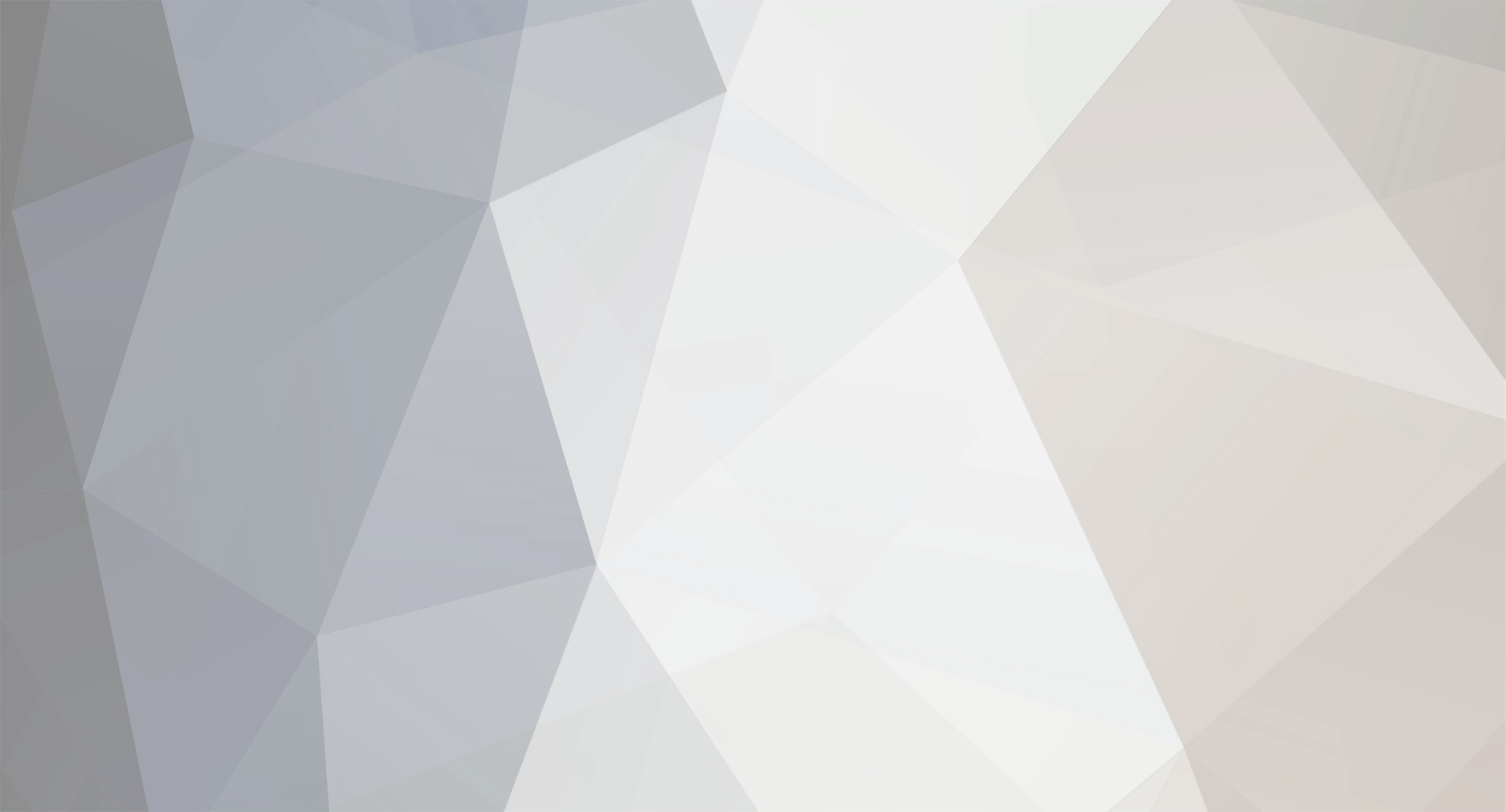
jabelar
Members-
Posts
3266 -
Joined
-
Last visited
-
Days Won
39
Everything posted by jabelar
-
[1.7.10] Player hitbox and hit mechanics - how to change stuff
jabelar replied to Thornack's topic in Modder Support
For changing reach, I would refer to Balkon's Weapon Mod source. In particular this event handling: https://github.com/Ckathode/balkons-weaponmod/blob/e223e96333734dcaff737a05482f5ce3b6eee968/src/main/java/ckathode/weaponmod/WMClientEventHandler.java Basically it looks like he creates a moving object position with a length of the reach, and if it hits something he calls the attack method. -
Whenever you want to change vanilla stuff, I recommend looking for solutions in this order: 1) Check if the vanilla classes have public fields or methods you can access directly. It is very inconsistent, but there are lots of public stuff -- for example, entity AI is contained in a public task list that you can fully edit. 2) Look for events. Forge and FML have provided a lot of events that intercept vanilla functionality that is interesting to modders. Furthermore, many events are cancelable, meaning that you can fully replace the vanilla behavior. 3) Use Java reflection to access private/protected fields and methods. You can't change the code itself with reflection, but can often affect it's execution or monitor for a field to hit a certain value. 4) Consider replacing the vanilla classes entirely with your own. This doesn't always work, but for example you can change the crafting recipes to output your stuff instead of the vanilla, or you can intercept all entity spawns (with an event) and replace it with your own version. The main problem with this is that in several places in the Minecraft code they compare against specific instance instead of using instanceof. In other words, even if you extend BlockLadder there are places in the code where it looks for Blocks.ladder which will be false for your extended class. If they were more consistent with using instanceof, this would actually be one of the most powerful techniques... 5) Advanced techniques like ASM.
-
One thing I'd mention is that the GuiScreen has a method called updateScreen() which is separate from drawScreen(). I usually put any logic for changing the GUI, such as hiding a button if I want it deactivated at some point, in that method. Regarding changing slots dynamically, the tricky part is that they will automatically be in the position you set even if your graphic changes so it looks like there is no slot. I'm not really sure how to avoid that, but maybe you could move the slot to a position off the screen or something. Alternatively, you could actually switch to a new GUI (of course you'd want to transfer the container contents over when doing so).
-
It's good you're exploring reflection. I don't use reflection often, but I'm surprised if your code works as posted. Don't you need to use getDeclaredField() instead of getField() if you're trying to access a private field? Also, I thought you'd need to set it to accessible before you got or set the value...
-
What does your KeyInputHandler code look like at this point? What is on line 19?
-
You would create a property for the block that contains some info you want to check. Then in your block model you'd map different textures to the different values of that property. This is also how you can make different textures for different sides of an orientable block like a furnace. You might want to check out my tutorial: http://jabelarminecraft.blogspot.com/p/minecraft-forge-18-block-modding.html As well as this one from TheGreyGhost: http://greyminecraftcoder.blogspot.com/2014/12/block-models-18.html
-
Yes, it doesn't actually matter what the name of the method is, or the name of the parameter either, rather the @EventHandler only looks at the parameter type passed. So a method that passes FMLInitializationEvent will be the same. Okay, in the code you posted, you're registering the key handler in the main class. That should be in the client proxy lik,e we've been saying. I personally would put all that other code into the CommonProxy and the KeyBinding stuff in the ClientProxy, although it is technically okay to have the common stuff in the main class method.
-
[1.7.10] Changing the camera - how to force 3rd person view
jabelar replied to Thornack's topic in Modder Support
I think it is orientCamera() method in EntityRenderer class. -
[1.7.10] Changing the camera - how to force 3rd person view
jabelar replied to Thornack's topic in Modder Support
it's weird to me that setting the third person view field isn't enough to make it work. Because unless someone presses F5 again, it should be stable even with respect to the rendering timing. I understand that if we were trying to overwrite the value while Minecraft was also trying to overwrite it, but I don't see how that is the case here. -
[1.7.10] Changing the camera - how to force 3rd person view
jabelar replied to Thornack's topic in Modder Support
In Eclipse, select that field and right-click and choose Call Hierarchy. It will tell you everywhere it is used. interestingly, in 1.8 I tried to put the following into the PlayerTickEvent handler: EntityPlayer thePlayer = event.player; World world = thePlayer.worldObj; if (world.isRemote) { Minecraft.getMinecraft().gameSettings.thirdPersonView = 2; // thePlayer.posY -= thePlayer.eyeHeight; } And the position is correct but it displays a girl skin instead of the "steve" normal skin! -
[1.7.10][Forge-10.13.2.1291] possible bug with ClientTickEvent
jabelar replied to N247S's topic in Modder Support
No, I'm saying that is the other way around. Minecraft#runTick() always calls the onPreClientTick() which posts the ClientTickEvent.START. So you should see the event as long as there is a Minecraft instance executing runTick(0. Anyway, as Ernio says "client" doesn't strictly mean "world". It just means user interface is running. -
How exactly are your 100 variants stored? In metadata? Yeah, JSON method can make algorithmic model/texture settings a bit complicated. I think you can override the Item#getModel() method to algorithmically reference different models, but I think that still requires a JSON per variant. Note that you can probably make a script (heck probably even just use EXCEL) to create the JSON content for you. Definitely a pain, but as you can see in the default Minecraft asset item JSON models, they went ahead and make a hundred of them. Maybe someone knows a better way though.
-
[1.7.10][Forge-10.13.2.1291] possible bug with ClientTickEvent
jabelar replied to N247S's topic in Modder Support
That does seem a bit weird. If you follow the call hierarchy for ClientTickEvent with START phase, it is only called in the onPreClientTick() method, and that is called in the Minecraft classes runTick() and it isn't really qualified in any way (no if-then that might prevent it). So I assume it is being posted as long as a Minecraft is doing a runTick(). So I guess that class is still instantiated and calling runTick(). -
On client side, is it only something visible in the GUI? Or are you doing something like making the block look different for all players (like show it turned on using different model, texture or particles)? In the first case, as mentioned by diesieben07 you should use container and gui handler (this creates built-in sync). In the second case, you'll need to send packet to all players.
-
Yeah, that's not the way to use proxies. In the approach that I use is that your main class should have methods that handle the FML "life cycle" events (what people typically call "pre-init", "init", "post-init"). Those should call the same method in the proxy instance. E.g. in my main class I'll have something like this: Then your Common Proxy should have the same methods and should do whatever is actually common, for example in my CommonProxy: Then in the ClientProxy which extends CommonProxy, call the super method for the common stuff then add the client-specific stuff: So main class handles the init life cycle event by calling the proxy, and proxy will have appropriate methods based on side.
-
One thing to be aware of is that some vanilla code compares against the instance of the block (e.g. Blocks.ladder) instead of checking for instanceof (e.g. instanceof BlockLadder). This means that extending a class and replacing the vanilla blocks may not work fully as expected. I suspect that many mods are also coded that way. If you want to really do a wholesale change to most blocks and have them work correctly, you need to actually make the built-in Blocks instance take on the value of your block. Since Blocks instances are static and final, you'll need to use techniques like reflection or ASM.
-
I give some explanation of this in one of my tutorials: http://jabelarminecraft.blogspot.com/p/minecraft-forge-1721710-gui-and-input.html
-
Yeah, key bindings are only supposed to be on the client -- this allows each player to have different key bindings if they really wanted. As explained above, use proxy to only do the key binding stuff on client side, then send packet to server to get it to respond in whatever way you want. I have a tutorial here (on the key binding part): http://jabelarminecraft.blogspot.com/p/minecraft-forge-1721710-keybinding.html
-
Zootaxz, I don't think you understand some of the important things about metadata. Here are some things to consider: 1) in 1.8, metadata is now sort of hidden from the code and instead you should use a Property for the block, which can vary in each block's IBlockState. There is still metadata though, so you need to have methods in your block to convert from state to metadata and from metadata to state. 2) The built-in metadata is only 4-bits per block position, so you can only have 16 variations in one block. 3) The JSONs map the property values to the models, and the models map to the textures. If you implement the above three points, then Minecraft will properly save the metadata and also sync it between client and server for you. It seems that you're trying to create some entirely new metadata system? How are you syncing between client and server? How is it getting saved and loaded? I think the problem is that you can certainly create all sorts of classes and fields to hold extended data, but you also have to consider the syncing and saving processes.
-
In the cases where you extend a class but it isn't being recognized as that class in Minecraft code, it is usually due to the fact that the Minecraft code must being doing a comparison with the actual instance (Blocks.ladder) instead of checking for instanceof BlockLadder. Your custom class will never test true against Blocks.ladder. You may have to play the sound yourself by checking if player is moving on it. Might be easy or might be tricky, not sure.
-
You shouldn't resurrect such old threads. But since you did, there is also the concept of rendering passes that helps sort through some of this, plus some other settings about side visibility and opaqueness. TheGreyGhost has a detailed explanation here: http://greyminecraftcoder.blogspot.com.au/2013/07/rendering-transparent-blocks.html
-
Hmmm, that's weird... the error message changed a bit. It previously was just complaining about the <init> which didn't give me much to go on. Okay, the null pointer exception is fairly obvious -- the entity is supposed to remember the entity that summoned it and I'm referencing that when it is null. But I need some advice on how to prevent that. In my "extended constructor" which I use when spawning the entity on the server side, I pass the summoning entity into the constructor. But it seems that the client calls the "simple constructor" -- which only sets this to null. What is best way to pass this extra data so that when client gets instruction to create the entity it also gets this extra data? I'm guessing I should use the additional spawn data interface?
-
Okay, I had my code working great -- in this case one entity can randomly spawn another entity. But then I made the mistake of doing a lot of code modifications without retesting this particular feature, now I checked it out and it is broken. I know I can go back to earlier code, but due to the extensiveness of my edits I'd like to see if I can just directly get some advise on what might be wrong. Here is the error: Here is the code that tries to spawn the entity: The code runs, as you can see by the console statements I show in the error log. Lastly, here is the constructor code for the entity I'm trying to spawn: You can see that my EntityMysteriousStranger constructor call happens on the server (console says "extended constructor"). The sync messages after that are for the entity that is trying to spawn the entity (it is supposed to set flag indicating that it has spawned, to prevent it from spawning in future). the EntityMysteriousStranger seems to have had an ID assigned and position set correctly. Then the client calls the simple constructor (the one that only take World parameter), and then it crashes. I thought it might be due to the registry, but I have plenty of other entities working in the mod. But in case it matters, my entity registration code looks like: Any ideas of what is causing that crash?
-
I've made a list of some of the things i had to change from 1.7.10 to 1.8. You can see that here: http://jabelarminecraft.blogspot.com/p/minecraft-forge-converting-your-mod.html My list doesn't cover everything, because my mods focus on entities, but it should give you a bunch of ideas on areas needing conversion.