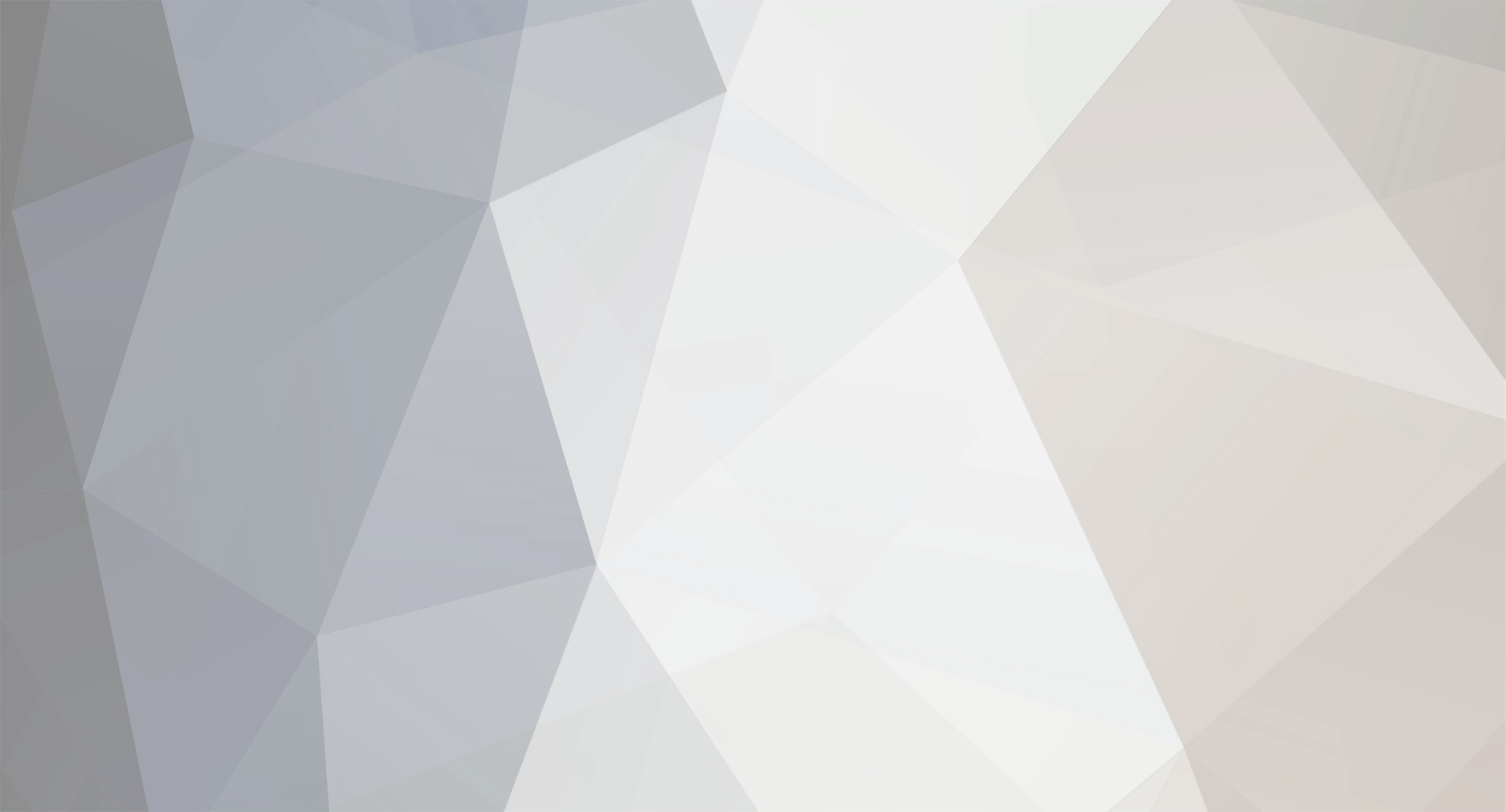
BoonieQuafter-CrAfTeR
Members-
Posts
337 -
Joined
-
Last visited
Everything posted by BoonieQuafter-CrAfTeR
-
i know this is completely and utterly wrong but what do I do from here, plus im having trouble finding examples in vanilla(the healthboost potion effect does help me) or the internet. How/What should I do to just + 10 to a health modifier. event.player.getEntityAttribute(SharedMonsterAttributes.maxHealth).applyModifier(new AttributeModifier(null, iconString, event.player.getMaxHealth() + 10D, 10)); yeah don't even say anything about the code.. but seriously how do I apply an attribute.
-
yeah so It worked slightly: here is every class im hoping this will help me solve the problem. MainRegistry: package com.OlympiansMod.Main; import net.minecraft.client.renderer.entity.RenderSnowball; import net.minecraftforge.event.entity.living.LivingDeathEvent; import com.OlympiansMod.Block.ModBlocks; import com.OlympiansMod.Item.DnaPosideonInfused; import com.OlympiansMod.Item.DnaStats; import com.OlympiansMod.Item.DnaStats2; import com.OlympiansMod.Item.DnaZeusInfused; import com.OlympiansMod.Item.ModItems; import com.OlympiansMod.creativetabs.MCreativeTabs; import com.OlympiansMod.entity.EntityCell; import com.OlympiansMod.entity.EntityGreekFire; import com.OlympiansMod.entity.MEntity; import com.OlympiansMod.lib.Refstrings; import com.OlympiansMod.world.MWorld; import cpw.mods.fml.client.registry.RenderingRegistry; import cpw.mods.fml.common.FMLCommonHandler; import cpw.mods.fml.common.Mod; import cpw.mods.fml.common.Mod.EventHandler; import cpw.mods.fml.common.Mod.Instance; import cpw.mods.fml.common.SidedProxy; import cpw.mods.fml.common.event.FMLInitializationEvent; import cpw.mods.fml.common.event.FMLPostInitializationEvent; import cpw.mods.fml.common.event.FMLPreInitializationEvent; import cpw.mods.fml.common.eventhandler.SubscribeEvent; import cpw.mods.fml.common.registry.GameRegistry; @Mod(modid = Refstrings.MODID , name = Refstrings.NAME , version = Refstrings.VERSION) public class MainRegistry { private static int modGuiIndex = 0; public static final int GUI_CUSTOM_INV = modGuiIndex++; @SidedProxy(clientSide = Refstrings.CLIENTSIDE , serverSide = Refstrings.SERVERSIDE) public static ServerProxy proxy; @Instance public static MainRegistry modInstance; @EventHandler public static void PreLoad(FMLPreInitializationEvent PreEvent) { MCreativeTabs.initialiseTabs(); ModBlocks.MainRegistry(); MEntity.MainRegistry(); ModItems.MainRegistry(); FMLCommonHandler.instance().bus().register(new DnaZeusInfused()); FMLCommonHandler.instance().bus().register(new DnaPosideonInfused()); MWorld.MainRegistry(); CraftingManager.mainRegistry(); } @EventHandler public static void Load(FMLInitializationEvent event) { proxy.registerRenderInfo(); } @EventHandler public static void PostLoad(FMLPostInitializationEvent PostEvent) { } } ItemClasses: package com.OlympiansMod.Item; import java.util.List; import com.sun.media.jfxmedia.events.PlayerEvent; import cpw.mods.fml.common.FMLCommonHandler; import cpw.mods.fml.common.eventhandler.SubscribeEvent; import cpw.mods.fml.common.gameevent.TickEvent.Phase; import cpw.mods.fml.common.gameevent.TickEvent.PlayerTickEvent; import net.minecraft.entity.Entity; import net.minecraft.entity.SharedMonsterAttributes; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.init.Items; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.potion.Potion; import net.minecraft.potion.PotionEffect; import net.minecraft.util.DamageSource; import net.minecraft.util.EnumChatFormatting; import net.minecraft.world.World; public class DnaZeusInfused extends Item { @SubscribeEvent public void playerTick(PlayerTickEvent event) { boolean hasItem = event.player.inventory.hasItem(ModItems.DnaZeusInfused); if (event.player.capabilities.isCreativeMode) { } else { if (hasItem == true) { event.player.capabilities.allowFlying = true; if (event.player.capabilities.isFlying) { if(!event.player.worldObj.isRemote){ event.player.getEntityAttribute(SharedMonsterAttributes.maxHealth).setBaseValue(40.0D); event.player.addPotionEffect(new PotionEffect( Potion.damageBoost.id, 1, 1)); event.player.addPotionEffect(new PotionEffect( Potion.resistance.id, 1, 1)); event.player.addPotionEffect(new PotionEffect( Potion.hunger.id, 3, 3)); } } else { if (hasItem == true) { if(!event.player.worldObj.isRemote){ event.player.getEntityAttribute(SharedMonsterAttributes.maxHealth).setBaseValue(30.0D); event.player.addPotionEffect(new PotionEffect(Potion.damageBoost.id, 0, 0)); event.player.addPotionEffect(new PotionEffect(Potion.resistance.id, 0, 0)); } } } } } if (event.player.capabilities.isCreativeMode) { } else { if (hasItem == false) { if(!event.player.worldObj.isRemote){ event.player.getEntityAttribute(SharedMonsterAttributes.maxHealth).removeAllModifiers(); event.player.getEntityAttribute(SharedMonsterAttributes.maxHealth).setBaseValue(20.0D); } event.player.capabilities.allowFlying = false; event.player.capabilities.isFlying = false; } if (event.player.isInWater() && hasItem == true) { if(!event.player.worldObj.isRemote){ event.player.addPotionEffect(new PotionEffect( Potion.weakness.id, 0, 0)); } } } } public ItemStack onItemRightClick(ItemStack stack, World world, EntityPlayer player) { if (!world.isRemote) { player.attackEntityFrom(DamageSource.causeIndirectMagicDamage(player, player), 10); player.addPotionEffect(new PotionEffect(Potion.blindness.id, 60, 5)); player.addPotionEffect(new PotionEffect(Potion.confusion.id, 200, 5)); player.addPotionEffect(new PotionEffect(Potion.wither.id, 60, 5)); player.inventory.addItemStackToInventory(new ItemStack( ModItems.DNAZeus)); return new ItemStack(ModItems.DnaInfuser); } return stack; } public void addInformation(ItemStack stack, EntityPlayer player, List list, boolean i) { list.add(EnumChatFormatting.AQUA + "Dna Infuser:"); list.add(" "); list.add(EnumChatFormatting.BLUE + "Mode:"); list.add(EnumChatFormatting.BLUE + "Actively Infusing:"); list.add(EnumChatFormatting.BLUE + "Dna of Zeus"); } } package com.OlympiansMod.Item; import java.util.List; import cpw.mods.fml.common.eventhandler.SubscribeEvent; import cpw.mods.fml.common.gameevent.TickEvent.PlayerTickEvent; import net.minecraft.entity.SharedMonsterAttributes; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.potion.Potion; import net.minecraft.potion.PotionEffect; import net.minecraft.util.DamageSource; import net.minecraft.util.EnumChatFormatting; import net.minecraft.world.World; public class DnaPosideonInfused extends Item{ @SubscribeEvent public void playerTick(PlayerTickEvent event) { boolean hasItem = event.player.inventory.hasItem(ModItems.DnaPosideonInfused); if (event.player.capabilities.isCreativeMode) { } else { if (hasItem == true) { if (event.player.isWet()) { if(!event.player.worldObj.isRemote){ event.player.getEntityAttribute(SharedMonsterAttributes.maxHealth).setBaseValue(45.0D); event.player.addPotionEffect(new PotionEffect(Potion.moveSpeed.id, 1, 1)); event.player.addPotionEffect(new PotionEffect(Potion.waterBreathing.id, 1, 1)); event.player.addPotionEffect(new PotionEffect(Potion.damageBoost.id, 3, 3)); event.player.addPotionEffect(new PotionEffect(Potion.resistance.id, 1, 1)); } } else { if (hasItem == true) { if(!event.player.worldObj.isRemote){ event.player.getEntityAttribute(SharedMonsterAttributes.maxHealth).setBaseValue(30.0D); event.player.addPotionEffect(new PotionEffect(Potion.damageBoost.id, 1, 1)); event.player.addPotionEffect(new PotionEffect(Potion.resistance.id, 0, 0)); } } } } } if (event.player.capabilities.isCreativeMode) { } else { if (hasItem == false) { if(!event.player.worldObj.isRemote){ event.player.getEntityAttribute(SharedMonsterAttributes.maxHealth).removeAllModifiers(); event.player.getEntityAttribute(SharedMonsterAttributes.maxHealth).setBaseValue(20.0D); } } if (event.player.isInWater() && hasItem == true) { if(!event.player.worldObj.isRemote){ event.player.addPotionEffect(new PotionEffect( Potion.regeneration.id, 1, 1)); } } } } public ItemStack onItemRightClick(ItemStack stack, World world, EntityPlayer player) { if (!world.isRemote) { player.attackEntityFrom( DamageSource.causeIndirectMagicDamage(player, player), 10); player.addPotionEffect(new PotionEffect(Potion.blindness.id, 60, 5)); player.addPotionEffect(new PotionEffect(Potion.confusion.id, 200, 5)); player.addPotionEffect(new PotionEffect(Potion.wither.id, 60, 5)); player.inventory.addItemStackToInventory(new ItemStack( ModItems.DNAPosideon)); return new ItemStack(ModItems.DnaInfuser); } return stack; } public void addInformation(ItemStack stack, EntityPlayer player, List list, boolean i) { list.add(EnumChatFormatting.AQUA + "Dna Infuser:"); list.add(" "); list.add(EnumChatFormatting.BLUE + "Mode:"); list.add(EnumChatFormatting.BLUE + "Actively Infusing:"); list.add(EnumChatFormatting.BLUE + "Dna of Posideon"); } } so yeah, the dnaposideoninfused works perfectly, problem is the dnaZeusInfused works, except for the health modifiers, and I can get the dnazeusInfused to work, but I have to place the event bus before the dna posideon infused. but then the dnaposideoninfused doesn't work. why is this?
-
everything is valid, except like I said earlier why, registering everything server side works just not with the event bus registered It works if the itemposideon is register after the zeus bus, and vis versa, its kinds hard to explain just look at the code. why is it doing this? it works perfectly if its registered second. and thanks about the potion effects, it works now, this is mainly about the health stuff though, meaning I just want to clarify that I don't think the main registry has anything to do with the potion effects.
-
I found a different problem, I think Im registrying my event busses wrong, what do you think, also registering one before another makes an effect, heres thee code: package com.OlympiansMod.Main; import net.minecraft.client.renderer.entity.RenderSnowball; import net.minecraftforge.event.entity.living.LivingDeathEvent; import com.OlympiansMod.Block.ModBlocks; import com.OlympiansMod.Item.DnaPosideonInfused; import com.OlympiansMod.Item.DnaZeusInfused; import com.OlympiansMod.Item.ModItems; import com.OlympiansMod.creativetabs.MCreativeTabs; import com.OlympiansMod.entity.EntityCell; import com.OlympiansMod.entity.EntityGreekFire; import com.OlympiansMod.entity.MEntity; import com.OlympiansMod.lib.Refstrings; import com.OlympiansMod.world.MWorld; import cpw.mods.fml.client.registry.RenderingRegistry; import cpw.mods.fml.common.FMLCommonHandler; import cpw.mods.fml.common.Mod; import cpw.mods.fml.common.Mod.EventHandler; import cpw.mods.fml.common.Mod.Instance; import cpw.mods.fml.common.SidedProxy; import cpw.mods.fml.common.event.FMLInitializationEvent; import cpw.mods.fml.common.event.FMLPostInitializationEvent; import cpw.mods.fml.common.event.FMLPreInitializationEvent; import cpw.mods.fml.common.eventhandler.SubscribeEvent; import cpw.mods.fml.common.registry.GameRegistry; @Mod(modid = Refstrings.MODID , name = Refstrings.NAME , version = Refstrings.VERSION) public class MainRegistry { private static int modGuiIndex = 0; public static final int GUI_CUSTOM_INV = modGuiIndex++; @SidedProxy(clientSide = Refstrings.CLIENTSIDE , serverSide = Refstrings.SERVERSIDE) public static ServerProxy proxy; @Instance public static MainRegistry modInstance; @EventHandler public static void PreLoad(FMLPreInitializationEvent PreEvent) { MCreativeTabs.initialiseTabs(); ModBlocks.MainRegistry(); MEntity.MainRegistry(); ModItems.MainRegistry(); FMLCommonHandler.instance().bus().register(new DnaPosideonInfused()); FMLCommonHandler.instance().bus().register(new DnaZeusInfused()); MWorld.MainRegistry(); CraftingManager.mainRegistry(); } @EventHandler public static void Load(FMLInitializationEvent event) { proxy.registerRenderInfo(); } @EventHandler public static void PostLoad(FMLPostInitializationEvent PostEvent) { } }
-
here is the new code: @SubscribeEvent public void playerTick(PlayerTickEvent event) { boolean hasItem = event.player.inventory.hasItem(ModItems.DnaPosideonInfused); if (event.player.capabilities.isCreativeMode) { } else { if (hasItem == true) { if (event.player.isWet()) { if(!event.player.worldObj.isRemote){ event.player.getEntityAttribute(SharedMonsterAttributes.maxHealth).setBaseValue(40.0D); event.player.addPotionEffect(new PotionEffect(Potion.moveSpeed.id, 1, 1)); event.player.addPotionEffect(new PotionEffect(Potion.waterBreathing.id, 1, 1)); event.player.addPotionEffect(new PotionEffect(Potion.damageBoost.id, 3, 3)); event.player.addPotionEffect(new PotionEffect(Potion.resistance.id, 1, 1)); } } else { if (hasItem == true) { if(!event.player.worldObj.isRemote){ event.player.getEntityAttribute(SharedMonsterAttributes.maxHealth).setBaseValue(30.0D); event.player.addPotionEffect(new PotionEffect(Potion.damageBoost.id, 1, 1)); event.player.addPotionEffect(new PotionEffect(Potion.resistance.id, 0, 0)); } } } } } if (event.player.capabilities.isCreativeMode) { } else { if (hasItem == false) { event.player.getEntityAttribute(SharedMonsterAttributes.maxHealth).setBaseValue(20.0D); } if (event.player.isInWater() && hasItem == true) { if(!event.player.worldObj.isRemote){ event.player.addPotionEffect(new PotionEffect( Potion.regeneration.id, 1, 1)); } } } }
-
so im making an item that does all the stuff below, problem is, adding health isn't working how should I fix this? @SubscribeEvent public void playerTick(PlayerTickEvent event) { boolean hasItem = event.player.inventory.hasItem(ModItems.DnaPosideonInfused); if (event.player.capabilities.isCreativeMode) { } else { if (hasItem == true) { if (event.player.isWet()) { event.player.getEntityAttribute(SharedMonsterAttributes.maxHealth).setBaseValue(40.0D); event.player.addPotionEffect(new PotionEffect(Potion.moveSpeed.id, 1, 1)); event.player.addPotionEffect(new PotionEffect(Potion.waterBreathing.id, 1, 1)); event.player.addPotionEffect(new PotionEffect(Potion.damageBoost.id, 3, 3)); event.player.addPotionEffect(new PotionEffect(Potion.resistance.id, 1, 1)); } else { if (hasItem == true) { event.player.getEntityAttribute(SharedMonsterAttributes.maxHealth).setBaseValue(30.0D); event.player.addPotionEffect(new PotionEffect(Potion.damageBoost.id, 1, 1)); event.player.addPotionEffect(new PotionEffect(Potion.resistance.id, 0, 0)); } } } } if (event.player.capabilities.isCreativeMode) { } else { if (hasItem == false) { event.player.getEntityAttribute(SharedMonsterAttributes.maxHealth).setBaseValue(20.0D); } if (event.player.isInWater() && hasItem == true) { event.player.addPotionEffect(new PotionEffect( Potion.regeneration.id, 1, 1)); } } }
-
[1.7.10] turn off allowFlying?
BoonieQuafter-CrAfTeR replied to BoonieQuafter-CrAfTeR's topic in Modder Support
yeah but it wont just let me add 1 to the time existed. I need an example, please. -
[1.7.10] turn off allowFlying?
BoonieQuafter-CrAfTeR replied to BoonieQuafter-CrAfTeR's topic in Modder Support
bump -
[1.7.10] turn off allowFlying?
BoonieQuafter-CrAfTeR replied to BoonieQuafter-CrAfTeR's topic in Modder Support
how do I get the next tick of the player may I ask? -
[1.7.10] turn off allowFlying?
BoonieQuafter-CrAfTeR replied to BoonieQuafter-CrAfTeR's topic in Modder Support
and what do I do if other flying items don't work now because of the event? also will this cause compatibility errors with other mods? Edit: Should I add potion effects on the server side? -
[1.7.10] turn off allowFlying?
BoonieQuafter-CrAfTeR replied to BoonieQuafter-CrAfTeR's topic in Modder Support
ok thanks it works now, well, not exactly the way I wanted it to, if your still flying it doesn't disable your ability to fly, but after you land it does. how should I fix this. -
[1.7.10] turn off allowFlying?
BoonieQuafter-CrAfTeR replied to BoonieQuafter-CrAfTeR's topic in Modder Support
where can I find a example of a playertickevent current code: public void playerTick(PlayerTickEvent event) { boolean hasItem = event.player.inventory.hasItem(ModItems.DnaZeusInfused); if (event.player.inventory.hasItem(ModItems.DnaZeusInfused)) { event.player.capabilities.allowFlying = true; if (hasItem == false) { event.player.capabilities.allowFlying = false; } } } -
[1.7.10] turn off allowFlying?
BoonieQuafter-CrAfTeR replied to BoonieQuafter-CrAfTeR's topic in Modder Support
I failed SORRY -
so im making a item that allows flying, but if the item is not in the inventory I want it to disable flying, and Ive tried doing this multiple ways. problem is not matter what I haven't been able to do it. so here is my last attempt at it. if there are any problems with the code please let me know. any help is greatly appreciated. thanks. onUpdate method: public class DnaZeusInfused extends Item { public void onUpdate(ItemStack stack, World world, Entity entity, int par4, boolean par5) { super.onUpdate(stack, world, entity, par4, par5); { EntityPlayer player = (EntityPlayer) entity; boolean hasItem = player.inventory.hasItem(ModItems.DnaZeusInfused); if (player.inventory.hasItem(ModItems.DnaZeusInfused)) { player.capabilities.allowFlying = true; } else if (hasItem == false) { player.capabilities.allowFlying = false; } if (player.capabilities.isFlying) { player.addPotionEffect(new PotionEffect(Potion.damageBoost.id, 1, 1)); player.addPotionEffect(new PotionEffect(Potion.resistance.id, 1, 1)); } else { player.addPotionEffect(new PotionEffect(Potion.damageBoost.id, 0, 0)); player.addPotionEffect(new PotionEffect(Potion.resistance.id, 0, 0)); } } }
-
Mob stuff. [1.7.10]
BoonieQuafter-CrAfTeR replied to BoonieQuafter-CrAfTeR's topic in Modder Support
a specific mob that is.