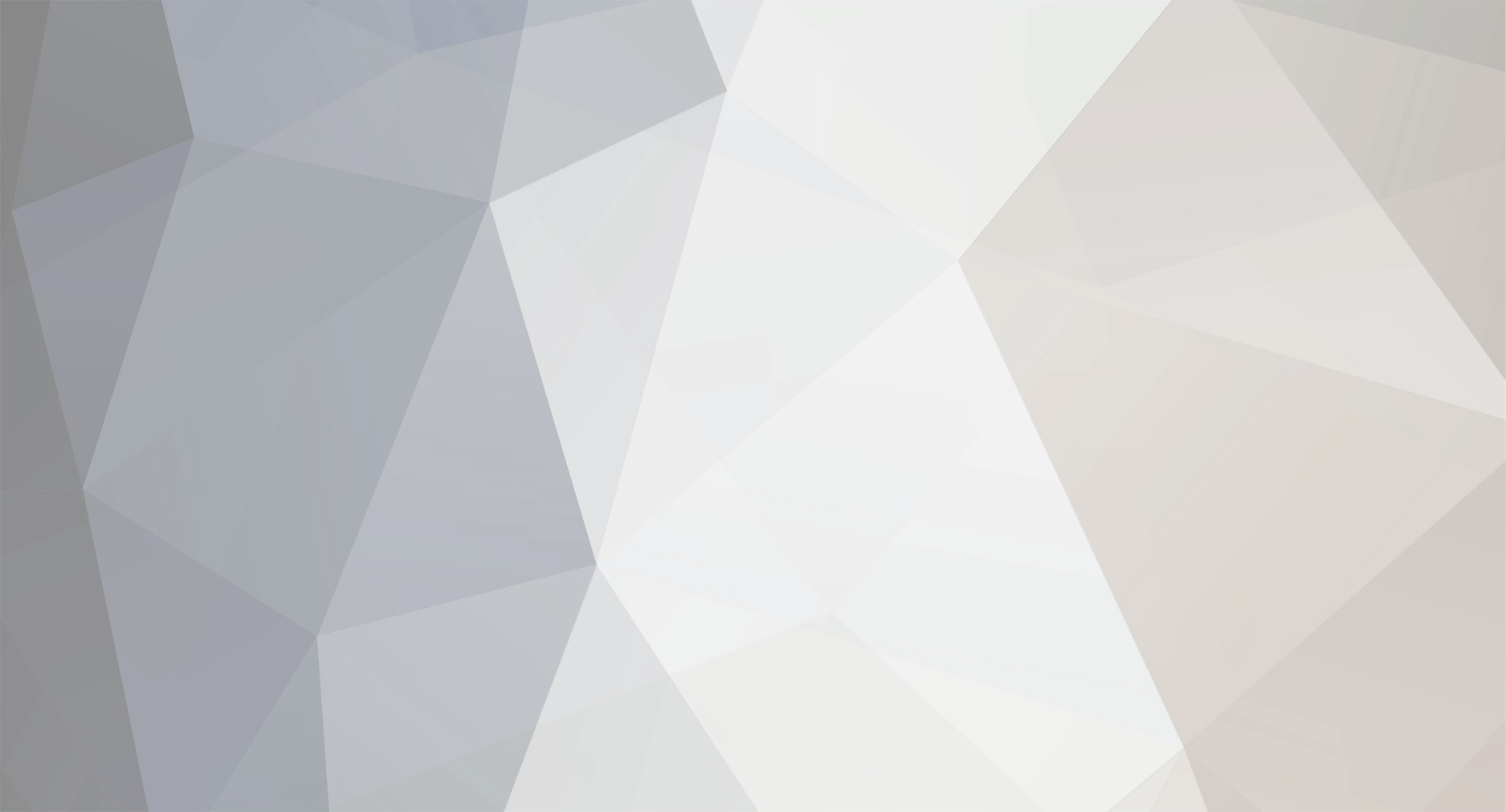
coolAlias
-
Posts
2805 -
Joined
-
Last visited
Posts posted by coolAlias
-
-
Wonder if it has anything to do with not using your modid when registering the sounds?
Otherwise, your last bit of code didn't have the change I suggested above - does it work if you use your modid both when registering and for the sound key in your sounds.json?
-
It's still looking in the Minecraft assets folder rather than your mod's. Maybe try prefixing your sound keys with your mod id:
"tetracraft:entity.terrakon.bark": {"category": "neutral", "sounds": ["tetracraft:entity/terrakon/bark1", "tetracraft:entity/terrakon/bark2", "tetracraft:entity/terrakon/bark3"]},
-
I don't mean to stream them since their sounds for my entity, do I have to have stream marked true?
No, you don't. Have a look at mine. Do note that it is for 1.8, though, so some things may have changed - it should still give you a general idea of what you need.
-
i get the feeling this is not the proper way to do it or am i wrong?
No idea - I generally abhor video code tutorials, and I'm certainly not spending 34+ minutes watching this one.
What specifically about Containers do you not understand? Or by 'container', do you mean the entire item storage / display system?
For a system like a chest, you need:
1. A Block to provide the TileEntity and open the GUI when activated
2. A TileEntity to store the inventory contents
3. An IInventory implementation to handle interactions with the inventory
4. A GUI to display the inventory contents
5. A Container to keep the client-side version of the IInventory in sync with the server while the GUI is open
6. An IGuiHandler to provide the correct GUI and Container when you call #openGui
I might be forgetting something, but each of the above has multiple steps and several have specific registration requirements, and none of it will work if your base mod class isn't set up correctly. Needless to say, this is a HUGE undertaking for a new modder.
While it's not impossible, I highly suggest you start with something simpler and work your way up. For example, start with a non-interactive Block. Then an interactive Block (doesn't have to be fancy). Then try a basic GUI - one that doesn't have an inventory, but is rather just a way to display some info. Etc.
-
You don't need terrakon.bark - that's the sound registry key (well, technically yours is labeled 'mob.terrakon.bark') that maps to the possible sounds, e.g. tetracraft:entity/terrakon/bark1, tetracraft:entity/terrakon/bark2, etc.
So it should look like:
"mob.terrakon.bark": {"category": "neutral", "sounds": [{"name": "tetracraft:entity/terrakon/bark1", "stream": true}, {"name": "tetracraft:entity/terrakon/bark2", "stream": true}, {"name": "tetracraft:entity/terrakon/bark3", "stream": true}]},
At least, I think - I haven't done streaming sound variants before, but that's how it works for other sounds.
-
I don't know that I would say unchangeable, but perhaps unlikely to change.
-
To be fair, the blocks in the for loop are not included in the preceding if statement, but that does not mean the for loop has a point.
Just think about it step by step:
for loop breaks down to this, basically: if (block correct and 0 == meta) return true; if (block correct and 1 == meta) return true; if (block correct and 2 == meta) return true; // and on up to 15
So, if at any point the metadata value is the same as i, you return true. The metadata value can only be a value from 0 to 15, and you check ALL of those values, so by very nature of the code, at some point i has to equal the metadata value, making the metadata value and thus your for loop completely unnecessary.
It literally does nothing different than simply checking if (block == clayWall) for those 3 blocks.
-
And if you're using Eclipse, it's about the same. There is also a small 'bug' icon next to the run button.
You should really set up a run configuration so you can test directly from within your IDE - you are using an IDE, right?
-
Interesting - I suppose I haven't ever tried it while actually moving, so... dang.
I wonder if that's why you have to stand still in the Nether Portal for a few seconds before teleporting? Lol. Maybe you can force the client-player velocity to zero either right before or right after moving? Might not work due to network lag, but it might alleviate it. Surely there is a real solution, though.
-
I believe itemInstance.getRegistryName() should return the full name including the modid, but I haven't set up a 1.9 workspace yet so I'm just guessing.
-
They could very well change if you add or remove a mod after the world is created.
-
Make a GUI the players can access either any time or by using an Item or Block.
Here is an outdated but still quite useful tutorial that explains a lot of things about how GUIs work, such as how you can have multiple pages, etc. I highly recommend it even in light of its age.
-
IDs have been completely removed (well, hidden). You should not have any reason to use them.
In your particular case:
if (stack == this.itemID) { // becomes: if (stack.getItem() == this) {
-
If you load your World with the same set of mods as the last time you loaded it, the Block IDs will probably be the same as the last time. I don't see any harm in trying it out in a 3rd party piece of software, since it sounds like it is not actually interacting with Minecraft while it is running.
However, there is no guarantee that the block IDs for that world will match a new world, even with the exact same mods.
-
They are the same as 1.7.2 Containers, which are the same as 1.6.4 Containers, which are probably the same as Containers in previous versions. Containers have been pretty stable over the various versions up to at least 1.8.9.
That all said, if you want to see one that is for your current version, check out vanilla Minecraft's ContainerFurnace - it does pretty much everything a Container can do. If you don't understand any part of it (e.g. #detectAndSendChanges or #addCraftingToCrafters), look for ANY Container tutorial and it will probably explain it.
-
Custom AI, specifically a custom Navigator (might be named something slightly different - I don't recall exactly).
Alternatively, you could first attempt overriding #getBlockPathWeight in your entity class and return 0 for any non-snow / non-snow-layer blocks. That should prevent your entity from pathing onto non-snow covered blocks.
-
That's my guess as well - it probably IS rendering, just not somewhere you can see it.
Run your mod in debugging mode so you can play with the GL translate and rotation values (among others) in real time.
-
Entity#setVelocity is client-side only due to the way the code is compiled / decompiled; since it is only ever called on the client side, it gets marked with the @SideOnly annotation.
To teleport entities, I prefer to use #setPosition; then I check if it's an EntityPlayer and if so, I additionally call #setPositionAndUpdate to make sure the client player gets the memo.
Works for me without ever getting that error message.
-
You set custom state mappers at the same time you register your block models, typically from the ClientProxy (or a method call originating from there). You don't have to implement anything special in your Block class to do so.
-
Your for loop is kinda... pointless.
for(int i=0; i<16; i++){ if ((block==TemBlocks.claywalls && meta ==i) || (block==TemBlocks.claybrickwalls && meta ==i) || (block==TemBlocks.brickswalls && meta ==i) ){ return true; } }
If you think about what that is doing, i starts at 0 and goes all the way to 15, so every value less than 16, which is every possible metadata value for a Block... why even check?
And if you DO want to check meta for some reason, why do it in a for loop when you can just check it like this:
if (meta < 16 && (block==TemBlocks.claywalls || block==TemBlocks.claybrickwalls || block==TemBlocks.brickswalls)) { // the block is one of the ones you want and the meta is from 0 to 15... // same exact logic as your for loop, but far more efficient and succinct }
-
Your mod instance is missing its annotation; it should be like this:
@Mod.Instance(TESItems.MODID,) public static TESItems instance;
Also, you are handling the FMLInitializationEvent THREE times... you should only do so once. There are also FMLPreInitializationEvent and FMLPostInitializationEvent events which you can use.
Do NOT use @SideOnly in your Main class. Ever. Use the proxy system, which you do not have at all in your mod...
-
I register it from
@EventHandler
public void preInit(FMLInitializationEvent event)
with NetworkRegistry.INSTANCE.registerGuiHandler(this, new CustomPlayerGUIHandler());
Why is your FMLInitializationEvent handled in a method named 'preInit'? Is that an accident?
Anyway, can you show your entire Main class? Something in there is not right.
-
Jabelar goes into some detail on how to render a sphere in one of his tutorials - might be worth checking out.
-
Where did you register your IGuiHandler from? It should be in your Main class during one of the FML init events (I usually use Init, but I don't think it really matters).
[1.9] NBT change resetting block break
in Modder Support
Posted
Nope, you can't effectively sub-class ItemStack to add your own stuff, though you can with EntityItem.