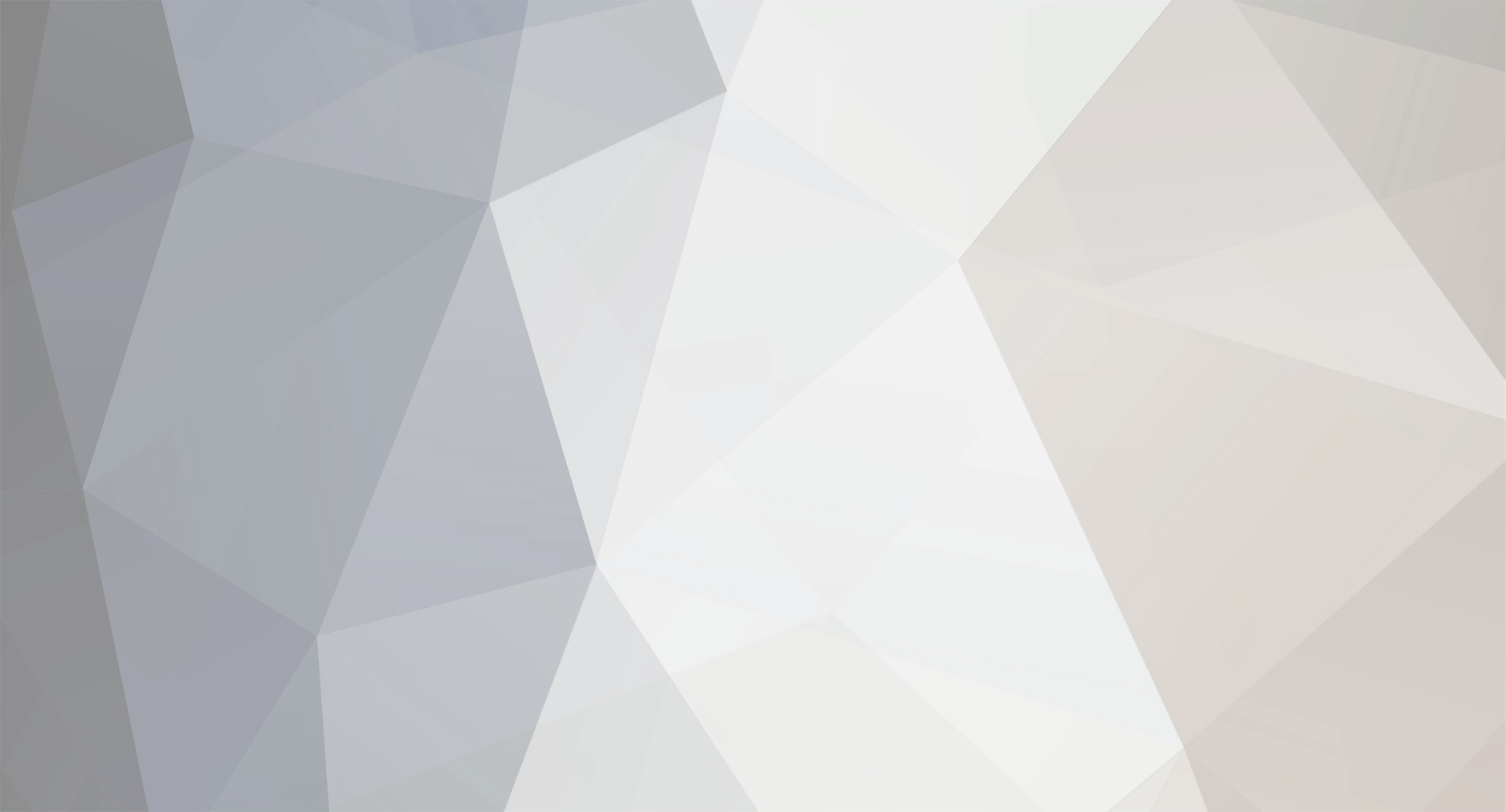
jabelar
Members-
Posts
3266 -
Joined
-
Last visited
-
Days Won
39
Everything posted by jabelar
-
Is there a limit to how big an NBTTagList can be?
jabelar replied to jotato's topic in Modder Support
Is it possible to be a bit "smarter" with your tagging. Like I cannot imagine that you actually have 200,000 fully unique slots -- what the heck are you trying to do anyway? Basically you should consider if there is "compression" algorithm you can come up with. For example, if there is any repetition or regularity in what is in the slots you may not need to send each slot individually. Maybe explain exactly what you're trying to do and we can suggest more efficient ways of storing the data. -
The texture gets associated in the Renderer class, so you'll need to make a custom one that links to a modified PNG asset and then register that as the renderer for the player.
-
Oh, I know you CAN, but I've never seen anyone do that before. I figured it was against convention or something. Anyway, thanks for the help! I think the issue with registering your block (or item or entity, etc) in their own constructor is that you potentially lose control of the order of registration, or at least you have to be more conscious of how the instantiation order will affect registration order. Sometimes you'll need to control the order. For example with ItemSeeds it is important to have the related blocks registered first. You can control it with instantiation, but many people might be tempted to still instantiate in the field declaration area of the main class. Anyway, just something to keep in mind if you go the route of registering classes within their own constructors.
-
See my tutorial on events: http://jabelarminecraft.blogspot.com/p/minecraft-forge-172-event-handling.html Note that a couple events are fired on the "wrong" bus -- meaning not the one you'd think based on the package. I've listed those cases that I'm aware of. One simple trick to tell which bus an event is fired on is to follow the "call hierarchy" for the event. Eclipse will show you where it was used and that will include the bus posts.
-
See my tutorial on events: http://jabelarminecraft.blogspot.com/p/minecraft-forge-172-event-handling.html
-
What event bus did you register this handler class to? These go on the FML bus, not the regular EVENT_BUS...
-
[SOLVED] How are rendering parameters determined?
jabelar replied to coolAlias's topic in Modder Support
Interesting. I've always found look vector trustworthy, but admit I mostly use it for the player not for other entities. I'll have to try that... I'm also wondering about your statement that "I clearly see the caster rotating on the screen". One thing to think about is that the actual head of some entities moves independent of the body. I'm not sure about your entity, but I can see a case where the yaw and look vector were based on the body rotation but not the head. Similarly, if you looked at the rendering / model of the caster itself i *must* have a field that is controlling the rotation of the render so it seems you should be able to hook onto that. Anyway, it seems you have a work around, but like you I hate having something that is not fully understood in my code... -
You might want to look at my tutorial on crops for 1.7.x: http://jabelarminecraft.blogspot.com/p/minecraft-forge-172-creating-custom.html
-
Why use EntityPlayer.openGui() which takes a bunch of "weird" parameters when you can use Minecraft.getMinecraft().displayGuiScreen() instead? Of course you'd only call that on client side. It just takes a GuiScreen as the parameter. It seems that openGui() is still intended for use with containers, as you can call it from server side, and it wants position information passed in, as well as mod instance, and some sort of "modGuiID" integer parameter which it then passes with an FMLMessage ... If you really just want a fully custom (non container) type GUI, seems to me that dipslayGuiScreen() is easier way to go. Just pass the GuiScreen and have that send packets back to server if needed.
-
Well, just write the code to handle it, but it is pretty easy. You just look at each neighbor and if ANY is a power source you can consider your cable powered. Just set the distance to -1 then loop through all neighbors and if you find a power source set distance to 1. Then, whether you encounter one or many power sources, you'll still get same answer, and you won't clear it again if you don't find a power source (if you get what I mean).
-
I'm just saying that doing any type of scanning like the way you're coding will drive you crazy if you want to be able to handle any type of configuration. Imagine a big loop of cable, or a spiral of cable, or cables that cross near each other but are connected to different sources -- those will not be easy to handle the way you're going. Definitely check out my way of doing it -- no big loops needed (except simple one to scan the directly neighboring blocks).
-
Getting different items from different stages, [Crops]
jabelar replied to Kander16's topic in Modder Support
Okay, here's the thing, this is your own custom block so you can code anything you want in Java. But it is also a Minecraft mod so you need to respond to those method calls that Minecraft Forge makes to your blocks. As diesieben07 says, the real entry to your block code is the getDrops() method. The other methods are used within your block and you can use those as well (like getItemDropped()), but not necessarily needed. In getDrops() you can do whatever you want -- it is your own Java code after all. You could drop something, or spawn another entity, or add an achievement or whatever. So the only thing you have to do is change the returned value based on the growth stage. You already can tell the stage of the crop based on the metadata, and the nice thing about the getDrops() method is that the metadata is passed as one of the parameters. So just do some conditional statements (like if statements) to check the metadata and return the type of item you want to drop. Make sense? In the end, the point is that you can code anything you want, but you need to put it in the getDrops() method because that is what will get called when Minecraft needs the drops. After that it is just Java, the possibilities are endless. -
Did you read through my suggestion on how to do the scanning? It is really simple as each cable block can figure out for itself whether it is connected and how long the shortest route is to power source, all without any complicated path finding! Basically each block should only have to scan its neighbors. Just like real energy, electricity, water, whatever, it will quickly within a few ticks adjust to any added block. You shouldn't need any loops (because technically Minecraft is already looping through all the block update code). Suggestions like Hugo's don't work well for complicated paths. Imagine if you made a spiral of cable -- the distance along the cable might be very long even if the power source and final piece of cable are very close to each other. Read my suggestion above again. I guarantee it is the simplest way to code power distribution networks.
-
I believe the easiest way to do this is that each cable block should have int field distanceFromPower that indicates how "close" (in terms of cable length) it is from a power source. Then each tick each block will check all surrounding neighbor blocks for other cable blocks and update its distanceToPower to be one more than the smallest non-zero distanceToPower field of its neighbors. Then it checks for any neighboring power source block and if there is then set distanceToPower to 1. If there is no powered cable or power source neighboring set the distanceToPower to be -1 (represents) unpowered. If you think through the above logic, within a few ticks of adding a cable or power source the whole power network will be up to date. You can configure the cables in any pattern, a mesh, 3d, a spiral, and it will work without needing complicated pathfinding or nested loops. Hope you understand what I mean. The trick then is only in the int field. You could use metadata but then you probably just need to make it a single bit to indicate "powered". You could also use TileEntity. I'd probably do that unless you planned to have a lot. Lastly you can just make a public int 3d array in your main class to store it. Note it is not important to save this data as it will recalculate next time the game runs. But if you want to animate you need to sync client and server.
-
Check out TheGreyGhost's tutorial: http://greyminecraftcoder.blogspot.com/2013/07/block-rendering.html
-
I don't think you can use the tempt AI because I don't think spiders use the new AI. You can check by printing out the returned value from the isAiEnabled() method. Basically, some entities use the AI task list and others use the onUpdate() method to control them. I think spiders use the latter. In the case of an entity that uses onUpdate, you may be able to control it using the LivingUpdateEvent. This is a cancelable event (meaning you can cancel the vanilla behavior and replace with your own). So in that event you'd check if the event.entity was instanceof EntitySpider and if that is true then you'd check if the player is holding the item (that might be a bit tricky though as no player is returned in that event, so you'd want to look for EntityPlayer within a certain distance) and if so then you'd want to cancel the vanilla behavior and replace with your own. The only issue is that you should copy a lot of the onUpdate() code to ensure that the behavior is still somewhat normal, and you'd want to implement proper pathfinding and such so that it moved properly.
-
prevPosXYZ and lastTickPosXYZ don't work for players
jabelar replied to Bedrock_Miner's topic in Modder Support
I've noticed some such inconsistency before. You may just have to create your own field to track it. Basically you can just use PlayerTickEvent and record value from last tick. Since it sounds like you just want to use these for instantaneous calculations of whether player has passed through some location, you shouldn't have to save the values as an actual extended property, although you could do that if you felt it was somehow necessary. -
[1.7.10] Proper way to modify blocks when populating chunks
jabelar replied to TheAwesomeGem's topic in Modder Support
I have a tutorial (really just records a method developed by diesieben07) here: http://jabelarminecraft.blogspot.com/p/minecraft-forge-172-modding-quick-tips.html The tutorial has a collection of tips related to blocks, look at the section down the page called: Replace Generated Blocks With Different Block -
Is there a forge event that triggers when all mods have loaded?
jabelar replied to UntouchedWagons's topic in Modder Support
That is actually my first suggestion, but Sequiteri didn't like the fact you couldn't make it generic (i.e. if you truly wanted all mods loaded, including ones you didn't know you needed to depend on). The OP already established they were interested on the client side which is why I considered it a suitable suggestion. I also don't think this is an "ugly hack". It is really a nice hook, just like any other forge hook: basically there is a method call at exactly the right point that is meant for you to have full control over. It seems pretty elegant to me because it is being used for exactly the same purpose -- the gui factory wants to know when all mods are loaded, just like we're trying to solve for. Anyway, this is obviously academic because what the OP wants to achieve doesn't seem to really need such specific "all mods loaded" solution. -
Is there a forge event that triggers when all mods have loaded?
jabelar replied to UntouchedWagons's topic in Modder Support
Actually, I think I just found a way to detect when all the mods are loaded! Right at the point when they are all finished. The new gui factory stuff for enabling the config guis calls the initialize() method for your guifactory right after all the mods are loaded in the finishMinecraftLoading() method in the FMLClientHandler class. So if you register a gui factory, whatever you do in the initialize() method will be performed right at the moment when all mods are finished loading! Ta da! -
Is there a forge event that triggers when all mods have loaded?
jabelar replied to UntouchedWagons's topic in Modder Support
I find that most of the time, people don't ask the question in the way that they need, otherwise they probably would have figured out the solution themselves! It's like all the forum posts that say "how do you edit base classes" when they mean they just want to change the drops for an entity, etc. If a person knew to ask: what event do I need to handle to change drops of a living entity, they probably would have found the LivingDropsEvent themselves... So I usually supply people with information "around" the question they asked. But back to your question you're certainly right that you can never be sure that other mods have honored the pre-init, init, and post-init in a way that you can trust. I was looking at this further, and it seems that you can actually poll the FMLClientLoader.isLoading() function. It returns a boolean called loading which goes to false at the very end of the post-init and in fact happens right around the message in the console that says "Forge Mod Loader has successfully loaded x mods". -
Is there a forge event that triggers when all mods have loaded?
jabelar replied to UntouchedWagons's topic in Modder Support
That's an interesting question. There is a server stopping event and a server stopped event, but I'm not sure what that does when you're a client only. You might still want to try it. I guess it depends on what you specifically want to do. I mean the client can be stopped in a number of ways, including just hitting the window close to force it to shut down. For example, if you just want to know when a player returns to the main menu, I think you can do that by handling the gui open event and checking for the main menu, or something like that. What exactly do you mean by "game itself is shutting down"? -
I am not totally sure but I'm suspicious of the world.getHeightValue() function. I think that once you build, it will return a different value. so I think that in each of your generates you shouldn't be adding so much to j. Like just use j+1 in each generate and see what that does. To really trace this, after each j=world.getHeightValue() you can print a console statement like System.out.println("j = "+j); and see what it says.
-
[1.7.10]Forge 1217 console messages too verbose?
jabelar replied to jabelar's topic in Modder Support
Thanks, I thought it was weird. -
I think what you would do is create a public field (or maybe multiple fields or an array depending on how many entities and textures can be affected) in your mod's main class. for example you can have a ResourceLocation field for your texturePigCurrent and textureCowCurrent, etc. Then in your item class you just update this field. And in the render class you would just reference that field. so the item doesn't directly do the rendering, but basically updates a public mod field to point to new resource location which is then used by the render class. Get the idea?