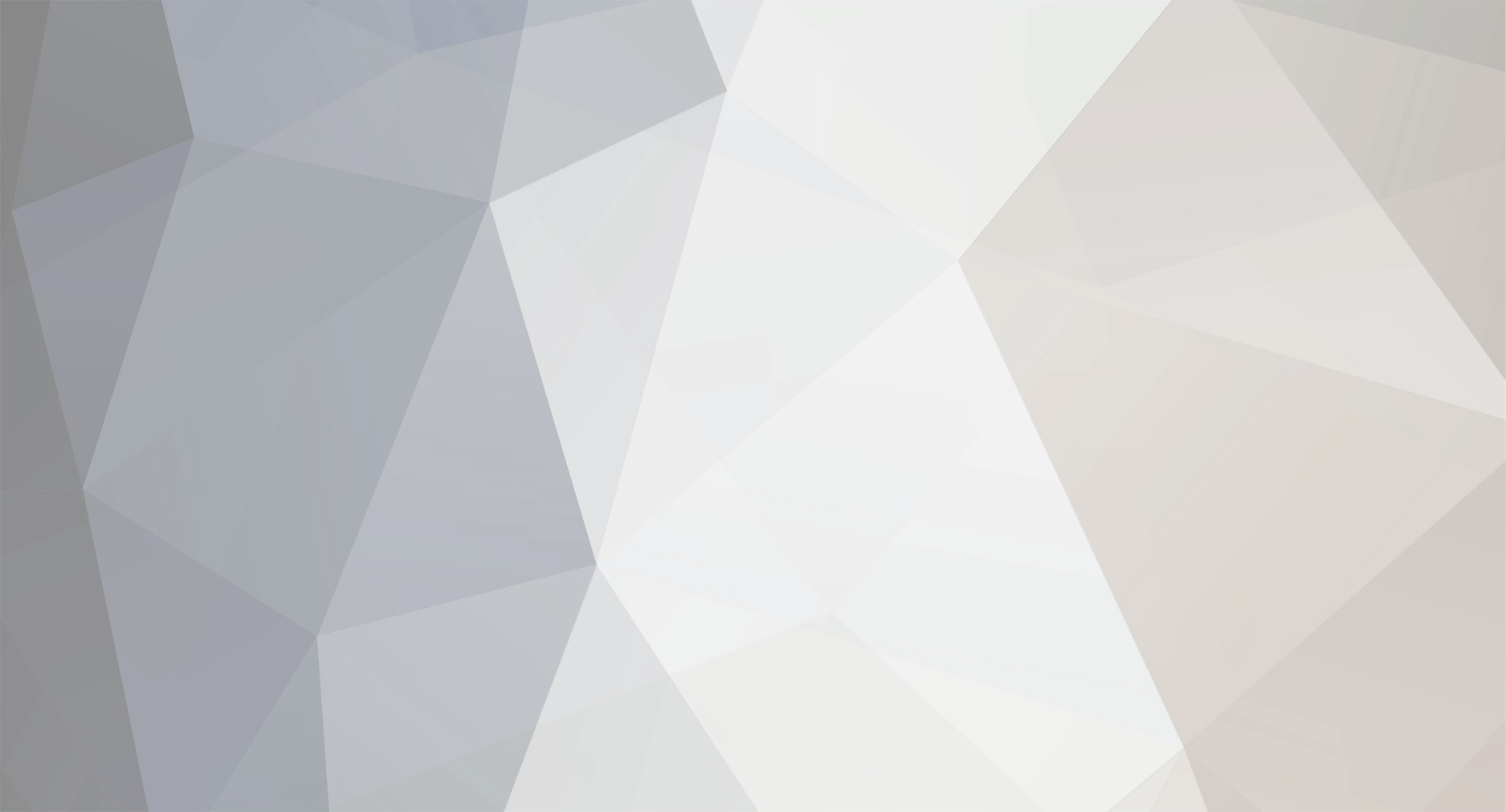
Earthcomputer
Forge Modder-
Posts
114 -
Joined
-
Last visited
Everything posted by Earthcomputer
-
[1.9] Assistance required in handling chest opening interactions
Earthcomputer replied to baegmon's topic in Modder Support
I suggest using the tile entity NBT data. If you're using your own custom chests (which is really the best option), override various methods in the block class instead of using evebts., store the player UUID in the tile entity and override readFromNBT and writeToNBT. If you're working with vanilla chests, you will have to use events. You may have to store the custom player UUID per-chunk like how diesieben07 said as well. -
[1.9] Use the vainilla spawner in custom structure
Earthcomputer replied to perromercenary00's topic in Modder Support
In addition to the comments above, you should always do an instanceof check before casting after world.getTileEntity. You cannot guarantee that this tile entity is an instance of TileEntityMobSpawner, and if it isn't, the game will crash. -
May I suggest that a recipe like this is registered after other mods register their recipes? In the postInit phase for example. Otherwise this recipe would override other recipes which may make items unobtainable is survival.
-
A simple way to go would be something like: if (!stack.hasTagCompound()) stack.stackTagCompound = new NBTTagCompound(); if (!stack.stackTagCompound.hasKey("AttributeModifiers", Constants.NBT.TAG_LIST) stack.stackTagCompound.setTag("AttributeModifiers", new NBTTagList()); NBTTagList attributeModifiers = stack.stackTagCompound.getTagList("AttributeModifiers"); NBTTagCompound speedModifier = new NBTTagCompound(); speedModifier.setString("AttributeName", "generic.movementSpeed"); // ... Other things in the speed modifier NBT tag ... attributeModifiers.appendTag(speedModifier); There may be a better way, but this would work.
-
[1.8.9] [UNSOLVED] Constantly get player's hand position
Earthcomputer replied to JelloMOO's topic in Modder Support
Please give more information, such as your code, why you need to do this, whether you are want the hand position in third person or first person, etc. I'd take a look at the vanilla code which calculates hand position. -
When the block detects it is in the correct structure you should change a property in the block state (using setBlockState in World), or use getActualState in your block class). Then your blockstate json file can detect if the block is in a different state and point to a different model file. Look at redstone wire. It has to detect whether there are redstone components nearby.
-
[1.9] [SOLVED] GUI doesn't open on right-click
Earthcomputer replied to GlowingWater's topic in Modder Support
In the GUI Handler on the client side you should be returning an instance of GuiCrate. Normally you only have one GUI Handler per mod. -
[1.7.10] Send files between server and client
Earthcomputer replied to Dijkstra's topic in Modder Support
There are plenty of tutorials on custom packets in Forge, such as http://greyminecraftcoder.blogspot.co.uk/2015/01/client-server-communication-using-your.html?m=1 -
[1.7.10] Send files between server and client
Earthcomputer replied to Dijkstra's topic in Modder Support
I think packets are the way to go. In vanilla the server can send an entire resource pack to the client via S48PacketResourcePackSend. Edit: that example is no good, but the server still sends entire chunks to the client -
http://minecraft.gamepedia.com/Model By the looks of things you're going to need 12 different elements per block: 2 per side, 1 with the shade tag set to false for the parts which do not "emit" light, 1 with the shade tag set to true, for the parts which do "emit" light.
-
[1.8.9] Language translation aliases
Earthcomputer replied to Earthcomputer's topic in Modder Support
Well, this seems to work: In my opinion this feature should be in Forge. You can do a lot of useful stuff with this, not just aliases. Edit: oops! That didn't work! This does, though: public class SmartTranslationRegistry { private static final Map<String, String> translationMap = ReflectionHelper.getPrivateValue(StringTranslate.class, ReflectionHelper.<StringTranslate, StringTranslate> getPrivateValue(StringTranslate.class, null, "field_74817_a", "instance"), "field_74816_c", "languageList"); private static final Map<String, String> i18nProps = ReflectionHelper.getPrivateValue(Locale.class, ReflectionHelper.<Locale, I18n> getPrivateValue(I18n.class, null, "field_135054_a", "i18nLocale"), "field_135032_a", "properties"); public static final Map<String, String> translations = Collections.unmodifiableMap(translationMap); private SmartTranslationRegistry() { } private static final Map<String, ISmartTranslation> smartTranslations = Maps.newHashMap(); static { ((IReloadableResourceManager) Minecraft.getMinecraft().getResourceManager()) .registerReloadListener(new ResourceManagerReloadListener()); } public static void registerTranslation(String key, ISmartTranslation translation) { smartTranslations.put(key, translation); } public static void registerAlias(String key1, String key2) { registerTranslation(key1, new AliasTranslation(key2)); } private static class AliasTranslation implements ISmartTranslation { private String otherKey; public AliasTranslation(String keyToTranslateTo) { this.otherKey = keyToTranslateTo; } @Override public String translateToLocal(String language, Map<String, String> translations) { return translations.get(otherKey); } } private static class ResourceManagerReloadListener implements IResourceManagerReloadListener { @Override public void onResourceManagerReload(IResourceManager resourceManager) { EasyEditorsApi.logger.info("Reloading resource manager"); String lang = FMLCommonHandler.instance().getCurrentLanguage(); for (Map.Entry<String, ISmartTranslation> entry : smartTranslations.entrySet()) { String translation = entry.getValue().translateToLocal(lang, translations); translationMap.put(entry.getKey(), translation); i18nProps.put(entry.getKey(), translation); } } } } And then in the preinit method of the main mod class: SmartTranslationRegistry.registerAlias("entity.MinecartChest.name", "item.minecartChest.name"); -
In addition to the above comment, I'd take a look into the flint and steel's code to see how it sets stuff on fire. I believe World has a method like setBlockOnFire, which also plays the sound effect. Also, if this isn't your own custom item you're working with, you may need to look into event handling, which there are plenty of tutorials for.
-
[1.8.9] Language translation aliases
Earthcomputer replied to Earthcomputer's topic in Modder Support
Well, for example, someone makes a resource pack that adds a new language, and they define a translation for item.minecartChest.name. They probably won't be defining a translation for entity.MinecartChest.name, so if I could set an alias, the translation wouldn't just map to whatever's in en_US. -
Hello everyone! I am trying to add a localization to entity.MinecartChest.name. I know that inserting "entity.MinecartChest.name=Minecart with Chest" into my language file will add a translation. However, I do not want to do this for every single language file, since there is already a translation key which will lead to the correct translation: item.minecartChest.name. So my question is: is there a way to tell Minecraft that whenever it wants to translate the key "entity.MinecartChest.name", it should translate it to the same translation as "item.minecartChest.name"? Something similar to LanguageRegistry.instance().addTranslationAlias("entity.MinecartChest.name", "item.minecartChest.name"), perhaps? If you know there isn't a way to do this, I'm happy to accept that answer. Thanks in advance for help
-
[1.6.4] setting harvest level of vanilla minecraft blocks
Earthcomputer replied to Ziplock_Jim's topic in Modder Support
Out of interest, why are you still modding in 1.6.4? -
[1.8] Natural vs. Manufactured block detection
Earthcomputer replied to tool_user's topic in Modder Support
It is impossible to do this fail-safe either way, because: If you're trying to tell whether an item can be manufactured, you have to know all the ways in which an item can be manufactured (crafted, smelted, etc.). If mods add their own ways of manufacturing (e.g. pulverizers), you may not always know if an item can be manufactured in that way. If you're trying to tell whether a block is naturally generated, you've basically got no chance. The best chance you've got is searching through ASM data, but even a best-effort algorithm to test if a block is naturally generated would still fail about 50% of the time. You've got a bit more chance with items generated in chests, as Forge has hooks for chest generation, and even more chance with mob drops, provided you have an instance of World. Even after all that, there still might be a way to be about 90% sure that an item can be manufactured: use NEI. Most mods which add extra machines will register their crafting recipes to NEI, so you have less chance of missing any manufacturing methods. You can also find a list of blocks and whether they are naturally generated on the Minecraft Wiki. Hope this helps -
[Solved] [1.8] Textures of radio buttons go black
Earthcomputer replied to Earthcomputer's topic in Modder Support
Solved: fixed with a call to GlStateManager.color(1, 1, 1, 1) before drawing the radio button -
Anyone have any idea why my second radio button is going black? The first radio button above it isn't going black. Screenshot: http://imgur.com/k2xqGA5 Radio button texture: http://imgur.com/0MIZeW3 Code: Thanks in advance for help
-
Well, after a week of playing around with this, I think I've got a working solution which works for me (with just one small bug): The small bug is in the method mouseReleased. Anyone know how to differentiate between different mouse buttons in that method?
-
I'm making a client-side mod which requires a GUI which may be bigger that the physical screen size. I've looked at the vanilla code for scroll bars (in GuiSlot), but I don't understand it. How would I implement a two-way scroll bar such that: Everything drawn on the screen is translated (shifted) in the opposite direction to the scroll bars The mouse co-ordinates are translated in the same direction as the scroll bars, so it is easy to determine what was clicked Are there examples of code that can already to this? If not, how would I go about implementing it? Thanks in advance for help. Edit: okay, I've made a start. It's just the commented parts of drawScreen I need help with now.
-
[Help] Prevent player from falling off mount
Earthcomputer replied to big_red_frog's topic in Modder Support
Dang, I forgot you get dismounted in water, I'll edit above -
[1.7.10][SOLVED] Coremod not loading in eclipse
Earthcomputer replied to Elix_x's topic in Modder Support
Never mind, just took a closer look at your code and I see why. It looks like you have tried it with the equals sign but showed us the log of a run without the equals sign, given that they're shown as two separate arguments. Try with the equals sign in the JVM arguemtns