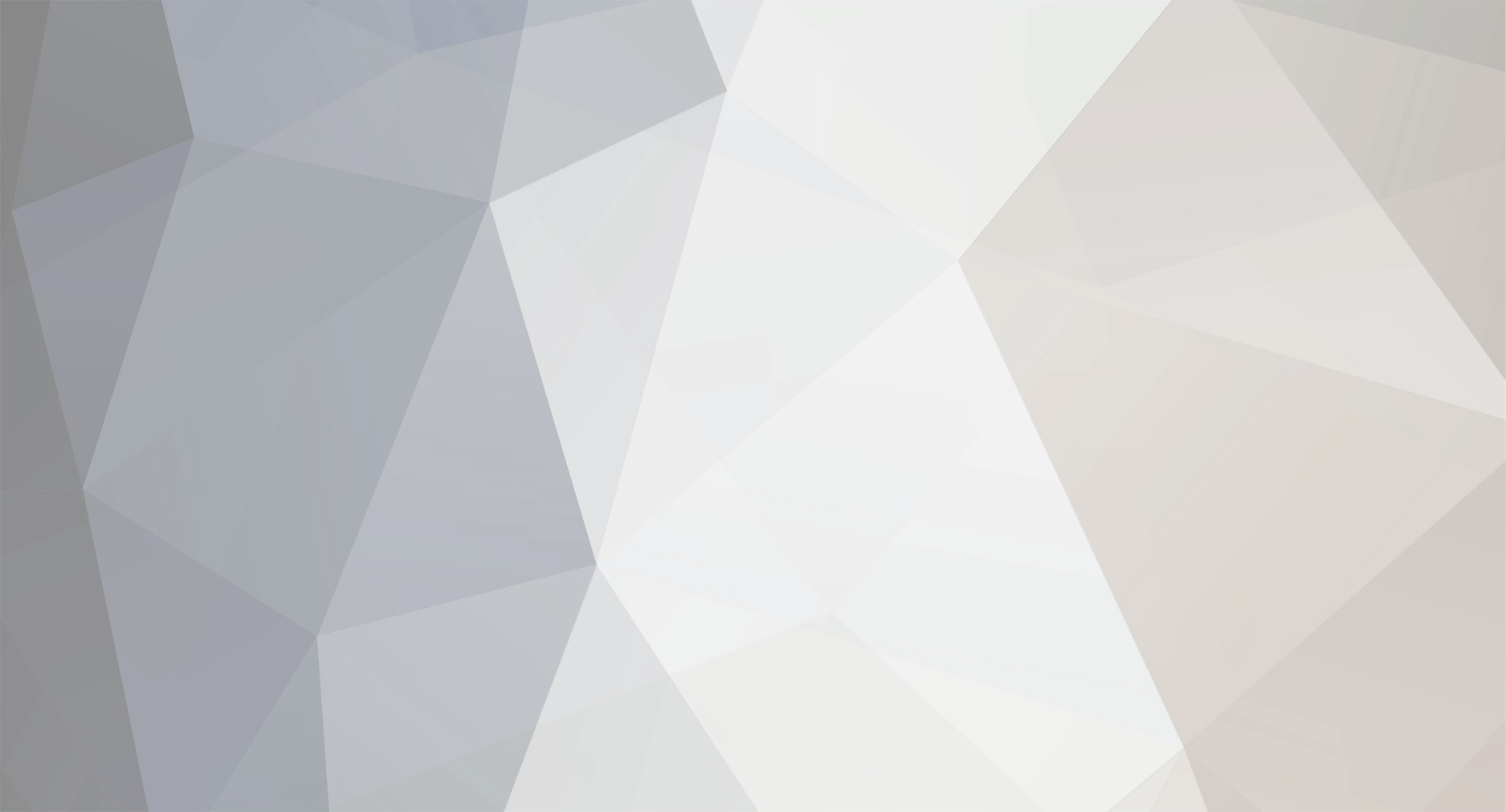
Toastrackenigma
Members-
Posts
49 -
Joined
-
Last visited
Everything posted by Toastrackenigma
-
[1.7.10] Problem moving items in custom GUI slots
Toastrackenigma replied to Toastrackenigma's topic in Modder Support
If I don't use Minecraft.getMinecraft(), then how do I call displayGuiScreen? Also, I changed InventoryEffectRenderer from GuiContainer, because I was reading through forum posts of people that had had similar issues, and someone said that changing that to that fixed a similar issue. I tried it on mine and it didn't really do... anything. I guess I just forgot to change it back. -
Hi everyone! I have been working on making a custom GUI for an item (so that when you shift right-click with the item in hand, the GUI opens), and have run into an issue when trying to render the player's inventory inside. Specifically, the main inventory slots work fine, however the hotbar slots do not work (if you try to pick an item up, it looks like it's about to move then returns to the original state). I have been debugging this problem for a few hours now, and so I have decided to ask you guys for help. Here is my code: magnet.java magnetGui.java magnetGuiContainer.java Thanks
-
@Abastro I wasn't wanting a right-click to happen anyway, I was just using that method as an example of something similar to what I want to do. To put it in clearer terms, I want the liquid to alter items dropped into it using the Q key - for example, if I drop in four gold the ItemStack changes into one diamond. I was wondering if there was a method similar to onItemRightClick but for detecting if an item was dropped into a liquid (onItemDropped maybe)
-
Hey, I have my fluid registered and working (it works in a bucket and also shows up in the world), but I want to know how to make it change items that are dropped in it (like PneumaticCraft's etching acid, or the fluix seeds in AE2). I have looked all over the internet and can't find a reference as to how to do something like this anywhere. I would imagine that the fluid could have some sort of function which fires when an item is dropped into it and gives me back the ItemStack to play with, but that sounds too easy I'm thinking along the lines of the public ItemStack onItemRightClick(ItemStack is, World world, EntityPlayer ep) function for items, but I could be completely wrong. Any suggestions? Thanks, -Toastrackenigma
-
I put a system.out.println("Hello World"); in the public void onBucketFill(FillBucketEvent event) { event and when I tried to pick up the liquid nothing was printed, so it's probably not getting called. I have tried changing the order in which I call my statements but it doesn't seem to do anything. Any advice on how to make that handler get called?
-
I assume that you're using System.out.println("Whatever Text"); - that's the correct way to do it. Have you looked through all of the console? It may be closer to the beginning rather than at the end.
-
Hey forum, I have been following this tutorial (http://www.minecraftforge.net/wiki/Create_a_Fluid) to create a custom liquid in forge 1.7.10. I have the source "block" which I can place in the world and it flows and works fine. I also have a custom bucket which can place down the liquid and then transforms into a vanilla bucket. The problem is that when I then proceed to right-click the liquid with a vanilla bucket to pick it up, I get a water bucket rather than a bucket of my custom liquid. Here is the code: From the main class (in pre-int method): Block diamondliquidblock = new DiamondLiquidBlock(diamondliquid); GameRegistry.registerBlock(diamondliquidblock, diamondliquidblock.getUnlocalizedName().substring(5)); Item diamondliquidbucket = new DiamondLiquidBucket(diamondliquidblock); GameRegistry.registerItem(diamondliquidbucket, diamondliquidbucket.getUnlocalizedName().substring(5)); FluidContainerRegistry.registerFluidContainer(diamondliquid, new ItemStack(diamondliquidbucket), new ItemStack(Items.bucket)); BucketHandler.INSTANCE.buckets.put(diamondliquidblock, diamondliquidbucket); MinecraftForge.EVENT_BUS.register(BucketHandler.INSTANCE); DiamondLiquidBlock class: package com.toastrackenigma.toastcraft; import cpw.mods.fml.relauncher.Side; import cpw.mods.fml.relauncher.SideOnly; import net.minecraft.block.material.Material; import net.minecraft.client.renderer.texture.IIconRegister; import net.minecraft.util.IIcon; import net.minecraft.world.IBlockAccess; import net.minecraft.world.World; import net.minecraftforge.fluids.BlockFluidClassic; import net.minecraftforge.fluids.Fluid; public class DiamondLiquidBlock extends BlockFluidClassic { @SideOnly(Side.CLIENT) protected IIcon stillIcon; @SideOnly(Side.CLIENT) protected IIcon flowingIcon; public DiamondLiquidBlock(Fluid fluid) { super(fluid,Material.water); this.setCreativeTab(Main.maintab); this.setBlockName("liquiddiamond"); } @Override public IIcon getIcon(int side, int meta) { IIcon result = stillIcon; if (side==1) { result = flowingIcon; } return result; } @SideOnly(Side.CLIENT) @Override public void registerBlockIcons(IIconRegister iir) { stillIcon = iir.registerIcon(Main.MODID + ":" + "diamondflowing"); flowingIcon = iir.registerIcon(Main.MODID + ":" + "diamondstill"); } @Override public boolean canDisplace(IBlockAccess world, int x, int y, int z) { if (world.getBlock(x, y, z).getMaterial().isLiquid()) { return false; } else { return super.canDisplace(world, x, y, z); } } @Override public boolean displaceIfPossible(World world, int x, int y, int z) { if (world.getBlock(x, y, z).getMaterial().isLiquid()) { return false; } else { return super.displaceIfPossible(world, x, y, z); } } } DiamondLiquidBucket Class: package com.toastrackenigma.toastcraft; import net.minecraft.block.Block; import net.minecraft.init.Items; import net.minecraft.item.ItemBucket; public class DiamondLiquidBucket extends ItemBucket{ public DiamondLiquidBucket(Block fluidblock) { super(fluidblock); this.setCreativeTab(Main.maintab); this.setContainerItem(Items.bucket); this.setUnlocalizedName("diamondliquidbucket"); this.setTextureName(Main.MODID + ":" + "diamondliquidbucket"); } } BucketHandler Class: package com.toastrackenigma.toastcraft; import java.util.HashMap; import java.util.Map; import cpw.mods.fml.common.eventhandler.Event.Result; import net.minecraft.block.Block; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.util.MovingObjectPosition; import net.minecraft.world.World; import net.minecraftforge.event.entity.player.FillBucketEvent; public class BucketHandler { public static BucketHandler INSTANCE = new BucketHandler(); public Map<Block, Item> buckets = new HashMap<Block, Item>(); public void onBucketFill(FillBucketEvent event) { ItemStack result = fillBucket(event.world, event.target); if (result == null) { return; } event.result = result; event.setResult(Result.ALLOW); } private ItemStack fillBucket(World world, MovingObjectPosition pos) { Block block = world.getBlock(pos.blockX, pos.blockY, pos.blockZ); Item bucket = buckets.get(block); if (bucket != null && world.getBlockMetadata(pos.blockX, pos.blockY, pos.blockZ) == 0) { world.setBlockToAir(pos.blockX, pos.blockY, pos.blockZ); return new ItemStack(bucket); } else { return null; } } } I at one point tried to change the super(fluid,Material.water); line in DiamondLiquidBlock to super(fluid,Material.lava); and the empty bucket turned into a lava bucket instead, so the bucket that I get is obviously determined by the material that I use. Infact, inside the ItemBucket Class there is and if statement for what is returned (water or lava bucket) based off the material of the liquid: if (material == Material.water && l == 0) { p_77659_2_.setBlockToAir(i, j, k); return this.func_150910_a(p_77659_1_, p_77659_3_, Items.water_bucket); } My question is, how to I override this default functionality and make an empty bucket pick up my liquid rather than water / lava? I have searched the internet and have found some people having a similar problem, but their fixes didn't resolve my issue and most were for 1.6 and 1.5 and not 1.7.10. Please help! -Toastrackenigma
-
Saving information after the game is quit?
Toastrackenigma replied to Toastrackenigma's topic in Modder Support
I'm trying to make something a little similar to the way Applied Energistics's Quantum Entangled Singularities work: I want it to be that every time that you perform the crafting recipe the item's damage value goes up one, i.e. GameRegistry.addShapelessRecipe(new ItemStack(Techcraft.magnetHalf, 2, , new Object[] { Techcraft.magnetItem}); Replacing 8 with the damage value. So, the first time you craft this the damage on both of these is 1, then the next time it's 2, etc. Pretty much only two are ever going to have the same damage, so they're a linked pair. I was going to store the damage in the WorldSavedData part -
Saving information after the game is quit?
Toastrackenigma replied to Toastrackenigma's topic in Modder Support
Whenever I'm on a site and the editor buttons don't work it makes me cringe because one of the first projects I did when I learnt how to program was make one that worked properly. It only took me < 1 hour and it never did this to anyone I've sorta fixed the problem myself, I put that code into my item and used onRightClick and it worked (incremented each time I right clicked and when I opened MC again continued where it left off). However, what would I do if I wanted it in my Mod's main class (where I had it initially)? I get that there is no reference to world in there, so how do I give it one? Thanks for all of your help -
Saving information after the game is quit?
Toastrackenigma replied to Toastrackenigma's topic in Modder Support
****Editor buttons still not working**** markDirty() is being called. Here's the worldsave.newChannel() code: public int newChannel() { markDirty(); return loadstoneChannels++; } I think I've posted the whole worldSave file earlier this post. -
Saving information after the game is quit?
Toastrackenigma replied to Toastrackenigma's topic in Modder Support
****Insert quote here, none of the editor buttons are working, who knows why?**** I know that, I just wasn't thinking about this worldSave thing the same way for some reason. I now have: worldSave worldsave = worldSave.get(world); int thesave = worldsave.newChannel(); While this counts up from zero every time it's called, when I close the game and reopen it the variable's forgotten, which kindof defeats the whole point. -
Yes, durability == max damage. There should be plenty of tutorials about this subject online ([lmgtfy]how to make a pickaxe forge 1.7.10[/lmgtfy])
-
[1.7.10] Redstone only emitted from one side of a block?
Toastrackenigma replied to Toastrackenigma's topic in Modder Support
Thanks for telling me that, I thought the params at the top of a method were what I was giving IT, rather than what IT was giving ME (big difference). Although it worked for my other public boolean canConnectRedstone(IBlockAccess world, int x, int y, int z, int side) method, it didn't work for my actual isProvidingStrongPower block. (While it used to provide power to all 6 sides, it now provides power to none). Here's the code: public int isProvidingStrongPower(IBlockAccess world, int x, int y, int z, int side) { if (side==3) { return 15; } else { return 0; } } -
[1.7.10] Redstone only emitted from one side of a block?
Toastrackenigma replied to Toastrackenigma's topic in Modder Support
I'm taking that my issue is the the latter (i.e. a noobish mistake). To be honest I'm only "fairly decent" with Java (not with programming, I'm great with Javascript, PHP, Python, etc), I've been learning it over the holidays and part of that is making this mod. What have I done wrong? -
Saving information after the game is quit?
Toastrackenigma replied to Toastrackenigma's topic in Modder Support
Good to know. So how do I actually do that? I've got this far: worldSave worldsave = worldSave.get(world); This gives me back a random string which looks like this com.toastrackenigma.techcraft.worldSave@26d68a6e (the number on the end is different on the client then it is on the server). How do I use this? -
[1.7.10] Redstone only emitted from one side of a block?
Toastrackenigma replied to Toastrackenigma's topic in Modder Support
Looking more closely at it in Eclipse so do I, but I don't know how to set it because if I do any of the following it doesn't work: public int isProvidingStrongPower(IBlockAccess p_149709_1_, int p_149709_2_, int p_149709_3_, int p_149709_4_, int side 2) public int isProvidingStrongPower(IBlockAccess p_149709_1_, int p_149709_2_, int p_149709_3_, int p_149709_4_, int 2 ) public int isProvidingStrongPower(IBlockAccess p_149709_1_, int p_149709_2_, int p_149709_3_, int p_149709_4_, int side) { side = 2; return 15; } public int side = 2; public int isProvidingStrongPower(IBlockAccess p_149709_1_, int p_149709_2_, int p_149709_3_, int p_149709_4_, side) public int side = 2; public int isProvidingStrongPower(IBlockAccess p_149709_1_, int p_149709_2_, int p_149709_3_, int p_149709_4_, int side) etc. Any tips or am I just making some noobish mistake -Toastrackenigma -
Hey guys, I know how to make a block emit a redstone signal by using public int isProvidingStrongPower(IBlockAccess p_149709_1_, int p_149709_2_, int p_149709_3_, int p_149709_4_, int p_149709_5_) { return 15; } but that emits a signal from all 6 sides of the block as if it was a redstone block. How do I make it only emit a signal from one side of the block like repeaters do (I have looked through the repeater's code in BlockRedstoneDiode.java and BlockRedstoneRepeater.java and found nothing). There also seems to be nothing online about this topic (no tutorials, etc) or topics asking the same question with no answers. -Toastrackenigma
-
If you're looking for the EntityBoat.java file, it's here: recompSrc > net > minecraft > entity > item > EntityBoat.java If you're looking for the EntityBoat.class file, it's here: recompCls > net > minecraft > entity > item > EntityBoat.class Windows Explorer / Finder both have a search bar. Use it.
-
Saving information after the game is quit?
Toastrackenigma replied to Toastrackenigma's topic in Modder Support
Well, because say I want a block to output an int I have stored in it to the chat (just as a test), this isn't working: public boolean onBlockActivated(World world, int x, int y, int z, EntityPlayer player, int par6, float par7, float par8, float par9) { int testthis = worldSave.readFromNBT("testthis"); if (world.isRemote) { player.addChatMessage(new ChatComponentTranslation("Number: "+testthis)); } return true; } Here's the worldSave.java file: package com.toastrackenigma.techcraft; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.world.WorldSavedData; import net.minecraft.world.World; public class worldSave extends WorldSavedData{ private static final String ID = "techcraft"; private int loadstoneChannels = 0; public worldSave() { super(ID); } public worldSave(String identifier) { super(identifier); } @Override public void readFromNBT(NBTTagCompound nbt) { loadstoneChannels = nbt.getInteger("loadstoneChannels"); } @Override public void writeToNBT(NBTTagCompound nbt) { nbt.setInteger("loadstoneChannels", loadstoneChannels); } public int newChannel() { markDirty(); return loadstoneChannels++; } public static worldSave get(World world) { worldSave data = (worldSave)world.loadItemData(worldSave.class, ID); if (data == null) { data = new worldSave(); world.setItemData(ID, data); } return data; } } I know it's because the readFromNBT function has a void return type, but when I change it from void to int and add a return statement it throws errors at me. -
Saving information after the game is quit?
Toastrackenigma replied to Toastrackenigma's topic in Modder Support
Ok, so I've got this worldSave class written now with no errors but how to I go about actually storing things in it? -
Saving information after the game is quit?
Toastrackenigma replied to Toastrackenigma's topic in Modder Support
I can't seem to find much info about this online - any pointers towards a tutorial, etc which could help me with this? -
Hey everybody, I have some information which I'm currently storing in a global variable in my block's class. The idea is that every time the block is placed down, a number stored in this global variable increments. This is important for reasons which I won't explain here Problem is, the global variable is deleted when the game is quit (as I had expected) and I need this value to stick around for when the game is next re-opened. I also need the value to be stored per world, so it can be say 7 in one and 12 in another. I know some mods use txt files in the the world save to store info, so maybe I could do something like that? Otherwise, is there something like a Javascript Cookie or localStorage storage object in Java which I could use to store this value? Thanks in advance -Toastrackenigma