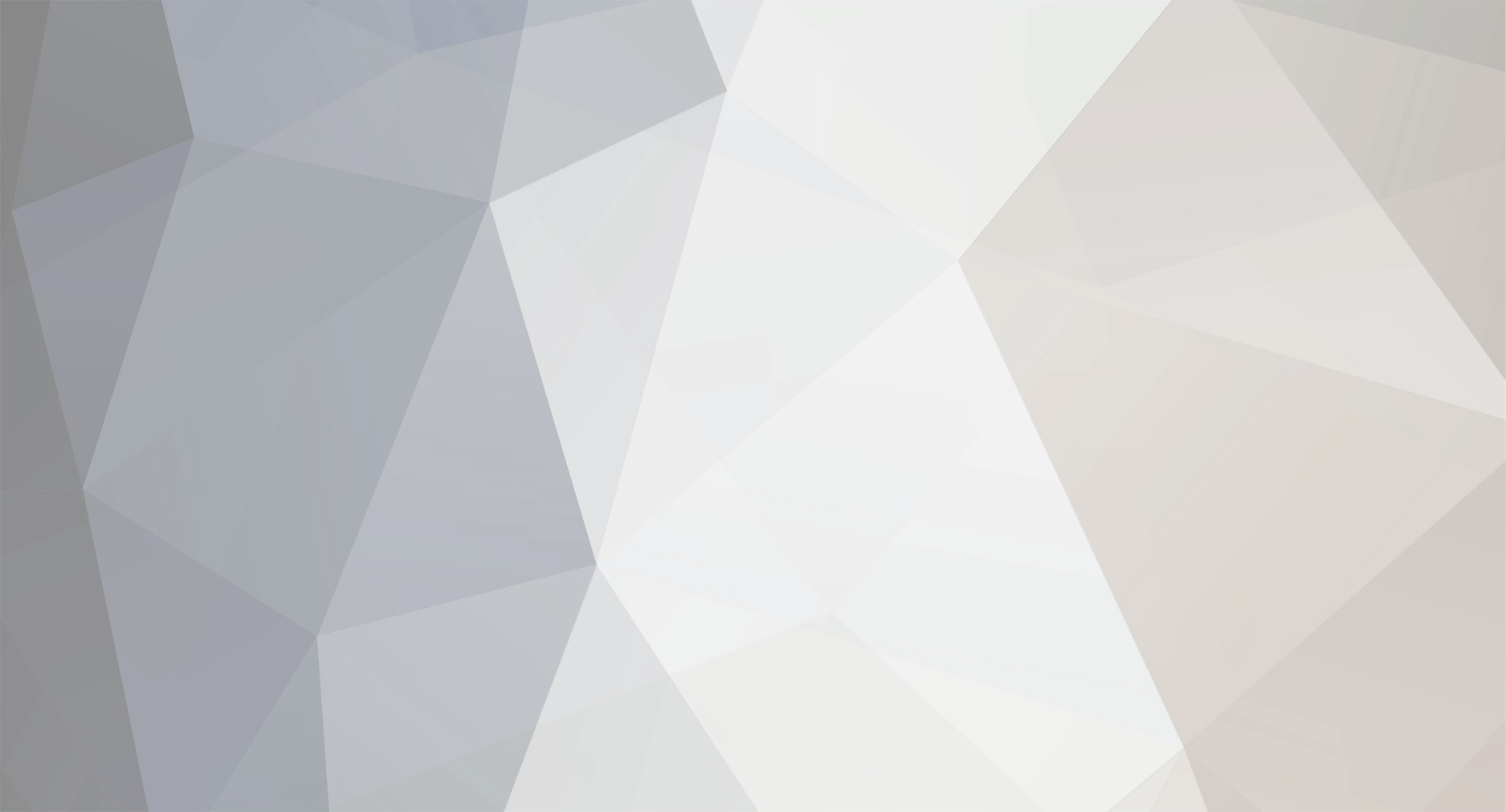
deerangle
Members-
Posts
259 -
Joined
-
Last visited
-
Days Won
1
Everything posted by deerangle
-
I'm making a Block that can store items. If the player right-clicks, he stores one item. When he shift-right-clicks, he stores the entire stack. But while sneaking, onBlockActivated is only triggered if the player's hand is empty. So which method/event should i use instead?
-
1.7.10 is actually no longer supported on this forum, but whatever... The setStepSound method sets the sound that the block makes when you walk on it. Like when you walk on sand, it makes a different sound than if you were to walk on grass
-
I was missing getMetadata..... ? public class ItemBlockStorage extends ItemBlock { public ItemBlockStorage(Block block) { super(block); this.setHasSubtypes(true); } @Override public int getMetadata(ItemStack stack) { return this.getMetadata(stack.getItemDamage()); } @Override public int getMetadata(int damage) { return damage; } @Override public String getUnlocalizedName(ItemStack stack) { return super.getUnlocalizedName(stack) + "_" + BlockStorage.EnumStorageType.byMeta(stack.getMetadata()).getName(); } }
-
I created a Block that has Blockstates. When I use the Item to place it though, it get's placed with the default state and not the state according to the metadata of the damage value of the item. Setblock delivers me the correct blockstate though. I know it must be something really trivial that i missed, but I can't seem to find anything... Here are my ItemBlock and Block classes:
-
It was so simple... I had to remove the ".json" from the resourcelocation. Solved!
-
You are getting this: java.lang.RuntimeException: One of more entry values did not copy to the correct id. Check log for details! In the github issue, moosegold mentions this error:
-
Have a look at this issue: https://github.com/MinecraftForge/MinecraftForge/issues/4079
-
I made a mob and want to use a LootTable for the loot. I added the LootTable json file and registered it in preinit (before entity initialization). The content is the exact same as the cow loot table. I overwrote EntityAnimal#getLootTable to return the exact same loot table resource loc as the one i registered in preinit. I put breakpoints in the code, and found that the loottable is empty after being loaded here LootTable loottable = this.world.getLootTableManager().getLootTableFromLocation(resourcelocation); The resourcelocation though, is correct in EntityLiving#dropLoot. I checked to make sure that my init code is called too: LootTableList.register(new ResourceLocation(MODID, "entities/hedgehog.json")); Why is my hedgehog LootTable not working? Any ideas?
-
Yeah right. I don't want that. Then i'll make it without auto-download.
-
Thank you!
-
I am currently trying to make a ModCreator. A simple GUI interface, that beginners or people who cannot code can create simple mods. Now I need to download the MDK from the forge-website, but it's currently hard-coded into the code and the user cant select the forge version. I saw that there is a version_manifest.json for minecraft itself. Is there something similar for forge?
-
I Solved my issue by setting "fboEnable" from the GameSettings to false. I assume that makes it possibile for me to use FBOs.
-
I did now. Sorry
-
I know that It has to do with the FBO. when I remove the BindFBO stuff, it doesn't freeze. FBO code: package com.deerangle.camera; import java.nio.ByteBuffer; import org.lwjgl.opengl.Display; import org.lwjgl.opengl.GL11; import org.lwjgl.opengl.GL12; import org.lwjgl.opengl.GL13; import org.lwjgl.opengl.GL14; import org.lwjgl.opengl.GL15; import org.lwjgl.opengl.GL20; import org.lwjgl.opengl.GL21; import org.lwjgl.opengl.GL30; import org.lwjgl.opengl.GL31; import org.lwjgl.opengl.GL32; import org.lwjgl.opengl.GL33; import org.lwjgl.opengl.GL40; import org.lwjgl.opengl.GL42; public class CameraFBO { public static final int NONE = 0; public static final int DEPTH_TEXTURE = 1; public static final int DEPTH_RENDER_BUFFER = 2; private final int width; private final int height; private int frameBuffer; private int colourTexture; private int depthTexture; private int depthBuffer; private int colourBuffer; /** * Creates an FBO of a specified width and height, with the desired type of * depth buffer attachment. * * @param width * - the width of the FBO. * @param height * - the height of the FBO. * @param depthBufferType * - an int indicating the type of depth buffer attachment that * this FBO should use. */ public CameraFBO(int width, int height, int depthBufferType) { this.width = width; this.height = height; initialiseFrameBuffer(depthBufferType); } /** * Deletes the frame buffer and its attachments when the game closes. */ public void cleanUp() { GL30.glDeleteFramebuffers(frameBuffer); GL11.glDeleteTextures(colourTexture); GL11.glDeleteTextures(depthTexture); GL30.glDeleteRenderbuffers(depthBuffer); GL30.glDeleteRenderbuffers(colourBuffer); } /** * Binds the frame buffer, setting it as the current render target. Anything * rendered after this will be rendered to this FBO, and not to the screen. */ public void bindFrameBuffer() { GL30.glBindFramebuffer(GL30.GL_DRAW_FRAMEBUFFER, frameBuffer); GL11.glViewport(0, 0, width, height); } /** * Unbinds the frame buffer, setting the default frame buffer as the current * render target. Anything rendered after this will be rendered to the screen, * and not this FBO. */ public void unbindFrameBuffer() { GL30.glBindFramebuffer(GL30.GL_FRAMEBUFFER, 0); GL11.glViewport(0, 0, Display.getWidth(), Display.getHeight()); } /** * Binds the current FBO to be read from (not used in tutorial 43). */ public void bindToRead() { GL11.glBindTexture(GL11.GL_TEXTURE_2D, 0); GL30.glBindFramebuffer(GL30.GL_READ_FRAMEBUFFER, frameBuffer); GL11.glReadBuffer(GL30.GL_COLOR_ATTACHMENT0); } /** * @return The ID of the texture containing the colour buffer of the FBO. */ public int getColourTexture() { return colourTexture; } /** * @return The texture containing the FBOs depth buffer. */ public int getDepthTexture() { return depthTexture; } /** * Creates the FBO along with a colour buffer texture attachment, and possibly a * depth buffer. * * @param type * - the type of depth buffer attachment to be attached to the FBO. */ private void initialiseFrameBuffer(int type) { createFrameBuffer(); createTextureAttachment(); if (type == DEPTH_RENDER_BUFFER) { createDepthBufferAttachment(); } else if (type == DEPTH_TEXTURE) { createDepthTextureAttachment(); } unbindFrameBuffer(); } /** * Creates a new frame buffer object and sets the buffer to which drawing will * occur - colour attachment 0. This is the attachment where the colour buffer * texture is. * */ private void createFrameBuffer() { frameBuffer = GL30.glGenFramebuffers(); GL30.glBindFramebuffer(GL30.GL_FRAMEBUFFER, frameBuffer); GL11.glDrawBuffer(GL30.GL_COLOR_ATTACHMENT0); } /** * Creates a texture and sets it as the colour buffer attachment for this FBO. */ private void createTextureAttachment() { colourTexture = GL11.glGenTextures(); GL11.glBindTexture(GL11.GL_TEXTURE_2D, colourTexture); GL11.glTexImage2D(GL11.GL_TEXTURE_2D, 0, GL11.GL_RGB8, width, height, 0, GL11.GL_RGB, GL11.GL_UNSIGNED_BYTE, (ByteBuffer) null); GL11.glTexParameteri(GL11.GL_TEXTURE_2D, GL11.GL_TEXTURE_MAG_FILTER, GL11.GL_LINEAR); GL11.glTexParameteri(GL11.GL_TEXTURE_2D, GL11.GL_TEXTURE_MIN_FILTER, GL11.GL_LINEAR); GL11.glTexParameteri(GL11.GL_TEXTURE_2D, GL11.GL_TEXTURE_WRAP_S, GL12.GL_CLAMP_TO_EDGE); GL11.glTexParameteri(GL11.GL_TEXTURE_2D, GL11.GL_TEXTURE_WRAP_T, GL12.GL_CLAMP_TO_EDGE); GL30.glFramebufferTexture2D(GL30.GL_FRAMEBUFFER, GL30.GL_COLOR_ATTACHMENT0, GL11.GL_TEXTURE_2D, colourTexture, 0); } /** * Adds a depth buffer to the FBO in the form of a texture, which can later be * sampled. */ private void createDepthTextureAttachment() { depthTexture = GL11.glGenTextures(); GL11.glBindTexture(GL11.GL_TEXTURE_2D, depthTexture); GL11.glTexImage2D(GL11.GL_TEXTURE_2D, 0, GL14.GL_DEPTH_COMPONENT24, width, height, 0, GL11.GL_DEPTH_COMPONENT, GL11.GL_FLOAT, (ByteBuffer) null); GL11.glTexParameteri(GL11.GL_TEXTURE_2D, GL11.GL_TEXTURE_MAG_FILTER, GL11.GL_LINEAR); GL11.glTexParameteri(GL11.GL_TEXTURE_2D, GL11.GL_TEXTURE_MIN_FILTER, GL11.GL_LINEAR); GL30.glFramebufferTexture2D(GL30.GL_FRAMEBUFFER, GL30.GL_DEPTH_ATTACHMENT, GL11.GL_TEXTURE_2D, depthTexture, 0); } /** * Adds a depth buffer to the FBO in the form of a render buffer. This can't be * used for sampling in the shaders. */ private void createDepthBufferAttachment() { depthBuffer = GL30.glGenRenderbuffers(); GL30.glBindRenderbuffer(GL30.GL_RENDERBUFFER, depthBuffer); GL30.glRenderbufferStorage(GL30.GL_RENDERBUFFER, GL14.GL_DEPTH_COMPONENT24, width, height); GL30.glFramebufferRenderbuffer(GL30.GL_FRAMEBUFFER, GL30.GL_DEPTH_ATTACHMENT, GL30.GL_RENDERBUFFER, depthBuffer); } }
-
I'm rendering minecraft to an FBO, from the perspective of a camera block. So far, almost everything works, except for a weird rendering bug. While the camera is rendering to the FBO, my menu does not disappear and the vignette seems to stack until it's a black circle. See vignette_mc.jpg Code:
-
I just tested with another Block and found that it was the Cauldrons's fault. And thanks to your tipp, I solved this issue! Thanks!
-
I just realized that I was using the wrong item. Now i am using ModItems.SCHOKO_INGOT. Still not working. Buti had the same thought too. But how can it be, that in the same function, ModCrafting#preInit(), i have this line, and it works: GameRegistry.addShapedRecipe(new ItemStack(ModBlocks.SCHOKO_BLOCK, 1, 2), "AAA", "AAA", "AAA", 'A', new ItemStack(ModItems.SCHOKO_INGOT, 1, 2)); (I will update the code at the top to how it is supposed to be. Still not working though)
-
My ShapedOreRecipe isn't working properly. I registered it with several other recipes. the only one that doesnt work is this one: new ShapedOreRecipe(new ItemStack(ModBlocks.PACKER), "I I", "WSW", "WCW", 'I', "ingotIron", 'S', new ItemStack(ModItems.SCHOKO_INGOT, 1, 0), 'C', Blocks.CAULDRON, 'W', "plankWood") to debug, I printed the recipes contents: ShapedOreRecipe sor = new ShapedOreRecipe(new ItemStack(ModBlocks.PACKER), "I I", "WSW", "WCW", 'I', "ingotIron", 'S', new ItemStack(ModItems.SCHOKO_INGOT, 1, 0), 'C', Blocks.CAULDRON, 'W', "plankWood"); for(Object o : sor.getInput()) { System.out.println(o); } System.out.println(sor.getRecipeOutput()); GameRegistry.addRecipe(sor); The output was following: [16:38:34] [Client thread/INFO] [STDOUT]: [com.deerangle.main.ModCrafting:preInit:43]: [1xitem.ingotIron@0] [16:38:34] [Client thread/INFO] [STDOUT]: [com.deerangle.main.ModCrafting:preInit:43]: null [16:38:34] [Client thread/INFO] [STDOUT]: [com.deerangle.main.ModCrafting:preInit:43]: [1xitem.ingotIron@0] [16:38:34] [Client thread/INFO] [STDOUT]: [com.deerangle.main.ModCrafting:preInit:43]: [1xtile.wood@32767] [16:38:34] [Client thread/INFO] [STDOUT]: [com.deerangle.main.ModCrafting:preInit:43]: 1xitem.null@0 [16:38:34] [Client thread/INFO] [STDOUT]: [com.deerangle.main.ModCrafting:preInit:43]: [1xtile.wood@32767] [16:38:34] [Client thread/INFO] [STDOUT]: [com.deerangle.main.ModCrafting:preInit:43]: [1xtile.wood@32767] [16:38:34] [Thread-6/INFO] [FML]: Using sync timing. 200 frames of Display.update took 125382604 nanos [16:38:34] [Client thread/INFO] [FML]: Applying holder lookups [16:38:34] [Client thread/INFO] [FML]: Holder lookups applied [16:38:34] [Client thread/INFO] [FML]: Injecting itemstacks [16:38:34] [Client thread/INFO] [FML]: Itemstack injection complete [16:38:34] [Client thread/ERROR] [FML]: Fatal errors were detected during the transition from PREINITIALIZATION to INITIALIZATION. Loading cannot continue [16:38:34] [Client thread/ERROR] [FML]: States: 'U' = Unloaded 'L' = Loaded 'C' = Constructed 'H' = Pre-initialized 'I' = Initialized 'J' = Post-initialized 'A' = Available 'D' = Disabled 'E' = Errored UCH mcp{9.19} [Minecraft Coder Pack] (minecraft.jar) UCH FML{8.0.99.99} [Forge Mod Loader] (forgeSrc-1.10.2-12.18.3.2511.jar) UCH Forge{12.18.3.2511} [Minecraft Forge] (forgeSrc-1.10.2-12.18.3.2511.jar) UCE noahschocolate{1.1.155} [Noah's Chocolate!] (bin) UCH JEI{3.9.7.260} [Just Enough Items] (jei_1.10.2-3.9.7.260.jar) [16:38:34] [Client thread/ERROR] [FML]: The following problems were captured during this phase [16:38:34] [Client thread/ERROR] [FML]: Caught exception from Noah's Chocolate! (noahschocolate) java.lang.NullPointerException at net.minecraft.item.ItemStack.toString(ItemStack.java:509) ~[forgeSrc-1.10.2-12.18.3.2511.jar:?] at java.lang.String.valueOf(String.java:2994) ~[?:1.8.0_171] at java.lang.StringBuilder.append(StringBuilder.java:131) ~[?:1.8.0_171] at net.minecraftforge.fml.common.TracingPrintStream.println(TracingPrintStream.java:43) ~[forgeSrc-1.10.2-12.18.3.2511.jar:?] at com.deerangle.main.ModCrafting.preInit(ModCrafting.java:43) ~[bin/:?] at com.deerangle.main.NoahsChocolate.preinit(NoahsChocolate.java:61) ~[bin/:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_171] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_171] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_171] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_171] at net.minecraftforge.fml.common.FMLModContainer.handleModStateEvent(FMLModContainer.java:618) ~[forgeSrc-1.10.2-12.18.3.2511.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_171] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_171] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_171] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_171] at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) ~[guava-17.0.jar:?] at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) ~[guava-17.0.jar:?] at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) ~[guava-17.0.jar:?] at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) ~[guava-17.0.jar:?] at com.google.common.eventbus.EventBus.post(EventBus.java:275) ~[guava-17.0.jar:?] at net.minecraftforge.fml.common.LoadController.sendEventToModContainer(LoadController.java:243) ~[forgeSrc-1.10.2-12.18.3.2511.jar:?] at net.minecraftforge.fml.common.LoadController.propogateStateMessage(LoadController.java:221) ~[forgeSrc-1.10.2-12.18.3.2511.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_171] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_171] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_171] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_171] at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) ~[guava-17.0.jar:?] at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) ~[guava-17.0.jar:?] at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) ~[guava-17.0.jar:?] at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) ~[guava-17.0.jar:?] at com.google.common.eventbus.EventBus.post(EventBus.java:275) ~[guava-17.0.jar:?] at net.minecraftforge.fml.common.LoadController.distributeStateMessage(LoadController.java:145) [LoadController.class:?] at net.minecraftforge.fml.common.Loader.preinitializeMods(Loader.java:624) [Loader.class:?] at net.minecraftforge.fml.client.FMLClientHandler.beginMinecraftLoading(FMLClientHandler.java:259) [FMLClientHandler.class:?] at net.minecraft.client.Minecraft.startGame(Minecraft.java:477) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:386) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_171] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_171] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_171] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_171] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_171] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_171] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_171] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_171] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [start/:?] at GradleStart.main(GradleStart.java:26) [start/:?] The error says, that there is a nullpointer in ItemStack#toString().
-
I was using classes which were only in the Mod but not in the API. Fixed it!
-
When I Build my Mod using gradlew build it crashes at :compileJava and says, that some packages dont exist. build.gradle Terminal output
-
I solved it with a simple workaround that requires me to manually configure it for every tileentity. Thanks anyways. If anyone has an answer to the actual question, feel free to answer!
-
I know, but how can i even test if it would be valid? I dont have access to the slots themselves from my custom ItemStackHandler.
-
Can I make the ItemStackHandler only insert items into a slot if they are valid for the slot?