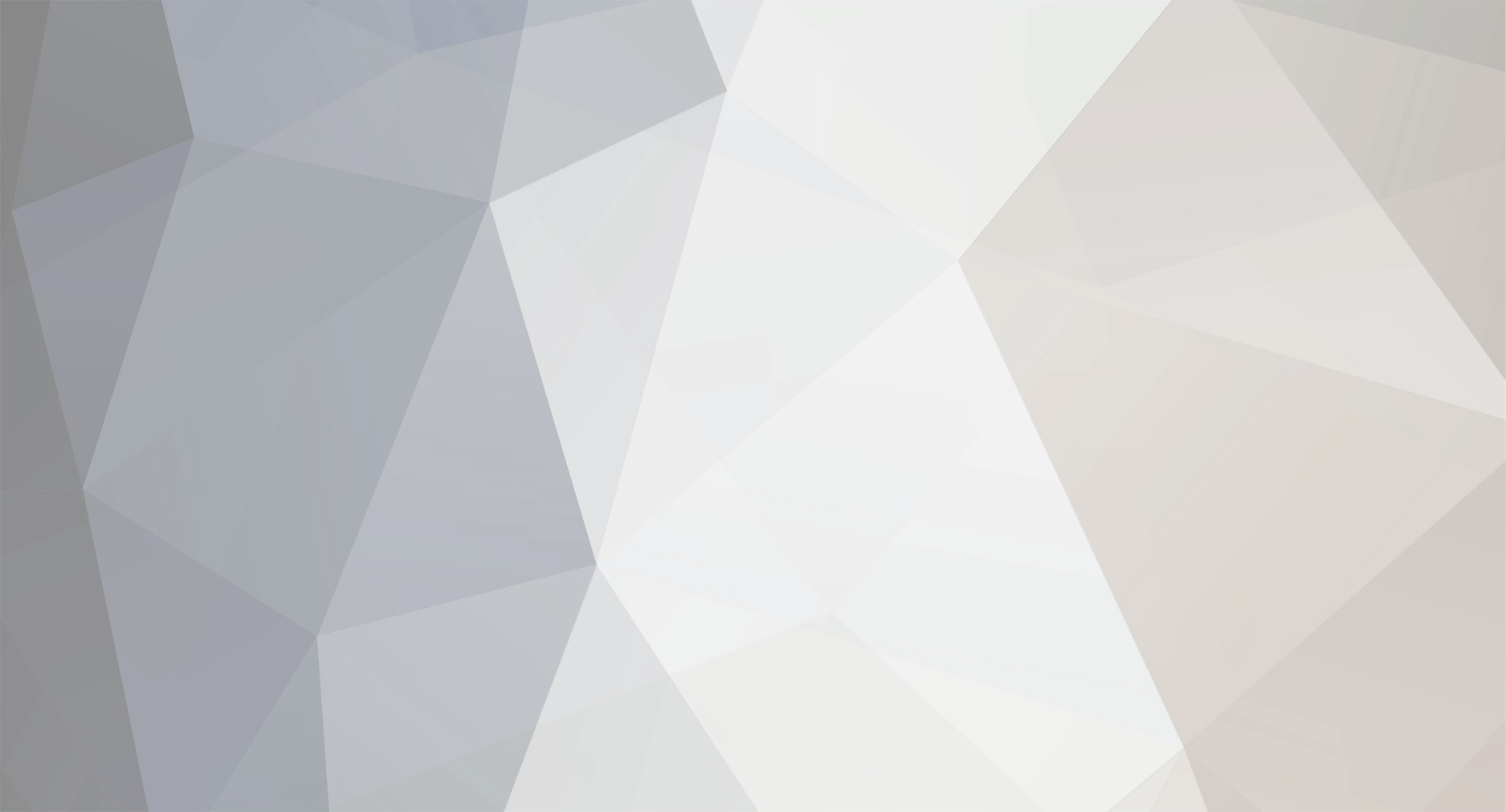
coolAlias
Members-
Posts
2805 -
Joined
-
Last visited
Everything posted by coolAlias
-
[1.8] Block and TileEntity Event (receiveClientEvent).
coolAlias replied to Ernio's topic in Modder Support
While I'm not sure exactly what the documentation is implying, I override shouldRefresh to return false for ALL of my TileEntities so that they behave identically to vanilla - if you look at the base implementation, NO vanilla TE will ever return true. It's a mystery why the default behavior for modded TEs is opposite that of vanilla (per diesieben: "SOMETHING had to be chosen for the default..."), but I know people have run into unexpected issues because of it: their TE data suddenly disappearing after setting a state property, for example, so clearly the server side must also be getting refreshed, though your experiment seems to suggest otherwise. -
A copy is defined however you decide to implement it for your object, just like 'equals' is defined however you implement it in Java. This is part of what makes C++ so powerful, though I don't miss memory management in the least - automatic memory management, e.g. via JVM, makes life 100x simpler. While Java does have clone() and you can implement a copy() constructor, it isn't quite the same in that you'd have to call the copy constructor yourself, whereas in C++ the copy constructor is called automatically whenever creating a copy of an object. However, as you say, this is going into much more detail than is required for the (solved!) issue. The one time in over a year that it slips by me and we get a whole freaking discussion thread about Java vs. C++ EDIT: Here is a good writeup about Java / C++ with respect to this issue.
-
[SOLVED] Preventing 1st-person item change animation?
coolAlias replied to coolAlias's topic in Modder Support
There is Item#getShareTag() which could be used to prevent the itemstack NBT from being sent to the client, which would presumably stop the animation change for that particular case, but then you couldn't use the NBT data at all for rendering. So, it seems that currently there is no possible way (i.e. without ASM) to do what I am attempting. In that light, does anyone have any comments on my proposed solution? Can you think of any situations it would not handle well? Is there a better way? I ask because I don't want to go to the trouble of learning how to submit a proper PR to Forge (I tried once before but I had just edited the files directly, which is apparently a big no-no) and putting one together only to have it rejected for something stupid I didn't consider. -
[1.8] [SOLVED] Overriding left mouse click on an Item
coolAlias replied to SnowyEgret's topic in Modder Support
You may be able to prevent the sound using event.useBlock = Result.DENY; But you will have to actually intercept the MouseEvent and cancel that if you want to prevent the arm swing animation, and then you'd have to call your Item methods manually (which involves sending packets to the server, since MouseEvent is client side only). -
Exactly, Java is always pass by value, though with non-primitive types it passes the value of the memory location rather than a copy of the entire object, thus acting like a C pointer passed by value, so you can always alter whatever is at that memory location, just not the initial reference to that memory location. As we all well know I just completely spaced out about the list and started focusing on other things as the possible cause, exacerbated by the fact that I was working on it in short amounts of time between waking up and going to work. So yeah, I got tripped up lol
-
It's not pass by reference / value that trips me up, I just totally forgot about it - in all my other code I use Lists.newArrayList(parentList), and I just kind of assumed that this was going on in the background somewhere... it didn't even cross my mind as I was looking at my code earlier that I was changing the parent list even though I know very well that's how it works. One of those facepalm moments for sure, and embarrassing to have posted about it
-
@jabelar Actually, that part of the tutorial is deprecated. There is a much better way to handle it, and that is PlayerEvent.Clone. This is fired each time a new player instance is created and provides you with both the old instance and the new one, giving you the perfect opportunity to copy whatever data you had in your IEEP class to the new instance. The only thing to keep in mind at that point is that if the client needs to be aware of that data, you still need to send a packet after cloning, and the best place (imo) to do so is in EntityJoinWorldEvent.
-
[Resolved]get were the player is looking 1.7.10
coolAlias replied to BoonieQuafter-CrAfTeR's topic in Modder Support
Not from my end, it isn't, but just in case, here it is, unquoted, again: "Also, why are you adding them at all? The raytrace MovingObjectPosition returns the block position that was hit, as in real world block coordinates. If you add the player's position to that, you will get very bizarre numbers. Example: Player is at 100,64,100 looking east (+x axis); MOP returns a block at 100,64,115 (directly east). Now you add those together, and spawn lightning at 200, 128, 215. Do you really expect to see it?" In summary, use the coordinates from the MovingObjectPosition, and DO NOT ADD anything, certainly not the player's position. It makes no sense at all to do so, as you can see in the example provided. -
Golems use world.setEntityState(flag) when they attackEntityAsMob - this sends a byte flag to the client side, which calls your entity's handleHealthUpdate(flag) method. Golems use that to set their attack timer on the client side as a flag for the model to begin animating, and the algorithm in the golem model is based on sine or cosine to create a smooth up/down motion all based on that one timer. As others have suggested, your best bet is to literally just sit and read through the vanilla code for an hour or however long it takes you to understand how it works, and then do something similar. Keep in mind that any variables you have in your entity are probably only known on the server side; thus, if you want to use them in your animation, you need to somehow inform the client of their current value. The method I described above is quite useful for most situations, or you can use DataWatcher or custom packets if you are in need of more precise control.
-
It's not very elegant, but the way I did it is to subscribe to LivingAttackEvent, check if the attacking entity (event.source.getEntity()) is under the 'stun' effect and, if so, cancel the event. That will at least prevent mobs from doing damage that way. As for creepers, there is now an explosion event of some kind that you should be able to cancel - I haven't used it yet, but I bet it has everything you need. There is also the cancelable EnderTeleportEvent which, as you might guess, you can use to prevent teleportation. This may or may not work for modded mobs, depending on whether the mod author follows convention and posts the event for their custom entities or not. Lots of events, as you can see
-
[Resolved]get were the player is looking 1.7.10
coolAlias replied to BoonieQuafter-CrAfTeR's topic in Modder Support
To quote myself in answer to your question: You didn't listen the first time, so perhaps the second? -
[Resolved]get were the player is looking 1.7.10
coolAlias replied to BoonieQuafter-CrAfTeR's topic in Modder Support
Yeah, that's the one: Vec3.createVectorHelper. Arguments are the coordinates of the vector you want to create. I don't know what you mean by 'defines the other int' - what int? Listen, I understand you are "desperate" to get this working, but perhaps a time-out to learn about vectors would be helpful? That and going through some basic Java tutorials, of course. -
[Resolved]get were the player is looking 1.7.10
coolAlias replied to BoonieQuafter-CrAfTeR's topic in Modder Support
Not to be mean, but you clearly don't understand what @Override actually does - it is simply an extra layer of error-checking within the IDE - it has no effect whatsoever on the compiled code. @OP You probably want to pay more attention to what you are writing in your code: Bolt.setPosition(player.posX = x , player.posY + y , player.posZ + z);; Surely you meant plus (+) and not equals (=) ? Also, why are you adding them at all? The raytrace MovingObjectPosition returns the block position that was hit, as in real world block coordinates. If you add the player's position to that, you will get very bizarre numbers. Example: Player is at 100,64,100 looking east (+x axis); MOP returns a block at 100,64,115 (directly east). Now you add those together, and spawn lightning at 200, 128, 215. Do you really expect to see it? -
[Resolved]get were the player is looking 1.7.10
coolAlias replied to BoonieQuafter-CrAfTeR's topic in Modder Support
There is no Vec3Pool any more, just use Vec3 whatever = new Vec3(args); Or, if you don't have that version yet, Vec3Helper.createVector or whatever it used to be. -
Caching the quads actually occurred to me just as I walked out the door for work after I posted this, and it works perfectly well so that's what I'm doing now. Haven't tested to see if it's any smoother in Minecraft vs. dev environment yet, though. Can you clarify what you mean by n/d quads and 'rookie's mistake' ? I'm not sure what you mean, except perhaps that I forgot to break out of the loop as soon as I found the quad that I wanted. Still, the mystery remains: why would my initial code be so much slower outside the dev environment?
-
Some of you may have seen my previous post about getting shields to render correctly - that works perfectly now, but when I played my mod with actual Minecraft rather than via Eclipse, every time I held the shield, especially in first person, it would cause massive client-side lag. This lag does not happen in the development environment. All I'm doing is adding one quad during getGeneralQuads(): Nothing out of the ordinary, right? Then in my Item class, I override getModel to return a different model location based on whether the player is using the item or not, but these locations are the same ones already registered: I have other items that do somewhat similar things, or things I would imagine would be even more resource intensive, but those all work smoothly. Is there any reason that the above code would cause lag only when playing via the actual launcher?
-
[1.7.10] Item onRightClick returning the same thing
coolAlias replied to KakesRevenge's topic in Modder Support
Well, you can't have two stacks in one inventory slot, so you'll need to put one of them somewhere else. You can use player.inventory.addItemStackToInventory(new ItemStack(whatever)) to do just that, and then return the original stack as you have it there. -
[1.7.10] Item onRightClick returning the same thing
coolAlias replied to KakesRevenge's topic in Modder Support
See that the return value is an ItemStack? Return a different one. The one you have, 'stack', is the ItemStack that called the method. -
See my edit.
-
Did you override Block#damageDropped(IBlockState) to return a non-zero value? EDIT: Actually, sorry, I meant in your ItemBlock, you need to override public int getMetadata(int damage) just like ItemMultiTexture does to return the actual damage value. The Block one is used mainly for pickBlock / item drops when harvested.
-
EDIT: Closed. Thanks Lex! I've tracked this down to ItemRenderer#renderItemInFirstPerson which is the only place that actually uses the equippedProgress and prevEquippedProgress to affect how the item is rendered. I've got an item that I don't want to have this 'animation' - I want it to appear in place just as it does in 3rd person, right when the player switches to it (it's actually to accommodate changing NBT [and no, I can't store it elsewhere in this case]). Has anyone ever come up with a solution for this? If not, how about the following solution: EDIT: The following solution is much better than the one in the spoiler above: Another possible solution would be to have something like Item#getFirstEquippedProgress(ItemStack current, ItemStack previous, float equippedProgress) at this point: if (this.equippedProgress < 0.1F) { this.equippedProgress = itemstack.getItem().getFirstEquippedProgress(itemstack, this.itemToRender, this.equippedProgress); this.itemToRender = itemstack; this.equippedItemSlot = entityplayersp.inventory.currentItem; } I added in the current equippedProgress so the default Item implementation has an easy return value, and note that returning 1.0F will prevent any unwanted equip animation - heck, it could even just be a boolean return value, really, but the float gives more control. Thanks for reading, coolAlias
-
[1.8] How to get config directory? [SOLVED]
coolAlias replied to JoelGodOfWar's topic in Modder Support
Couldn't you just use your language file, then? -
[1.8] [SOLVED] Dyed Armor crafting recipe
coolAlias replied to ADaringEnchilada's topic in Modder Support
Another solution is to implement an IRecipe for your items, then all you have to do to add all of the dye recipes is this: CraftingManager.getInstance().getRecipeList().add(new YourIRecipeImplementation()); CraftingManager.getInstance().addRecipe(new ItemStack(YourItems.yourItem)," X ", " X ", "XXX", 'X', new ItemStack(Blocks.wool, 1, OreDictionary.WILDCARD_VALUE)); // or whatever the recipe is Granted, the IRecipe implementation for dyes is a bit more verbose than your solution, but it ends up looking cleaner, imo, and you could easily generalize it to account for any variety of items by creating an IDyable interface or some such. -
[1.8] How to get config directory? [SOLVED]
coolAlias replied to JoelGodOfWar's topic in Modder Support
I'm not quite sure what you are trying to accomplish with this particular line of reasoning, but you'd need to figure out a way to open the .jar file contents (e.g. 7-zip utility to open it as an archive) and find the file you want within that directory structure. Is this text file supposed to contain configuration settings for your mod that are not user-modifiable? If so, you are still hard-coding it because you have to change the text file - why not just set the values you want in your code? If it is supposed to be modifiable by users, why place it in your .jar file when you can use the mod configuration directory and open / modify whatever file you want there?