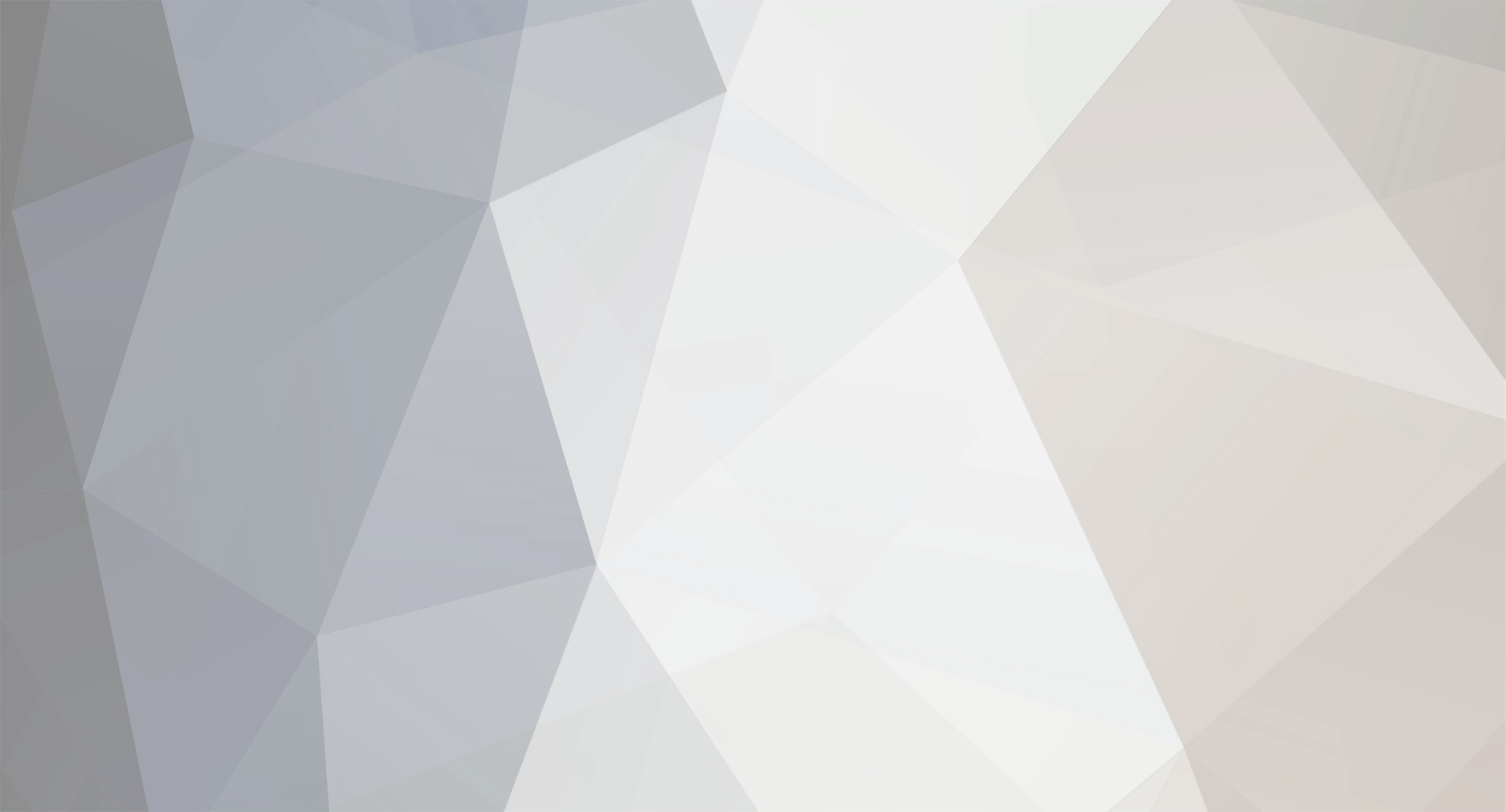
jabelar
Members-
Posts
3266 -
Joined
-
Last visited
-
Days Won
39
Everything posted by jabelar
-
With regard to multiple hit boxes, most discussions on that topic have concluded that you can't expect them to be very exact. heck, even the main hitbox is not matched at all to the entity model. To be exact you need to consider that the built-in hit box doesn't rotate, that there can be lag between server and client, and that you'd need a lot of math to get it exact. I personally think you should just be happy figuring out if you hit the head (i.e. hit at certain height or above). Beyond that I'm not aware of many successful attempts at multiple small critical hit boxes.
-
I have 1.7 living entity tutorial here: http://jabelarminecraft.blogspot.com/p/creating-custom-entities.html I'm working on creating the 1.8 version, but as mentioned above the entities haven't changed much. The steps required are listed in the tutorial, and I think mostly you can copy the 1.7 code and then just do standard update process -- your IDE will flag those methods that have changed and then you can compare the 1.7 and 1.8 source to see what the equivalent is now likely to be. I've also created a tutorial listing a number of things I've encountered while updating my 1.7 mods to 1.8: http://jabelarminecraft.blogspot.com/p/minecraft-forge-converting-your-mod.html Lastly, if you get stuck figuring out the update you can post the specific question here and we can help.
-
[1.8][Java?] Loading function (math) from string.
jabelar replied to Ernio's topic in Modder Support
Why are you concerned about performance -- do you really need to run this every tick? In terms of performance optimization, generally you should only worry about it if it is proven to be a problem. You shouldn't be stupid about performance, but you also shouldn't compromise the goal of making simple, logical, readable code unless truly necessary. A parser would be very logical and readable, so I'd only get fancy if needed. -
[SOLVED]Enabling/Disabling Custom Task for Custom AI
jabelar replied to najib333's topic in Modder Support
As Draco says, look at the type hierarchy for ItemStack and familiarize yourself with all the methods available. Anyway, there is a getItem() method for the ItemStack that you should compare to see if it is equal to the instance of your custom item. -
[SOLVED]Enabling/Disabling Custom Task for Custom AI
jabelar replied to najib333's topic in Modder Support
You need to make a boolean field in your entity as well. Then in the interact() method of your entity, check the type of item being held by the player. If it is the right item, then set the field in your entity. Your AI task should have access to the entity, and so it can then look up the field value since you copied it to the entity in the interact() method. -
[SOLVED]Enabling/Disabling Custom Task for Custom AI
jabelar replied to najib333's topic in Modder Support
Your AI class should have a method called shouldExecute(). You can check the conditions you want there to prevent it from executing if you don't want it to. There is also a method called continueExecuting() where you can check to see if there is any condition met for stopping the AI. -
I guess the other question is: what will the levels do? Like will the entities have more health, or do more damage, or something? Changing actual behavior of vanilla entities will probably require handling events.
-
Vec3 class has methods for rotation. rotateAroundX(), rotateAroundY(), rotateAroundZ(). You can take the look vector of the entity and do these rotations on that.
-
[1.8] [SOLVED] Spawning Particles Behind the Player
jabelar replied to saxon564's topic in Modder Support
You should just use the player's look vector and reverse it and multiply by distance you want behind. Add the resulting vector to the player position and spawn particles there. -
On the horizontal plane, I think you can use the rotateAroundY() method in Vec3. rotate by 90 or -90 degrees to get orthogonal.
-
Hmmm, wonder if it is a JVM thing or an Eclipse setting thing? I've got to go out for rest of today, but I'll take a video to show people that I'm not crazy...
-
[SOLVED] Is there any tool to update obfuscation mapping for mod codes?
jabelar replied to herbix's topic in Modder Support
Yes. It's easy to replace func_aaa_b to something, since aaa is an unique number. And I can simply use text replacement to achieve this. However, if func_aaa_b is mapped to A in old mappings and B in new mappings. I have to look up A in old mappings to get func_aaa_b, and then look up it in new mappings to get B. It cost more time and I can't simply replace text because it's not unique. I have to do it manually in case change other methods with the same name. That's why I am looking for a tool. I think the func_aaa_b names may be unique across entire workspace but someone else would have to confirm. -
Sure. https://github.com/jabelar/WildAnimalsPlus-1.7.10 The EntityBirdsOfPrey class is the one of interest. I have the code now using the entity rand (i.e. it works), but to see what I was complaining about, change it to worldObj.rand instead. Then you have to create several of the entities in game. There is a spawn egg for "eagle" in the creative tab. The randomness will be okay on initial creation, so then you need to save and load the game. When they are reconstructed that's when the problem occurs. Thanks for looking in to it.
-
No, it just means that the object is constant. But objects are mutable. The only place I see that sets the seed for the world Random is some stuff in the structure generation. That shouldn't cause the seed to be constant... As a workaround you could use the rand field in Entity instead. I ended up just using a new Random since it is a one-off type of thing, but using entity rand is good idea -- I checked and it works properly. I mostly wanted to understand the issue because I feel like it is fairly natural to want to do something random in entity constructor. The last thing that surprises me though is that I thought a set seed makes the sequence predictable but I thought there is still a sequence -- doesn't nextInt() generate different values even if seed is constant? Calling nextInt() several times on the same Random should provide different values even if seed is constant, right? It is really acting like the world rand instance is being re-created with a set seed each time.
-
Okay, that makes sense that it is the hashcode. Although I think a constant hashcode would indicate that the seed is constant, right? With breakpoint on the line that does next int, the seed for the world rand went like this as it went through the server constructors (these four are being loaded from save game): 93667606441033 93667606441033 93667606441033 93667606441033 then in the client constructors: 139379158755069 195327285708415 89798151390929 154026912586867 Then if I create the entity later, on the server seed is: 182302737784538 (now it is different!) And on client: 182192877199158
-
[SOLVED] Is there any tool to update obfuscation mapping for mod codes?
jabelar replied to herbix's topic in Modder Support
While a bit of a pain it isn't to bad to do it manually. Your IDE (like Eclipse) will highlight everything that changed as an error and you look at the mapping CSV file to look up what it changed to. You can usually do it in about 15 minutes. -
There is no getSeed() function but I think toString() shows the seed so I printed that out. Here is what my constructor looks like currently: public EntityBirdOfPrey(World parWorld) { super(parWorld); // DEBUG System.out.println("EntityBirdOfPrey constructor(), "+"on Client=" +parWorld.isRemote+", EntityID = "+getEntityId()+", ModEntityID = "+entityUniqueID); setSize(2.0F, 3.0F); // DEBUG System.out.println("World random seed = "+worldObj.rand.toString()); randFactor = worldObj.rand.nextInt(10); // DEBUG System.out.println("randFactor = "+randFactor); initSyncDataCompound(); setupAI(); } Here is console output for the entities constructed from loading the save game: [17:16:37] [server thread/INFO] [sTDOUT]: [com.blogspot.jabelarminecraft.wildanimals.entities.birdsofprey.EntityBirdOfPrey:<init>:70]: EntityBirdOfPrey constructor(), on Client=false, EntityID = 26, ModEntityID = 9b21931b-67ff-4ae4-be5c-6503abe1c41d [17:16:37] [server thread/INFO] [sTDOUT]: [com.blogspot.jabelarminecraft.wildanimals.entities.birdsofprey.EntityBirdOfPrey:<init>:75]: World random seed = java.util.Random@5905e924 [17:16:37] [server thread/INFO] [sTDOUT]: [com.blogspot.jabelarminecraft.wildanimals.entities.birdsofprey.EntityBirdOfPrey:<init>:78]: randFactor = 1 [17:16:37] [server thread/INFO] [sTDOUT]: [com.blogspot.jabelarminecraft.wildanimals.entities.birdsofprey.EntityBirdOfPrey:<init>:70]: EntityBirdOfPrey constructor(), on Client=false, EntityID = 27, ModEntityID = 691ba2b6-afe7-4370-9bd4-3a64a01a861b [17:16:37] [server thread/INFO] [sTDOUT]: [com.blogspot.jabelarminecraft.wildanimals.entities.birdsofprey.EntityBirdOfPrey:<init>:75]: World random seed = java.util.Random@5905e924 [17:16:37] [server thread/INFO] [sTDOUT]: [com.blogspot.jabelarminecraft.wildanimals.entities.birdsofprey.EntityBirdOfPrey:<init>:78]: randFactor = 1 [17:16:37] [server thread/INFO] [sTDOUT]: [com.blogspot.jabelarminecraft.wildanimals.entities.birdsofprey.EntityBirdOfPrey:<init>:70]: EntityBirdOfPrey constructor(), on Client=false, EntityID = 39, ModEntityID = cf32a9a0-af33-4e24-a920-15dd1926bfb2 [17:16:37] [server thread/INFO] [sTDOUT]: [com.blogspot.jabelarminecraft.wildanimals.entities.birdsofprey.EntityBirdOfPrey:<init>:75]: World random seed = java.util.Random@5905e924 [17:16:37] [server thread/INFO] [sTDOUT]: [com.blogspot.jabelarminecraft.wildanimals.entities.birdsofprey.EntityBirdOfPrey:<init>:78]: randFactor = 1 [17:16:37] [server thread/INFO] [sTDOUT]: [com.blogspot.jabelarminecraft.wildanimals.entities.birdsofprey.EntityBirdOfPrey:<init>:70]: EntityBirdOfPrey constructor(), on Client=false, EntityID = 40, ModEntityID = 64fbcf89-c902-4820-af27-9779c9c1dd98 [17:16:37] [server thread/INFO] [sTDOUT]: [com.blogspot.jabelarminecraft.wildanimals.entities.birdsofprey.EntityBirdOfPrey:<init>:75]: World random seed = java.util.Random@5905e924 [17:16:37] [server thread/INFO] [sTDOUT]: [com.blogspot.jabelarminecraft.wildanimals.entities.birdsofprey.EntityBirdOfPrey:<init>:78]: randFactor = 1 Possibly relevant is console ouptut for entities construction on the client side (it seems to be properly random): [17:16:39] [Client thread/INFO] [sTDOUT]: [com.blogspot.jabelarminecraft.wildanimals.entities.birdsofprey.EntityBirdOfPrey:<init>:70]: EntityBirdOfPrey constructor(), on Client=true, EntityID = 519, ModEntityID = e2af7ddc-47fa-47c9-b345-cad610ed0b2e [17:16:39] [Client thread/INFO] [sTDOUT]: [com.blogspot.jabelarminecraft.wildanimals.entities.birdsofprey.EntityBirdOfPrey:<init>:75]: World random seed = java.util.Random@19d4ee95 [17:16:39] [Client thread/INFO] [sTDOUT]: [com.blogspot.jabelarminecraft.wildanimals.entities.birdsofprey.EntityBirdOfPrey:<init>:78]: randFactor = 0 [17:16:39] [Client thread/INFO] [sTDOUT]: [com.blogspot.jabelarminecraft.wildanimals.entities.birdsofprey.EntityBirdOfPrey:<init>:70]: EntityBirdOfPrey constructor(), on Client=true, EntityID = 523, ModEntityID = b5a59dc1-f32e-4235-9171-30d6396a9c82 [17:16:39] [Client thread/INFO] [sTDOUT]: [com.blogspot.jabelarminecraft.wildanimals.entities.birdsofprey.EntityBirdOfPrey:<init>:75]: World random seed = java.util.Random@19d4ee95 [17:16:39] [Client thread/INFO] [sTDOUT]: [com.blogspot.jabelarminecraft.wildanimals.entities.birdsofprey.EntityBirdOfPrey:<init>:78]: randFactor = 5 [17:16:39] [Client thread/INFO] [sTDOUT]: [com.blogspot.jabelarminecraft.wildanimals.entities.birdsofprey.EntityBirdOfPrey:<init>:70]: EntityBirdOfPrey constructor(), on Client=true, EntityID = 526, ModEntityID = 948012db-6716-41fe-9e91-c120f708f8c4 [17:16:39] [Client thread/INFO] [sTDOUT]: [com.blogspot.jabelarminecraft.wildanimals.entities.birdsofprey.EntityBirdOfPrey:<init>:75]: World random seed = java.util.Random@19d4ee95 [17:16:39] [Client thread/INFO] [sTDOUT]: [com.blogspot.jabelarminecraft.wildanimals.entities.birdsofprey.EntityBirdOfPrey:<init>:78]: randFactor = 2 In both cases (server and client) the seed is constant but only on client does nextInt seem to give random number...
-
It seems logic to have it only for a singleplayer, because on a server it would be annoying(Every time somebody joins it becomes night) Checking whether you're in single player is different than checking if world is remote. Even in single player there is a server running. I think there is some other way to detect if you're in single player (and you still want to do the time change on the server in that case).
-
Any ideas about my problem though? Even with a fixed seed, my results don't make sense (unless the rand instance is somehow cloned or reset to the seed at the time of each constructor).
-
It is really simply this (other variations yield similar results). public EntityBirdOfPrey(parWorld) { super(parWorld); System.out.println("Rand ="+parWorld.rand.nextInt(6)); }
-
I agree that that is what it seems to act like -- like multiple rands are starting with same seed. But I'm pretty sure Minecraft isn't multi-threaded like that. I don't know if anyone else can replicate the issue. It is pretty simple -- take a custom entity and add line of code to print out random int in constructor, then create multiple entities and save and load the game and see what prints out.
-
I already posted the code. It is in the entity constructor. it is not just the number happening to be the same due to random chance. It returns same number no matter how many times it runs. I've got 5 entities in the save game and they all print same value, over multiple save then load cycles, including exiting fully then restarting. I'm pretty familiar with random generation in other languages and even with fixed seed I think next int method should retuRN different numbers on each subsequent call. The code can produce different numbers because entities constructed later (with spawn egg) have different values. Only those constructed during loading of saved game have same value.
-
I was trying to add some randomization so my entities would not act in sync. So in the constructor I set some fields to random values using the worldObj.rand from the entity. The entities were not random, so I tested by putting following statement into the constructor: System.out.println("Rand ="+worldObj.rand.nextInt(6)); And as multiple entities were created (from a save game, so all are being constructed in same tick): [22:10:38] [server thread/INFO] [sTDOUT]: [com.blogspot.jabelarminecraft.wildanimals.entities.birdsofprey.EntityBirdOfPrey:initSyncDataCompound:87]: Rand =3 [22:10:38] [server thread/INFO] [sTDOUT]: [com.blogspot.jabelarminecraft.wildanimals.entities.birdsofprey.EntityBirdOfPrey:initSyncDataCompound:87]: Rand =3 [22:10:38] [server thread/INFO] [sTDOUT]: [com.blogspot.jabelarminecraft.wildanimals.entities.birdsofprey.EntityBirdOfPrey:initSyncDataCompound:87]: Rand =3 Basically it looks like the worldObj.rand.nextInt(5) is giving the same value. If I stop and reload the save game, it has same value, and also if I exit completely and run again. However, I get a different value for any I construct later. In other words, all the ones being constructed during loading a game have same value, but ones that get constructed later in the game all have different values. Note that I'm running from Eclipse -- I know some dev environments will fix the seed value to make debugging easier. Is that happening here? Or when a save game is loading, maybe the world's random number generator isn't set up yet? Any other explanation?
-
Did you look at squid code? Anyway, I think in onLivingUpdate() you can check to make sure you're on server then set motionY to 0.