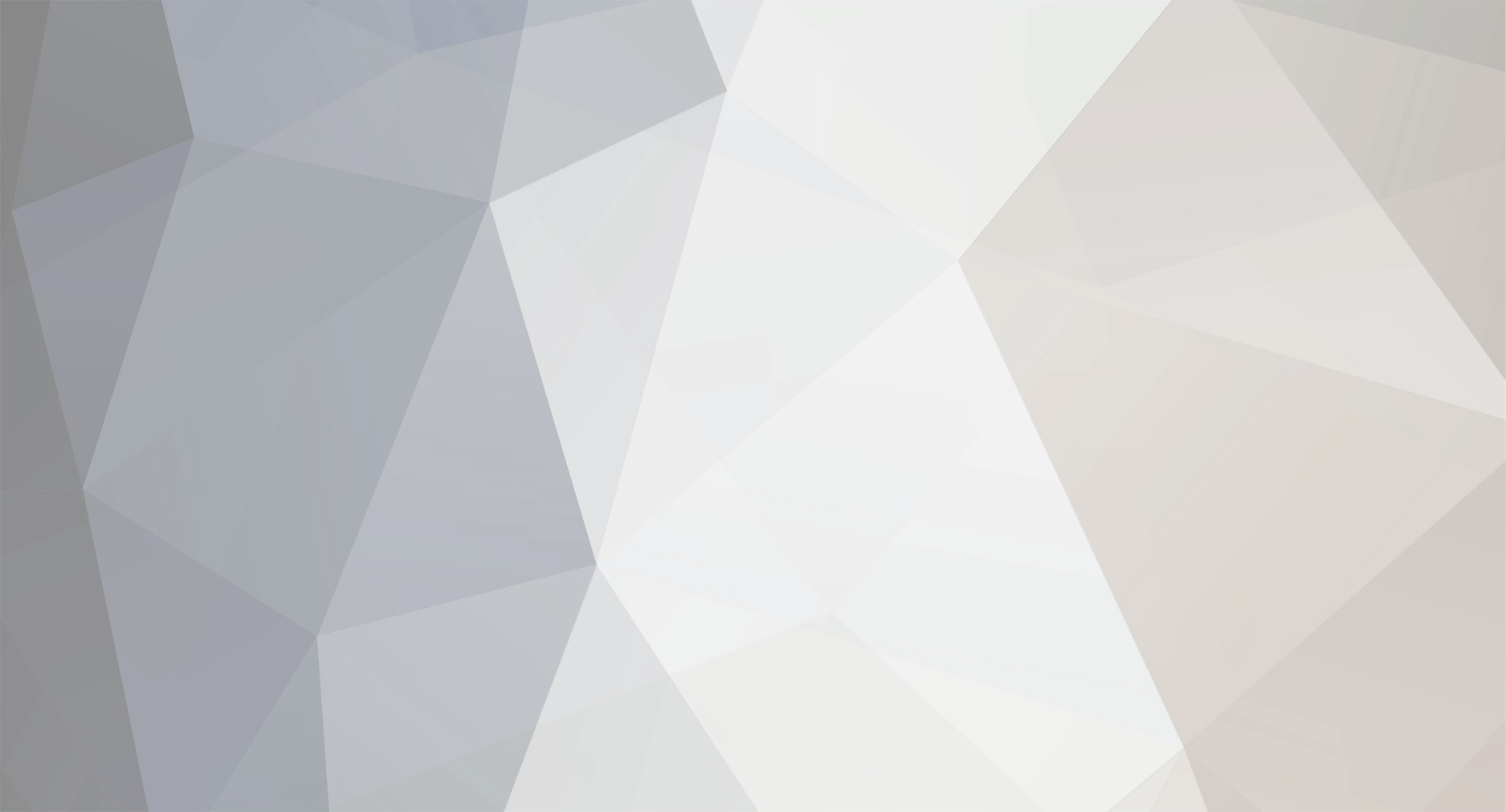
tattyseal
-
Posts
194 -
Joined
-
Last visited
Posts posted by tattyseal
-
-
Updated last post, I forgot to actually change the code, sorry!
-
You need to have @EventHandler above the preInit and Init like:
@EventHandler
public void preInit(FMLPreInitializationEvent event){
proxy.initRenderers();
}
@EventHandler
public void init(FMLInitializationEvent event){
GameRegistry.registerTileEntity(TileEntityTower1Entity.class, "TileEntityTower1");
GameRegistry.registerItem(Wand, ITEMSINFO.WAND_UNLOCALIZED_NAME);
LanguageRegistry.addName(Wand, ITEMSINFO.WAND_NAME);
GameRegistry.registerBlock(TileEntityTower1, BLOCKSINFO.TOWER1_UNLOCALIZED_NAME);
LanguageRegistry.addName(TileEntityTower1, BLOCKSINFO.TOWER1_NAME);
}
@EventHandler
public void modIntegration(FMLPostInitializationEvent event){
}
-
You can't compare strings like that.s.getItem().getUnlocalizedName() == DynamicBlocks.dynGlass.getUnlocalizedName()
WHAT?Block.getBlockFromName(i.getStackInSlot(0).getUnlocalizedName())I got it working
CODE:
package org.db.block;
import net.minecraft.block.Block;
import net.minecraft.inventory.InventoryCrafting;
import net.minecraft.item.ItemBlock;
import net.minecraft.item.ItemStack;
import net.minecraft.item.crafting.IRecipe;
import net.minecraft.nbt.NBTTagCompound;
import net.minecraft.world.World;
import org.db.DynamicBlocks;
public class DynamicBlockRecipe implements IRecipe
{
@Override
public boolean matches(InventoryCrafting i, World w)
{
if(i != null && i.getStackInSlot(0) != null && i.getStackInSlot(1) != null && i.getStackInSlot(2) != null && i.getStackInSlot(0).getItem() instanceof ItemBlock && DynamicBlock.isBlockValidForInv(i.getStackInSlot(0)) && i.getStackInSlot(2).getItem() instanceof ItemBlock && DynamicBlock.isBlockValidForInv(i.getStackInSlot(2)))
{
ItemStack is = i.getStackInSlot(1);
if(is != null && is.getItem() != null && is.getItem().getUnlocalizedName().contains(DynamicBlocks.dynBlock.getUnlocalizedName()))
{
return true;
}
}
return false;
}
@Override
public ItemStack getCraftingResult(InventoryCrafting i)
{
ItemStack is = new ItemStack(DynamicBlocks.dynBlock, 1);
NBTTagCompound tag = new NBTTagCompound();
tag.setInteger("meta", Block.getIdFromBlock(Block.getBlockFromItem(i.getStackInSlot(0).getItem())));
tag.setInteger("blockMeta", Block.getIdFromBlock(Block.getBlockFromItem(i.getStackInSlot(2).getItem())));
is.stackTagCompound = tag;
return is;
}
@Override
public int getRecipeSize()
{
return 9;
}
@Override
public ItemStack getRecipeOutput()
{
return null;
}
}
-
Code
package org.db.block;
import net.minecraft.block.Block;
import net.minecraft.inventory.InventoryCrafting;
import net.minecraft.item.ItemBlock;
import net.minecraft.item.ItemStack;
import net.minecraft.item.crafting.IRecipe;
import net.minecraft.nbt.NBTTagCompound;
import net.minecraft.world.World;
import org.db.DynamicBlocks;
public class GlassRecipe implements IRecipe
{
@Override
public boolean matches(InventoryCrafting i, World w)
{
if(i != null && i.getStackInSlot(0) != null && i.getStackInSlot(1) != null && i.getStackInSlot(2) != null && i.getStackInSlot(0).getItem() instanceof ItemBlock && DynamicGlass.isBlockValidForInv(i.getStackInSlot(0)) && i.getStackInSlot(2).getItem() instanceof ItemBlock && DynamicGlass.isBlockValidForInv(i.getStackInSlot(2)))
{
ItemStack is = i.getStackInSlot(1);
if(is != null && is.getItem() != null && is.getItem().getUnlocalizedName() == DynamicBlocks.dynGlass.getUnlocalizedName())
{
return true;
}
}
return false;
}
@Override
public ItemStack getCraftingResult(InventoryCrafting i)
{
ItemStack is = new ItemStack(DynamicBlocks.dynGlass, 1);
NBTTagCompound tag = new NBTTagCompound();
tag.setInteger("meta", Block.getIdFromBlock(Block.getBlockFromName(i.getStackInSlot(0).getUnlocalizedName())));
tag.setInteger("blockMeta", Block.getIdFromBlock(Block.getBlockFromName(i.getStackInSlot(2).getUnlocalizedName())));
is.stackTagCompound = tag;
return is;
}
@Override
public int getRecipeSize()
{
return 9;
}
@Override
public ItemStack getRecipeOutput()
{
return null;
}
}
Main mod file in preInit:
GameRegistry.addRecipe(new GlassRecipe());
But the recipe does not work ingame
-
Hello.
So basically I want to be able to craft my block with any blocks, then use a CraftingEvent to check if it is valid for my block.
So I want it to be like:
BDB
B being the block
D being my block
But I cannot figure out how to make it B to be any block, does anyone know how to do this?
-
You did not install the mod on your server
-
1) Click your project
2) Press F5
-
You are adding a recipe before Registering your item
1) ALWAYS POST CODE
-
How would i make the other layer?
-
I want my whole armor to be colored, not like Leather with the stripe
-
**BUMP**
-
@Override public Object getClientGuiElement(int id, EntityPlayer player, World world, int x, int y, int z){ TileEntity te = world.getTileEntity(x, y, z); if(te instanceof TileEntityBlockCrafter){ return new ContainerBlockCrafter(player.inventory, (TileEntityBlockCrafter) te); } return null; }
You are returning your Container on the client, return your Gui class not the Container
-
ZaetRegistry.registerColoredArmor("zaet", "dyeArmor", ArmorMaterial.CLOTH, tabZaetMech);
public static void registerColoredArmor(String folder, String name, ArmorMaterial material, CreativeTabs creativeTabs) { for(int i = 0; i < 16; i++) { for(int x = 0; x < 4; x++) { Item armor = new ColoredArmor(i, folder, name, material, 0, x, creativeTabs); GameRegistry.registerItem(armor, armor.getUnlocalizedName()); } }
I assume CLOTH is leather
-
ZaetRegistry.registerColoredArmor("zaet", "dyeArmor", ArmorMaterial.CLOTH, tabZaetMech);
public static void registerColoredArmor(String folder, String name, ArmorMaterial material, CreativeTabs creativeTabs) { for(int i = 0; i < 16; i++) { for(int x = 0; x < 4; x++) { Item armor = new ColoredArmor(i, folder, name, material, 0, x, creativeTabs); GameRegistry.registerItem(armor, armor.getUnlocalizedName()); } }
-
Updated the OP with an image and updated code
package org.zaet.api.armor; import net.minecraft.client.renderer.texture.IIconRegister; import net.minecraft.creativetab.CreativeTabs; import net.minecraft.entity.Entity; import net.minecraft.init.Blocks; import net.minecraft.item.ItemArmor; import net.minecraft.item.ItemStack; import org.zaet.api.ZaetRegistry; public class ColoredArmor extends ItemArmor { public static final int helm = 0, chest = 1, legs = 2, boots = 3; public String folder; public String armorName; public int color; public ColoredArmor(int color, String folder, String armorName, ArmorMaterial material, int render, int type, CreativeTabs tab) { super(material, render, type); this.color = color; this.folder = folder; this.armorName = armorName; setTextureName(folder + ":" + armorName + "_" + type); setUnlocalizedName(folder + "_" + armorName + "_" + type + color); setCreativeTab(tab); } @Override public void registerIcons(IIconRegister r) { this.itemIcon = r.registerIcon(folder + ":" + armorName + "_" + armorType); } @Override public String getArmorTexture(ItemStack stack, Entity entity, int slot, String type) { switch(this.armorType) { case helm: return folder + ":" + "textures/armor/" + armorName + "_1" + ".png"; case chest: return folder + ":" + "textures/armor/" + armorName + "_1" + ".png"; case legs: return folder + ":" + "textures/armor/" + armorName + "_2" + ".png"; case boots: return folder + ":" + "textures/armor/" + armorName + "_1" + ".png"; default: return folder + ":" + "textures/armor/" + armorName + "_1" + ".png"; } } @Override public boolean hasColor(ItemStack item) { return true; } @Override public int getColor(ItemStack item) { return ZaetRegistry.getColorHexCode(color); } @Override public int getColorFromItemStack(ItemStack item, int unUsed) { return getColor(null); } }
-
That only affects the Item icon, not the armor texture. I haven't done the Item Textures.
-
I do not see anything different either... Odd.
-
*BUMP*
I would not bump unless very desperate. Sorry.
-
**BUMP**
-
I would say save it to NBT to be safe
@Override public void writeToNBT(NBTTagCompound tag) { tag.setInteger("rot", rot); } @Override public void readFromNBT(NBTTagCompound tag) { rot = tag.getInteger("rot"); }
-
Hello, I am trying to make colored armor but I cant get it working, even though it renders the color in the item, it does not when you wear it.
package org.zaet.api.armor; import net.minecraft.client.renderer.texture.IIconRegister; import net.minecraft.creativetab.CreativeTabs; import net.minecraft.entity.Entity; import net.minecraft.init.Blocks; import net.minecraft.item.ItemArmor; import net.minecraft.item.ItemStack; import org.zaet.api.ZaetRegistry; public class ColoredArmor extends ItemArmor { public static final int helm = 0, chest = 1, legs = 2, boots = 3; public String folder; public String armorName; public int color; public ColoredArmor(int color, String folder, String armorName, ArmorMaterial material, int render, int type, CreativeTabs tab) { super(material, render, type); this.color = color; this.folder = folder; this.armorName = armorName; setTextureName(folder + ":" + armorName + "_" + type); setUnlocalizedName(folder + "_" + armorName + "_" + type + color); setCreativeTab(tab); } @Override public void registerIcons(IIconRegister r) { this.itemIcon = r.registerIcon(folder + ":" + armorName + "_" + armorType); } @Override public String getArmorTexture(ItemStack stack, Entity entity, int slot, String type) { switch(this.armorType) { case helm: return folder + ":" + "textures/armor/" + armorName + "_1" + ".png"; case chest: return folder + ":" + "textures/armor/" + armorName + "_1" + ".png"; case legs: return folder + ":" + "textures/armor/" + armorName + "_2" + ".png"; case boots: return folder + ":" + "textures/armor/" + armorName + "_1" + ".png"; default: return folder + ":" + "textures/armor/" + armorName + "_1" + ".png"; } } @Override public boolean hasColor(ItemStack item) { return true; } @Override public int getColor(ItemStack item) { return ZaetRegistry.getColorHexCode(color); } @Override public int getColorFromItemStack(ItemStack item, int unUsed) { return getColor(null); } }
Does anyone have any ideas?
-
In your leaf constructor where you put super(id, material); put setStepSound(Block.soundTypeLeaves); it will be similar
and in your block class put
@Override public boolean isOpaqueCube() { return false; }
-
Thank. I don't know why I did not think of that
.
if((new Random()).nextInt(2500) == 0) { tattyseal.setStupid(true); }
-
So I wanted to make a new tool (not like a new Pickaxe) but, as an example a Paxel. I looked in the Pickaxe class and it seemed like it only had things like Blocks.wood and not materials and I cannot get my head around it. Does anyone know how?
[1.6.4] Another Problem... I can't take the items out of my inventory ._.
in Modder Support
Posted
Where is your GUI class file? How are we meant to help you with no class?