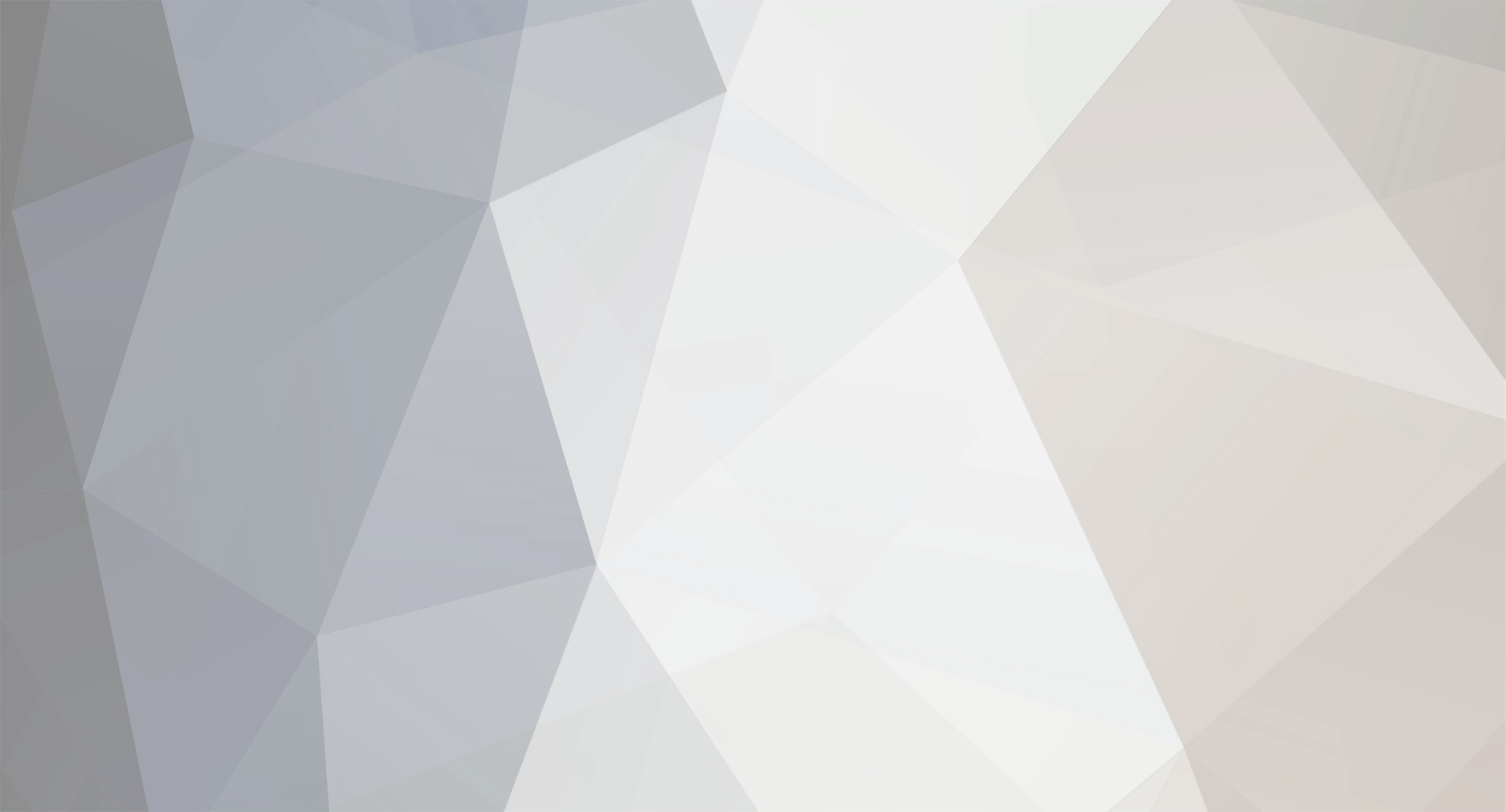
coolAlias
-
Posts
2805 -
Joined
-
Last visited
Posts posted by coolAlias
-
-
Arrows have some special code to set the shooting entity on the client side when they spawn, which is what let's the arrow know not to hit the shooter right away. Your issue is partially that the client doesn't know about that, so the client arrow immediately hits the shooting player while the server arrow goes on its merry way.
To fix it, implement IEntityAdditionalSpawnData and send the shooting entity's ID, then fetch the entity from the world when reading the data and set it as the shooting entity.
Also, anything extending EntityArrow should have a tracking update frequency of 20; most other projectiles have a frequency of 10.
Furthermore, I suggest you do not simply copy/paste entire vanilla classes - things like
private static final String __OBFID = "CL_00001715";
and parameter names like 'p_12326_a' really hint at sloppy coding / lack of effort to understand what's going on in the class.
-
Removing a player from another player's tracker wouldn't technically make them 'invisible' to code - the tracker is only responsible for sending updates about an entity's position / state to the various client players tracking it; the server still knows everything, but the player would probably appear 'stuck' to other players. At least that's how I understand the tracking system.
However, if you want the player to be basically invincible + unmovable etc, the simplest way I have found is to cancel the LivingUpdateEvent, possibly in addition to the LivingHurtEvent.
-
#isKeyDown (previously #getKeyIsPressed) is the one to use for continuous querying - #isPressed will return false after calling it the first time.
Also, you should not register KeyBindings in your CommonHandler - they are client side only and should be registered in your ClientProxy or its equivalent.
Show your registration code.
-
If you want to move it left/right with respect to its current heading, then yes, you need to account for yaw, and that involves lots of math (just look at EntityArrow or EntityThrowable constructors).
Alternatively, and I'm not sure how well this will work, you could try using this.getLookVec() - this should be defined for all entities, and hopefully will give you the current directing the spell projectile is heading.
However, given that you seem to be spawning a projectile for your flame thrower, surely the projectile was sent in the direction the player was initially facing, right? And you keep spawning new entities each tick, right?
Personally, I find an entity-based implementation to be somewhat unwieldy for something like a flamethrower - I prefer to simply spawn particles in the direction the player is facing each tick (while using the item), and check for entities within that area to damage / set on fire. Then you can use the player's look vector and current position to figure out where to spawn the particles and what values to give them for motion (e.g. in the same direction as the player is looking).
-
That's the method you have to use - specify EntityItem as the class of Entity to retrieve, and you will have a list of only EntityItems. Iterate through the list, cast to EntityItem, and check if it is the item you want.
-
Only use 20 for the update frequency if your entity extends EntityArrow (otherwise you may end up with weird behavior) - all other vanilla projectiles use 10, including things like fireballs.
-
That has a somewhat complicated answer, but basically you check if the mouse is clicking within your scroll bar's current position and whether it is dragging up or down, then modify the position accordingly. You can also check for the mouse wheel if you want.
Each time the screen renders, you use the current position of the scroll bar to determine what part of your image to render, or how much of whatever needs to be inside that specific area to render.
While for 1.6.4, VSWE's Climbing the Interface Ladder series is excellent and explains very well how scroll bars function (among many other things). I highly recommend it.
It would be easiest to follow in 1.6.4 and then update to 1.8 later, but you can probably manage to do it on the fly as not much has changed since then as far as interfaces go.
-
If you need 32 states, then no, I wouldn't recommend a separate icon for each one. I only suggested that because it sounded like you had 4 states, 6 at most, in which case it wouldn't be so bad.
I assume you have a TileEntity, then, to store that many states? Metadata can only handle up to 16, so you may want to use metadata for block rotation and the TE for whatever else you need, or vice versa.
Yes, you need to call #draw after you have added all the vertices, otherwise nothing will show up on the screen
-
Honestly I'm not really sure what those mean, but if you are intent on using the ISBRH to render 1 icon with different rotations, you should look at using the Tessellator, specifically #addVertexWithUV.
Depending on the block's metadata (which you would set based on the player's facing / pitch when the block is placed), you would change the order of the rendering vertices, e.g. starting at x=16 and going to x=0 instead of 0->16 to invert the icon along the x axis.
The easy route, though 'less optimal', is to simply create 4 different icons, one for the arrow facing each direction, and register all 4 as your block icons, then return the correct one from #getIcon based on the block's metadata. Simple and effective, no need to mess with difficult rendering code - sounds like a win to me, though I know some would disagree.
-
You should be able to simply use metadata to handle which face shows the icon, or if it's not about which face, which version of the icon is shown, but that would require 1 icon per direction.
Since you are using ISBRH anyway, you can instead use the GL11.glRotatef function to rotate the icon before you render it - take a look at the vanilla block rendering code, especially for buttons, levers, and that kind of thing, for examples.
-
Extend EntityThrowable (easier to work with) or EntityArrow; EntityArrow has a constructor specifically used by skeletons to send the projectile towards a target, rather than in the direction the shooting entity is facing. You should be able to either use it directly or copy / adapt it to suit your needs.
-
Only create the explosion on the server side, not both. The client thinks those blocks got destroyed, so it doesn't render them, but the server disagrees
-
Block bounds go from minimum to maximum, i.e. minX, minY, minZ, maxX, maxY, maxZ.
You have 12 * f as the minimum z, which is greater than the maximum z of 4 * f. Switch them.
-
If diesieben's succinct but 100% accurate explanation doesn't get you there, here are video tutorials from Lex Manos:
-
Vanilla almost ALWAYS has the answers.
I know it can be hard to find things sometimes in the vanilla code, but you should at least try. In this case, the answer lies in ServerConfigurationManager#respawnPlayer, which uses 'verifyRespawnCoordinates' to check if the bed is still valid, and below is code that I have adapted from that for my own use:
ChunkCoordinates cc = player.getBedLocation(player.dimension); if (cc != null) { cc = EntityPlayer.verifyRespawnCoordinates(player.worldObj, cc, player.isSpawnForced(player.dimension)); } if (cc == null) { cc = player.worldObj.getSpawnPoint(); } if (cc != null) { // now you have the correct spawn coordinates }
-
It's #setTagCompound... but you should have been able to find that out in < 1 second by just typing 'stack.set' in your IDE.
-
I'm pretty sure you need to set the player's position in the Teleporter class, too. Why don't you start with the Teleporter class I showed you earlier, rather than creating one with all empty methods?
Also, it's important to adjust the player's Y position, otherwise you often end up falling through the world into the void.
-
That's bizarre. Does the packet teleportation still work as expected now that you have modified the Teleporter class? If you print out the bed coordinates in both cases, are they the same?
-
It involves lots of trigonometry, but basically you subtract the target's position from the player's position on both the horizontal axes and run that through atan to get the angle, then subtract the player's current rotation yaw from that angle and make sure it's clamped between -180 and 180. I found I had to add 90 to it after the fact, probably as the result of some quirk in Minecraft's coordinate system.
You can use similar math for the vertical axis, but be sure to include the entities' respective heights and/or eye positions in your calculations.
Once you have both angles, call player#setAngles(yaw, pitch) and you're good to go. Perhaps consider setting the player's head yaw instead of their entire body, depending on your situation. I do this on the client side, btw, but you may want to do the calculations on both sides.
-
Why are you sending a packet at all? PlayerTickEvent happens on both sides, so run your teleportation code directly on the server instead of messing with packets. Furthermore, if you are worried about it processing more than once, then set a flag while it is processing to prevent it from resending, but this will not be a problem if you process directly on the server as suggested.
As for your teleporter putting you in the nearest portal, that's because that's what it does unless you override more methods like I did in my code, specifically #placeInPortal. I suggest you take a closer look at the vanilla teleporter code.
-
You're not listening: do NOT send in an NBTTagCompound to that method - that makes NO sense at all, and you don't even use it in your method:
public void setSummoned(ItemStack itemstack, int Index, NBTTagCompound compound) //NBTTagCompound compound) { // where do you use 'properties' ? Right, nowhere. Where do you use 'compound'? Again, nowhere. Get rid of them. NBTTagCompound properties = (NBTTagCompound) compound.getTag(CELESITAL_CRAFT_INFO); Index = itemstack.getItemDamage(); // 'Index' already equals the stack damage, so this is pointless, too, meaning the ItemStack parameter is not needed at all if (summonedState[index] == false) { summonedState[index] = true; }else{ summonedState[index] = false; } // that's a lot of lines for a simple operation (toggling a boolean value): summonedState[index] = !summonedState[index]; this.sync(); // not necessary unless you plan to display your information in a GUI, and even then, this is overkill - use a more specific packet that sends only the information that has changed }
Also, you should NOT use #getEntityData when you are already using IEEP.
Furthermore, you have 12 private boolean fields, and then a boolean array in your IEEP class - the 12 fields are not used except as defaults for the array, but you never initialize them (which is fine - they default to false, but so does the array). This is silly:
private boolean aquariusActive, taurusActive, cancerActive, virgoActive, sagittariusActive, leoActive, ariesActive, scorpioActive, geminiActive, capricornActive, piscesActive, libraActive; public boolean[] summonedState = new boolean[]{aquariusActive, taurusActive, cancerActive, virgoActive, sagittariusActive, leoActive, ariesActive, scorpioActive, geminiActive, capricornActive, piscesActive, libraActive}; // why not this instead: public boolean[] summonedState = new boolean[12];
You also have two identical arrays, 'celKeys' and 'celKeys2', in your Item class...
As for your question - it works because of all of the reasons I already explained, as well as basic logic: set a flag and use it as a conditional check before allowing an action. I really don't know what to tell you other than that, because that is all that it is, and seems so rudimentary and fundamental a concept as to not even need further explanation. If you don't get it, perhaps take a programming 101 course? At the very least, start from the beginning of the Java tutorials I linked earlier. You will thank yourself for it later.
It has nothing to do with the packets which, in your case, you do not even need since you are not displaying any information on the client side. You should remove all of the packet code unless and until you actually need it.
-
It looks like you are trying to set NBT data directly in your IExtendedEntityProperties class - this is not the way. Store data in appropriate class fields, such as a boolean array.
E.g. your #setSummoned method should take neither an ItemStack nor an NBTTagCompound (where the heck is that coming from anyway?), nor should you pass in an 'int Index' simply to set it equal to the stack's damage value. None of that makes any sense. All you want this method to do is mark a specific entity type as summoned or not, so you need 2 arguments: the type of entity, and whether it is currently summoned or not.
When you call this method from the Item, you will almost certainly only ever be passing 'true' as the 2nd argument, and you would call this method again in your entity's 'setDead()' method to make sure it gets reset to 'false' for the summoning player and summoned entity type.
I know you say you learn from examples, so let me direct you to some basic Java tutorials that cover methods and method arguments. Seriously - go through all of the lessons, practice what each one discusses, and you will find your current problems to be trivial.
-
You need to get the IEEP instance for the given player, and then call whatever class methods you have, e.g.
YourIeepClass instance = YourIeepClass.get(player); instance.setSummoned(6, true);
-
1. Do NOT use 'static' on your mutable class fields
2. You need to actually save the value of the variables - your save method currently sets every one to 'false'
3. Getting and setting these values is about as basic of Java as it gets - just make methods to do what you want
[1.8] [SOLVED] Offset a projectile in a certain direction
in Modder Support
Posted
If you just want to move it down:
If you want to move it to one side or the other: