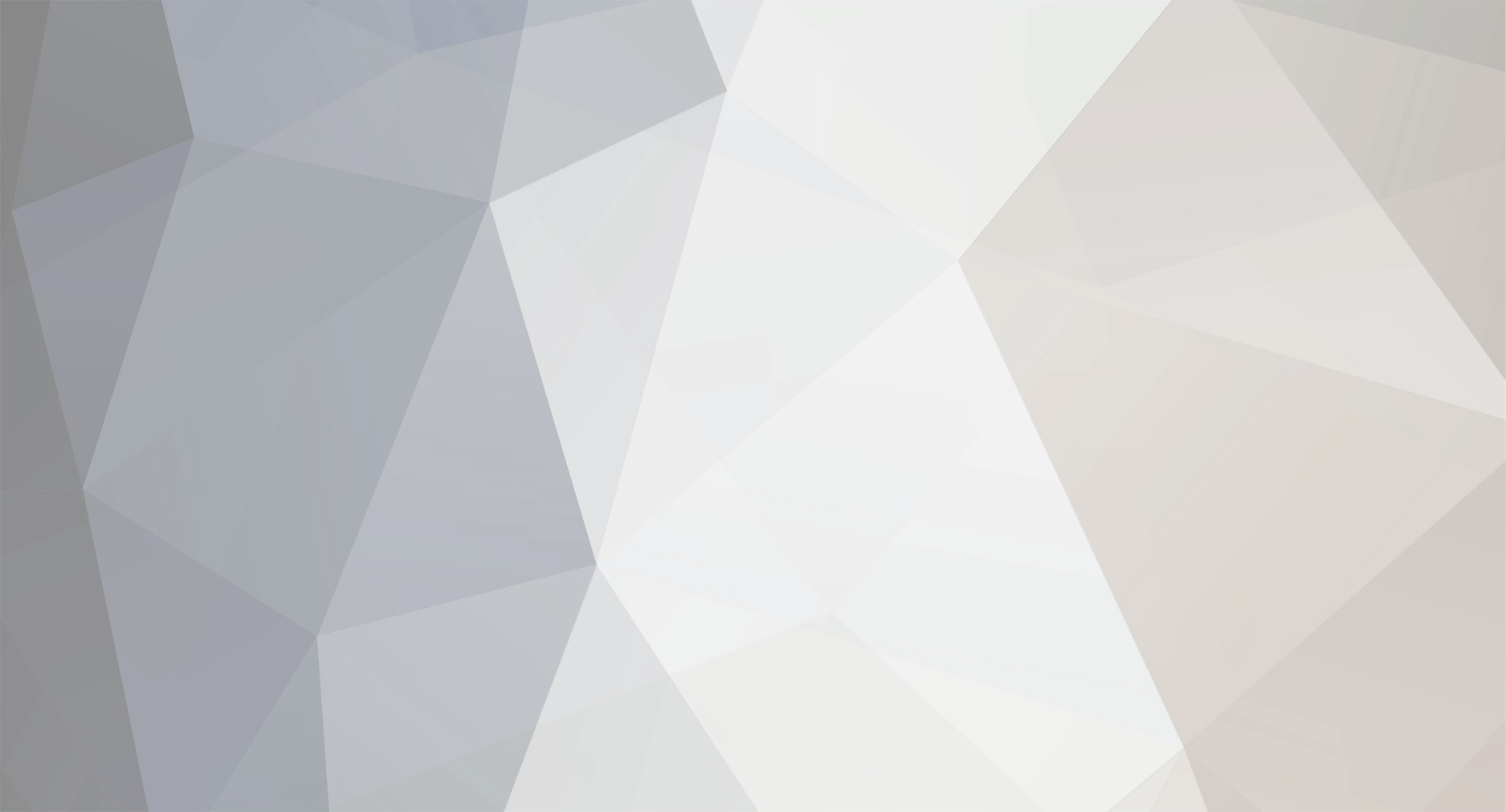
knokko
Members-
Posts
255 -
Joined
-
Last visited
Converted
-
Gender
Male
-
Location
Holland
-
Personal Text
I am speaking Dutch, sorry if my English is bad.
Recent Profile Visitors
The recent visitors block is disabled and is not being shown to other users.
knokko's Achievements

Diamond Finder (5/8)
15
Reputation
-
Wow, that worked, now the packets also arrive at the client side. Thank you for the help.
-
There are several methods in the Item class, override one of the methods and destroy your item.
-
I am trying to render energy in cables in my mod. The TileEntity sends a packet to the Client that says where to render the energy. But between toBytes(ByteBuf and fromBytes(ByteBuf, it causes several Exceptions and a crash. There seems to be a problem with the discriminator, it seems to be -1 when the ID of the message is 0. Here is the message registered: public static SimpleNetworkWrapper network; @EventHandler public void preInit(FMLPreInitializationEvent event){ HyperItems.load(); HyperBlocks.load(); network = NetworkRegistry.INSTANCE.newSimpleChannel("knokko Hyper Combat"); network.registerMessage(CableMessage.Handler.class, CableMessage.class, 0, Side.CLIENT); } Here is the packet class: public class CableMessage implements IMessage { public static final byte[] BYTES = new byte[]{64,32,16,8,4,2,1}; private int x; private int y; private int z; private boolean[] energy; public CableMessage() {} public CableMessage(int x, int y, int z, boolean[] energy){ this.x = x; this.y = y; this.z = z; this.energy = energy; } @Override public void fromBytes(ByteBuf buf) { System.out.println("decode from bytes..."); x = buf.getInt(0); y = buf.getInt(4); z = buf.getInt(; energy = toBinair(buf.getByte(12)); System.out.println("decoded from bytes"); } @Override public void toBytes(ByteBuf buf) { System.out.println("convert to bytes..."); buf.setInt(0, x); buf.setInt(4, y); buf.setInt(8, z); buf.setByte(12, fromBinair(new boolean[]{energy[0], energy[1], energy[2], energy[3], energy[4], energy[5], false, false})); System.out.println("converted to bytes"); } public static class Handler implements IMessageHandler<CableMessage, IMessage> { //Gets the TileEntity at the given position and marks the places to render the energy @Override public IMessage onMessage(final CableMessage message, MessageContext ctx) { IThreadListener listener = Minecraft.getMinecraft(); listener.addScheduledTask(new Runnable(){ @Override public void run(){ World world = Minecraft.getMinecraft().theWorld; TileEntity entity = world.getTileEntity(new BlockPos(message.x, message.y, message.z)); if(world.isRemote && entity instanceof TileEntityCable) ((TileEntityCable)entity).isEnergy = new boolean[]{message.energy[0], message.energy[1], message.energy[2], message.energy[3], message.energy[4], message.energy[5]}; System.out.println("packet arrived: energy = " + message.energy + " and entity = " + entity); }}); return null; } } //gets 8 booleans from 1 byte public static boolean[] toBinair(byte b){ boolean[] bools = new boolean[8]; if(b >= 0) bools[7] = true; else { b++; b *= -1; } byte t = 0; while(t < 7){ if(b >= BYTES[t]){ b -= BYTES[t]; bools[t] = true; } ++t; } return bools; } //stores 8 booleans into 1 byte public static byte fromBinair(boolean[] bools){ byte b = 0; int t = 0; while(t < 7){ if(bools[t]) b += BYTES[t]; ++t; } if(!bools[7]){ b *= -1; b--; } return b; } } Here is the method where the packet is sent: @Override public int drainEnergy(EnumFacing facing, int requestedAmount) { //isEnergy = new boolean[6]; System.out.println("drainEnergy..."); int energy = 0; //isDraining is here to prevent infinite loops if(!isDraining){ if(capacity == 0){ Block block = worldObj.getBlockState(pos).getBlock(); if(block instanceof BlockCable) capacity = ((BlockCable) block).getCapacity(); } if(requestedAmount > capacity) requestedAmount = capacity; isDraining = true; int t = 0; while(t < EnumFacing.values().length){ if(energy >= requestedAmount){ isDraining = false; //sending the packet... System.out.println("starting to send message..."); HyperCombat.network.sendToAllAround(new CableMessage(pos.getX(), pos.getY(), pos.getZ(), isEnergy), new TargetPoint(worldObj.provider.getDimensionId(), pos.getX(), pos.getY(), pos.getZ(), 32)); System.out.println("message send... hopefully"); return energy; } if(facing != EnumFacing.values()[t].getOpposite()){ int extra = drain(EnumFacing.values()[t], requestedAmount - energy); energy += extra; if(extra > 0) isEnergy[t] = true; } ++t; } } isDraining = false; if(energy > 0) isEnergy[facing.ordinal()] = true; System.out.println("starting to send message..."); HyperCombat.network.sendToAllAround(new CableMessage(pos.getX(), pos.getY(), pos.getZ(), isEnergy), new TargetPoint(worldObj.provider.getDimensionId(), pos.getX(), pos.getY(), pos.getZ(), 32)); System.out.println("message send... hopefully"); return energy; } Here is the important part of the console log: Does anybody understand what goes wrong here? Why is the discriminator -1?
-
[1.8] How to make a Item not consumable in the crafting table
knokko replied to windows300's topic in Modder Support
This code works for me in 1.7.10, maybe it will work too in 1.8: @Override public boolean doesContainerItemLeaveCraftingGrid(ItemStack itemStack) { return false; } -
Copy your source code, install forge, and paste your source code there. And than you can start fixing a few hundred errors probably
-
Read your code very carefully, you probably forgot a ';' or bracket'('.
-
[1.7.2] Trying to Insta-Kill Player with potion
knokko replied to yanksrock1019's topic in Modder Support
As potioneffect, you can use new PotionEffect(7, 1, 100); -
Here is the superclass: public abstract class Dragon extends EntityFlying implements IMob{ protected EntityLivingBase target; public Dragon(World worldIn) { super(worldIn); setSize(30, 3); isImmuneToFire = true; } public void onUpdate(){ super.onUpdate(); if(target == null){ target = worldObj.getClosestPlayerToEntity(this, getEntityAttribute(SharedMonsterAttributes.followRange).getAttributeValue()); } if(target != null){ followTarget(); shootingUpdate(); faceEntity(target, 30, 30); } attackEntities(); } public abstract void shootingUpdate(); public void attackEntities(){ AxisAlignedBB aabb = getEntityBoundingBox(); List list = worldObj.getEntitiesWithinAABBExcludingEntity(this, aabb); int times = 0; while(times < list.size()){ Entity entity = (Entity) list.get(times); if(!(entity instanceof EntityItem)){ entity.attackEntityFrom(DamageSource.causeMobDamage(this), (float) getEntityAttribute(SharedMonsterAttributes.attackDamage).getAttributeValue()); } ++times; } } public void followTarget(){ Line line = new Line(new Position(this), new Position(target)); double speed = getEntityAttribute(SharedMonsterAttributes.movementSpeed).getAttributeValue(); motionX = ExtraUtils.divineAccurate(line.distanceXT, line.distance) * speed; motionY = ExtraUtils.divineAccurate(line.distanceYT, line.distance) * speed; motionZ = ExtraUtils.divineAccurate(line.distanceZT, line.distance) * speed; } public void setAttackTarget(EntityLivingBase entity){ target = entity; } public void setRevengeTarget(EntityLivingBase entity){ target = entity; } public EntityLivingBase getAttackTarget(){ return target; } public EntityLivingBase getAITarget(){ return target; } public void applyEntityAttributes(){ super.applyEntityAttributes(); getAttributeMap().registerAttribute(SharedMonsterAttributes.attackDamage); } public AxisAlignedBB getCollisionBox(Entity in){ return getEntityBoundingBox(); } public AxisAlignedBB getBoundingBox(){ return getEntityBoundingBox(); } } Here is the 'real' class: public class FireDragon extends Dragon{ public int cooldown; public int fireTimer; public boolean shootCooldown; public FireDragon(World worldIn) { super(worldIn); setSize(30,3); } @Override public void shootingUpdate() { if(!worldObj.isRemote){ if(cooldown <= 0){ if(fireTimer < 5 && shootCooldown){ EntityLargeFireball fire = new EntityLargeFireball(worldObj, this, 1, 1, 1); fire.explosionPower = 2; worldObj.spawnEntityInWorld(fire); ++fireTimer; shootCooldown = false; } else if(fireTimer >= 5){ cooldown = 100; fireTimer = 0; } else { shootCooldown = true; } } else { --cooldown; } } } @Override public void applyEntityAttributes(){ super.applyEntityAttributes(); getEntityAttribute(SharedMonsterAttributes.attackDamage).setBaseValue(10); getEntityAttribute(SharedMonsterAttributes.movementSpeed).setBaseValue(0.3); getEntityAttribute(SharedMonsterAttributes.followRange).setBaseValue(64); getEntityAttribute(SharedMonsterAttributes.maxHealth).setBaseValue(400); } } Any ideas what can go wrong?
-
Are you sure you have exported all required folders? You needed to export 3 folders. Can you open the source folders from the mod that imports the other mod?
-
I have tested it's hitbar with arrows. The hitbar for arrows is different than the hitbar for normal attacks. Really strange, isn't it?
-
I have looked in EntityZombie and EntityDragon (of minecraft) and there block size is used. Can it be a problem with max lenght or something?
-
I think like the normal blocks, but they have a lot of subblocks.(metadata, data values...)
-
It isn't as difficult as you think. It was pretty easy for me, even when I hardly knew java. Eclipse has an export option, you need to export src/main/java, src/main/resources and the folder src. In eclipse you have a toolbar above your files, you need project/properties/libraries/add external jar and than choose the jar you created with the export funtion.
-
I allready have that, "setSize(30,3)". The hitbar is still very small. But the hitbar isn't large enough.
-
I think bFull3D was the return his IDE used to auto-complete his method I think he is a beginnen because he didn't find his problem himself and what diesieben said.