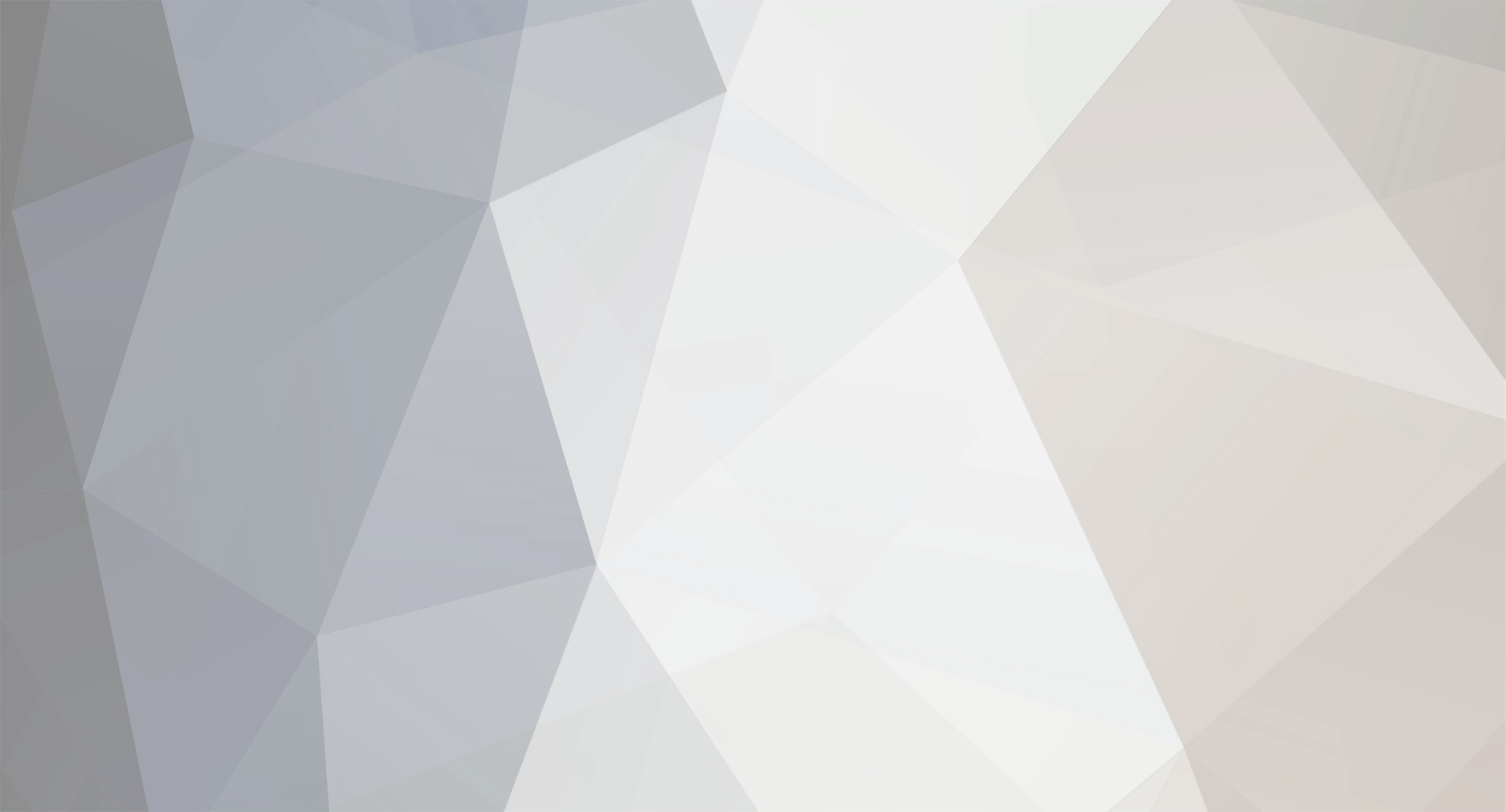
shadowmage4513
Forge Modder-
Posts
157 -
Joined
-
Last visited
-
Days Won
1
Everything posted by shadowmage4513
-
Entity wont spawn when created in GUIScreen
shadowmage4513 replied to shucke's topic in Modder Support
This is because everything that happens in a non-container GUI happens client-side only. You will need to enact some sort of packets/packethandlers--and when the client pressses the button/does the action to spawn the entity--send a packet to the server and have the server do the spawning. You will need to send whatever information you need from the GUI, as the server has no idea you even have GUI open (it doesn't deal with GUIs--they are client-side only). -
Block Break Event and NBTTagCompound Tag Key iteration?
shadowmage4513 replied to Xulai's topic in Modder Support
Thirded! They seem so common sense, I thought they already existed and was looking for them earlier in my Forge dist to no avail. -
Aye, I think this should all be able to be handled in the blockbreak/blockharvest event, or even in the itemtool itself (overriding one of the methods). You should basically be able to tell the game to spit out your item whenever a dirt block is broken with your tool. I'll try and take a closer look and see if I can find the specific methods/etc. Ahh, yes..in Item.java: public boolean onBlockDestroyed(ItemStack par1ItemStack, World par2World, int par3, int par4, int par5, int par6, EntityLiving par7EntityLiving) { return false; } implement an override here, and make either the player drop the item, or merely spawn it in the world. You might even be able to call the block-drop method to have the block spit it out. You will have to check that the block destroyed==Block.dirt or ==Block.grass (by calling world.getblockID(x,y,z) and comparing that to dirt/grass blockIDs). Either way..no base-edits. No block overrides. No compatibility issues. Please let me know if it helps.
-
From a quick glance: You are only opening your GUI on one side. The correct call for opening GUIs is FMLNetworkHandler.openGui(EntityPlayer player, <mod_class> mod.instance, int GuiID, World world, int x, int y,int z); It must be called both client AND server side simultaneously (or as close as possible) in order for the GUIs to open, and the server to be aware of what GUI you are interacting with (populate the proper containers for both client/server side). So..in your block class..change public boolean onBlockActivated(World par1World, int par2, int par3, int par4, EntityPlayer par5EntityPlayer, int par6, float par7, float par8, float par9){ if(!par1World.isRemote){ return true; } Object obj = (TileEntityRocketAssembly)par1World.getBlockTileEntity(par2, par3, par4); //ModLoader.openGUI(par5EntityPlayer, new GuiRocketAssembly(par5EntityPlayer.inventory, (TileEntityRocketAssembly)(obj))); par5EntityPlayer.openGui((Object)BaseRocketEngineering.instance, 0, par1World, par2, par3, par4); return true; } to: public boolean onBlockActivated(World par1World, int par2, int par3, int par4, EntityPlayer par5EntityPlayer, int par6, float par7, float par8, float par9) { TileEntityRocketAssembly obj = (TileEntityRocketAssembly)par1World.getBlockTileEntity(par2, par3, par4); if(obj!=null) { FMLNetworkHandler.openGui(par5EntityPlayer, BaseRocketEngineering.instance, 0, par1World, par2, par3, par4); } return true; } Hope that helps. I recently fought with this issue myself with multiple different GUIs. Now--I think I've a pretty good idea on whats going on. Shadowmage
-
I was recently watching a discussion on this topic, can't quite remember where (redpower forums, or slowpoke's twitch...who knows)... And it looks/sounded like the best way to implement this would be to implement a transparent light-emitting block that follows the player around. It sounded like it was mostly a workaround for a lack of moving/positional lights in MCs lighting engine. Apparently there have been _some_ mods that did moving lights, but it was said they were extremely laggy. While its not telling you _how_ to do it, hopefully this can offer some insight as to how you should tackle your problem. Shadowmage
-
Yes, it pretty much gets the direction you are looking, does some vector math, then grabs a MobileObjectPosition to do a proper ray-trace. This ray trace will get the block that is hit. The code I posted wasn't cleaned up very much, and was pretty much taken out of one of the vanilla items....a boat I think? I guess the important lines are: float var4 = 1.0F; float var5 = player.prevRotationPitch + (player.rotationPitch - player.prevRotationPitch) * var4; float var6 = player.prevRotationYaw + (player.rotationYaw - player.prevRotationYaw) * var4; double var7 = player.prevPosX + (player.posX - player.prevPosX) * var4; double var9 = player.prevPosY + (player.posY - player.prevPosY) * var4 + 1.62D - player.yOffset; double var11 = player.prevPosZ + (player.posZ - player.prevPosZ) * var4; Vec3 var13 = Vec3.getVec3Pool().getVecFromPool(var7, var9, var11); float var14 = MathHelper.cos(-var6 * 0.017453292F - (float)Math.PI); float var15 = MathHelper.sin(-var6 * 0.017453292F - (float)Math.PI); float var16 = -MathHelper.cos(-var5 * 0.017453292F); float var17 = MathHelper.sin(-var5 * 0.017453292F); float var18 = var15 * var16; float var20 = var14 * var16; double var21 = 5.0D; Vec3 var23 = var13.addVector(var18 * var21, var17 * var21, var20 * var21); MovingObjectPosition var24 = world.rayTraceBlocks_do(var13, var23, true);///this right here holds hit values. if it is not null, there was a hit. it will contain info for what type of hit (entity/tile), as /////well as exact coordinates for the hit. //// if(var24!=null) { if(var24.typeOfHit == EnumMovingObjectType.TILE) { ////then var24.blockHitX~Z will contain the coordinates of the block hit } }
-
in an Item /** * Called whenever this item is equipped and the right mouse button is pressed. Args: itemStack, world, entityPlayer */ @Override public ItemStack onItemRightClick(ItemStack stack, World world, EntityPlayer player) { float var4 = 1.0F; float var5 = player.prevRotationPitch + (player.rotationPitch - player.prevRotationPitch) * var4; float var6 = player.prevRotationYaw + (player.rotationYaw - player.prevRotationYaw) * var4; double var7 = player.prevPosX + (player.posX - player.prevPosX) * var4; double var9 = player.prevPosY + (player.posY - player.prevPosY) * var4 + 1.62D - player.yOffset; double var11 = player.prevPosZ + (player.posZ - player.prevPosZ) * var4; Vec3 var13 = Vec3.getVec3Pool().getVecFromPool(var7, var9, var11); float var14 = MathHelper.cos(-var6 * 0.017453292F - (float)Math.PI); float var15 = MathHelper.sin(-var6 * 0.017453292F - (float)Math.PI); float var16 = -MathHelper.cos(-var5 * 0.017453292F); float var17 = MathHelper.sin(-var5 * 0.017453292F); float var18 = var15 * var16; float var20 = var14 * var16; double var21 = 5.0D; Vec3 var23 = var13.addVector(var18 * var21, var17 * var21, var20 * var21); MovingObjectPosition var24 = world.rayTraceBlocks_do(var13, var23, true); if (var24 == null) { return stack; } else { Vec3 var25 = player.getLook(var4); boolean var26 = false; float var27 = 1.0F; List var28 = world.getEntitiesWithinAABBExcludingEntity(player, player.boundingBox.addCoord(var25.xCoord * var21, var25.yCoord * var21, var25.zCoord * var21).expand(var27, var27, var27)); Iterator var29 = var28.iterator(); while (var29.hasNext()) { Entity var30 = (Entity)var29.next(); if (var30.canBeCollidedWith()) { float var31 = var30.getCollisionBorderSize(); AxisAlignedBB var32 = var30.boundingBox.expand(var31, var31, var31); if (var32.isVecInside(var13)) { var26 = true; } } } if (var26) { return stack; } else { if (var24.typeOfHit == EnumMovingObjectType.TILE) { int var42 = var24.blockX; int var43 = var24.blockY; int var44 = var24.blockZ; System.out.println("hitX: " +var42+" hitY: "+var43+" hitZ: "+var44); System.out.println("hitXMax: " +(var42+1)+" hitYMax: "+(var43+1)+" hitZMax: "+(var44+1)); } return stack; } } } That code in an item will return the min/max bounds of the block you clicked on (if a block was present)..I.E. the block bounded by the block-pick-outline. Wont' work if an entity is in the way, or the block has an onBlockActivated method. But gets you basic x,y,z coords of the block clicked otherwise. Really..you can use similar code anywhere to get the block the player is looking at, not just in an item...I have it there because I was using the item to print meta-data for whatever block I clicked on (just modified the output for your purposes). You could then call world.setBlockWithNotify(x,y,z,blockID); to update the block clicked to whatever you want. Hope that helps
-
Nobody with any experience overriding vanilla network move-code? I guess I'll give it a go and let you all know how it went, and the steps necessary if it works. Basically, I just want to keep network traffic down and completely stop the sending of vanilla move-packets for my custom entities. Thanks,
-
Hi all. I'm currently writing a mod involving some mountable vehicle-type entities. My issue is that the stock minecraft MP movement code is...well...terrible. I've pretty much overriden the entire vanilla movement packet stuff with my own. The problem is, the vanilla packets are still sent, I just ignore them (technically, I've overridden setPositionAndRotation2 with an empty method ). My movement packets are tiny. They consist of two bytes to transmit the input from the player to the server and relay it to other players. Occasionally I send a synch packet to make sure position is lined up between server and players. So, the real question: What are the downfalls of not registering entities with EntityRegistry.RegisterModEntity()? What it does is handle the initial spawning of the entities, and adding them to the Vanilla network entity tracking list. If I do NOT register my entities with the EntityRegistry.registerModEntity(...) and handle things manually, the only problem I can see is the initial spawn information won't be sent. I don't think this will be an issue because I am blanking out the method it uses to set everything anyway. It would mean I would have to manually spawn the entities into the client world (likely using the same FML methods used by the original packet). TLDR: My move code works great, but vanilla packets are still being sent even though I just ignore them. Can I un-register the entity with FML? (but leave the other registerGlobalEntityID, so it will save/load from NBT still). Anyone else have experience with complete custom entity network movement code and overriding vanilla behavior? Thanks