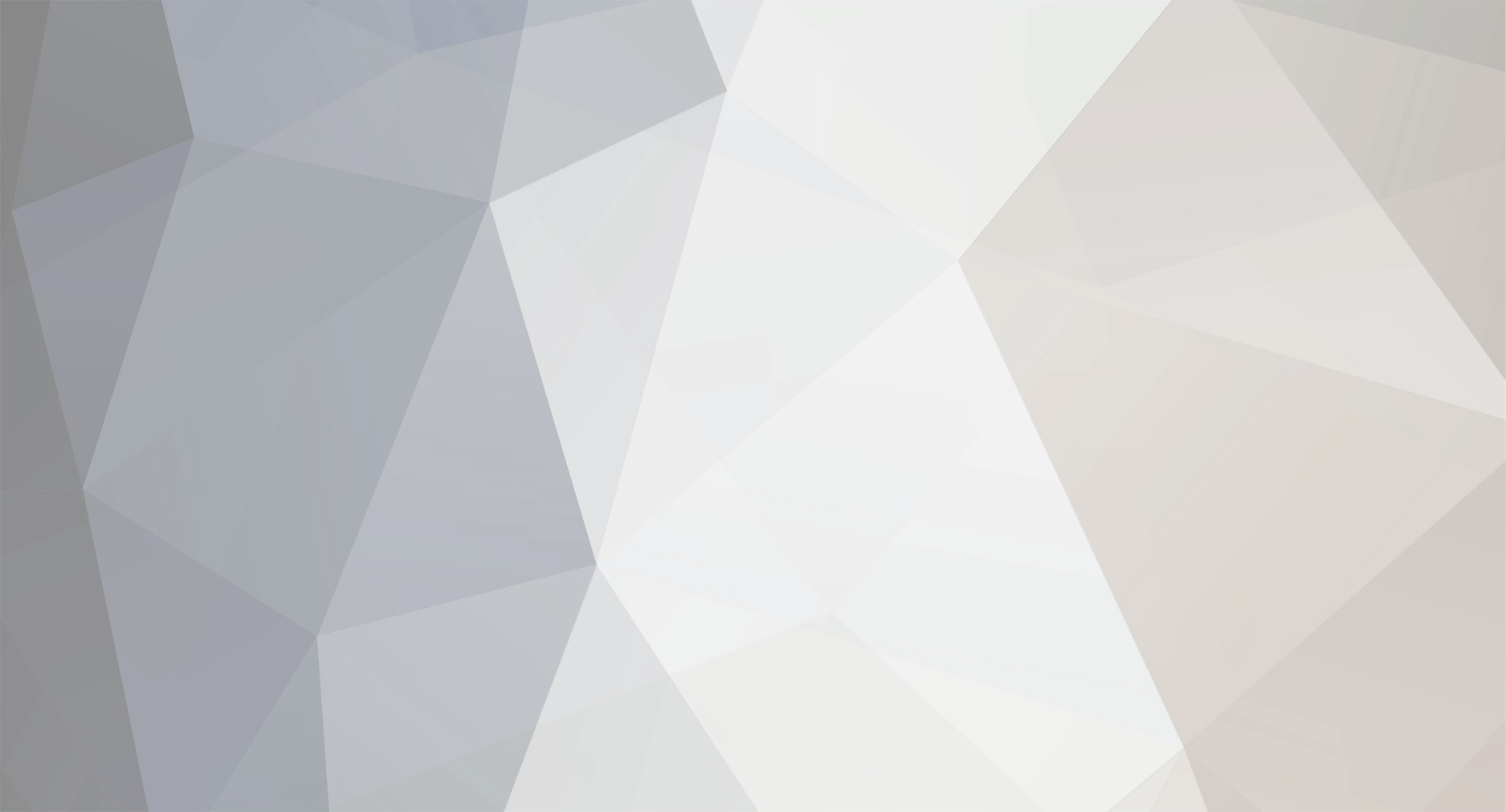
Toastrackenigma
Members-
Posts
49 -
Joined
-
Last visited
Everything posted by Toastrackenigma
-
Thanks, that worked I was going to use the local is variable as what to set the new item to, I never thought that that would change the item in the player's hand For future reference my final code now is: public boolean onBlockActivated(World world, int x, int y, int z, EntityPlayer player, int par6, float par7, float par8, float par9) { if (player.getHeldItem()!=null) { String theitem = player.getHeldItem().getUnlocalizedName().substring(5); if (theitem.equals("unmagneticMagnet")) { player.setCurrentItemOrArmor(0, new ItemStack(Techcraft.normalMagnet,1)); } } return true; }
-
1.7.10 Please help me with my parallel dimension idea.
Toastrackenigma replied to Jordor's topic in Modder Support
Because making a new dimension which looks exactly the same as the overworld (block changes and all) is, as Draco18s said, going to be neigh impossible, why don't you make it so that you don't actually CHANGE dimensions, you just stay in the overworld but get some sort of debuff which lasts until you go through the portal back. The player's made invisible to other people in the "overworld", and the players in the "custom dimension" are made invisible to them. Then just use some sort of shader / renderer / thing to make the blocks look like the ones from your mod. When you mine them you can change them to be not the "overworld" ones but the "custom dimension"'s ones. That seems to me to be the only way to do it, unless you completely re-generate the custom dimension each time as a modified copy of the overworld, which I'm not sure is possible. EDIT: Actually fully read the post, saw you were going to do this anyway Maybe try replacing the vanilla grass, stone, etc with blocks from your mod which look and work the exact same way, and then do a setBlockTextureName() call on them when you go through the portal. That could be how you could go about changing textures, and quite easily -
Hey everyone, I'm trying to make a block give take the item that it's right-clicked with and give you back another one (for example, right click it with a diamond and you get an emerald). Surprisingly, this seems harder than it sounds. I know how to do something similar with items when they are right clicked like so: public ItemStack onItemRightClick(ItemStack is, World world, EntityPlayer ep) { --is.stackSize; is = new ItemStack(Techcraft.unmagneticMagnet,1); } Hopefully I explained what I want to do well enough So far the block removes the currently held item but doesn't replace it. I have looked through vanilla code and nothing in there seems to have the same functionality (things like jukeboxes just take the item which I know how to do, and when you take the item out it puts it on the ground, not into the inventory). Here's my code so far: public boolean onBlockActivated(World world, int x, int y, int z, EntityPlayer player, int par6, float par7, float par8, float par9) { if (player.getHeldItem()!=null) { String theitem = player.getHeldItem().getUnlocalizedName().substring(5); if (theitem.equals("unmagneticMagnet")) { ItemStack is = player.getHeldItem(); --is.stackSize; is = new ItemStack(Techcraft.normalMagnet,1); } } return true; } Please help -Toastrackenigma
-
[SOLVED] Detect what item is held in the player's hand
Toastrackenigma replied to Toastrackenigma's topic in Modder Support
Thanks for all of your help, it works now! That's interesting about == not working in Java, I'm better at python / javascript so hence why I used it as an operator Thanks again, -Toastrackenigma -
[SOLVED] Detect what item is held in the player's hand
Toastrackenigma replied to Toastrackenigma's topic in Modder Support
I do know what value to use Also, toLowerCase() doesn't seem to change anything -
It won't render in the inventory by default (see the hopper or saplings or levers or redstone from vanilla to see what I mean) however if you want it to you've got to make another class: package com.jonnyetiz.mtrade.block; import org.lwjgl.opengl.GL11; import net.minecraft.client.renderer.tileentity.TileEntitySpecialRenderer; import net.minecraft.item.ItemStack; import net.minecraft.tileentity.TileEntity; import net.minecraftforge.client.IItemRenderer; public class BlockCondenserItemRenderer implements IItemRenderer { TileEntitySpecialRenderer render; private TileEntity entity; public BlockCondenserItemRenderer(TileEntitySpecialRenderer render, TileEntity entity) { this.entity = entity; this.render = render; } @Override public boolean handleRenderType(ItemStack item, ItemRenderType type) { return true; } @Override public boolean shouldUseRenderHelper(ItemRenderType type, ItemStack item, ItemRendererHelper helper) { return true; } @Override public void renderItem(ItemRenderType type, ItemStack item, Object... data) { if(type == IItemRenderer.ItemRenderType.ENTITY) GL11.glTranslatef(-0.5F, 0.0F, -0.5F); this.render.renderTileEntityAt(this.entity, 0.0D, 0.0D, 0.0D, 0.0F); } } Then add this to your client proxy just under where you render the block: MinecraftForgeClient.registerItemRenderer(Item.getItemFromBlock(ModBlocks.blockCondenser), new RenderCondenser(render0, new TileEntityCondenser())); Finally, add this to the bottom of your BlockCondenser file: @SideOnly(Side.CLIENT) public void registerBlockIcons(IIconRegister iconRegister) { this.blockIcon = iconRegister.registerIcon("modid:textures/model/texturename.png"); } Hope that helps, -Toastrackenigma
-
[SOLVED] Detect what item is held in the player's hand
Toastrackenigma replied to Toastrackenigma's topic in Modder Support
Thanks for your reply, however that a) gives me an error (diamond cannot be resolved or is not a field) and b) it still just returns "Not a diamond". -
That's weird that par1ItemStack.damageItem(1, player); doesn't damage the item, it works for me in 1.7.10. Have you done setMaxDamage(n) at the beginning of the item class? Also, what version of Minecraft are you modding? I don't know if they changed the way that you damage items between 1.6 - 1.7 but it might have something to do with it.
-
Hello everyone, I've made a block which should do something when right-clicked on if the player currently has a diamond in their hand. If they don't, it should do nothing. However, the if statement always says that there is no diamond in the player's hand, even if there is. Here is my code: public boolean onBlockActivated(World world, int x, int y, int z, EntityPlayer player, int par6, float par7, float par8, float par9) { if (player.getHeldItem()!=null) { String theitem = player.getHeldItem().getUnlocalizedName().substring(5); if (theitem == "diamond") { System.out.println("Is a diamond!"); } else { System.out.println("Not a diamond"); } } return true; } In the above code, Not a diamond is always printed. I have tried putting "diamond" in a variable and then comparing the two variables, I used System.out.println(player.getHeldItem().getUnlocalizedName().substring(5)); to output whatever item the player had in their hand and it said "diamond" in the console. So does that mean that "diamond" != "diamond"? I even put this in before the if statement: System.out.println(player.getHeldItem().getUnlocalizedName().substring(5)=="diamond"); It always returned false. So, could someone please help / tell me what I'm doing wrong? Thanks in advance, -Toastrackenigma
-
It's a very hard thing to do - in my mod I've got an item that when right-clicked sucks everything within 32 blocks towards it, here's the whole class incase it helps [embed=425,349] package com.toastrackengima.techcraft; //Imports import java.util.List; import net.minecraft.creativetab.CreativeTabs; import net.minecraft.entity.item.EntityItem; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.world.World; public class TechDiamondMag extends Item{ public static CreativeTabs tabTech; //Creative tab public TechDiamondMag() { setMaxStackSize(1); //Basic stuff, no stacking, textures, damage, etc setUnlocalizedName("DiamondMag"); this.setCreativeTab(MainMod.tabTech); this.setTextureName("techcraft:DiamondMagnet"); setMaxDamage(1028); } public void addInformation(ItemStack par1ItemStack, EntityPlayer par2EntityPlayer, List par3List, boolean par4) { par3List.add("A golden magnet embued"); //Add some of the item lore par3List.add("with the power of diamond"); par3List.add("Max pull distance: 32"); } @Override public ItemStack onItemRightClick(ItemStack is, World world, EntityPlayer ep) { //While the item is being right-clicked double radius = 5; List<EntityItem> items = world.getEntitiesWithinAABB(EntityItem.class, ep.boundingBox.expand(32, 32, 32)); //The area to pull items from (change 32 to any number) for(EntityItem it : items){ double distX = ep.posX - it.posX; //Move those items! double distZ = ep.posZ - it.posZ; double distY = it.posY+1.5D - ep.posY; double dir = Math.atan2(distZ, distX); double speed = 1F / it.getDistanceToEntity(ep) * 15; //Adjust the speed here (change 15) if (distY<0) { it.motionY += speed; } it.motionX = Math.cos(dir) * speed; it.motionZ = Math.sin(dir) * speed; } is.damageItem(1, ep); //Damage the item (those magnets don't last forever, you know) return is; } } [/embed] You'd have to change some of that to make it into a block, but that should give you a starting point -Toastrackenigma
-
Anywhere between where your item's class is defined and where it ends, you can put this code. Note that you can add as many par3Lists as you want to create new lines of the description, and also that it doesn't auto-wrap (hence why I've split the text up onto two lines): public void addInformation(ItemStack par1ItemStack, EntityPlayer par2EntityPlayer, List par3List, boolean par4) { par3List.add("A regular fish only"); par3List.add("found in water"); } For a specifically coloured name I've been using the section symbol in my en_us.lang file followed by the letter that defines the colour, like so: §eFish That probably isn't the best way to do it, but it works! -Toastrackenigma P.S. Note that for some reason that Eclipse (on my Mac) doesn't encode the section symbol correctly; I would suggest just typing all of the contents of the en_us.lang file in a separate text editor (like notepad or textedit) to make it work
-
Ok so the title basically says it all: how can I create a non-standard block (block that is not a full 1 * 1 * 1 opaque cube) like an anvil, torch, repeater, etc from vanilla minecraft. I have looked in all of the relevant code but I didn't understand it further than fact that they all had these booleans and different textures for each side / part of the block. public boolean renderAsNormalBlock() { return false; } public boolean isOpaqueCube() { return false; } I have included both booleans in my code and have the following code rendering the different textures for the top, side and bottom of the block: protected IIcon MagStandBottom; protected IIcon MagStandSide; protected IIcon MagStandTop; @Override public void registerBlockIcons(IIconRegister p_149651_1_) { MagStandBottom = p_149651_1_.registerIcon("techcraft:MagStandBottom"); MagStandSide = p_149651_1_.registerIcon("techcraft:MagStandSide"); MagStandTop = p_149651_1_.registerIcon("techcraft:MagStandTop"); } @Override public IIcon getIcon(int side, int metadata) { if (side == 0) { return MagStandBottom; } else if (side==1) { return MagStandTop; } else { return MagStandSide; } } It renders the different sides and I can't place torches or redstone on it, but how can move the sides inwards or have legs on it (like the cauldron)? Thanks for helping, Toastrackenigma
-
[SOLVED][1.7.10] Newbie stepping over to Forge
Toastrackenigma replied to AnotherDeveloper's topic in Modder Support
The directory to put the textures for your blocks is /src/main/resources/assets/[modname]/textures/blocks Put your item's textures in /src/main/resources/assets/[modname]/textures/items If you don't have the assets, textures, blocks or items folders then create them Also, your en_US.lang file (and all other lang files) should go into /src/main/resources/assets/[modname]/lang rather than the resources folder. You can find an up-to-date tutorial for some of the basic things you might want to do here: http://www.minecraftforum.net/forums/mapping-and-modding/mapping-and-modding-tutorials/1571558-1-7-2-forge-add-new-block-item-entity-ai-creative Hope that helps -
Here's how to generate ores in 1.7.10 (assuming you already have made the block to be your ore) In your mod's main class, type in this before the @EventHandler: public static MyBlockWG myblockwg = new MyBlockWG(); You can call myblockwg and MyBlockWG anything you want, but make sure that both instances of MyBlockWG are the same. After the @EventHandler add this: GameRegistry.registerWorldGenerator(myblockwg, 1); Make sure that myblockwg is the same as what you changed myblockwg to in the first line. Now make a new class called whatever you changed MyBlockWG to (in this demo it'll be MyBlockWG) and copy and paste this over everything: package com.toastrackengima.mymod; //Change to your package's name //Import stuff import java.util.Random; import net.minecraft.world.World; import net.minecraft.world.chunk.IChunkProvider; import net.minecraft.world.gen.feature.WorldGenMinable; import cpw.mods.fml.common.IWorldGenerator; public class LoadOreWG implements IWorldGenerator { @Override public void generate(Random random, int chunkX, int chunkZ, World world, IChunkProvider chunkGenerator, IChunkProvider chunkProvider) { //Do different things to generate / not generate in the overworld, the nether and the end switch(world.provider.dimensionId) { case -1 : generateInNether(world,random, chunkX*16, chunkZ*16); break; case 0 : generateInOverworld(world,random, chunkX*16, chunkZ*16); break; case 1 : generateInEnd(world,random, chunkX*16, chunkZ*16); break; } } public void generateInEnd(World world, Random random, int x, int y) { //Since we don't want it in the end, this is empty } public void generateInOverworld(World world, Random random, int x, int z) { for(int i=0; i<6; i++) { //The number after i (in this case 6) is how many veins spawn per chunk (16*16*16 area) int xcoord = x + random.nextInt(16); //Sets random coords for the x axis int ycoord = random.nextInt(256); //Sets random coords for the y axis int zcoord = z + random.nextInt(16); //Sets random coords for the z axis new WorldGenMinable(MainMod.loadore, 15).generate(world, random, xcoord, ycoord, zcoord); //Generates it (the number in the World Gen Minable function (in this case 15) is the max amount of blocks in each vein). } } public void generateInNether(World world, Random random, int x, int y) { //Since we don't want it in the nether, this is empty } } Hope this helps
-
How to make a block light up the area around it?
Toastrackenigma replied to Toastrackenigma's topic in Modder Support
Good to know. I think that must have been how they made the blackout curtains in Extra Utilities. Also, after thinking about it some more I thought that there could be an item that you throw on the ground and it makes everything dark. That would be awesome @Kriki98 So you're basically adding more than one blocks emitting 1.0F light but they're sky blocks so they just surround the block that looks like it's emitting the light. Sounds like a plan! -
How to move items towards the player?
Toastrackenigma replied to Toastrackenigma's topic in Modder Support
@MultiMote Thank you so much that worked! Now my magnet sucks items towards it! :) :) -
How to make a block light up the area around it?
Toastrackenigma replied to Toastrackenigma's topic in Modder Support
@Kwibble That's fine It was my second day modding and I hadn't even realised I could look at MC's source code so you had a point! @drok0920 That's actually a pretty interesting question. Also, before I figured out that 1.0F was the highest you could go I set the block to 16.0F and it actually darkened rather than lit... sort of acting as a blackout torch. It was kinda cool and I had half a mind to keep it in the mod -
How to move items towards the player?
Toastrackenigma replied to Toastrackenigma's topic in Modder Support
@MultiMote The code was way to complex @ArcaneFractal That sounds like it could actually work... does anyone know the method used to set the velocity? So far I've got @Override public ItemStack onItemRightClick(ItemStack itemstack, World world, EntityPlayer player) { world.getEntitiesWithinAABBExcludingEntity(player, player.boundingBox.expand(8D, 8D, 8D)); return itemstack; } Pretty sure that should select the items, I think that the world.getEntites part should return a list of entites around the player in a 8x8x8 radius. For the velocity I'm imagining something along the lines of setItemVelocity(), setItemSpeed(), ItemVelocity(), etc. I could be completely wrong Toastrackenigma -
How to move items towards the player?
Toastrackenigma replied to Toastrackenigma's topic in Modder Support
Thanks for the help, but after a bit more research I've decided that moving items is too hard for my third day modding! After thinking about it, removing the items from the world and then adding them to the player's inventory would achieve the same goal as moving them towards the player. Do you guys think that would be easier than actually moving them towards the player? If so, do you have any pointers or advice that you can give me to achieve this? Thanks again Toastrackenigma P.S. @MultiMote handleMaterialAcceleration is in the world.java class and only handles the movement of items when they are in water. -
Hi, I've got an item in my mod which I want (while right-click is held) to begin pulling items within 8 blocks towards the player. So far I have this code: ... @Override public ItemStack onItemRightClick(ItemStack itemstack, World world, EntityPlayer player) { System.out.println("Hello World!"); return itemstack; } That prints out Hello World to the terminal while I am pressing right click, however I have no idea where to start with actually pulling items towards the player. Thanks in advance Toastrackenigma P.S. I'm modding Minecraft 1.7.10
-
How to make a block light up the area around it?
Toastrackenigma replied to Toastrackenigma's topic in Modder Support
@F1repl4ce Thanks, it worked! @Kwibble Thanks also, I didn't think to look there and had just found it when @F1repl4ce replied. I also didn't know that about numbers higher than 1.0F - I thought the higher the float the more light would be emitted... well there's why it wasn't working! For anyone that's intrested / having the same problem my final code is now setLightLevel(1.0F); -
How to make a block light up the area around it?
Toastrackenigma replied to Toastrackenigma's topic in Modder Support
@Phyyrus: Although that used to work in 1.6.4 I am trying to mod 1.7 (sorry, I forgot to mention that ) and setLightValue has been replaced with setLightLevel which for some reason is not working for me @Kwibble: I tried looking through the Vanilla code for Minecraft (specifically at the glowstone and torch java files) but I couldn't see any reference to emitting light no matter how hard I looked. Also, there seem to be no tutorials on Google/Youtube for adding this kind of functionality. Thanks for your replies! -
The question's basically the title How can I make my custom block like up the area around it? I tried setLightLevel(32F); but the appears to be inside the block rather than outside it. (Basically I want it to act like Glowstone) I'm new to modding (I started my first mod yesterday) and so I'm sure it's a basic problem but I searched Google for over an hour without finding anything, so I came here! Thanks in advance! -Toastrackenigma