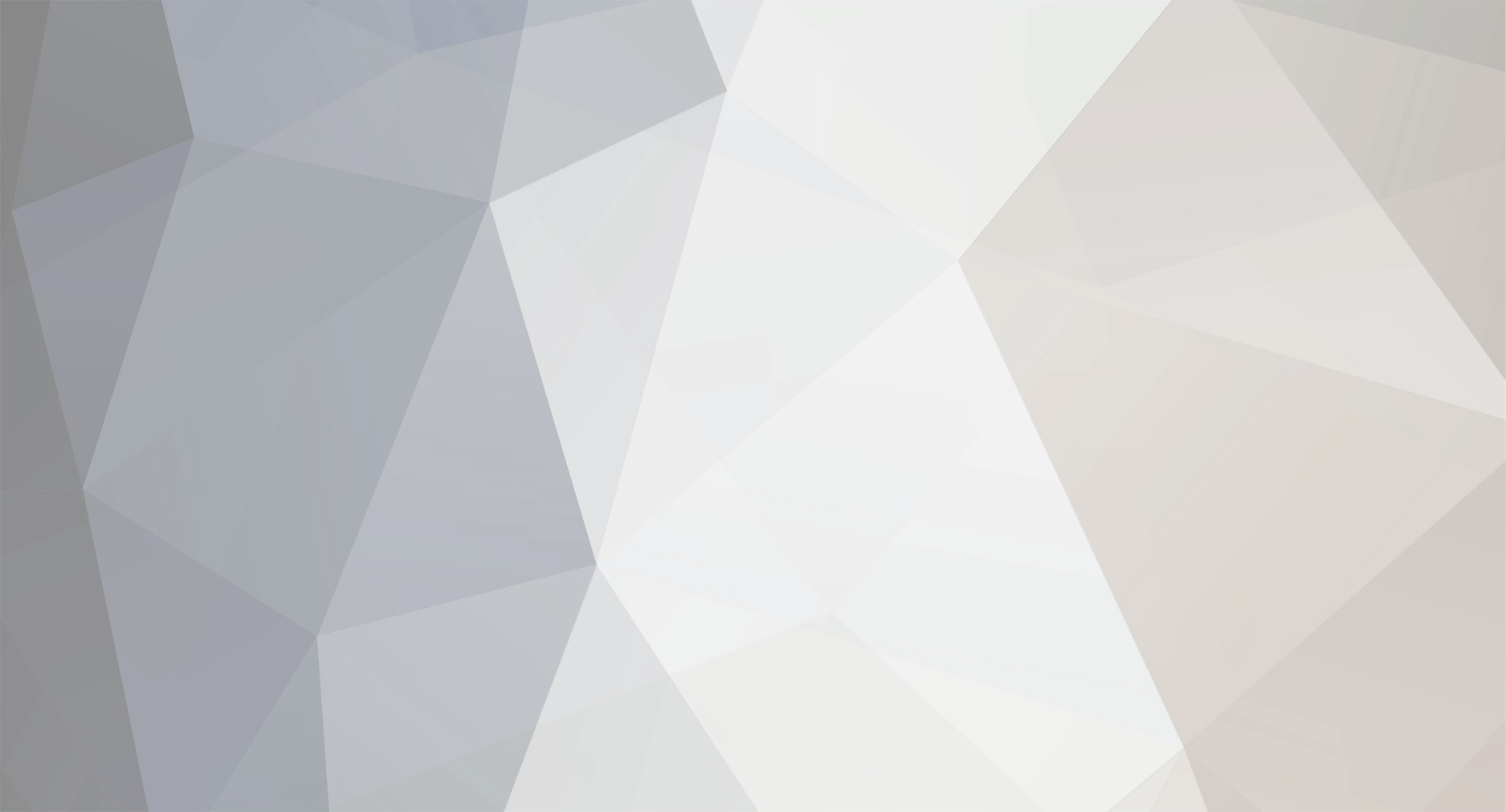
grossik
Members-
Posts
88 -
Joined
-
Last visited
Everything posted by grossik
-
[SOLVED] [1.15.2] Why my custom item entity not renderer?
grossik replied to grossik's topic in Modder Support
This is correct? @Override public IPacket<?> createSpawnPacket() { return NetworkHooks.getEntitySpawningPacket(this); } -
[SOLVED] [1.15.2] Why my custom item entity not renderer?
grossik replied to grossik's topic in Modder Support
Because I test this Ok this: package cz.grossik.farmcraft.entity; import cz.grossik.farmcraft.init.ItemInit; import net.minecraft.block.material.Material; import net.minecraft.entity.EntityType; import net.minecraft.entity.item.ItemEntity; import net.minecraft.item.ItemStack; import net.minecraft.network.IPacket; import net.minecraft.network.datasync.DataParameter; import net.minecraft.network.datasync.DataSerializers; import net.minecraft.network.datasync.EntityDataManager; import net.minecraft.network.play.server.SSpawnObjectPacket; import net.minecraft.world.World; public class FarmCraftItemEntity extends ItemEntity { private static final DataParameter<ItemStack> ITEM = EntityDataManager.createKey(FarmCraftItemEntity.class, DataSerializers.ITEMSTACK); public FarmCraftItemEntity(EntityType<? extends ItemEntity> p_i50217_1_, World p_i50217_2_) { super(p_i50217_1_, p_i50217_2_); } @Override protected void registerData() { this.getDataManager().register(ITEM, ItemStack.EMPTY); } @Override public IPacket<?> createSpawnPacket() { return new SSpawnObjectPacket(this); } @Override public void tick() { System.err.println("tick"); if(this.isInWater()) { System.err.println("water"); ItemStack newItemStack = new ItemStack(ItemInit.soaked_barley.get()); ItemEntity newItem = new ItemEntity(this.world, this.getPosition().getX(), this.getPosition().getY(), this.getPosition().getZ(), newItemStack); this.world.addEntity(newItem); this.remove(); } super.tick(); } } -
[SOLVED] [1.15.2] Why my custom item entity not renderer?
grossik replied to grossik's topic in Modder Support
Render: package cz.grossik.farmcraft.renderer; import com.mojang.blaze3d.matrix.MatrixStack; import cz.grossik.farmcraft.entity.FarmCraftItemEntity; import java.util.Random; import net.minecraft.client.Minecraft; import net.minecraft.client.renderer.IRenderTypeBuffer; import net.minecraft.client.renderer.Vector3f; import net.minecraft.client.renderer.entity.EntityRenderer; import net.minecraft.client.renderer.entity.EntityRendererManager; import net.minecraft.client.renderer.model.IBakedModel; import net.minecraft.client.renderer.model.ItemCameraTransforms; import net.minecraft.client.renderer.texture.AtlasTexture; import net.minecraft.client.renderer.texture.OverlayTexture; import net.minecraft.entity.LivingEntity; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.util.ResourceLocation; import net.minecraft.util.math.MathHelper; import net.minecraftforge.api.distmarker.Dist; import net.minecraftforge.api.distmarker.OnlyIn; @SuppressWarnings("deprecation") public class FarmCraftItemEntityRenderer extends EntityRenderer<FarmCraftItemEntity> { private final net.minecraft.client.renderer.ItemRenderer itemRenderer; private final Random random = new Random(); public FarmCraftItemEntityRenderer(EntityRendererManager renderManagerIn) { super(renderManagerIn); this.itemRenderer = Minecraft.getInstance().getItemRenderer(); this.shadowSize = 0.15F; this.shadowOpaque = 0.75F; } protected int getModelCount(ItemStack stack) { int i = 1; if (stack.getCount() > 48) { i = 5; } else if (stack.getCount() > 32) { i = 4; } else if (stack.getCount() > 16) { i = 3; } else if (stack.getCount() > 1) { i = 2; } return i; } public void render(FarmCraftItemEntity entityIn, float entityYaw, float partialTicks, MatrixStack matrixStackIn, IRenderTypeBuffer bufferIn, int packedLightIn) { matrixStackIn.push(); ItemStack itemstack = entityIn.getItem(); int i = itemstack.isEmpty() ? 187 : Item.getIdFromItem(itemstack.getItem()) + itemstack.getDamage(); this.random.setSeed((long)i); IBakedModel ibakedmodel = this.itemRenderer.getItemModelWithOverrides(itemstack, entityIn.world, (LivingEntity)null); boolean flag = ibakedmodel.isGui3d(); int j = this.getModelCount(itemstack); float f = 0.25F; float f1 = shouldBob() ? MathHelper.sin(((float)entityIn.getAge() + partialTicks) / 10.0F + entityIn.hoverStart) * 0.1F + 0.1F : 0; float f2 = ibakedmodel.getItemCameraTransforms().getTransform(ItemCameraTransforms.TransformType.GROUND).scale.getY(); matrixStackIn.translate(0.0D, (double)(f1 + 0.25F * f2), 0.0D); float f3 = ((float)entityIn.getAge() + partialTicks) / 20.0F + entityIn.hoverStart; matrixStackIn.rotate(Vector3f.YP.rotation(f3)); if (!flag) { float f7 = -0.0F * (float)(j - 1) * 0.5F; float f8 = -0.0F * (float)(j - 1) * 0.5F; float f9 = -0.09375F * (float)(j - 1) * 0.5F; matrixStackIn.translate((double)f7, (double)f8, (double)f9); } for(int k = 0; k < j; ++k) { matrixStackIn.push(); if (k > 0) { if (flag) { float f11 = (this.random.nextFloat() * 2.0F - 1.0F) * 0.15F; float f13 = (this.random.nextFloat() * 2.0F - 1.0F) * 0.15F; float f10 = (this.random.nextFloat() * 2.0F - 1.0F) * 0.15F; matrixStackIn.translate(shouldSpreadItems() ? f11 : 0, shouldSpreadItems() ? f13 : 0, shouldSpreadItems() ? f10 : 0); } else { float f12 = (this.random.nextFloat() * 2.0F - 1.0F) * 0.15F * 0.5F; float f14 = (this.random.nextFloat() * 2.0F - 1.0F) * 0.15F * 0.5F; matrixStackIn.translate(shouldSpreadItems() ? f12 : 0, shouldSpreadItems() ? f14 : 0, 0.0D); } } this.itemRenderer.renderItem(itemstack, ItemCameraTransforms.TransformType.GROUND, false, matrixStackIn, bufferIn, packedLightIn, OverlayTexture.NO_OVERLAY, ibakedmodel); matrixStackIn.pop(); if (!flag) { matrixStackIn.translate(0.0, 0.0, 0.09375F); } } matrixStackIn.pop(); super.render(entityIn, entityYaw, partialTicks, matrixStackIn, bufferIn, packedLightIn); } /** * Returns the location of an entity's texture. */ public ResourceLocation getEntityTexture(FarmCraftItemEntity entity) { return AtlasTexture.LOCATION_BLOCKS_TEXTURE; } /*==================================== FORGE START ===========================================*/ /** * @return If items should spread out when rendered in 3D */ public boolean shouldSpreadItems() { return true; } /** * @return If items should have a bob effect */ public boolean shouldBob() { return true; } /*==================================== FORGE END =============================================*/ } Entity: package cz.grossik.farmcraft.entity; import java.util.Objects; import java.util.UUID; import javax.annotation.Nullable; import cz.grossik.farmcraft.init.ItemInit; import net.minecraft.block.material.Material; import net.minecraft.entity.Entity; import net.minecraft.entity.EntityType; import net.minecraft.entity.MoverType; import net.minecraft.entity.item.ItemEntity; import net.minecraft.entity.player.PlayerEntity; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.item.Items; import net.minecraft.nbt.CompoundNBT; import net.minecraft.nbt.NBTUtil; import net.minecraft.network.IPacket; import net.minecraft.network.datasync.DataParameter; import net.minecraft.network.datasync.DataSerializers; import net.minecraft.network.datasync.EntityDataManager; import net.minecraft.network.play.server.SSpawnObjectPacket; import net.minecraft.stats.Stats; import net.minecraft.tags.FluidTags; import net.minecraft.util.DamageSource; import net.minecraft.util.SoundEvents; import net.minecraft.util.math.BlockPos; import net.minecraft.util.math.MathHelper; import net.minecraft.util.math.Vec3d; import net.minecraft.util.text.ITextComponent; import net.minecraft.util.text.TranslationTextComponent; import net.minecraft.world.World; import net.minecraft.world.dimension.DimensionType; import net.minecraftforge.api.distmarker.Dist; import net.minecraftforge.api.distmarker.OnlyIn; public class FarmCraftItemEntity extends ItemEntity { private static final DataParameter<ItemStack> ITEM = EntityDataManager.createKey(FarmCraftItemEntity.class, DataSerializers.ITEMSTACK); private int age; private int pickupDelay; private int health = 5; private UUID thrower; private UUID owner; public int lifespan = 6000; public final float hoverStart = (float)(Math.random() * Math.PI * 2.0D); public FarmCraftItemEntity(EntityType<? extends ItemEntity> p_i50217_1_, World p_i50217_2_) { super(p_i50217_1_, p_i50217_2_); } @Override protected boolean canTriggerWalking() { return false; } @Override protected void registerData() { this.getDataManager().register(ITEM, ItemStack.EMPTY); } @Override public void tick() { if (getItem().onEntityItemUpdate(this)) return; if (this.getItem().isEmpty()) { this.remove(); } else { super.tick(); if (this.pickupDelay > 0 && this.pickupDelay != 32767) { --this.pickupDelay; } this.prevPosX = this.getPosX(); this.prevPosY = this.getPosY(); this.prevPosZ = this.getPosZ(); Vec3d vec3d = this.getMotion(); if (this.areEyesInFluid(FluidTags.WATER)) { this.applyFloatMotion(); } else if (!this.hasNoGravity()) { this.setMotion(this.getMotion().add(0.0D, -0.04D, 0.0D)); } if (this.world.isRemote) { this.noClip = false; } else { this.noClip = !this.world.func_226669_j_(this); if (this.noClip) { this.pushOutOfBlocks(this.getPosX(), (this.getBoundingBox().minY + this.getBoundingBox().maxY) / 2.0D, this.getPosZ()); } } if (!this.onGround || horizontalMag(this.getMotion()) > (double)1.0E-5F || (this.ticksExisted + this.getEntityId()) % 4 == 0) { this.move(MoverType.SELF, this.getMotion()); float f = 0.98F; if (this.onGround) { BlockPos pos = new BlockPos(this.getPosX(), this.getPosY() - 1.0D, this.getPosZ()); f = this.world.getBlockState(pos).getSlipperiness(this.world, pos, this) * 0.98F; } this.setMotion(this.getMotion().mul((double)f, 0.98D, (double)f)); if (this.onGround) { this.setMotion(this.getMotion().mul(1.0D, -0.5D, 1.0D)); } } boolean flag = MathHelper.floor(this.prevPosX) != MathHelper.floor(this.getPosX()) || MathHelper.floor(this.prevPosY) != MathHelper.floor(this.getPosY()) || MathHelper.floor(this.prevPosZ) != MathHelper.floor(this.getPosZ()); int i = flag ? 2 : 40; if (this.ticksExisted % i == 0) { if (this.world.getFluidState(new BlockPos(this)).isTagged(FluidTags.LAVA)) { this.setMotion((double)((this.rand.nextFloat() - this.rand.nextFloat()) * 0.2F), (double)0.2F, (double)((this.rand.nextFloat() - this.rand.nextFloat()) * 0.2F)); this.playSound(SoundEvents.ENTITY_GENERIC_BURN, 0.4F, 2.0F + this.rand.nextFloat() * 0.4F); } if (!this.world.isRemote && this.func_213857_z()) { this.searchForOtherItemsNearby(); } } if (this.age != -32768) { ++this.age; } this.isAirBorne |= this.handleWaterMovement(); if (!this.world.isRemote) { double d0 = this.getMotion().subtract(vec3d).lengthSquared(); if (d0 > 0.01D) { this.isAirBorne = true; } } ItemStack item = this.getItem(); if (!this.world.isRemote && this.age >= lifespan) { int hook = net.minecraftforge.event.ForgeEventFactory.onItemExpire(this, item); if (hook < 0) this.remove(); else this.lifespan += hook; } if (item.isEmpty()) { this.remove(); } } } private void applyFloatMotion() { Vec3d vec3d = this.getMotion(); this.setMotion(vec3d.x * (double)0.99F, vec3d.y + (double)(vec3d.y < (double)0.06F ? 5.0E-4F : 0.0F), vec3d.z * (double)0.99F); } private void searchForOtherItemsNearby() { if (this.func_213857_z()) { for(FarmCraftItemEntity itementity : this.world.getEntitiesWithinAABB(FarmCraftItemEntity.class, this.getBoundingBox().grow(0.5D, 0.0D, 0.5D), (p_213859_1_) -> { return p_213859_1_ != this && p_213859_1_.func_213857_z(); })) { if (itementity.func_213857_z()) { this.func_226530_a_(itementity); if (this.removed) { break; } } } } } private boolean func_213857_z() { ItemStack itemstack = this.getItem(); return this.isAlive() && this.pickupDelay != 32767 && this.age != -32768 && this.age < 6000 && itemstack.getCount() < itemstack.getMaxStackSize(); } private void func_226530_a_(FarmCraftItemEntity p_226530_1_) { ItemStack itemstack = this.getItem(); ItemStack itemstack1 = p_226530_1_.getItem(); if (Objects.equals(this.getOwnerId(), p_226530_1_.getOwnerId()) && func_226532_a_(itemstack, itemstack1)) { if (itemstack1.getCount() < itemstack.getCount()) { func_213858_a(this, itemstack, p_226530_1_, itemstack1); } else { func_213858_a(p_226530_1_, itemstack1, this, itemstack); } } } public static boolean func_226532_a_(ItemStack p_226532_0_, ItemStack p_226532_1_) { if (p_226532_1_.getItem() != p_226532_0_.getItem()) { return false; } else if (p_226532_1_.getCount() + p_226532_0_.getCount() > p_226532_1_.getMaxStackSize()) { return false; } else if (p_226532_1_.hasTag() ^ p_226532_0_.hasTag()) { return false; }else if (!p_226532_0_.areCapsCompatible(p_226532_1_)) { return false; } else { return !p_226532_1_.hasTag() || p_226532_1_.getTag().equals(p_226532_0_.getTag()); } } public static ItemStack func_226533_a_(ItemStack p_226533_0_, ItemStack p_226533_1_, int p_226533_2_) { int i = Math.min(Math.min(p_226533_0_.getMaxStackSize(), p_226533_2_) - p_226533_0_.getCount(), p_226533_1_.getCount()); ItemStack itemstack = p_226533_0_.copy(); itemstack.grow(i); p_226533_1_.shrink(i); return itemstack; } private static void func_226531_a_(FarmCraftItemEntity p_226531_0_, ItemStack p_226531_1_, ItemStack p_226531_2_) { ItemStack itemstack = func_226533_a_(p_226531_1_, p_226531_2_, 64); p_226531_0_.setItem(itemstack); } private static void func_213858_a(FarmCraftItemEntity p_213858_0_, ItemStack p_213858_1_, FarmCraftItemEntity p_213858_2_, ItemStack p_213858_3_) { func_226531_a_(p_213858_0_, p_213858_1_, p_213858_3_); p_213858_0_.pickupDelay = Math.max(p_213858_0_.pickupDelay, p_213858_2_.pickupDelay); p_213858_0_.age = Math.min(p_213858_0_.age, p_213858_2_.age); if (p_213858_3_.isEmpty()) { p_213858_2_.remove(); } } @Override protected void dealFireDamage(int amount) { this.attackEntityFrom(DamageSource.IN_FIRE, (float)amount); } @Override public boolean attackEntityFrom(DamageSource source, float amount) { if (this.world.isRemote || this.removed) return false; //Forge: Fixes MC-53850 if (this.isInvulnerableTo(source)) { return false; } else if (!this.getItem().isEmpty() && this.getItem().getItem() == Items.NETHER_STAR && source.isExplosion()) { return false; } else { this.markVelocityChanged(); this.health = (int)((float)this.health - amount); if (this.health <= 0) { this.remove(); } return false; } } @Override public void writeAdditional(CompoundNBT compound) { compound.putShort("Health", (short)this.health); compound.putShort("Age", (short)this.age); compound.putShort("PickupDelay", (short)this.pickupDelay); compound.putInt("Lifespan", lifespan); if (this.getThrowerId() != null) { compound.put("Thrower", NBTUtil.writeUniqueId(this.getThrowerId())); } if (this.getOwnerId() != null) { compound.put("Owner", NBTUtil.writeUniqueId(this.getOwnerId())); } if (!this.getItem().isEmpty()) { compound.put("Item", this.getItem().write(new CompoundNBT())); } } @Override public void readAdditional(CompoundNBT compound) { this.health = compound.getShort("Health"); this.age = compound.getShort("Age"); if (compound.contains("PickupDelay")) { this.pickupDelay = compound.getShort("PickupDelay"); } if (compound.contains("Lifespan")) lifespan = compound.getInt("Lifespan"); if (compound.contains("Owner", 10)) { this.owner = NBTUtil.readUniqueId(compound.getCompound("Owner")); } if (compound.contains("Thrower", 10)) { this.thrower = NBTUtil.readUniqueId(compound.getCompound("Thrower")); } CompoundNBT compoundnbt = compound.getCompound("Item"); this.setItem(ItemStack.read(compoundnbt)); if (this.getItem().isEmpty()) { this.remove(); } } @Override public void onCollideWithPlayer(PlayerEntity entityIn) { if (!this.world.isRemote) { if (this.pickupDelay > 0) return; ItemStack itemstack = this.getItem(); Item item = itemstack.getItem(); int i = itemstack.getCount(); int hook = net.minecraftforge.event.ForgeEventFactory.onItemPickup(this, entityIn); if (hook < 0) return; ItemStack copy = itemstack.copy(); if (this.pickupDelay == 0 && (this.owner == null || lifespan - this.age <= 200 || this.owner.equals(entityIn.getUniqueID())) && (hook == 1 || i <= 0 || entityIn.inventory.addItemStackToInventory(itemstack))) { copy.setCount(copy.getCount() - getItem().getCount()); net.minecraftforge.fml.hooks.BasicEventHooks.firePlayerItemPickupEvent(entityIn, this, copy); entityIn.onItemPickup(this, i); if (itemstack.isEmpty()) { entityIn.onItemPickup(this, i); this.remove(); itemstack.setCount(i); } entityIn.addStat(Stats.ITEM_PICKED_UP.get(item), i); } } } @Override public ITextComponent getName() { ITextComponent itextcomponent = this.getCustomName(); return (ITextComponent)(itextcomponent != null ? itextcomponent : new TranslationTextComponent(this.getItem().getTranslationKey())); } @Override public boolean canBeAttackedWithItem() { return false; } @Nullable @Override public Entity changeDimension(DimensionType destination, net.minecraftforge.common.util.ITeleporter teleporter) { Entity entity = super.changeDimension(destination, teleporter); if (!this.world.isRemote && entity instanceof FarmCraftItemEntity) { ((FarmCraftItemEntity)entity).searchForOtherItemsNearby(); } return entity; } @Override public ItemStack getItem() { return this.getDataManager().get(ITEM); } @Override public void setItem(ItemStack stack) { this.getDataManager().set(ITEM, stack); } @Nullable @Override public UUID getOwnerId() { return this.owner; } @Override public void setOwnerId(@Nullable UUID p_200217_1_) { this.owner = p_200217_1_; } @Nullable @Override public UUID getThrowerId() { return this.thrower; } @Override public void setThrowerId(@Nullable UUID p_200216_1_) { this.thrower = p_200216_1_; } @Override public int getAge() { return this.age; } @Override public void setDefaultPickupDelay() { this.pickupDelay = 10; } @Override public void setNoPickupDelay() { this.pickupDelay = 0; } @Override public void setInfinitePickupDelay() { this.pickupDelay = 32767; } @Override public void setPickupDelay(int ticks) { this.pickupDelay = ticks; } @Override public boolean cannotPickup() { return this.pickupDelay > 0; } @Override public void setNoDespawn() { this.age = -6000; } @Override public void makeFakeItem() { this.setInfinitePickupDelay(); this.age = getItem().getEntityLifespan(world) - 1; } @Override public IPacket<?> createSpawnPacket() { return new SSpawnObjectPacket(this); } } I copy all code of ItemEntity -
[SOLVED] [1.15.2] Why my custom item entity not renderer?
grossik replied to grossik's topic in Modder Support
Ok. Thank you. But it didn't solve my problem. Renderer is initialized but render method not called -
Hello. I make custom item entity, but if I press Q my item not render. I dont know why Entity: package cz.grossik.farmcraft.entity; import cz.grossik.farmcraft.init.ItemInit; import net.minecraft.block.material.Material; import net.minecraft.entity.EntityType; import net.minecraft.entity.item.ItemEntity; import net.minecraft.item.ItemStack; import net.minecraft.world.World; public class FarmCraftItemEntity extends ItemEntity { public FarmCraftItemEntity(EntityType<? extends ItemEntity> p_i50217_1_, World p_i50217_2_) { super(p_i50217_1_, p_i50217_2_); } public FarmCraftItemEntity(World worldIn, double x, double y, double z) { super(worldIn, x, y, z); } public FarmCraftItemEntity(World worldIn, double x, double y, double z, ItemStack stack) { this(worldIn, x, y, z); } @Override public void tick() { System.err.println("tick"); if(this.isInWater()) { System.err.println("water"); ItemStack newItemStack = new ItemStack(ItemInit.soaked_barley.get()); ItemEntity newItem = new ItemEntity(this.world, this.getPosition().getX(), this.getPosition().getY(), this.getPosition().getZ(), newItemStack); this.world.addEntity(newItem); this.remove(); } super.tick(); } } Render: package cz.grossik.farmcraft.renderer; import com.mojang.blaze3d.matrix.MatrixStack; import cz.grossik.farmcraft.entity.FarmCraftItemEntity; import java.util.Random; import net.minecraft.client.Minecraft; import net.minecraft.client.renderer.IRenderTypeBuffer; import net.minecraft.client.renderer.Vector3f; import net.minecraft.client.renderer.entity.EntityRenderer; import net.minecraft.client.renderer.entity.EntityRendererManager; import net.minecraft.client.renderer.model.IBakedModel; import net.minecraft.client.renderer.model.ItemCameraTransforms; import net.minecraft.client.renderer.texture.AtlasTexture; import net.minecraft.client.renderer.texture.OverlayTexture; import net.minecraft.entity.LivingEntity; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.util.ResourceLocation; import net.minecraft.util.math.MathHelper; import net.minecraftforge.api.distmarker.Dist; import net.minecraftforge.api.distmarker.OnlyIn; @OnlyIn(Dist.CLIENT) public class FarmCraftItemEntityRenderer extends EntityRenderer<FarmCraftItemEntity> { private final net.minecraft.client.renderer.ItemRenderer itemRenderer; private final Random random = new Random(); public FarmCraftItemEntityRenderer(EntityRendererManager renderManagerIn) { super(renderManagerIn); this.itemRenderer = Minecraft.getInstance().getItemRenderer(); this.shadowSize = 0.15F; this.shadowOpaque = 0.75F; } protected int getModelCount(ItemStack stack) { int i = 1; if (stack.getCount() > 48) { i = 5; } else if (stack.getCount() > 32) { i = 4; } else if (stack.getCount() > 16) { i = 3; } else if (stack.getCount() > 1) { i = 2; } return i; } public void render(FarmCraftItemEntity entityIn, float entityYaw, float partialTicks, MatrixStack matrixStackIn, IRenderTypeBuffer bufferIn, int packedLightIn) { matrixStackIn.push(); ItemStack itemstack = entityIn.getItem(); int i = itemstack.isEmpty() ? 187 : Item.getIdFromItem(itemstack.getItem()) + itemstack.getDamage(); this.random.setSeed((long)i); IBakedModel ibakedmodel = this.itemRenderer.getItemModelWithOverrides(itemstack, entityIn.world, (LivingEntity)null); boolean flag = ibakedmodel.isGui3d(); int j = this.getModelCount(itemstack); float f = 0.25F; float f1 = shouldBob() ? MathHelper.sin(((float)entityIn.getAge() + partialTicks) / 10.0F + entityIn.hoverStart) * 0.1F + 0.1F : 0; float f2 = ibakedmodel.getItemCameraTransforms().getTransform(ItemCameraTransforms.TransformType.GROUND).scale.getY(); matrixStackIn.translate(0.0D, (double)(f1 + 0.25F * f2), 0.0D); float f3 = ((float)entityIn.getAge() + partialTicks) / 20.0F + entityIn.hoverStart; matrixStackIn.rotate(Vector3f.YP.rotation(f3)); if (!flag) { float f7 = -0.0F * (float)(j - 1) * 0.5F; float f8 = -0.0F * (float)(j - 1) * 0.5F; float f9 = -0.09375F * (float)(j - 1) * 0.5F; matrixStackIn.translate((double)f7, (double)f8, (double)f9); } for(int k = 0; k < j; ++k) { matrixStackIn.push(); if (k > 0) { if (flag) { float f11 = (this.random.nextFloat() * 2.0F - 1.0F) * 0.15F; float f13 = (this.random.nextFloat() * 2.0F - 1.0F) * 0.15F; float f10 = (this.random.nextFloat() * 2.0F - 1.0F) * 0.15F; matrixStackIn.translate(shouldSpreadItems() ? f11 : 0, shouldSpreadItems() ? f13 : 0, shouldSpreadItems() ? f10 : 0); } else { float f12 = (this.random.nextFloat() * 2.0F - 1.0F) * 0.15F * 0.5F; float f14 = (this.random.nextFloat() * 2.0F - 1.0F) * 0.15F * 0.5F; matrixStackIn.translate(shouldSpreadItems() ? f12 : 0, shouldSpreadItems() ? f14 : 0, 0.0D); } } this.itemRenderer.renderItem(itemstack, ItemCameraTransforms.TransformType.GROUND, false, matrixStackIn, bufferIn, packedLightIn, OverlayTexture.NO_OVERLAY, ibakedmodel); matrixStackIn.pop(); if (!flag) { matrixStackIn.translate(0.0, 0.0, 0.09375F); } } matrixStackIn.pop(); super.render(entityIn, entityYaw, partialTicks, matrixStackIn, bufferIn, packedLightIn); } /** * Returns the location of an entity's texture. */ public ResourceLocation getEntityTexture(FarmCraftItemEntity entity) { return AtlasTexture.LOCATION_BLOCKS_TEXTURE; } /*==================================== FORGE START ===========================================*/ /** * @return If items should spread out when rendered in 3D */ public boolean shouldSpreadItems() { return true; } /** * @return If items should have a bob effect */ public boolean shouldBob() { return true; } /*==================================== FORGE END =============================================*/ } Registry in FMLClientSetupEvent: RenderingRegistry.registerEntityRenderingHandler(EntityInit.ITEM_ENTITY.get(), FarmCraftItemEntityRenderer::new); Entity init: public static RegistryObject<EntityType<FarmCraftItemEntity>> ITEM_ENTITY = ENTITIES .register("item_farmcraft", () -> EntityType.Builder.<FarmCraftItemEntity>create(FarmCraftItemEntity::new, EntityClassification.MISC) .size(0.25F, 0.25F) .build(new ResourceLocation("minecraft:item").toString()));
-
[1.15.2] JigsawManager register new structure in village problem
grossik replied to grossik's topic in Modder Support
Any idea how to solve this? -
Hello. I try to generate desert village structure to plains village, but this code not work @SubscribeEvent public void setup(FMLCommonSetupEvent event){ JigsawPattern old = JigsawManager.REGISTRY.get(new ResourceLocation("minecraft", "village/plains/houses")); List<JigsawPiece> shuffled = old.getShuffledPieces(new Random()); List<Pair<JigsawPiece, Integer>> newPieces = new ArrayList<>(); for(JigsawPiece p : shuffled){ newPieces.add(new Pair<>(p, 1)); } newPieces.add(new Pair<>(new SingleJigsawPiece("minecraft:village/desert/houses/desert_tool_smith_1"), 10)); ResourceLocation something = old.func_214948_a(); JigsawManager.REGISTRY.register(new JigsawPattern(new ResourceLocation("minecraft", "village/plains/houses"), something, newPieces, PlacementBehaviour.RIGID)); } What is wrong?
-
Hello I'm trying to add a new structure to a desert village, but I don't know how. I try it through generation over JigsawManager.REGISTRY JigsawManager.REGISTRY.register(new JigsawPattern(new ResourceLocation("village/desert/houses"), new ResourceLocation("empty"), ImmutableList.of(new Pair<>(new SingleJigsawPiece("village/desert/houses/teststruktura"), 1)), JigsawPattern.PlacementBehaviour.RIGID)); But I don't think that's a good way.
-
[1.15.2] Tile entity renderer does not see items
grossik replied to grossik's topic in Modder Support
I add send update packet and now its works. Do you know any good tutorials for more complex things like animation in tile entity etc? Thank you all -
[1.15.2] Tile entity renderer does not see items
grossik replied to grossik's topic in Modder Support
I know, I've already deleted it. But it didn't solve my problem. I guess I shouldn't use it LockableTileEntity right? -
[1.15.2] Tile entity renderer does not see items
grossik replied to grossik's topic in Modder Support
Oh ok. Thank you. Now is better? package cz.grossik.farmcraft.block; import java.util.ArrayList; import java.util.List; import java.util.Map; import java.util.Map.Entry; import javax.annotation.Nonnull; import javax.annotation.Nullable; import com.google.common.collect.Lists; import com.google.common.collect.Maps; import cz.grossik.farmcraft.recipe.FarmCraftRecipeType; import cz.grossik.farmcraft.container.JuicerContainer; import cz.grossik.farmcraft.init.FarmCraftTileEntityTypes; import cz.grossik.farmcraft.init.ItemInit; import cz.grossik.farmcraft.recipe.AbstractJuicerRecipe; import net.minecraft.block.BlockState; import net.minecraft.entity.item.ExperienceOrbEntity; import net.minecraft.entity.player.PlayerEntity; import net.minecraft.entity.player.PlayerInventory; import net.minecraft.inventory.IRecipeHelperPopulator; import net.minecraft.inventory.IRecipeHolder; import net.minecraft.inventory.ISidedInventory; import net.minecraft.inventory.ItemStackHelper; import net.minecraft.inventory.container.Container; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.item.Items; import net.minecraft.item.crafting.IRecipe; import net.minecraft.item.crafting.IRecipeType; import net.minecraft.item.crafting.RecipeItemHelper; import net.minecraft.nbt.CompoundNBT; import net.minecraft.tileentity.ITickableTileEntity; import net.minecraft.tileentity.LockableTileEntity; import net.minecraft.util.Direction; import net.minecraft.util.IIntArray; import net.minecraft.util.IItemProvider; import net.minecraft.util.ResourceLocation; import net.minecraft.util.math.MathHelper; import net.minecraft.util.text.ITextComponent; import net.minecraft.util.text.TranslationTextComponent; import net.minecraftforge.common.capabilities.Capability; import net.minecraftforge.common.util.LazyOptional; import net.minecraftforge.items.CapabilityItemHandler; import net.minecraftforge.items.IItemHandlerModifiable; import net.minecraftforge.items.ItemStackHandler; public class JuicerTileEntity extends LockableTileEntity implements ISidedInventory, IRecipeHolder, IRecipeHelperPopulator, ITickableTileEntity { private static final int[] SLOTS_UP = new int[]{0}; private static final int[] SLOTS_DOWN = new int[]{2, 1}; private static final int[] SLOTS_HORIZONTAL = new int[]{1}; private ItemStackHandler items; private LazyOptional<IItemHandlerModifiable> itemHandler = LazyOptional.of(() -> items); private int burnTime; private int recipesUsed; private int cookTime; private int cookTimeTotal; protected final IIntArray furnaceData = new IIntArray() { public int get(int index) { switch(index) { case 0: return JuicerTileEntity.this.burnTime; case 1: return JuicerTileEntity.this.recipesUsed; case 2: return JuicerTileEntity.this.cookTime; case 3: return JuicerTileEntity.this.cookTimeTotal; default: return 0; } } public void set(int index, int value) { switch(index) { case 0: JuicerTileEntity.this.burnTime = value; break; case 1: JuicerTileEntity.this.recipesUsed = value; break; case 2: JuicerTileEntity.this.cookTime = value; break; case 3: JuicerTileEntity.this.cookTimeTotal = value; } } public int size() { return 4; } }; private final Map<ResourceLocation, Integer> field_214022_n = Maps.newHashMap(); protected final IRecipeType<?> recipeType; public JuicerTileEntity() { super(FarmCraftTileEntityTypes.juicer.get()); items = new FarmCraftItemsHandler(); this.recipeType = FarmCraftRecipeType.JUICER_RECIPE; } protected ITextComponent getDefaultName() { return new TranslationTextComponent("farmcraft.container.juicer"); } protected Container createMenu(int id, PlayerInventory player) { return new JuicerContainer(id, player, this, this.furnaceData); } @Deprecated //Forge - get burn times by calling ForgeHooks#getBurnTime(ItemStack) public static Map<Item, Integer> getBurnTimes() { Map<Item, Integer> map = Maps.newLinkedHashMap(); addItemBurnTime(map, ItemInit.juice_glass, 200); return map; } private static void addItemBurnTime(Map<Item, Integer> map, IItemProvider itemProvider, int burnTimeIn) { map.put(itemProvider.asItem(), burnTimeIn); } public boolean isBurning() { return this.burnTime > 0; } public void read(CompoundNBT compound) { super.read(compound); //this.items = NonNullList.withSize(this.getSizeInventory(), ItemStack.EMPTY); items.deserializeNBT(compound.getCompound("juicer:slots")); //ItemStackHelper.loadAllItems(compound, this.items); this.burnTime = compound.getInt("BurnTime"); this.cookTime = compound.getInt("CookTime"); this.cookTimeTotal = compound.getInt("CookTimeTotal"); this.recipesUsed = this.getBurnTime(this.items.getStackInSlot(1)); int i = compound.getShort("RecipesUsedSize"); for(int j = 0; j < i; ++j) { ResourceLocation resourcelocation = new ResourceLocation(compound.getString("RecipeLocation" + j)); int k = compound.getInt("RecipeAmount" + j); this.field_214022_n.put(resourcelocation, k); } } public CompoundNBT write(CompoundNBT compound) { super.write(compound); compound.putInt("BurnTime", this.burnTime); compound.putInt("CookTime", this.cookTime); compound.putInt("CookTimeTotal", this.cookTimeTotal); //ItemStackHelper.saveAllItems(compound, this.items); compound.put("juicer:slots", items.serializeNBT()); compound.putShort("RecipesUsedSize", (short)this.field_214022_n.size()); int i = 0; for(Entry<ResourceLocation, Integer> entry : this.field_214022_n.entrySet()) { compound.putString("RecipeLocation" + i, entry.getKey().toString()); compound.putInt("RecipeAmount" + i, entry.getValue()); ++i; } return compound; } public void tick() { boolean flag = this.isBurning(); boolean flag1 = false; if (this.isBurning()) { --this.burnTime; } if (!this.world.isRemote) { ItemStack itemstack = this.items.getStackInSlot(1); if (this.isBurning() || !itemstack.isEmpty() && !this.items.getStackInSlot(0).isEmpty()) { IRecipe<?> irecipe = this.world.getRecipeManager().getRecipe((IRecipeType<AbstractJuicerRecipe>)this.recipeType, this, this.world).orElse(null); if (!this.isBurning() && this.canSmelt(irecipe)) { this.burnTime = this.getBurnTime(itemstack); this.recipesUsed = this.burnTime; if (this.isBurning()) { flag1 = true; if (itemstack.hasContainerItem()) this.items.insertItem(1, itemstack.getContainerItem(), false); else if (!itemstack.isEmpty()) { Item item = itemstack.getItem(); //itemstack.shrink(1); if (itemstack.isEmpty()) { this.items.insertItem(1, itemstack.getContainerItem(), false); } } } } if (this.isBurning() && this.canSmelt(irecipe)) { ++this.cookTime; if (this.cookTime == this.cookTimeTotal) { this.cookTime = 0; this.cookTimeTotal = this.func_214005_h(); this.func_214007_c(irecipe); flag1 = true; } } else { this.cookTime = 0; this.burnTime = 0; } } else if (!this.isBurning() && this.cookTime > 0) { this.cookTime = MathHelper.clamp(this.cookTime - 2, 0, this.cookTimeTotal); } if (flag != this.isBurning()) { flag1 = true; this.world.setBlockState(this.pos, this.world.getBlockState(this.pos).with(JuicerBlock.VYPALUJE, Boolean.valueOf(this.isBurning())), 3); } BlockState blockstate = this.world.getBlockState(this.getPos()); if (!(blockstate.getBlock() instanceof JuicerBlock)) { return; } if(itemstack.getItem() != ItemInit.juice_glass) { blockstate = blockstate.with(JuicerBlock.HAS_BOTTLE, false); } else { blockstate = blockstate.with(JuicerBlock.HAS_BOTTLE, true); } this.world.setBlockState(this.pos, blockstate, 3); } if (flag1) { this.markDirty(); } } protected boolean canSmelt(@Nullable IRecipe<?> recipeIn) { if (!this.items.getStackInSlot(0).isEmpty() && !this.items.getStackInSlot(1).isEmpty() && recipeIn != null) { ItemStack itemstack = recipeIn.getRecipeOutput(); if (itemstack.isEmpty()) { return false; } else { ItemStack itemstack1 = this.items.getStackInSlot(2); if (itemstack1.isEmpty()) { return true; } else if (!itemstack1.isItemEqual(itemstack)) { return false; } else if (itemstack1.getCount() + itemstack.getCount() <= this.getInventoryStackLimit() && itemstack1.getCount() + itemstack.getCount() <= itemstack1.getMaxStackSize()) { // Forge fix: make furnace respect stack sizes in furnace recipes return true; } else { return itemstack1.getCount() + itemstack.getCount() <= itemstack.getMaxStackSize(); // Forge fix: make furnace respect stack sizes in furnace recipes } } } else { return false; } } private void func_214007_c(@Nullable IRecipe<?> p_214007_1_) { if (p_214007_1_ != null && this.canSmelt(p_214007_1_)) { ItemStack itemstack = this.items.getStackInSlot(0); ItemStack itemstack1 = p_214007_1_.getRecipeOutput(); ItemStack itemstack2 = this.items.getStackInSlot(2); ItemStack itemstack3 = this.items.getStackInSlot(1); if (itemstack2.isEmpty()) { this.items.insertItem(2, itemstack1.copy(), false); } else if (itemstack2.getItem() == itemstack1.getItem()) { itemstack2.grow(itemstack1.getCount()); } if (!this.world.isRemote) { this.setRecipeUsed(p_214007_1_); } itemstack.shrink(1); itemstack3.shrink(1); } } protected int getBurnTime(ItemStack p_213997_1_) { Item item = p_213997_1_.getItem(); if (p_213997_1_.isEmpty()) { return 0; } else { if(getBurnTimes().containsKey(item)) { return getBurnTimes().get(item); } else { return 0; } } } /*protected int getBurnTime(ItemStack p_213997_1_) { if (p_213997_1_.isEmpty()) { return 0; } else { Item item = p_213997_1_.getItem(); if(item == ItemInit.juice_glass) { return 200; } else { return 0; } //return net.minecraftforge.common.ForgeHooks.getBurnTime(p_213997_1_); } }*/ protected int func_214005_h() { return this.world.getRecipeManager().getRecipe((IRecipeType<AbstractJuicerRecipe>)this.recipeType, this, this.world).map(AbstractJuicerRecipe::getCookTime).orElse(200); } public int[] getSlotsForFace(Direction side) { if (side == Direction.DOWN) { return SLOTS_DOWN; } else { return side == Direction.UP ? SLOTS_UP : SLOTS_HORIZONTAL; } } public boolean canInsertItem(int index, ItemStack itemStackIn, @Nullable Direction direction) { return this.isItemValidForSlot(index, itemStackIn); } public boolean canExtractItem(int index, ItemStack stack, Direction direction) { if (direction == Direction.DOWN && index == 1) { Item item = stack.getItem(); if (item != Items.WATER_BUCKET && item != Items.BUCKET) { return false; } } return true; } public int getSizeInventory() { return this.items.getSlots(); } public boolean isEmpty() { for(int i = 0; i < items.getSlots(); i++) { ItemStack itemstack = this.items.getStackInSlot(i); if (!itemstack.isEmpty()) { return false; } } return true; } public ItemStack getStackInSlot(int index) { return this.items.getStackInSlot(index); } public ItemStack decrStackSize(int index, int count) { return index >= 0 && index < items.getSlots() && !items.getStackInSlot(index).isEmpty() && count > 0 ? items.getStackInSlot(index).split(count) : ItemStack.EMPTY; } public ItemStack removeStackFromSlot(int index) { return index >= 0 && index < items.getSlots() ? items.insertItem(index, ItemStack.EMPTY, false) : ItemStack.EMPTY; } public void setInventorySlotContents(int index, ItemStack stack) { ItemStack itemstack = this.items.getStackInSlot(index); boolean flag = !stack.isEmpty() && stack.isItemEqual(itemstack) && ItemStack.areItemStackTagsEqual(stack, itemstack); this.items.insertItem(index, stack, false); if (stack.getCount() > this.getInventoryStackLimit()) { stack.setCount(this.getInventoryStackLimit()); } if (index == 0 && !flag) { this.cookTimeTotal = this.func_214005_h(); this.cookTime = 0; this.markDirty(); } } public boolean isUsableByPlayer(PlayerEntity player) { if (this.world.getTileEntity(this.pos) != this) { return false; } else { return player.getDistanceSq((double)this.pos.getX() + 0.5D, (double)this.pos.getY() + 0.5D, (double)this.pos.getZ() + 0.5D) <= 64.0D; } } public boolean isItemValidForSlot(int index, ItemStack stack) { if (index == 2) { return false; } else if (index != 1) { return true; } else { ItemStack itemstack = this.items.getStackInSlot(1); if(itemstack.getItem() == ItemInit.juice_glass) { return true; } else { return false; } } } public void clear() { for(int i = 0; i < items.getSlots(); i++) { items.insertItem(i, ItemStack.EMPTY, false); } } public void setRecipeUsed(@Nullable IRecipe<?> recipe) { if (recipe != null) { this.field_214022_n.compute(recipe.getId(), (p_214004_0_, p_214004_1_) -> { return 1 + (p_214004_1_ == null ? 0 : p_214004_1_); }); } } @Nullable public IRecipe<?> getRecipeUsed() { return null; } public void onCrafting(PlayerEntity player) { } public void func_213995_d(PlayerEntity p_213995_1_) { List<IRecipe<?>> list = Lists.newArrayList(); for(Entry<ResourceLocation, Integer> entry : this.field_214022_n.entrySet()) { p_213995_1_.world.getRecipeManager().getRecipe(entry.getKey()).ifPresent((p_213993_3_) -> { list.add(p_213993_3_); func_214003_a(p_213995_1_, entry.getValue(), ((AbstractJuicerRecipe)p_213993_3_).getExperience()); }); } p_213995_1_.unlockRecipes(list); this.field_214022_n.clear(); } private static void func_214003_a(PlayerEntity p_214003_0_, int p_214003_1_, float p_214003_2_) { if (p_214003_2_ == 0.0F) { p_214003_1_ = 0; } else if (p_214003_2_ < 1.0F) { int i = MathHelper.floor((float)p_214003_1_ * p_214003_2_); if (i < MathHelper.ceil((float)p_214003_1_ * p_214003_2_) && Math.random() < (double)((float)p_214003_1_ * p_214003_2_ - (float)i)) { ++i; } p_214003_1_ = i; } while(p_214003_1_ > 0) { int j = ExperienceOrbEntity.getXPSplit(p_214003_1_); p_214003_1_ -= j; p_214003_0_.world.addEntity(new ExperienceOrbEntity(p_214003_0_.world, p_214003_0_.func_226277_ct_(), p_214003_0_.func_226278_cu_() + 0.5D, p_214003_0_.func_226281_cx_() + 0.5D, j)); } } public void fillStackedContents(RecipeItemHelper helper) { for(int i = 0; i < items.getSlots(); i++) { ItemStack itemstack = this.items.getStackInSlot(i); } } @Override public <T> LazyOptional<T> getCapability(@Nonnull Capability<T> cap, @Nonnull Direction side) { if (cap == CapabilityItemHandler.ITEM_HANDLER_CAPABILITY) { return itemHandler.cast(); } return super.getCapability(cap, side); } @Override public void remove() { super.remove(); if(itemHandler != null) { itemHandler.invalidate(); } } public ItemStackHandler getInventory() { return this.items; } } But still not work. -
[1.15.2] Tile entity renderer does not see items
grossik replied to grossik's topic in Modder Support
I dont know what you mean. -
Hello, I use tile entity from the furnace for my tile entity and I wanted to make the renderer for this block, but my renderer does not see items that are in block inventory. I still have empty inventory and I don't know why. Tile entity: package cz.grossik.farmcraft.block; import java.util.List; import java.util.Map; import java.util.Map.Entry; import javax.annotation.Nullable; import com.google.common.collect.Lists; import com.google.common.collect.Maps; import cz.grossik.farmcraft.recipe.FarmCraftRecipeType; import cz.grossik.farmcraft.container.JuicerContainer; import cz.grossik.farmcraft.init.FarmCraftTileEntityTypes; import cz.grossik.farmcraft.init.ItemInit; import cz.grossik.farmcraft.recipe.AbstractJuicerRecipe; import net.minecraft.block.BlockState; import net.minecraft.block.BrewingStandBlock; import net.minecraft.entity.item.ExperienceOrbEntity; import net.minecraft.entity.player.PlayerEntity; import net.minecraft.entity.player.PlayerInventory; import net.minecraft.inventory.IRecipeHelperPopulator; import net.minecraft.inventory.IRecipeHolder; import net.minecraft.inventory.ISidedInventory; import net.minecraft.inventory.ItemStackHelper; import net.minecraft.inventory.container.Container; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.item.Items; import net.minecraft.item.crafting.IRecipe; import net.minecraft.item.crafting.IRecipeType; import net.minecraft.item.crafting.RecipeItemHelper; import net.minecraft.nbt.CompoundNBT; import net.minecraft.tileentity.ITickableTileEntity; import net.minecraft.tileentity.LockableTileEntity; import net.minecraft.util.Direction; import net.minecraft.util.IIntArray; import net.minecraft.util.IItemProvider; import net.minecraft.util.NonNullList; import net.minecraft.util.ResourceLocation; import net.minecraft.util.math.MathHelper; import net.minecraft.util.text.ITextComponent; import net.minecraft.util.text.TranslationTextComponent; import net.minecraftforge.common.capabilities.Capability; import net.minecraftforge.common.util.LazyOptional; import net.minecraftforge.items.CapabilityItemHandler; import net.minecraftforge.items.ItemStackHandler; public class JuicerTileEntity extends LockableTileEntity implements ISidedInventory, IRecipeHolder, IRecipeHelperPopulator, ITickableTileEntity { private static final int[] SLOTS_UP = new int[]{0}; private static final int[] SLOTS_DOWN = new int[]{2, 1}; private static final int[] SLOTS_HORIZONTAL = new int[]{1}; protected NonNullList<ItemStack> items = NonNullList.withSize(3, ItemStack.EMPTY); private int burnTime; private int recipesUsed; private int cookTime; private int cookTimeTotal; protected final IIntArray furnaceData = new IIntArray() { public int get(int index) { switch(index) { case 0: return JuicerTileEntity.this.burnTime; case 1: return JuicerTileEntity.this.recipesUsed; case 2: return JuicerTileEntity.this.cookTime; case 3: return JuicerTileEntity.this.cookTimeTotal; default: return 0; } } public void set(int index, int value) { switch(index) { case 0: JuicerTileEntity.this.burnTime = value; break; case 1: JuicerTileEntity.this.recipesUsed = value; break; case 2: JuicerTileEntity.this.cookTime = value; break; case 3: JuicerTileEntity.this.cookTimeTotal = value; } } public int size() { return 4; } }; private final Map<ResourceLocation, Integer> field_214022_n = Maps.newHashMap(); protected final IRecipeType<?> recipeType; public JuicerTileEntity() { super(FarmCraftTileEntityTypes.juicer.get()); this.recipeType = FarmCraftRecipeType.JUICER_RECIPE; } protected ITextComponent getDefaultName() { return new TranslationTextComponent("farmcraft.container.juicer"); } protected Container createMenu(int id, PlayerInventory player) { return new JuicerContainer(id, player, this, this.furnaceData); } @Deprecated //Forge - get burn times by calling ForgeHooks#getBurnTime(ItemStack) public static Map<Item, Integer> getBurnTimes() { Map<Item, Integer> map = Maps.newLinkedHashMap(); addItemBurnTime(map, ItemInit.juice_glass, 200); return map; } private static void addItemBurnTime(Map<Item, Integer> map, IItemProvider itemProvider, int burnTimeIn) { map.put(itemProvider.asItem(), burnTimeIn); } public boolean isBurning() { return this.burnTime > 0; } public void read(CompoundNBT compound) { super.read(compound); this.items = NonNullList.withSize(this.getSizeInventory(), ItemStack.EMPTY); ItemStackHelper.loadAllItems(compound, this.items); this.burnTime = compound.getInt("BurnTime"); this.cookTime = compound.getInt("CookTime"); this.cookTimeTotal = compound.getInt("CookTimeTotal"); this.recipesUsed = this.getBurnTime(this.items.get(1)); int i = compound.getShort("RecipesUsedSize"); for(int j = 0; j < i; ++j) { ResourceLocation resourcelocation = new ResourceLocation(compound.getString("RecipeLocation" + j)); int k = compound.getInt("RecipeAmount" + j); this.field_214022_n.put(resourcelocation, k); } } public CompoundNBT write(CompoundNBT compound) { super.write(compound); compound.putInt("BurnTime", this.burnTime); compound.putInt("CookTime", this.cookTime); compound.putInt("CookTimeTotal", this.cookTimeTotal); ItemStackHelper.saveAllItems(compound, this.items); compound.putShort("RecipesUsedSize", (short)this.field_214022_n.size()); int i = 0; for(Entry<ResourceLocation, Integer> entry : this.field_214022_n.entrySet()) { compound.putString("RecipeLocation" + i, entry.getKey().toString()); compound.putInt("RecipeAmount" + i, entry.getValue()); ++i; } return compound; } public void tick() { boolean flag = this.isBurning(); boolean flag1 = false; if (this.isBurning()) { --this.burnTime; } if (!this.world.isRemote) { ItemStack itemstack = this.items.get(1); if (this.isBurning() || !itemstack.isEmpty() && !this.items.get(0).isEmpty()) { IRecipe<?> irecipe = this.world.getRecipeManager().getRecipe((IRecipeType<AbstractJuicerRecipe>)this.recipeType, this, this.world).orElse(null); if (!this.isBurning() && this.canSmelt(irecipe)) { this.burnTime = this.getBurnTime(itemstack); this.recipesUsed = this.burnTime; if (this.isBurning()) { flag1 = true; if (itemstack.hasContainerItem()) this.items.set(1, itemstack.getContainerItem()); else if (!itemstack.isEmpty()) { Item item = itemstack.getItem(); //itemstack.shrink(1); if (itemstack.isEmpty()) { this.items.set(1, itemstack.getContainerItem()); } } } } if (this.isBurning() && this.canSmelt(irecipe)) { ++this.cookTime; if (this.cookTime == this.cookTimeTotal) { this.cookTime = 0; this.cookTimeTotal = this.func_214005_h(); this.func_214007_c(irecipe); flag1 = true; } } else { this.cookTime = 0; this.burnTime = 0; } } else if (!this.isBurning() && this.cookTime > 0) { this.cookTime = MathHelper.clamp(this.cookTime - 2, 0, this.cookTimeTotal); } if (flag != this.isBurning()) { flag1 = true; this.world.setBlockState(this.pos, this.world.getBlockState(this.pos).with(JuicerBlock.VYPALUJE, Boolean.valueOf(this.isBurning())), 3); } BlockState blockstate = this.world.getBlockState(this.getPos()); if (!(blockstate.getBlock() instanceof JuicerBlock)) { return; } if(itemstack.getItem() != ItemInit.juice_glass) { blockstate = blockstate.with(JuicerBlock.HAS_BOTTLE, false); } else { blockstate = blockstate.with(JuicerBlock.HAS_BOTTLE, true); } this.world.setBlockState(this.pos, blockstate, 3); } if (flag1) { this.markDirty(); } } protected boolean canSmelt(@Nullable IRecipe<?> recipeIn) { if (!this.items.get(0).isEmpty() && !this.items.get(1).isEmpty() && recipeIn != null) { ItemStack itemstack = recipeIn.getRecipeOutput(); if (itemstack.isEmpty()) { return false; } else { ItemStack itemstack1 = this.items.get(2); if (itemstack1.isEmpty()) { return true; } else if (!itemstack1.isItemEqual(itemstack)) { return false; } else if (itemstack1.getCount() + itemstack.getCount() <= this.getInventoryStackLimit() && itemstack1.getCount() + itemstack.getCount() <= itemstack1.getMaxStackSize()) { // Forge fix: make furnace respect stack sizes in furnace recipes return true; } else { return itemstack1.getCount() + itemstack.getCount() <= itemstack.getMaxStackSize(); // Forge fix: make furnace respect stack sizes in furnace recipes } } } else { return false; } } private void func_214007_c(@Nullable IRecipe<?> p_214007_1_) { if (p_214007_1_ != null && this.canSmelt(p_214007_1_)) { ItemStack itemstack = this.items.get(0); ItemStack itemstack1 = p_214007_1_.getRecipeOutput(); ItemStack itemstack2 = this.items.get(2); ItemStack itemstack3 = this.items.get(1); if (itemstack2.isEmpty()) { this.items.set(2, itemstack1.copy()); } else if (itemstack2.getItem() == itemstack1.getItem()) { itemstack2.grow(itemstack1.getCount()); } if (!this.world.isRemote) { this.setRecipeUsed(p_214007_1_); } itemstack.shrink(1); itemstack3.shrink(1); } } protected int getBurnTime(ItemStack p_213997_1_) { Item item = p_213997_1_.getItem(); if (p_213997_1_.isEmpty()) { return 0; } else { if(getBurnTimes().containsKey(item)) { return getBurnTimes().get(item); } else { return 0; } } } /*protected int getBurnTime(ItemStack p_213997_1_) { if (p_213997_1_.isEmpty()) { return 0; } else { Item item = p_213997_1_.getItem(); if(item == ItemInit.juice_glass) { return 200; } else { return 0; } //return net.minecraftforge.common.ForgeHooks.getBurnTime(p_213997_1_); } }*/ protected int func_214005_h() { return this.world.getRecipeManager().getRecipe((IRecipeType<AbstractJuicerRecipe>)this.recipeType, this, this.world).map(AbstractJuicerRecipe::getCookTime).orElse(200); } public int[] getSlotsForFace(Direction side) { if (side == Direction.DOWN) { return SLOTS_DOWN; } else { return side == Direction.UP ? SLOTS_UP : SLOTS_HORIZONTAL; } } public boolean canInsertItem(int index, ItemStack itemStackIn, @Nullable Direction direction) { return this.isItemValidForSlot(index, itemStackIn); } public boolean canExtractItem(int index, ItemStack stack, Direction direction) { if (direction == Direction.DOWN && index == 1) { Item item = stack.getItem(); if (item != Items.WATER_BUCKET && item != Items.BUCKET) { return false; } } return true; } public int getSizeInventory() { return this.items.size(); } public boolean isEmpty() { for(ItemStack itemstack : this.items) { if (!itemstack.isEmpty()) { return false; } } return true; } public ItemStack getStackInSlot(int index) { return this.items.get(index); } public ItemStack decrStackSize(int index, int count) { return ItemStackHelper.getAndSplit(this.items, index, count); } public ItemStack removeStackFromSlot(int index) { return ItemStackHelper.getAndRemove(this.items, index); } public void setInventorySlotContents(int index, ItemStack stack) { ItemStack itemstack = this.items.get(index); boolean flag = !stack.isEmpty() && stack.isItemEqual(itemstack) && ItemStack.areItemStackTagsEqual(stack, itemstack); this.items.set(index, stack); if (stack.getCount() > this.getInventoryStackLimit()) { stack.setCount(this.getInventoryStackLimit()); } if (index == 0 && !flag) { this.cookTimeTotal = this.func_214005_h(); this.cookTime = 0; this.markDirty(); } } public boolean isUsableByPlayer(PlayerEntity player) { if (this.world.getTileEntity(this.pos) != this) { return false; } else { return player.getDistanceSq((double)this.pos.getX() + 0.5D, (double)this.pos.getY() + 0.5D, (double)this.pos.getZ() + 0.5D) <= 64.0D; } } public boolean isItemValidForSlot(int index, ItemStack stack) { if (index == 2) { return false; } else if (index != 1) { return true; } else { ItemStack itemstack = this.items.get(1); if(itemstack.getItem() == ItemInit.juice_glass) { return true; } else { return false; } } } public void clear() { this.items.clear(); } public void setRecipeUsed(@Nullable IRecipe<?> recipe) { if (recipe != null) { this.field_214022_n.compute(recipe.getId(), (p_214004_0_, p_214004_1_) -> { return 1 + (p_214004_1_ == null ? 0 : p_214004_1_); }); } } @Nullable public IRecipe<?> getRecipeUsed() { return null; } public void onCrafting(PlayerEntity player) { } public void func_213995_d(PlayerEntity p_213995_1_) { List<IRecipe<?>> list = Lists.newArrayList(); for(Entry<ResourceLocation, Integer> entry : this.field_214022_n.entrySet()) { p_213995_1_.world.getRecipeManager().getRecipe(entry.getKey()).ifPresent((p_213993_3_) -> { list.add(p_213993_3_); func_214003_a(p_213995_1_, entry.getValue(), ((AbstractJuicerRecipe)p_213993_3_).getExperience()); }); } p_213995_1_.unlockRecipes(list); this.field_214022_n.clear(); } private static void func_214003_a(PlayerEntity p_214003_0_, int p_214003_1_, float p_214003_2_) { if (p_214003_2_ == 0.0F) { p_214003_1_ = 0; } else if (p_214003_2_ < 1.0F) { int i = MathHelper.floor((float)p_214003_1_ * p_214003_2_); if (i < MathHelper.ceil((float)p_214003_1_ * p_214003_2_) && Math.random() < (double)((float)p_214003_1_ * p_214003_2_ - (float)i)) { ++i; } p_214003_1_ = i; } while(p_214003_1_ > 0) { int j = ExperienceOrbEntity.getXPSplit(p_214003_1_); p_214003_1_ -= j; p_214003_0_.world.addEntity(new ExperienceOrbEntity(p_214003_0_.world, p_214003_0_.func_226277_ct_(), p_214003_0_.func_226278_cu_() + 0.5D, p_214003_0_.func_226281_cx_() + 0.5D, j)); } } public void fillStackedContents(RecipeItemHelper helper) { for(ItemStack itemstack : this.items) { helper.accountStack(itemstack); } } net.minecraftforge.common.util.LazyOptional<? extends net.minecraftforge.items.IItemHandler>[] handlers = net.minecraftforge.items.wrapper.SidedInvWrapper.create(this, Direction.UP, Direction.DOWN, Direction.NORTH); @Override public <T> net.minecraftforge.common.util.LazyOptional<T> getCapability(net.minecraftforge.common.capabilities.Capability<T> capability, @Nullable Direction facing) { if (!this.removed && facing != null && capability == net.minecraftforge.items.CapabilityItemHandler.ITEM_HANDLER_CAPABILITY) { if (facing == Direction.UP) return handlers[0].cast(); else if (facing == Direction.DOWN) return handlers[1].cast(); else return handlers[2].cast(); } return super.getCapability(capability, facing); } /** * invalidates a tile entity */ @Override public void remove() { super.remove(); for (int x = 0; x < handlers.length; x++) handlers[x].invalidate(); } public NonNullList<ItemStack> getInventory() { return this.items; } } Renderer: public class TileEntityJuicerRenderer extends TileEntityRenderer<JuicerTileEntity> { public TileEntityJuicerRenderer(TileEntityRendererDispatcher p_i226006_1_) { super(p_i226006_1_); } @Override public void func_225616_a_(JuicerTileEntity te, float p_225616_2_, MatrixStack p_225616_3_, IRenderTypeBuffer p_225616_4_, int p_225616_5_, int p_225616_6_) { BlockState blockstate = te.getBlockState(); if (!(blockstate.getBlock() instanceof JuicerBlock)) { return; } if(blockstate.get(JuicerBlock.VYPALUJE)) { System.err.println(te.getInventory()); } } } Console still log: [1 air, 1 air, 1 air] Anyone know what's wrong?
-
Thank you.
-
I tried it, but same.
-
Hi I have a problem with my new farmland block. https://i.imgur.com/HZe7Oo4.png My code: package com.example.examplemod.block; import java.util.Random; import com.example.examplemod.TestBlocks; import net.minecraft.block.Block; import net.minecraft.block.BlockDirt; import net.minecraft.block.material.MapColor; import net.minecraft.block.material.Material; import net.minecraft.block.properties.IProperty; import net.minecraft.block.properties.PropertyInteger; import net.minecraft.block.state.BlockFaceShape; import net.minecraft.block.state.BlockStateContainer; import net.minecraft.block.state.IBlockState; import net.minecraft.entity.Entity; import net.minecraft.init.Blocks; import net.minecraft.item.Item; import net.minecraft.util.BlockRenderLayer; import net.minecraft.util.EnumFacing; import net.minecraft.util.math.AxisAlignedBB; import net.minecraft.util.math.BlockPos; import net.minecraft.world.IBlockAccess; import net.minecraft.world.World; import net.minecraftforge.fml.relauncher.Side; import net.minecraftforge.fml.relauncher.SideOnly; public class BlockFarmlandGrass extends Block { public static final PropertyInteger MOISTURE = PropertyInteger.create("moisture", 0, 7); protected static final AxisAlignedBB FARMLAND_AABB = new AxisAlignedBB(0.0D, 0.0D, 0.0D, 1.0D, 1.0D, 1.0D); protected static final AxisAlignedBB field_194405_c = new AxisAlignedBB(0.0D, 1.0D, 0.0D, 1.0D, 1.0D, 1.0D); public BlockFarmlandGrass() { super(Material.GRASS, MapColor.GRASS); this.setDefaultState(this.blockState.getBaseState().withProperty(MOISTURE, Integer.valueOf(0))); this.setTickRandomly(true); this.setLightOpacity(255); } public AxisAlignedBB getBoundingBox(IBlockState state, IBlockAccess source, BlockPos pos) { return FARMLAND_AABB; } public void updateTick(World worldIn, BlockPos pos, IBlockState state, Random rand) { int i = ((Integer)state.getValue(MOISTURE)).intValue(); if (!this.hasWater(worldIn, pos) && !worldIn.isRainingAt(pos.up())) { if (i > 0) { worldIn.setBlockState(pos, state.withProperty(MOISTURE, Integer.valueOf(i - 1)), 2); } else if (!this.hasCrops(worldIn, pos)) { turnToDirt(worldIn, pos); } } else if (i < 7) { worldIn.setBlockState(pos, state.withProperty(MOISTURE, Integer.valueOf(7)), 2); } } /** * Block's chance to react to a living entity falling on it. */ public void onFallenUpon(World worldIn, BlockPos pos, Entity entityIn, float fallDistance) { if (!worldIn.isRemote && entityIn.canTrample(worldIn, this, pos, fallDistance)) // Forge: Move logic to Entity#canTrample { turnToDirt(worldIn, pos); } super.onFallenUpon(worldIn, pos, entityIn, fallDistance); } protected static void turnToDirt(World p_190970_0_, BlockPos worldIn) { p_190970_0_.setBlockState(worldIn, Blocks.DIRT.getDefaultState()); AxisAlignedBB axisalignedbb = field_194405_c.offset(worldIn); for (Entity entity : p_190970_0_.getEntitiesWithinAABBExcludingEntity((Entity)null, axisalignedbb)) { double d0 = Math.min(axisalignedbb.maxY - axisalignedbb.minY, axisalignedbb.maxY - entity.getEntityBoundingBox().minY); entity.setPositionAndUpdate(entity.posX, entity.posY + d0 + 0.001D, entity.posZ); } } private boolean hasCrops(World worldIn, BlockPos pos) { Block block = worldIn.getBlockState(pos.up()).getBlock(); return block instanceof net.minecraftforge.common.IPlantable && canSustainPlant(worldIn.getBlockState(pos), worldIn, pos, net.minecraft.util.EnumFacing.UP, (net.minecraftforge.common.IPlantable)block); } private boolean hasWater(World worldIn, BlockPos pos) { for (BlockPos.MutableBlockPos blockpos$mutableblockpos : BlockPos.getAllInBoxMutable(pos.add(-4, 0, -4), pos.add(4, 1, 4))) { if (worldIn.getBlockState(blockpos$mutableblockpos).getMaterial() == Material.WATER) { return true; } } return false; } /** * Called when a neighboring block was changed and marks that this state should perform any checks during a neighbor * change. Cases may include when redstone power is updated, cactus blocks popping off due to a neighboring solid * block, etc. */ public void neighborChanged(IBlockState state, World worldIn, BlockPos pos, Block blockIn, BlockPos fromPos) { super.neighborChanged(state, worldIn, pos, blockIn, fromPos); if (worldIn.getBlockState(pos.up()).getMaterial().isSolid()) { turnToDirt(worldIn, pos); } } /** * Called after the block is set in the Chunk data, but before the Tile Entity is set */ public void onBlockAdded(World worldIn, BlockPos pos, IBlockState state) { super.onBlockAdded(worldIn, pos, state); if (worldIn.getBlockState(pos.up()).getMaterial().isSolid()) { turnToDirt(worldIn, pos); } } @SideOnly(Side.CLIENT) public boolean shouldSideBeRendered(IBlockState blockState, IBlockAccess blockAccess, BlockPos pos, EnumFacing side) { switch (side) { case UP: return true; case NORTH: case SOUTH: case WEST: case EAST: IBlockState iblockstate = blockAccess.getBlockState(pos.offset(side)); Block block = iblockstate.getBlock(); return !iblockstate.isOpaqueCube() && block != TestBlocks.farmland_grass && block != Blocks.GRASS_PATH; default: return super.shouldSideBeRendered(blockState, blockAccess, pos, side); } } /** * Get the Item that this Block should drop when harvested. */ public Item getItemDropped(IBlockState state, Random rand, int fortune) { return Blocks.DIRT.getItemDropped(Blocks.DIRT.getDefaultState().withProperty(BlockDirt.VARIANT, BlockDirt.DirtType.DIRT), rand, fortune); } /** * Convert the given metadata into a BlockState for this Block */ public IBlockState getStateFromMeta(int meta) { return this.getDefaultState().withProperty(MOISTURE, Integer.valueOf(meta & 7)); } /** * Convert the BlockState into the correct metadata value */ public int getMetaFromState(IBlockState state) { return ((Integer)state.getValue(MOISTURE)).intValue(); } protected BlockStateContainer createBlockState() { return new BlockStateContainer(this, new IProperty[] {MOISTURE}); } @Override @SideOnly(Side.CLIENT) public BlockRenderLayer getBlockLayer() { return BlockRenderLayer.CUTOUT_MIPPED; } public BlockFaceShape getBlockFaceShape(IBlockAccess p_193383_1_, IBlockState p_193383_2_, BlockPos p_193383_3_, EnumFacing p_193383_4_) { return p_193383_4_ == EnumFacing.DOWN ? BlockFaceShape.SOLID : BlockFaceShape.UNDEFINED; } } Json: { "variants": { "moisture=0": { "model": "test:grassfarmland_dry" }, "moisture=1": { "model": "test:grassfarmland_dry" }, "moisture=2": { "model": "test:grassfarmland_dry" }, "moisture=3": { "model": "test:grassfarmland_dry" }, "moisture=4": { "model": "test:grassfarmland_dry" }, "moisture=5": { "model": "test:grassfarmland_dry" }, "moisture=6": { "model": "test:grassfarmland_dry" }, "moisture=7": { "model": "test:grassfarmland_moist" } } } { "parent": "test:block/farmland", "textures": { "dirt": "blocks/dirt", "strana": "blocks/grass_side", "top": "test:blocks/grassfarmland_dry" } } { "parent": "test:block/farmland", "textures": { "dirt": "blocks/dirt", "strana": "blocks/grass_side", "top": "test:blocks/grassfarmland_wet" } } I don't know what to do.
-
In my mod. Trade List: and register: VillagerRegistry.VillagerCareer career_fc2_farmer = new VillagerRegistry.VillagerCareer(villagerProfession_farmer, Main.MODID + ".farmer"); career_fc2_farmer.addTrade(1, new FC2VillagerTrades.ItemstackForSeeds(ItemHandler.BarleySeeds, new EntityVillager.PriceInfo(1, 2));
-
Thanks, it works.
-
Sorry. But when I give GlStateManager.translate(-1.0F, 0.0F, -0.5F). It olny works in one direction.
-
No.
-
I figured it out myself. package cz.grossik.farmcraft2.racks; import javax.annotation.Nullable; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.entity.player.InventoryPlayer; import net.minecraft.inventory.Container; import net.minecraft.inventory.ISidedInventory; import net.minecraft.inventory.ItemStackHelper; import net.minecraft.item.ItemStack; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.nbt.NBTTagList; import net.minecraft.network.NetworkManager; import net.minecraft.network.play.server.SPacketUpdateTileEntity; import net.minecraft.tileentity.TileEntityLockable; import net.minecraft.util.EnumFacing; import net.minecraft.util.ITickable; import net.minecraftforge.common.capabilities.Capability; import net.minecraftforge.items.CapabilityItemHandler; import net.minecraftforge.items.wrapper.SidedInvWrapper; public class TileEntityRacks extends TileEntityLockable implements ITickable, ISidedInventory { private ItemStack[] wineItemStacks = new ItemStack[9]; private String furnaceCustomName; private NBTTagCompound update_packet; public int getSizeInventory() { return this.wineItemStacks.length; } public ItemStack getStackInSlot(int index) { return this.wineItemStacks[index]; } @Nullable public ItemStack decrStackSize(int index, int count) { return ItemStackHelper.getAndSplit(this.wineItemStacks, index, count); } @Nullable public ItemStack removeStackFromSlot(int index) { return ItemStackHelper.getAndRemove(this.wineItemStacks, index); } public void setCustomInventoryName(String p_145951_1_) { this.furnaceCustomName = p_145951_1_; } public void setInventorySlotContents(int index, ItemStack stack) { boolean flag = stack != null && stack.isItemEqual(this.wineItemStacks[index]) && ItemStack.areItemStackTagsEqual(stack, this.wineItemStacks[index]); this.wineItemStacks[index] = stack; if (stack != null && stack.stackSize > this.getInventoryStackLimit()) { stack.stackSize = this.getInventoryStackLimit(); } } @Override public int getInventoryStackLimit() { return 1; } @Override public void readFromNBT(NBTTagCompound compound) { super.readFromNBT(compound); int i; for(i = 0; i < getSizeInventory(); i++) { NBTTagCompound tag = (NBTTagCompound)compound.getTag("Item_" + String.valueOf(i)); if(tag != null) { ItemStack stack = null; if(!tag.getBoolean("empty")) { stack = ItemStack.loadItemStackFromNBT(tag); } wineItemStacks[i] = stack; } } } protected final void updateInventoryItem(int slot) { if(worldObj.isRemote) { return; } if(update_packet == null) { update_packet = new NBTTagCompound(); super.writeToNBT(update_packet); } writeInventoryItemToNBT(update_packet,slot); } protected final void writeInventoryItemToNBT(NBTTagCompound compound,int slot) { ItemStack is = wineItemStacks[slot]; NBTTagCompound tag = new NBTTagCompound(); if(is != null) { tag.setBoolean("empty", false); is.writeToNBT(tag); } else { tag.setBoolean("empty", true); } compound.setTag("Item_" + String.valueOf(slot), tag); } protected void writeTileToNBT(NBTTagCompound compound) { super.writeToNBT(compound); } @Override public NBTTagCompound writeToNBT(NBTTagCompound compound) { if(compound == null) { compound = new NBTTagCompound(); } int i; super.writeToNBT(compound); for(i = 0; i < getSizeInventory(); i++) { writeInventoryItemToNBT(compound,i); } return compound; } public boolean isUseableByPlayer(EntityPlayer player) { return this.worldObj.getTileEntity(this.pos) != this ? false : player.getDistanceSq((double)this.pos.getX() + 0.5D, (double)this.pos.getY() + 0.5D, (double)this.pos.getZ() + 0.5D) <= 64.0D; } public void openInventory(EntityPlayer player) { } public void closeInventory(EntityPlayer player) { } public boolean isItemValidForSlot(int index, ItemStack stack) { return false; } @Override public int getField(int id) { return 0; } @Override public void setField(int id, int value) { } @Override public int getFieldCount() { return 0; } public void clear() { for (int i = 0; i < this.wineItemStacks.length; ++i) { this.wineItemStacks[i] = null; } } public String getName() { return this.hasCustomName() ? this.furnaceCustomName : "Racks"; } public boolean hasCustomName() { return this.furnaceCustomName != null && !this.furnaceCustomName.isEmpty(); } @Override public Container createContainer(InventoryPlayer playerInventory, EntityPlayer playerIn) { return new ContainerRacks(playerInventory, this); } @Override public String getGuiID() { return "10"; } @Override public int[] getSlotsForFace(EnumFacing side) { return null; } @Override public boolean canInsertItem(int index, ItemStack itemStackIn, EnumFacing direction) { return this.isItemValidForSlot(index, itemStackIn); } public boolean canExtractItem(int index, ItemStack stack, EnumFacing direction) { return true; } @Override public void update() { } public boolean Check0(){ if(wineItemStacks[0] != null) { return true; } else { return false; } } public boolean Check1(){ if(wineItemStacks[1] != null) { return true; } else { return false; } } public boolean Check2(){ if(wineItemStacks[2] != null) { return true; } else { return false; } } public boolean Check3(){ if(wineItemStacks[3] != null) { return true; } else { return false; } } public boolean Check4(){ if(wineItemStacks[4] != null) { return true; } else { return false; } } public boolean Check5(){ if(wineItemStacks[5] != null) { return true; } else { return false; } } public boolean Check6(){ if(wineItemStacks[6] != null) { return true; } else { return false; } } public boolean Check7(){ if(wineItemStacks[7] != null) { return true; } else { return false; } } public boolean Check8(){ if(wineItemStacks[8] != null) { return true; } else { return false; } } @SuppressWarnings("unchecked") @Override public <T> T getCapability(Capability<T> capability, EnumFacing facing) { if (facing != null && capability == CapabilityItemHandler.ITEM_HANDLER_CAPABILITY) { return (T) new SidedInvWrapper(this, facing); } return super.getCapability(capability, facing); } @Override public final SPacketUpdateTileEntity getUpdatePacket() { NBTTagCompound nbt = new NBTTagCompound(); writeToNBT(nbt); return new SPacketUpdateTileEntity(getPos(), 0, nbt); } @Override public NBTTagCompound getUpdateTag() { return writeToNBT(null); } @Override public final void onDataPacket(NetworkManager net, SPacketUpdateTileEntity pkt) { super.onDataPacket(net, pkt); if(worldObj.isRemote) { readFromNBT(pkt.getNbtCompound()); } } } But I have problem with rotation Wine model. package cz.grossik.farmcraft2.racks; import org.lwjgl.opengl.GL11; import cz.grossik.farmcraft2.Main; import net.minecraft.client.renderer.GlStateManager; import net.minecraft.client.renderer.tileentity.TileEntitySpecialRenderer; import net.minecraft.util.ResourceLocation; import net.minecraftforge.fml.relauncher.Side; import net.minecraftforge.fml.relauncher.SideOnly; @SideOnly(Side.CLIENT) public class RenderRacks extends TileEntitySpecialRenderer<TileEntityRacks> { private static final ModelWine0 model0 = new ModelWine0(); private static final ModelWine1 model1 = new ModelWine1(); private static final ModelWine2 model2 = new ModelWine2(); private static final ModelWine3 model3 = new ModelWine3(); private static final ModelWine4 model4 = new ModelWine4(); private static final ModelWine5 model5 = new ModelWine5(); private static final ModelWine6 model6 = new ModelWine6(); private static final ModelWine7 model7 = new ModelWine7(); private static final ModelWine8 model8 = new ModelWine8(); private static final ResourceLocation tWine = new ResourceLocation(Main.MODID + ":textures/wine.png"); @Override public void renderTileEntityAt(TileEntityRacks te, double x, double y, double z, float partialTicks, int destroyStage) { GlStateManager.pushMatrix(); GlStateManager.translate(x, y, z); int rotationAngle = 0; int metadata = te.getBlockMetadata(); if (metadata%4 == 0) { rotationAngle = 270; } if (metadata%4 == 1) { rotationAngle = 90; } if (metadata%4 == 2) { rotationAngle = 180; } if (metadata%4 == 3) { rotationAngle = 0; } GL11.glRotatef(rotationAngle, 0, 1, 0); ResourceLocation texture0 = tWine; bindTexture(texture0); GlStateManager.pushMatrix(); if(te.Check0() == true) { model0.render(); } if(te.Check1() == true) { model1.render(); } if(te.Check2() == true) { model2.render(); } if(te.Check3() == true) { model3.render(); } if(te.Check4() == true) { model4.render(); } if(te.Check5() == true) { model5.render(); } if(te.Check6() == true) { model6.render(); } if(te.Check7() == true) { model7.render(); } if(te.Check8() == true) { model8.render(); } GlStateManager.popMatrix(); GlStateManager.popMatrix(); } }
-
What? I don't know how to override it.
-
Yes, I don't know how.
-
Nobody help?
-
Whenever I connect the world, rendering disappears. When I click on the block to appear. TileEntity: Render: Sorry for my bad English.