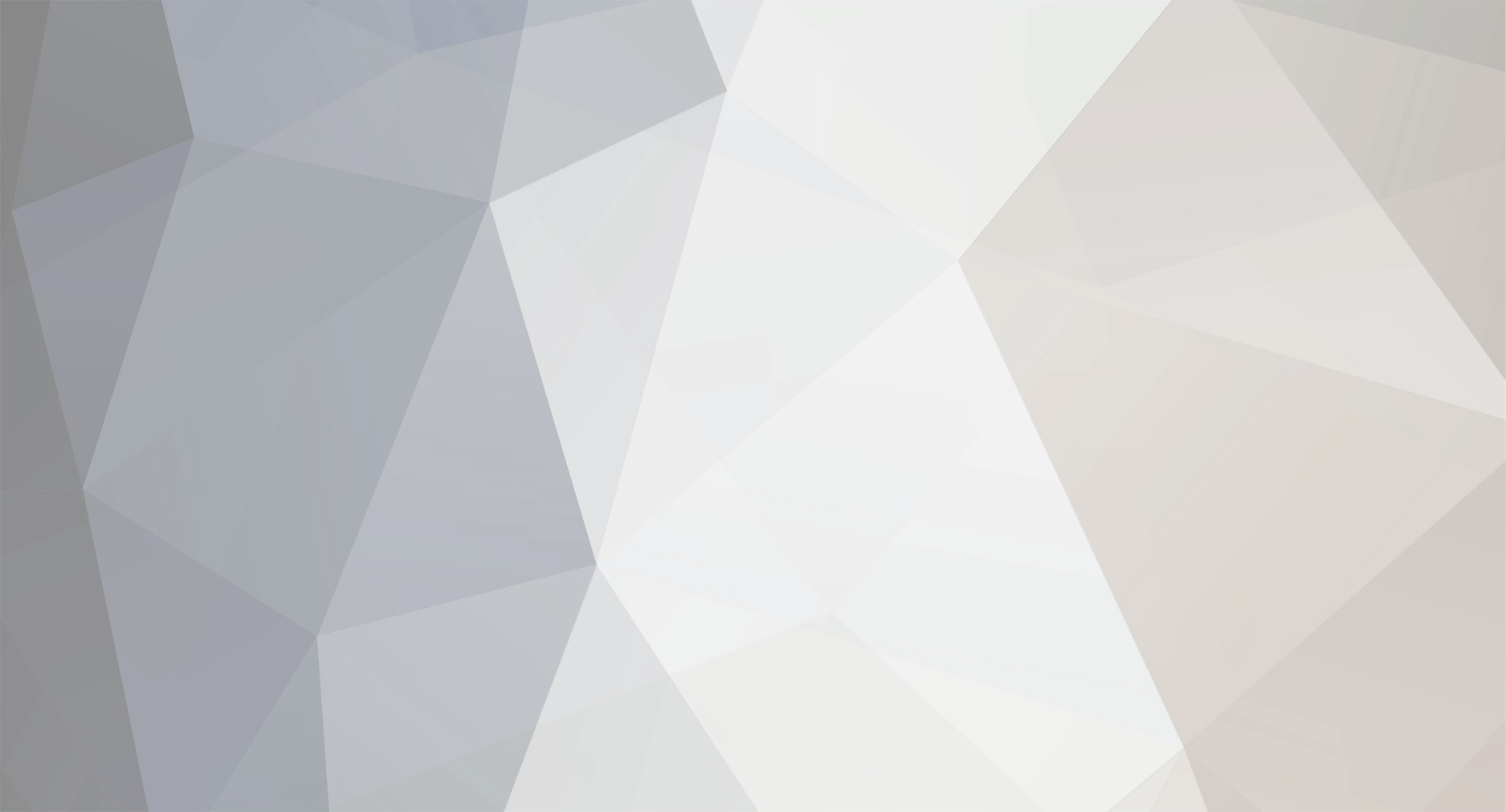
Thornack
Members-
Posts
629 -
Joined
-
Last visited
Everything posted by Thornack
-
[1.7.10] How to Change Player Model and Texture
Thornack replied to Thornack's topic in Modder Support
I have solved this problem but I do have a question. How do you access the players height and width and the bounding box and the camera zoom. I need to alter these fields to work with my model. I have been trying to figure that out for a while and cant seem to figure it out. My solution (posted below) involves cancelling the default player render and then rendering my own model. The model shows up with the correct texture. I had to change my renderer to extend RendererLivingEntity otherwise if I extend RenderLiving I got a crash Im not sure why. Anyway, this is the solution I came up with below. I do have a question as I have very little experience with changing the player - will my solution work in multiplayer to display the model to other players? Or do I have to do something special to achieve this? Currently I dont have a way to test for this multiplayer behaviour so That is why I am asking. My Renderer ChangePlayerModel Event -
[1.7.10] How to Change Player Model and Texture
Thornack replied to Thornack's topic in Modder Support
bump -
[1.7.10] How to Change Player Model and Texture
Thornack replied to Thornack's topic in Modder Support
Any help with this is greatly appreciated -
Hi Everyone, I have figured out how to change the players model using the ObfuscationReflectionHelper but i have not been able to figure out a way to change the model and texture. Using the method directly below (spoiler 1) the default player texture is overlaid onto the model you choose. Also this method is currently inefficient since I believe it is called multiple times a frame but I am not positive about this. What I want it to do is something more like cancel the player render event and then render my own model in its place using my custom renderer so that I can have custom textures. But I have run into a problem. I know how to cancel the player event but dont know how to add my own renderer in its place after cancelling so that the player model is replaced with the custom one I choose. Im not sure what the problem is since the method addCustomRenderer works perfectly when I use it in my ClientProxy to render my mobs when they are spawned in game. In my ClientProxy I use the following method(s) to register my entities and this works perfectly where my mobs show up normal and rendered correctly whether i use my custom spawning event or using a monster egg. I have also tried to call my renderer directly in place of the addCustomRenderer Method using -> RenderCustomModel() render = new RenderCustomModel(new ModelMonster(), "Monster.png", 1f); but this didnt work either. Am I missing something stupid I have been at this for a while. The ClientProxy Methods my entity renderer class (works) [note**] I removed a function that I use to render custom entity information cause I dont wish to share that and it shouldnt be relevant to my problem
-
[1.7.10] Getting Entity Data based off of look vector doesnt work
Thornack replied to Thornack's topic in Modder Support
Thanks coolAlias for the code snippet but I went my own route. I solved my problem by updating the entity by sending a packet from server to client and then getting the entity data client side only. It seems to work ok for what I needed. Although getting it server side would be ideal. For now Im just keeping it client side since Im using my item to test the stats generation that I have implemented. This is my solution (Its pretty ugly but seems to do the job) I open a gui to display the stats that my mob has by using the entityID of the custom mob that was clicked on where its ID is passed through the x coordinate. Don't really know how else to do it and any suggestions would be appreciated. public ItemStack onItemRightClick(ItemStack itemStack, World world, EntityPlayer player) { if (world.isRemote){ int maxCheckRange = 15; Vec3 vec3 = player.getLookVec().normalize(); for(int initialCheckRange = 1;initialCheckRange<maxCheckRange;initialCheckRange++){ AxisAlignedBB aabb = AxisAlignedBB.getBoundingBox(player.posX + vec3.xCoord * initialCheckRange, player.posY + vec3.yCoord * initialCheckRange, player.posZ + vec3.zCoord * initialCheckRange, player.posX + vec3.xCoord * initialCheckRange, player.posY + vec3.yCoord * initialCheckRange, player.posZ + vec3.zCoord * initialCheckRange); List list = world.getEntitiesWithinAABB(EntityMyMob.class, aabb); if(list.iterator().hasNext()){ EntityMyMob ent = (EntityMyMob)list.get(0); //Open a GuiStatsDisplay which displays info about the custom mob the player clicked on. //(kind of a hack) - the entityID of the custom mob clicked on is passed through the x coordinate. //Don't really know how else to do it. player.openGui(CustomMod.instance, PWGuiHandler.STATS_GUI_ID, world, (int)ent.getEntityId(), 0, 0); } } } return itemStack; } -
The Comment above the getWorldTime() method says ** * Get current world time */ public long getWorldTime() { return this.worldTime; } " and the comment above the worldTime says /** The current world time in ticks, ranging from 0 to 23999. */ private long worldTime; which is why I expected it to wrap from 23999 back to 0
-
I solved the issue by doing if (time >=24000){ this.worldObj.getWorldInfo().setWorldTime(0); } but it is still weird why it wont wrap on its own like the method description says it should
-
I have a tile entity that gets the world time. The method description says that this time wraps from 23999 to 0 and the then counts back to 23999. However that is not what I find when I try to use it. When I print it to consol the world time is additive past 23999 it seems forever.Any ideas why the time doesnt wrap? My Tile Entities updateEntity method in the //DO STUFF HERE section the stuff I do stops happening cause of the world time issue where the time doesnt wrap. [Edit] I solved the previous stuff that was posted below this it was a stupid error on my part
-
[1.7.10] Getting Entity Data based off of look vector doesnt work
Thornack replied to Thornack's topic in Modder Support
Ya Ive been looking, If anyone has a solution to getting entity data based on a look vector/raytrace please let me know ill keep looking and post a solution if i come across one. -
[1.7.10] Getting Entity Data based off of look vector doesnt work
Thornack replied to Thornack's topic in Modder Support
@Override public ItemStack onItemRightClick(ItemStack itemStack, World world, EntityPlayer player) { if (!world.isRemote){ MovingObjectPosition mop = this.getMovingObjectPositionFromPlayer(world, player, true); System.out.println(mop); } return itemStack; } } this outprints either null or type hit = block and never outprints type hit to be of type Entity -
[1.7.10] Getting Entity Data based off of look vector doesnt work
Thornack replied to Thornack's topic in Modder Support
I tried the following didnt work turns out raytraces are hard. Im not sure what else to try at this point I cant seem to be able to get the entity @Override public ItemStack onItemRightClick(ItemStack itemStack, World world, EntityPlayer player) { Entity Target = this.getEntityFromLookVector(world, player); System.out.println(Target); if (!world.isRemote & Target != null) { if (Target instanceof EntityMyMob) { System.out.println("getting entity "); int maxHP = ((EntityMyMob) Target).stats.hp; System.out.println("This mobs maxHP is " + maxHP); } } return itemStack; } @SideOnly(Side.CLIENT) public Entity getEntityFromLookVector (World world, EntityPlayer player){ MovingObjectPosition mop = player.rayTrace(15, 1.0f); if (mop != null) { System.out.println("mop isnt null" + mop); if (mop.typeOfHit == MovingObjectPosition.MovingObjectType.ENTITY) { System.out.println("hit ent"); return mop.entityHit; } } return null; } } this code never returned an entity as being hit. Even if I stood right next to my mob or even a cow/sheep it would either return one of the following mop isnt nullHitResult{type=BLOCK, x=206, y=3, z=888, f=1, pos=(206.5229233287801, 4.0, 888.9466416142508), entity=null} mop isnt nullHitResult{type=MISS, x=219, y=12, z=892, f=4, pos=(219.0, 12.750675644892668, 892.5001750429458), entity=null} -
[1.7.10] Getting Entity Data based off of look vector doesnt work
Thornack replied to Thornack's topic in Modder Support
WOW I feel dumb.... Thanks so much. <FacePalm> Is right -
[1.7.10] Getting Entity Data based off of look vector doesnt work
Thornack replied to Thornack's topic in Modder Support
I hope this clarifies it, The System.out.print at line 6 in the code below outprints just fine meaning the method is called on the server just fine The System.out.print at line 20 inside the for loop does not outprint when I am on the server - when I am on the client however this line DOES work. <- Really weird [Line 1] @Override [Line 2] public ItemStack onItemRightClick(ItemStack itemStack, World world, EntityPlayer player) { [Line 3] if (!world.isRemote) { [Line 4] int maxCheckRange = 15; [Line 5] Vec3 vec3 = player.getLookVec().normalize(); [Line 6] System.out.println("On Server"); // This outprints just fine meaning this method gets called on the server successfully [Line 7] for (int initialCheckRange = 1; initialCheckRange < maxCheckRange; initialCheckRange++) { [Line 8] [Line 9] AxisAlignedBB aabb = AxisAlignedBB.getBoundingBox( [Line 10] player.posX + vec3.xCoord * initialCheckRange, [Line 11] player.posY + vec3.yCoord * initialCheckRange, [Line 12] player.posZ + vec3.zCoord * initialCheckRange, [Line 13] player.posX + vec3.xCoord * initialCheckRange, [Line 14] player.posY + vec3.yCoord * initialCheckRange, [Line 15] player.posZ + vec3.zCoord * initialCheckRange); [Line 16] List list = world.getEntitiesWithinAABB(EntityMyMob.class,aabb); [Line 17] if (list.iterator().hasNext()) { [Line 18] EntityMyMob ent = (EntityMyMob) list.get(0); [Line 19] int maxHP = ent.getEntityData().getInteger("MaxHP"); [Line 20] System.out.println("This mobs maxHP is " + maxHP); // This does not out print when I am on the server But does outprint when I am on the client [Line 21] [Line 22] } [Line 23] } [Line 24] [Line 25] } [Line 26] return itemStack; [Line 27] } I have now moved on from trying the method above and have written the following one and it works correctly displaying the hp stats of each entity but I need this method to have a much greater range. Any ideas? @Override public ItemStack onItemRightClick(ItemStack itemStack, World world, EntityPlayer player) { if (!world.isRemote){ Minecraft mc = Minecraft.getMinecraft(); MovingObjectPosition objectMouseOver = mc.objectMouseOver; if(mc.objectMouseOver != null && mc.objectMouseOver.entityHit != null) { Entity Target = objectMouseOver.entityHit; if(Target instanceof EntityMyMob){ System.out.println("getting entity "); int maxHP = ((EntityMyMob) Target).stats.hp; System.out.println("This mobs maxHP is " + maxHP); } } } return itemStack; } int maxHP = ent.getEntityData().getInteger("MaxHP"); wouldnt work at all and would return 0 no matter what. My stats are being saved to NBT but apparently I cannot get them back from NBT in this way for each instance of MyMob entity in game. Dont know why I have no idea why -
[1.7.10] Getting Entity Data based off of look vector doesnt work
Thornack replied to Thornack's topic in Modder Support
I have no idea why but for some reason I cant seem to get the EntityData server side. Does anyone know why? If I change the if (world.isRemote){} to if (!world.isRemote){} then for some reason none of the code inside this if statement gets executed. -
[1.7.10] Getting Entity Data based off of look vector doesnt work
Thornack replied to Thornack's topic in Modder Support
I guess what Im trying to say is It seems my code doesnt get the entity from the look vector then I dont think, since It doesnt display anything to the consol when I try to outprint the server values for my stats -
[1.7.10] Getting Entity Data based off of look vector doesnt work
Thornack replied to Thornack's topic in Modder Support
So I should use !world.isRemote to get the entity server side then send a packet to the client to update the values? If I do !world.isRemote for some reason nothing is displayed to the consol on right click except "Right-Clicked". Shouldnt I be able to see the value of the entities stat that exists on the server if i use !world.isRemote? -
[1.7.10] Getting Entity Data based off of look vector doesnt work
Thornack replied to Thornack's topic in Modder Support
I know that the stats are being saved correctly and calculated correctly Since I have a method to check this where the item that I use to catch my mobs stores their stats and displays them and so I know the stats work correctly. I just cant seem to be able to access these stats using this new item based on a look vector and I dunno y -
I have an item that is a 3D custom rendered item. I want to give it the ability to check a mobs stats that have been saved to NBT on Right click based on the players look vector. For some reason I always get a 0 for all the stats I try to check and im not sure why. I think I am successfully getting the entity my consol prints "This mobs maxHP is 0" and it doesnt matter which stat I try to implement they are all 0. Anyone know why My on Item Right Click Method In My custom mobs class I am saving the stats in the following manner My Entity Class My Stats Container class
-
[1.7.10] Changing mob stats without using IExtendedProperties
Thornack replied to Thornack's topic in Modder Support
Thanks guys Ill try to get something together and ill post it once i have it working. -
[1.7.10] Changing mob stats without using IExtendedProperties
Thornack replied to Thornack's topic in Modder Support
Do you recommend then to create my own way to determine each of my mobs actual stats for example Spd by altering fields in the Entity class such as motionX, motionY, motionZ. Atm this value is just a calculated value that is stored in NBT data for each entity and when a player tames the mob the item used to tame the mob stores the instance of the mob and lets the player release and recapture the mob at a whim where the stats are saved preserved throughout this process. I guess my question is how would I alter the actual HP of the mob that was assigned by vanilla to equal the value that I calculated (to replace the default HP value that vanilla gives my mob (i think it is 20 but i am not sure). Currently my stats.hp doesnt link with the vanilla hp so all instances of my mob have the same hp when I hit them they die with the same number of hits and Im not sure how this works in Vanilla -
Hi everyone, I am working on a tameable mob that uses a stats container class and a stats calculation class to determine its stats. Currently I have the tameable bit down I use a custom system and the stats are generated and stored per world where each instance of my mob has a unique version of the stats due to my implementation of a "genes" factor. Given this structure, I was wondering how could I use it to alter the entities properties. If you look at the read/write EntityFromNBT methods contained in my entity class I want to use the stats that have been saved for each instance of my mob to alter the mobs movement speed, armour value, hp, exp, and also want to implement custom stuff like stamina bars for my mob (I already know how to add a stamina bar to a player using IExtendedPlayerProperties). Is there a way to use my stats system to affect the mobs "minecraft stats" so that movement speed, armour value, hp, exp etc are set to my values? To start off I am just trying to make my calculated HP be the actual mobs HP at the moment. Do I have to use IExtendedEntityProperties or is there another way I could implement this since my stats are already being saved to NBT per each instance of the mob? My Entity Class My Stats Container class
-
Hi everyone, My goal is to open a youtube video in minecraft to create a story for minecraft using cut-scenes that get triggered by events that occur in game. So far I have a GUI class and am able to open the youtube video by clicking the P key - I arbitrarily set it to this. But the way I have it figured out currently uses the following method that is called whenever the player clicks the p key In my GUI class I have the following methods for opening the web browser @Override public void handleKeyboardInput() { if (Keyboard.getEventKeyState()){ this.keyTyped(Keyboard.getEventCharacter(), Keyboard.getEventKey()); } else{ this.keyReleased(Keyboard.getEventKey(), Keyboard.getEventKey()); } this.mc.func_152348_aa(); } @Override protected void keyTyped(char key, int event){ super.keyTyped(key, event); if (key=='p'||key=='P'){ this.openWebpage(); } } public static String openWebpage() { if (Desktop.isDesktopSupported()) { try { Desktop.getDesktop().browse(new URI("http://www.Youtube.com")); } catch (IOException e) { e.printStackTrace(); return "Can't open browser for some reason"; } catch (URISyntaxException e) { e.printStackTrace(); return "Bug Detected: The URL is invalid for some reason."; } } else { return "For some reason You Can't open the browser"; } return null; } It works perfectly to open a webpage using the browser but I have a question, I want the url to open inside the GUI where currently it opens in the desktop (outside of MC). Not sure how to implement this part. I know you have to use the following two methods but I am not sure exactly how to get the last little bit so that I have a working cutscene @Override public void drawScreen(int mouseX, int mouseY, float p_73863_3_){} private void drawTexturedRect(Rectangle guiRect, Rectangle drawRect, Rectangle textureRect, int textureSize){} any help is greatly appreciated
-
[1.8] custom gui with textured buttons and scrollpanes
Thornack replied to Failender's topic in Modder Support
I know of the following bits of code that may be useful to you screen resolution int width = event.resolution.getScaledWidth(); int height = event.resolution.getScaledHeight(); Mouse clicked @Override protected void mouseClicked(int x, int y, int event){ if(event != 0 || !this.SelectList.func_148179_a(x, y, event)){ super.mouseClicked(x, y, event); } } Mouse Moved @Override protected void mouseMovedOrUp(int mouseX, int mouseY, int event){ if (event != 0 || !this.SelectList.func_148181_b(mouseX, mouseY, event)){ super.mouseMovedOrUp(mouseX, mouseY, event); } } -
Hi everyone I have made a stamina bar that regenerates every tick. But when I change its max value from 100 to 300 lets say the bar displays the 300 as the max value but stops refilling once it reaches 100. anyone know why? This is the code for the Extended Player Class, PacketSyncPlayerStamina class, and the Stamina GUI class. Note in the Extended Player Properties class I want the maxStamina variable to change while the player is logged in based on some condition (I am implementing a level up system which I want to use to change the value of maxStamina so that the player gets more stamina the higher their level.) currently the max stamina value changes but the current stamina value does not recognize that it changed unless you create a new world. - Anyone know why?? The Extended Player class where If I change the maxStamina variable the max value updates but the current value does not PacketSyncPlayerStamina The GUI for the stamina Bar where I cancel the food bar event only and then replace it with my stamina bar I use the PlayerTickEvent to increment the stamina bar every x number of ticks to allow stamina regen let me know if this is necessary to solve the issue but I doubt it I think it has to do with DataWatchers/packets and my limited understanding with how they work
-
[1.7.10]How would I check if the players inventory is open?
Thornack replied to starwarsmace's topic in Modder Support
try this it happens the first time but after that it doesnt work... move the items around remove them from the hud it isnt that simple. @SubscribeEvent public void onPlayerJoin(PlayerLoggedInEvent event) { System.out.println("This is called Log In"); if(!event.player.worldObj.isRemote && !event.player.capabilities.isCreativeMode){ System.out.println("on server side and not in creative"); if(event.player.inventory.mainInventory[0] == null){ System.out.println("Adding itemCustom1"); event.player.inventory.addItemStackToInventory(new ItemStack(CommonProxy.itemCustom1)); } if(event.player.inventory.mainInventory[1] == null){ System.out.println("Adding itemCustom2"); event.player.inventory.addItemStackToInventory(new ItemStack(CommonProxy.itemCustom2)); } if(event.player.inventory.mainInventory[2] == null){ System.out.println("Adding itemCustom3"); event.player.inventory.addItemStackToInventory(new ItemStack(CommonProxy.itemCustom3)); } } } [EDIT] the weird thing is if you move the item -> itemCustom3 lets say to a different slot lets say slot 5 and then relog. Now slot 3 is empty and so you should get itemCustom 3 placed into slot 3 but you dont you get it in slot 5 now. If you throw the item away then it works again upon relog you get item 3 in slot 3.