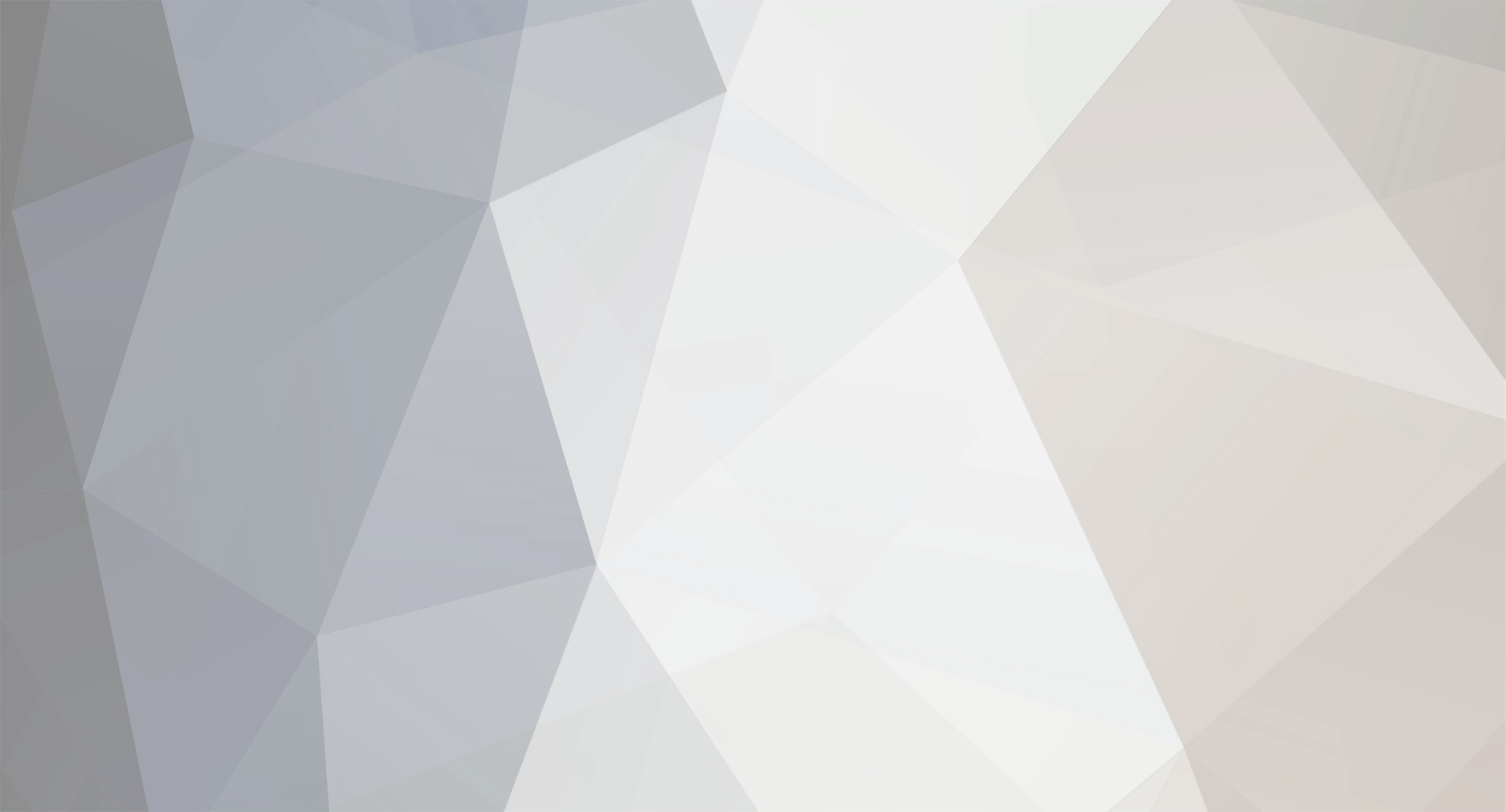
coolAlias
-
Posts
2805 -
Joined
-
Last visited
Posts posted by coolAlias
-
-
1. Create a class that extends TileEntity
2. Implement ITileEntityProvider in your Block class and return an instance of the TileEntity class you just created from #createNewTileEntity
3. Register your TileEntity:
GameRegistry.registerTileEntity(YourTileEntityClass.class, ModInfo.ID + ":tileEntityUniqueName");
That's pretty much it. I like to use the mod_id in the registration to help prevent potential conflicts with other mods.
So now you have a TileEntity... but what do you want it for?
-
The problem with a config, though, is that you are using the values on the SERVER to set the defaults in the ItemStack NBT, so the players themselves, unless they are playing single-player, won't actually be able to set their preference at all. Any changes they make to their own config will be completely ignored.
You could get around that by using the client-side config values as the defaults for the config GUI, and then overwriting those with any that appear in the ItemStack NBT. That should work fine. Also, you probably don't want changes in the GUI to be saved to the clients config file, since the settings are per-ItemStack, so I would only send the values back to the server to set the ItemStack NBT and not call config.save() at all.
-
You shouldn't need a TileEntity nor to set the light value, just use the method added by Forge that gives you the world and coordinates:
public int getLightValue(IBlockAccess world, int x, int y, int z) { int meta = world.getBlockMetadata(x, y, z); return value based on metadata; }
-
Yes, config files can be saved in-game.
Oh, you want the settings to be unique per item, not just globally applied... well, in that case, why even use the Config? Just use the ItemStack NBT, as that is automatically synced between server (where you set values) and client (where you can read them).
When you open your GUI (server-side), you can check the ItemStack's tag compound and set any values that are missing; then from the GUI, allow the user to change settings and when the close the GUI, send a packet to the server containing the new NBTTagCompound for the ItemStack and copy over your settings from that tag to the server-side tag.
Don't just set it directly - that would allow clients to potentially cheat and add their own enchantments and other things from your GUI.
-
Since these are Config settings that pertain only to the client's rendering of the inventory, you can actually get away with setting them only on the client side. Every client has their own config file that is separate from the server's, so pass the Config instance to your GUI and be sure to save it when the GUI closes so any changes the user makes are saved.
Along with that, the values are already saved in the Config file, so you don't need to mess around with them at all in the IInventory class - just get the values directly from the Config when you need them.
-
Ok so after printing the values I've found that although they're all zero when the GUI opens, and all 0 when reading/writing the NBT. The GUI is currently a GuiScreen so doesn't have a container, so I guess I'll have to change that. How come if items can be saved to NBT without a packet, integers and other raw data types do?
Any GUI that involves manipulating ItemStacks typically has a Container to keep it in sync; the fact that you don't makes me wonder how anything works at all
Perhaps my inventory-storing-item tutorial will help you out.
When the data is loaded I want to prioritise the NBT, and then if the the tags are missing use the defaults from the config file.
To do that, simply set the values of your various fields to the Config values in the Inventory constructor - it is read from NBT after it is constructed, so any value in NBT will overwrite the default.
-
You shouldn't need any kind of special Registry at all - registering your Item to the normal GameRegistry and setting its unlocalized name will automatically check your language file for the string "item.unlocalized_name.name".
As for the directory structure, everyone sets it up differently, so it was probably fine where it was before, but the default workspace setup usually has the folder as src/main/resources/assets/mod_id/actual_folders.
-
It should be under src/main/resources/assets/your_mod_id/lang.
In it, you should have the exact string to match what you see in game:
item.bacon.name=Bacon
-
You're using CLIENT only code in your #onImpact method:
if(!Keyboard.isKeyDown(42)){
Not good. Also, your 'spiderMan' field will always be null on the client side unless you specifically send it with the spawn packet - implement IEntityAdditionalSpawnData.
-
A little standard debugging should help narrow down the issue:
If you print out the values of the config color values when you open up your GUI, what do you get?
If you print out the values of the NBT color values when loading the inventory, what do you get?
If you print out the values of the config color values when saving the inventory, what do you get?
I'm guessing that the values are fine, but Inventory NBT is probably only read on the server and so the client GUI has no idea what the values are. The Container class takes care of synchronizing the actual Inventory, but unless you override #detectAndSendChanges to also sync your color values, it does not send them.
Also, you will want to send a packet to the server from your GUI to change the config values - setting them on the client will result in them not getting saved.
-
You're missing the @Override annotation above #randomDisplayTick which, if you had put it there, would have informed you that you have the incorrect method signature, thus your method is never called and your particles never spawn.
-
@Override public void onUpdate() { }
... need I say more?
-
PlayerRespawnEvent is on the FML event bus, not the MinecraftForge event bus:
FMLCommonHandler.instance().bus().register(new YourFmlEventHandler());
I believe they are merging all of the event buses into one for Forge 1.8.8, so eventually you won't have to worry about that anymore.
EDIT: Pretty standard day - ninja'd by diesieben.
-
Not really sure off the top of my head, but I did notice a few things:
1. You're calling #setBlockState TWICE in a row for both active and inactive... there's no point in doing that, as the latest call #setBlockState will overwrite all previous calls. Plus you used the same arguments for both calls.
2. You don't need a static method to set the block state - you can do it directly from within the TileEntity:
this.worldObj.setBlockState(this.pos, this.blockType.getDefaultState().withProperty(...)...);
As for the texture not changing, block state is synced automatically with the client side, so that's not the issue; are you sure your model .JSONs are correct and point to different textures?
-
The GameData class can get any Item by ID, including modded items, but keep in mind that lots of Items are subtyped or (in the case of modded items) may use NBT to specify characteristics. You'll also want to check that your random integer value returns a valid item, as not all IDs will be taken.
I'm not sure if there is a better way than that, but it's somewhere to start.
-
Maybe glRotate specifically isn't supported, but if you're adventurous, take a look at the rendering for levers. You can do that same type of code in an ISBRH and it will be able to render your block in various rotations - painful, yes, but it can be done.
-
glRotate should most definitely only be rotating your block, not the whole chunk. I'm guessing you put glRotate between glPush and glPop and rendered your block afterward, but you have to render your block after rotating and before popping.
Show your code.
-
You can get a specific modifier by passing it the correct UUID - luckily, Item uses a static one for weapon damage:
AttributeModifier itemDamage = player.getAttributeMap().getAttributeInstance(SharedMonsterAttributes.attackDamage).getModifier(itemDamageUUID);
Of course, with modded weapons, there is no guarantee the modder would use that, though they typically should. Not much you can do about those kinds of cases.
EDIT: Forgot to mention that the Item field is private, so I made a local public static copy (you could also use Reflection if you want):
/** Copy of Item#field_111210_e which is the UUID used to store weapon damage modifiers */ public static final UUID itemDamageUUID = UUID.fromString("CB3F55D3-645C-4F38-A497-9C13A33DB5CF");
-
1. You should NEVER call World#setTileEntity yourself - this is done automatically by Minecraft when a block with a TE is placed
2. Don't compare String with the '==' operator; use #equals instead
ted.username.equals(player.getCommandSenderName()) instead of ted.username == player.getCommandSenderName()
3. If your TE #username field can be NULL, you'll want to either check that first or swap the order in #2
4. You probably don't want your TE's #username field to be static - that means every TE will have the same player name, all the time
5. Don't create a new Random every time the Block is activated - the World object already has a Random instance, as does Block. Use one of those instead, OR, if you really want a new Random, add it as a class field.
-
You also need to show your Gui, IGuiHandler, and code where you open the gui.
I suspect you probably changed something else while adding buttons, so try this little test: in your Gui class constructor / init methods, comment out anything to do with buttons. Make ONLY this change, and try again - does the method get called now? I'm guessing it won't, but I may be wrong. The answer will help us help you.
-
Usually, modders will add ores and recipes using Forge's OreDictionary - this is what allows things like using copper from different mods in the same recipe.
If you're making a modpack, you can make a separate add-on type mod that adds any ores or recipes that you feel should have been in the Ore Dictionary for compatibility but were overlooked by the mod authors, and / or you can ask the mod author to use the Ore Dictionary for future releases.
-
I wouldn't say never.
"Never" is almost always relative to one's experience - for the @SideOnly annotations, I think telling people to NEVER* use them is quite appropriate. By the time they have enough experience to realize they can and should use it in some very few cases, they will probably know how to do so without causing all sorts of mayhem like the OP did in this thread.
* Except when a parent method or class uses the annotation, in which case yours should have it, too, which I noted in my first comment about this.
-
diesieben does a much better job of explaining it than I probably would.
-
Hey @coolAlias, is that possible to implement in the RenderBow.class i have?
No and yes - no because that is an Item method that goes in your bow class, yes because you call that method from your render class to get the icon you want to render.
Look at the vanilla ItemBow class and bow rendering - highlight ItemBow#getIcon and open the call hierarchy for it to find the bow rendering code.
[1.8] [SOLVED] Help with right-clickable blocks
in Modder Support
Posted
You don't need a TileEntity for a block to be a clickable, and in fact a TileEntity has nothing at all to do with any type of block-player interaction, at least not directly.
You need to override #onBlockActivated in your Block class and put whatever code you want to happen there.
Show what you tried.