void initClient(FMLStateEvent state) { super.initClient(state); if(state instanceof FMLInitializationEvent) { registerItemRenderer(ItemScalaeXP.INSTANCE); } } private static void registerItemRenderer(Item i) { ModelLoader.setCustomModelResourceLocation(i, 0, new ModelResourceLocation(i.getRegistryName(), "inventory")); } } Item File: public class ItemScalaeXP extends Item { public static final ItemScalaeXP INSTANCE = new ItemScalaeXP(); public static final String NAME = "scalae_xp"; private ItemScalaeXP() { setRegistryName(NAME); setUnlocalizedName(NAME); setCreativeTab(CreativeTabs.COMBAT); } ...
-
I realize that, but if I listened for whenever the player was about to pickup an XP orb, added its value to the player's current XP, and checked the sum against the new XP bar cap, wouldn't it work?
-
I'm trying to detect whenever the player levels up. I thought this check would work, but it doesn't. Event Class: package tlhpoe.scalae.handler; import net.minecraft.entity.player.*; import net.minecraftforge.event.entity.player.*; import net.minecraftforge.fml.common.eventhandler.*; public class Events { @SubscribeEvent public void playerPickupXP(PlayerPickupXpEvent e) { EntityPlayer p = e.getEntityPlayer(); if(!p.getEntityWorld().isRemote) { if((p.experience + e.getOrb().xpValue) >= p.xpBarCap()) { System.out.println("Level up!"); } } } }
-
I have Mine and Blade: Battlegear 2 installed and it patched the ModelBiped class. I removed it to see if it caused anything, nope. I'm going to try to make my own biped model to render.
-
Upon further research I discovered some past problems with rendering entities in 1.8 that were fixed by registering the renderers after the pre-initialization stage. I moved my entity renderer registerings to the initialization stage, but nothing changed.
-
I believe you're looking for the OreDictionary, but I don't think Minecraft uses the OreDictionary for blaze rods. You're going to have to iterate through the recipes list and clone each recipe in it that contains the blaze rod and replace the blaze rod with your blaze rod. Not sure if you'll need to make your mod load last or just add the recipes post-initialization.
-
Do you mean this? Minecraft.getMinecraft().getRenderManager() I didn't know which render manager to get, so I figured the Minecraft class had an instance of it. Also if you mean this: this.addLayer(new LayerBipedArmor(this)); this.addLayer(new LayerHeldItem(this)); this.addLayer(new LayerArrow(this)); I plan on making the entity equip armor and hold items.
-
If you mean the ModelBiped function, I overrode it so I could put the print in to check if it was rendering. I also called the super so that it would render the model if it did, which it didn't.
-
I'm an idiot. The render isn't being called at all, even though it's registered. Render: package enlistment.render.entity; import org.lwjgl.opengl.GL11; import enlistment.Enlistment; import enlistment.entity.EntityTroop; import net.minecraft.client.Minecraft; import net.minecraft.client.model.ModelBiped; import net.minecraft.client.renderer.entity.RendererLivingEntity; import net.minecraft.client.renderer.entity.layers.LayerArrow; import net.minecraft.client.renderer.entity.layers.LayerBipedArmor; import net.minecraft.client.renderer.entity.layers.LayerHeldItem; import net.minecraft.entity.Entity; import net.minecraft.entity.EntityLivingBase; import net.minecraft.util.ResourceLocation; public class RenderTroop extends RendererLivingEntity { public static final ResourceLocation TROOP_TEXTURE = new ResourceLocation(Enlistment.ID + ":textures/entity/troop.png"); public RenderTroop() { super(Minecraft.getMinecraft().getRenderManager(), new ModelBiped(1F) { @Override public void render(Entity entityIn, float p_78088_2_, float p_78088_3_, float p_78088_4_, float p_78088_5_, float p_78088_6_, float scale) { super.render(entityIn, p_78088_2_, p_78088_3_, p_78088_4_, p_78088_5_, p_78088_6_, scale); System.out.println("RENDERING TROOP MODEL AT X: " + entityIn.posX + ", Y: " + entityIn.posY + ", Z: " + entityIn.posZ); } }, 1F); this.addLayer(new LayerBipedArmor(this)); this.addLayer(new LayerHeldItem(this)); this.addLayer(new LayerArrow(this)); } @Override protected void preRenderCallback(EntityLivingBase entity, float f) { if(entity instanceof EntityTroop) { EntityTroop troop = (EntityTroop) entity; GL11.glScalef(1F, 1F, 1F); System.out.println("PRE-RENDERING TROOP AT X: " + troop.posX + ", Y: " + troop.posY + ", Z: " + troop.posZ); } } @Override protected ResourceLocation getEntityTexture(Entity entity) { return TROOP_TEXTURE; /* if(entity instanceof EntityTroop) { EntityTroop troop = (EntityTroop) entity; switch(troop.getTier()) { case (69): return null; default: return TROOP_TEXTURE; } } return null; */ } }
-
It's called in my ClientProxy, which in turn is called client-side from my mod class, which extends an abstract class that automatically calls the proxy. ClientProxy: package enlistment.network; import enlistment.entity.EntityHandler; import enlistment.gui.GuiTroopsOverlay; import net.minecraftforge.common.MinecraftForge; public class ClientProxy extends ServerProxy { @Override public void init() { super.init(); MinecraftForge.EVENT_BUS.register(new GuiTroopsOverlay()); EntityHandler.initRenders(); } } The overlay that is also registered is being rendered.
-
Yes EntityHandler: package enlistment.entity; import enlistment.Enlistment; import enlistment.render.entity.RenderTroop; import net.minecraft.entity.EntityLiving; import net.minecraft.entity.EnumCreatureType; import net.minecraft.world.biome.BiomeGenBase; import net.minecraftforge.fml.client.registry.RenderingRegistry; import net.minecraftforge.fml.common.registry.EntityRegistry; import net.minecraftforge.fml.relauncher.Side; import net.minecraftforge.fml.relauncher.SideOnly; public class EntityHandler { public static void init() { registerEntity(EntityTroop.class, "Troop"); } @SideOnly(Side.CLIENT) public static void initRenders() { RenderingRegistry.registerEntityRenderingHandler(EntityTroop.class, new RenderTroop()); } private static void registerEntity(Class<? extends EntityLiving> clazz, String name) { EntityRegistry.registerGlobalEntityID(clazz, name, EntityRegistry.findGlobalUniqueEntityId()); EntityRegistry.addSpawn(clazz, 100, 6, 8, EnumCreatureType.CREATURE, BiomeGenBase.plains, BiomeGenBase.forest, BiomeGenBase.desert, BiomeGenBase.desertHills, BiomeGenBase.forestHills); Enlistment.instance.entities.add(name, clazz); } }
-
I put a print inside of my entity's update method to check if they are in the world and it is being called. Render: package enlistment.render.entity; import org.lwjgl.opengl.GL11; import enlistment.Enlistment; import enlistment.entity.EntityTroop; import net.minecraft.client.Minecraft; import net.minecraft.client.model.ModelBiped; import net.minecraft.client.renderer.entity.RendererLivingEntity; import net.minecraft.client.renderer.entity.layers.LayerArrow; import net.minecraft.client.renderer.entity.layers.LayerBipedArmor; import net.minecraft.client.renderer.entity.layers.LayerHeldItem; import net.minecraft.entity.Entity; import net.minecraft.entity.EntityLivingBase; import net.minecraft.util.ResourceLocation; public class RenderTroop extends RendererLivingEntity { public static final ResourceLocation TROOP_TEXTURE = new ResourceLocation(Enlistment.ID + ":textures/entity/troop.png"); public RenderTroop() { super(Minecraft.getMinecraft().getRenderManager(), new ModelBiped(1F), 1F); this.addLayer(new LayerBipedArmor(this)); this.addLayer(new LayerHeldItem(this)); this.addLayer(new LayerArrow(this)); } @Override protected void preRenderCallback(EntityLivingBase entity, float f) { if(entity instanceof EntityTroop) { EntityTroop troop = (EntityTroop) entity; GL11.glScalef(1F, 1F, 1F); } } @Override protected ResourceLocation getEntityTexture(Entity entity) { return TROOP_TEXTURE; /* if(entity instanceof EntityTroop) { EntityTroop troop = (EntityTroop) entity; switch(troop.getTier()) { case (69): return null; default: return TROOP_TEXTURE; } } return null; */ } } Entity: package enlistment.entity; import java.util.UUID; import net.minecraft.entity.EntityCreature; import net.minecraft.entity.EntityLiving; import net.minecraft.entity.SharedMonsterAttributes; import net.minecraft.entity.ai.EntityAIAttackOnCollide; import net.minecraft.entity.ai.EntityAIMoveThroughVillage; import net.minecraft.entity.ai.EntityAINearestAttackableTarget; import net.minecraft.entity.ai.EntityAISwimming; import net.minecraft.entity.ai.EntityAIWander; import net.minecraft.entity.ai.EntityAIWatchClosest; import net.minecraft.entity.ai.EntityAIWatchClosest2; import net.minecraft.entity.monster.EntityMob; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.util.DamageSource; import net.minecraft.world.World; public class EntityTroop extends EntityCreature { protected FoodStatsTroop foodStats = new FoodStatsTroop(); protected int tier; protected boolean hired = false; protected int morale; protected int relationWithPlayer; protected boolean stationary = false; public EntityTroop(World world) { super(world); this.setSize(0.6F, 1.95F); this.setCanPickUpLoot(false); applyEntityAI(); } public EntityTroop(World world, boolean hired) { this(world); this.hired = hired; } @Override protected void entityInit() { super.entityInit(); this.dataWatcher.addObject(17, ""); } protected void applyEntityAI() { this.tasks.addTask(0, new EntityAISwimming(this)); this.tasks.addTask(5, new EntityAITroopFollow(this, 1.0D, 10.0F, 2.0F)); this.tasks.addTask(9, new EntityAIWatchClosest2(this, EntityPlayer.class, 3F, 1F)); this.tasks.addTask(9, new EntityAIWander(this, 0D)); this.tasks.addTask(10, new EntityAIWatchClosest(this, EntityLiving.class, 8F)); this.tasks.addTask(4, new EntityAIAttackOnCollide(this, EntityMob.class, 1D, true)); this.tasks.addTask(6, new EntityAIMoveThroughVillage(this, 1D, false)); this.targetTasks.addTask(1, new EntityAINearestAttackableTarget(this, EntityMob.class, true)); } protected void applyEntityAttributes() { super.applyEntityAttributes(); this.getEntityAttribute(SharedMonsterAttributes.followRange).setBaseValue(16D); this.getEntityAttribute(SharedMonsterAttributes.maxHealth).setBaseValue(10D); this.getEntityAttribute(SharedMonsterAttributes.followRange).setBaseValue(35D); this.getEntityAttribute(SharedMonsterAttributes.movementSpeed).setBaseValue(0.1D); this.getEntityAttribute(SharedMonsterAttributes.knockbackResistance).setBaseValue(1D); this.getAttributeMap().registerAttribute(SharedMonsterAttributes.attackDamage).setBaseValue(1D); } @Override public void onLivingUpdate() { super.onLivingUpdate(); if(getFoodStats().needFood() && this.ticksExisted % 10 == 0) { getFoodStats().setFoodLevel(this.foodStats.getFoodLevel() + 1); } } @Override public void onUpdate() { super.onUpdate(); if(!this.worldObj.isRemote) { System.out.println("Hi! I'm here at X: " + this.posX + ", Z: " + this.posZ); getFoodStats().onUpdate(this); } } @Override public void readEntityFromNBT(NBTTagCompound comp) { super.readEntityFromNBT(comp); foodStats.readNBT(comp); NBTTagCompound nbt = comp.getCompoundTag("Enlistment_NBT"); setTier(nbt.getInteger("Tier")); setHired(nbt.getBoolean("Hired")); setMorale(nbt.getInteger("Morale")); setRelationWithPlayer(nbt.getInteger("Relation_with_Player")); setOwnerID(nbt.getString("Owner_ID")); setStationary(nbt.getBoolean("Stationary")); } @Override public void writeEntityToNBT(NBTTagCompound comp) { super.writeEntityToNBT(comp); foodStats.writeNBT(comp); NBTTagCompound nbt = comp.getCompoundTag("Enlistment_NBT"); nbt.setInteger("Tier", tier); nbt.setBoolean("Hired", hired); nbt.setInteger("Morale", morale); nbt.setInteger("Relation_with_Player", relationWithPlayer); nbt.setString("Owner_ID", getOwnerID()); nbt.setBoolean("Stationary", isStationary()); comp.setTag("Enlistment_NBT", nbt); } @Override public boolean interact(EntityPlayer player) { if(getOwnerID().equals("")) { setOwnerID(player.getUniqueID().toString()); return true; } else if(getOwnerID().equals(player.getUniqueID().toString())) { setStationary(!isStationary()); return true; } return super.interact(player); } @Override public boolean attackEntityFrom(DamageSource src, float par2) { if(this.isEntityInvulnerable(src)) { return false; } else { //retalliate return super.attackEntityFrom(src, par2); } } public boolean canEat() { return getFoodStats().needFood(); } public boolean shouldHeal() { return this.getHealth() > 0.0F && this.getHealth() < this.getMaxHealth(); } public void upgradeTier() { setTier(getTier() + 1); System.out.println("Troop #" + this.getEntityId() + " has ranked up!"); this.getEntityAttribute(SharedMonsterAttributes.attackDamage).setBaseValue(1D + (2D * tier)); this.getEntityAttribute(SharedMonsterAttributes.maxHealth).setBaseValue(10D + (7.5D * tier)); } @Override protected String getHurtSound() { return "game.player.hurt"; } @Override protected String getDeathSound() { return "game.player.die"; } public String getOwnerID() { return this.dataWatcher.getWatchableObjectString(17); } public void setOwnerID(String ownerUuid) { this.dataWatcher.updateObject(17, ownerUuid); } public EntityPlayer getOwner() { try { UUID uuid = UUID.fromString(getOwnerID()); return uuid == null ? null : this.worldObj.getPlayerEntityByUUID(uuid); } catch(IllegalArgumentException illegalargumentexception) { return null; } } public FoodStatsTroop getFoodStats() { return foodStats; } public void setFoodStats(FoodStatsTroop foodStats) { this.foodStats = foodStats; } public int getTier() { return tier; } public void setTier(int tier) { this.tier = tier; } public boolean isHired() { return hired; } public void setHired(boolean hired) { this.hired = hired; } public int getMorale() { return morale; } public void setMorale(int morale) { this.morale = morale; } public int getRelationWithPlayer() { return relationWithPlayer; } public void setRelationWithPlayer(int relationWithPlayer) { this.relationWithPlayer = relationWithPlayer; } public boolean isStationary() { return stationary; } public void setStationary(boolean stationary) { this.stationary = stationary; } } Sorry if I sound vague, I am completely in the dark myself when it comes to rendering.
-
Make Player Model AIM whilst holding specific item
TLHPoE replied to ScottehBoeh's topic in Modder Support
Maybe you have to override the Item#getMaxItemUseDuration and Item#onItemRightClick methods? By default the first one is zero and the second one is empty (except for a return). Look in the ItemBow class to see what to add. -
I'm trying to install CodeChickenCore in my workspace so I can run other mods. I'm creating an addon to another mod so it is easier to see what I'm doing.
-
Hi, I inputted "C:\Users\phank\.gradle\caches\minecraft\net\minecraftforge\forge\1.7.10-10.13.4.1558-1.7.10\unpacked\conf" for the "mcp conf dir for the deobfuscator" popup when launching, and got this error: [18:43:11] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: java.lang.RuntimeException: Could not find methods.csv [18:43:11] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at codechicken.lib.asm.ObfMapping$MCPRemapper.parseConfDir(ObfMapping.java:150) [18:43:11] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at codechicken.lib.asm.ObfMapping$MCPRemapper.getConfFiles(ObfMapping.java:96) [18:43:11] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at codechicken.lib.asm.ObfMapping$MCPRemapper.<init>(ObfMapping.java:162) [18:43:11] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at codechicken.lib.asm.ObfMapping.loadMCPRemapper(ObfMapping.java:205) [18:43:11] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at codechicken.lib.asm.ObfMapping.<clinit>(ObfMapping.java:217) [18:43:11] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at codechicken.core.asm.MCPDeobfuscationTransformer.load(MCPDeobfuscationTransformer.java:113) [18:43:11] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at codechicken.core.asm.MCPDeobfuscationTransformer$LoadPlugin.injectData(MCPDeobfuscationTransformer.java:52) [18:43:11] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at net.minecraftforge.fml.relauncher.CoreModManager$FMLPluginWrapper.injectIntoClassLoader(CoreModManager.java:137) [18:43:11] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at net.minecraft.launchwrapper.Launch.launch(Launch.java:115) [18:43:11] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [18:43:11] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at net.minecraftforge.gradle.GradleStartCommon.launch(Unknown Source) [18:43:11] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at GradleStart.main(Unknown Source) [18:43:12] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: java.lang.RuntimeException: Could not find methods.csv [18:43:12] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at codechicken.lib.asm.ObfMapping$MCPRemapper.parseConfDir(ObfMapping.java:150) [18:43:12] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at codechicken.lib.asm.ObfMapping$MCPRemapper.getConfFiles(ObfMapping.java:96) [18:43:12] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at codechicken.lib.asm.ObfMapping$MCPRemapper.<init>(ObfMapping.java:162) [18:43:12] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at codechicken.lib.asm.ObfMapping.loadMCPRemapper(ObfMapping.java:205) [18:43:12] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at codechicken.lib.asm.ObfMapping.<clinit>(ObfMapping.java:217) [18:43:12] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at codechicken.core.asm.MCPDeobfuscationTransformer.load(MCPDeobfuscationTransformer.java:113) [18:43:12] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at codechicken.core.asm.MCPDeobfuscationTransformer$LoadPlugin.injectData(MCPDeobfuscationTransformer.java:52) [18:43:12] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at net.minecraftforge.fml.relauncher.CoreModManager$FMLPluginWrapper.injectIntoClassLoader(CoreModManager.java:137) [18:43:12] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at net.minecraft.launchwrapper.Launch.launch(Launch.java:115) [18:43:12] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [18:43:12] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at net.minecraftforge.gradle.GradleStartCommon.launch(Unknown Source) [18:43:12] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at GradleStart.main(Unknown Source) [18:43:12] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: java.lang.RuntimeException: Could not find methods.csv [18:43:12] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at codechicken.lib.asm.ObfMapping$MCPRemapper.parseConfDir(ObfMapping.java:150) [18:43:12] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at codechicken.lib.asm.ObfMapping$MCPRemapper.getConfFiles(ObfMapping.java:96) [18:43:12] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at codechicken.lib.asm.ObfMapping$MCPRemapper.<init>(ObfMapping.java:162) [18:43:12] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at codechicken.lib.asm.ObfMapping.loadMCPRemapper(ObfMapping.java:205) [18:43:12] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at codechicken.lib.asm.ObfMapping.<clinit>(ObfMapping.java:217) [18:43:12] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at codechicken.core.asm.MCPDeobfuscationTransformer.load(MCPDeobfuscationTransformer.java:113) [18:43:12] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at codechicken.core.asm.MCPDeobfuscationTransformer$LoadPlugin.injectData(MCPDeobfuscationTransformer.java:52) [18:43:12] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at net.minecraftforge.fml.relauncher.CoreModManager$FMLPluginWrapper.injectIntoClassLoader(CoreModManager.java:137) [18:43:12] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at net.minecraft.launchwrapper.Launch.launch(Launch.java:115) [18:43:12] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [18:43:12] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at net.minecraftforge.gradle.GradleStartCommon.launch(Unknown Source) [18:43:12] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at GradleStart.main(Unknown Source) Exception in thread "main" [18:43:12] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: java.lang.ExceptionInInitializerError [18:43:12] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at codechicken.core.asm.MCPDeobfuscationTransformer.load(MCPDeobfuscationTransformer.java:113) [18:43:12] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at codechicken.core.asm.MCPDeobfuscationTransformer$LoadPlugin.injectData(MCPDeobfuscationTransformer.java:52) [18:43:12] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at net.minecraftforge.fml.relauncher.CoreModManager$FMLPluginWrapper.injectIntoClassLoader(CoreModManager.java:137) [18:43:12] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at net.minecraft.launchwrapper.Launch.launch(Launch.java:115) [18:43:12] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [18:43:12] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at net.minecraftforge.gradle.GradleStartCommon.launch(Unknown Source) [18:43:12] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at GradleStart.main(Unknown Source) [18:43:12] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: Caused by: java.lang.RuntimeException: Failed to select mappings directory, set it manually in the config [18:43:12] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at codechicken.lib.asm.ObfMapping$MCPRemapper.getConfFiles(ObfMapping.java:107) [18:43:12] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at codechicken.lib.asm.ObfMapping$MCPRemapper.<init>(ObfMapping.java:162) [18:43:12] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at codechicken.lib.asm.ObfMapping.loadMCPRemapper(ObfMapping.java:205) [18:43:12] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: at codechicken.lib.asm.ObfMapping.<clinit>(ObfMapping.java:217) [18:43:12] [main/INFO] [sTDERR]: [java.lang.Throwable$WrappedPrintStream:println:748]: ... 7 more Java HotSpot(TM) 64-Bit Server VM warning: Using incremental CMS is deprecated and will likely be removed in a future release I looked in the directory and the methods.csv were, of course, not there. Did I setup my workspace incorrectly? I ran the command "gradlew setupDecompWorkspace eclipse" Also sorry if I'm in the wrong forum.
-
Entity teleports upwards whenever something collides with it
TLHPoE replied to Asweez's topic in Modder Support
I agree with coolAlias, many problems I've encountered with entities have stemmed from not calling the supers in each method I override. If that doesn't work, try slowly removing each method you override to see which one is causing the issue. -
I have little to no knowledge of world generation (besides basic ore generation), and making a new village home seemed like a good place to start. This is what I have so far VillageHandler: package enlistment.village; import java.util.ArrayList; import java.util.Random; import net.minecraft.world.gen.structure.StructureVillagePieces; import net.minecraftforge.fml.common.registry.VillagerRegistry; public class VillageHandler { public static void init() { ArrayList<StructureVillagePieces.PieceWeight> pieces = new ArrayList<StructureVillagePieces.PieceWeight>(); pieces.add(new StructureVillagePieces.PieceWeight(VillageTroopHome.class, 100, 100)); VillagerRegistry.addExtraVillageComponents(pieces, new Random(), 100); } } VIllageTroopHome: package enlistment.village; import java.util.Random; import net.minecraft.init.Blocks; import net.minecraft.util.EnumFacing; import net.minecraft.world.World; import net.minecraft.world.gen.structure.StructureBoundingBox; import net.minecraft.world.gen.structure.StructureVillagePieces; public class VillageTroopHome extends StructureVillagePieces.Village { public VillageTroopHome(StructureVillagePieces.Start start, int type, Random random, StructureBoundingBox bb, EnumFacing direction) { super(start, type); this.coordBaseMode = direction; this.boundingBox = bb; } @Override public boolean addComponentParts(World world, Random random, StructureBoundingBox bb) { System.out.println("HI"); if(this.field_143015_k < 0) { this.field_143015_k = this.getAverageGroundLevel(world, bb); if(this.field_143015_k < 0) { return true; } this.boundingBox.offset(0, this.field_143015_k - this.boundingBox.maxY + 12 - 1, 0); } this.fillWithBlocks(world, bb, 1, 1, 1, 3, 3, 3, Blocks.cobblestone.getDefaultState(), Blocks.cobblestone.getDefaultState(), false); this.fillWithBlocks(world, bb, 2, 2, 2, 2, 100, 2, Blocks.glass.getDefaultState(), Blocks.glass.getDefaultState(), false); this.spawnVillagers(world, bb, 2, 1, 2, 1); return true; } } Looking through the source code of Minecraft, I haven't been able to decipher what the cryptic integers in the VIllagerRegistry#addExtraVillageComponents method does, so I set them to 100 for testing purposes. Also I set the integers in the StructureVillagePieces#PieceWeight constructor to 100 for the same reason. The questions I have besides the integers are if I'm using the correct methods, extending the right classes, and passing the right objects.
-
I have been told that 1.8 is buggy and that I should use 1.7.10 and skip to 1.9, but others say that 1.8 will be stable enough and that it will replace 1.7.10 as the staple modding version for a long time. In all honesty, I haven't been listening to any Minecraft/Forge related news at all since I just returned from a hiatus.