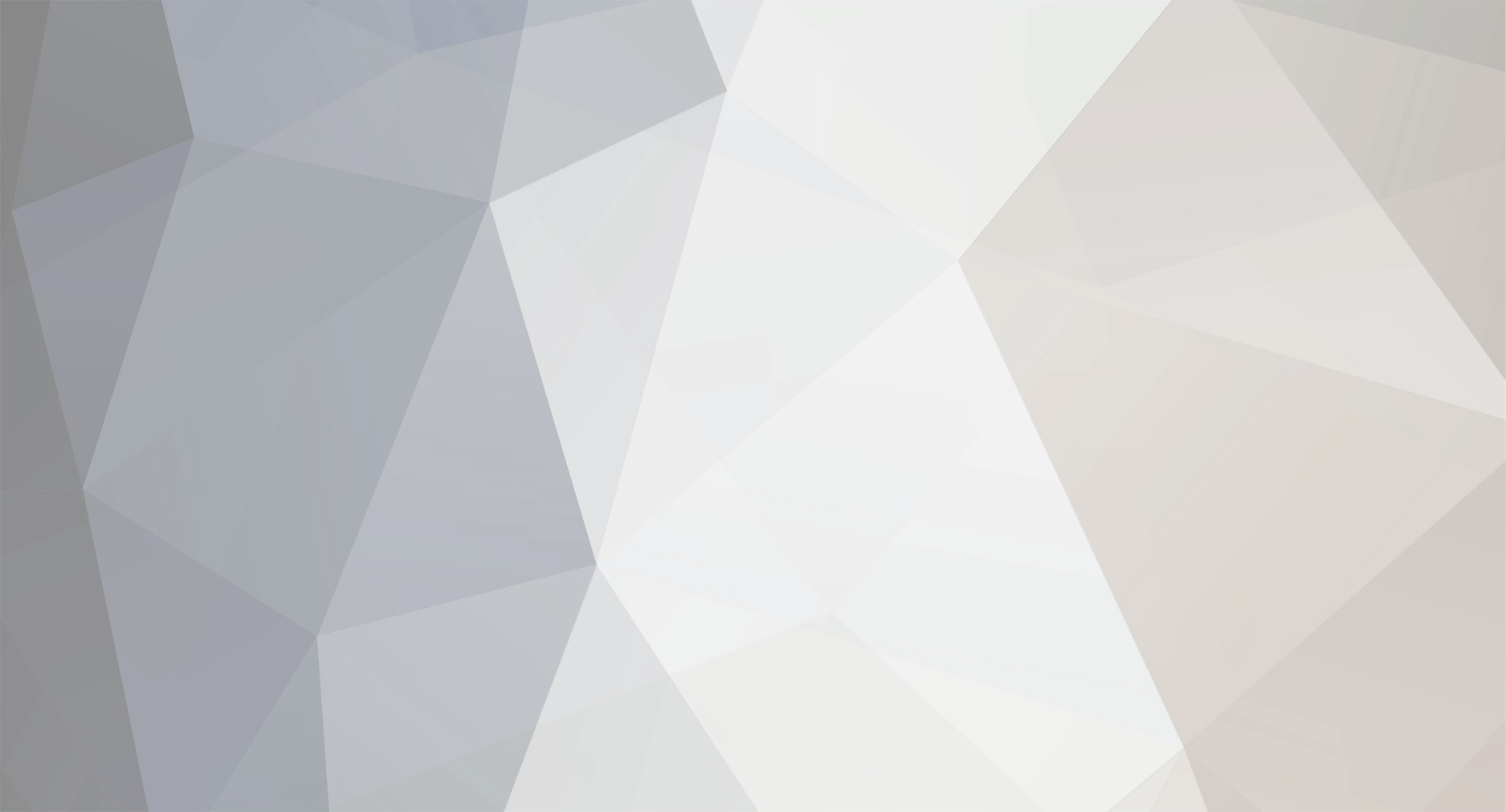
coolAlias
-
Posts
2805 -
Joined
-
Last visited
Posts posted by coolAlias
-
-
I highly doubt that's going to work - ray casting is used to fetch 'real' objects in the world that the player is looking at, not elements in a GUI. Also, a ray cast only ever returns a Block, an Entity, or null, so the best you could expect would be to get the client player being rendered, and that's usually Minecraft.getMinecraft().thePlayer.
Basically, if you're trying to determine specific parts of the player model that are being clicked, your approach is not going to work.
You may be able to estimate based on the current mouse position in relation to where the standard model biped is displaying within your GUI: e.g. if your display box starts at (0, 0) and goes to (100, 50) and the model renders from (10, 10) to (90, 40), then if the mouse is between say (15, 10) and (35, 40), you could assume the mouse is within the head region.
You should probably explain what you are actually trying to accomplish.
-
I'm not familiar with how Forge's in-game config GUIs function, but it seems to me like calling #loadConfig again would overwrite any changes you may have made from the GUI with the values from the actual file.
Speaking of which, GUIs are client-side only, so any config settings that you allow to be configured from there either need to only be used on the client side, OR you need to have a way of getting the new settings to the server so the config file on the server (which may or may not be on the player's computer) can actually save them.
Take a look at Battlegear2, for example, specifically their GUI's #onGuiClosed method, and their Config class' #refreshConfig method.
-
Try returning true instead of false within your if statements - that signals Minecraft that something happened.
-
PlayerTickEvent should occur on BOTH client and server - where are you registering your event handler from?
-
Since 1.8.9, all of the event buses have been consolidated so there is only one bus, the MinecraftForge.EVENT_BUS.
As for the block damage, yeah, that can work, or you could check if motionX^2 + motionZ^2 is greater than a certain threshold and not worry about sneaking at all.
-
Show your code - it should look something like this:
@Mod.EventHandler public void onServerStarting(FMLServerStartingEvent event) { event.registerServerCommand(new YourCommand()); // or: event.registerServerCommand(YourCommand.INSTANCE); }
-
Hm, the Forge version shouldn't affect it, no. Are you getting any interesting messages in your console log?
Are your skeleton aids stuck in the ground at all? I notice you don't really adjust the y position other than +1, but with the random x/z position, that may not be enough.
Otherwise, your spawn code looks pretty standard, especially if your entity is working as expected otherwise... I dunno, maybe show the code that calls the #wakeTheDamned method?
-
One thing is that the way you are registering your entity renderer, you need to do it during the FML init event, not pre-init, because the render manager should be null during pre-init. How are you not crashing?
-
You either click the '#' symbol in the toolbar or manually type [ code ] your code here [ /code ] without the spaces.
As for your issue, you need to set the block state with the appropriate AGE property, e.g.
world.setBlockState(pos, state.withProperty(AGE, 1));
Note that the actual code may be slightly different - I don't have an IDE in front of me.
-
Those types of GUIs use the Container as an intermediary between the server and the client.
If you take a look at the ContainerFurnace class, you will see how it calls the IInventory methods as well as #detectAndSendChanges and another method like #addCraftingToCrafters - the former is the one responsible for updating the GUI values from the TE values, and the latter is one that typically adds players that need to be informed of changes.
Ideally, your TE-based Container/GUI combo wouldn't ever need to explicitly send messages or block updates, but would all be handled via the Container.
-
Post your EntitySacrificialSkeleton code, I'm assuming it has something to do with
onSpawnWithEgg(null)
Nah, that method rarely gets passed a non-null object, though I suppose the skeleton / custom implementation of it might be responsible?
@OP Mind posting your entity code?
-
No registry name set for object guru.tbe.item.ItemAirOrb@7747c (guru.tbe.item.ItemAirOrb)
You need to set the registry name of your Items before registering.
Use itemInstance.setRegistryName("item_name"), not the unlocalized name.
-
Please post the crash report as well.
May I also suggest that you use standard Java naming conventions? Specifically, variables should start with a lower-case letter - airOrb instead of AirOrb - because AirOrb would be the name of a class.
-
Going from memory:
client side: player.currentScreen
server side: player.openContainer
Those will give you the actual GUI and Container instances, if any, which you can cast to yours and do whatever you want.
Also, since GUI screens are client-side only, you could technically get away with using static fields, e.g. MyGui.field = 42, but keep in mind that it is almost always better to use an actual instance of the class and non-static members.
-
* Mark all (I think you can even mark @Mod, but idk) your classes with @SideOnly(Side.SERVER) - classes will be present only on dedicated server.
I just want to stress the bolded portion there - marking your classes @SideOnly(Side.SERVER) will preclude the use of your mod in single player.
Is that really what you want? In the vast majority of cases, there isn't any reason to make a mod specifically server-side only. You could just as easily make it a general mod that performs its duties in the various mod init phases, events, etc., all running on the logical server i.e. when the World object is not remote, and it will have the same effect but be usable also in single player. Just a thought.
-
Looking at this all in retrospect, would I just use static fields to get the information to other classes, such as my gui class in this scenario?
Absolutely not. They will not do what you think they will.
Each IMessage is created once when you send it, then a new instance is created on the other side and reads the data sent over the network. Once that's done, an instance of the IMessageHandler is created and the message passed to it.
So, if you send a message in return, it will be recreated on the other side and another new instance of the IMessageHandler created to handle that.
This is why a single handler can handle both sides, but if doing so you have to check which side you are on before doing anything if your message behaves differently on different sides.
When a single message can be handled on both sides, you need to register it twice - once on each side - and using the same discriminator byte (at least that's what I've found).
However, it's somewhat rare for a single message to be useful on both sides, so you may want to carefully evaluate whether one message type is sufficient, or whether you really need two.
-
If you read the crash log, you will see it:
cpw.mods.fml.common.LoaderException: java.lang.NoClassDefFoundError: net/malisis/core/network/MalisisNetwork
Whatever mod 'malisis' is, that is your culprit.
-
Sounds like you are setting the block on the client side - only ever call setBlock on the server side, i.e. when the world is not remote.
if (!worldObj.isRemote) { // logical server - okay to set block worldObj.setBlock(...); }
-
ClientTickEvent itself isn't doing anything - it is posted twice per Minecraft run tick on the client side, from the Minecraft class. The first time is the START phase, and the second time is the END phase.
Since it is CLIENT side only, any player data retrieved at this point must have been kept updated from the server side via packets. Several things are kept in sync for you by Minecraft, but most things are not because they are not normally needed client-side.
What are you trying to do that made you look at ClientTickEvent?
-
Sorry, you don't actually use the Container directly - send the TE coordinates in the packet, as Draco mentioned, then use those to fetch the TE and add the stack to the inventory. If your inventory is coded correctly, the Container will notice the changes and send an update to the client.
If it's your first time using packets, I suggest following diesieben's tutorial to get the basics down; if you end up making lots of packets and get sick of writing IMessageHandler classes, then take a look at my tutorial.
I'm not sure what you mean about linking a world to a URL - could you explain that more?
-
@jeffryfisher Or if he doesn't want anyone nearby to hear and figure out that he's using a hoe, which is what I assumed*
* given that he was wanting to play the sound on the client, but perhaps that was the only way he found? or is that how vanilla tools or just the hoes behave? I don't have an IDE to check right now, but I kinda think that tool sounds are client only - do you actually hear other players chopping and mining? Not that I recall, but it's been ages since I've played.
-
I only meant to set persistence for the purpose of testing, not permanently
I don't recall where you can find the vanilla spawn rates, but most of them are in the range of 10 to 100 if I recall correctly. For my own mobs, I tend to give them spawn rates of 5-10 and they spawn regularly enough to be fun, but aren't an overwhelming presence.
I'd just play around with it until you find something you like to use as the default, and then give your users the option to set it to what they like in via your configuration file.
-
There may other checks going on that prevent it from spawning after #getCanSpawnHere, though one would think that the spawn event would be correct. That's odd.
It's possible that it is simply despawning before you get there. Try setting the entity to be persistent, or return false for #canDespawn.
Alternatively, you could try increasing your entity's spawn rate to something ridiculous, like 10,000, just to see if it will spawn.
Unrelated to your issue, but in 1.8.9+ (and maybe earlier), EntityRegistry#registerModEntity optionally takes additional parameters for the egg colors and will automatically register the spawn egg for you; it is recommended to use only that method and not add spawn eggs manually.
-
No, most definitely do NOT use the TE description packet for this - that is not what it is designed for.
Whenever you have a client-side action, such as a GUI button, that you want to change something on the server, you need to send your own custom packet that only knows what action the player requested, e.g. clicked GUI button 'X', and then when that packet is handled on the server, you validate the action, e.g.:
- is the player interacting with the appropriate container / TE for this to happen?
- is the player allowed to press that button?
- etc.
Once you determine the action is allowed, then you can alter the inventory contents via the server side Container, which will automatically update the client side view.
IF you are sure the action will succeed and IF you want your GUI to be extremely snappy, you can go ahead and update the inventory client-side first before you send the packet, BUT NEVER let the client-side dictate what the server-side values are. That is, still do the server side validation before altering the inventory, and let the server update the client with the correct values (which should be the same, but you never know).
Minecraft Forge 1.8 Throwable Entity Not Rendering
in Modder Support
Posted
You are missing the closing brace.