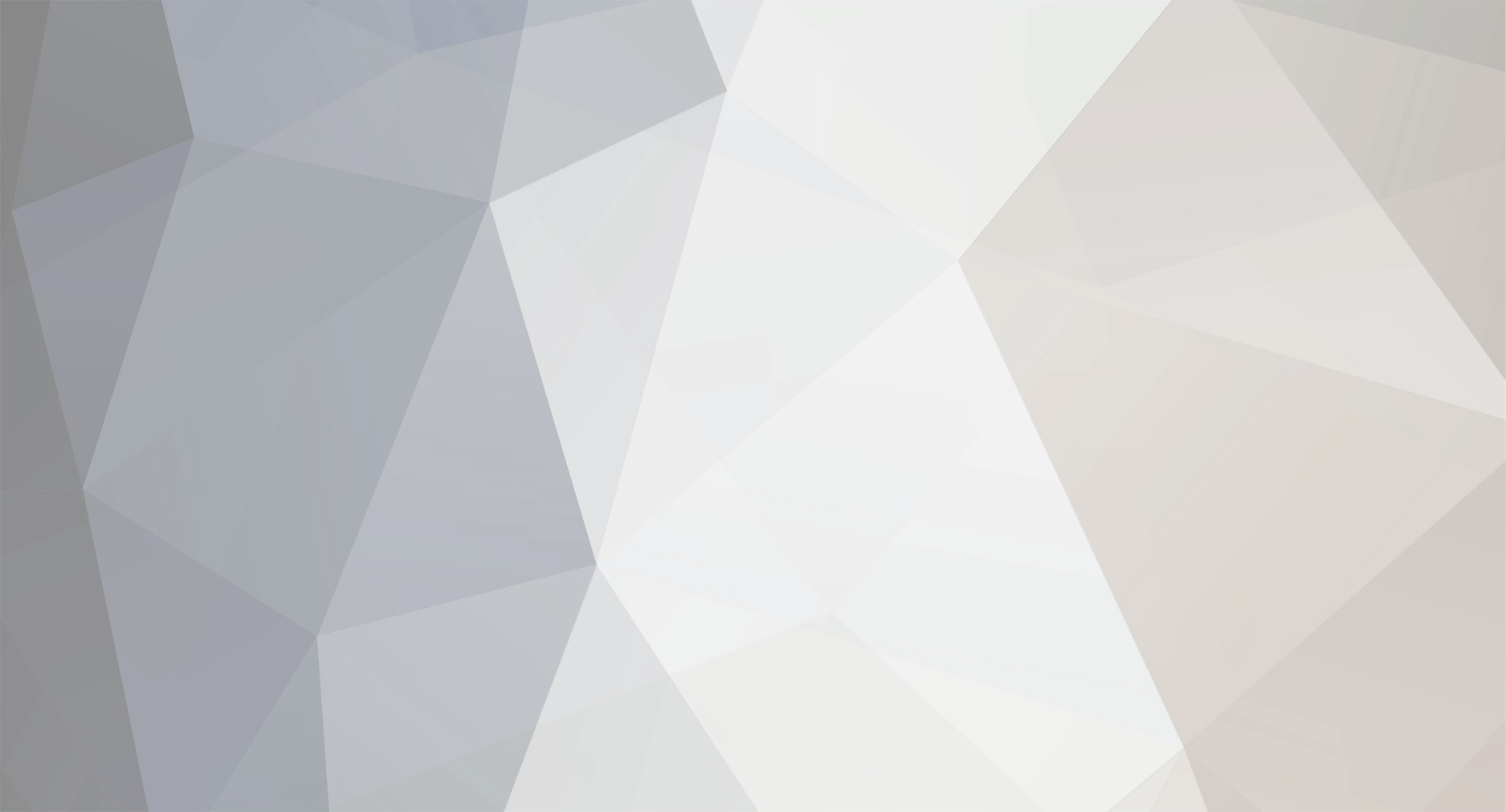
TheEpicTekkit
Members-
Posts
361 -
Joined
-
Last visited
Everything posted by TheEpicTekkit
-
setting depth mast to true resulted in this: [/img] and [/img] So the water and other TESR models behind it such as the wind turbine, are now getting rendered, but now the pipe itself has really odd transparency. Things like the inside of the pipe looks like it is in front of the outside of the pipe, and in image two, the pipe in the background looks to be in front of the pipe in the foreground.Also, there are really annoying and messy looking dark and light bits. What can I do? I either need a way to fix this, or how do I do the render last fix that you suggested?
-
Hi everyone. I am working on a pipe that will transport items (like ItemDucts or Buildcrafts pipes, I haven't actually got to the actual item transport part yet, I am just working on rendering today) and I have decided to make the sides transparent so that you can see the items inside. I have successfully achieved this, but unfortunately, there is some weird rendering of the world behind it. Full blocks are fine, but anything else (for some reason, excluding lava) isn't rendered, so this includes water, and any other models. Here are some screenshots to illustrate what I mean: [/img] and [/img] As you can see, the water behind, and my wind turbine model aren't getting rendered behind the transparent part of the pipe. There are also some transparent bits inside the pipe when looked at as some angles (seen in pic 1) My current code for rendering this is this: Any suggestions on how to fix this? Thanks.
-
There really isn't any code you couldn't have already guessed I have. but anyway... //Side id - Relative -ForgeDirection public IIcon sideBottom; //Side 0 - Bottom -DOWN public IIcon sideTop; //Side 1 - Top -UP public IIcon sideFrontOn, sideFrontOff; //Side 2 - Front -NORTH public IIcon blockIcon; //Side 3 - Back -SOUTH public IIcon sideLeft; //Side 4 - Left -WEST public IIcon sideRight; //Side 5 - Right -EAST @Override @SideOnly(Side.CLIENT) public IIcon getIcon(int side, int meta) { if (side == 0 || side == 1) { if (side == 0) return this.sideBottom; if (side == 1) return this.sideTop; } else if (tileMachine != null) { if (meta == 0 || meta == 1) { if (this.tileMachine.isActive) return side == 2 ? this.sideFrontOn : this.blockIcon; else return side == 2 ? this.sideFrontOff : this.blockIcon; } if (meta == 2 || meta == 3) { if (this.tileMachine.isActive) return side == 5 ? this.sideFrontOn : this.blockIcon; else return side == 5 ? this.sideFrontOff : this.blockIcon; } if (meta == 4 || meta == 5) { if (this.tileMachine.isActive) return side == 3 ? this.sideFrontOn : this.blockIcon; else return side == 3 ? this.sideFrontOff : this.blockIcon; } if (meta == 6 || meta == 7) { if (this.tileMachine.isActive) return side == 4 ? this.sideFrontOn : this.blockIcon; else return side == 4 ? this.sideFrontOff : this.blockIcon; } } return blockIcon; } @Override @SideOnly(Side.CLIENT) public void registerBlockIcons(IIconRegister icon) { this.sideBottom = icon.registerIcon(modid + ":" + this.getUnlocalizedName().substring(5) + "_Bottom"); this.sideTop = icon.registerIcon(modid + ":" + this.getUnlocalizedName().substring(5) + "_Top"); this.sideFrontOn = icon.registerIcon(modid + ":" + this.getUnlocalizedName().substring(5) + "_Front_on"); this.sideFrontOff = icon.registerIcon(modid + ":" + this.getUnlocalizedName().substring(5) + "_Front_off"); this.sideLeft = icon.registerIcon(modid + ":" + this.getUnlocalizedName().substring(5) + "_Left"); this.sideRight = icon.registerIcon(modid + ":" + this.getUnlocalizedName().substring(5) + "_Right"); this.blockIcon = icon.registerIcon(modid + ":" + this.getUnlocalizedName().substring(5) + "_Back"); }
-
Hi everyone. So, I am a bit stumped on a problem here. I can't seem to figure out how to display the side icons on my machine in a GUI. The icons work fine when in the world, but when as an ItemBlock in a GUI, the top and bottom icons are fine, as they are constant, but the front, back, left and right don't get displayed, instead, they default to the "blockIcon" IIcon, which I use as the back icon. How can I set the side each icon goes on when my machine is an ItemBlock? Thanks.
-
[1.7.10] [SOLVED] Every furnace/campfire burns
TheEpicTekkit replied to Bektor's topic in Modder Support
Sigh.... This is basic Java. You are incorrectly using the static modifier. The static modifier means that it will be the same for all other instances of that class, it is basically like a universal variable, that is the same everywhere. Your burning boolean is static, so if one is burning, every other instance of your tileentity will also be burning. Read up on the static modifier. -
Is this pipe a custom rendered block that connects when placed next to another? or is it a full 1x1x1 block? Also, do you mean that depending on the amount of fluid in the pipe, a different height of fluid is rendered? (kind of like BuilcCrafts pipes) If so, then there would be a little bit of maths to do this, not a tone though, really just getting the currently stored amount, dividing it by fluid capacity of the pipe, and multiplying it by height of the maximum amount to be rendered (I am not 100% sure of this, but it will be something along those lines). Also, you would need a custom block renderer to do this in. You would also need a method in the renderer to get the current texture of the fluid in the pipe. I can't tell you how to do this at the moment (I am on a school pc, and don't have access to my code at home to make sure if I am correct, and I don't want to be giving wrong answers) but will do later if nobody already has by then.
-
I need some help with making a mod :c
TheEpicTekkit replied to RibbonHeart's topic in Modder Support
Well... I guess he has some inefficient techniques of doing things, but the key is weather you understand what he is doing or not. He does explain what he is doing well, so it shouldn't be too hard. -
I need some help with making a mod :c
TheEpicTekkit replied to RibbonHeart's topic in Modder Support
ScratchForFun - Very detailed and clear tutorials. -
Okay, do you understand GL11.glRotatef now? if so, all you really need to achieve that then is some variable in the tileentity that stores the rotation, and on what axis, then in renderTileEntityAt, just do a check to make sure this is the right tileentity, then cast the tileentity variable to your tileentity, and then you can access those variables from your renderer.
-
If it is a model, you have a rendering class for it, registered in your ClientProxy, If you don't have this, I don't quite know how or what you are doing, and you should look into setting it up like that. So, in your renderTileEntityAt method, put the GL11.glRotatef method after your first GL11.glPushMatrix. The first parameter is the angle you want to rotate it, and the second, third, and fourth are the x, y, and z axis. So for example, to rotate it -90 degrees on the y axis, you would have: GL11.glRotatef(-90, 0.0F, 1.0F, 0.0F); The numbers for the x, y, z axis are a bit like a percentage of how much it will affect that axis. So 1, is like 100% and will rotate it for the full -90 degrees on that axis, and 0 will not affect the axis at all. You can do something like 0.5F to only rotate it 50% so the result will be -45 degrees. In your case, you want to use metadata to determine this rotation. So in your block, set the blocks metadata depending on the players rotationYaw (see the vanilla furnace to understand how this is done, if you don't already understand it. You would want to look at the methods onBlockPlacedBy, and onBlockAdded), and in the renderTileEntityAt method, you can use the TileEntity parameter to retrieve the metadata.
-
GL11.glRotatef(angle, xAxis, yAxis, zAxis); You just have to figure out the axis.
-
[1.7.10]Rendering Custom Rendered Block - Alpha Problem
TheEpicTekkit replied to Mecblader's topic in Modder Support
I have just noticed something. You don't have a GL11.glPushMatrix(); and a GL11.glPopMatrix(); How is this working without that? Before you start anything with GL11, start with GL11.glPushMatrix, and when you have finished, end with GL11.glPopMatrix. -
[1.7.10]Rendering Custom Rendered Block - Alpha Problem
TheEpicTekkit replied to Mecblader's topic in Modder Support
I'm not quite sure what you mean by "weird line artifact" explain? And have you tried enabling GL_CULLING where I said to, then disabling it again at the end, just before GL11.glPopMatrix(); so that you enable it, render everything, then disable it again? -
[1.7.10]Rendering Custom Rendered Block - Alpha Problem
TheEpicTekkit replied to Mecblader's topic in Modder Support
In your renderTileEntityAt method, try putting GL11.glDisable(GL11.GL_CULLING); Quick question, is your pipes connections rendering properly? just curious, because the way I am doing it in my mod uses a lot less code for the rendering. Looks like you have followed ScratchForFun's pipes tutorial. -
Hello everyone. I was wondering how I would set the metadata for a block as an ItemBlock. Normally this doesn't matter, but when it has multiple textures, specifically, a front texture, I want to know how I orientate it in the players inventory. Currently, all the icons are just the default blockIcon (I normally use this icon as the back, and sides). I can't figure out how vanilla blocks such as the furnace, dispenser, or piston do it. Thanks.
-
ScratchForFun - He does basic and advanced tutorials.Even as advanced as cables and power.
-
Recipes are normally loaded in post-Init, right? try clearing the list in pre-Init or Init.
-
Hello everyone. I don't have a problem that needs solving, but a method that I want to understand better than I do. I am making many blocks with custom GUIs in my mod, and so far, they have had pretty simple GUIs, and I have just copied the transferStackInSlot method from ContainerFurnace with minor modifications that I still barely understood. But now I am making a more complicated GUI, and I have 1 input slot (ID 0), and 6 output slots (ID 1 to 7), I am trying to set up the transferStackInSlot method for this, but am getting confused and stuck. All I really want is a detailed explanation to help me understand what this method does, how it works, and how to use it for custom GUIs. Thanks.