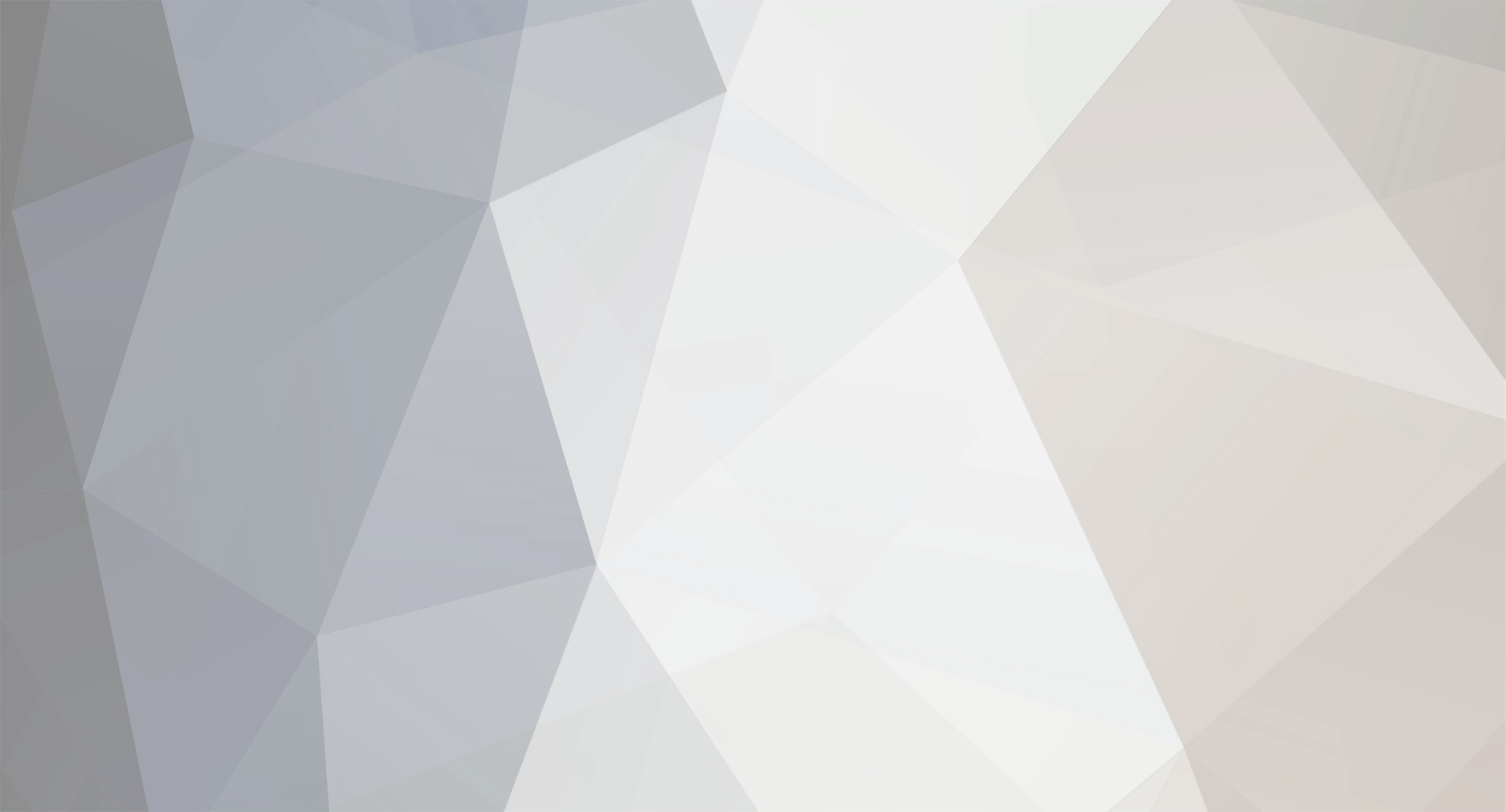
jabelar
Members-
Posts
3266 -
Joined
-
Last visited
-
Days Won
39
Everything posted by jabelar
-
I think you can handle the harvest block event.
-
To use the debugger, you need to use the debug run configuration. If you do that, then the execution (for that thread) will actually stop when the breakpoint is reached. You can usually tell because in Eclipse instead of a bunch of updating the information will sort of stop updating. So if you had a breakpoint set for onBlockActivated() you would play the game and watch the debug window and then at the moment of the block activation you should see it sort of capture the information in the debug windows. Once the execution is frozen, you can inspect the field values. That should be in another pane in the Eclipse debug interface. Then there are special buttons on the toolbar for stepping through the next lines of code. So you can go one line at a time. The difference between the various step buttons is sometimes you want to go right into the various methods and other times you trust a method so want to let it execute completely as a single step. It's kinda complicated to explain, but should be sort of obvious if you do it and watch what is happening. Also, just look up tutorials on Eclipse debugger as this is just standard Java and has nothing to do with modding or Minecraft.
-
The PacketBuffer class has a writeItemStack() and readItemStack() method. You can look at SPacketWindowItems for ideas. So I would make a custom packet using SPacketCustomPayload and pass it a PacketBuffer where you've used those methods for your ItemStack.
-
Yes, that is the whole point of advancements -- to trigger when criteria conditions are met. The format for the JSON is described here: https://minecraft.gamepedia.com/Advancements
-
I think you can just use the Log4j library to create your own logger. Standard Java stuff so google for tutorials and examples.
-
I think there are two types of people who don't post enough information to get the help they need: 1) people who think that the "experts" on the forum magically know all the answers off the top of their heads, 2) people who think that their mod is so special that they are worried about people stealing it. For type #1, I try to educate them that experts are mostly people that are just really good at researching. Even if we think we know the answer, we usually double-check our information because programming is about being exact -- the name of the method has to be exactly right, and so forth. If I'm answering a person's question I will usually pull up the Minecraft or Forge source, pull up my own implementations, look in github, check StackOverflow, etc. to gain confidence in my answer. The most important thing for these people to know is that the problem is usually very specific to their code. It is very difficult for anyone else to know what sort of typo they put into their code, or what sort of logic error, without seeing the code. Secondly, people often don't understand that the logs usually give a very good idea of what is wrong, so teaching people to really look at the error log is helpful. In a perfect world a person who comes here for help posts all the information, but also has put in their own effort to try to understand it. For type #2, I can only say that honestly no one here is interested in stealing other people's ideas and if you are stuck on coding then it is doubtful your mod is so advanced that it is worth stealing. But I suppose it is natural for people to be protective of their ideas, it's just that it is hard to help someone who isn't sharing.
-
As far as I know you need the JSON. It shouldn't be that much work and they are small text files so don't take up too much space. Even if you have a lot, it only should take about 10 minutes to create all the ones you need.
-
I don't think that is going to work. The textures "layers" is hardcoded in and you don't get events for each layer. I think what you need to do is have textures that are already pre-colored and set that for the horse armor texture.
-
I don't really understand your code. Of course if you change the color in the living entity pre render it will color the whole entity. But I'm not sure why you're doing it that way. First of all, where are you rendering the armor at all? I don't see any code for that. You have two, possibly three, options for coloring the armor. First of all, in a place where you actually render the armor you could actually choose a pre-colored texture. Secondly, you could use the GL11 color there but make sure you return the color to white in order to allow the remainder of the horse to have normal color. Thirdly you might be able to use IItemColor and tinting process. But the most fundamental thing is you need to render the actual armor. So where is the model and rendering code for that?
-
in 1.12.x, you can simply register a registry element with the same name, including the minecraft domain.
-
What exactly do you want to happen if you click on a water block?
-
Okay, so I think the problem goes back to what someone said earlier -- the regular right click does not interact with the fluid block. So you need to look at how the buckets do it. So look at the ItemBucket source code. I looked at it briefly and here is what I think. I think that the position that is passed to the onItemRightClick() method isn't right in the case of water. So what the bucket code does its own "ray trace" to double check if there is any water in the path to the position. You might be able to mostly copy the code for the bucket, but instead of filling the bucket and replacing the water with air you can do whatever you want with your item.
-
Okay, that wasn't quite what I meant by debug mode. Assuming you're using Eclipse you would set a breakpoint (google how to do that) on the first line of code in your onItemRightClick() method. Then use a debug run configuration to run the game. Eclipse should then reorganize into a debug view and what will happen is that you can start to play but as soon as you do a right click interaction with your item the execution of the game should halt and a bunch of information should show up in Eclipse that allows you to see what the values of all the fields are. Then there are buttons in Eclipse that allow you to progress one "step" at time (meaning one line of code) and again you can check what is happening. If that is too advanced for you, the other way is to add your own console print statements to give you information about what is going on. For example, using your previous code as an example, I would do something like this: public EnumActionResult onItemRightClick(ItemStack stack, EntityPlayer playerIn, World worldIn, BlockPos pos, EnumHand hand, EnumFacing facing, float hitX, float hitY, float hitZ) { System.out.println("onItemRightClick with item = "+stack.getItem()+" at position = "+pos); IBlockState iblockstate = worldIn.getBlockState(pos); Block block = iblockstate.getBlock(); if (worldIn.getBlockState(pos.up()).getMaterial() == Material.AIR && block == Blocks.WATER || block == Blocks.FLOWING_WATER) { System.out.println("Condition met -- block pos is surface water"); worldIn.playSound(playerIn, pos, SoundEvents.ITEM_BUCKET_FILL, SoundCategory.BLOCKS, 1.0F, 1.0F); if(!worldIn.isRemote) { worldIn.spawnEntityInWorld(new EntityItem(worldIn, playerIn.posX, playerIn.posY, playerIn.posZ, new ItemStack(net.dev.premiermod.init.ModItems.speck_gold, 1))); stack.damageItem(1, playerIn); } return EnumActionResult.SUCCESS; } else { System.out.println("Condition not met -- block pos isn't surface water"); return EnumActionResult.PASS; } } With the added lines above, run the game normally but look at the console when you right click. It will tell you firstly whether your code is running at all and secondly it will tell you which code path it is taking. Depending on what you see, then you can dig in further to figure out what is going wrong.
-
What do you mean it "doesn't work"? And have you done regular debug. Like did you either use print statements to trace the execution to see what your code is doing, or did you use breakpoints and debug mode in Eclipse. If you want to know why code isn't working, simply follow the execution and see if it is doing what you expect.
-
I think you just need to handle the AnvilUpdateEvent.
-
Probable Bug Resource Packs Are unable To Override Modded Lang
jabelar replied to jredfox's topic in Modder Support
So the Forge documentation on resources is here: https://mcforge.readthedocs.io/en/latest/concepts/resources/ It says: So it is specifically saying that lang files are treated a bit differently. Are other resources also acting the same way? Also I'm not sure when the lang file is applied to the item, but you're not registering your item in the proper order. You should not be doing it in pre-init, but rather you should be doing it in the registry event for items and you should access the registry passed through the event. I'm not sure it is related to your issue, but I could imagine that the order might matter for any "merging" of resources that happens. -
[SOLVED] Playing sounds causes ConcurrentModificationException
jabelar replied to leduyquang753's topic in Modder Support
Yes -
Can anyone help me update a mod from 1.7.10 to 1.12?
jabelar replied to Rackneh's topic in Modder Support
I already said that. Your name and logo (and other artistic / identity assets) are under separate copyright. But as long as you're not pretending to have the same identity and give credit according to the license and change all the artwork (or otherwise honor the art's license), the use of the code can't be restricted. -
Can anyone help me update a mod from 1.7.10 to 1.12?
jabelar replied to Rackneh's topic in Modder Support
No, basically open source means you can't restrict at all what other people do with it. For example, in a FAQ at https://opensource.org/faq#restrict they say: Now, in the license it can ask that you give credit, and frankly the modding community would probably not like you if you did not. Also sites like CurseForge can add whatever restriction they want for things posted on their sites. However, as far as I know, there is no legal restriction for taking someone's GPL code and redistributing it, modified or not, without any permission from the original author. HOWEVER: It is important to understand that the artistic assets -- like any textures and sounds -- are considered separate works and so may be licensed differently. If there is no license I'm not sure what the legal default would be, but you can definitely put in a license that is not open source to cover your artistic assets. So if you modify and redistribute without the authors' permission you need to make sure you understand the licensing of the resource assets, and to be safe you should make your own.. -
Well, it just takes some math. Obviously the position will need to be relative to the pigman holding the chain. After that it is physics. I would probably code it using standard physics ideas. Like I would have a field for the chain's "force". The boulder would move freely until its distance is equal to the length of the chain at which point the chain will exert force in the direction of the tight chain. I would probably "cheat" the physics a bit when starting an attack -- like I would have the pigman start spinning and automatically give the boulder some lift and rotational motion, but then use physics after that. When the chain is tight, you can use vector dot product to help eliminate any outward motion. An alternative way, maybe simpler if you're not strong in physics, is to plan out the motion with an array and step through it like a standard animation. However, you would also check for collisions and when those happen you'd revert to physics calculations.
-
Yes, but I was worried not about the biome filler but about the chunk generator "filler" block. In the chunk generator, such as the vanilla overworld it fills the chunk with STONE. After that the biome might add dirt and grass as filler and top. But the vanilla cave gen uses the canReplaceBlock() method which checks for a bunch of standard stuff including STONE. If you think about it, caves go deeper than the biome filler. If you have your own custom block which is used as tje main block in the chunk generator but not in your biome top or filler, then the caves will have trouble. Of course the solution is to simply override the canReplaceBlock() in your own custom cave generator.
-
Yeah, I guess you were creating a new biomeprovider over and over or something, rather than a single instance that gets referenced again. Usually when I have these problems with world gen I try to simply the amount of overriding and it helps narrow it down. Glad it worked!
-
You can see my example code here: https://github.com/jabelar/ExampleMod-1.12/tree/master/src/main/java/com/blogspot/jabelarminecraft/examplemod/worldgen The way the WorldType works, is you MUST use the super constructor that just takes a string for the name. That constructor will add your custom world type to the available options in the new world creation menu. See https://github.com/jabelar/ExampleMod-1.12/blob/master/src/main/java/com/blogspot/jabelarminecraft/examplemod/worldgen/WorldTypeCloud.java. In that you'll see you need to override the key methods for providing the BiomeProvider and ChunkGenerator. I give a list of the things that need to be hooked together in this tutorial: http://jabelarminecraft.blogspot.com/p/minecraft-modding-custom-dimension.html.
-
I think you just need to go through standard debug -- add some console or logger print statements at critical points in your code and have it print out useful info like what location it is trying to modify. Basically you need to catch the cascading generation "in the act". Once you observe the failing case it is usually pretty obvious what went wrong.
-
In your CMWorldProvider class I think you're overriding too many methods. One of the tricks with Minecraft world gen is that the classes and methods are very convoluted and you can run into trouble where you register things but also override methods in ways that don't match. For example, you shouldn't provide the chunk generator directly. It should come from the WorldType (in the terrainType field) automatically. So I don't think you should override the createChunkGenerator() method. You also shouldn't have to provide the biomes. I don't think you should override the getBiomeProvider() method. That should also come from the WorldType automatically. So I don't think you should override the getBiomeProvider() or the getBiomeForCoords() methods. I suggest properly creating a WordType and having those methods automatically give the results in the WorldProvider (using the parent class without overriding), cause I've found mixups that can occur can cause lag. Another thing I notices is that you're using your own "corrupted stone" to fill in the chunk. In your mineable world gen you properly use a predicate that also checks for corrupted stone, but I wonder if there are other features that are struggling because they look for regular stone or something. For example, if a ravine or mineshaft was looking for stone and couldn't find it then I would expect the chunk generation to take longer. For example MapGenCaves looks for specific blocks which may not be present in your case. To isolate this, I recommend commenting out each of the feature generators and see if any of them are causing lag; if so you might need to do a custom version.