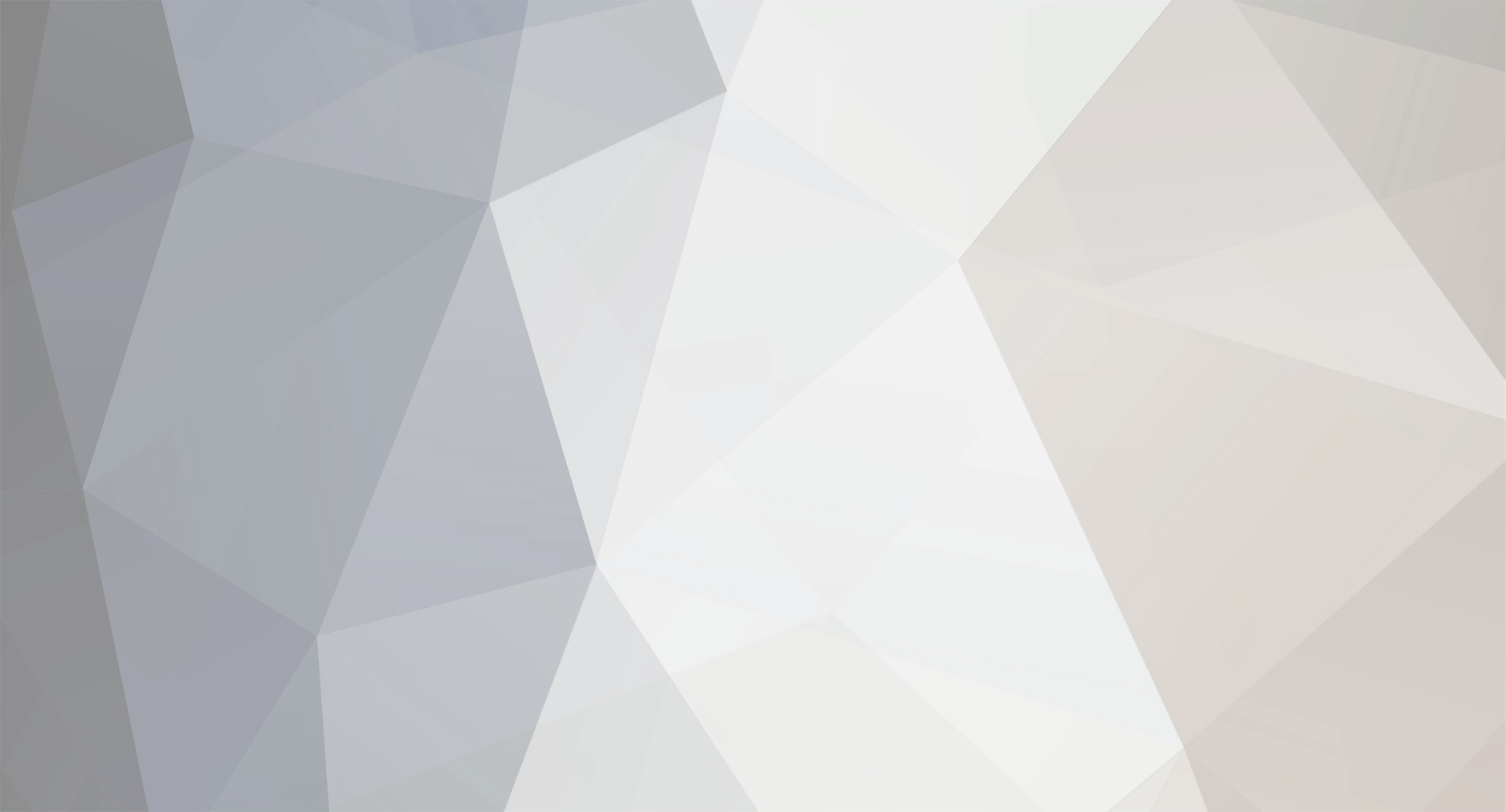
BoonieQuafter-CrAfTeR
Members-
Posts
337 -
Joined
-
Last visited
Everything posted by BoonieQuafter-CrAfTeR
-
[1.7.10] switching a mobs ai. [SOLVED] 100%
BoonieQuafter-CrAfTeR replied to BoonieQuafter-CrAfTeR's topic in Modder Support
well, I fixed the crash report but my bigger problem is, I don't want to make my own ai classes, and my I cant figure out how to make my entity attack on collide in the first place. package com.OlympiansMod.entity; import java.util.Iterator; import java.util.List; import com.OlympiansMod.Item.ModItems; import cpw.mods.fml.relauncher.Side; import cpw.mods.fml.relauncher.SideOnly; import net.minecraft.block.Block; import net.minecraft.entity.Entity; import net.minecraft.entity.EntityLiving; import net.minecraft.entity.EntityLivingBase; import net.minecraft.entity.IRangedAttackMob; import net.minecraft.entity.SharedMonsterAttributes; import net.minecraft.entity.ai.EntityAIArrowAttack; import net.minecraft.entity.ai.EntityAIAttackOnCollide; import net.minecraft.entity.ai.EntityAIHurtByTarget; import net.minecraft.entity.ai.EntityAILookIdle; import net.minecraft.entity.ai.EntityAIMoveTowardsTarget; import net.minecraft.entity.ai.EntityAINearestAttackableTarget; import net.minecraft.entity.ai.EntityAISwimming; import net.minecraft.entity.ai.EntityAIWander; import net.minecraft.entity.ai.EntityAIWatchClosest; import net.minecraft.entity.boss.BossStatus; import net.minecraft.entity.boss.EntityDragon; import net.minecraft.entity.boss.IBossDisplayData; import net.minecraft.entity.monster.EntityGolem; import net.minecraft.entity.monster.EntityMob; import net.minecraft.entity.monster.EntitySlime; import net.minecraft.entity.monster.EntityWitch; import net.minecraft.entity.monster.EntityZombie; import net.minecraft.entity.monster.IMob; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.entity.projectile.EntityPotion; import net.minecraft.init.Blocks; import net.minecraft.init.Items; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.potion.Potion; import net.minecraft.potion.PotionEffect; import net.minecraft.util.DamageSource; import net.minecraft.util.MathHelper; import net.minecraft.world.World; public class Zeus extends EntityGolem implements IBossDisplayData, IRangedAttackMob { private EntityAIArrowAttack aiArrowAttack = new EntityAIArrowAttack(this, 1.0D, 20, 60, 15.0F); private EntityAIAttackOnCollide aiAttackOnCollide = new EntityAIAttackOnCollide(this, EntityPlayer.class, 1.2D, false); private int attackTimer; private int healthupdate; private int attackMelee;; public Zeus(World world) { super(world); { this.getNavigator().setAvoidsWater(true); this.isImmuneToFire = true; this.setHealth(this.getMaxHealth()); this.setSize(1.1F, 2F); this.getNavigator().setAvoidsWater(true); this.tasks.addTask(1, new EntityAISwimming(this)); this.tasks.addTask(2, new EntityAIWander(this, 0.5D)); this.tasks.addTask(4, new EntityAIWatchClosest(this, EntityPlayer.class, 1.0F)); this.tasks.addTask(5, new EntityAIWatchClosest(this, EntityUndead.class, 1.0F)); this.tasks.addTask(6, new EntityAILookIdle(this)); this.tasks.addTask(7, new EntityAIMoveTowardsTarget(this, 0.7D, 100.0F)); this.targetTasks.addTask(1, new EntityAIHurtByTarget(this, true)); this.targetTasks.addTask(2, new EntityAINearestAttackableTarget(this, EntityPlayer.class, 0, true)); this.targetTasks.addTask(3, new EntityAINearestAttackableTarget(this, EntityLiving.class, 0, false, true, IMob.mobSelector)); if (world != null && !world.isRemote) { this.setCombatTask(); } } } public void onLivingUpdate() { super.onLivingUpdate(); BossStatus.setBossStatus(this, true); if (this.getHealth() <= 1000) { --this.healthupdate; } if (this.attackTimer > 0) { --this.attackTimer; } if (this.attackTimer > 0) { --this.attackMelee; } } { } public boolean isAIEnabled() { return true; } @Override protected void entityInit() { super.entityInit(); dataWatcher.addObject(21, (byte) 0); } public void setAggressive(boolean par2) { this.getDataWatcher().updateObject(21, Byte.valueOf((byte) (par2 ? 1 : 0))); } public boolean getAggressive() { return this.getDataWatcher().getWatchableObjectByte(21) == 1; } protected void addRandomArmor() { super.addRandomArmor(); this.setCurrentItemOrArmor(0, new ItemStack(ModItems.MasterBolt)); } protected void applyEntityAttributes() { super.applyEntityAttributes(); this.getEntityAttribute(SharedMonsterAttributes.followRange).setBaseValue(70.0D); this.getEntityAttribute(SharedMonsterAttributes.maxHealth).setBaseValue(5000.0D); this.getEntityAttribute(SharedMonsterAttributes.movementSpeed).setBaseValue(0.7D); } public void setCombatTask() { { this.tasks.addTask(4, this.aiArrowAttack); this.tasks.addTask(4, this.aiAttackOnCollide); } } @Override public boolean attackEntityAsMob(Entity entity) { this.attackTimer = 10; this.worldObj.setEntityState(this, (byte) 4); return entity.attackEntityFrom(DamageSource.causeMobDamage(this), (float) (7 + this.rand.nextInt(15))); } @Override public void attackEntityWithRangedAttack(EntityLivingBase entity, float par1) { this.attackTimer = 10; this.worldObj.setEntityState(this, (byte) 4); this.worldObj.playSoundEffect(this.posX, this.posY, this.posZ, "portal.portal", 10000.0F, 0.8F + this.rand.nextFloat() * 0.2F); EntityMasterBolt Bolt = new EntityMasterBolt(this.worldObj, this); Bolt.rotationPitch -= -20.0F; double d0 = entity.posX + entity.motionX - this.posX; double d1 = entity.posY + (double) entity.getEyeHeight() - 1.900000023841858D - this.posY; double d2 = entity.posZ + entity.motionZ - this.posZ; float f1 = MathHelper.sqrt_double(d0 * d0 + d2 * d2); Bolt.setThrowableHeading(d0, d1 + (double) (f1 * 0.2F), d2, 0.75F, 8.0F); this.worldObj.spawnEntityInWorld(Bolt); if (this.getHealth() <= 1500) { for (int i = 0; i < 10; i++) { this.worldObj.playSoundEffect(this.posX, this.posY, this.posZ, "portal.portal", 10000.0F, 0.8F + this.rand.nextFloat() * 0.2F); EntityThunderBolt Bolt2 = new EntityThunderBolt( this.worldObj, this); Bolt.rotationPitch -= -20.0F; double d0l = entity.posX + entity.motionX - this.posX + 1; double d1l = entity.posY + (double) entity.getEyeHeight() - 0.900000023841858D - this.posY; double d2l = entity.posZ + entity.motionZ - this.posZ; float f1l = MathHelper.sqrt_double(d0 * d0 + d2 * d2); Bolt2.setThrowableHeading(d0l, d1l + (double) (f1l * 0.2F), d2l, 0.75F, 8.0F); this.worldObj.spawnEntityInWorld(Bolt2); } } } @SideOnly(Side.CLIENT) public void handleHealthUpdate(byte p_70103_1_) { if (p_70103_1_ == 4) { this.attackTimer = 10; } else { super.handleHealthUpdate(p_70103_1_); } } @SideOnly(Side.CLIENT) public int getattackTimer() { return this.attackTimer; } protected String getHurtSound() { return "mob.enderdragon.hit"; } protected String getDeathSound() { return "mob.blaze.death"; } protected void func_145780_a(int p_145780_1_, int p_145780_2_, int p_145780_3_, Block p_145780_4_) { this.playSound("step.cloth", 1.0F, 1.0F); } public void setDead() { for (int i = 0; i < 2; ++i) { worldObj.spawnParticle("lava", this.posX, this.posY, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("lava", this.posX, this.posY + 1, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("lava", this.posX, this.posY + 2, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("lava", this.posX, this.posY + 3, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("lava", this.posX, this.posY + 4, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("lava", this.posX, this.posY + 5, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("lava", this.posX, this.posY + 6, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("lava", this.posX + 1, this.posY + 1, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("lava", this.posX + 1, this.posY + 2, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("lava", this.posX + 1, this.posY + 3, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("lava", this.posX + 1, this.posY + 4, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("lava", this.posX + 1, this.posY + 5, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("lava", this.posX + 1, this.posY + 6, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("explode", this.posX, this.posY, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("explode", this.posX, this.posY + 1, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("explode", this.posX, this.posY + 2, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("explode", this.posX, this.posY + 3, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("explode", this.posX, this.posY + 4, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("explode", this.posX, this.posY + 5, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("explode", this.posX, this.posY + 6, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("explode", this.posX + 1, this.posY + 1, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("explode", this.posX + 1, this.posY + 2, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("explode", this.posX + 1, this.posY + 3, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("explode", this.posX + 1, this.posY + 4, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("explode", this.posX + 1, this.posY + 5, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("explode", this.posX + 1, this.posY + 6, this.posZ, 50.0F, 50.0F, 50.0F); worldObj.spawnParticle("hugeexplosion", this.posX, this.posY, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("hugeexplosion", this.posX, this.posY + 1, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("hugeexplosion", this.posX, this.posY + 2, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("hugeexplosion", this.posX, this.posY + 3, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("hugeexplosion", this.posX, this.posY + 4, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("hugeexplosion", this.posX, this.posY + 5, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("hugeexplosion", this.posX, this.posY + 6, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("hugeexplosion", this.posX + 1, this.posY + 1, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("hugeexplosion", this.posX + 1, this.posY + 2, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("hugeexplosion", this.posX + 1, this.posY + 3, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("hugeexplosion", this.posX + 1, this.posY + 4, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("hugeexplosion", this.posX + 1, this.posY + 5, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("hugeexplosion", this.posX + 1, this.posY + 6, this.posZ, 0.0F, 0.0F, 0.0F); } if (!this.worldObj.isRemote) { ZeusExtended zeus = new ZeusExtended(worldObj); zeus.setPosition(this.posX, this.posY, this.posZ); this.worldObj.spawnEntityInWorld(zeus); this.worldObj.playSoundEffect(this.posX, this.posY, this.posZ, "ambient.weather.thunder", 10000.0F, 0.8F + this.rand.nextFloat() * 0.2F); } super.setDead(); } } after I know why it wont even attempt to attack on collide I think I will be able to fix the problem on my own. thanks for the quick response. -
[1.7.10] Mobs AI and Attributes
BoonieQuafter-CrAfTeR replied to American2050's topic in Modder Support
the witdth and the height sets the size of the hit box, a better way to do that might be this.setSize(3F, 8F) -
so im making an attackai for my mob, I want it so switch back and forth from ranged to melee. so I tried adding a distance method, but so far it hasn't gotten me any were accept a crash report. so here is my code, im not asking for anything accept for why it doesn't work. thank you. public void setCombatTask() { { if(this.getAttackTarget().getDistanceSqToEntity(this) > 121.0D){ this.tasks.addTask(4, this.aiArrowAttack); }else{ this.tasks.addTask(4, this.aiAttackOnCollide); } } }
-
[1.7.10] Mobs AI and Attributes
BoonieQuafter-CrAfTeR replied to American2050's topic in Modder Support
ok dude, u need to realize that all this stuff is in vanilla mob classes. so you failed to the upmost extent. you need to enable the ai.. which every other mob class in minecraft has (well pretty much). } public boolean isAIEnabled() { return true; } and for getting ur boss bar?? look at wither or enderdragon code, seriously. vanilla has everything you need. so for the boss bar you have to make an onlivingupdate method, like this.. public void onLivingUpdate() { super.onLivingUpdate(); and to get the boss bar all you need is this line of code, which set the boss bar, believe it or not. BossStatus.setBossStatus(this, true); basically for the future. look at vanilla code first, and look for tutorials. It will help you out ALLOT. -
this is sort of a messy outline I made of what I want to do(you last idea) I tried making a onupdate for distance measurement, like the witch does for whether or not it should through a potion, depending on how far away its attack target is. so this is what I have so far. btw it doesn't work. package com.OlympiansMod.entity; import java.util.Iterator; import java.util.List; import com.OlympiansMod.Item.ModItems; import cpw.mods.fml.relauncher.Side; import cpw.mods.fml.relauncher.SideOnly; import net.minecraft.block.Block; import net.minecraft.entity.Entity; import net.minecraft.entity.EntityLiving; import net.minecraft.entity.EntityLivingBase; import net.minecraft.entity.IRangedAttackMob; import net.minecraft.entity.SharedMonsterAttributes; import net.minecraft.entity.ai.EntityAIArrowAttack; import net.minecraft.entity.ai.EntityAIAttackOnCollide; import net.minecraft.entity.ai.EntityAIHurtByTarget; import net.minecraft.entity.ai.EntityAILookIdle; import net.minecraft.entity.ai.EntityAIMoveTowardsTarget; import net.minecraft.entity.ai.EntityAINearestAttackableTarget; import net.minecraft.entity.ai.EntityAISwimming; import net.minecraft.entity.ai.EntityAIWander; import net.minecraft.entity.ai.EntityAIWatchClosest; import net.minecraft.entity.boss.BossStatus; import net.minecraft.entity.boss.EntityDragon; import net.minecraft.entity.boss.IBossDisplayData; import net.minecraft.entity.monster.EntityGolem; import net.minecraft.entity.monster.EntityMob; import net.minecraft.entity.monster.EntitySlime; import net.minecraft.entity.monster.EntityWitch; import net.minecraft.entity.monster.EntityZombie; import net.minecraft.entity.monster.IMob; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.entity.projectile.EntityPotion; import net.minecraft.init.Blocks; import net.minecraft.init.Items; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.potion.Potion; import net.minecraft.potion.PotionEffect; import net.minecraft.util.DamageSource; import net.minecraft.util.MathHelper; import net.minecraft.world.World; public class Zeus extends EntityGolem implements IBossDisplayData, IRangedAttackMob { private EntityAIArrowAttack aiArrowAttack = new EntityAIArrowAttack(this, 1.0D, 20, 60, 15.0F); private EntityAIAttackOnCollide aiAttackOnCollide = new EntityAIAttackOnCollide(this, EntityPlayer.class, 1.2D, false); private int attackTimer; private int healthupdate; private int attackMelee;; public Zeus(World world) { super(world); { this.getNavigator().setAvoidsWater(true); this.isImmuneToFire = true; this.setHealth(this.getMaxHealth()); this.setSize(1.1F, 2F); this.getNavigator().setAvoidsWater(true); this.tasks.addTask(1, new EntityAISwimming(this)); this.tasks.addTask(2, new EntityAIWander(this, 0.5D)); this.tasks.addTask(4, new EntityAIWatchClosest(this, EntityPlayer.class, 1.0F)); this.tasks.addTask(5, new EntityAIWatchClosest(this, EntityUndead.class, 1.0F)); this.tasks.addTask(6, new EntityAILookIdle(this)); this.tasks.addTask(7, new EntityAIMoveTowardsTarget(this, 0.7D, 100.0F)); this.targetTasks.addTask(1, new EntityAIHurtByTarget(this, true)); this.targetTasks.addTask(2, new EntityAINearestAttackableTarget(this, EntityPlayer.class, 0, true)); this.targetTasks.addTask(3, new EntityAINearestAttackableTarget(this, EntityLiving.class, 0, false, true, IMob.mobSelector)); if (world != null && !world.isRemote) { this.setCombatTask(); } } } public void onLivingUpdate() { super.onLivingUpdate(); BossStatus.setBossStatus(this, true); if (this.getHealth() <= 1000) { --this.healthupdate; } if (this.attackTimer > 0) { --this.attackTimer; } if (this.rand.nextFloat() < 0.25F && this.getAttackTarget() != null && this.getAttackTarget().getDistanceSqToEntity(this) > 121.0D) { --this.attackMelee; } } { } public boolean isAIEnabled() { return true; } @Override protected void entityInit() { super.entityInit(); dataWatcher.addObject(21, (byte) 0); } public void setAggressive(boolean par2) { this.getDataWatcher().updateObject(21, Byte.valueOf((byte) (par2 ? 1 : 0))); } public boolean getAggressive() { return this.getDataWatcher().getWatchableObjectByte(21) == 1; } protected void addRandomArmor() { super.addRandomArmor(); this.setCurrentItemOrArmor(0, new ItemStack(ModItems.MasterBolt)); } protected void applyEntityAttributes() { super.applyEntityAttributes(); this.getEntityAttribute(SharedMonsterAttributes.followRange).setBaseValue(70.0D); this.getEntityAttribute(SharedMonsterAttributes.maxHealth).setBaseValue(5000.0D); this.getEntityAttribute(SharedMonsterAttributes.movementSpeed).setBaseValue(0.7D); } public void setCombatTask() { { this.tasks.addTask(4, this.aiArrowAttack); } { this.attackMelee = 10; this.tasks.addTask(4, this.aiAttackOnCollide); } } @Override public boolean attackEntityAsMob(Entity entity) { this.attackTimer = 10; this.worldObj.setEntityState(this, (byte) 4); return entity.attackEntityFrom(DamageSource.causeMobDamage(this), (float) (7 + this.rand.nextInt(15))); } @Override public void attackEntityWithRangedAttack(EntityLivingBase entity, float par1) { this.attackTimer = 10; this.worldObj.setEntityState(this, (byte) 4); this.worldObj.playSoundEffect(this.posX, this.posY, this.posZ, "portal.portal", 10000.0F, 0.8F + this.rand.nextFloat() * 0.2F); EntityMasterBolt Bolt = new EntityMasterBolt(this.worldObj, this); Bolt.rotationPitch -= -20.0F; double d0 = entity.posX + entity.motionX - this.posX; double d1 = entity.posY + (double) entity.getEyeHeight() - 1.900000023841858D - this.posY; double d2 = entity.posZ + entity.motionZ - this.posZ; float f1 = MathHelper.sqrt_double(d0 * d0 + d2 * d2); Bolt.setThrowableHeading(d0, d1 + (double) (f1 * 0.2F), d2, 0.75F, 8.0F); this.worldObj.spawnEntityInWorld(Bolt); if (this.getHealth() <= 1500) { for (int i = 0; i < 10; i++) { this.worldObj.playSoundEffect(this.posX, this.posY, this.posZ, "portal.portal", 10000.0F, 0.8F + this.rand.nextFloat() * 0.2F); EntityThunderBolt Bolt2 = new EntityThunderBolt( this.worldObj, this); Bolt.rotationPitch -= -20.0F; double d0l = entity.posX + entity.motionX - this.posX + 1; double d1l = entity.posY + (double) entity.getEyeHeight() - 0.900000023841858D - this.posY; double d2l = entity.posZ + entity.motionZ - this.posZ; float f1l = MathHelper.sqrt_double(d0 * d0 + d2 * d2); Bolt2.setThrowableHeading(d0l, d1l + (double) (f1l * 0.2F), d2l, 0.75F, 8.0F); this.worldObj.spawnEntityInWorld(Bolt2); } } } @SideOnly(Side.CLIENT) public void handleHealthUpdate(byte p_70103_1_) { if (p_70103_1_ == 4) { this.attackTimer = 10; } else { super.handleHealthUpdate(p_70103_1_); } } @SideOnly(Side.CLIENT) public int getattackTimer() { return this.attackTimer; } protected String getHurtSound() { return "mob.enderdragon.hit"; } protected String getDeathSound() { return "mob.blaze.death"; } protected void func_145780_a(int p_145780_1_, int p_145780_2_, int p_145780_3_, Block p_145780_4_) { this.playSound("step.cloth", 1.0F, 1.0F); } public void setDead() { for (int i = 0; i < 2; ++i) { worldObj.spawnParticle("lava", this.posX, this.posY, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("lava", this.posX, this.posY + 1, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("lava", this.posX, this.posY + 2, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("lava", this.posX, this.posY + 3, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("lava", this.posX, this.posY + 4, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("lava", this.posX, this.posY + 5, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("lava", this.posX, this.posY + 6, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("lava", this.posX + 1, this.posY + 1, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("lava", this.posX + 1, this.posY + 2, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("lava", this.posX + 1, this.posY + 3, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("lava", this.posX + 1, this.posY + 4, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("lava", this.posX + 1, this.posY + 5, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("lava", this.posX + 1, this.posY + 6, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("explode", this.posX, this.posY, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("explode", this.posX, this.posY + 1, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("explode", this.posX, this.posY + 2, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("explode", this.posX, this.posY + 3, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("explode", this.posX, this.posY + 4, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("explode", this.posX, this.posY + 5, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("explode", this.posX, this.posY + 6, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("explode", this.posX + 1, this.posY + 1, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("explode", this.posX + 1, this.posY + 2, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("explode", this.posX + 1, this.posY + 3, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("explode", this.posX + 1, this.posY + 4, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("explode", this.posX + 1, this.posY + 5, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("explode", this.posX + 1, this.posY + 6, this.posZ, 50.0F, 50.0F, 50.0F); worldObj.spawnParticle("hugeexplosion", this.posX, this.posY, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("hugeexplosion", this.posX, this.posY + 1, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("hugeexplosion", this.posX, this.posY + 2, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("hugeexplosion", this.posX, this.posY + 3, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("hugeexplosion", this.posX, this.posY + 4, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("hugeexplosion", this.posX, this.posY + 5, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("hugeexplosion", this.posX, this.posY + 6, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("hugeexplosion", this.posX + 1, this.posY + 1, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("hugeexplosion", this.posX + 1, this.posY + 2, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("hugeexplosion", this.posX + 1, this.posY + 3, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("hugeexplosion", this.posX + 1, this.posY + 4, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("hugeexplosion", this.posX + 1, this.posY + 5, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("hugeexplosion", this.posX + 1, this.posY + 6, this.posZ, 0.0F, 0.0F, 0.0F); } if (!this.worldObj.isRemote) { ZeusExtended zeus = new ZeusExtended(worldObj); zeus.setPosition(this.posX, this.posY, this.posZ); this.worldObj.spawnEntityInWorld(zeus); this.worldObj.playSoundEffect(this.posX, this.posY, this.posZ, "ambient.weather.thunder", 10000.0F, 0.8F + this.rand.nextFloat() * 0.2F); } super.setDead(); } } also ingame sometimes the entity looks as though its trying to attack.
-
ok so I tried what cool alias said but it still isn't working for me: package com.OlympiansMod.entity; import java.util.Iterator; import java.util.List; import com.OlympiansMod.Item.ModItems; import cpw.mods.fml.relauncher.Side; import cpw.mods.fml.relauncher.SideOnly; import net.minecraft.block.Block; import net.minecraft.entity.Entity; import net.minecraft.entity.EntityLiving; import net.minecraft.entity.EntityLivingBase; import net.minecraft.entity.IRangedAttackMob; import net.minecraft.entity.SharedMonsterAttributes; import net.minecraft.entity.ai.EntityAIArrowAttack; import net.minecraft.entity.ai.EntityAIAttackOnCollide; import net.minecraft.entity.ai.EntityAIHurtByTarget; import net.minecraft.entity.ai.EntityAILookIdle; import net.minecraft.entity.ai.EntityAIMoveTowardsTarget; import net.minecraft.entity.ai.EntityAINearestAttackableTarget; import net.minecraft.entity.ai.EntityAISwimming; import net.minecraft.entity.ai.EntityAIWander; import net.minecraft.entity.ai.EntityAIWatchClosest; import net.minecraft.entity.boss.BossStatus; import net.minecraft.entity.boss.EntityDragon; import net.minecraft.entity.boss.IBossDisplayData; import net.minecraft.entity.monster.EntityGolem; import net.minecraft.entity.monster.EntityMob; import net.minecraft.entity.monster.EntitySlime; import net.minecraft.entity.monster.EntityWitch; import net.minecraft.entity.monster.EntityZombie; import net.minecraft.entity.monster.IMob; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.entity.projectile.EntityPotion; import net.minecraft.init.Blocks; import net.minecraft.init.Items; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.potion.Potion; import net.minecraft.potion.PotionEffect; import net.minecraft.util.DamageSource; import net.minecraft.util.MathHelper; import net.minecraft.world.World; public class Zeus extends EntityGolem implements IBossDisplayData, IRangedAttackMob { private EntityAIArrowAttack aiArrowAttack = new EntityAIArrowAttack(this, 1.0D, 20, 60, 15.0F); private EntityAIAttackOnCollide aiAttackOnCollide = new EntityAIAttackOnCollide(this, EntityPlayer.class, 1.2D, true); private int attackTimer; private int healthupdate; private static final String __OBFID = "CL_00001697"; public Zeus(World world) { super(world); { this.getNavigator().setAvoidsWater(true); this.isImmuneToFire = true; this.setHealth(this.getMaxHealth()); this.setSize(1.1F, 2F); this.getNavigator().setAvoidsWater(true); this.tasks.addTask(1, new EntityAISwimming(this)); this.tasks.addTask(2, new EntityAIWander(this, 0.5D)); this.tasks.addTask(4, new EntityAIWatchClosest(this, EntityPlayer.class, 1.0F)); this.tasks.addTask(5, new EntityAIWatchClosest(this, EntityUndead.class, 1.0F)); this.tasks.addTask(6, new EntityAILookIdle(this)); this.tasks.addTask(7, new EntityAIMoveTowardsTarget(this, 0.7D, 100.0F)); this.targetTasks.addTask(1, new EntityAIHurtByTarget(this, true)); this.targetTasks.addTask(2, new EntityAINearestAttackableTarget(this, EntityPlayer.class, 0, true)); this.targetTasks.addTask(3, new EntityAINearestAttackableTarget(this, EntityLiving.class, 0, false, true, IMob.mobSelector)); if (world != null && !world.isRemote) { this.setCombatTask(); } } } public void onLivingUpdate() { super.onLivingUpdate(); BossStatus.setBossStatus(this, true); if (this.getHealth() <= 1000) { --this.healthupdate; } if (this.attackTimer > 0) { --this.attackTimer; } } { } public boolean isAIEnabled() { return true; } @Override protected void entityInit() { super.entityInit(); dataWatcher.addObject(21, (byte) 0); } public void setAggressive(boolean par2) { this.getDataWatcher().updateObject(21, Byte.valueOf((byte) (par2 ? 1 : 0))); } public boolean getAggressive() { return this.getDataWatcher().getWatchableObjectByte(21) == 1; } protected void addRandomArmor() { super.addRandomArmor(); this.setCurrentItemOrArmor(0, new ItemStack(ModItems.MasterBolt)); } protected void applyEntityAttributes() { super.applyEntityAttributes(); this.getEntityAttribute(SharedMonsterAttributes.followRange).setBaseValue(70.0D); this.getEntityAttribute(SharedMonsterAttributes.maxHealth).setBaseValue(5000.0D); this.getEntityAttribute(SharedMonsterAttributes.movementSpeed).setBaseValue(0.7D); } public void setCombatTask() { { this.tasks.addTask(4, this.aiArrowAttack); } { this.tasks.addTask(4, this.aiAttackOnCollide); } } @Override public void attackEntityWithRangedAttack(EntityLivingBase entity, float par1) { this.attackTimer = 10; this.worldObj.setEntityState(this, (byte) 4); this.worldObj.playSoundEffect(this.posX, this.posY, this.posZ, "portal.portal", 10000.0F, 0.8F + this.rand.nextFloat() * 0.2F); EntityMasterBolt Bolt = new EntityMasterBolt(this.worldObj, this); Bolt.rotationPitch -= -20.0F; double d0 = entity.posX + entity.motionX - this.posX; double d1 = entity.posY + (double) entity.getEyeHeight() - 1.900000023841858D - this.posY; double d2 = entity.posZ + entity.motionZ - this.posZ; float f1 = MathHelper.sqrt_double(d0 * d0 + d2 * d2); Bolt.setThrowableHeading(d0, d1 + (double) (f1 * 0.2F), d2, 0.75F, 8.0F); this.worldObj.spawnEntityInWorld(Bolt); if (this.getHealth() <= 1500) { for (int i = 0; i < 10; i++) { this.worldObj.playSoundEffect(this.posX, this.posY, this.posZ, "portal.portal", 10000.0F, 0.8F + this.rand.nextFloat() * 0.2F); EntityThunderBolt Bolt2 = new EntityThunderBolt( this.worldObj, this); Bolt.rotationPitch -= -20.0F; double d0l = entity.posX + entity.motionX - this.posX + 1; double d1l = entity.posY + (double) entity.getEyeHeight() - 0.900000023841858D - this.posY; double d2l = entity.posZ + entity.motionZ - this.posZ; float f1l = MathHelper.sqrt_double(d0 * d0 + d2 * d2); Bolt2.setThrowableHeading(d0l, d1l + (double) (f1l * 0.2F), d2l, 0.75F, 8.0F); this.worldObj.spawnEntityInWorld(Bolt2); } } } @Override public boolean attackEntityAsMob(Entity entity) { this.attackTimer = 10; this.worldObj.setEntityState(this, (byte) 4); return entity.attackEntityFrom(DamageSource.causeMobDamage(this), (float) (7 + this.rand.nextInt(15))); } @SideOnly(Side.CLIENT) public void handleHealthUpdate(byte p_70103_1_) { if (p_70103_1_ == 4) { this.attackTimer = 10; } else { super.handleHealthUpdate(p_70103_1_); } } @SideOnly(Side.CLIENT) public int getattackTimer() { return this.attackTimer; } protected String getHurtSound() { return "mob.enderdragon.hit"; } protected String getDeathSound() { return "mob.blaze.death"; } protected void func_145780_a(int p_145780_1_, int p_145780_2_, int p_145780_3_, Block p_145780_4_) { this.playSound("step.cloth", 1.0F, 1.0F); } public void setDead() { for (int i = 0; i < 2; ++i) { worldObj.spawnParticle("lava", this.posX, this.posY, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("lava", this.posX, this.posY + 1, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("lava", this.posX, this.posY + 2, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("lava", this.posX, this.posY + 3, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("lava", this.posX, this.posY + 4, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("lava", this.posX, this.posY + 5, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("lava", this.posX, this.posY + 6, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("lava", this.posX + 1, this.posY + 1, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("lava", this.posX + 1, this.posY + 2, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("lava", this.posX + 1, this.posY + 3, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("lava", this.posX + 1, this.posY + 4, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("lava", this.posX + 1, this.posY + 5, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("lava", this.posX + 1, this.posY + 6, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("explode", this.posX, this.posY, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("explode", this.posX, this.posY + 1, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("explode", this.posX, this.posY + 2, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("explode", this.posX, this.posY + 3, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("explode", this.posX, this.posY + 4, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("explode", this.posX, this.posY + 5, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("explode", this.posX, this.posY + 6, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("explode", this.posX + 1, this.posY + 1, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("explode", this.posX + 1, this.posY + 2, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("explode", this.posX + 1, this.posY + 3, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("explode", this.posX + 1, this.posY + 4, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("explode", this.posX + 1, this.posY + 5, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("explode", this.posX + 1, this.posY + 6, this.posZ, 50.0F, 50.0F, 50.0F); worldObj.spawnParticle("hugeexplosion", this.posX, this.posY, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("hugeexplosion", this.posX, this.posY + 1, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("hugeexplosion", this.posX, this.posY + 2, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("hugeexplosion", this.posX, this.posY + 3, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("hugeexplosion", this.posX, this.posY + 4, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("hugeexplosion", this.posX, this.posY + 5, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("hugeexplosion", this.posX, this.posY + 6, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("hugeexplosion", this.posX + 1, this.posY + 1, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("hugeexplosion", this.posX + 1, this.posY + 2, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("hugeexplosion", this.posX + 1, this.posY + 3, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("hugeexplosion", this.posX + 1, this.posY + 4, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("hugeexplosion", this.posX + 1, this.posY + 5, this.posZ, 0.0F, 0.0F, 0.0F); worldObj.spawnParticle("hugeexplosion", this.posX + 1, this.posY + 6, this.posZ, 0.0F, 0.0F, 0.0F); } if (!this.worldObj.isRemote) { ZeusExtended zeus = new ZeusExtended(worldObj); zeus.setPosition(this.posX, this.posY, this.posZ); this.worldObj.spawnEntityInWorld(zeus); this.worldObj.playSoundEffect(this.posX, this.posY, this.posZ, "ambient.weather.thunder", 10000.0F, 0.8F + this.rand.nextFloat() * 0.2F); } super.setDead(); } } im probably missing something big
-
[SOLVED]ItemStack consumption problems..
BoonieQuafter-CrAfTeR replied to BoonieQuafter-CrAfTeR's topic in Modder Support
thanks, that actually helped quite allot! -
this doesn't work.. protected void collideWithEntity(Entity entity) { if (!this.getAggressive()) { if (this.getHealth() <= 1000) { this.attackTimer = 10; this.worldObj.setEntityState(this, (byte) 4); entity.attackEntityFrom(DamageSource.causeMobDamage(this), (float) (20 + this.rand.nextInt(15))); } } super.collideWithEntity(entity); } btw I formatted it
-
I actually came up with a different method, but it has a few bugs. A: it doesn't attack when its aggressive, (any mob that touches it takes damage.) B: it doesn't work with the entity player. and I can add EntityPlayer into the method. Here is the code protected void collideWithEntity(Entity entity) { if(this.getHealth()<=3300){ if(!this.getAggressive()){ this.attackTimer = 10; this.worldObj.setEntityState(this, (byte)4); entity.attackEntityFrom(DamageSource.causeMobDamage(this), (float)(20 + this.rand.nextInt(15))); } } super.collideWithEntity(entity); } What should I do
-
[1.7.10] Item Icons are not working?
BoonieQuafter-CrAfTeR replied to BoonieQuafter-CrAfTeR's topic in Modder Support
kk -
[SOLVED]ItemStack consumption problems..
BoonieQuafter-CrAfTeR replied to BoonieQuafter-CrAfTeR's topic in Modder Support
I FIXED IT, it took ur advice cool alias, I kept trying yay! -
[SOLVED]ItemStack consumption problems..
BoonieQuafter-CrAfTeR replied to BoonieQuafter-CrAfTeR's topic in Modder Support
actually this is unrelated but my player.isSneaking is ineffective, any ideas? Edit: Ive tried doing this: public ItemStack onItemRightClick(ItemStack stack, World world, EntityPlayer player){ if(player.inventory.hasItem(ModItems.Riptide)){ player.inventory.consumeInventoryItem(ModItems.Riptide); }else{ player.isSneaking(); } } return new ItemStack(ModItems.anklamolses); } } and this if(player.isSneaking()||player.inventory.consumeInventoryItem(ModItems.anklamolses)){ but it doesn't work, im sure im missing something big im just not sure what it is -
[SOLVED]ItemStack consumption problems..
BoonieQuafter-CrAfTeR replied to BoonieQuafter-CrAfTeR's topic in Modder Support
Thank you, It worked -
so im making an item that consumes itself, but adds another item to the inventory. and vise versa for the added item. Problem is, if the Item is in the first hotbar slot it doesn't add the item to anywhere in the inventory. Here is my method. public ItemStack onItemRightClick(ItemStack stack, World world, EntityPlayer player){ if(player.isSneaking()){ if (player.inventory.hasItem(ModItems.Riptide)){ player.inventory.consumeInventoryItem(ModItems.Riptide); player.inventory.addItemStackToInventory(new ItemStack(ModItems.anklamolses)); } } return stack; } } Thank you all for your time.
-
[1.7.10] Item Icons are not working?
BoonieQuafter-CrAfTeR replied to BoonieQuafter-CrAfTeR's topic in Modder Support
that wasn't a bump I was trying an emoji -
[1.7.10] Item Icons are not working?
BoonieQuafter-CrAfTeR replied to BoonieQuafter-CrAfTeR's topic in Modder Support
sup