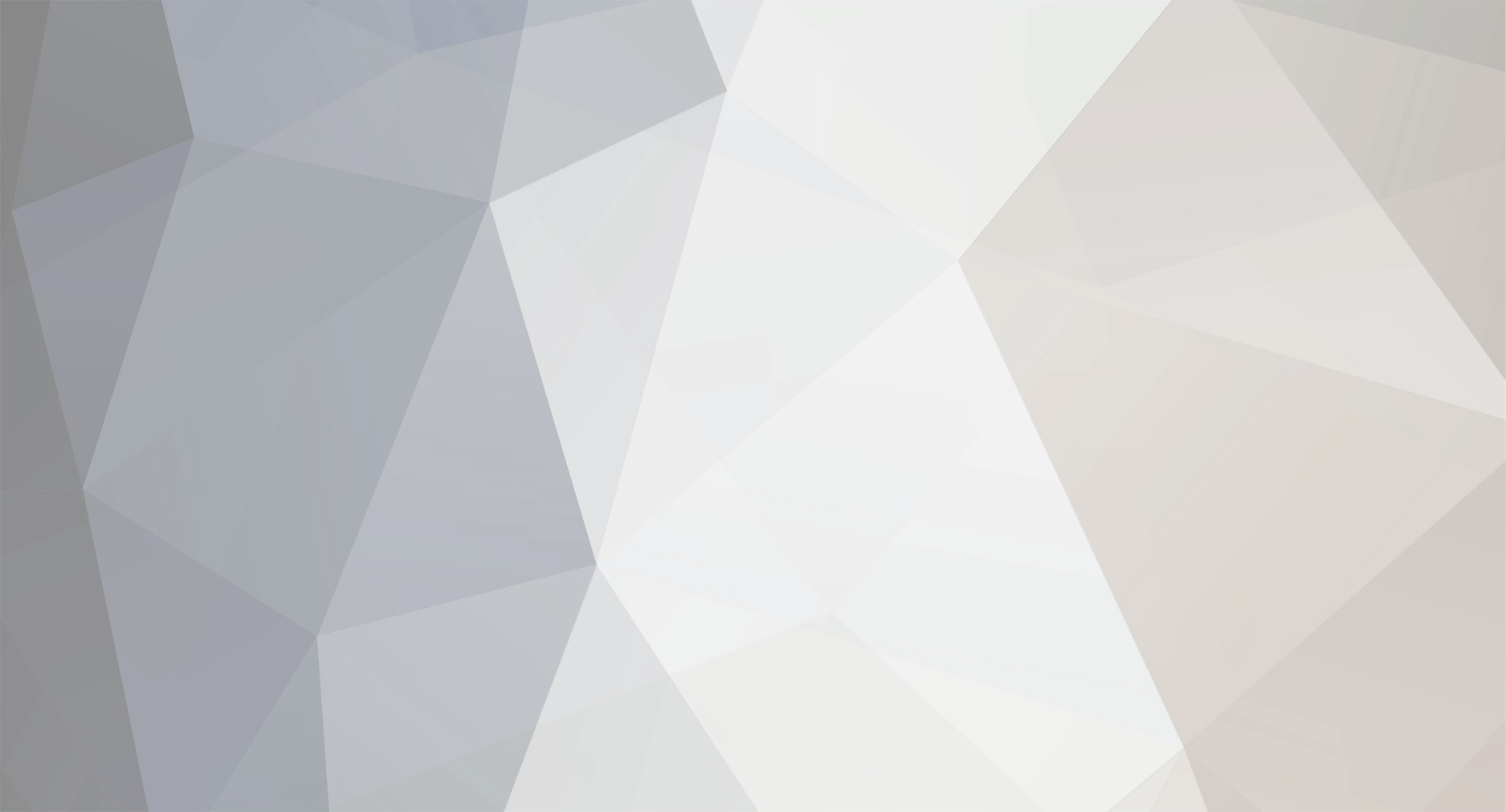
coolAlias
Members-
Posts
2805 -
Joined
-
Last visited
Everything posted by coolAlias
-
You really shouldn't need to take such drastic measures. You can do all of that rendering directly from RenderHandEvent, which is only fired in first person anyway (I believe), or you could move it to happen with your Item's IPerspectiveAwareModel and do your rendering of the player's hand from the first person perspective there.
-
[SOLVED] Preventing 1st-person item change animation?
coolAlias replied to coolAlias's topic in Modder Support
It's not something I have done, it's something I am proposing we add to Forge so that we can avoid crazy workarounds like the one you implemented. The fact that some modders go to such great lengths to prevent this stupid forced animation is, in my opinion, a strong indicator that my proposal is at least in the right direction, and I think it would solve these issues pretty elegantly with minimal code. Again, if anyone can think of a better way to do it, or has suggestions of how to improve mine, I'm all ears, but something needs to be done. If Lex Manos or anyone else on the Forge team happens to read this, would there be any reasons you can think of that my suggestion would be rejected as a PR? -
Always great gifs from Ernio @OP As others have said, you need to compare the ItemStack in the slot to what you are looking for, but also, if the player is in Creative Mode, you shouldn't even bother looping from 0-9 unless you need to set ammo for creative players, too, rather than just letting them shoot whatever the default is. What I would do is create a list of Items / ItemStacks that are acceptable ammo, and compare those: // Elsewhere in your code: private final List<Item> acceptableAmmo = Lists.newArrayList(); static { acceptableAmmo.add(Items.wheat_seeds); // or whatever items you want as ammo } // In Item#onItemRightClick method: ItemStack ammo = null; // adding in '&& ammo == null' to the for loop will cause it to exit as soon as acceptable ammo is found for (int i = 0; i < 9 && ammo == null; ++i) { ItemStack stack = player.inventory.getStackInSlot(i); if (stack != null && acceptableAmmo.contains(stack.getItem())) { ammo = stack; // now the loop will stop, and you have your ammo type } } if (ammo != null) { // here you can decrement the stack size of the ammo stack and spawn in correct projectile, etc. }
-
[SOLVED] Preventing 1st-person item change animation?
coolAlias replied to coolAlias's topic in Modder Support
@EverythingGames That is exactly the kind of hacky solution that I am trying to avoid with my proposal. -
The only way to do so is to iterate through each hotbar slot and check the inventory stack. Unless your item can shoot itself, you can safely iterate without worrying about the currently held item, since the currently held item must, by definition, be the one that was right-clicked, i.e. your item, and wouldn't be a valid ammo type.
-
If your block variants, you need to add each one to the ModelBakery using the unlocalized name by which you plan to retrieve it. Then, make a custom ItemBlock to return the correct unlocalized names for each damage value, or set up your names to use a standardized format that can take advantage of the naming function from ItemMultiTexture, e.g. either using your Block's EnumType or as 'tile.your_block_base_unlocalized_name.specific_type'.
-
Look at World#setBlockState - each time the stem grows, that method is called, which should allow you to make your checks (using the block snapshot event) and see if you want to place a melon block on top of a hopper.
-
[1.8]Using aabb to give near entitys a potion effect.
coolAlias replied to Looke81's topic in Modder Support
With Java. If you don't already know it, learn it. Google and Java documentation / tutorials are your friend. -
What do you think the 'Entity' parameter is for? There is an entity parameter in nearly every single Render class method. I'd really like to help you further, but nothing I say is going to teach you Java, and that's what you need. Just look at your code: private String getZertumType() { if (entity instanceof EntityZertum) { return ""; } else if (entity instanceof EntityRedZertum) { return "r"; } else if (entity instanceof EntityMetalZertum) { return "m"; } else if (entity instanceof EntityIceZertum) { return "i"; } else if (entity instanceof EntityForisZertum) { return "f"; } else if (entity instanceof EntityDestroZertum) { return "des"; } else if (entity instanceof EntityDarkZertum) { return "d"; } return ""; } For one, that will never work, because all subclasses are also instances of the parent class, so only your first condition is ever evaluated. Next, I assume this is the method in which you 'need an entity' - so send it one as a parameter! Finally, why have a method like this at all, when you can simply add it to your EntityZertum class and have each subclass override it to return the appropriate value? Much better encapsulation and extendability. Best of luck to you.
-
You're not being very clear about what you want: do you want to render a different model based on the entity's state, or render TWO models at the same time for the same entity? In any case, you still need to create or provide all the models you want when instantiating your render class, then choose amongst them when rendering. As I've both explained and demonstrated. On a side note, all that time you've spent looking at other people's code hoping to find what you're looking for would have been better spent learning the fundamentals of Java - then you could write your own code.
-
[1.8]Using aabb to give near entitys a potion effect.
coolAlias replied to Looke81's topic in Modder Support
AxisAlignedBB takes 6 arguments: minX/Y/Z, maxX/Y/Z. Easiest way to use it for an area surrounding an entity is getEntityBoundingBox().expand(dx, dy, dz) where dX/Y/Z are the amount you want to expand along that axis (it expands in both directions, so 8.0D actually adds 16.0D to the total dimensions). this.getEntityBoundingBox().expand(8.0D, 4.0D, 8.0D) // gets all entities around this entity within 8 blocks, but only 4 along the y axis -
Well that certainly is a mystery :\ You should at least implement createChild to not return null and see if that makes any difference (don't see why it would, but then I don't see why what you have isn't working). Other than that, I have no idea, sorry.
-
1.8 Game crashes after I tried to add a block
coolAlias replied to Kingz101's topic in Modder Support
package proxy; // <== that looks very very wrong public class ClientProxy extends CommonProxy { // etc. ... Are you not getting any errors in Eclipse that tell you your package structure is wrong? A "package" is basically the same as a directory, and it needs to include the full directory structure up to and including your main class: package kingz.cdm.proxy; // also, package names should be all lower case public class ClientProxy extends CommonProxy { // etc. -
1.8 Game crashes after I tried to add a block
coolAlias replied to Kingz101's topic in Modder Support
Look in your ClientProxy class, what is the FULL package name? I highly doubt it is just 'proxy'. full.package.name.ClientProxy, e.g. yourmodname.proxy.ClientProxy -
Heh, more like I've just run into all the same problems before
-
1.8 Game crashes after I tried to add a block
coolAlias replied to Kingz101's topic in Modder Support
You're looking in the wrong place for your ClientProxy; or looked at in another way, you put your ClientProxy in the wrong package. Make sure CDMRef.CLIENT_PROXY_CLASS is the actual package name for your ClientProxy. -
Put the main model as a separate argument, then something that's not a ModelBase next, and then the array of alternate models, or just pass the first element of the array to the super method. If you don't understand what I'm saying, Google / Java tutorials are your friend.
-
1.8 Game crashes after I tried to add a block
coolAlias replied to Kingz101's topic in Modder Support
Well, what does the crash log say? -
Dear lord. ModelBase... model is an ARRAY. RenderLiving expects ONE model. Basic Java. Learn it.
-
Are you logging in with the same name each time? The default development environment settings log you in with a new user name each time - you need to set it in the run-time configuration: --username Me. You can even add your password (if the username is your real one) to log in using your actual account, if you want.
-
There is something wrong in your code, then, that you didn't show, because I can call super on RenderLiving's constructor just fine while adding any parameters I want to my own constructor: public class RenderGenericLiving extends RenderLiving { public RenderGenericLiving(RenderManager renderManager, ModelBase model, float shadowSize, float scale, String texturePath) { super(renderManager, model, shadowSize); // other stuff } } No errors there. I bet you left in an empty constructor, e.g. public RenderZertumModel() { super(); }. This just reinforces my point even more...
-
There's a whole topic about it that may help, but basically: 1. Set your entity's held item to what you want (use addRandomArmor or whatever else you need upon spawning) 2. To make your life easy, extend both RenderBiped and ModelBiped where appropriate 3. Make sure whatever attack AI you are using calls #swingItem when attacking - EntityAIAttackOnCollide does this. 4. Make sure you call updateArmSwingProgress() from your entity's onLivingUpdate method, otherwise the swing animation won't work despite your entity holding the sword
-
The second parameter in EntityAIAttackOnCollide is the speed at which your entity will move towards the target. Most of the time, it should be the same as or just slightly more than your entity's movement speed, yet you set it to 4.0D, which is roughly 4 blocks per tick. Take it down a notch
-
Use an AttributeModifier for a permanent (or temporary) boost to max health.