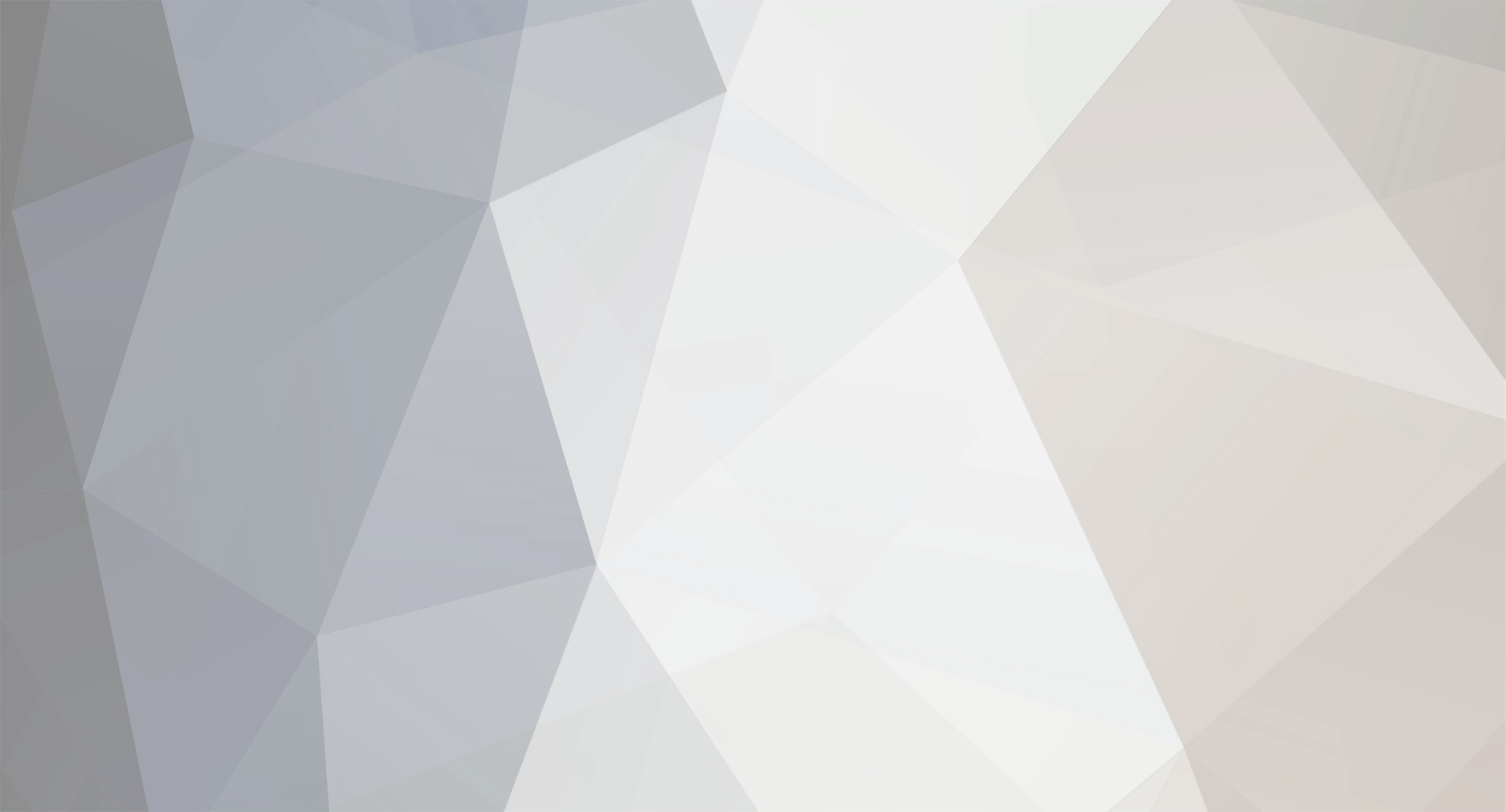
coolAlias
-
Posts
2805 -
Joined
-
Last visited
Posts posted by coolAlias
-
-
From your code, it looks like you are trying to return exactly the same transformations / quads that vanilla would... is that just a placeholder? If your model is all messed up, maybe the problem is in your JSON.
-
The whole point of IPerspectiveAwareModel is to let you define exactly which transforms you want to apply in each perspective, and it would be much more difficult to do that if it applied all of the vanilla transformations for you first.
If your model needs to have different transformations in one or more perspectives but you still want to use the vanilla transformations as a base, you can easily do so:
switch (cameraTransformType) { case GUI: RenderItem.applyVanillaTransform(parent.getItemCameraTransforms().gui); break; // etc. for other cases
-
You don't need to make it so complicated - just use the improved for loop to iterate over the actual list:
for (EntityPlayer player : world.playerEntities) { BlockPos pos = new BlockPos(player); // or if you want to use coordinates directly: double x = player.posX; double y = player.posY; double z = player.posZ; // now spawn in the item entity }
-
> You can use player.isUsingItem() without any issues, and if that's true, use player.getHeldItem() to get the item being used. No need for Reflection here.
Didn't think of that... it's probably safe to assume they're always the same... probably...
At least until 1.9
-
You can use player.isUsingItem() without any issues, and if that's true, use player.getHeldItem() to get the item being used. No need for Reflection here.
-
the thing is is that I cannot use a string array since every time someone shares the waypoint with someone else it must add a player name to the list.
So assign a new array of the appropriate size or use a Collection such as Set or List.
-
I've localized the problem... I think...
When sneding the message I write the line like this:
GearsAndClouds.network.sendToServer(new GnCPacket("player invisible"));
I think they mean that new. How do I work around that?
No, that's not it. You have to make a new instance of the message class to send it, and the error message specifically said it crashed trying to create a new instance of GnCPacketHandler, which the above is not.
Show your entire GnCPacket class, including the inner GnCPacketHandler class.
-
Can I just get a number please
8
On a serious note, Draco told you exactly where to look to find the information about block updates in connection with setBlock - are you for some reason unable to read it? It only takes like 3 seconds, and if you have trouble finding it, then it is a very good exercise for you to improve your IDE skills. Learning to use your IDE will go a long way toward helping you help yourself in the future.
-
Don't store the seed as a field in your Block class - Items are not yet initialized (i.e. all null) when you initialize your blocks. Return MyItems.seed or whatever instead.
Did not work...
But I think your wrong since it still drops the product without me doing that!?
You said the seed wasn't dropping, now you say it is, so which is it?
If you have the following:
// in your main class public static Item seed; public static Block block; // during FMLPreInitializationEvent block = new SomeCropBlock(seed); seed = new SomeSeedItem() // in your Block public SomeCropBlock(Item seed) { this.seed = seed; }
Then your block, when it tries to drop the seed, will return a NULL pointer because the seed Item was null when given to it. If instead you reference it via YourMainClass.seed in the method to drop the seed, you won't get a null pointer.
-
I think it is safe to assume that the item in the hand is the one taken from the crafting
Really? You never click an item and put it in any of the other 30 some slots in your inventory? You always place it in your current slot? I don't think I ever put it in my current slot, as I'm usually already carrying something.
Sorry, but I don't think using any kind of ticking event is the answer to this question.
-
Don't store the seed as a field in your Block class - Items are not yet initialized (i.e. all null) when you initialize your blocks. Return MyItems.seed or whatever instead.
-
http://www.minecraftforge.net/forum/index.php/topic,23133.0.html
There you go! Using this technique u can make recipes that can only be crafted under specific circumstances! Should be exactly what u want! Go and bumb diesieben's karma up, he did a great job there
That only works for new recipes, though. The OP seems to be looking for a way to restrict vanilla recipes. I guess you could remove all of the recipes and replace them with custom IRecipes... seems like a lot of work
-
Put this as your parent model in your block's model:
"parent": "minecraft:block/cross", // textures go here
That's all there is to it.
-
I may be wrong, but I don't think there is any easy Forge hook that lets you change that.
The only way to restrict it for certain players that I have ever seen is to substitute in your own crafting matrix for the vanilla one, so that you can inject your own logic. Not very inter-mod compatible, but it gets the job done.
That assumes you already have a way to know which recipes are restricted for which players, such as storing their 'learned recipes' in IExtendedEntityProperties or whatever it is you are doing.
-
You can remove recipes entirely so no one can craft that item - would that be an acceptable solution, or are you trying to restrict recipes just for certain players?
-
I also heard of adding something like NBT into another NBT (cant remember correctly)
So can someone explain a bit on this and give me an example?
Make a new NBTTagCompound, add stuff to it, then add that compound to the first one. Simple as that.
Your best bet is to just start playing around with it, looking at vanilla examples (tile entities and mob-like entities are all good places to start), etc.
-
Where can I find all of this info? And do I need to pretty much learn how Java and whatnot to fully nail this down?
Yes. How else were you planning on obtaining all of this information, if not through code? I also posted some information in your other thread.
-
Set an expiry date in the ItemStack NBT, e.g. world.getWorldTime() + duration_in_ticks, then compare the current world time to the expiration date.
You won't really be able to reliably 'destroy' the ItemStack if it's in some random container, though, since you'd need to have a reference to the container (i.e. the IInventory) in order to set the contents of the slot to null - just setting the ItemStack to null won't actually affect the container or any other place that has a reference to it. You could, however, decrement the stack size.
Once the stack size is 0, it should (I think...) get removed when a player interacts with it, but to make sure you can override the Item's update tick (which fires every tick the item is in a player's inventory) to remove the itemstack if the stack size is 0.
-
Also, you can simply send the player's current entityId instead of UUID, but as diesieben and others have said, you already have the player from the packet.
The only reason you would even want to do something like that is if you needed a DIFFERENT player in the packet, e.g. player 1 is interacting with player 2, and you need player 2 (in addition to player 1, which you will have by default) when sending the packet to player 1.
-
Provided that Mojang / any modders correctly implement their weapon-holding mobs, you'd get the correct attack damage simply by getting the current value for the mob's SharedMonsterAttribute for attackDamage.
However, I don't think that mobs calculate their actual total in the same way players do, but you can still use that to get their base damage value (usually) and then use entity#getHeldItem() to get their held ItemStack. Once you have the ItemStack, you can find all of its AttributeModifiers and Enchantments using various methods in ItemStack/Item and via NBT for enchantments.
-
entityitem#delayBeforeCanPickup = x; // changes to setter in 1.8
-
CMEs typically occur when dealing with Collections, usually when one thread is iterating over one and another thread is removing items to that same Collection.
From your log, it looks as though you have an entity that might be doing some Collections processing when reading/writing NBT, specifically writeWatchedListToPacketBuffer.
Putting that section of code within a synchronized block will probably fix it, or by using Iterators to traverse your list when you are removing entries.
-
Don't set onGround to true unless the player is on the ground.
Your trouble is caused because you are setting fall distance on the CLIENT, but the player's fall distance on the SERVER is what results in the player taking damage.
You need to send a packet telling the server that your player is trying to double-jump and let the server say, 'okay, s/he can do that, and now I'm resetting fallDistance'.
-
I understand what you are doing but that is not exactly what I am going for, basically I intend on using something to test if they are airborne (and yes I know there already is something for that), then let them fly for a short amount of time when they double tab space bar and then boost them where they are looking, but I hit a wall and deleted all my code like an idiot because where I was going was not going to work, I had a friend who worked with bukkit servers and that is how he said to do it, but he never explained how, so now I am stuck
Well you should have said that from the beginning, rather than just 'double-jump'.
What exactly do you mean by 'let them fly'? Like in Creative Mode, or only in a certain direction? Doesn't seem much like a double-jump if they can fly... What about this boost, does it trigger automatically after their fly-time is over, or is it another button press?
Anyway, the basic premise is exactly the same:
if (jump key pressed and entity already in air) { allow flying for short time; }
each tick:
if (fly time > 0) {decrement fly time; if (fly time is now zero) { boost in direction player is facing; }}
'Boosting' a player in a certain direction can be done simply by getting their look vector and then adding some multiple of that to their current motion values.
[1.7.10] Controlling Enchantments of Enchanted Books when added to Loot Chests
in Modder Support
Posted
If I read your post correctly, you have an ITEM that gets USED, and when used it gives an enchanted book? If so, then there is absolutely no need for WeightedRandomChestContent - that's for randomly generating loot inside of inventories, but you just need a single enchanted book generating onItemRightClick.
Instead, you can use what ItemEnchantedBook uses when it is determining random enchantments for said loot:
For more or less powerful enchantments, on average, increase or decrease the last parameter.