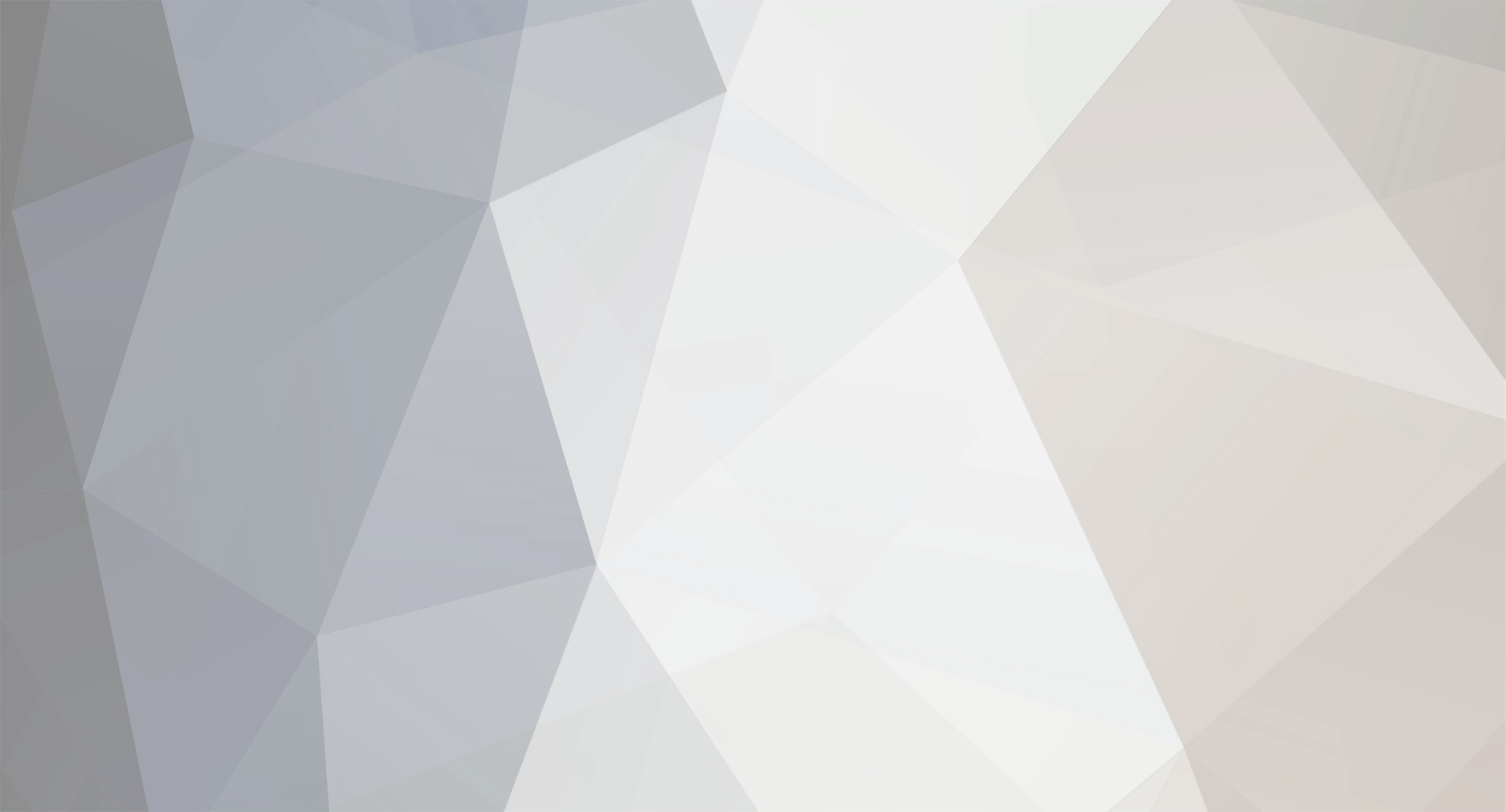
GhostSnyperRecon
-
Posts
50 -
Joined
-
Last visited
Posts posted by GhostSnyperRecon
-
-
Techne is a program that is like a 3d modeling software for minecraft : http://techne.zeux.me/?download
-
its all good, i just needed to look at the brewing stand code a little better, thanks anyway
-
hi guys, ive created a dual input furnace that works and all, but i cant seem to make the shift-clicking into the right slots to work.
here is the code, it will put fuel in the right palce, but only insert an item into one of the input slots. much help appreciated.
package mod.warp.blocks;
import java.util.List;
import cpw.mods.fml.common.Side;
import cpw.mods.fml.common.asm.SideOnly;
import mod.warp.InfuserRecipes;
import net.minecraft.src.Container;
import net.minecraft.src.EntityPlayer;
import net.minecraft.src.FurnaceRecipes;
import net.minecraft.src.ICrafting;
import net.minecraft.src.InventoryPlayer;
import net.minecraft.src.ItemStack;
import net.minecraft.src.Slot;
import net.minecraft.src.SlotFurnace;
import net.minecraft.src.TileEntityFurnace;
// Referenced classes of package net.minecraft.src:
// Container, Slot, SlotFurnace, InventoryPlayer,
// ICrafting, TileEntityFurnace, ItemStack, EntityPlayer
public class ContainerWarpInfuser extends Container
{
private TileEntityWarpInfuser WarpInfuser;
private int CookTime;
private int BurnTime;
private int ItemBurnTime;
public ContainerWarpInfuser(InventoryPlayer inventoryplayer, TileEntityWarpInfuser tileentityWarpInfuser)
{
CookTime = 0;
BurnTime = 0;
ItemBurnTime = 0;
WarpInfuser = tileentityWarpInfuser;
this.addSlotToContainer(new Slot(tileentityWarpInfuser, 0, 44, 17));
this.addSlotToContainer(new Slot(tileentityWarpInfuser, 1, 56, 53));
this.addSlotToContainer(new SlotFurnace(inventoryplayer.player, tileentityWarpInfuser, 2, 116, 35));
this.addSlotToContainer(new Slot(tileentityWarpInfuser, 3, 67, 17));
for(int i = 0; i < 3; i++)
{
for(int k = 0; k < 9; k++)
{
this.addSlotToContainer(new Slot(inventoryplayer, k + i * 9 + 9, 8 + k * 18, 84 + i * 18));
}
}
for(int j = 0; j < 9; j++)
{
this.addSlotToContainer(new Slot(inventoryplayer, j, 8 + j * 18, 142));
}
}
public void updateCraftingResults()
{
super.updateCraftingResults();
for(int i = 0; i < crafters.size(); i++)
{
ICrafting icrafting = (ICrafting)crafters.get(i);
if(CookTime != WarpInfuser.InfuserCookTime)
{
icrafting.updateCraftingInventoryInfo(this, 0, WarpInfuser.InfuserCookTime);
}
if(CookTime != WarpInfuser.InfuserCookTime)
{
icrafting.updateCraftingInventoryInfo(this, 3, WarpInfuser.InfuserCookTime);
}
if(BurnTime != WarpInfuser.InfuserBurnTime)
{
icrafting.updateCraftingInventoryInfo(this, 1, WarpInfuser.InfuserBurnTime);
}
if(ItemBurnTime != WarpInfuser.currentItemBurnTime)
{
icrafting.updateCraftingInventoryInfo(this, 2, WarpInfuser.currentItemBurnTime);
}
}
CookTime = WarpInfuser.InfuserCookTime;
BurnTime = WarpInfuser.InfuserBurnTime;
ItemBurnTime = WarpInfuser.currentItemBurnTime;
}
@SideOnly(Side.CLIENT)
public void updateProgressBar(int par1, int par2)
{
if (par1 == 0)
{
this.WarpInfuser.InfuserCookTime = par2;
}
if (par1 == 1)
{
this.WarpInfuser.InfuserBurnTime = par2;
}
if (par1 == 2)
{
this.WarpInfuser.currentItemBurnTime = par2;
}
}
public boolean canInteractWith(EntityPlayer entityplayer)
{
return WarpInfuser.canInteractWith(entityplayer);
}
private boolean func_28125_a(ItemStack itemstack1, int i, int j, boolean B) {
// TODO Auto-generated method stub
return false;
}
public ItemStack func_82846_b(EntityPlayer par1EntityPlayer, int par2)
{
ItemStack var3 = null;
Slot var4 = (Slot)this.inventorySlots.get(par2);
if (var4 != null && var4.getHasStack())
{
ItemStack var5 = var4.getStack();
var3 = var5.copy();
if (par2 == 2)
{
if (!this.mergeItemStack(var5, 3, 39, true))
{
return null;
}
var4.onSlotChange(var5, var3);
}
else if (par2 != 1 && par2 != 0 && par2 != 3)
{
if (InfuserRecipes.infusing().getInfusingResult(var5) != null)
{
if (!this.mergeItemStack(var5, 0, 1, false))
{
return null;
}
else if (!this.mergeItemStack(var5, 2, 3, false))
{
return null;
}
}
else if (TileEntityWarpInfuser.isItemFuel(var5))
{
if (!this.mergeItemStack(var5, 1, 2, false))
{
return null;
}
}
else if (par2 >= 3 && par2 < 30)
{
if (!this.mergeItemStack(var5, 30, 39, false))
{
return null;
}
}
else if (par2 >= 30 && par2 < 39 && !this.mergeItemStack(var5, 3, 30, false))
{
return null;
}
}
else if (!this.mergeItemStack(var5, 3, 39, false))
{
return null;
}
if (var5.stackSize == 0)
{
var4.putStack((ItemStack)null);
}
else
{
var4.onSlotChanged();
}
if (var5.stackSize == var3.stackSize)
{
return null;
}
var4.func_82870_a(par1EntityPlayer, var5);
}
return var3;
}
}
-
well i found the code in entity item but i dont understand the event thing, any help is much appreciated
-
hi can anyone tel me how i can make an achievement trigger on an itempick up?
-
hi all, im trying to get player gravity in my dimension, however, i dont want to edit any base files, how do i go about this using forge hooks?
-
fo you have caves that generate through custom blocks at all?
-
do you know anyone who could do a tut?
-
hi, ive got a new dimension and all that, but heres something i cant work out. i have a world that is totaly plains, is there i way i can make the world be plains but have the air style of the nether
-
please help me with this, ive got the files done but i cant get it to work
MapGenCavesWarp.java
package mod.warp;
import java.util.Random;
import net.minecraft.src.Block;
import net.minecraft.src.MapGenBase;
import net.minecraft.src.MathHelper;
import net.minecraft.src.World;
public class MapGenCavesWarp extends MapGenBase
{
/**
* Generates a larger initial cave node than usual. Called 25% of the time.
*/
protected void generateLargeCaveNode(long par1, int par3, int par4, byte[] par5ArrayOfByte, double par6, double par8, double par10)
{
this.generateCaveNode(par1, par3, par4, par5ArrayOfByte, par6, par8, par10, 1.0F + this.rand.nextFloat() * 6.0F, 0.0F, 0.0F, -1, -1, 0.5D);
}
/**
* Generates a node in the current cave system recursion tree.
*/
protected void generateCaveNode(long par1, int par3, int par4, byte[] par5ArrayOfByte, double par6, double par8, double par10, float par12, float par13, float par14, int par15, int par16, double par17)
{
double var19 = (double)(par3 * 16 +
;
double var21 = (double)(par4 * 16 +
;
float var23 = 0.0F;
float var24 = 0.0F;
Random var25 = new Random(par1);
if (par16 <= 0)
{
int var26 = this.range * 16 - 16;
par16 = var26 - var25.nextInt(var26 / 4);
}
boolean var54 = false;
if (par15 == -1)
{
par15 = par16 / 2;
var54 = true;
}
int var27 = var25.nextInt(par16 / 2) + par16 / 4;
for (boolean var28 = var25.nextInt(6) == 0; par15 < par16; ++par15)
{
double var29 = 1.5D + (double)(MathHelper.sin((float)par15 * (float)Math.PI / (float)par16) * par12 * 1.0F);
double var31 = var29 * par17;
float var33 = MathHelper.cos(par14);
float var34 = MathHelper.sin(par14);
par6 += (double)(MathHelper.cos(par13) * var33);
par8 += (double)var34;
par10 += (double)(MathHelper.sin(par13) * var33);
if (var28)
{
par14 *= 0.92F;
}
else
{
par14 *= 0.7F;
}
par14 += var24 * 0.1F;
par13 += var23 * 0.1F;
var24 *= 0.9F;
var23 *= 0.75F;
var24 += (var25.nextFloat() - var25.nextFloat()) * var25.nextFloat() * 2.0F;
var23 += (var25.nextFloat() - var25.nextFloat()) * var25.nextFloat() * 4.0F;
if (!var54 && par15 == var27 && par12 > 1.0F && par16 > 0)
{
this.generateCaveNode(var25.nextLong(), par3, par4, par5ArrayOfByte, par6, par8, par10, var25.nextFloat() * 0.5F + 0.5F, par13 - ((float)Math.PI / 2F), par14 / 3.0F, par15, par16, 1.0D);
this.generateCaveNode(var25.nextLong(), par3, par4, par5ArrayOfByte, par6, par8, par10, var25.nextFloat() * 0.5F + 0.5F, par13 + ((float)Math.PI / 2F), par14 / 3.0F, par15, par16, 1.0D);
return;
}
if (var54 || var25.nextInt(4) != 0)
{
double var35 = par6 - var19;
double var37 = par10 - var21;
double var39 = (double)(par16 - par15);
double var41 = (double)(par12 + 2.0F + 16.0F);
if (var35 * var35 + var37 * var37 - var39 * var39 > var41 * var41)
{
return;
}
if (par6 >= var19 - 16.0D - var29 * 2.0D && par10 >= var21 - 16.0D - var29 * 2.0D && par6 <= var19 + 16.0D + var29 * 2.0D && par10 <= var21 + 16.0D + var29 * 2.0D)
{
int var55 = MathHelper.floor_double(par6 - var29) - par3 * 16 - 1;
int var36 = MathHelper.floor_double(par6 + var29) - par3 * 16 + 1;
int var57 = MathHelper.floor_double(par8 - var31) - 1;
int var38 = MathHelper.floor_double(par8 + var31) + 1;
int var56 = MathHelper.floor_double(par10 - var29) - par4 * 16 - 1;
int var40 = MathHelper.floor_double(par10 + var29) - par4 * 16 + 1;
if (var55 < 0)
{
var55 = 0;
}
if (var36 > 16)
{
var36 = 16;
}
if (var57 < 1)
{
var57 = 1;
}
if (var38 > 120)
{
var38 = 120;
}
if (var56 < 0)
{
var56 = 0;
}
if (var40 > 16)
{
var40 = 16;
}
boolean var58 = false;
int var42;
int var45;
for (var42 = var55; !var58 && var42 < var36; ++var42)
{
for (int var43 = var56; !var58 && var43 < var40; ++var43)
{
for (int var44 = var38 + 1; !var58 && var44 >= var57 - 1; --var44)
{
var45 = (var42 * 16 + var43) * 128 + var44;
if (var44 >= 0 && var44 < 128)
{
if (par5ArrayOfByte[var45] == Block.waterMoving.blockID || par5ArrayOfByte[var45] == Block.waterStill.blockID)
{
var58 = true;
}
if (var44 != var57 - 1 && var42 != var55 && var42 != var36 - 1 && var43 != var56 && var43 != var40 - 1)
{
var44 = var57;
}
}
}
}
}
if (!var58)
{
for (var42 = var55; var42 < var36; ++var42)
{
double var59 = ((double)(var42 + par3 * 16) + 0.5D - par6) / var29;
for (var45 = var56; var45 < var40; ++var45)
{
double var46 = ((double)(var45 + par4 * 16) + 0.5D - par10) / var29;
int var48 = (var42 * 16 + var45) * 128 + var38;
boolean var49 = false;
if (var59 * var59 + var46 * var46 < 1.0D)
{
for (int var50 = var38 - 1; var50 >= var57; --var50)
{
double var51 = ((double)var50 + 0.5D - par8) / var31;
if (var51 > -0.7D && var59 * var59 + var51 * var51 + var46 * var46 < 1.0D)
{
byte var53 = par5ArrayOfByte[var48];
if (var53 == WarpCraft.warpStoneBlock.blockID)
{
var49 = true;
}
if (var53 == WarpCraft.warpStoneBlock.blockID || var53 == Block.dirt.blockID || var53 == Block.grass.blockID)
{
if (var50 < 10)
{
par5ArrayOfByte[var48] = (byte)Block.lavaMoving.blockID;
}
else
{
par5ArrayOfByte[var48] = 0;
if (var49 && par5ArrayOfByte[var48 - 1] == Block.dirt.blockID)
{
par5ArrayOfByte[var48 - 1] = this.worldObj.getBiomeGenForCoords(var42 + par3 * 16, var45 + par4 * 16).topBlock;
}
}
}
}
--var48;
}
}
}
}
if (var54)
{
break;
}
}
}
}
}
}
/**
* Recursively called by generate() (generate) and optionally by itself.
*/
protected void recursiveGenerate(World par1World, int par2, int par3, int par4, int par5, byte[] par6ArrayOfByte)
{
int var7 = this.rand.nextInt(this.rand.nextInt(this.rand.nextInt(40) + 1) + 1);
if (this.rand.nextInt(15) != 0)
{
var7 = 0;
}
for (int var8 = 0; var8 < var7; ++var8)
{
double var9 = (double)(par2 * 16 + this.rand.nextInt(16));
double var11 = (double)this.rand.nextInt(this.rand.nextInt(120) +
;
double var13 = (double)(par3 * 16 + this.rand.nextInt(16));
int var15 = 1;
if (this.rand.nextInt(4) == 0)
{
this.generateLargeCaveNode(this.rand.nextLong(), par4, par5, par6ArrayOfByte, var9, var11, var13);
var15 += this.rand.nextInt(4);
}
for (int var16 = 0; var16 < var15; ++var16)
{
float var17 = this.rand.nextFloat() * (float)Math.PI * 2.0F;
float var18 = (this.rand.nextFloat() - 0.5F) * 2.0F / 8.0F;
float var19 = this.rand.nextFloat() * 2.0F + this.rand.nextFloat();
if (this.rand.nextInt(10) == 0)
{
var19 *= this.rand.nextFloat() * this.rand.nextFloat() * 3.0F + 1.0F;
}
this.generateCaveNode(this.rand.nextLong(), par4, par5, par6ArrayOfByte, var9, var11, var13, var19, var17, var18, 0, 0, 1.0D);
}
}
}
}
ChunkProviderWarp.java
package mod.warp;
import java.util.List;
import java.util.Random;
import net.minecraft.src.BiomeGenBase;
import net.minecraft.src.Block;
import net.minecraft.src.BlockSand;
import net.minecraft.src.Chunk;
import net.minecraft.src.ChunkPosition;
import net.minecraft.src.EnumCreatureType;
import net.minecraft.src.IChunkProvider;
import net.minecraft.src.IProgressUpdate;
import net.minecraft.src.MapGenBase;
import net.minecraft.src.MapGenCaves;
import net.minecraft.src.MapGenCavesHell;
import net.minecraft.src.MapGenRavine;
import net.minecraft.src.MapGenScatteredFeature;
import net.minecraft.src.MapGenStronghold;
import net.minecraft.src.MathHelper;
import net.minecraft.src.NoiseGeneratorOctaves;
import net.minecraft.src.SpawnerAnimals;
import net.minecraft.src.World;
import net.minecraft.src.WorldGenDungeons;
import net.minecraft.src.WorldGenFire;
import net.minecraft.src.WorldGenFlowers;
import net.minecraft.src.WorldGenGlowStone1;
import net.minecraft.src.WorldGenHellLava;
import net.minecraft.src.WorldGenLakes;
public class ChunkProviderWarp implements IChunkProvider
{
/** RNG. */
private Random rand;
/** A NoiseGeneratorOctaves used in generating terrain */
private NoiseGeneratorOctaves noiseGen1;
/** A NoiseGeneratorOctaves used in generating terrain */
private NoiseGeneratorOctaves noiseGen2;
/** A NoiseGeneratorOctaves used in generating terrain */
private NoiseGeneratorOctaves noiseGen3;
/** A NoiseGeneratorOctaves used in generating terrain */
private NoiseGeneratorOctaves noiseGen4;
/** A NoiseGeneratorOctaves used in generating terrain */
public NoiseGeneratorOctaves noiseGen5;
/** A NoiseGeneratorOctaves used in generating terrain */
public NoiseGeneratorOctaves noiseGen6;
public NoiseGeneratorOctaves mobSpawnerNoise;
/** Reference to the World object. */
private World worldObj;
/** are map structures going to be generated (e.g. strongholds) */
private final boolean mapFeaturesEnabled;
/** Holds the overall noise array used in chunk generation */
private double[] noiseArray;
private double[] stoneNoise = new double[256];
private MapGenBase caveGenerator = new MapGenCavesWarp();
/** Holds StronghoFld Generator */
private MapGenStronghold strongholdGenerator = new MapGenStronghold();
private MapGenVillagesWarp villageswarpGenerator = new MapGenVillagesWarp(0);
/** Holds Village Generator */
/** Holds Mineshaft Generator */
private MapGenScatteredFeature scatteredFeatureGenerator = new MapGenScatteredFeature();
/** Holds ravine generator */
private MapGenBase ravineGenerator = new MapGenRavine();
/** The biomes that are used to generate the chunk */
private BiomeGenBase[] biomesForGeneration;
/** A double array that hold terrain noise from noiseGen3 */
double[] noise3;
/** A double array that hold terrain noise */
double[] noise1;
/** A double array that hold terrain noise from noiseGen2 */
double[] noise2;
/** A double array that hold terrain noise from noiseGen5 */
double[] noise5;
/** A double array that holds terrain noise from noiseGen6 */
double[] noise6;
/**
* Used to store the 5x5 parabolic field that is used during terrain generation.
*/
float[] parabolicField;
int[][] field_73219_j = new int[32][32];
public ChunkProviderWarp(World par1World, long par2, boolean par4)
{
this.worldObj = par1World;
this.mapFeaturesEnabled = true;
this.rand = new Random(par2);
this.noiseGen1 = new NoiseGeneratorOctaves(this.rand, 16);
this.noiseGen2 = new NoiseGeneratorOctaves(this.rand, 16);
this.noiseGen3 = new NoiseGeneratorOctaves(this.rand,
;
this.noiseGen4 = new NoiseGeneratorOctaves(this.rand, 4);
this.noiseGen5 = new NoiseGeneratorOctaves(this.rand, 10);
this.noiseGen6 = new NoiseGeneratorOctaves(this.rand, 16);
this.mobSpawnerNoise = new NoiseGeneratorOctaves(this.rand,
;
}
/**
* Generates the shape of the terrain for the chunk though its all stone though the water is frozen if the
* temperature is low enough
*/
public void generateTerrain(int par1, int par2, byte[] par3ArrayOfByte)
{
byte var4 = 4;
byte var5 = 16;
byte var6 = 63;
int var7 = var4 + 1;
byte var8 = 17;
int var9 = var4 + 1;
this.biomesForGeneration = this.worldObj.getWorldChunkManager().getBiomesForGeneration(this.biomesForGeneration, par1 * 4 - 2, par2 * 4 - 2, var7 + 5, var9 + 5);
this.noiseArray = this.initializeNoiseField(this.noiseArray, par1 * var4, 0, par2 * var4, var7, var8, var9);
for (int var10 = 0; var10 < var4; ++var10)
{
for (int var11 = 0; var11 < var4; ++var11)
{
for (int var12 = 0; var12 < var5; ++var12)
{
double var13 = 0.125D;
double var15 = this.noiseArray[((var10 + 0) * var9 + var11 + 0) * var8 + var12 + 0];
double var17 = this.noiseArray[((var10 + 0) * var9 + var11 + 1) * var8 + var12 + 0];
double var19 = this.noiseArray[((var10 + 1) * var9 + var11 + 0) * var8 + var12 + 0];
double var21 = this.noiseArray[((var10 + 1) * var9 + var11 + 1) * var8 + var12 + 0];
double var23 = (this.noiseArray[((var10 + 0) * var9 + var11 + 0) * var8 + var12 + 1] - var15) * var13;
double var25 = (this.noiseArray[((var10 + 0) * var9 + var11 + 1) * var8 + var12 + 1] - var17) * var13;
double var27 = (this.noiseArray[((var10 + 1) * var9 + var11 + 0) * var8 + var12 + 1] - var19) * var13;
double var29 = (this.noiseArray[((var10 + 1) * var9 + var11 + 1) * var8 + var12 + 1] - var21) * var13;
for (int var31 = 0; var31 < 8; ++var31)
{
double var32 = 0.25D;
double var34 = var15;
double var36 = var17;
double var38 = (var19 - var15) * var32;
double var40 = (var21 - var17) * var32;
for (int var42 = 0; var42 < 4; ++var42)
{
int var43 = var42 + var10 * 4 << 11 | 0 + var11 * 4 << 7 | var12 * 8 + var31;
short var44 = 128;
var43 -= var44;
double var45 = 0.25D;
double var49 = (var36 - var34) * var45;
double var47 = var34 - var49;
for (int var51 = 0; var51 < 4; ++var51)
{
if ((var47 += var49) > 0.0D)
{
par3ArrayOfByte[var43 += var44] = (byte)WarpCraft.warpStoneBlock.blockID;
}
else if (var12 * 8 + var31 < var6)
{
par3ArrayOfByte[var43 += var44] = (byte)Block.waterStill.blockID;
}
else
{
par3ArrayOfByte[var43 += var44] = 0;
}
}
var34 += var38;
var36 += var40;
}
var15 += var23;
var17 += var25;
var19 += var27;
var21 += var29;
}
}
}
}
}
/**
* Replaces the stone that was placed in with blocks that match the biome
*/
public void replaceBlocksForBiome(int par1, int par2, byte[] par3ArrayOfByte, BiomeGenBase[] par4ArrayOfBiomeGenBase)
{
byte var5 = 63;
double var6 = 0.03125D;
this.stoneNoise = this.noiseGen4.generateNoiseOctaves(this.stoneNoise, par1 * 16, par2 * 16, 0, 16, 16, 1, var6 * 2.0D, var6 * 2.0D, var6 * 2.0D);
for (int var8 = 0; var8 < 16; ++var8)
{
for (int var9 = 0; var9 < 16; ++var9)
{
BiomeGenBase var10 = par4ArrayOfBiomeGenBase[var9 + var8 * 16];
float var11 = var10.getFloatTemperature();
int var12 = (int)(this.stoneNoise[var8 + var9 * 16] / 3.0D + 3.0D + this.rand.nextDouble() * 0.25D);
int var13 = -1;
byte var14 = var10.topBlock;
byte var15 = var10.fillerBlock;
for (int var16 = 127; var16 >= 0; --var16)
{
int var17 = (var9 * 16 + var8) * 128 + var16;
if (var16 <= 0 + this.rand.nextInt(5))
{
par3ArrayOfByte[var17] = (byte)Block.bedrock.blockID;
}
else
{
byte var18 = par3ArrayOfByte[var17];
if (var18 == 0)
{
var13 = -1;
}
else if (var18 != WarpCraft.warpStoneBlock.blockID)
{
if (var13 == -1)
{
if (var12 == 0)
{
var14 = 0;
var15 = (byte)WarpCraft.warpStoneBlock.blockID;
}
else if (var16 >= var5 - 4 && var16 <= var5 + 1)
{
var14 = var10.topBlock;
var15 = var10.fillerBlock;
}
if (var16 < var5 && var14 == 0)
{
if (var11 < 0.15F)
{
var14 = (byte)Block.ice.blockID;
}
else
{
var14 = (byte)Block.waterStill.blockID;
}
}
var13 = var12;
if (var16 >= var5 - 1)
{
par3ArrayOfByte[var17] = var14;
}
else
{
par3ArrayOfByte[var17] = var15;
}
}
else if (var13 > 0)
{
--var13;
par3ArrayOfByte[var17] = var15;
if (var13 == 0 && var15 == Block.sand.blockID)
{
var13 = this.rand.nextInt(4);
var15 = (byte)Block.sandStone.blockID;
}
}
}
}
}
}
}
}
/**
* loads or generates the chunk at the chunk location specified
*/
public Chunk loadChunk(int par1, int par2)
{
return this.provideChunk(par1, par2);
}
/**
* Will return back a chunk, if it doesn't exist and its not a MP client it will generates all the blocks for the
* specified chunk from the map seed and chunk seed
*/
public Chunk provideChunk(int par1, int par2)
{
BlockWarpStone warpstoneblock = WarpCraft.warpStoneBlock;
this.rand.setSeed((long)par1 * 341873128712L + (long)par2 * 132897987541L);
byte[] var3 = new byte[32768];
this.generateTerrain(par1, par2, var3);
this.biomesForGeneration = this.worldObj.getWorldChunkManager().loadBlockGeneratorData(this.biomesForGeneration, par1 * 16, par2 * 16, 16, 16);
this.replaceBlocksForBiome(par1, par2, var3, this.biomesForGeneration);
byte[] warpstoneblock1 = new byte[32768];
this.caveGenerator.generate(this, this.worldObj, par1, par2, warpstoneblock1);
this.ravineGenerator.generate(this, this.worldObj, par1, par2, var3);
if (this.mapFeaturesEnabled)
{
this.villageswarpGenerator.generate(this, this.worldObj, par1, par2, var3);
this.strongholdGenerator.generate(this, this.worldObj, par1, par2, var3);
this.scatteredFeatureGenerator.generate(this, this.worldObj, par1, par2, var3);
}
Chunk var4 = new Chunk(this.worldObj, var3, par1, par2);
byte[] var5 = var4.getBiomeArray();
var4.generateSkylightMap();
return var4;
}
/**
* generates a subset of the level's terrain data. Takes 7 arguments: the [empty] noise array, the position, and the
* size.
*/
private double[] initializeNoiseField(double[] par1ArrayOfDouble, int par2, int par3, int par4, int par5, int par6, int par7)
{
if (par1ArrayOfDouble == null)
{
par1ArrayOfDouble = new double[par5 * par6 * par7];
}
if (this.parabolicField == null)
{
this.parabolicField = new float[25];
for (int var8 = -2; var8 <= 2; ++var8)
{
for (int var9 = -2; var9 <= 2; ++var9)
{
float var10 = 10.0F / MathHelper.sqrt_float((float)(var8 * var8 + var9 * var9) + 0.2F);
this.parabolicField[var8 + 2 + (var9 + 2) * 5] = var10;
}
}
}
double var44 = 684.412D;
double var45 = 684.412D;
this.noise5 = this.noiseGen5.generateNoiseOctaves(this.noise5, par2, par4, par5, par7, 1.121D, 1.121D, 0.5D);
this.noise6 = this.noiseGen6.generateNoiseOctaves(this.noise6, par2, par4, par5, par7, 200.0D, 200.0D, 0.5D);
this.noise3 = this.noiseGen3.generateNoiseOctaves(this.noise3, par2, par3, par4, par5, par6, par7, var44 / 80.0D, var45 / 160.0D, var44 / 80.0D);
this.noise1 = this.noiseGen1.generateNoiseOctaves(this.noise1, par2, par3, par4, par5, par6, par7, var44, var45, var44);
this.noise2 = this.noiseGen2.generateNoiseOctaves(this.noise2, par2, par3, par4, par5, par6, par7, var44, var45, var44);
boolean var43 = false;
boolean var42 = false;
int var12 = 0;
int var13 = 0;
for (int var14 = 0; var14 < par5; ++var14)
{
for (int var15 = 0; var15 < par7; ++var15)
{
float var16 = 0.0F;
float var17 = 0.0F;
float var18 = 0.0F;
byte var19 = 2;
BiomeGenBase var20 = this.biomesForGeneration[var14 + 2 + (var15 + 2) * (par5 + 5)];
for (int var21 = -var19; var21 <= var19; ++var21)
{
for (int var22 = -var19; var22 <= var19; ++var22)
{
BiomeGenBase var23 = this.biomesForGeneration[var14 + var21 + 2 + (var15 + var22 + 2) * (par5 + 5)];
float var24 = this.parabolicField[var21 + 2 + (var22 + 2) * 5] / (var23.minHeight + 2.0F);
if (var23.minHeight > var20.minHeight)
{
var24 /= 2.0F;
}
var16 += var23.maxHeight * var24;
var17 += var23.minHeight * var24;
var18 += var24;
}
}
var16 /= var18;
var17 /= var18;
var16 = var16 * 0.9F + 0.1F;
var17 = (var17 * 4.0F - 1.0F) / 8.0F;
double var47 = this.noise6[var13] / 8000.0D;
if (var47 < 0.0D)
{
var47 = -var47 * 0.3D;
}
var47 = var47 * 3.0D - 2.0D;
if (var47 < 0.0D)
{
var47 /= 2.0D;
if (var47 < -1.0D)
{
var47 = -1.0D;
}
var47 /= 1.4D;
var47 /= 2.0D;
}
else
{
if (var47 > 1.0D)
{
var47 = 1.0D;
}
var47 /= 8.0D;
}
++var13;
for (int var46 = 0; var46 < par6; ++var46)
{
double var48 = (double)var17;
double var26 = (double)var16;
var48 += var47 * 0.2D;
var48 = var48 * (double)par6 / 16.0D;
double var28 = (double)par6 / 2.0D + var48 * 4.0D;
double var30 = 0.0D;
double var32 = ((double)var46 - var28) * 12.0D * 128.0D / 128.0D / var26;
if (var32 < 0.0D)
{
var32 *= 4.0D;
}
double var34 = this.noise1[var12] / 512.0D;
double var36 = this.noise2[var12] / 512.0D;
double var38 = (this.noise3[var12] / 10.0D + 1.0D) / 2.0D;
if (var38 < 0.0D)
{
var30 = var34;
}
else if (var38 > 1.0D)
{
var30 = var36;
}
else
{
var30 = var34 + (var36 - var34) * var38;
}
var30 -= var32;
if (var46 > par6 - 4)
{
double var40 = (double)((float)(var46 - (par6 - 4)) / 3.0F);
var30 = var30 * (1.0D - var40) + -10.0D * var40;
}
par1ArrayOfDouble[var12] = var30;
++var12;
}
}
}
return par1ArrayOfDouble;
}
/**
* Checks to see if a chunk exists at x, y
*/
public boolean chunkExists(int par1, int par2)
{
return true;
}
/**
* Populates chunk with ores etc etc
*/
public void populate(IChunkProvider par1IChunkProvider, int par2, int par3)
{
BlockSand.fallInstantly = true;
int var4 = par2 * 16;
int var5 = par3 * 16;
BiomeGenBase var6 = this.worldObj.getBiomeGenForCoords(var4 + 16, var5 + 16);
this.rand.setSeed(this.worldObj.getSeed());
long var7 = this.rand.nextLong() / 2L * 2L + 1L;
long var9 = this.rand.nextLong() / 2L * 2L + 1L;
this.rand.setSeed((long)par2 * var7 + (long)par3 * var9 ^ this.worldObj.getSeed());
boolean var11 = false;
if (this.mapFeaturesEnabled)
{
this.villageswarpGenerator.generateStructuresInChunk(this.worldObj, this.rand, par2, par3);
this.strongholdGenerator.generateStructuresInChunk(this.worldObj, this.rand, par2, par3);
this.scatteredFeatureGenerator.generateStructuresInChunk(this.worldObj, this.rand, par2, par3);
}
int var12;
int var13;
int var14;
if (!var11 && this.rand.nextInt(4) == 0)
{
var12 = var4 + this.rand.nextInt(16) + 8;
var13 = this.rand.nextInt(128);
var14 = var5 + this.rand.nextInt(16) + 8;
(new WorldGenLakes(Block.waterStill.blockID)).generate(this.worldObj, this.rand, var12, var13, var14);
}
if (!var11 && this.rand.nextInt(
== 0)
{
var12 = var4 + this.rand.nextInt(16) + 8;
var13 = this.rand.nextInt(this.rand.nextInt(120) +
;
var14 = var5 + this.rand.nextInt(16) + 8;
if (var13 < 63 || this.rand.nextInt(10) == 0)
{
(new WorldGenLakes(Block.waterStill.blockID)).generate(this.worldObj, this.rand, var12, var13, var14);
}
}
for (var12 = 0; var12 < 8; ++var12)
{
var13 = var4 + this.rand.nextInt(16) + 8;
var14 = this.rand.nextInt(128);
int var15 = var5 + this.rand.nextInt(16) + 8;
if ((new WorldGenDungeons()).generate(this.worldObj, this.rand, var13, var14, var15))
{
;
}
}
var6.decorate(this.worldObj, this.rand, var4, var5);
SpawnerAnimals.performWorldGenSpawning(this.worldObj, var6, var4 + 8, var5 + 8, 16, 16, this.rand);
var4 += 8;
var5 += 8;
for (var12 = 0; var12 < 16; ++var12)
{
for (var13 = 0; var13 < 16; ++var13)
{
var14 = this.worldObj.getPrecipitationHeight(var4 + var12, var5 + var13);
if (this.worldObj.isBlockFreezable(var12 + var4, var14 - 1, var13 + var5))
{
this.worldObj.setBlockWithNotify(var12 + var4, var14 - 1, var13 + var5, Block.ice.blockID);
}
if (this.worldObj.canSnowAt(var12 + var4, var14, var13 + var5))
{
this.worldObj.setBlockWithNotify(var12 + var4, var14, var13 + var5, Block.snow.blockID);
}
}
}
if (this.rand.nextInt(1) == 0)
{
var12 = var4 + this.rand.nextInt(16) + 8;
var13 = this.rand.nextInt(128);
var14 = var5 + this.rand.nextInt(16) + 8;
(new WorldGenWarpBush()).generate(this.worldObj, this.rand, var12, var13, var14);
}
WorldGenTreesWarp worldgentreeswarp = new WorldGenTreesWarp();
{
var12 = var4 + this.rand.nextInt(16) + 8;
var14 = var5 + this.rand.nextInt(16) + 8;
var13 = this.rand.nextInt(128);
worldgentreeswarp.generate(this.worldObj, this.rand, var12, var13, var14);
}
BlockSand.fallInstantly = false;
}
/**
* Two modes of operation: if passed true, save all Chunks in one go. If passed false, save up to two chunks.
* Return true if all chunks have been saved.
*/
public boolean saveChunks(boolean par1, IProgressUpdate par2IProgressUpdate)
{
return true;
}
/**
* Unloads the 100 oldest chunks from memory, due to a bug with chunkSet.add() never being called it thinks the list
* is always empty and will not remove any chunks.
*/
public boolean unload100OldestChunks()
{
return false;
}
/**
* Returns if the IChunkProvider supports saving.
*/
public boolean canSave()
{
return true;
}
/**
* Converts the instance data to a readable string.
*/
public String makeString()
{
return "RandomLevelSource";
}
/**
* Returns a list of creatures of the specified type that can spawn at the given location.
*/
public List getPossibleCreatures(EnumCreatureType par1EnumCreatureType, int par2, int par3, int par4)
{
BiomeGenBase var5 = this.worldObj.getBiomeGenForCoords(par2, par4);
return var5 == null ? null : var5.getSpawnableList(par1EnumCreatureType);
}
/**
* Returns the location of the closest structure of the specified type. If not found returns null.
*/
public ChunkPosition findClosestStructure(World par1World, String par2Str, int par3, int par4, int par5)
{
return "Stronghold".equals(par2Str) && this.strongholdGenerator != null ? this.strongholdGenerator.getNearestInstance(par1World, par3, par4, par5) : null;
}
public int getLoadedChunkCount()
{
return 16;
}
}
-
i want to get caves to spawn in my custom dimension but they wont, any help?
-
thanks, ive got it working now
-
could you post the main file and the custom leaves file so i can actually see what ive done wrong?
-
how would one go about registering a tick handler? im new to crreating stuff like this so i have no idea what im doing.
-
do i need to add something to my mainmodfile.java? if i do then im an idiot
package mod.warp;
import java.util.Map;
import java.util.logging.Level;
import net.minecraft.src.BiomeDecorator;
import net.minecraft.src.BiomeGenBase;
import net.minecraft.src.BiomeGenPlains;
import net.minecraft.src.Block;
import net.minecraft.src.BlockFire;
import net.minecraft.src.DimensionAPI;
import net.minecraft.src.EnumCreatureType;
import net.minecraft.src.EnumToolMaterial;
import net.minecraft.src.Item;
import net.minecraft.src.ItemBlock;
import net.minecraft.src.ItemStack;
import net.minecraft.src.Material;
import net.minecraft.src.WorldProvider;
import net.minecraftforge.client.MinecraftForgeClient;
import net.minecraftforge.common.Configuration;
import net.minecraftforge.common.DimensionManager;
import cpw.mods.fml.common.FMLCommonHandler;
import cpw.mods.fml.common.FMLLog;
import cpw.mods.fml.common.Mod;
import cpw.mods.fml.common.Mod.Init;
import cpw.mods.fml.common.Mod.Instance;
import cpw.mods.fml.common.Mod.PostInit;
import cpw.mods.fml.common.Mod.PreInit;
import cpw.mods.fml.common.SidedProxy;
import cpw.mods.fml.common.event.FMLInitializationEvent;
import cpw.mods.fml.common.event.FMLPostInitializationEvent;
import cpw.mods.fml.common.event.FMLPreInitializationEvent;
import cpw.mods.fml.common.network.NetworkMod;
import cpw.mods.fml.common.registry.EntityRegistry;
import cpw.mods.fml.common.registry.GameRegistry;
import cpw.mods.fml.common.registry.LanguageRegistry;
import java.awt.Color;
@Mod(modid = "WarpCraft", name = "WarpCraft", version = "In-Dev 1.0")
@NetworkMod(
channels = { "WarpCraft" },
clientSideRequired = true,
serverSideRequired = false,
packetHandler = PacketHandler.class )
public class WarpCraft
{
@Instance
public static WarpCraft instance;
@SidedProxy(clientSide = "mod.warp.ClientProxy", serverSide = "mod.warp.CommonProxy")
public static CommonProxy proxy;
//Dimension
public static BiomeGenWarp warp;
//Blocks
public static BlockWarpOre warpOreBlock;
public static BlockWarpBlock warpBlockBlock;
public static BlockWarpLight warpLight;
public static BlockWarpLightON warpLightON;
public static BlockWarpCorupt warpCoruptBlock;
public static BlockPortalWarp warpPortal;
public static BlockWarpFrame warpFrame;
public static BlockWarpFire warpFire;
public static BlockWarpStone warpStoneBlock;
public static BlockWarpBush warpBushBlock;
public static BlockWarpCoalOre warpCOBlock;
public static BlockWarpLog warpLogBlock;
public static BlockWarpLeaves warpLeavesBlock;
//Items
public static ItemWarpDust warpDustItem;
public static ItemWarpIngot warpIngotItem;
public static ItemWarpPaxel warpPaxelItem;
public static ItemWarpCDust warpCDustItem;
public static ItemWarpFS warpFSItem;
public static ItemWarpBerry warpBerryItem;
public static ItemWarpCoal warpCoalItem;
//Config Values
// Blocks
private int bWarpOreID;
private int bWarpBlockID;
private int bWarpLightID;
private int bWarpLightONID;
private int bWarpCoruptBlockID;
private int bWarpPortalID;
private int bWarpFrameID;
private int bWarpFireID;
private int bWarpStoneID;
private int bWarpBushID;
private int bWarpCOID;
private int bWarpLogID;
private int bWarpLeavesID;
// Items
private int iWarpDustID;
private int iWarpIngotID;
private int iWarpPaxelID;
private int iWarpCDustID;
private int iWarpFSID;
private int iWarpBerryID;
private int iWarpCoalID;
@PreInit
public void preInit(FMLPreInitializationEvent event)
{
// Loading in Configuration Data
Configuration cfg = new Configuration(event.getSuggestedConfigurationFile());
try {
cfg.load();
// Load Block IDs
bWarpOreID = cfg.getOrCreateBlockIdProperty("Warp Ore", 140).getInt(140);
bWarpBlockID = cfg.getOrCreateBlockIdProperty("Warp Block", 141).getInt(141);
bWarpLightID = cfg.getOrCreateBlockIdProperty("Warp Lamp", 142).getInt(142);
bWarpLightONID = cfg.getOrCreateBlockIdProperty("Warp LampON", 143).getInt(143);
bWarpCoruptBlockID = cfg.getOrCreateBlockIdProperty("Corupt Warp Ore", 144).getInt(144);
bWarpPortalID = cfg.getOrCreateBlockIdProperty("Warp Portal", 145).getInt(145);
bWarpFrameID = cfg.getOrCreateBlockIdProperty("Warp Portal Frame", 146).getInt(146);
bWarpFireID = cfg.getOrCreateBlockIdProperty("Warp Fire", 147).getInt(147);
bWarpStoneID = cfg.getOrCreateBlockIdProperty("Warp Stone", 148).getInt(148);
bWarpBushID = cfg.getOrCreateBlockIdProperty("Warp Bush", 149).getInt(149);
bWarpCOID = cfg.getOrCreateBlockIdProperty("Warp Coal Ore", 150).getInt(150);
bWarpLogID = cfg.getOrCreateBlockIdProperty("Infused Log", 151).getInt(151);
bWarpLeavesID = cfg.getOrCreateBlockIdProperty("Infused Leaves", 152).getInt(152);
// Load Item IDs
iWarpDustID = cfg.getOrCreateIntProperty("Warp Dust", Configuration.CATEGORY_ITEM, 400).getInt(400);
iWarpIngotID = cfg.getOrCreateIntProperty("Warp Ingot", Configuration.CATEGORY_ITEM, 401).getInt(401);
iWarpPaxelID = cfg.getOrCreateIntProperty("Warp Paxel", Configuration.CATEGORY_ITEM, 402).getInt(402);
iWarpCDustID = cfg.getOrCreateIntProperty("Corupt Warp Dust", Configuration.CATEGORY_ITEM, 403).getInt(403);
iWarpFSID = cfg.getOrCreateIntProperty("Warp Lighter", Configuration.CATEGORY_ITEM, 404).getInt(404);
iWarpBerryID = cfg.getOrCreateIntProperty("Warp Berry", Configuration.CATEGORY_ITEM, 405).getInt(405);
iWarpCoalID = cfg.getOrCreateIntProperty("Warp Coal", Configuration.CATEGORY_ITEM, 406).getInt(406);
} catch (Exception e) {
FMLLog.log(Level.SEVERE, e, "WarpCraft's configuration failed to load.");
} finally {
cfg.save();
}
}
@Init
public void init(FMLInitializationEvent evt)
{
// Entity
int redColor = (35 << 16)+ (35 <<
;
int orangeColor = (107 << 16)+ (68 <<
;
LanguageRegistry.instance().addStringLocalization("entity.Rat.name", "en_US", "Rat");
EntityRegistry.registerGlobalEntityID(EntityRat.class, "Rat", EntityRegistry.findGlobalUniqueEntityId(), redColor, orangeColor);
// Initialize Blocks
warpOreBlock = new BlockWarpOre(bWarpOreID);
warpBlockBlock = new BlockWarpBlock(bWarpBlockID);
warpLight = new BlockWarpLight(bWarpLightID);
warpLightON = new BlockWarpLightON(bWarpLightONID);
warpCoruptBlock = new BlockWarpCorupt(bWarpCoruptBlockID);
warpPortal = new BlockPortalWarp(bWarpPortalID);
warpFrame = new BlockWarpFrame(bWarpFrameID);
warpFire = new BlockWarpFire(bWarpFireID, bWarpBlockID);
warpStoneBlock = new BlockWarpStone(bWarpStoneID);
warpBushBlock = new BlockWarpBush(bWarpBushID);
warpCOBlock = new BlockWarpCoalOre(bWarpCOID);
warpLogBlock = new BlockWarpLog(bWarpLogID);
warpLeavesBlock = new BlockWarpLeaves(bWarpLeavesID, 0);
// Initialize Items
warpDustItem = (ItemWarpDust) new ItemWarpDust(iWarpDustID).setIconIndex(0).setItemName("Warp Dust");
warpIngotItem = (ItemWarpIngot) new ItemWarpIngot(iWarpIngotID).setIconIndex(1).setItemName("Warp Ingot");
warpPaxelItem = (ItemWarpPaxel) new ItemWarpPaxel(iWarpPaxelID, EnumToolMaterial.EMERALD).setIconIndex(2).setItemName("Warp Paxel");
warpCDustItem = (ItemWarpCDust) new ItemWarpCDust(iWarpCDustID).setIconIndex(3).setItemName("Corupt Warp Dust");
warpFSItem = (ItemWarpFS) new ItemWarpFS(iWarpFSID).setIconIndex(4).setItemName("Warp Lighter");
warpBerryItem = (ItemWarpBerry) new ItemWarpBerry(iWarpBerryID, 1, true).setIconIndex(5).setItemName("Warp Berry");
warpCoalItem = (ItemWarpCoal) new ItemWarpCoal(iWarpCoalID).setIconIndex(6).setItemName("Warp Coal");
// Entity
// Register Blocks
GameRegistry.registerBlock(warpOreBlock);
GameRegistry.registerBlock(warpBlockBlock);
GameRegistry.registerBlock(warpLight);
GameRegistry.registerBlock(warpLightON);
GameRegistry.registerBlock(warpCoruptBlock);
GameRegistry.registerBlock(warpPortal);
GameRegistry.registerBlock(warpFrame);
GameRegistry.registerBlock(warpFire);
GameRegistry.registerBlock(warpStoneBlock);
GameRegistry.registerBlock(warpBushBlock);
GameRegistry.registerBlock(warpCOBlock);
GameRegistry.registerBlock(warpLogBlock);
GameRegistry.registerBlock(warpLeavesBlock);
// Add Localization Data
// Blocks
LanguageRegistry.instance().addStringLocalization(warpOreBlock.getBlockName() + ".name", "en_US", "Warp Ore");
LanguageRegistry.instance().addStringLocalization(warpBlockBlock.getBlockName() + ".name", "en_US", "Warp Block");
LanguageRegistry.instance().addStringLocalization(warpLight.getBlockName() + ".name", "en_US", "Warp Lamp");
LanguageRegistry.instance().addStringLocalization(warpLightON.getBlockName() + ".name", "en_US", "Warp Lamp");
LanguageRegistry.instance().addStringLocalization(warpCoruptBlock.getBlockName() + ".name", "en_US", "Corupt Warp Ore");
LanguageRegistry.instance().addStringLocalization(warpPortal.getBlockName() + ".name", "en_US", "Warp Portal");
LanguageRegistry.instance().addStringLocalization(warpFrame.getBlockName() + ".name", "en_US", "Warp Portal Frame");
LanguageRegistry.instance().addStringLocalization(warpFire.getBlockName() + ".name", "en_US", "Warp Fire");
LanguageRegistry.instance().addStringLocalization(warpStoneBlock.getBlockName() + ".name", "en_US", "Warp Stone");
LanguageRegistry.instance().addStringLocalization(warpBushBlock.getBlockName() + ".name", "en_US", "Warp Bush");
LanguageRegistry.instance().addStringLocalization(warpCOBlock.getBlockName() + ".name", "en_US", "Warp Coal Ore");
LanguageRegistry.instance().addStringLocalization(warpLogBlock.getBlockName() + ".name", "en_US", "Infused Log");
LanguageRegistry.instance().addStringLocalization(warpLeavesBlock.getBlockName() + ".name", "en_US", "Infused Leaves");
// Items
LanguageRegistry.instance().addStringLocalization(warpDustItem.getItemName() + ".name", "en_US", "Warp Dust");
LanguageRegistry.instance().addStringLocalization(warpIngotItem.getItemName() + ".name", "en_US", "Warp Ingot");
LanguageRegistry.instance().addStringLocalization(warpPaxelItem.getItemName() + ".name", "en_US", "Warp Paxel");
LanguageRegistry.instance().addStringLocalization(warpCDustItem.getItemName() + ".name", "en_US", "Corupt Warp Dust");
LanguageRegistry.instance().addStringLocalization(warpFSItem.getItemName() + ".name", "en_US", "Warp Lighter");
LanguageRegistry.instance().addStringLocalization(warpBerryItem.getItemName() + ".name", "en_US", "Warp Berry");
LanguageRegistry.instance().addStringLocalization(warpCoalItem.getItemName() + ".name", "en_US", "Warp Coal");
// Entity
// Register Recipes
// Block Recipes
GameRegistry.addRecipe(new ItemStack(warpBlockBlock, 1),
"xx",
"xx",
'x', warpDustItem
);
GameRegistry.addRecipe(new ItemStack(warpLight, 1),
" x ",
"xyx",
" x ",
'x', Block.fenceIron,
'y', warpDustItem
);
GameRegistry.addRecipe(new ItemStack(warpFrame, 4),
" x ",
"xyx",
" x ",
'x', Block.obsidian,
'y', warpDustItem
);
// Item Recipes
GameRegistry.addRecipe(new ItemStack(warpPaxelItem, 1),
"xxx",
"xyx",
"xyx",
'x', warpIngotItem,
'y', Item.stick
);
GameRegistry.addRecipe(new ItemStack(warpDustItem, 4),
"x",
'x', warpBlockBlock
);
GameRegistry.addRecipe(new ItemStack(warpFSItem, 1),
"xyx",
"xzx",
"xxx",
'x', Item.ingotIron,
'y', Item.flint,
'z', warpIngotItem
);
// Fuel
GameRegistry.registerFuelHandler(new WarpFuel());
// Register Smelting
GameRegistry.addSmelting(warpDustItem.shiftedIndex, new ItemStack(warpIngotItem), 0.1F);
// Ore Generation
GameRegistry.registerWorldGenerator(new WorldGeneratorWarpCraft());
GameRegistry.addBiome(warp = new BiomeGenWarp(65));
// Register Rendering Information
proxy.registerRenderInformation();
// Register Dimension
DimensionAPI.registerDimension(new WorldProviderWarp());
// Entities
}
@PostInit
public void postInit(FMLPostInitializationEvent evt)
{
// TODO: Add Post-Initialization code such as mod hooks
}
}
-
i added it and i still dont get any texture changes, anybody willing to post a custom leaves code with working fancy / fast textures that i could have a look at?
-
even when its liek this
public void setGraphicsLevel(boolean par1)
{
this.graphicsLevel = par1;
this.blockIndexInTexture = this.baseIndexInPNG + (par1 ? 0 : 1);
}
it only give me one texture
-
i have tried using that but i cant seem to get it to work, heres the code
package mod.warp;
import cpw.mods.fml.common.Side;
import cpw.mods.fml.common.asm.SideOnly;
import java.util.ArrayList;
import java.util.List;
import java.util.Random;
import net.minecraft.src.Block;
import net.minecraft.src.BlockLeavesBase;
import net.minecraft.src.ColorizerFoliage;
import net.minecraft.src.CreativeTabs;
import net.minecraft.src.EntityPlayer;
import net.minecraft.src.IBlockAccess;
import net.minecraft.src.Item;
import net.minecraft.src.ItemStack;
import net.minecraft.src.Material;
import net.minecraft.src.World;
import net.minecraftforge.common.IShearable;
public class BlockWarpLeaves extends BlockLeavesBase implements IShearable
{
/**
* The base index in terrain.png corresponding to the fancy version of the leaf texture. This is stored so we can
* switch the displayed version between fancy and fast graphics (fast is this index + 1).
*/
private int baseIndexInPNG;
int[] adjacentTreeBlocks;
protected BlockWarpLeaves(int par1, int par2)
{
super(par1, par2, Material.leaves, false);
this.baseIndexInPNG = par2;
this.setTickRandomly(true);
blockIndexInTexture = 9;
setBlockName("Infused Leaves");
this.setCreativeTab(CreativeTabs.tabDeco);
}
/**
* ejects contained items into the world, and notifies neighbours of an update, as appropriate
*/
public void breakBlock(World par1World, int par2, int par3, int par4, int par5, int par6)
{
byte var7 = 1;
int var8 = var7 + 1;
if (par1World.checkChunksExist(par2 - var8, par3 - var8, par4 - var8, par2 + var8, par3 + var8, par4 + var8))
{
for (int var9 = -var7; var9 <= var7; ++var9)
{
for (int var10 = -var7; var10 <= var7; ++var10)
{
for (int var11 = -var7; var11 <= var7; ++var11)
{
int var12 = par1World.getBlockId(par2 + var9, par3 + var10, par4 + var11);
if (Block.blocksList[var12] != null)
{
Block.blocksList[var12].beginLeavesDecay(par1World, par2 + var9, par3 + var10, par4 + var11);
}
}
}
}
}
}
/**
* Ticks the block if it's been scheduled
*/
public void updateTick(World par1World, int par2, int par3, int par4, Random par5Random)
{
if (!par1World.isRemote)
{
int var6 = par1World.getBlockMetadata(par2, par3, par4);
if ((var6 &
!= 0 && (var6 & 4) == 0)
{
byte var7 = 4;
int var8 = var7 + 1;
byte var9 = 32;
int var10 = var9 * var9;
int var11 = var9 / 2;
if (this.adjacentTreeBlocks == null)
{
this.adjacentTreeBlocks = new int[var9 * var9 * var9];
}
int var12;
if (par1World.checkChunksExist(par2 - var8, par3 - var8, par4 - var8, par2 + var8, par3 + var8, par4 + var8))
{
int var13;
int var14;
int var15;
for (var12 = -var7; var12 <= var7; ++var12)
{
for (var13 = -var7; var13 <= var7; ++var13)
{
for (var14 = -var7; var14 <= var7; ++var14)
{
var15 = par1World.getBlockId(par2 + var12, par3 + var13, par4 + var14);
Block block = Block.blocksList[var15];
if (block != null && block.canSustainLeaves(par1World, par2 + var12, par3 + var13, par4 + var14))
{
this.adjacentTreeBlocks[(var12 + var11) * var10 + (var13 + var11) * var9 + var14 + var11] = 0;
}
else if (block != null && block.isLeaves(par1World, par2 + var12, par3 + var13, par4 + var14))
{
this.adjacentTreeBlocks[(var12 + var11) * var10 + (var13 + var11) * var9 + var14 + var11] = -2;
}
else
{
this.adjacentTreeBlocks[(var12 + var11) * var10 + (var13 + var11) * var9 + var14 + var11] = -1;
}
}
}
}
for (var12 = 1; var12 <= 4; ++var12)
{
for (var13 = -var7; var13 <= var7; ++var13)
{
for (var14 = -var7; var14 <= var7; ++var14)
{
for (var15 = -var7; var15 <= var7; ++var15)
{
if (this.adjacentTreeBlocks[(var13 + var11) * var10 + (var14 + var11) * var9 + var15 + var11] == var12 - 1)
{
if (this.adjacentTreeBlocks[(var13 + var11 - 1) * var10 + (var14 + var11) * var9 + var15 + var11] == -2)
{
this.adjacentTreeBlocks[(var13 + var11 - 1) * var10 + (var14 + var11) * var9 + var15 + var11] = var12;
}
if (this.adjacentTreeBlocks[(var13 + var11 + 1) * var10 + (var14 + var11) * var9 + var15 + var11] == -2)
{
this.adjacentTreeBlocks[(var13 + var11 + 1) * var10 + (var14 + var11) * var9 + var15 + var11] = var12;
}
if (this.adjacentTreeBlocks[(var13 + var11) * var10 + (var14 + var11 - 1) * var9 + var15 + var11] == -2)
{
this.adjacentTreeBlocks[(var13 + var11) * var10 + (var14 + var11 - 1) * var9 + var15 + var11] = var12;
}
if (this.adjacentTreeBlocks[(var13 + var11) * var10 + (var14 + var11 + 1) * var9 + var15 + var11] == -2)
{
this.adjacentTreeBlocks[(var13 + var11) * var10 + (var14 + var11 + 1) * var9 + var15 + var11] = var12;
}
if (this.adjacentTreeBlocks[(var13 + var11) * var10 + (var14 + var11) * var9 + (var15 + var11 - 1)] == -2)
{
this.adjacentTreeBlocks[(var13 + var11) * var10 + (var14 + var11) * var9 + (var15 + var11 - 1)] = var12;
}
if (this.adjacentTreeBlocks[(var13 + var11) * var10 + (var14 + var11) * var9 + var15 + var11 + 1] == -2)
{
this.adjacentTreeBlocks[(var13 + var11) * var10 + (var14 + var11) * var9 + var15 + var11 + 1] = var12;
}
}
}
}
}
}
}
var12 = this.adjacentTreeBlocks[var11 * var10 + var11 * var9 + var11];
if (var12 >= 0)
{
par1World.setBlockMetadata(par2, par3, par4, var6 & -9);
}
else
{
this.removeLeaves(par1World, par2, par3, par4);
}
}
}
}
@SideOnly(Side.CLIENT)
/**
* A randomly called display update to be able to add particles or other items for display
*/
public void randomDisplayTick(World par1World, int par2, int par3, int par4, Random par5Random)
{
if (par1World.canLightningStrikeAt(par2, par3 + 1, par4) && !par1World.doesBlockHaveSolidTopSurface(par2, par3 - 1, par4) && par5Random.nextInt(15) == 1)
{
double var6 = (double)((float)par2 + par5Random.nextFloat());
double var8 = (double)par3 - 0.05D;
double var10 = (double)((float)par4 + par5Random.nextFloat());
par1World.spawnParticle("dripWater", var6, var8, var10, 0.0D, 0.0D, 0.0D);
}
}
private void removeLeaves(World par1World, int par2, int par3, int par4)
{
this.dropBlockAsItem(par1World, par2, par3, par4, par1World.getBlockMetadata(par2, par3, par4), 0);
par1World.setBlockWithNotify(par2, par3, par4, 0);
}
/**
* Returns the quantity of items to drop on block destruction.
*/
public int quantityDropped(Random par1Random)
{
return par1Random.nextInt(20) == 0 ? 1 : 0;
}
/**
* Returns the ID of the items to drop on destruction.
*/
public int idDropped(int par1, Random par2Random, int par3)
{
return Block.sapling.blockID;
}
/**
* Drops the block items with a specified chance of dropping the specified items
*/
public void dropBlockAsItemWithChance(World par1World, int par2, int par3, int par4, int par5, float par6, int par7)
{
if (!par1World.isRemote)
{
byte var8 = 20;
if ((par5 & 3) == 3)
{
var8 = 40;
}
if (par1World.rand.nextInt(var8) == 0)
{
int var9 = this.idDropped(par5, par1World.rand, par7);
this.dropBlockAsItem_do(par1World, par2, par3, par4, new ItemStack(var9, 1, this.damageDropped(par5)));
}
if ((par5 & 3) == 0 && par1World.rand.nextInt(200) == 0)
{
this.dropBlockAsItem_do(par1World, par2, par3, par4, new ItemStack(Item.appleRed, 1, 0));
}
}
}
/**
* Called when the player destroys a block with an item that can harvest it. (i, j, k) are the coordinates of the
* block and l is the block's subtype/damage.
*/
public void harvestBlock(World par1World, EntityPlayer par2EntityPlayer, int par3, int par4, int par5, int par6)
{
super.harvestBlock(par1World, par2EntityPlayer, par3, par4, par5, par6);
}
/**
* Determines the damage on the item the block drops. Used in cloth and wood.
*/
protected int damageDropped(int par1)
{
return par1 & 3;
}
/**
* Is this block (a) opaque and (b) a full 1m cube? This determines whether or not to render the shared face of two
* adjacent blocks and also whether the player can attach torches, redstone wire, etc to this block.
*/
public boolean isOpaqueCube()
{
return !this.graphicsLevel;
}
public int getRenderBlockPass()
{
return 0;
}
@SideOnly(Side.CLIENT)
/**
* Pass true to draw this block using fancy graphics, or false for fast graphics.
*/
public void setGraphicsLevel(boolean par1)
{
this.graphicsLevel = par1;
this.blockIndexInTexture = this.baseIndexInPNG + 1;
}
@Override
public boolean isShearable(ItemStack item, World world, int x, int y, int z)
{
return true;
}
@Override
public ArrayList<ItemStack> onSheared(ItemStack item, World world, int x, int y, int z, int fortune)
{
ArrayList<ItemStack> ret = new ArrayList<ItemStack>();
ret.add(new ItemStack(this, 1, world.getBlockMetadata(x, y, z) & 3));
return ret;
}
@Override
public void beginLeavesDecay(World world, int x, int y, int z)
{
world.setBlockMetadata(x, y, z, world.getBlockMetadata(x, y, z) |
;
}
@Override
public boolean isLeaves(World world, int x, int y, int z)
{
return true;
}
@Override
public String getTextureFile() {
return "/warpcraft/blocks/Blocks.png";
}
}
-
hi, im creating some trees for a new dimension, and ive come to a texture issue, i cant work out how to make the graphics work with fancy and fast variations, any help?
-
hi, ive managed to create a dimension, new biome etc, however, i want the new biome to only spawn in the dimension, not the overworld, can anyone help with this, thanks
-
also if its any help i want the fire to be green, but ive been trying for about a day with no luck
-
hi, can anyone help me to change the colour of my custom fire to green instead of the default fire texture?
Mob Spawn Item
in Modder Support
Posted
right, ive got this item which spawns in a mob, however, i want to know, is there a way i can spawn the mob in already tamed?