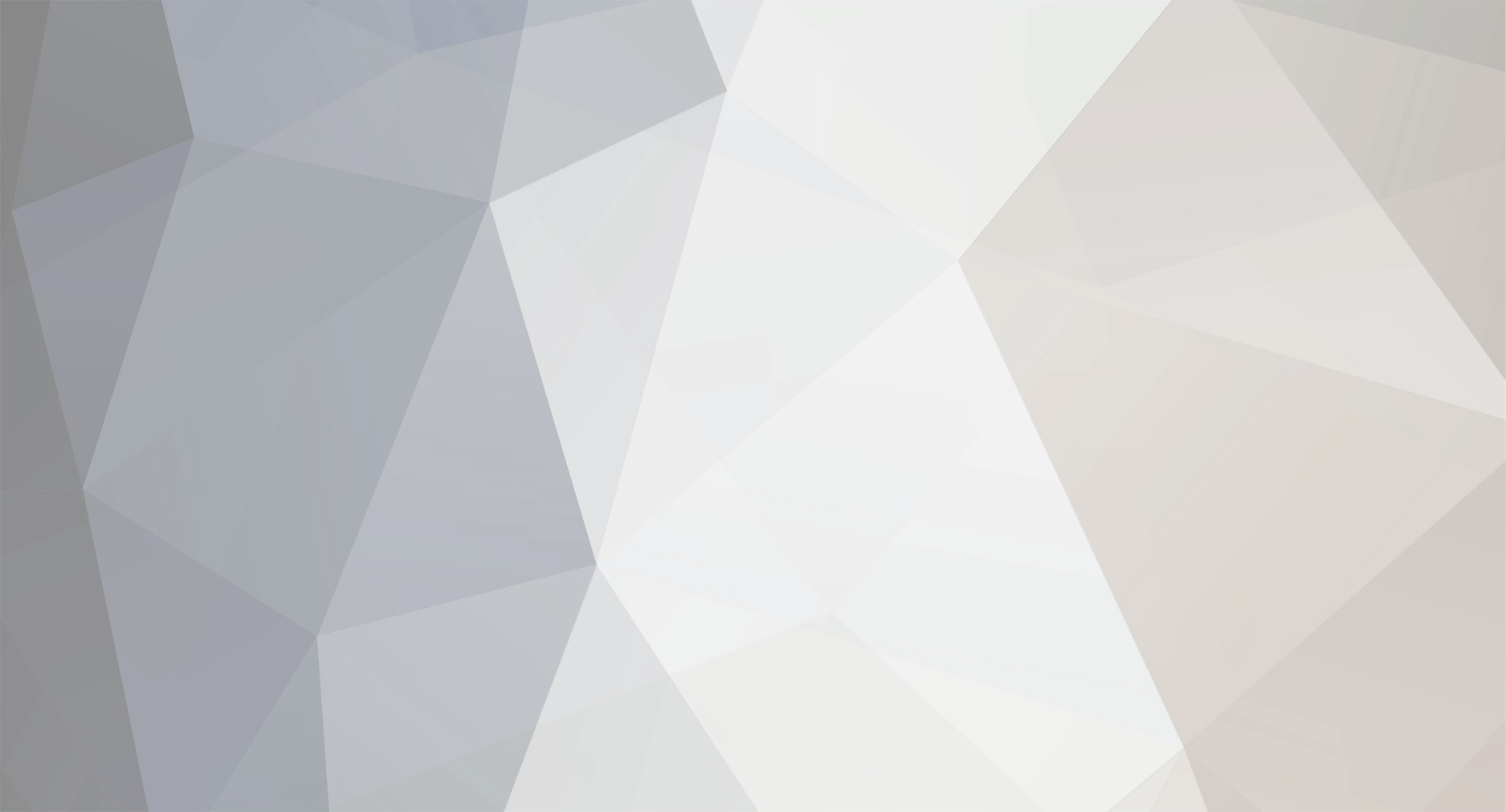
jabelar
Members-
Posts
3266 -
Joined
-
Last visited
-
Days Won
39
Everything posted by jabelar
-
Opening a player's inventory on another player's screen
jabelar replied to Asweez's topic in Modder Support
Okay, you seem a bit confused. Let me try to break it down for you. You want to do something custom, so you're going to have to do some work. Custom GUIs aren't hard, but there are a couple tricky points and it takes a fair bit of coding to get right. If you have a GUI that shows an inventory (I mean it has slots that you can move things into and out from) then it is recommended you use a Container with IGuiHandler. A Container helps map the slots in the GUI to whatever inventory you want, and the IGuiHandler ensures everything gets synced between client and server. The only thing different about your GUI is you want the inventory to be from a different player. Like we've said above, that isn't really that different -- you can simply pass that in like you would the local player's inventory. But the big question is how you find the player. Are you clicking on the other player, or are you just opening this GUI at some other point? If you're clicking on the player, then you can just treat it like an Entity interaction, check that it is EntityPlayer, cast it to EntityPlayer then take the inventory. If you are not clicking on the player, please describe when you want the GUI to open so we can provide suggestions. -
[1.8] [SOLVED]Display GUI when interacting with an entity
jabelar replied to Brickfix's topic in Modder Support
For a container Gui you should be using openGui() not displayGui(). The displayGui() doesn't do any of the container stuff. -
Opening a player's inventory on another player's screen
jabelar replied to Asweez's topic in Modder Support
Do you just want to display it? Or interact with it (like take things in and out)? I'm not sure but I think you can make a container that combines any number of inventories. The "glue" that holds a container together is the inventorySlots List. You can make a custom Container that takes in various inventories (like another player's) and then map those to slots in the container. Then they should be available for display, and they should sync between client and server (provided you implement an IGuiHandler as well). -
[1.7.10] Testing mod on server - with other players
jabelar replied to Thornack's topic in Modder Support
I think (someone can confirm) that you have to be in offline mode in the dev environment. But anyway, I just have cloned repositories on three computers, use eclipse to run a dedicated server run configuration on one computer and client run configuration on the other two computers. They seem to be able to join and play properly. Is there something beyond that that you need to do? -
I think so, yeah. Did it work?
-
Warning: This is only for 1.7.10. I haven't figured out what the equivalent for 1.8 is yet. You may want to draw the texture of an Item in a custom GuiScreen. Items don't directly have a ResourceLocation for their texture. Instead, they have an IIcon object which holds the UV-coordinates of the texture on a giant image which holds all the Item textures. The ResourceLocation is located at TextureMap.locationItemsTexture. If you want to use this in a GUI, you have to get the IIcon of the Item first, bind the TextureMap.locationItemsTexture, and then call the drawTexturedModelRectFromIcon() method to draw the IIcon of the item.
-
What do you mean that it forces you to reload the world? Does it crash, or does it come up with a message, or do you mean nothing happens until the next time you load the world?
-
Okay, it is a bit complicated but important to understand when modding. Minecraft is a client-server game, but such games often run a lot of code on the client in parallel with the server in order to prevent stuttering and lag due to network issues. But there is some code that only runs on the client and some that only runs on the server. To save memory space (I guess that's the reason), Minecraft only loads some classes on one side. If it is not on the side, Java can't see it and you'll get the class not found exception. However, a further complication is that when you run the game "client" it also runs a server and does it in the same Java executable. This means that the sided classes are actually loaded for both sides. So some code might appear to behave when you really did it wrong. Anyway, one of the solutions is to use the right classes. And furthermore there is a mechanism called the "proxy" which can help you collect methods that depend on sided classes so they only load what is appropriate. I have a tutorial on some of this here: http://jabelarminecraft.blogspot.com/p/minecraft-forge-17217x-quick-tips-for.html
-
Entities have a "navigator" that is used in the AI. I would copy the EnityAIWander code and adjust it how you want. Basically the key point is that after determining where you want to go, you need to set the enitity's navigator to tryForXYZ() where you put in the position and speed.
-
[1.7.10] local entity coordinates to global coordinates
jabelar replied to MCRaichu's topic in Modder Support
Why not look at EntityArrow code? If you look at the constructor for EntityArrow, it has this code for setting the initial position and motion of the arrow: I'm not actually sure why it calls setLocationAndAngles() because the code that follows basically changes the position and angles. Anyway, what it is doing is fairly simple: - it sets the posY to 1.0D. Since a player's eye is at 1.75D I guess that might be right to be a bit lower than eye level. - it sets the posX and posZ based on the rotationYaw, simply using trigonometry (cosine and sine functions) to pick up each dimensional component of the angle. The key here is the 0.16D multiplier -- this is how far the offset is from the entity's center. You'll probably need to change this based on the size of your entity. You'll note that it is basically shooting from the middle of the body, but slightly in front. For you then, the question is whether your weapon is in the middle of the front of the entity or not. If your weapon is in the middle it is easy, just adjust the 0.16D to value that matches distance from center of your model to the weapon. Also adjust the height from 1.0D to whatever is the weapon height. If your weapon is off-center then you have a bit more to do. You have to take the offset distance and use a trig function on the rotationYaw again. I think it would actually be the opposite function as used for the position since you wan't to offset at right angles to the way the entity is looking. You'll probably have to play around with it, but the key is that the rotationYaw and some trig functions are likely required. -
Checking if sound is playing && ISound[srsly noone?]
jabelar replied to Lua's topic in Modder Support
Awesome! Good work. -
I thought the Explosion Events were added recently to Forge for 1.8. If that's accurate, it won't help for 1.7.10. It was also added to 1.7.10 but you need to update your forge to 1299 or later. They are still updating Forge for 1.7.10. They're up to 1389 now...
-
To prevent your rockets from being affected by the explosion of other rockets, you simply need to handle the ExplosionEvent.Detonate event. In that event a list is passed in as a parameter that contains all entities that are in range of the explosion. You can simply remove your rockets from the list (if any are there) and they won't be affected.
-
[1.8] [Solved] Adding custom Mob to the entities a Zombie will attack
jabelar replied to Brickfix's topic in Modder Support
The AI lists (tasks and targetTasks) for all vanilla entities are public fields. So you can totally change the AI of any mob the way you want. You can just add to the existing list, or you can clear the existing list and build it back up with your own. -
Okay, first of all there are a few things going on with how different AI work with each other. First of all, within each list (tasks and targetTasks) there is the priority value. It will check if each AI should start based on the priority. The lower the number, the higher the priority. If two have the same priority, then they'll be checked in the order they were added to the list. You can have multiple AI running at the same time, but sometimes you don't want that (you don't want it wandering at the same time it is attacking), so there are the "mutex" bits. This is a masking system which indicates which tasks should be prevented from running together. This is why the priority matters -- if two AI are prevented by the mutex from running simultaneously, then the one that starts first will always get precedence. Lastly, there are two lists that are both simultaneously active: tasks and targetTasks. The priority list is independent in each, so they don't interact. Technically the targetTasks should simply be setting targets and the tasks should be acting on that information, but practically they can be mixed up -- they are just two lists of functionality running side by side. I have a tutorial on this here: http://jabelarminecraft.blogspot.com/p/minecraft-forge-1721710-custom-entity-ai.html Okay, however the original poster's problem is probably not related to the AI. There are a couple other things that can prevent custom entities from successfully attacking. You should read my tutorial on custom entity attacks: http://jabelarminecraft.blogspot.com/p/minecraft-forge-1721710-custom-entity.html
-
[1.8] MinecraftByExample sample code project
jabelar replied to TheGreyGhost's topic in Modder Support
One of the reasons I like answering questions and making tutorials is to get me into new topics. Sometimes the simplest question ("why isn't my custom slime entity transparent") can make you really go back and review what you know. -
You could make a custom dirt block that extends BlockDirt and behaves differently. The problem though is that Minecraft code didn't use instanceof checks (I think it should have) but instead uses instance checks (== Blocks.dirt) so your custom dirt probably won't work fully as proper dirt. I wish Minecraft code used instanceof consistently as it would give modders a lot more power -- you could extend things very easily. Anyway, this might still be the way to go since you already want it to not be normal dirt.
-
Once you figure out custom packets, like CoolAlias says it is actually pretty easy. Getting a solid packet handling system is a major step to becoming a serious modder, as there are many occasions where you will need it. So invest the time and figure it out. diesieben07 has the basic packet setup using the simple network wrapper tutorial here: http://www.minecraftforge.net/forum/index.php/topic,20135.0.html CoolAlias has a more comprehensive (but bit more complicated) system that overcomes a few of the limitations of the simple network wrapper here: http://www.minecraftforum.net/forums/mapping-and-modding/mapping-and-modding-tutorials/2137055-1-7-2-customizing-packet-handling-with
-
The player whose client sends the packet can be obtained from the message context passed with the message. In terms of server "deciding" you just have to pass enough information or instruction in the packet payload. Like I have a GUI that gives an item when closed, so when the Done button is clicked in the GUI I send a packet with a special ID number (just the first int I put into the payload) and then when the server receives the packet it acts on that ID. So the client is telling the server what to do.
-
Cool. Your code looks good to me, you have similar coding style. Regarding whether your event handling methods are in single class or separate classes, I personally throw them all into one class per event bus. Then I don't have to do the extra step of registering the class on the bus each time I decide to add some event handling. But it there is nothing wrong with having them separate.
-
[SOLVED] [1.7.10] Sticking Tile Entities Where They Don't Belong
jabelar replied to Science!'s topic in Modder Support
I think the "proper" way to do it would be to create custom blocks that extend each of the vanilla blocks you want to add tile entities to, and when you want to add a tile entity you actually replace the block with your own (which would provide tile entity). The one possible problem with this approach is that a lot of the Minecraft code compares with exact instances rather than using instanceof against the class. So when you extend a class, some code may not recognize your block. I think it is a shame that Minecraft was coded that way, because modding would be a lot more powerful if you could extend vanilla classes and expect them to be treated as equivalent to the superclass where relevant. What are you trying to achieve exactly? If you just want to store some data associated with a position in the world, there may be other ways of achieving that. -
[1.8] [SOLVED] TintIndex: Anyone gotten it to do anything useful?
jabelar replied to Zaerudath's topic in Modder Support
The description here: http://minecraft.gamepedia.com/Block_models says the following about tint index: "Determines whether to tint the texture using a hardcoded tint index. The default is not using the tint, and any number causes it to use tint. Note that only certain blocks have a tint index, all others will be unaffected." So basically they seem to be saying that it is pretty much a boolean (0 is false, all other numbers are true) and it only works for those blocks that already have a tint index "hardcoded". A search for any field with tint in the name only gives useInventoryTint in the RendererBlocks class. Overall I'm not sure what you can really do. The BlockLeaves and BlockGrass have a getRenderColor() method but they return hardcoded stuff so you can't really directly change them. -
I'm saying if you read the max health first you'd see 20 and know that you should change it. Basically, instead of just setting something, check it first. But anyway, yes, you could also figure out way to save whether you've changed things.
-
[1.7.10] How to stop potion effect particles from being rendered?
jabelar replied to Geforce's topic in Modder Support
Since particles are Entities (aren't they), can't you just use player tick and detect all entities nearby, and check for instance of EntityFX and kill those that you find? -
I think you can do it the way you're doing it but you need to check whether the entity is already damaged before setting the health. Like if the health is less than your full health value, leave it alone.