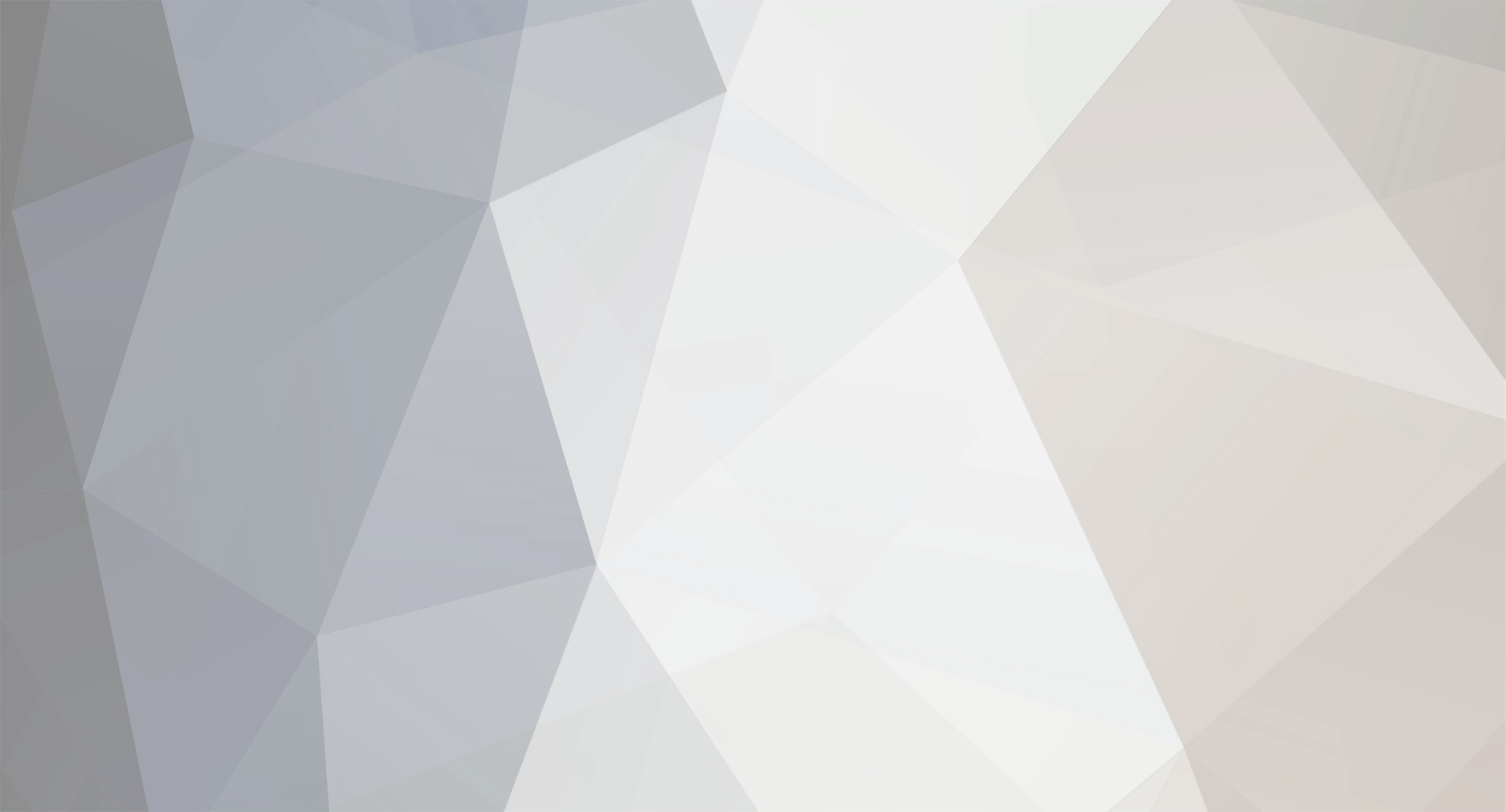
coolAlias
Members-
Posts
2805 -
Joined
-
Last visited
Everything posted by coolAlias
-
It should work just fine from onUpdate. Did you call 'super.onUpdate' as well? You should. Here's some code I have for a projectile Entity that spawns particles in either single- or multi-player just fine. You really only need to spawn on the client side, like Goto mentioned, but I didn't check for that in the code here. @Override public void onUpdate() { super.onUpdate(); if (!this.isDead && !this.inGround) { for (int k = 0; k < 4; ++k) { float f4 = 0.25F; this.worldObj.spawnParticle("crit", this.posX - this.motionX * (double)f4, this.posY - this.motionY * (double)f4, this.posZ - this.motionZ * (double)f4, this.motionX, this.motionY, this.motionZ); } } }
-
[SOLVED] Read/Write Compressed NBT from/to DataStream
coolAlias replied to coolAlias's topic in Modder Support
Should read/writeCompressed work for any format of NBTTagCompound? One would think so, and I haven't done anything weird with mine, so far as I know. It's all pretty basic stuff in the Inventory and ExtendedPlayer classes. Like I mentioned earlier, I got it working by writing each ItemStack to the outputStream, but I'm curious why the NBT method didn't work. I make the call to 'sync' onEntityJoinWorld and the packet is handled like I showed earlier with the compressed stream tools. I've checked and everything is getting called properly, it's just the NBT read/write that was messed up. Code: -
Can I use Entity.isCollidedHorizontally server side?
coolAlias replied to coolAlias's topic in Modder Support
I have yet to sort this one out, if anyone has suggestions. Cheers. -
itemMaterial doesn't exist, unless you made a new class. Did you mean EnumToolMaterial? If so, that doesn't store a damage value, though it does have 'maxUses'. Your 'Items.materialOne' is just another Item, by the looks of it. No different than using 'energizedDust' as far as your furnace is concerned. What exactly are you trying to accomplish? Do you want to damage the fuel itemstack? Why? You are already returning a burn time, just consume the item and let the furnace burn. You can make it so the furnace doesn't use power if there is no item inside to smelt if that's what you want. If you really want to have 'damaged' fuel stacks, like a half-used battery, you could return a damaged itemstack from the 'getStackInSlot' and 'getStackInSlotOnClosing' methods, but keep in mind damageable items cannot be stacked.
-
[SOLVED] Read/Write Compressed NBT from/to DataStream
coolAlias replied to coolAlias's topic in Modder Support
EDIT: Ok, sorry I'm a bit slow... I don't know what I was thinking trying to send the entire inventory in a single NBT compound <facepalm>. I used Packet.write/readItemStack, iterating through the inventory, and it works great. Wow... thank you guys for your patience. I'm sure it must be tough sometimes Thanks for the ideas. I tried using read/writeCompressed instead, but it has the same result. The NBT tag is correct when preparing the packet, but either during the writing or reading process it gets translated into null. It shouldn't make a difference, but the NBT Tag is for an IInventory storing some ItemStacks. Actually, I think it does. I just tried storing another variable directly into the tag compound and it was sent and received correctly, but the items stored in a tag list appended to the compound don't get written to the packet. Sending the tag list compound shows the tag list correctly, but it doesn't contain any tags. So it looks like I have to manually go through and write/read every individual tag compound? There has to be a better way than that, right? The Packet methods were where I found the code with the byte array from earlier. I didn't use the actual methods because I didn't make a subclass of Packet, just a static method to build the packet as a customPayload250, and writeNBTTagCompound is protected. Code with read/writeCompressed instead: -
Then the problem may be that the client doesn't know your minecart has fuel, so always returns false. When you add fuel, does the client know about it? Things that work in single player easily break in multi-player due to these kinds of problems.
-
SOLUTION: Don't try to send the entire inventory as NBT. Just iterate through the contents and use Packet.writeItemStack to write each itemstack to the stream. I'm trying to synchronize an inventory stored in IExtendedEntityProperties on loading so as to display something in the HUD. It works fine as soon as I open the inventory at least once, but before that, all the client-side data is null. I tried sending a compressed version of the NBT from server to client, much like the TileEntity packet, and printing parNBTTagCompound.toString() shows it has all the correct information before being sent. However, once I send and receive the data, there's nothing there. Is this one of those cases where you can't use the assignment operator '=' ? Or is there something else going on here? Code:
-
Where do you register/input Throwable Entity's texture?
coolAlias replied to SevenDeadly's topic in Modder Support
EDIT: Goto was right, of course. I fired up the Eclipse server and tried my custom entity there, didn't render. Moving 'registerModEntity' to the load method of my main mod class resolved the issue. Too bad that's not mentioned in the Forge wiki tutorial, unless there's another one that deals with registering entities besides the SpaceMarine blasterRifle one. If there is, it's not easy to find. Thanks for clearing that up Goto. I've been looking through your mods on github and I can't seem to find where you register your entities... I only see lines like the following: RenderingRegistry.registerEntityRenderingHandler(EntitySteamBoat.class, new RenderSteamBoat()); I know that's in ClientProxy only, but do you register the EntitySteamBoat? Where? I don't see it in CommonProxy or the main mod class. Thanks again. It's nice to have someone who really understands the code - like I said, the method I used above seems to work fine, and that's the way it says to do it in the Blaster Rifle tutorial on the wiki, but what you say makes sense about needing to register on both sides. -
Will do. Thanks for the clear answer!
-
Is there a difference between using entityClientPlayerMP.sendQueue.addToSendQueue(packet) and PacketDispatcher.sendPacketToServer(Packet packet)? The wiki tutorial says they can both be used, but what I'm worried about is using the latter method, is the Player parameter in onPacketData necessarily the same as the one that sent it? I'm guessing it must be, as it should know which client the packet came from and create the parameter accordingly.
-
If you have the time, I highly recommend you check out the lectures below on 'Climbing the Interface Ladder' - it covers everything you will need to know to get the mouse click in inventory and then some. http://courses.vswe.se/?course=4
-
Where do you register/input Throwable Entity's texture?
coolAlias replied to SevenDeadly's topic in Modder Support
You mentioned this is in your CommonProxy? I think you should only register entities in ClientProxy, which could be the cause of your null network mod handler. At least, I only register mine in ClientProxy and it works for me on the Eclipse server. I can't claim any expertise in this particular area other than that. EDIT: This is wrong. Entities should be registered on both sides, so do it from main mod load method or somewhere similar. Thanks Goto again for the correction. -
DataWatcher.addObject: init vs. constructor
coolAlias replied to coolAlias's topic in Modder Support
Awesome, thanks! Just wanted to make sure I wasn't missing something really obvious -
I've noticed that data watcher objects are usually if not always added in init methods, such as EntityInit, in vanilla code. Is there a specific reason this is the case, or could it just as well be added in the entity constructor? In my specific case, I add a watchable in a class that extends IExtendedEntityProperties. It seems to work fine in the constructor, but I could just as easily move it to the init method as well, if need be. Just curious if it makes a difference. Thanks!
-
One way I've seen and used is to store the IExtendedEntityProperty data temporarily outside of the player when the player dies, then reload it when the player rejoins. There is also PERSISTED_NBT_TAG (I think that's what it's called, I don't have Eclipse handy at the moment) in EntityPlayer, but I haven't been able to use that successfully yet. Check here for a working example: http://www.minecraftforum.net/topic/1952901-eventhandler-and-iextendedentityproperties/#entry24051513
-
Can I use Entity.isCollidedHorizontally server side?
coolAlias replied to coolAlias's topic in Modder Support
It's true when pressing forward into a block, even when going down, but NOT falling, you are right. That's what is weird. If I go up like a ladder and come down like a ladder, I still take damage even though I technically wasn't falling, except that 'isOnLadder' is not true, since I'm not on a ladder. That's why I was trying to use onCollidedHorizontally, as it is still true coming down or I wouldn't be able to come down using the sneak key, I would just fall. So the problem is, even though onCollidedHorizontally is true client side, allowing me to move correctly, server side it is NOT true, so it thinks I'm falling and increments fallDistance regardless of whether I'm actually falling or not, because I have downward motion and the server doesn't know about onCollidedHorizontally, from what I can tell. -
I don't think that annotation means what you think it means. --Inigo Montoya (Hint: That particular annotation only has an effect inside your IDE to trigger additional error checking; leaving it off has no effect once the program is compiled) Adding it helped him figure out he had the incorrect method signature. I'd say that's a pretty useful purpose even if at compile time it doesn't change the outcome.
-
Getting EntityPlayer parameter outside of the method itself
coolAlias replied to Flenix's topic in Modder Support
@diesieben07 - That's what I meant to write... (EntityClientPlayerMP, that is) Didn't know about using Minecraft.getMinecraft().thePlayer, though, I've always used the FMLClientHandler for some reason. -
Getting EntityPlayer parameter outside of the method itself
coolAlias replied to Flenix's topic in Modder Support
You can use 'FMLClientHandler.instance().getClient().thePlayer;' to get an EntityPlayerMP instance of the player using the Gui, but remember that Gui and EntityPlayerMP are all client-side. Anything you do to the player here will not be stored on the server. Your best bet would be to use the above to get the player in order to send a packet to that player on the server, then do whatever you need to do there. Your PacketHandler class should already have the player object available for you. -
Looking for GUI tutorials - not ItemContainer
coolAlias replied to Lycanus Darkbinder's topic in Modder Support
I'll put this in a spoiler so we can all move on: So anyway, how is your Gui coming along? I know this has been mentioned, but GuiEditSign and TileEntitySign should both contain loads of useful information for you, as that's an example of a non-Container-based Gui that edits a text field. -
Where do you register/input Throwable Entity's texture?
coolAlias replied to SevenDeadly's topic in Modder Support
That depends on how you set the texture before. I always used an Item for the projectile and just used that Item texture for the Entity. For example, if I have a cannon I'll make an Item cannonBall. You can either register the texture in the class with registerIcons or, if you don't want to make a class, use setTextureName("texture_name") - that's the updated name for func_111somethingsometing_d from before, so you still need to prefix the texture name with your mod id + ":" When you register your throwable entity renderer, if it's set up similar to RenderSnowball, you just pass in the Item and it will use the correct texture. -
Can I use Entity.isCollidedHorizontally server side?
coolAlias replied to coolAlias's topic in Modder Support
It's true when going up/down because the player is still moving forward, just like the way ladders/vines work. I'm still not sure why it's only true client side. This has the unfortunate side-effect of forcing me to update the server with client information, which I don't want to do if I can help it. Any ideas how to avoid that? -
GotoLink's absolutely correct. Just do what he says and you'll be fine Check the Forge Wiki for a generic tutorial on Gui Overlay: http://www.minecraftforge.net/wiki/Gui_Overlay It's quite good and deals with getting images to display on the screen, rather than just text, which should be enough to get you started.
-
Try this tutorial: http://www.minecraftforum.net/topic/1952901-eventhandler-and-iextendedentityproperties/#entry24051513