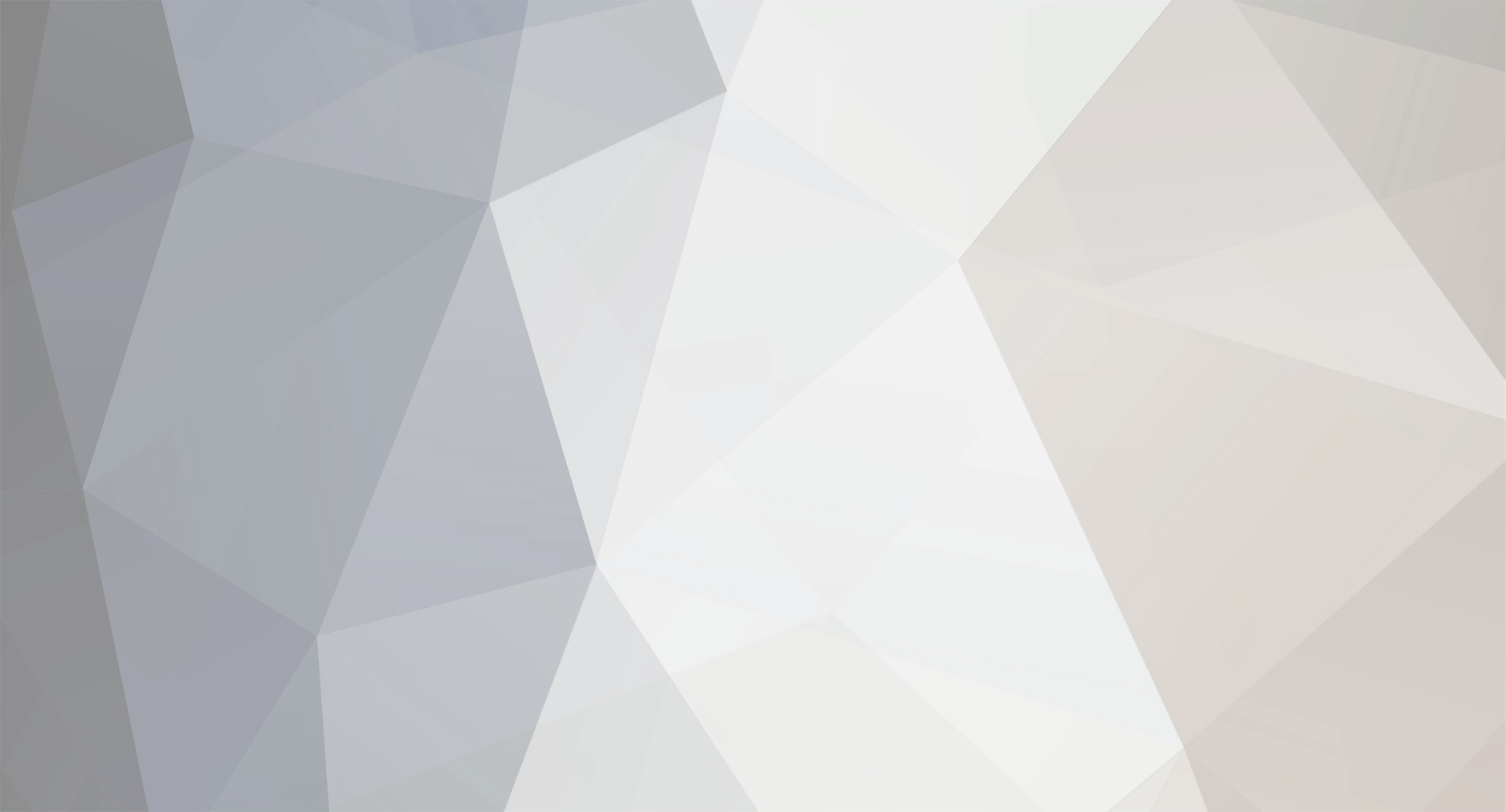
SureenInk
Members-
Posts
118 -
Joined
-
Last visited
Everything posted by SureenInk
-
So...I'm not sure what to put for coding here...so I'll put what I think will help and if you guys can think of something, let me know. In either case, I'm having a problem where I've created an item that can be thrown. However, when I throw the item, it isn't rendering it. In my ClientProxy, I registered the following: RenderingRegistry.registerEntityRenderingHandler(EntityPopMMOIngot.class, new RenderYoutuberBall(YoutubersItems.PopMMOIngot)); From what I've seen, that should be all the coding I need...but it's not doing anything. Here's the coding for the Ingot: PopMMOIngot = new SureenItem(false, 3).setUnlocalizedName("popmmosingot").setCreativeTab(YoutubersMain.FuriousDestroyerTab).setTextureName("youtubers:popmmos_ingot"); And here's SureenItem: package sureen.core.items; import cpw.mods.fml.relauncher.Side; import cpw.mods.fml.relauncher.SideOnly; import net.minecraft.item.EnumRarity; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; public class SureenItem extends Item { public boolean field_effect; public int rarity; public SureenItem(boolean par1, int par2) { this.field_effect = par1; this.rarity = par2; } @SideOnly(Side.CLIENT) public boolean hasEffect(ItemStack par1ItemStack) { return field_effect; } public EnumRarity getRarity(ItemStack par1ItemStack) { if (rarity == 0) { return EnumRarity.common; } if (rarity == 1) { return EnumRarity.uncommon; } if (rarity == 2) { return EnumRarity.rare; } else return EnumRarity.epic; } } And here's the item creating the attack. It works 100% and summons the entity and everything...but it doesn't render it: package youtubers.items; import java.util.List; import net.minecraft.client.renderer.texture.IIconRegister; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.item.EnumRarity; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.item.ItemSword; import net.minecraft.world.World; import youtubers.entity.EntityPopMMOIngot; import youtubers.registry.YoutubersItems; public class YoutuberSword extends ItemSword { public int rarity; public YoutuberSword(ToolMaterial material, int par1) { super(material); this.rarity = par1; } public EnumRarity getRarity(ItemStack par1ItemStack) { if (rarity == 0) { return EnumRarity.common; } if (rarity == 1) { return EnumRarity.uncommon; } if (rarity == 2) { return EnumRarity.rare; } else return EnumRarity.epic; } public boolean hasEffect(ItemStack itemstack) { return false; } public ItemStack onItemRightClick(ItemStack ItemStack, World par2World, EntityPlayer par3EntityPlayer) { if (ItemStack.getItem() == YoutubersItems.PopMMOSword) { par2World.playSoundAtEntity(par3EntityPlayer, "random.bow", 0.5F, 0.4F / (Item.itemRand.nextFloat() * 0.4F + 0.8F)); if (!par2World.isRemote) { par2World.spawnEntityInWorld(new EntityPopMMOIngot(par2World, par3EntityPlayer)); ItemStack.damageItem(3, par3EntityPlayer); } } return ItemStack; } public void addInformation(ItemStack item, EntityPlayer player, List list, boolean par4) { if (item.getItem() == YoutubersItems.PopMMOSword) { list.add("Right click for an Explosion of Epic Proportions!"); } } } And lastly, the Entity coding: package youtubers.entity; import net.minecraft.entity.Entity; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.entity.projectile.EntityThrowable; import net.minecraft.util.DamageSource; import net.minecraft.util.MovingObjectPosition; import net.minecraft.world.World; public class EntityPopMMOIngot extends EntityThrowable { private int field_92057_e = 2; public EntityPopMMOIngot(World par1World) { super(par1World); } public EntityPopMMOIngot(World par1World, EntityPlayer par3EntityPlayer) { super(par1World, par3EntityPlayer); } public EntityPopMMOIngot(World par1World, double par2, double par4, double par6) { super(par1World, par2, par4, par6); } private void explode() { this.worldObj.newExplosion((Entity)null, this.posX, this.posY, this.posZ, this.field_92057_e, true, this.worldObj.getGameRules().getGameRuleBooleanValue("mobGriefing")); } protected void onImpact(MovingObjectPosition par1MovingObjectPosition) { if (par1MovingObjectPosition.entityHit != null) { byte var2 = 0; par1MovingObjectPosition.entityHit.attackEntityFrom(DamageSource.causeThrownDamage(this, getThrower()), 13.0F); } for (int var3 = 0; var3 < 8; var3++) { this.worldObj.spawnParticle("Snowballpoof", this.posX, this.posY, this.posZ, 0.0D, 0.0D, 0.0D); } if (!this.worldObj.isRemote) { explode(); setDead(); } } } That's honestly all the coding I can think of to copy in...Can anyone help with this?
-
Having an entity check for a Blocks Metadata
SureenInk replied to SureenInk's topic in Modder Support
Uhh...hmm...I'm confused still (sorry!) How do I use that in the ai coding? Also...I can't do an instance of BlockDoublePlant for an item...so how would I do that? -
Having an entity check for a Blocks Metadata
SureenInk replied to SureenInk's topic in Modder Support
Yeah, I'm checking to see if the player has the itemblock in their hand. How do I go about checking for it's damage, then? -
So, I'm a little confused...I need to be able to check for a block's metadata, but I'm unsure how to do it. In my coding, I have these 2 lines of code: this.tasks.addTask(2, new EntityAITempt(this, 1.0D, Item.getItemFromBlock(Blocks.double_plant), false)); if ((helditem != null) && (helditem.getItem() == Item.getItemFromBlock(Blocks.double_plant))) I want to be able to check for the lilac, which as far as I can tell is metadata 1, but both of these are only allowing me to check for Item, which doesn't have the parameters to do metadata. I've tried using ItemStack instead, but that doesn't work because I have to search for Item, not ItemStack...could anyone help?
-
I was hoping someone would show up to give you a better system then mine...because mine is horrible to code, but since it's been a day and no one has, I'll give you mine. But please, someone who has a better system, please help this guy out! Mine only lets me do minor default things, and I know there's a way to code more advanced things! Anyway... TileEntityMobSpawner ttsnimspawner = (TileEntityMobSpawner)world.getTileEntity(i + 6, j + 11, k + 6); if (ttsnimspawner == null) { System.err.printf("TTSnimSpawner is null!?!\n"); } else { ttsnimspawner.func_145881_a().setEntityName("Skeleton"); } The main thing you'll want is to use that if statement, cause if, for some reason, the spawner isn't at the location you specified (maybe terrain grew over it or something) then it will bug out and crash the mod. The if statement prevents that from happening. Unfortunately, this only works for default standard mob spawners... I'd love to know how to code something like...baby zombie spawners with armor or something like that, though (seriously, I know there's a way!)
-
-facehoof- ugh...it only posted half of my post...sorry about that. I edited it.
-
I'm a little confused...so I would need to run my world generation using EVENT_BUS instead if I added that into it? I can see your generation there is doing both the PopulateChunkEvent and the world generation, so that's why it confused me. Cause right now my world generator is just: GameRegistry.registerWorldGenerator(new CraftyGirlsWorldGeneration(), 1); Would that mean I have to make it this instead? MinecraftForge.EVENT_BUS.register(new CraftyGirlsWorldGeneration());
-
Okay, so, I have my coding for my treasure chests, and I can get anything I want into it, but I can't figure out enchanted books. I can add books with enchants on them, but I can't get Enchanted Books (that you can put into an anvil and put the enchantment on your item) into them. TileEntityChest chest20 = (TileEntityChest) world.getTileEntity(i + 46, j + 19, k + 13); if (chest20 == null) { System.err.printf("TileEntityChest20 is null!?!\n"); } else { for (int slot = 0; slot < chest20.getSizeInventory(); slot++) { int slotran = random1.nextInt(30); int itemran = random2.nextInt(3); if (slotran == 20){ ItemStack stack = new ItemStack(Items.enchanted_book); stack.addEnchantment(Enchantment.featherFalling, itemran+1); //this just adds a book with an enchant, not an enchanted book. chest21.setInventorySlotContents(slot, stack); } if (slotran == 25){ ItemStack stack = new ItemStack(Items.enchanted_book, 1, 0); WeightedRandomChestContent tmp = new WeightedRandomChestContent(stack, 1, 1, 1); //This adds an "Enchanted Book" that has no enchants. chest21.setInventorySlotContents(slot, stack); } } }
-
Yeap...turns out a jungle biome spawned inside of my structure...so a leaf block replaced the spawner...is there any way to prevent that from happening? I mean, it works now and stuff, but I'd like my dungeons to not be destroyed by the biomes around them.
-
Wow! Thanks a lot! This helps a ton! EDIT: New problem...I have this coding for my mob spawners TileEntityMobSpawner ttsnimspawner = (TileEntityMobSpawner)world.getTileEntity(i + 6, j + 11, k + 6); ttsnimspawner.func_145881_a().setEntityName("Skeleton"); When a second structure spawns in, it seems to error on this code. It works fine when there's one structure, but I flew to a second structure and it errored and crashed. I don't understand why... Also, how would I go about adding loot to the chests I have in my dungeon?
-
[1.7.2] Using the Ore Dictionary in Crafting Recipes?
SureenInk replied to saxon564's topic in Modder Support
You could try making each of the individual crafting recipes. Like, you could tell it you want a shaped recipe this is like: XY CB Then make another one that is: YB XC And so on. I'm not sure that you can use the Ore Dictionary for it, because that basically lets you make an item the same as an item from any other mod (so like, a copper ingot from your mod could be used in Metallurgy). On the other hand, I'm still a noob myself and haven't used the Ore Dictionary at all myself, so I don't know. -
Is this beyond what someone is willing to help with? Cause I really can't find any information on doing this...Again, I'd like to do a sort of "Twilight Forest" thing where the structures spawn in specific locations. Like, maybe every 300 blocks or so (so on like 0,0, then 0,300, and 0,-300, and 300,0, etc.)
-
Unfortunately, that's what I tried to do. The random number generates a random number between 0.0 and 1.0. It's set to 0.9 (10% of the time, it's about as low as I can set it). It runs about 32 times per chunk, though...it's almost guaranteed to be set at least once. As for checking for coordinates and such, I don't have the slightest idea how to do that. What I would like to do is make it spawn every 300 blocks (like - 0, 300, 600, 900) then run some random code to then tell it "Okay, at these coordinates run a random number to determine which structure to generate there". I have no idea how to do that, though. I've tried some things, but nothing has worked, so I'm not sure what to do.
-
-nods- okay. That...doesn't really help my structure problem... (and yeah, I figured out the hardened clay thing)
-
O...kay...I'm not sure where to even begin with checking for that...Sounds like a great idea, though. Could you link me to a tutorial or explain how to do so? I've tried looking for one, but can't find any.
-
I didn't, do I need to? The parent of EntityNeola is EntityAnimal. The parent of NeolaTameable is EntityTameable.
-
Problem 1 [solved] Problem 2: New problem...I have this coding for my mob spawners TileEntityMobSpawner ttsnimspawner = (TileEntityMobSpawner)world.getTileEntity(i + 6, j + 11, k + 6); ttsnimspawner.func_145881_a().setEntityName("Skeleton"); When a second structure spawns in, it seems to error on this code. It works fine when there's one structure, but I flew to a second structure and it errored and crashed. I don't understand why... Also, how would I go about adding loot to the chests I have in my dungeon?
-
Suddenly missing textures in the newest forge?
SureenInk replied to SureenInk's topic in Modder Support
Actually...that was a typo myself...the problem was...I didn't put them in the "assets" folder x.x I figured it out, btw. I just didn't bother saying so cause the topic was almost at the page of the page, and I didn't want to bump it just to say it was fixed. -
Well, I can't seem to spawn in tameable entities via the regular code because it's hard coded in. Someone said the only way to do that is to learn ASM and I can't find anything on that (nor do I even know what it means...which makes it hard to look for). So, I found a tutorial on how to spawn in entities the game won't normally let you spawn in. What this does is make it so that whenever an entity that I want to be tameable is spawned it, it spawns in the tameable version on top of the non-tameable version, then removes the non-tameable version.
-
So, I looked up a tutorial, and it said that to replace an entity with another one on spawn, I had to run an event handler. I programmed my event handler the way he did, and it didn't work. I then saw someone else's code and it worked for them but was different, so I tried that code. So far, neither works, and the second causes errors while the first just outright doesn't work. Can anyone help me figure out why? Original Code: package craftygirls.core.events; import cpw.mods.fml.common.eventhandler.SubscribeEvent; import cpw.mods.fml.relauncher.Side; import cpw.mods.fml.relauncher.SideOnly; import craftygirls.core.entity.EntityNeola; import craftygirls.core.entity.NeolaTameable; import net.minecraftforge.event.entity.living.LivingSpawnEvent; public class CraftyEventHandling { @SubscribeEvent public void onEntityLivingSpawn(LivingSpawnEvent event) { if (!event.world.isRemote) { if (event.entityLiving instanceof EntityNeola) { EntityNeola oldNeola = (EntityNeola)event.entityLiving; if (oldNeola.getCanSpawnHere()) { NeolaTameable newNeola = new NeolaTameable(event.world); newNeola.setLocationAndAngles(oldNeola.posX, oldNeola.posY, oldNeola.posZ, oldNeola.rotationYaw, oldNeola.rotationPitch); event.world.spawnEntityInWorld(newNeola); oldNeola.setDead(); } } } } } Second Code (that errors): package craftygirls.core.events; import cpw.mods.fml.common.eventhandler.SubscribeEvent; import cpw.mods.fml.relauncher.Side; import cpw.mods.fml.relauncher.SideOnly; import craftygirls.core.entity.EntityNeola; import craftygirls.core.entity.NeolaTameable; import net.minecraft.world.World; import net.minecraftforge.event.entity.living.LivingSpawnEvent; public class CraftyEventHandling { @SubscribeEvent public void onEntityLivingSpawn(LivingSpawnEvent event) { if (!event.entity.worldObj.isRemote && event.entityLiving instanceof EntityNeola) { EntityNeola oldNeola = (EntityNeola)event.entity; World world = oldNeola.worldObj; NeolaTameable newNeola = new NeolaTameable(world); newNeola.setPosition(event.entity.posX, event.entity.posY, event.entity.posZ); event.world.spawnEntityInWorld(newNeola); oldNeola.setDead(); } } } Error with code: [01:17:34] [Client thread/FATAL]: Unreported exception thrown! java.lang.NullPointerException at net.minecraft.client.network.NetHandlerPlayClient.handleSpawnMob(NetHandlerPlayClient.java:853) ~[NetHandlerPlayClient.class:?] at net.minecraft.network.play.server.S0FPacketSpawnMob.processPacket(S0FPacketSpawnMob.java:129) ~[s0FPacketSpawnMob.class:?] at net.minecraft.network.play.server.S0FPacketSpawnMob.processPacket(S0FPacketSpawnMob.java:222) ~[s0FPacketSpawnMob.class:?] at net.minecraft.network.NetworkManager.processReceivedPackets(NetworkManager.java:232) ~[NetworkManager.class:?] at net.minecraft.client.multiplayer.PlayerControllerMP.updateController(PlayerControllerMP.java:321) ~[PlayerControllerMP.class:?] at net.minecraft.client.Minecraft.runTick(Minecraft.java:1647) ~[Minecraft.class:?] at net.minecraft.client.Minecraft.runGameLoop(Minecraft.java:994) ~[Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:910) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:112) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.7.0_51] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.7.0_51] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.7.0_51] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.7.0_51] at net.minecraft.launchwrapper.Launch.launch(Launch.java:134) [launchwrapper-1.9.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.9.jar:?] ---- Minecraft Crash Report ---- // I'm sorry, Dave. Time: 4/24/14 1:17 AM Description: Unexpected error java.lang.NullPointerException: Unexpected error at net.minecraft.client.network.NetHandlerPlayClient.handleSpawnMob(NetHandlerPlayClient.java:853) at net.minecraft.network.play.server.S0FPacketSpawnMob.processPacket(S0FPacketSpawnMob.java:129) at net.minecraft.network.play.server.S0FPacketSpawnMob.processPacket(S0FPacketSpawnMob.java:222) at net.minecraft.network.NetworkManager.processReceivedPackets(NetworkManager.java:232) at net.minecraft.client.multiplayer.PlayerControllerMP.updateController(PlayerControllerMP.java:321) at net.minecraft.client.Minecraft.runTick(Minecraft.java:1647) at net.minecraft.client.Minecraft.runGameLoop(Minecraft.java:994) at net.minecraft.client.Minecraft.run(Minecraft.java:910) at net.minecraft.client.main.Main.main(Main.java:112) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at net.minecraft.launchwrapper.Launch.launch(Launch.java:134) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) A detailed walkthrough of the error, its code path and all known details is as follows: --------------------------------------------------------------------------------------- -- Head -- Stacktrace: at net.minecraft.client.network.NetHandlerPlayClient.handleSpawnMob(NetHandlerPlayClient.java:853) at net.minecraft.network.play.server.S0FPacketSpawnMob.processPacket(S0FPacketSpawnMob.java:129) at net.minecraft.network.play.server.S0FPacketSpawnMob.processPacket(S0FPacketSpawnMob.java:222) at net.minecraft.network.NetworkManager.processReceivedPackets(NetworkManager.java:232) at net.minecraft.client.multiplayer.PlayerControllerMP.updateController(PlayerControllerMP.java:321) -- Affected level -- Details: Level name: MpServer All players: 1 total; [EntityClientPlayerMP['Player763'/170, l='MpServer', x=-176.53, y=87.23, z=362.89]] Chunk stats: MultiplayerChunkCache: 314, 314 Level seed: 0 Level generator: ID 00 - default, ver 1. Features enabled: false Level generator options: Level spawn location: World: (8,64,256), Chunk: (at 8,4,0 in 0,16; contains blocks 0,0,256 to 15,255,271), Region: (0,0; contains chunks 0,0 to 31,31, blocks 0,0,0 to 511,255,511) Level time: 6049 game time, 6049 day time Level dimension: 0 Level storage version: 0x00000 - Unknown? Level weather: Rain time: 0 (now: false), thunder time: 0 (now: false) Level game mode: Game mode: creative (ID 1). Hardcore: false. Cheats: false Forced entities: 75 total; [EntityChicken['Chicken'/0, l='MpServer', x=-191.50, y=67.00, z=384.50], EntityChicken['Chicken'/1, l='MpServer', x=-190.47, y=81.00, z=409.44], EntityChicken['Chicken'/2, l='MpServer', x=-185.61, y=78.00, z=398.56], EntityChicken['Chicken'/3, l='MpServer', x=-185.19, y=76.00, z=422.56], EntityChicken['Chicken'/4, l='MpServer', x=-186.59, y=76.00, z=422.59], EntitySkeleton['Skeleton'/5, l='MpServer', x=-180.50, y=62.00, z=443.50], EntitySkeleton['Skeleton'/6, l='MpServer', x=-187.69, y=60.00, z=443.50], EntityBat['Bat'/1368, l='MpServer', x=-240.63, y=35.46, z=398.08], EntityZombie['Zombie'/7, l='MpServer', x=-181.50, y=62.00, z=445.50], EntityBat['Bat'/1364, l='MpServer', x=-253.63, y=35.10, z=402.75], EntitySkeleton['Skeleton'/14, l='MpServer', x=-164.47, y=64.00, z=373.16], EntitySkeleton['Skeleton'/15, l='MpServer', x=-160.50, y=63.00, z=374.50], EntityCreeper['Creeper'/21, l='MpServer', x=-149.50, y=58.00, z=431.50], EntityZombie['Zombie'/20, l='MpServer', x=-151.84, y=64.00, z=374.38], EntitySpider['Spider'/23, l='MpServer', x=-140.22, y=24.00, z=427.09], EntityChicken['Chicken'/22, l='MpServer', x=-159.47, y=70.00, z=422.53], EntitySkeleton['Skeleton'/25, l='MpServer', x=-138.50, y=24.00, z=432.50], EntityChicken['Chicken'/24, l='MpServer', x=-123.63, y=71.00, z=432.47], EntityChicken['Chicken'/27, l='MpServer', x=-141.58, y=78.00, z=440.06], EntitySpider['Spider'/26, l='MpServer', x=-136.50, y=24.00, z=436.50], EntitySpider['Spider'/29, l='MpServer', x=-117.50, y=42.00, z=384.50], EntityChicken['Chicken'/28, l='MpServer', x=-129.53, y=71.00, z=437.47], EntitySkeleton['Skeleton'/30, l='MpServer', x=-125.94, y=13.00, z=410.47], EntityCreeper['Creeper'/1609, l='MpServer', x=-253.50, y=30.00, z=423.50], EntityCreeper['Creeper'/32, l='MpServer', x=-115.69, y=19.00, z=411.34], EntityCreeper['Creeper'/33, l='MpServer', x=-124.41, y=14.00, z=411.00], EntityZombie['Zombie'/38, l='MpServer', x=-106.34, y=33.00, z=335.41], EntityCreeper['Creeper'/39, l='MpServer', x=-124.66, y=29.00, z=341.95], EntitySheep['Sheep'/37, l='MpServer', x=-102.88, y=67.00, z=312.28], EntityBat['Bat'/784, l='MpServer', x=-113.66, y=32.10, z=350.75], EntitySkeleton['Skeleton'/42, l='MpServer', x=-103.50, y=35.00, z=364.50], EntitySkeleton['Skeleton'/43, l='MpServer', x=-107.50, y=35.00, z=364.50], EntitySheep['Sheep'/41, l='MpServer', x=-101.34, y=66.00, z=322.59], EntityZombie['Zombie'/46, l='MpServer', x=-109.50, y=36.00, z=388.50], EntityZombie['Zombie'/47, l='MpServer', x=-105.50, y=32.00, z=384.50], EntityZombie['Zombie'/44, l='MpServer', x=-106.50, y=32.00, z=383.50], EntityChicken['Chicken'/50, l='MpServer', x=-105.56, y=86.00, z=428.66], EntityChicken['Chicken'/49, l='MpServer', x=-107.47, y=86.00, z=431.47], EntityBat['Bat'/773, l='MpServer', x=-245.53, y=46.00, z=426.75], EntityZombie['Zombie'/48, l='MpServer', x=-107.50, y=32.00, z=385.50], EntityZombie['Zombie'/1853, l='MpServer', x=-118.50, y=21.00, z=312.50], EntityCreeper['Creeper'/1855, l='MpServer', x=-115.53, y=24.00, z=320.34], EntityBat['Bat'/883, l='MpServer', x=-117.00, y=24.00, z=333.55], EntityZombie['Zombie'/1854, l='MpServer', x=-120.50, y=21.00, z=310.50], EntitySkeleton['Skeleton'/1029, l='MpServer', x=-137.97, y=12.00, z=414.59], EntityBat['Bat'/871, l='MpServer', x=-245.46, y=32.00, z=435.40], EntityBat['Bat'/872, l='MpServer', x=-244.02, y=35.00, z=444.80], EntityCreeper['Creeper'/1591, l='MpServer', x=-162.53, y=69.28, z=337.91], EntityZombie['Zombie'/851, l='MpServer', x=-114.50, y=42.00, z=385.50], EntityBat['Bat'/2303, l='MpServer', x=-247.15, y=16.84, z=303.65], EntityZombie['Zombie'/850, l='MpServer', x=-114.50, y=42.00, z=387.50], EntityBat['Bat'/2300, l='MpServer', x=-225.60, y=23.51, z=309.68], EntityZombie['Zombie'/849, l='MpServer', x=-119.50, y=42.00, z=384.50], EntityZombie['Zombie'/852, l='MpServer', x=-116.50, y=42.00, z=382.50], EntitySpider['Spider'/2281, l='MpServer', x=-157.87, y=63.00, z=380.07], EntityZombie['Zombie'/2032, l='MpServer', x=-102.50, y=19.00, z=417.50], EntityCreeper['Creeper'/2034, l='MpServer', x=-104.25, y=18.00, z=413.50], EntityBat['Bat'/2305, l='MpServer', x=-235.63, y=18.65, z=291.13], EntityChicken['Chicken'/186, l='MpServer', x=-223.84, y=77.00, z=412.41], EntityChicken['Chicken'/187, l='MpServer', x=-223.53, y=76.00, z=407.97], EntityChicken['Chicken'/184, l='MpServer', x=-222.50, y=80.00, z=378.50], EntityChicken['Chicken'/185, l='MpServer', x=-222.50, y=80.00, z=378.50], EntityChicken['Chicken'/190, l='MpServer', x=-202.44, y=62.52, z=376.56], EntityChicken['Chicken'/191, l='MpServer', x=-192.50, y=66.00, z=383.50], EntityChicken['Chicken'/188, l='MpServer', x=-222.50, y=81.00, z=412.50], EntityChicken['Chicken'/189, l='MpServer', x=-222.50, y=81.00, z=412.50], EntityChicken['Chicken'/178, l='MpServer', x=-225.91, y=81.00, z=375.56], EntityCreeper['Creeper'/2365, l='MpServer', x=-247.50, y=44.00, z=422.50], EntityChicken['Chicken'/183, l='MpServer', x=-220.50, y=80.00, z=378.50], EntityCreeper['Creeper'/2366, l='MpServer', x=-243.50, y=44.00, z=424.50], EntitySkeleton['Skeleton'/1433, l='MpServer', x=-125.31, y=29.00, z=343.60], EntitySkeleton['Skeleton'/193, l='MpServer', x=-192.50, y=39.00, z=425.50], EntityChicken['Chicken'/192, l='MpServer', x=-193.50, y=70.00, z=382.50], EntityClientPlayerMP['Player763'/170, l='MpServer', x=-176.53, y=87.23, z=362.89], EntityBat['Bat'/1456, l='MpServer', x=-243.75, y=26.98, z=410.41]] Retry entities: 0 total; [] Server brand: fml,forge Server type: Integrated singleplayer server Stacktrace: at net.minecraft.client.multiplayer.WorldClient.addWorldInfoToCrashReport(WorldClient.java:412) at net.minecraft.client.Minecraft.addGraphicsAndWorldToCrashReport(Minecraft.java:2521) at net.minecraft.client.Minecraft.run(Minecraft.java:939) at net.minecraft.client.main.Main.main(Main.java:112) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at net.minecraft.launchwrapper.Launch.launch(Launch.java:134) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) -- System Details -- Details: Minecraft Version: 1.7.2 Operating System: Windows 7 (amd64) version 6.1 Java Version: 1.7.0_51, Oracle Corporation Java VM Version: Java HotSpot(TM) 64-Bit Server VM (mixed mode), Oracle Corporation Memory: 745461440 bytes (710 MB) / 1037959168 bytes (989 MB) up to 1037959168 bytes (989 MB) JVM Flags: 3 total; -Xincgc -Xmx1024M -Xms1024M AABB Pool Size: 9299 (520744 bytes; 0 MB) allocated, 2 (112 bytes; 0 MB) used IntCache: cache: 0, tcache: 0, allocated: 13, tallocated: 95 FML: MCP v9.01-pre FML v7.2.156.1060 Minecraft Forge 10.12.1.1060 7 mods loaded, 7 mods active mcp{8.09} [Minecraft Coder Pack] (minecraft.jar) Unloaded->Constructed->Pre-initialized->Initialized->Post-initialized->Available->Available->Available->Available FML{7.2.156.1060} [Forge Mod Loader] (forgeSrc-1.7.2-10.12.1.1060.jar) Unloaded->Constructed->Pre-initialized->Initialized->Post-initialized->Available->Available->Available->Available Forge{10.12.1.1060} [Minecraft Forge] (forgeSrc-1.7.2-10.12.1.1060.jar) Unloaded->Constructed->Pre-initialized->Initialized->Post-initialized->Available->Available->Available->Available sureencore{V1.0} [sureen Core] (bin) Unloaded->Constructed->Pre-initialized->Initialized->Post-initialized->Available->Available->Available->Available craftygirls{Alpha v0.6} [The Crafty Girls Core Mod] (bin) Unloaded->Constructed->Pre-initialized->Initialized->Post-initialized->Available->Available->Available->Available gemsmod{Alpha v0.1} [Gems Mod] (bin) Unloaded->Constructed->Pre-initialized->Initialized->Post-initialized->Available->Available->Available->Available youtubers{Alpha v0.1} [Youtubers Mod] (bin) Unloaded->Constructed->Pre-initialized->Initialized->Post-initialized->Available->Available->Available->Available Launched Version: 1.6 LWJGL: 2.9.0 OpenGL: GeForce GT 635/PCIe/SSE2 GL version 4.3.0, NVIDIA Corporation Is Modded: Definitely; Client brand changed to 'fml,forge' Type: Client (map_client.txt) Resource Packs: [] Current Language: English (US) Profiler Position: N/A (disabled) Vec3 Pool Size: 1787 (100072 bytes; 0 MB) allocated, 22 (1232 bytes; 0 MB) used Anisotropic Filtering: Off (1) #@!@# Game crashed! Crash report saved to: #@!@# C:\Users\Sureen Ink\Desktop\Minecraft Mods\forge-1.7.2-1060\eclipse\.\crash-reports\crash-2014-04-24_01.17.34-client.txt AL lib: (EE) alc_cleanup: 1 device not closed
-
So, I've been working on a mod for the last few weeks, and everything has worked fine. I updated to the recommended forge build (1.7.2-1060), but now all of my textures are missing...I have changed none of the coding, nor have I changed the locations of any files. All of my textures are in the "main/resources/gemsblock/textures/blocks folder...and my coding for textures is .setBlockTextureName("gemsmod:amethystblock") I get the following error: [18:08:59] [Client thread/ERROR]: Using missing texture, unable to load gemsmod:textures/blocks/amethystblock.png java.io.FileNotFoundException: gemsmod:textures/blocks/amethystblock.png at net.minecraft.client.resources.SimpleReloadableResourceManager.getResource(SimpleReloadableResourceManager.java:71) ~[simpleReloadableResourceManager.class:?] at net.minecraft.client.renderer.texture.TextureMap.loadTextureAtlas(TextureMap.java:126) [TextureMap.class:?] at net.minecraft.client.renderer.texture.TextureMap.loadTexture(TextureMap.java:91) [TextureMap.class:?] at net.minecraft.client.renderer.texture.TextureManager.loadTexture(TextureManager.java:89) [TextureManager.class:?] at net.minecraft.client.renderer.texture.TextureManager.onResourceManagerReload(TextureManager.java:170) [TextureManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.notifyReloadListeners(SimpleReloadableResourceManager.java:134) [simpleReloadableResourceManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.reloadResources(SimpleReloadableResourceManager.java:118) [simpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.refreshResources(Minecraft.java:624) [Minecraft.class:?] at cpw.mods.fml.client.FMLClientHandler.finishMinecraftLoading(FMLClientHandler.java:283) [FMLClientHandler.class:?] at net.minecraft.client.Minecraft.startGame(Minecraft.java:583) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:890) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:112) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.7.0_51] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.7.0_51] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.7.0_51] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.7.0_51] at net.minecraft.launchwrapper.Launch.launch(Launch.java:134) [launchwrapper-1.9.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.9.jar:?]
-
hmm...I don't see a "renderLivingLabel" method in the Render class. I'm using forge 1024 for Minecraft 1.7. The setCustomNameTag works, but is there a way to keep it up on the character? When I mouse off of the entity, it disappears.
-
So, I've been looking over the Minecraft coding a bit, but I can't figure out how to do this... I want to put a name plate over an entity's head (like the player has). I want to do this because I'm making a mod that involves minecraft players (it's a youtubers mod) and I want to put their names over their heads as if they were actual characters. How would I go about doing that? I can't seem to find that coding in Minecraft (unless it's the NBTTagCompound in EntityPlayer, in which case, I don't understand it...)
-
Huh...I will have to give that a try I hadn't thought of doing anything like that. That could be awesome.
-
Nice. So I just put that under each spawner? Is there a way to give mobs armor using this? The original idea was for each floor to have mobs with the next tier armor and enchant (like, the bottom is leather with Protection 1, floor 2 is iron with Protection 2, etc.) Also, is there a way to spawn baby versions of the entities? The person this tower is based off of has a hate for Baby Zombies as opposed to normal Zombies, so I wanted it to spawn a bunch of Baby Zombies.