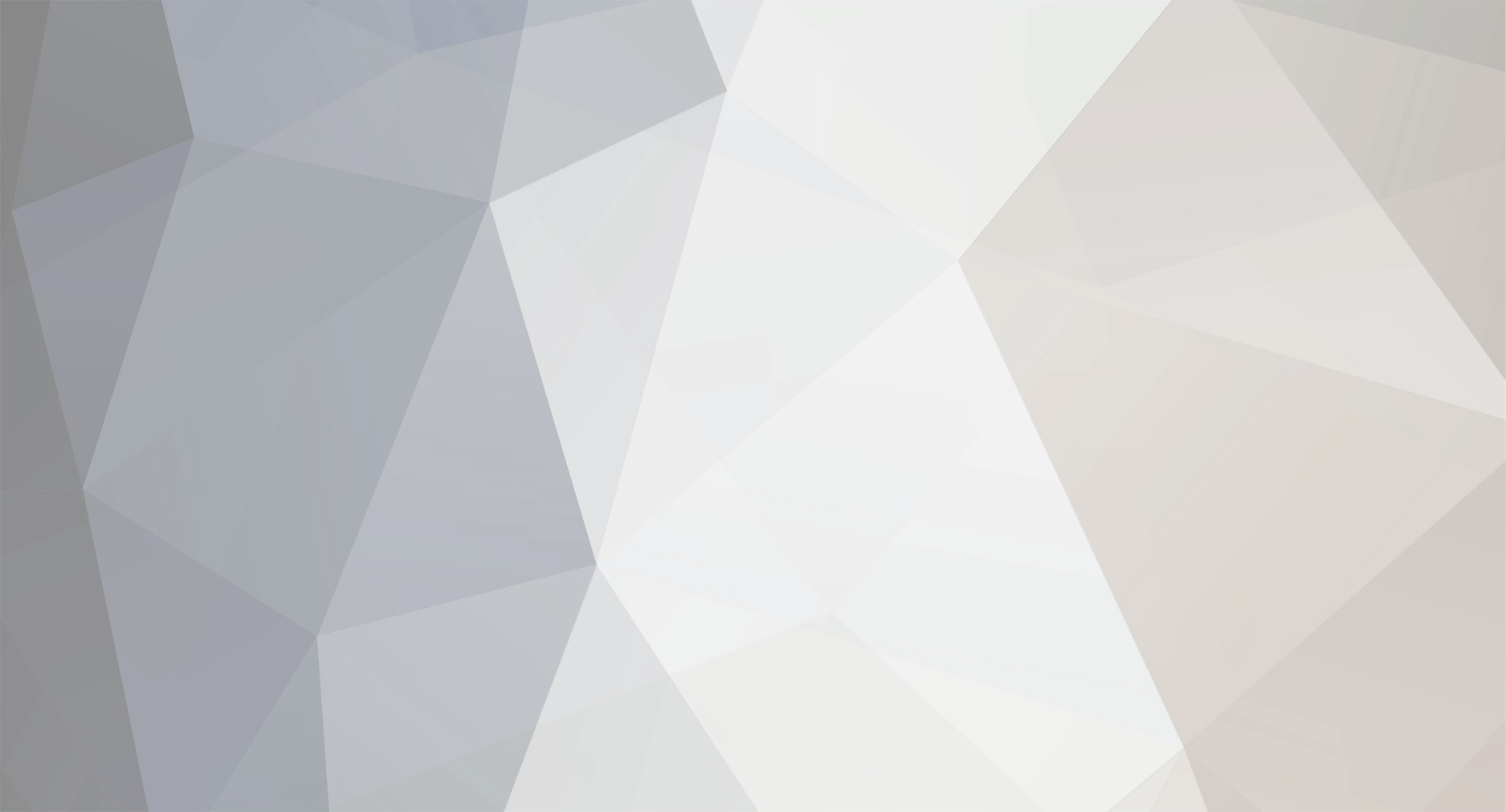
jabelar
Members-
Posts
3266 -
Joined
-
Last visited
-
Days Won
39
Everything posted by jabelar
-
Yes, that's exactly what I said. He is only guaranteed to get a GuiScreen from getGui(), so I told him to use instanceof to confirm it is a GuiCreateWorld and told him to cast it to make the method accept it.
-
Yes, I know T is a superclass of T. But in his example above his "T" is GuiScreen and his ? is GuiCreateWorld. So isn't he trying to put in a type Bar that doesn't extend Foo?
-
I'm not great at generics, but doesn't <? super T? mean that it needs to be a super class of T. I don't think GuiCreateWorld is a superclass of GuiScreen. But if you've already tested that the event.getGui() is instanceof GuiCreateWorld then maybe you can cast it to GuiCreateWorld to satisfy the generic?
-
It depends on what you're trying to do. I think there is a player capability called isFlying which is synced with the packet CPacketPlayerAbilities. So as mentioned maybe you can handle the player tick event and check the isFlying capability. Of course that would be any type of flying, so maybe there are cases that won't work for you. But if you catch isFlying in the first tick it is happening, that might be good for what you're trying to do.
-
[1.10.2] [UNSOLVABLE] Make the player light like a torch?
jabelar replied to Differentiation's topic in Modder Support
Here is source code: https://github.com/jabelar/MovingLightSource-1.12 Note in that mod entities on fire also become moving light sources, so kind of fun to set the time to night and light them up -- and for fun I make torches weapons that can set entities on fire. In terms of performance, perhaps you should also consider what Draco is mentioning. However, performance is a relative thing and I have the luxury of playing on good gaming rigs and it is quite smooth (you can see in my video), and everyone's computers are getting better every day (just go buy a NVIDIA mid-range GeForce card and you'll do fine) so I'm not sure how much it is worth looking at. -
[1.10.2] [UNSOLVABLE] Make the player light like a torch?
jabelar replied to Differentiation's topic in Modder Support
Why did you say "I guess not" when he just told you how to do it? I did it exactly the way draco said and made the player emit light (in my case I only turned it on when holding a light emitting item, but you could do exactly the same when wearing an item). See my video here: -
Potions wont recolor even when i change the liquidColor...?
jabelar replied to powns's topic in Modder Support
Events don't work that way. Events are coded by Forge as patches against the vanilla code, so you can't just add the same code yourself in an older version. However, if you look at the code around where the event is called in 1.11+ you might get ideas about other ways to intercept it. For example, I noticed that the event is actually fired during the metadata update and interestingly the metadata includes the potion color that is set to the data manager. So it may well be possible to set the datamanager directly. Here is the code in the event: protected void updatePotionMetadata() { if (this.activePotionsMap.isEmpty()) { this.resetPotionEffectMetadata(); this.setInvisible(false); } else { Collection<PotionEffect> collection = this.activePotionsMap.values(); net.minecraftforge.event.entity.living.PotionColorCalculationEvent event = new net.minecraftforge.event.entity.living.PotionColorCalculationEvent(this, PotionUtils.getPotionColorFromEffectList(collection), areAllPotionsAmbient(collection), collection); net.minecraftforge.common.MinecraftForge.EVENT_BUS.post(event); this.dataManager.set(HIDE_PARTICLES, event.areParticlesHidden()); this.dataManager.set(POTION_EFFECTS, event.getColor()); this.setInvisible(this.isPotionActive(MobEffects.INVISIBILITY)); } } However, I can't remember if the datamanager was used in 1.8.9 or it might have been the data watcher instead. If so, you should look at the data watcher for potions to see if the color is in that. If so, then you might be able to override it directly. -
You need to note what I said about using Reflection helper. Even though your code might work in a development environment, it won't work once you build it unless you handle the obfuscated name of the field. It is very likely not called "heal" in the actual Minecraft jar.
-
How does entity's hitbox and block's hitbox interact
jabelar replied to TheRPGAdventurer's topic in Modder Support
Well, entities have both planned and unplanned movement. For planned movement, which is usually figured out in the entity AI, it uses a "navigator" to find a path through the blocks. The navigators basically finds a series of positions that the entity can move between where its collision box doesn't collide. Then there is unplanned movement like if you knock it back. I haven't looked at that code specifically, but typically in games it will (a) look for collision before moving the entity into a location (b) reset position to the previous one if it finds itself in a collision. All the collision boxes (block and entity) in Minecraft are rectangular, restricted in size and cannot rotate so it is really simple math to detect a collision. Why are you asking? -
Actually it is usually good to have your user account info in the run configuration because otherwise during testing the various inventory stuff, any ownership data (like tamed animals), spawn points, and so forth aren't consistent. But in this case you should create a copy of the run configuration without the user account information (you probably entered it in the program arguments in the run configuration editor). Then you can either run with or without account information as you need to. But the main point is yes you should be able to run multiple instances of Minecraft from Eclipse, a server and a couple clients, whenever it is useful.
-
Well a few things. First of all you shouldn't have to guess whether the code is running -- you should either add console statements in the methods or you should set breakpoints and run the code in debug mode. For something like this I would use console statements because then you'd get a good sense of when it might be saving or not, and you can also print out the values of key fields at that point in the execution. That way you can figure out if the code simply isn't running, or if it running but the values loaded don't match for some reason. Secondly, it looks like you're saying that the NBT code is in the shield charm, but your title says "entity nbt". Which is it? Is that data for the shield item or for the entity? If it is for the shield item, at what point do you expect it to be saved? Thirdly, most of these sorts of problems are confusion about how to sync the client and the server. There are vanilla processes to sync the vanilla stuff, and some ability to have extended data communicated, but you often have to make sure either by initiating packets (through share tag methods, send packet methods, or mark dirty methods depending on the thing you're trying to sync), or even going as far as sending custom packets if necessary. Also, I've found there is some tricks about NBT used together with item capabilities. Not clear if you're using capabilities for this item, but if you are the order in which the initi capabilities happens versus the reading of the NBT data might have to be sorted out -- in other words sometimes the NBT data reads but then the capability can get initialized to overwrite it. But my main point is add console statements so you can actually track the execution and then it should be really easy to see what is wrong.
-
[1.12.x/1.11.x] [UNSOLVED] Bounding Boxes IndexOutOfBoundsException
jabelar replied to Bektor's topic in Modder Support
-
[SOLVED][1.12.2] Question about genPatches when contributing to Forge
jabelar replied to jabelar's topic in Modder Support
Thanks guys. -
[1.12.x/1.11.x] [UNSOLVED] Bounding Boxes IndexOutOfBoundsException
jabelar replied to Bektor's topic in Modder Support
First of all, in computing you count starting at zero. So the maximum your index should be is the size-1. In a bounding box there are six elements (three for each of the opposite min max corners) so you should only ever index up to 5. The error says you're indexing to 6 and your code does a <= size() when it should be simply < size(). -
Well, there are a lot of things to consider when you are doing entity AI. As mentioned before, there is a priority to the AI. There is also something called "mutex" which stands for mutually exclusive. This indicates which AI can run at the same time and which cannot. I have an explanation of entity AI in a tutorial here: http://jabelarminecraft.blogspot.com/p/minecraft-forge-1721710-custom-entity-ai.html To debug, you should just use the normal debug strategies -- set breakpoints and use Eclipse debug mode to make sure that your code is executing and further to check the value of key fields during the execution. Alternatively you can add console print statements, but assuming you're using the built-in AI classes that can be a pain because you'll have to copy them and make your own versions in order to add the prints. So I suggest starting with breakpoints. Based on that, confirm that your AI is executing and then it is usually pretty easy to figure out what is wrong after that.
-
One thing to look at is the scoreboard class already allows you to create teams, and it already has a field to enable/disable friendly fire. Not sure if that works for what you want, but you might want to look at it. At least you can check out how it works.
-
Are you sure there is a field called "heal"? I looked at the Potion class and these are the fields I see: public class Potion extends net.minecraftforge.registries.IForgeRegistryEntry.Impl<Potion> { public static final RegistryNamespaced<ResourceLocation, Potion> REGISTRY = net.minecraftforge.registries.GameData.getWrapper(Potion.class); private final Map<IAttribute, AttributeModifier> attributeModifierMap = Maps.<IAttribute, AttributeModifier>newHashMap(); private final boolean isBadEffect; private final int liquidColor; private String name = ""; private int statusIconIndex = -1; private double effectiveness; private boolean beneficial; Also, if you really need to use Reflection (you usually don't though, so we should see if there is a way to accomplish what you want without it) you need to use the ReflectionHelper class. This is because the field and method names are obfuscated in the real game but in our development environment they are deobfuscated. So you need to reference the SRG mapped name as well, and the ReflectionHelper helps with that. If you don't, it will appear to work in the development environment but will fail when you build your mod.
-
[SOLVED][1.12.2] Question about genPatches when contributing to Forge
jabelar replied to jabelar's topic in Modder Support
Okay, so I shouldn't modify clean at all then (makes sense). Under Forge there is the src/main/Java which contains Minecraft code and I should do the edits there then the genPatchs compares that with clean. Thanks for the clarification. What about my other question, if my changes are only in the Forge stuff (under main-java), I don't need to generate a patch right? It will get directly merged? -
I'm working on my first (simple) contribution to Forge. So I think I'm ready to submit a pull request, but want to clarify a couple things. I have my fork, which I've been working on (on the 1.12 branch), have a working test mod, etc. The change I'm making is only on Forge code, not Minecraft. So in that case do I need to generate patches? I assume my change will just get merged without needing patches -- generating patches is for cases when a change to the vanilla code is being proposed, right? My next contribution will want to do some changes to vanilla behavior. So in that case, I do the change in the Clean part of the code and then generate patches? Also, the instructions say to run the gradlew genPatches on the "Forge root". Does that mean the top of my workspace (which contains both the Clean and Forge, or actually run inside the Forge folder? Lastly, once the patch is generated I sync that back to my github repository? Sorry for the noob questions...
-
I haven't tried it recently, but in some versions you could use: player.canCommandSenderUseCommand(2, "")
-
[1.12.2] Run Configurations When Trying To Test Contribution To Forge
jabelar replied to jabelar's topic in Modder Support
Thanks Leviathan, that helps a lot. -
[1.12.2] Run Configurations When Trying To Test Contribution To Forge
jabelar replied to jabelar's topic in Modder Support
Regarding the test code, I do see that there are a bunch of files in the debug and test folders and many in the debug folders are annotated as mods which also show up when I do the Forge Client or Forge Server run configurations. So I'm guessing that we're supposed to put our test mod code into a single file which we put into the debug folder? That seems a bit limiting as I wanted to do some pull requests related to fluids but would need a fair number of classes and such -- would I still try to put that all into a single file? Also, with all those test mods loading, isn't there potential for trouble? I don't want the test mods interfering with my testing ... / -
So I've never contributed to Forge before, so figured I should give it a try. I forked and cloned forge, got the setupForge working, imported the projects and can see it all in Eclipse. However, what is the proper way to test it, and what do the various run configurations do exactly? In terms of actual testing, I would like to write some test mod to apply. Do I need to fully build everything, or can I test it all in Eclipse somehow? I tried the Forge Client run configuration, but got a lot of errors related to missing models (I didn't change anything related to that): 2017-10-08 00:02:11,279 main WARN Unable to instantiate org.fusesource.jansi.WindowsAnsiOutputStream 2017-10-08 00:02:11,284 main WARN Unable to instantiate org.fusesource.jansi.WindowsAnsiOutputStream [00:02:11] [main/INFO] [GradleStart]: Extra: [] [00:02:11] [main/INFO] [GradleStart]: Running with arguments: [--userProperties, {}, --assetsDir, C:/Users/Aaron/.gradle/caches/minecraft/assets, --assetIndex, 1.12, --accessToken{REDACTED}, --version, @@MCVERSION@@, --tweakClass, net.minecraftforge.fml.common.launcher.FMLTweaker, --tweakClass, net.minecraftforge.gradle.tweakers.CoremodTweaker] [00:02:11] [main/INFO] [LaunchWrapper]: Loading tweak class name net.minecraftforge.fml.common.launcher.FMLTweaker [00:02:11] [main/INFO] [LaunchWrapper]: Using primary tweak class name net.minecraftforge.fml.common.launcher.FMLTweaker [00:02:11] [main/INFO] [LaunchWrapper]: Loading tweak class name net.minecraftforge.gradle.tweakers.CoremodTweaker [00:02:11] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.common.launcher.FMLTweaker [00:02:11] [main/INFO] [FML]: Forge Mod Loader version 14.23.0.0 for Minecraft 1.12.2 loading [00:02:11] [main/INFO] [FML]: Java is Java HotSpot(TM) 64-Bit Server VM, version 1.8.0_144, running on Windows 10:amd64:10.0, installed at C:\Program Files\Java\jre1.8.0_144 [00:02:11] [main/INFO] [FML]: Managed to load a deobfuscated Minecraft name- we are in a deobfuscated environment. Skipping runtime deobfuscation [00:02:11] [main/INFO] [FML]: Ignoring missing certificate for coremod FMLCorePlugin (net.minecraftforge.fml.relauncher.FMLCorePlugin), we are in deobf and it's a forge core plugin [00:02:11] [main/INFO] [FML]: Ignoring missing certificate for coremod FMLForgePlugin (net.minecraftforge.classloading.FMLForgePlugin), we are in deobf and it's a forge core plugin [00:02:11] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.gradle.tweakers.CoremodTweaker [00:02:11] [main/INFO] [GradleStart]: Injecting location in coremod net.minecraftforge.fml.relauncher.FMLCorePlugin [00:02:11] [main/INFO] [GradleStart]: Injecting location in coremod net.minecraftforge.classloading.FMLForgePlugin [00:02:11] [main/INFO] [LaunchWrapper]: Loading tweak class name net.minecraftforge.fml.common.launcher.FMLInjectionAndSortingTweaker [00:02:11] [main/INFO] [LaunchWrapper]: Loading tweak class name net.minecraftforge.fml.common.launcher.FMLDeobfTweaker [00:02:11] [main/INFO] [LaunchWrapper]: Loading tweak class name net.minecraftforge.gradle.tweakers.AccessTransformerTweaker [00:02:11] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.common.launcher.FMLInjectionAndSortingTweaker [00:02:11] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.common.launcher.FMLInjectionAndSortingTweaker [00:02:11] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.relauncher.CoreModManager$FMLPluginWrapper 2017-10-08 00:02:12,388 main WARN Unable to instantiate org.fusesource.jansi.WindowsAnsiOutputStream 2017-10-08 00:02:12,406 main WARN Unable to instantiate org.fusesource.jansi.WindowsAnsiOutputStream 2017-10-08 00:02:12,407 main WARN Unable to instantiate org.fusesource.jansi.WindowsAnsiOutputStream [00:02:27] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.relauncher.CoreModManager$FMLPluginWrapper [00:02:27] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.common.launcher.FMLDeobfTweaker [00:02:28] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.gradle.tweakers.AccessTransformerTweaker [00:02:28] [main/INFO] [LaunchWrapper]: Loading tweak class name net.minecraftforge.fml.common.launcher.TerminalTweaker [00:02:28] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.common.launcher.TerminalTweaker [00:02:28] [main/INFO] [LaunchWrapper]: Launching wrapped minecraft {net.minecraft.client.main.Main} [00:02:30] [main/INFO]: Setting user: Player943 [00:02:39] [main/INFO]: LWJGL Version: 2.9.4 [00:02:42] [main/INFO] [FML]: Could not load splash.properties, will create a default one [00:02:42] [main/INFO] [FML]: -- System Details -- Details: Minecraft Version: 1.12.2 Operating System: Windows 10 (amd64) version 10.0 Java Version: 1.8.0_144, Oracle Corporation Java VM Version: Java HotSpot(TM) 64-Bit Server VM (mixed mode), Oracle Corporation Memory: 125307952 bytes (119 MB) / 278921216 bytes (266 MB) up to 1895825408 bytes (1808 MB) JVM Flags: 0 total; IntCache: cache: 0, tcache: 0, allocated: 0, tallocated: 0 FML: Loaded coremods (and transformers): GL info: ' Vendor: 'NVIDIA Corporation' Version: '4.5.0 NVIDIA 384.76' Renderer: 'GeForce GTX 760/PCIe/SSE2' [00:02:42] [main/INFO] [FML]: MinecraftForge v14.23.0.0 Initialized [00:02:42] [main/INFO] [FML]: Starts to replace vanilla recipe ingredients with ore ingredients. [00:02:42] [main/INFO] [FML]: Replaced 1036 ore ingredients [00:02:43] [main/INFO] [FML]: Found 0 mods from the command line. Injecting into mod discoverer [00:02:43] [main/INFO] [FML]: Searching F:\Forge Workspace\MinecraftForge\projects\run\mods for mods [00:02:44] [Thread-3/INFO] [FML]: Using sync timing. 200 frames of Display.update took 70113143 nanos [00:02:51] [main/INFO] [recipetest]: Mod recipetest is missing the required element 'name'. Substituting recipetest [00:02:51] [main/INFO] [registry_override_test]: Mod registry_override_test is missing the required element 'name'. Substituting registry_override_test [00:02:51] [main/WARN] [transparent_fast_tesr_test]: Mod transparent_fast_tesr_test is missing the required element 'version' and no fallback can be found. Substituting '1.0'. [00:02:54] [main/INFO] [FML]: Forge Mod Loader has identified 83 mods to load [00:02:55] [main/INFO] [FML]: Attempting connection with missing mods [minecraft, mcp, FML, forge, advancements_pagination, animal_tame_event_test, big_packet_test, ainodetypetest, block_place_event_test, forge_blockstate_retexture_test, bonemealeventtest, breedingtest, brewingreciperegistrytest, canapplyatenchantingtabletest, checkspawntest, chunkcapabilitypollutiontest, clientchateventtest, clientcommandtest, clientexceptiontest, command_tree_base_test, config_test, crafting_system_test, createfluidsourcetest, criticalhiteventtest, customentityselectortest, customspawndimensiontest, custom_sprite_test, debugsearchtab, decorateeventdebug, difficultychangeeventtest, dynbuckettest, enchantmentlevelsettest, entitytraveltodimensioneventtest, entityupdateblockedtest, enumplanttypetest, equipment_change_test, fluidhandlertest, forgedebugfluidplacement, fogcolorinsidematerialtest, forgeblockstatesloader, fovmodifiertest, furnacefuelburntimeeventtest, guicontainereventtest, is_book_enchantable_test, item_can_destroy_blocks_in_creative_test, itemfishtest, forgedebugitemlayermodel, forgedebugitemtile, loottable_load_event_test, loot_table_debug, mapdatatest, forgedebugmodelanimation, forgedebugmodelbakeevent, forgedebugmodelfluid, forgedebugmodelloaderregistry, forgemodnametooltip, forgedebugmultilayermodel, nbtsharetagitemtest, neighbornotifyeventtest, forgedebugnobedsleeping, nopotioneffect, objectholdertest, onitemusefirsttest, oredict_predicate, permission_test, playerdamagereworktest, playerinteracteventtest, playersetspawntest, potion_curative_item_debug, forgepotionregistry, recipetest, registry_override_test, shield_test, slipperiness_test, forge.testcapmod, forge_texture_dump, te_loading_test, transparent_fast_tesr_test, professiontest, worldperiodicrainchecktest, wrenchrotatedebug, wrnormal, forgenetworktest] at CLIENT [00:02:55] [main/INFO] [FML]: Attempting connection with missing mods [minecraft, mcp, FML, forge, advancements_pagination, animal_tame_event_test, big_packet_test, ainodetypetest, block_place_event_test, forge_blockstate_retexture_test, bonemealeventtest, breedingtest, brewingreciperegistrytest, canapplyatenchantingtabletest, checkspawntest, chunkcapabilitypollutiontest, clientchateventtest, clientcommandtest, clientexceptiontest, command_tree_base_test, config_test, crafting_system_test, createfluidsourcetest, criticalhiteventtest, customentityselectortest, customspawndimensiontest, custom_sprite_test, debugsearchtab, decorateeventdebug, difficultychangeeventtest, dynbuckettest, enchantmentlevelsettest, entitytraveltodimensioneventtest, entityupdateblockedtest, enumplanttypetest, equipment_change_test, fluidhandlertest, forgedebugfluidplacement, fogcolorinsidematerialtest, forgeblockstatesloader, fovmodifiertest, furnacefuelburntimeeventtest, guicontainereventtest, is_book_enchantable_test, item_can_destroy_blocks_in_creative_test, itemfishtest, forgedebugitemlayermodel, forgedebugitemtile, loottable_load_event_test, loot_table_debug, mapdatatest, forgedebugmodelanimation, forgedebugmodelbakeevent, forgedebugmodelfluid, forgedebugmodelloaderregistry, forgemodnametooltip, forgedebugmultilayermodel, nbtsharetagitemtest, neighbornotifyeventtest, forgedebugnobedsleeping, nopotioneffect, objectholdertest, onitemusefirsttest, oredict_predicate, permission_test, playerdamagereworktest, playerinteracteventtest, playersetspawntest, potion_curative_item_debug, forgepotionregistry, recipetest, registry_override_test, shield_test, slipperiness_test, forge.testcapmod, forge_texture_dump, te_loading_test, transparent_fast_tesr_test, professiontest, worldperiodicrainchecktest, wrenchrotatedebug, wrnormal, forgenetworktest] at SERVER [00:02:56] [main/INFO]: Reloading ResourceManager: Default, FMLFileResourcePack:Forge Mod Loader, FMLFileResourcePack:Minecraft Forge, FMLFileResourcePack:Advancements Pagination test mod, FMLFileResourcePack:AnimalTameEvent test mod, FMLFileResourcePack:Big network message test mod, FMLFileResourcePack:AiNodeTypeTest, FMLFileResourcePack:BlockPlaceEvent test mod, FMLFileResourcePack:BlockstateRetextureTest, FMLFileResourcePack:Bonemeal Event Test, FMLFileResourcePack:BreedingTest, FMLFileResourcePack:BrewingRecipeRegistryTest, FMLFileResourcePack:CanApplyAtEnchantingTableTest, FMLFileResourcePack:CheckSpawnTest, FMLFileResourcePack:Chunk Capability Test, FMLFileResourcePack:Client Chat Event Test, FMLFileResourcePack:Client Command Test, FMLFileResourcePack:Client Exception Test, FMLFileResourcePack:CommandTreeBaseTest, FMLFileResourcePack:ConfigTest, FMLFileResourcePack:CraftingTestMod, FMLFileResourcePack:CreateFluidSourceTest, FMLFileResourcePack:CriticalHitEventTest, FMLFileResourcePack:Custom Entity Selector Test, FMLFileResourcePack:CustomSpawnDimensionTest, FMLFileResourcePack:Custom sprite test, FMLFileResourcePack:Debug Search Tab, FMLFileResourcePack:DecorateEventDebug, FMLFileResourcePack:DifficultyChangeEventTest, FMLFileResourcePack:DynBucketTest, FMLFileResourcePack:EnchantmentLevelSetTest, FMLFileResourcePack:EntityTravelToDimensionEventTest, FMLFileResourcePack:Entity Update Blocked Test, FMLFileResourcePack:EnumPlantTypeTest, FMLFileResourcePack:Equipment Change Test, FMLFileResourcePack:FluidHandlerTest, FMLFileResourcePack:ForgeDebugFluidPlacement, FMLFileResourcePack:FogColor inside material debug., FMLFileResourcePack:ForgeBlockStatesLoader, FMLFileResourcePack:FOV Modifier Test, FMLFileResourcePack:Test for FurnaceFuelBurnTimeEvent, FMLFileResourcePack:GuiContainer Event Tests!, FMLFileResourcePack:Test for isBookEnchantable, FMLFileResourcePack:Item.canDestroyBlockInCreative() Test, FMLFileResourcePack:ItemFishTest, FMLFileResourcePack:ForgeDebugItemLayerModel, FMLFileResourcePack:ForgeDebugItemTile, FMLFileResourcePack:LootTableLoadEventTest, FMLFileResourcePack:Loot Table Debug, FMLFileResourcePack:mapdatatest, FMLFileResourcePack:ForgeDebugModelAnimation, FMLFileResourcePack:ForgeDebugModelBakeEvent, FMLFileResourcePack:ForgeDebugModelFluid, FMLFileResourcePack:ForgeDebugModelLoaderRegistry, FMLFileResourcePack:ForgeModNameTooltip, FMLFileResourcePack:ForgeDebugMultiLayerModel, FMLFileResourcePack:NBTShareTag Item Test, FMLFileResourcePack:NeighborNotifyEventTest, FMLFileResourcePack:ForgeDebugNoBedSleeping, FMLFileResourcePack:No Potion Effect Render Test, FMLFileResourcePack:ObjectHolderTests, FMLFileResourcePack:OnItemUseFirstTest, FMLFileResourcePack:Oredict Item Predicate Test, FMLFileResourcePack:PermissionTest, FMLFileResourcePack:PlayerDamageReworkTest, FMLFileResourcePack:PlayerInteractEventTest, FMLFileResourcePack:Player Set Spawn Test, FMLFileResourcePack:Potion Curative Item Debug, FMLFileResourcePack:ForgePotionRegistry, FMLFileResourcePack:recipetest, FMLFileResourcePack:registry_override_test, FMLFileResourcePack:Shield Test, FMLFileResourcePack:Slipperiness Test, FMLFileResourcePack:Forge TestCapMod, FMLFileResourcePack:Forge Texture Atlas Dump, FMLFileResourcePack:TileEntity#onLoad() test mod, FMLFileResourcePack:TransparentFastTESRTest, FMLFileResourcePack:ProfessionTest2000, FMLFileResourcePack:World Periodic Rain Check Test, FMLFileResourcePack:Wrench Rotate Debug, FMLFileResourcePack:WRNormal, FMLFileResourcePack:forgenetworktest [00:02:57] [main/INFO] [FML]: Processing ObjectHolder annotations [00:02:57] [main/INFO] [FML]: Found 1215 ObjectHolder annotations [00:02:57] [main/INFO] [FML]: Identifying ItemStackHolder annotations [00:02:57] [main/INFO] [FML]: Found 0 ItemStackHolder annotations [00:02:57] [main/INFO] [FML]: Configured a dormant chunk cache size of 0 [00:02:57] [Forge Version Check/INFO] [ForgeVersionCheck]: [forge] Starting version check at http://files.minecraftforge.net/maven/net/minecraftforge/forge/promotions_slim.json [00:02:57] [main/INFO] [forge.testcapmod]: IExampleCapability was registered wheeeeee! [00:02:57] [main/WARN] [FML]: A mod has attempted to assign Block Block{null} to the Fluid 'water' but this Fluid has already been linked to the Block Block{minecraft:water}. You may have duplicate Fluid Blocks as a result. It *may* be possible to configure your mods to avoid this. [00:02:57] [main/WARN] [FML]: **************************************** [00:02:57] [main/WARN] [FML]: * Dangerous alternative prefix `minecraft` for name `bookshelf`, expected `registry_override_test` invalid registry invocation/invalid name? [00:02:57] [main/WARN] [FML]: * at net.minecraftforge.registries.IForgeRegistryEntry$Impl.setRegistryName(IForgeRegistryEntry.java:85) [00:02:57] [main/WARN] [FML]: * at net.minecraftforge.registries.IForgeRegistryEntry$Impl.setRegistryName(IForgeRegistryEntry.java:95) [00:02:57] [main/WARN] [FML]: * at net.minecraftforge.debug.RegistryOverrideTest$BlockReplacement.<init>(RegistryOverrideTest.java:56) [00:02:57] [main/WARN] [FML]: * at net.minecraftforge.debug.RegistryOverrideTest$BlockReplacement.<init>(RegistryOverrideTest.java:53) [00:02:57] [main/WARN] [FML]: * at net.minecraftforge.debug.RegistryOverrideTest.registerBlocks(RegistryOverrideTest.java:46) [00:02:57] [main/WARN] [FML]: * at net.minecraftforge.fml.common.eventhandler.ASMEventHandler_61_RegistryOverrideTest_registerBlocks_Register.invoke(.dynamic)... [00:02:57] [main/WARN] [FML]: **************************************** [00:02:57] [main/INFO] [FML]: Applying holder lookups [00:02:57] [main/INFO] [FML]: Holder lookups applied [00:02:57] [main/INFO] [FML]: Applying holder lookups [00:02:57] [main/INFO] [FML]: Holder lookups applied [00:02:57] [main/INFO] [FML]: Applying holder lookups [00:02:57] [main/INFO] [FML]: Holder lookups applied [00:02:57] [Forge Version Check/INFO] [ForgeVersionCheck]: [forge] Found status: OUTDATED Target: 14.23.0.2491 [00:02:57] [main/INFO] [FML]: Applying holder lookups [00:02:57] [main/INFO] [FML]: Holder lookups applied [00:02:57] [main/INFO] [FML]: Injecting itemstacks [00:02:57] [main/INFO] [FML]: Itemstack injection complete [00:03:06] [Sound Library Loader/INFO]: Starting up SoundSystem... [00:03:06] [Thread-5/INFO]: Initializing LWJGL OpenAL [00:03:06] [Thread-5/INFO]: (The LWJGL binding of OpenAL. For more information, see http://www.lwjgl.org) [00:03:06] [Thread-5/INFO]: OpenAL initialized. [00:03:06] [Sound Library Loader/INFO]: Sound engine started [00:03:21] [main/INFO] [FML]: Max texture size: 16384 [00:03:22] [main/INFO]: Created: 512x512 textures-atlas [00:03:24] [main/ERROR] [FML]: Exception loading model for variant transparent_fast_tesr_test:fluid-tesr-block#fluid=15 for blockstate "transparent_fast_tesr_test:fluid-tesr-block[fluid=15]" net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model transparent_fast_tesr_test:fluid-tesr-block#fluid=15 with loader VariantLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader.registerVariant(ModelLoader.java:233) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadBlock(ModelBakery.java:153) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.loadBlocks(ModelLoader.java:221) ~[ModelLoader.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:159) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.registerReloadListener(SimpleReloadableResourceManager.java:121) [SimpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.init(Minecraft.java:512) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:377) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_144] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_144] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_144] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_144] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [bin/:?] at GradleStart.main(GradleStart.java:26) [bin/:?] Caused by: net.minecraft.client.renderer.block.model.ModelBlockDefinition$MissingVariantException at net.minecraft.client.renderer.block.model.ModelBlockDefinition.getVariant(ModelBlockDefinition.java:83) ~[ModelBlockDefinition.class:?] at net.minecraftforge.client.model.ModelLoader$VariantLoader.loadModel(ModelLoader.java:1242) ~[ModelLoader$VariantLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 21 more [00:03:24] [main/ERROR] [FML]: Exception loading blockstate for the variant transparent_fast_tesr_test:fluid-tesr-block#fluid=15: java.lang.Exception: Could not load model definition for variant transparent_fast_tesr_test:fluid-tesr-block at net.minecraftforge.client.model.ModelLoader.getModelBlockDefinition(ModelLoader.java:266) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadBlock(ModelBakery.java:121) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.loadBlocks(ModelLoader.java:221) ~[ModelLoader.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:159) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.registerReloadListener(SimpleReloadableResourceManager.java:121) [SimpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.init(Minecraft.java:512) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:377) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_144] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_144] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_144] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_144] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [bin/:?] at GradleStart.main(GradleStart.java:26) [bin/:?] Caused by: java.lang.RuntimeException: Encountered an exception when loading model definition of model transparent_fast_tesr_test:blockstates/fluid-tesr-block.json at net.minecraft.client.renderer.block.model.ModelBakery.loadMultipartMBD(ModelBakery.java:228) ~[ModelBakery.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.getModelBlockDefinition(ModelBakery.java:208) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.getModelBlockDefinition(ModelLoader.java:262) ~[ModelLoader.class:?] ... 20 more Caused by: java.io.FileNotFoundException: transparent_fast_tesr_test:blockstates/fluid-tesr-block.json at net.minecraft.client.resources.SimpleReloadableResourceManager.getAllResources(SimpleReloadableResourceManager.java:83) ~[SimpleReloadableResourceManager.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadMultipartMBD(ModelBakery.java:221) ~[ModelBakery.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.getModelBlockDefinition(ModelBakery.java:208) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.getModelBlockDefinition(ModelLoader.java:262) ~[ModelLoader.class:?] ... 20 more [00:03:24] [main/ERROR] [FML]: Exception loading model for variant transparent_fast_tesr_test:fluid-tesr-block#fluid=14 for blockstate "transparent_fast_tesr_test:fluid-tesr-block[fluid=14]" net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model transparent_fast_tesr_test:fluid-tesr-block#fluid=14 with loader VariantLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader.registerVariant(ModelLoader.java:233) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadBlock(ModelBakery.java:153) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.loadBlocks(ModelLoader.java:221) ~[ModelLoader.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:159) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.registerReloadListener(SimpleReloadableResourceManager.java:121) [SimpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.init(Minecraft.java:512) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:377) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_144] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_144] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_144] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_144] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [bin/:?] at GradleStart.main(GradleStart.java:26) [bin/:?] Caused by: net.minecraft.client.renderer.block.model.ModelBlockDefinition$MissingVariantException at net.minecraft.client.renderer.block.model.ModelBlockDefinition.getVariant(ModelBlockDefinition.java:83) ~[ModelBlockDefinition.class:?] at net.minecraftforge.client.model.ModelLoader$VariantLoader.loadModel(ModelLoader.java:1242) ~[ModelLoader$VariantLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 21 more [00:03:24] [main/ERROR] [FML]: Exception loading model for variant transparent_fast_tesr_test:fluid-tesr-block#fluid=13 for blockstate "transparent_fast_tesr_test:fluid-tesr-block[fluid=13]" net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model transparent_fast_tesr_test:fluid-tesr-block#fluid=13 with loader VariantLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader.registerVariant(ModelLoader.java:233) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadBlock(ModelBakery.java:153) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.loadBlocks(ModelLoader.java:221) ~[ModelLoader.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:159) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.registerReloadListener(SimpleReloadableResourceManager.java:121) [SimpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.init(Minecraft.java:512) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:377) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_144] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_144] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_144] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_144] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [bin/:?] at GradleStart.main(GradleStart.java:26) [bin/:?] Caused by: net.minecraft.client.renderer.block.model.ModelBlockDefinition$MissingVariantException at net.minecraft.client.renderer.block.model.ModelBlockDefinition.getVariant(ModelBlockDefinition.java:83) ~[ModelBlockDefinition.class:?] at net.minecraftforge.client.model.ModelLoader$VariantLoader.loadModel(ModelLoader.java:1242) ~[ModelLoader$VariantLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 21 more [00:03:24] [main/ERROR] [FML]: Exception loading model for variant transparent_fast_tesr_test:fluid-tesr-block#fluid=12 for blockstate "transparent_fast_tesr_test:fluid-tesr-block[fluid=12]" net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model transparent_fast_tesr_test:fluid-tesr-block#fluid=12 with loader VariantLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader.registerVariant(ModelLoader.java:233) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadBlock(ModelBakery.java:153) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.loadBlocks(ModelLoader.java:221) ~[ModelLoader.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:159) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.registerReloadListener(SimpleReloadableResourceManager.java:121) [SimpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.init(Minecraft.java:512) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:377) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_144] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_144] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_144] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_144] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [bin/:?] at GradleStart.main(GradleStart.java:26) [bin/:?] Caused by: net.minecraft.client.renderer.block.model.ModelBlockDefinition$MissingVariantException at net.minecraft.client.renderer.block.model.ModelBlockDefinition.getVariant(ModelBlockDefinition.java:83) ~[ModelBlockDefinition.class:?] at net.minecraftforge.client.model.ModelLoader$VariantLoader.loadModel(ModelLoader.java:1242) ~[ModelLoader$VariantLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 21 more [00:03:24] [main/ERROR] [FML]: Exception loading model for variant forgeblockstatesloader:custom_wall#east=false,north=false,south=false,up=false,variant=cobblestone,west=false for blockstate "forgeblockstatesloader:custom_wall[east=false,north=false,south=false,up=false,variant=cobblestone,west=false]" net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model forgeblockstatesloader:custom_wall#east=false,north=false,south=false,up=false,variant=cobblestone,west=false with loader VariantLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader.registerVariant(ModelLoader.java:233) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadBlock(ModelBakery.java:153) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.loadBlocks(ModelLoader.java:221) ~[ModelLoader.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:159) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.registerReloadListener(SimpleReloadableResourceManager.java:121) [SimpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.init(Minecraft.java:512) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:377) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_144] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_144] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_144] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_144] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [bin/:?] at GradleStart.main(GradleStart.java:26) [bin/:?] Caused by: net.minecraft.client.renderer.block.model.ModelBlockDefinition$MissingVariantException at net.minecraft.client.renderer.block.model.ModelBlockDefinition.getVariant(ModelBlockDefinition.java:83) ~[ModelBlockDefinition.class:?] at net.minecraftforge.client.model.ModelLoader$VariantLoader.loadModel(ModelLoader.java:1242) ~[ModelLoader$VariantLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 21 more [00:03:24] [main/ERROR] [FML]: Exception loading blockstate for the variant forgeblockstatesloader:custom_wall#east=false,north=false,south=false,up=false,variant=cobblestone,west=false: java.lang.Exception: Could not load model definition for variant forgeblockstatesloader:custom_wall at net.minecraftforge.client.model.ModelLoader.getModelBlockDefinition(ModelLoader.java:266) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadBlock(ModelBakery.java:121) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.loadBlocks(ModelLoader.java:221) ~[ModelLoader.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:159) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.registerReloadListener(SimpleReloadableResourceManager.java:121) [SimpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.init(Minecraft.java:512) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:377) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_144] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_144] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_144] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_144] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [bin/:?] at GradleStart.main(GradleStart.java:26) [bin/:?] Caused by: java.lang.RuntimeException: Encountered an exception when loading model definition of model forgeblockstatesloader:blockstates/custom_wall.json at net.minecraft.client.renderer.block.model.ModelBakery.loadMultipartMBD(ModelBakery.java:228) ~[ModelBakery.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.getModelBlockDefinition(ModelBakery.java:208) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.getModelBlockDefinition(ModelLoader.java:262) ~[ModelLoader.class:?] ... 20 more Caused by: java.io.FileNotFoundException: forgeblockstatesloader:blockstates/custom_wall.json at net.minecraft.client.resources.FallbackResourceManager.getAllResources(FallbackResourceManager.java:104) ~[FallbackResourceManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.getAllResources(SimpleReloadableResourceManager.java:79) ~[SimpleReloadableResourceManager.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadMultipartMBD(ModelBakery.java:221) ~[ModelBakery.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.getModelBlockDefinition(ModelBakery.java:208) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.getModelBlockDefinition(ModelLoader.java:262) ~[ModelLoader.class:?] ... 20 more [00:03:24] [main/ERROR] [FML]: Exception loading model for variant forgeblockstatesloader:custom_wall#east=false,north=true,south=true,up=true,variant=mossy_cobblestone,west=false for blockstate "forgeblockstatesloader:custom_wall[east=false,north=true,south=true,up=true,variant=mossy_cobblestone,west=false]" net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model forgeblockstatesloader:custom_wall#east=false,north=true,south=true,up=true,variant=mossy_cobblestone,west=false with loader VariantLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader.registerVariant(ModelLoader.java:233) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadBlock(ModelBakery.java:153) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.loadBlocks(ModelLoader.java:221) ~[ModelLoader.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:159) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.registerReloadListener(SimpleReloadableResourceManager.java:121) [SimpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.init(Minecraft.java:512) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:377) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_144] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_144] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_144] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_144] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [bin/:?] at GradleStart.main(GradleStart.java:26) [bin/:?] Caused by: net.minecraft.client.renderer.block.model.ModelBlockDefinition$MissingVariantException at net.minecraft.client.renderer.block.model.ModelBlockDefinition.getVariant(ModelBlockDefinition.java:83) ~[ModelBlockDefinition.class:?] at net.minecraftforge.client.model.ModelLoader$VariantLoader.loadModel(ModelLoader.java:1242) ~[ModelLoader$VariantLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 21 more [00:03:24] [main/ERROR] [FML]: Exception loading model for variant forgeblockstatesloader:custom_wall#east=false,north=true,south=true,up=false,variant=mossy_cobblestone,west=false for blockstate "forgeblockstatesloader:custom_wall[east=false,north=true,south=true,up=false,variant=mossy_cobblestone,west=false]" net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model forgeblockstatesloader:custom_wall#east=false,north=true,south=true,up=false,variant=mossy_cobblestone,west=false with loader VariantLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader.registerVariant(ModelLoader.java:233) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadBlock(ModelBakery.java:153) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.loadBlocks(ModelLoader.java:221) ~[ModelLoader.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:159) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.registerReloadListener(SimpleReloadableResourceManager.java:121) [SimpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.init(Minecraft.java:512) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:377) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_144] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_144] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_144] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_144] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [bin/:?] at GradleStart.main(GradleStart.java:26) [bin/:?] Caused by: net.minecraft.client.renderer.block.model.ModelBlockDefinition$MissingVariantException at net.minecraft.client.renderer.block.model.ModelBlockDefinition.getVariant(ModelBlockDefinition.java:83) ~[ModelBlockDefinition.class:?] at net.minecraftforge.client.model.ModelLoader$VariantLoader.loadModel(ModelLoader.java:1242) ~[ModelLoader$VariantLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 21 more [00:03:24] [main/ERROR] [FML]: Exception loading model for variant forgeblockstatesloader:custom_wall#east=true,north=true,south=true,up=true,variant=mossy_cobblestone,west=false for blockstate "forgeblockstatesloader:custom_wall[east=true,north=true,south=true,up=true,variant=mossy_cobblestone,west=false]" net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model forgeblockstatesloader:custom_wall#east=true,north=true,south=true,up=true,variant=mossy_cobblestone,west=false with loader VariantLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader.registerVariant(ModelLoader.java:233) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadBlock(ModelBakery.java:153) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.loadBlocks(ModelLoader.java:221) ~[ModelLoader.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:159) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.registerReloadListener(SimpleReloadableResourceManager.java:121) [SimpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.init(Minecraft.java:512) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:377) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_144] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_144] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_144] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_144] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [bin/:?] at GradleStart.main(GradleStart.java:26) [bin/:?] Caused by: net.minecraft.client.renderer.block.model.ModelBlockDefinition$MissingVariantException at net.minecraft.client.renderer.block.model.ModelBlockDefinition.getVariant(ModelBlockDefinition.java:83) ~[ModelBlockDefinition.class:?] at net.minecraftforge.client.model.ModelLoader$VariantLoader.loadModel(ModelLoader.java:1242) ~[ModelLoader$VariantLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 21 more [00:03:24] [main/ERROR] [FML]: Exception loading model for variant slipperiness_test:boat_blaster#normal for blockstate "slipperiness_test:boat_blaster" net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model slipperiness_test:boat_blaster#normal with loader VariantLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader.registerVariant(ModelLoader.java:233) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadBlock(ModelBakery.java:153) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.loadBlocks(ModelLoader.java:221) ~[ModelLoader.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:159) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.registerReloadListener(SimpleReloadableResourceManager.java:121) [SimpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.init(Minecraft.java:512) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:377) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_144] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_144] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_144] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_144] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [bin/:?] at GradleStart.main(GradleStart.java:26) [bin/:?] Caused by: net.minecraft.client.renderer.block.model.ModelBlockDefinition$MissingVariantException at net.minecraft.client.renderer.block.model.ModelBlockDefinition.getVariant(ModelBlockDefinition.java:83) ~[ModelBlockDefinition.class:?] at net.minecraftforge.client.model.ModelLoader$VariantLoader.loadModel(ModelLoader.java:1242) ~[ModelLoader$VariantLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 21 more [00:03:24] [main/ERROR] [FML]: Exception loading blockstate for the variant slipperiness_test:boat_blaster#normal: java.lang.Exception: Could not load model definition for variant slipperiness_test:boat_blaster at net.minecraftforge.client.model.ModelLoader.getModelBlockDefinition(ModelLoader.java:266) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadBlock(ModelBakery.java:121) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.loadBlocks(ModelLoader.java:221) ~[ModelLoader.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:159) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.registerReloadListener(SimpleReloadableResourceManager.java:121) [SimpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.init(Minecraft.java:512) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:377) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_144] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_144] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_144] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_144] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [bin/:?] at GradleStart.main(GradleStart.java:26) [bin/:?] Caused by: java.lang.RuntimeException: Encountered an exception when loading model definition of model slipperiness_test:blockstates/boat_blaster.json at net.minecraft.client.renderer.block.model.ModelBakery.loadMultipartMBD(ModelBakery.java:228) ~[ModelBakery.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.getModelBlockDefinition(ModelBakery.java:208) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.getModelBlockDefinition(ModelLoader.java:262) ~[ModelLoader.class:?] ... 20 more Caused by: java.io.FileNotFoundException: slipperiness_test:blockstates/boat_blaster.json at net.minecraft.client.resources.SimpleReloadableResourceManager.getAllResources(SimpleReloadableResourceManager.java:83) ~[SimpleReloadableResourceManager.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadMultipartMBD(ModelBakery.java:221) ~[ModelBakery.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.getModelBlockDefinition(ModelBakery.java:208) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.getModelBlockDefinition(ModelLoader.java:262) ~[ModelLoader.class:?] ... 20 more [00:03:24] [main/ERROR] [FML]: Exception loading model for variant slipperiness_test:boat_blaster#inventory for item "slipperiness_test:boat_blaster", normal location exception: net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model slipperiness_test:item/boat_blaster with loader VanillaLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader.loadItemModels(ModelLoader.java:297) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadVariantItemModels(ModelBakery.java:175) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:160) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.registerReloadListener(SimpleReloadableResourceManager.java:121) [SimpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.init(Minecraft.java:512) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:377) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_144] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_144] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_144] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_144] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [bin/:?] at GradleStart.main(GradleStart.java:26) [bin/:?] Caused by: java.io.FileNotFoundException: slipperiness_test:models/item/boat_blaster.json at net.minecraft.client.resources.SimpleReloadableResourceManager.getResource(SimpleReloadableResourceManager.java:69) ~[SimpleReloadableResourceManager.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadModel(ModelBakery.java:334) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.access$8(ModelLoader.java:1) ~[ModelLoader.class:?] at net.minecraftforge.client.model.ModelLoader$VanillaLoader.loadModel(ModelLoader.java:933) ~[ModelLoader$VanillaLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 20 more [00:03:24] [main/ERROR] [FML]: Exception loading model for variant slipperiness_test:boat_blaster#inventory for item "slipperiness_test:boat_blaster", blockstate location exception: net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model slipperiness_test:boat_blaster#inventory with loader VariantLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader.loadItemModels(ModelLoader.java:305) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadVariantItemModels(ModelBakery.java:175) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:160) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.registerReloadListener(SimpleReloadableResourceManager.java:121) [SimpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.init(Minecraft.java:512) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:377) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_144] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_144] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_144] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_144] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [bin/:?] at GradleStart.main(GradleStart.java:26) [bin/:?] Caused by: net.minecraft.client.renderer.block.model.ModelBlockDefinition$MissingVariantException at net.minecraft.client.renderer.block.model.ModelBlockDefinition.getVariant(ModelBlockDefinition.java:83) ~[ModelBlockDefinition.class:?] at net.minecraftforge.client.model.ModelLoader$VariantLoader.loadModel(ModelLoader.java:1242) ~[ModelLoader$VariantLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 20 more [00:03:24] [main/ERROR] [FML]: Exception loading model for variant mapdatatest:custom_map#inventory for item "mapdatatest:custom_map", normal location exception: net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model mapdatatest:item/custom_map with loader VanillaLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader.loadItemModels(ModelLoader.java:297) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadVariantItemModels(ModelBakery.java:175) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:160) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.registerReloadListener(SimpleReloadableResourceManager.java:121) [SimpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.init(Minecraft.java:512) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:377) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_144] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_144] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_144] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_144] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [bin/:?] at GradleStart.main(GradleStart.java:26) [bin/:?] Caused by: java.io.FileNotFoundException: mapdatatest:models/item/custom_map.json at net.minecraft.client.resources.SimpleReloadableResourceManager.getResource(SimpleReloadableResourceManager.java:69) ~[SimpleReloadableResourceManager.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadModel(ModelBakery.java:334) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.access$8(ModelLoader.java:1) ~[ModelLoader.class:?] at net.minecraftforge.client.model.ModelLoader$VanillaLoader.loadModel(ModelLoader.java:933) ~[ModelLoader$VanillaLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 20 more [00:03:24] [main/ERROR] [FML]: Exception loading model for variant mapdatatest:custom_map#inventory for item "mapdatatest:custom_map", blockstate location exception: net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model mapdatatest:custom_map#inventory with loader VariantLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader.loadItemModels(ModelLoader.java:305) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadVariantItemModels(ModelBakery.java:175) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:160) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.registerReloadListener(SimpleReloadableResourceManager.java:121) [SimpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.init(Minecraft.java:512) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:377) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_144] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_144] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_144] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_144] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [bin/:?] at GradleStart.main(GradleStart.java:26) [bin/:?] Caused by: net.minecraft.client.renderer.block.model.ModelBlockDefinition$MissingVariantException at net.minecraft.client.renderer.block.model.ModelBlockDefinition.getVariant(ModelBlockDefinition.java:83) ~[ModelBlockDefinition.class:?] at net.minecraftforge.client.model.ModelLoader$VariantLoader.loadModel(ModelLoader.java:1242) ~[ModelLoader$VariantLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 20 more [00:03:24] [main/FATAL] [FML]: Suppressed additional 60 model loading errors for domain forgeblockstatesloader [00:03:24] [main/FATAL] [FML]: Suppressed additional 12 model loading errors for domain transparent_fast_tesr_test [00:03:24] [main/ERROR] [FML]: Parsing error loading recipe crafting_system_test:conditions_property_not_array com.google.gson.JsonSyntaxException: Expected conditions to be a JsonArray, was an object ({"no...y"}) at net.minecraft.util.JsonUtils.getJsonArray(JsonUtils.java:255) ~[JsonUtils.class:?] at net.minecraft.util.JsonUtils.getJsonArray(JsonUtils.java:263) ~[JsonUtils.class:?] at net.minecraftforge.common.crafting.CraftingHelper.lambda$27(CraftingHelper.java:709) ~[CraftingHelper.class:?] at net.minecraftforge.common.crafting.CraftingHelper.findFiles(CraftingHelper.java:822) ~[CraftingHelper.class:?] at net.minecraftforge.common.crafting.CraftingHelper.loadRecipes(CraftingHelper.java:668) ~[CraftingHelper.class:?] at java.util.ArrayList.forEach(Unknown Source) [?:1.8.0_144] at net.minecraftforge.common.crafting.CraftingHelper.loadRecipes(CraftingHelper.java:620) [CraftingHelper.class:?] at net.minecraftforge.fml.common.Loader.initializeMods(Loader.java:717) [Loader.class:?] at net.minecraftforge.fml.client.FMLClientHandler.finishMinecraftLoading(FMLClientHandler.java:348) [FMLClientHandler.class:?] at net.minecraft.client.Minecraft.init(Minecraft.java:534) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:377) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_144] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_144] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_144] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_144] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_144] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [bin/:?] at GradleStart.main(GradleStart.java:26) [bin/:?] [00:03:24] [main/INFO] [FML]: Registering Test Recipes: [00:03:24] [main/INFO] [FML]: Applying holder lookups [00:03:24] [main/INFO] [FML]: Holder lookups applied [00:03:24] [main/INFO] [FML]: Injecting itemstacks [00:03:24] [main/INFO] [FML]: Itemstack injection complete [00:03:25] [main/INFO] [FML]: Forge Mod Loader has successfully loaded 83 mods [00:03:25] [main/INFO]: Narrator library for x64 successfully loaded [00:03:26] [Realms Notification Availability checker #1/INFO]: Could not authorize you against Realms server: Invalid session id Anyway, I'd like to understand the proper way to run Forge, and also how to apply some test mod code to the project. Thanks.
-
I agree it is good f we're moving towards something we can keep stable for the long term. Up until now there has been a major overhaul of some aspect of Forge literally every couple months which is well-intentioned but has fractured the modding code base and left a lot of people and mods behind. Hopefully we'll be finished upgrading everything soon and we let the modding community catch up!
-
Well, I meant it is conceptually more difficult as well as inconsistent with the other registries. You want to register an entity, but you need to wrap that in an entity entry. That would be okay except you can't simply construct that but rather you need to build it through a builder class then chain the methods -- and don't forget to actually call the build() method which is different than create()! Basically, you can see the OP in this thread ran into all the problems conceptually -- initially thought they could register entities directly, finally figured out that they needed a builder for entries but then forgot to do the build(). I guess I'd like it better if it was consistent across all the registries. I think the problem is that Forge is moving to more high-end programmer style -- lots of factories, functional interfaces, builders, and such. These are all really good programming style things and of course the "proper" way to do things. However, I believe it greatly reduces the accessibility to the general modder who is just dabbling. Also, the point with an API is that it is supposed to be a "contract" that is only modified under extreme situations. The amount of work it takes now to port a mod is literally weeks per mod. Forge ideally would be an API that stands the test of time (with all the changes happening invisibly under the hood). This is why there are so many people stuck modding back on 1.7.10 -- they simply can't keep up either conceptually or effort-wise. Basically, I think Forge is doing great stuff but is getting way ahead of the actual modding community both in terms of skill level required as well as simply keeping up porting mods. Heck this morning I upgraded my 1.12.1 mod to 1.12.2 and had to spend some serious time to clear out the errors. That is a problem when a modder like me who tries to keep up and has intermediate Java level has to scratch their head just to keep their previously working mod working. It is really fracturing the modding community -- if you don't believe me go to Minecraft Forums modification development forum and you'll see that only about 1 in 10 questions is about anything after 1.8! I guess I'm hoping that all these big changes get solid soon and then stay stable for a few years. Otherwise it is really disheartening to see all your learning and work get obsolete so quickly.