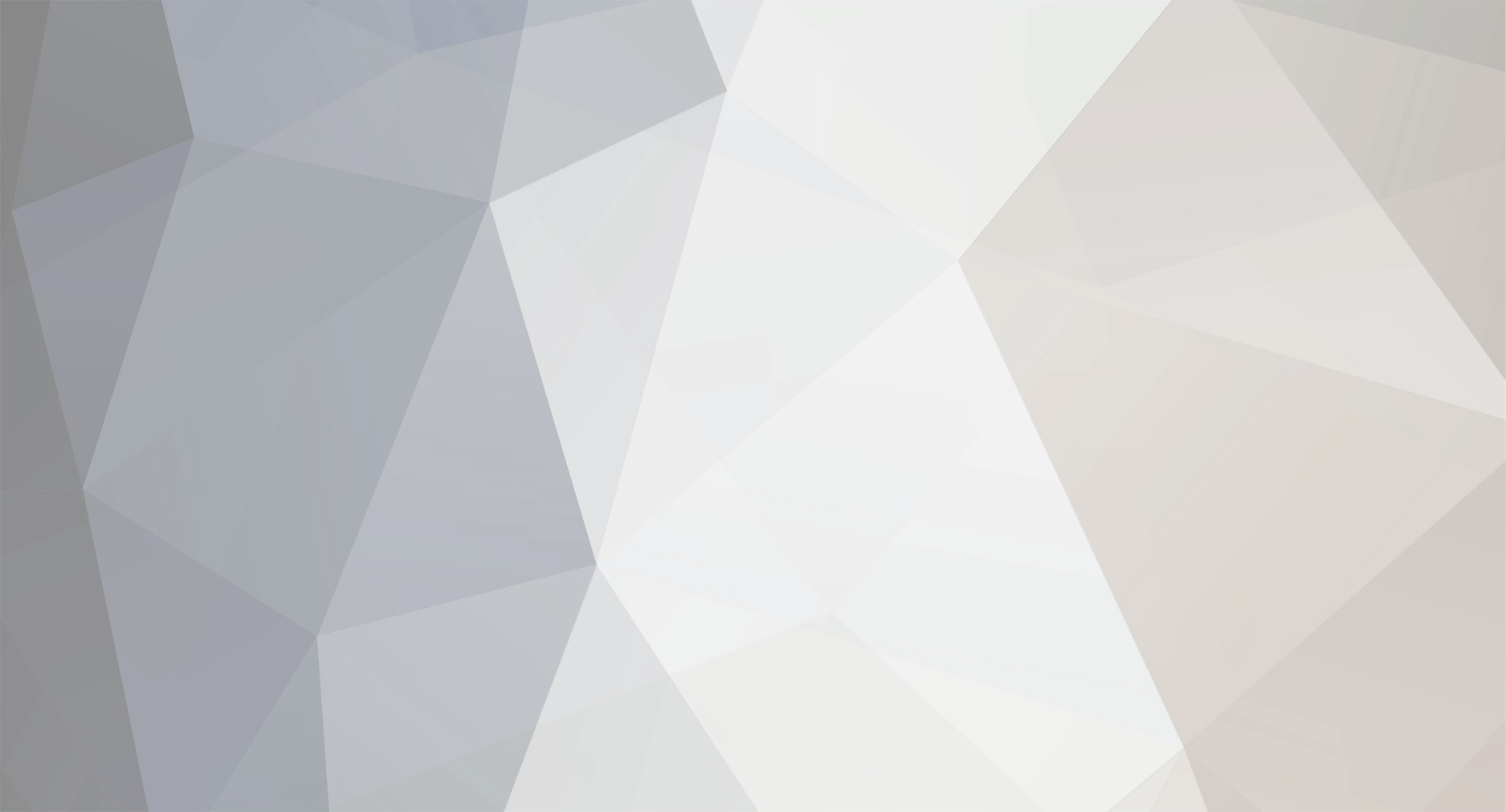
coolAlias
Members-
Posts
2805 -
Joined
-
Last visited
Everything posted by coolAlias
-
So create a custom output slot with this method: @Override public int getSlotStackLimit() { return 1; } When adding slots to your Container, be sure to use your custom slot only for your output. Look through the Slot class to see the other methods mentioned by MineMaarten - you will need them to prevent the player from putting things into the slot. From your recipe code, it didn't look random at all. It's still a recipe requiring two specific items to return a specific output... I'd suggest you take a good look at the vanilla FurnaceRecipes class. Do you see where it returns the smelting result? Well, instead of returning a specific ItemStack, store a custom int or whatever in the HashMap that tells you what random list to pull the item from: // I'll modify the vanilla for 2 itemstacks public ItemStack getSmeltingResult(ItemStack item1, ItemStack item2) { if (item1 == null || item2 == null) { return null; } int randomList = -1; if (metaSmeltingList.containsKey(Arrays.asList(item1.itemID, item1.getItemDamage(), item2.itemID, item2.getItemDamage())) randomList = metaSmeltingList.get(Arrays.asList(item1.itemID, item1.getItemDamage(), item2.itemID, item2.getItemDamage())); ItemStack output = null; switch(randomList) { case 1: // you put in an arrow and a stick or whatever // output = select from random list one containing gold, cobblestone, etc. case 2: // you put in dirt and gunpowder or whatever // output = select from random list two default: // for value of -1 or other unhandled cases return output; } } Something of that nature should work.
-
Find a way to store information about the player's state (afraid or not) <I'd use IExtendedEntityProperties>, then if the player is afraid, for the duration of the effect every update tick move the player in a random direction. The player may still have a little control, but it probably won't feel like it. I'm sure there's a way to intercept the player's movement commands as well and completely remove player control, but I'm not sure how to do that off the top of my head.
-
He's asking how to limit the stack size to 1, so it wouldn't be stackable in the slot, while vanilla stacking behavior would still be in effect in the normal player inventory. Since it's a furnace, you should have a TileEntity with this method in it already (assuming you implemented ISidedInventory, which you should have if you're making a furnace): @Override public int getInventoryStackLimit() { return 64; } Do I need to say more? For the random output, how are you storing your recipes? Does it matter what the inputs are at all, or should it be totally random? If it's totally random, then one option would be to make a List of ItemStacks and use list.get(rand.nextInt(list.size())) to return a random stack from within the list.
-
Is it possible your TickHandler is being called on more than just one type of tick? For instance, both SERVER and PLAYER? According to Forge documentation, PLAYER ticks occur on both the client and the server, which would explain why it's called twice (once on the server tick and once on the player server-side tick).
-
You put a space when you registered the mob: EntityRegistry.registerModEntity(EntityBlueChicken.class,"Blue Chicken", Try "BlueChicken" instead.
-
If you use attackEntityAsMob as Draco suggested, you can inflict damage directly to the entity attacked by using the attackEntityFrom method with no need for using attributes. public boolean attackEntityAsMob(Entity par1Entity) { // This will inflice 3 damage as a mob to the entity struck: par1Entity.attackEntityFrom(DamageSource.causeMobDamage(this), 3.0F); this.setLastAttacker(par1Entity); return false; } If you really want to use the attribute system, you will have to add the correct attribute to the attribute map before you can set it, if it has not been added already by a super class.
-
Your SpiritBase class requires a fully initialized EntityPlayer, but you are trying to access it from within the IExtendedEntityProperties constructor which is called during Entity construction, got it? You can't do that. Also, what are you thinking trying to access your extended properties from within SpiritBase, which is needed to initialize your extended properties? Get rid of all of that stuff and come up with a better way to initialize your variables. IExtendedEntityProperties already has an entity, in this case an EntityPlayer, and you're in the class already, so why not initialize them there? At the very least, make your SpiritBase class not require an EntityPlayer (or entity of any sort) and just make it a class that stores and handles things to do with mana.
-
[1.6] Help adding a potion effect when my item is held
coolAlias replied to Seroe's topic in Modder Support
I don't know where you came up with that onUpdate method, but the correct signature only has 5 parameters: public void onUpdate(ItemStack par1ItemStack, World par2World, Entity par3Entity, int par4, boolean par5) {} With the incorrect signature, this method will never get called unless you call it yourself. Adding '@Override' above methods such as this will help prevent you from making simple mistakes in the future. par5 is true if the itemstack is currently being used, so you could just check 'if (par5) add potion effect' -
[Partially Solved] HELP: Strange PlayerEvent Phenomenon
coolAlias replied to MrArcane111's topic in Modder Support
That's because you are only adding motionY if motionY is already greater than zero, which it will never be because the LivingJumpEvent is called when the player jumps (i.e. presses the space bar) but before any upward motion is applied. Removing that if statement should fix your problem. -
That's pretty cool - I was trying to figure out how to get the loaded mods I know this isn't quite what you're looking for, but the way I get around creating variables for every single block is to create a single int modBlockIndex and increment it each time I initialize a Block: private static int modBlockIndex; public static Block yourBlock1, yourBlock2, etc; // pre-init: get modBlockIndex start from config // init: yourBlock1 = new Block(modBlockIndex++); yourBlock2 = new Block(modBlockIndex++); etc. This way your ids are still configurable as a group, but saves the hassle of all those extra variables. I do the same for items.
-
[1.6.4] Ore Generation in the Nether and the End [Solved]
coolAlias replied to ItsTheRuski's topic in Modder Support
That's because you are using WorldGenMinable without specifying what type of block to replace. There is a three-parameter version of the constructor, the 3rd parameter of which is the block to replace; without that, it defaults to the stone block, of which there is none in the Nether. No blocks to replace = no custom blocks. -
Custom Mob attacks other Custom Mob [Solved]
coolAlias replied to SilasOtoko's topic in Modder Support
This is directly from EntityLiving. Override it in your class and add or substitute your class into the return equation. public boolean canAttackClass(Class par1Class) { return EntityCreeper.class != par1Class && EntityGhast.class != par1Class; } -
Custom Mob attacks other Custom Mob [Solved]
coolAlias replied to SilasOtoko's topic in Modder Support
Perhaps you didn't understand my earlier post. By overriding canAttackClass, you can check if the target is an instance of your entity and return false. You don't even need the IMob.mobSelector parameter, just use EntityMob.class as the target. -
That is absolutely amazing - Mojang should hire you to handle their lighting code!
-
Custom Mob attacks other Custom Mob [Solved]
coolAlias replied to SilasOtoko's topic in Modder Support
Simply add a target task to attack the nearest EntityMob. You'll have to override the canAttackClass method in your Entity if you want it to attack creepers and ghasts. this.targetTasks.addTask(4, new EntityAINearestAttackableTarget(this, EntityMob.class, 0, true)); -
Looks like 'SpiritBase' is your mod? Why are you using your mod instance to getMana()? Shouldn't mana be from each instance of an EntityPlayer or some other Entity, rather than what looks like a static method with no parameters? Additionally, it looks like you're trying to make a call to getMana() in the constructor for the IExtendedEntityProperties, which is called while the parent entity is still loading - like GotoLink said, whatever player object you get at this point will not work.
-
Use LivingJumpEvent: @ForgeSubscribe public void onLivingJumpEvent(LivingJumpEvent event) { if (event.entity instanceof EntityPlayer && wearing your armor) { event.entity.motionY += 0.2D; // or whatever value you want } } If you don't want to use events, you could try using the Forge method onArmorTickUpdate (in the Item class) and change the player's jump movement factor. I haven't tried that personally, though.
-
Static means that there is only one instance of a variable for all members of a class, so if block coordinates were static, all blocks would have the same coordinates. If a member variable is not static, that means you need an instance of the class to access the variable. In other words, the object has to exist in order for you to access its data; in this case, you need an existing TileEntity object in order to access its x/y/z coordinates, because if it doesn't exist, how could it have a position? In a nutshell, this is not a case in which you want to use static objects, and Mazetar's suggestion is your best bet.
-
Whenever one of these items is added to a player's inventory, store its UUID in a Map in IExtendedEntityProperties or someplace. Then, either find a way to inject code (ASM?) or edit base classes so that the InventoryPlayer checks for your Item onInventoryChanged, decrStackSize etc. and before setting the itemstack to null, remove its UUID from the map. That's the only way I can think of that wouldn't require doing lots of checks every tick.
-
For structures such as villages and such, whenever one generates the chunk coordinates are stored in a map in MapGenStructure: if (this.canSpawnStructureAtCoords(par2, par3)) { StructureStart structurestart = this.getStructureStart(par2, par3); this.structureMap.put(Long.valueOf(ChunkCoordIntPair.chunkXZ2Int(par2, par3)), structurestart); this.func_143026_a(par2, par3, structurestart); } This way the generator can check 'structureMap' to see if the coordinates currently generating already have a structure and, if so, skip it. I'm sure you could do something similar for your scattered feature.
-
TileEntitySign is the name of the vanilla class. Try changing your custom sign to a different name.
-
If that code didn't work, then the problem lies elsewhere, which you've suggested yourself in that the method isn't being called client side. How and where do you register your new entity? That's my guess as to the source of the problem. Check http://www.minecraftforge.net/forum/index.php/topic,12577.0.html for how to properly do so.
-
Custom furnace that uses longer time when... [solved]
coolAlias replied to AppliedOnce's topic in Modder Support
Just make a method that returns the time required to 'cook' and replace all instances of '400' with that method. public static int getTimeRequired(ItemStack stack) { if (stack.itemID == Item.diamond.itemID) return 800; else return 400; } Something like that. -
[SOLVED] Read/Write Compressed NBT from/to DataStream
coolAlias replied to coolAlias's topic in Modder Support
Ah, it's left over from the wiki Packet Handling tutorial Didn't know it was any more costly to use than worldObj.isRemote. Anyway, yes, my packet handler uses ids, I write a byte to the beginning of every custom payload packet that is the packet id, I just left that part out of the earlier code that used compressed stream tools because the problem wasn't sending/receiving the packet. That was working fine. The problem was that using the code from earlier took my inventory nbt tag with stuff in it and wrote or read it as null. I mentioned several of the steps I took to debug, including writing other things to the nbt tag that were successful, so the packets and handling are fine. For example, I could use 'compound.setInteger("Tag1", 5)' in the inventory writeNBT method and it would be handled fine by the packet, but adding a TagList to 'compound' didn't work. I forget what I did, but at one point I got the name of the TagList to process correctly, but the list was empty on the receiving end (but not on the sending end). The Inventory loads and saves fine, which suggests the NBT is all in proper order. It seems like writeCompressed only writes data directly in the tag compound, but not any other tags stored within the tag. That seems dead wrong to me, but from what I've tried that's how it appears to be.