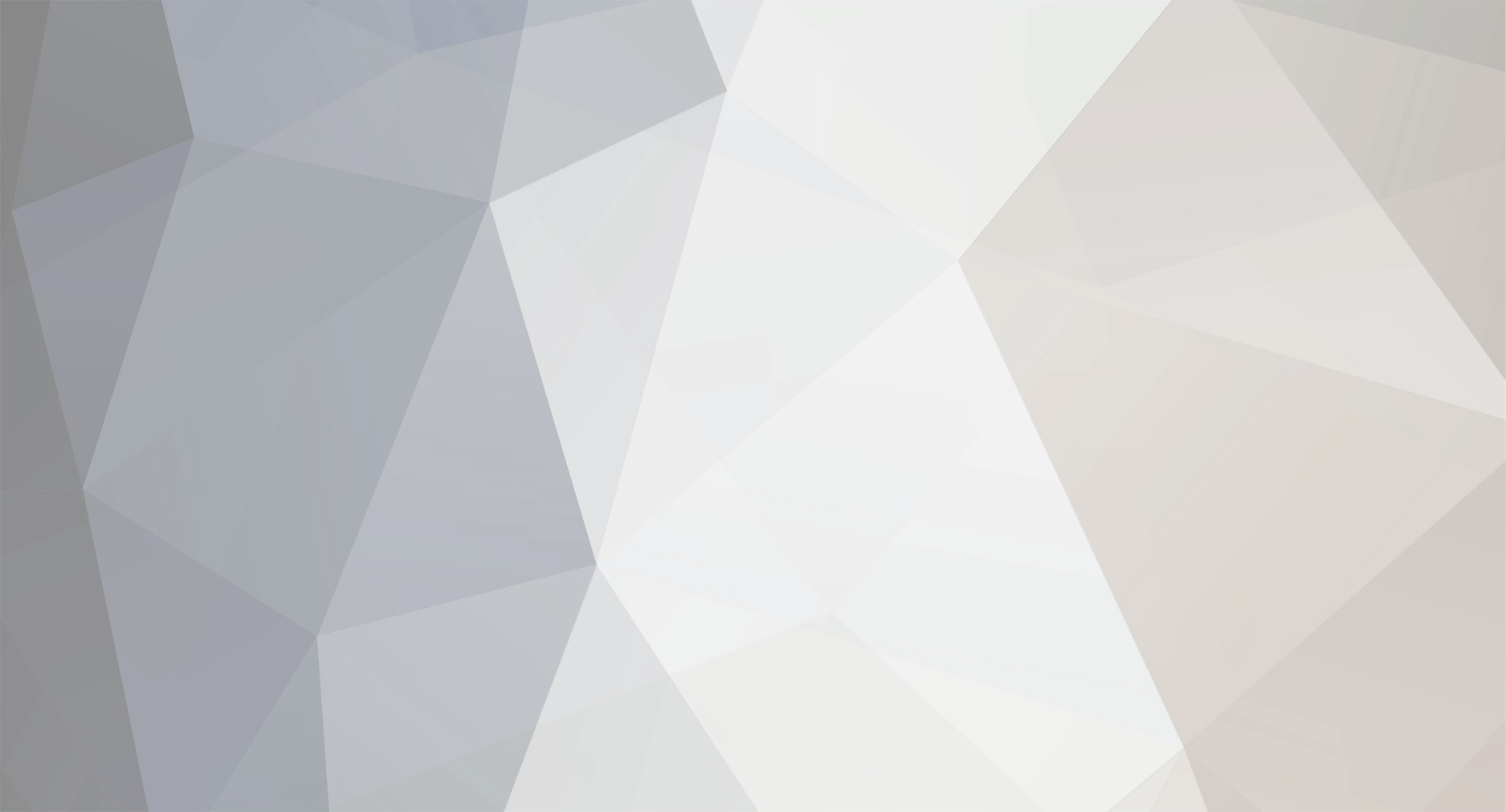
coolAlias
-
Posts
2805 -
Joined
-
Last visited
Posts posted by coolAlias
-
-
The PacketPipeline has long been deprecated in favor of SimpleNetworkWrapper and IMessage - you should really switch.
Also, you don't need to instantiate your mod instance, i.e. '= new ModMain()', as that is done automatically when your mod is loaded.
Everything else looks good, though, from what I can tell, except you didn't show your packet class which presumably is what actually makes the call to open the GUI. Are you sure your packet is working properly? You need to show us that code.
EDIT: Ninja'd by diesieben again...
-
1. Don't make your method static unless you pass either your Entity or the World object to it
2. Use one of the World#getClosestPlayer methods to find the closest player
-
-
That means you did not correctly implement #transferStackInSlot. Perhaps this explanation will help you, specifically the very last spoiler.
-
You've got it backwards - you can't run CLIENT code multiplayer because it will crash the server, so your mod, as is, will crash the server if you run it.
Unless of course you are trying to make a client-side only mod, which you didn't say that you were; in that case, then yes, you wouldn't be able to run any server side code.
But generally, when someone refers to a mod without specifying, that mod will be running on both the client and the server, and you should therefore always prefer to use the server's data over the client's.
-
This works perfectly on singleplayer and multiplayer, except when the server uses packets to fake mobs.
What? The server sends a packet to spawn the mob client-side so the client can render it, so really the client-side mob is the fake one.
Looks like you are running your code all client-side (Minecraft.getMinecraft() is client-side only), but you should be using the server version of the entity list - you can send chat messages from the server side just fine.
-
There is the Gui Open event or some such thing that would be much more effective than a tick handler, just keep in mind that there are plenty of mods that are doing that exact same thing...
Personally, I would suggest having an 'alternate' inventory menu the player can open to access their additional slots, but if you don't anticipate your mod being played alongside any others that try to replace the player inventory, then go for it.
Another alternative is to look into adding a button / tab to the player inventory screen to access your special slots; mods like Battlegear2 and several others take this route, though I haven't looked into how it's done.
-
You need to tell the config to save, i.e. 'config.save();'.
And why would you need an else / else if? What are you going to do if the recipe is not enabled, other than not adding it? You already only add it if it is enabled, so......
-
This line:
if(disableRFtLrecipe) { add recipe... wait, what?! read what the condition says... }
You say "set to true to disable the recipe", but then if it's true, you add the recipe... Either re-write your config option to say "set to true to ENABLE the recipe" and rename your variable to 'enableRFtLrecipe', or invert the condition using the '!' (called 'NOT') operator.
Also, as larsgerrits mentioned, either remove the the ConfigHandler class and read in your config from preInit, or remove the config-reading code you have in preInit and replace it with a call to initialize the ConfigHandler.
-
Registering it later shouldn't make any difference - you can generally register your Forge / FML event handlers at any time during the entire initialization process, as most of them aren't fired until after that has completed.
I'm guessing your issue is that you are not adjusting the Y position at all when trying to spawn, so the entities may be trying to spawn in the ground or in the air - try using getWorldHeight(x, z) + 1 as the y position.
Also, to be absolutely certain that your event is getting called properly, look in your console log - based on the code you posted, you should see "Spawn Watch" basically spammed all over in it; if not, then your event handler class is somehow set up incorrectly.
-
Try calling super.breakBlock from within #breakBlock.
-
Hm, actually, that method only exists in 1.8, but pre-1.8, the Block method #onBlockPlacedBy will still be called when the block is placed by a player and has an ItemStack parameter, so if you write the TileEntity to NBT when the block is broken by a player and store that tag in the ItemStack, you can restore the TileEntity to its previous state using that tag.
In pseudo-code with possibly made-up method names (they should be close to real ones, but I didn't type this in Eclipse or anything, so...):
// if #breakBlock is called before this, the TileEntity will have been removed, so you'll have to spawn the EntityItem from there instead // and then don't drop anything from here #dropBlockAsItem { TileEntity te = world.getTileEntity(x, y, z); NBTTagCompound nbt = new NBTTagCompound(); te.writeToNBT(nbt); parItemStack.setTagCompound(nbt); // this will store the TileEntity data in the ItemStack tag compound } #onBlockPlacedBy { TileEntity te = world.getTileEntity(x, y, z); // TileEntity should already exist since the block has already been placed te.readFromNBT(parItemStack.getTagCompound()); // now just read the data back in and you're done }
-
I have seen this done before, but rather than destroying the block, the player right clicked it instead. and then the backpack "block" would disappear and appear in the players inventory.
Oh yeah, you could do it that way, too. ItemBlock calls a method to effectively recreate the TileEntity from the ItemStack NBT when placed, so as long as you got it in there correctly, then that would obviate the need for IEEP to store the inventory and you'd be able to store as many as you wanted. So many options!
-
Why a Block and not an Item?
If you must use a Block, then you will need to make it like the Ender Chest and store the inventory not in the block, but in the player's IExtendedEntityProperties. You won't need a TileEntity, then, either, as you can create the GUI / Container directly from the IEEP when clicking on the Block.
If you want to make it so the player can have multiple backpacks, however, you need to store the inventory in the ItemStack NBT.
-
Once you have the direct target vector, you can find the difference between the current trajectory and the target vector and apply a portion of that to the current trajectory, e.g.:
double arcRate = 0.25D; double dx = current.xCoord - target.xCoord; current.xCoord += dx * arcRate;
Something like that anyway - I'm just typing off the top of my head here.
-
Then you will need IExtendedEntityProperties to store the friends list, at least.
There should be lots of tutorials on opening GUIs, and you will need to send packets as well to inform the server of anything that changes in the client-side gui (e.g. if you add a friend, the server needs to know about it so it can be saved to the player's file later via IExtendedEntityProperties).
Have you ever made a mod before? While not really that complicated, it could be pretty challenging if you don't know anything about modding / Java.
-
You need to provide the crash report.
Aside from that, you have some minor issues in your code:
You don't need to check if the world is remote when you are using the ClientTickEvent - it's already guaranteed to be client side.
You should compare the type of hit with a type of hit, not using the ordinal:
// No mc.getMinecraft().objectMouseOver.typeOfHit.ordinal() == 1 // Yes mc.getMinecraft().objectMouseOver.typeOfHit == MovingObjectPosition.MovingObjectType.ENTITY
You also have an unnecessary if statement:
if(mc.getMinecraft().objectMouseOver == null) { //Do nothing extra } else { // mouse over cannot be null here, because this is the else statement for the previous null check if(mc.getMinecraft().objectMouseOver != null) { // unnecessary...
[/code]
-
NBT is used to store data in all sorts of places, not just TileEntities.
It sounds like what you want is going to require IExtendededEntityProperties - it lets you store additional data (which gets saved to NBT, though you shouldn't store it in NBT for general use) per player or other entity, so if you want to add extra data to your character, that's the way to do it.
-
Or you could just check for null in the code you have... I don't think calling Minecraft's clickMouse() function constantly would be a good idea.
-
The object mouse over can be null, e.g. when looking into the sky.
-
Just use mc.objectMouseOver to get the entity - you're client side already anyway, and ray tracing is only used to find block collisions. If you look in EntityRenderer#getMouseOver, you'll see how it's done, but you don't need to copy that code when you can already use the result of it directly.
-
As long as you have both the player (which, being client-side, you can get from the Minecraft class) and the target entity, you can perform the same action as left-click using the player controller:
Minecraft.getMinecraft().playerController.attackEntity(player, targetEntity);
Note that it will still check distance server-side, so the player must be close enough to hit the mob.
-
Simulate a click for whom? You can't just 'click' a mouse without a player to perform the action - what's your specific scenario, and why can't the player click the mouse themselves?
-
Do non-player entities move correctly? Players are a bit 'special', in that their motion is determined almost entirely client-side - you need to send a packet to the client telling it what is new motion should be.
[SOLVED][1.8][FORGE] GuiHandler not firing
in Modder Support
Posted
Yeah, that's not my packet tutorial, mate. You may have seen some code like that in my old 1.7.2 tutorials, but all the notes on updating and more recent tutorials use the SimpleNetworkWrapper.
EDIT: Unless you are referring to the IEEP tutorial on Minecraft Forums - since their forum editor sucks so bad, I haven't bothered updating it since 1.7.2... but I do have an updated network tutorial which I mention in the custom inventory tutorials.