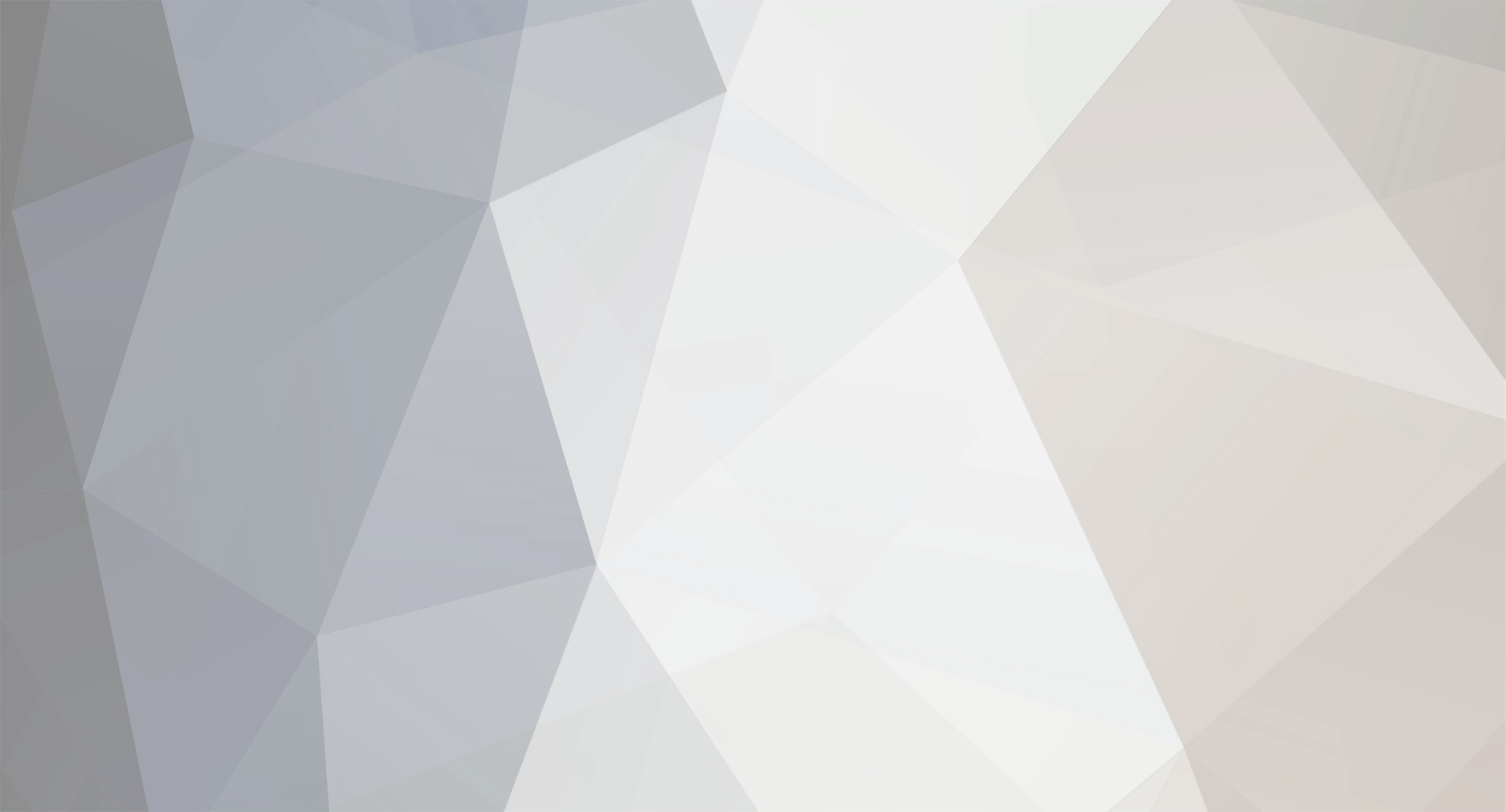
jabelar
Members-
Posts
3266 -
Joined
-
Last visited
-
Days Won
39
Everything posted by jabelar
-
A structure is just a bunch of blocks, so you just place blocks like you would normally with the world.setBlock() method.
-
Direct rotation (not "smooth" and prettier 359 -> 0)
jabelar replied to david476's topic in Modder Support
I'm still not sure of your problem. You're saying that if you change the rotation, that it will slowly go towards that rotation and in case where it is near 360 it will go the wrong way around? I thought setting the rotation would immediately rotate it. I'm not sure where it is doing the "smooth rotation" code. But you need to find that because that is where it would have to handle the case of going past the full circle. -
I've coded my own structure generator, but it isn't really ready to share. There are some others out there you may want to look at. But here is the the general approach. First of all, you need to decide whether your structures are made of "regular" pieces (like straight walls, perfect boxes, etc.) or irregular. If they are regular, you can make methods for each regular part like generateWall() or generateHollowCube(). These would simply consist of nested for loops that cycle through all the locations and place the desired block. I personally tend to have irregular structures. So in that case I use a "schematic" method. This means I have a file that basically maps out the placement of every block. Then my generation method simply reads the file and places blocks as indicated. There are several tricks though. First of all, a structure has a LOT of blocks in it. A 20 x 20 x 20 room could have 8000 blocks! So at the time of generation there can be significant lag. I have worked on a way where the structure is built over several game ticks so that there is no lag, but frankly it is an intermediate programming level to get this right. Also, because there are so many blocks, you need to use an external text file for the schematic -- otherwise you'll reach the limit of the size of a Java class. Secondly, the order that you place some blocks matter, like if you try to place a torch before there is a wall to attach it it will cause trouble. So I actually do three "passes" in the generation. First I place all the regular blocks with no metadata that are also not special (I'll explain that in a sec). Then I place blocks with metadata. Then I place some special blocks like tripwire. If you place tripwire before there is ground to place it on, it will just become an EntityItem rather than proper block. Thirdly, rotation of a structure is EXTREMELY difficult because unfortunately the metadata is not consistent. Rotating a bed to the East is different than rotating a door to the East, so you have to do a huge number of if statements to get it right. I personally find it easier to make separate schematics that are already rotated than to have one schematic and try to rotate it via code. But generally the idea is simple -- create a schematic in a text file (make this an asset resource), read the file back and place the blocks according to the schematic.
-
What about doing this differently -- why not try to intercept the textures where they are used, or during the render? Also, as diesieben07 says, you should be able to replace textures using resource pack instead.
-
Direct rotation (not "smooth" and prettier 359 -> 0)
jabelar replied to david476's topic in Modder Support
I'm not really sure why that code would cause any problem with rotating past 360. You're just setting the rotationYaw of your ship to match your player that is riding it. Where is the "smooth" rotation happening that is causing the problem? -
Direct rotation (not "smooth" and prettier 359 -> 0)
jabelar replied to david476's topic in Modder Support
Can you post your code? You're probably just not handling that case (of going past 360) properly. -
[Solved]How to find out if a player is on fire
jabelar replied to The_Fireplace's topic in Modder Support
I think you need to check the field in Entity called fire. That is an int field that indicates how much longer you will burn (and isn't masked by immunity, although immunity will make it count down faster). The problem is that the field is private. So you will need to use Java reflection. So basically use Java reflection to see if the fire field is > 0, if true then it is on fire (whether or not it is taking damage). -
how to detect if a class implements an interface
jabelar replied to memcallen's topic in Modder Support
We're just saying that it should work. And when something that should work doesn't work, then it is time to trace things really carefully. The code you've posted it is not at all obvious that you've confirmed that the te is really what you think it is. Maybe you did try it, but you haven't shown any proof. Based on the code you listed, there is is only a few possible causes: 1) the class isn't actually an instance of that interface. Can you post the code for the tile entity class? 2) the tile entity is null. Again the code you've shown so far would not prevent that case, so it would be good to show proof. 3) there is a tile entity there (not null) but not the one you expect. Maybe you have this last case. As strumshot mentions, another way to confirm whether it is actually an instance is to try to cast it into the interface. If that fails then it would tell you something. Anyway, debugging isn't about trusting that you know something is correct. Obviously something you think is correct is actually wrong. This happens all the time in programming -- every bug is something you think that should work but doesn't. The only thing to do is to exactly trace everything all at the same time. You can check any source on Java programming and instanceof should work for testing classes that implement an interface, as per the stackoverflow link above also attests. You can also try the isApplicable() method alternative. -
Detect all nearby entities to player and then shoot lightning at them
jabelar replied to Xile's topic in Modder Support
I'm not sure, but I think the bounding box minX, maxX, etc. are absolute positions. I mean you're looking between 0 and 10 but do you even know where that is in the world? How are you sure there are even any entities there? I think that might be way underground or something. -
how to detect if a class implements an interface
jabelar replied to memcallen's topic in Modder Support
In programming, if something isn't working the way you expect then one of your assumptions is wrong. You have to check everything explicitly. You think you have a tile entity there, but obviously it isn't being detected. So you need to prove that the position you're checking is correct, that the tile entity is actually not null, and so forth. This is debugging. You don't fix it by asking "what's wrong with my code", you fix it by *tracing* your code exactly. At some point your code is going wrong, so you need to go step by step and prove where it goes wrong. You have to prove that the te is not null at that position before you complain about the instanceof not working. Just saying "I'm sure" isn't enough -- print it out right at the point in the code where you are checking it. -
getIcon() for BlockDoor always returning NullPointerException [SOLVED]
jabelar replied to Althonos's topic in Modder Support
Can you show the code where you declare the icons[] array? Do you do it differently in each case, because in your first example it seems you've already got a length implying that the array is declared with a size already, and possibly already initialized somehow... Can you show the code where you register the block itself? It is possible that perhaps you're somehow placing a block that is improperly registered I guess. But it is a strange problem, as the code generally looks okay to me. -
Yes, just add different amounts to the rotation depending on how far along you are in the animation.
-
how to detect if a class implements an interface
jabelar replied to memcallen's topic in Modder Support
Well even though you might be confident that the tile entity below the position is there, in computer debugging usually you'll find that something you're sure of isn't actually true. So if I were you I'd add more console output to confirm. I would output the x, y, z coordinates and I would output the name of the block found at the position. I would also put in the else clauses for each if statement and put a console printout there too. for example, you test whether the te is null but it is not clear if that is true or not because you don't get any output. so if you have console statement showing all the information (position, whether te is null), then you'll be able to debug. -
getIcon() for BlockDoor always returning NullPointerException [SOLVED]
jabelar replied to Althonos's topic in Modder Support
Yeah problem is definitely that icons[0] is null at the time. But the register block icons is the common way to do it so I think the timing should already be controlled. Maybe put console statements in each method to confirm which order they are called in. -
getIcon() for BlockDoor always returning NullPointerException [SOLVED]
jabelar replied to Althonos's topic in Modder Support
Well, if you look closely at your code you're checking if icons[0] is null and then you are returning it (you assign it to newIcon, but then newIcon will be null) -- so that is probably why you're getting the null pointer exception. The more interesting question is why icons[0] is null in the first place. Your registration method looks correct to me. -
You can't use a for loop because it will be executed all at once and no one will see the animation because it will complete faster than they can see. Instead you need to do what I said which is create a counter that is incremented each time the render is called.
-
Well, you just have to use Java to control the animation. You already have a render() function I assume and you will just need some variable to keep track of the progress of the animation and change that into rotation (or whatever you need for the animation). So when the GUI opens you might set a counter to 40 (for two seconds) and then count it down each time you render. In the render you'd have an array with the rotation angle you want per tick, and the index would be this counter. Then just use GL11 to rotate by the amount you need. Does that make sense? Have an array of rotation angles and step through it with a counter to create the animation duration over time.
-
how to detect if a class implements an interface
jabelar replied to memcallen's topic in Modder Support
instanceof is the proper way to do it. What do you mean it didn't work? -
Okay, it is okay if you're new to modding. Basically, in Minecraft Forge they have broken up the execution into "sides", meaning client and server. Apparently to be more efficient, they decided they'd rather not load client classes (like rendering and keyboard input) on the server side. So they came up with an annotation @SidedOnly which will actually prevent classes from being loaded if they're not needed on the current side. However, this causes trouble for Java runtime because it will scan all your code and may find something that references something that will only be loaded on the other side and this can cause a crash. So sometimes you need to ensure to similarly annotate the side of your class. Furthermore, they understood that whole processes of loading the mod would differ for each side. For example, you wouldn't want to register renderers on the server. So they came up with the concept of a sided "proxy". Basically, while not technically accurate you could think of the proxy as containing those parts of your main class that are loaded based on the side you're on. People often handle the pre-init, init, post-init FML life cycle events in the proxy because there are some things (like renderers, keybindings, networking, etc.) that may differ by side. Anyway, you should read up on this concept. It can take a while to get an understanding. But basically you take the stuff from your main class that depends on side and put that into the appropriate proxy class. Back to the specific topic of a version update checker, on the client and server side you likely want different behavior on different sides -- on client you might display an actual GUI or chat message, whereas on server you might just output a console or admin interface message. So that is why I suggested running it from the proxy. I suggest reading TheGreyGhost's tutorial here: http://greyminecraftcoder.blogspot.com/2013/11/how-forge-starts-up-your-code.html
-
As diesieben07 pointed out, you shouldn't really be doing the version check on the server, or at least if you want to do that you'd probably do it specifically for server. Mostly you want to tell the client, especially in shieldbug1's attempt where he was doing it every time a player joined. So I just run the thread in the client proxy, so then it is only run on client (or integrated client). From your question, it sounds like maybe you're not confident in how the proxys work. Do you need further explanation for that? Anyway, if you run it in your main class, then it will be run on both sides (both client and server) and that may not be really appropriate for this purpose.
-
I know, I already noted in my post above that the catches needed further work -- I just coded it and posted quickly to confirm the general approach.
-
I'm not really sure. There are even (I think) some additional buses like networking happening behind the scenes as well.
-
Okay, I tried an actual implementation of Jwosty's suggestion and this seemed to work. First I made a version checker class as a runnable (allows it to execute in own thread). import java.io.IOException; import java.io.InputStream; import java.net.MalformedURLException; import java.net.URL; import org.apache.commons.io.IOUtils; /** * @author jabelar * */ public class VersionChecker implements Runnable { public static boolean isLatestVersion = false; public static String latestVersion = ""; /* (non-Javadoc) * @see java.lang.Runnable#run() */ @Override public void run() { InputStream in = null; try { in = new URL("https://raw.githubusercontent.com/jabelar/MagicBeans-1.7.10/master/src/main/java/com/blogspot/jabelarminecraft/magicbeans/version_file").openStream(); } catch (MalformedURLException e) { // TODO Auto-generated catch block e.printStackTrace(); } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } try { latestVersion = IOUtils.toString(in); } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } finally { IOUtils.closeQuietly(in); } System.out.println("Latest mod version = "+latestVersion); isLatestVersion = MagicBeans.MODVERSION.equals(latestVersion); System.out.println("Are you running latest version = "+isLatestVersion); } } Then in ClientProxy class (because we want to check on client) I had this code: @Override public void fmlLifeCycleEvent(FMLPostInitializationEvent event) { // DEBUG System.out.println("on Client side"); // do common stuff super.fmlLifeCycleEvent(event); // do client-specific stuff Thread versionCheckThread = new Thread(new VersionChecker(), "Version Check"); versionCheckThread.start(); } Lastly, I created a text file in my project containing just a version number on the first line and synced it with github. I then found the URL of that text file (make sure you select the raw view) and that is what I put into the VersionChecker class above. Note also that "MagicBeans" in my code above is just name of my mod's main class, you should use your own. I left the catch statements as TODO, but you can put more explicit error statements. diesieben07, does this meet your scrutiny? Any problems with this?
-
Okay, but if he has similar code on the client side and kicks it off in its own thread is it a valid way to do it? Or do you have another suggestion?