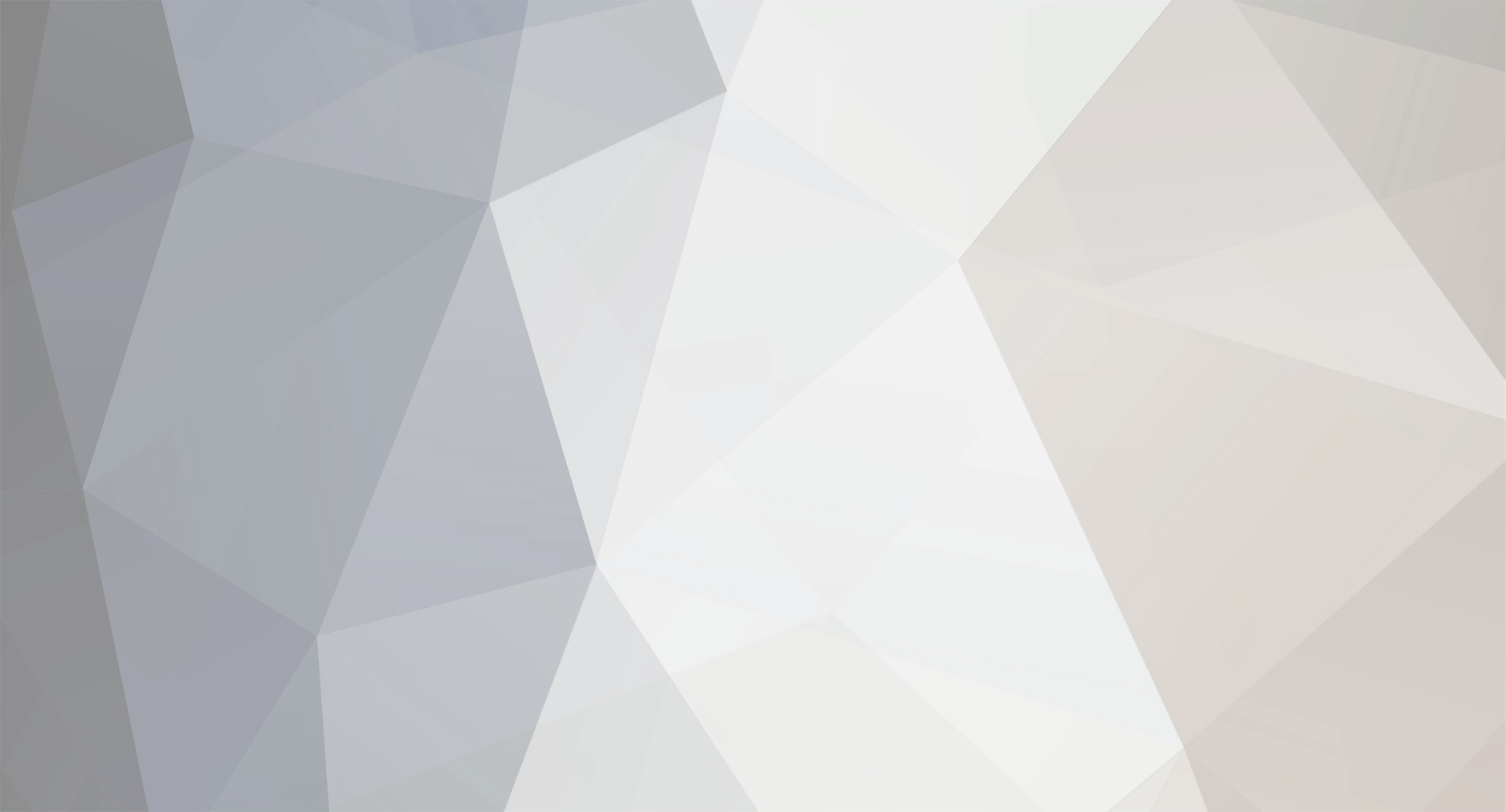
jabelar
Members-
Posts
3266 -
Joined
-
Last visited
-
Days Won
39
Everything posted by jabelar
-
I'd recommend my own. ? http://jabelarminecraft.blogspot.com/ I also give some links to other tutorial sites there. Plus google should show lots of hits, also if you prefer videos search YouTube.
-
I have a tutorial and example code for custom trees here: http://jabelarminecraft.blogspot.com/p/minecraft-modding-custom-tree-with.html Maybe something there will help.
-
Maybe this would be clearer. In a tick handling event like ClientTickEvent, in one phase of that event, you would update the position in a public static field. This would have a very regular steady movement. In the render handling event, you would get the position from that public static field and add a multiple of the partial ticks times the increment. Note that the tick value can be also updated in other ways. For example, the world keeps track of total time, so you could record the world time when the GUI was just opened and then calculate how many ticks it was open in the render event. I didn't mean to cause confusion. Just wanted to point out the way Minecraft normally handles the conversion from ticks to render frames for animations.
-
Doing your own delta time works, but there is another more common approach which I figured is worth mentioning. The game logic runs in a loop that "ticks" 20 frames per second. The render refresh rate can vary depending on your monitor, settings and power of your GPU. So whenever you're doing animations in Minecraft you should set the positions and angles based on the game ticks, not the refresh rate. So you would really increment the position every tick rather than every event (which fires at the refresh rate). However, doing that has the side effect that it will look a bit jerky because it will only update position 20 times per second which is a bit less than needed for perception of smooth motion (traditional movie film used 24 fps). So to smooth it out, there is the concept of "partial ticks" that are passed to the rendering methods (and events). So an alternative, arguably more common, way to do animations is to update the position using the ticks plus a bit of adjustment using the partial tick. For example, the game overlay event has a getPartialTicks() method to help with this. Just thought you'd be interested. Point is that partial ticks are used to communicate difference between refresh rate and tick rate and are commonly used to control animation speed.
-
Also, a block pos contains fields for each of x, y, z so if you can do any math you want. Although most common operations, like getting block beside or above already have dedicated methods as mentioned. But don't be confused by BlockPos -- it just contains x, y, and z which are directly accessible if you need it.
-
When asking a question you should explain why you're asking. There is probably a proper way to achieve what you want. For example, if you just want to enable a block in the game based on user settings, that is quite possible, but not by mucking with conditional registration. Let us know what you're trying to do and we can advise on best way to achieve it.
-
The problem is that the "getHeldItem" methods actually return ItemStack not Item. The method should really be named getHeldItemStackOffHand() instead. So you simply have to get the Item from the Itemstack, so add .getItem() at the end. like: playerSP.getHeldItemOffhand().getItem() instanceof ItemBlock
-
You can also just have some patience and wait until the Forge 1.13 is made available. You'll be able to see all the source then, as well as all the ways Forge has been modified to work with it.
-
Is that your own code? Because you are already checking the items in the hands in your code, so it seems you already know the right methods (getHeldItemOffHand and getHeldItemMainHand) and that code shows good knowledge of Java programming? So what exactly is your problem then?
-
[1.12.2] How to draw a black rectangle in the code?
jabelar replied to Romsik788's topic in Modder Support
If you're running your code in a Gui or child class there are methods for drawing rectangles. For transparency that can be the alpha value in the color, but you may also need to set the blend mode using GlStateManager. -
Why shadow size instead of the actual width of the entity?
-
The way modding works is actually pretty interesting. Java is a weird language because it was designed originally to be interpreted in real time by a "virtual machine". This had the advantage that the same program file (in this case the JAR) could be used on any type of computer -- you don't have to download different one for Windows, Android, Linux, Mac, etc. or different hardware targets (like x86 versus 64-bit, ARM, SPARC, MIPS, etc.) because the Java virtual machine and related runtime environment would take care of doing the final step of turning your program into machine language. The downside of this approach is that it is easier to reverse-engineer the code because the program contains information at the higher level language rather than machine bytecode. You can try to make it difficult for people to reverse-engineer by obfuscating the code meaning to take all your code and turn the names into meaningless things, and making all the formatting sort of random. For example, instead of having a field called firstNameString it will be changed to something like f_354356_b. The computer doesn't care because a field can have any name, but it makes it harder for any humans to guess at the functionality. However, it isn't impossible to guess. So you can basically de-obfuscate if you have a list of better names. So modding starts with that idea -- you can take the minecraft JAR and de-obfuscate it and start to understand it. Once you have the deobfuscated code, you can modify it and run it yourself except each modification would be on its own and you couldn't combine them. So instead people decided to add patches to the code that provided "hooks" (an API) that then allow code in your own source to execute in line with the vanilla code. Forge events are a good example -- the patch to the minecraft source just adds a call to a forge event post method in a forge source class. Then modders can run their own code without actually modifying the minecraft source. From a licensing point of view, forge is still honoring the fact that you are not allowed to redistribute the minecraft source. Instead, it relies on people getting the minecraft jar and going through the deobfuscation step to make it accessible to modding. So it SEEMS like Forge is giving you all that source code, but really it is just aiding the process of making it available to be human-readable in your programming environment.
-
You're confusing things. Most of the source code in any of the minecraft packages is minecraft code -- meaning those lines were written by minecraft coders, not forge coders. Forge does apply patches to many of the classes, but only very few lines. In fact if you contribute to Forge you are specifically asked to minimize the amount of change including even avoiding adding any extra whitespace. The methods being discussed in this thread are minecraft methods.
-
Weird visual error. Can't figure out cause. Help?
jabelar replied to Fakeiman's topic in Modder Support
It is definitely difficult when a problem only happens occasionally to debug what is happening because it can take a while to even confirm that a suspicious mod is indeed the problem. However, it is mostly likely an interaction between your mods. Meaning it is some combination that isn't working. And that is hard to isolate when you have so many mods. You might start by figuring out which mods affect rendering so much, since not all mods do. If that list is somewhat short you can experiment with combinations of them. Also, is there any errors in your logs? Usually when things start to go poorly a mod will spit out an error. Like missing texture or missing model or something. -
Use Eclipse (or whatever IDE you're using) and look at the declaration for that method and then look at the Call Hierarchy for that method. The declaration with show you the code within the method and the Call Hierachy will tell you everywhere it is used (and you can check those out to get better examples).
-
In Minecraft, the Gui#drawTexturedModalRect() method is a good reference. It assumes a texture that is already bound but then takes in the x, y position to draw, the textureX and textureY position within the texture, and the height and width.
-
[1.11.2] How to generate structures bigger than a chunk
jabelar replied to GooberGunter's topic in Modder Support
He still has some old tutorials posted on GitHub: https://github.com/doctorhealt3/TheXFactor117s-Forge-Tutorials/blob/master/OldTutorials.md but I can't find anything relevant to this topic. -
Is this item capability only relevant when it is held? If so you might want to change it from being an item capability to being a player capability, as that might be easier to keep synced. Basically have player capability for "holding special item in my main hand" or whatever you need to trigger the rest of your logic.
-
Weird visual error. Can't figure out cause. Help?
jabelar replied to Fakeiman's topic in Modder Support
If you're lucky someone might have seen this before and know the answer. Otherwise you'll have to figure it out the old-fashioned way. You have to remove mods until the problem goes away to try to isolate the mod(s) that are causing it. You have a heck of a lot of mods, so that will take some work. I would start with a "binary search". I mean I would get rid of half the mods and see if the problem still occurs. If not try the other half and confirm it still occurs. Then take the half that has the problem and cut that in half and try both halves. You keep doing that until you get down to the specific mod. This is a fast approach because even though you have 253 mods, you only have to cut it in half 8 times (groups of 128, 64, 32, 16, 8, 4, 2, then 1) Now it is very possible that the problem is due to an interaction between two mods. For example you could cut the mod pack in half and find that neither half shows the problem. In that case you're going to have to crawl through one by one until you find one that is involved and then crawl through the rest to figure out which one is interacting poorly. This forum may not be the best place to ask. This is for people who write mods. Other forums for making mod packs are more likely to find someone who has seen this before. -
[1.12.2] How to translate parts of custom entity up and down? [SOLVED]
jabelar replied to Electric's topic in Modder Support
Wow, that's a pretty crazy model (in terms of there is a lot of boxes). Instead of changing the offset I think you need to use the setRotationPoint() method. That is what places the rotation point in 3D space relative to the entity position. The way it works, if I remember correctly is: 1) You create a box by specifying the size in x, y, z. 2) You also specify the offset which is the point WITHIN the box where rotation occurs. 3) You then need to specify the rotation point which is actually placing the block in space. 4) You can then set rotation angle (around that point). Note that I believe that the naming of the methods is sort of misleading. You would think that setting the rotation point would imply setting the point within the block where it rotates; but it is the opposite, the offset does that and the set rotation point actually moves the whole block. Anyway, that is what I remember from when I used to do complicated animations. Also, for easier debugging you might want to try a much simpler model. Like maybe just make one block that you can control up and down first. -
II don't have time to review your code, but I've got some tutorial information on fluids here: http://jabelarminecraft.blogspot.com/p/minecraft-modding-fluids_18.html. Maybe something there will point out your errors.
-
[1.12.2] How to translate parts of custom entity up and down? [SOLVED]
jabelar replied to Electric's topic in Modder Support
In your model class you should be able to change the position of each box part. Note that changing offset will change the rotation point within the block -- it will appear to move but will also rotate differently. Changing the pos will move the whole thing and keep the rotation point in same location. In any case can you post your whole code, at least for the model class? -
If you handle the GuiOpenEvent you can check for an inventory gui and replace it with your own version. What exactly are you trying to achieve?
-
Best practice? Branching versus copying to start new mod
jabelar replied to jabelar's topic in Modder Support
Library idea is good for my utility classes, I will look at setting it up that way. But mostly my starter mod is more like a template -- already has all the classes and packages organized the way I like so for a new mod I can just get right into coding the new stuff. So probably just copying makes the most sense. -
Best practice? Branching versus copying to start new mod
jabelar replied to jabelar's topic in Modder Support
Well the reason I even contemplated it is that my starter mod contains all my mechanisms I like -- all registrations and things are all set up, etc. Of course copying the files gives me all that too. But even for different versions, do you recommend using branches or copy? I found that the amount of change is so large that the commonality is barely worth it. Once you change all the registration mechanisms (like the move to registry events), change achievements to advancements, IEEP to capabilities, go through and fix all the SRG name changes, get rid of hacks that are now handled by proper new Forge mechanisms, and so forth, seems like I have to touch almost everything anyway.