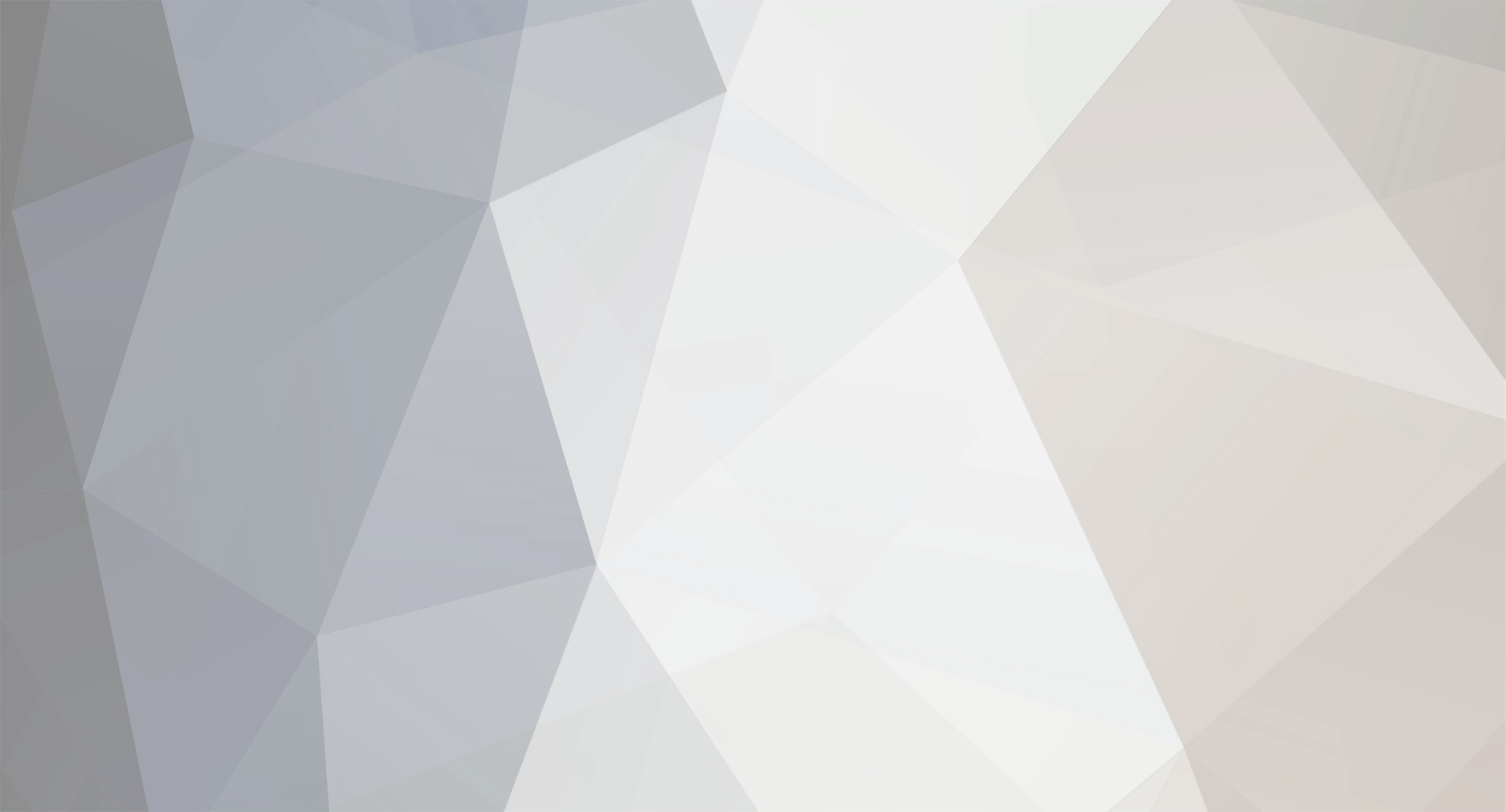
deerangle
Members-
Posts
259 -
Joined
-
Last visited
-
Days Won
1
Everything posted by deerangle
-
Why don't you measure the time it took to complete 20 ticks, then divide 1000ms by the measured time, and multiply that number with the 20 ticks you counted. long measuredTime = 2000L; //this means it took 2000ms for 20 ticks to complete float tps = 20 * (1000f / measuredTime); // 1000/2000 = 0.5; 0.5 * 20 = 10 tps To count the time 20 ticks take, create a field in your eventhandler that counts up every server tick, and when it reaches 20, you calculate the time difference between the last time it reached 20, and then reset the counter. You can effectively vary the 20 ticks and use whatever value you want. The higher the number, the slower the tps updates.
-
[SOLVED][1.13.2] Blockstate submodels not appearing.
deerangle replied to deerangle's topic in Modder Support
I decided to use a different way to solve my problem, since I found that using blockstates wouldn't be beneficial in this situation. I will mark this as solved. -
You should put it in the pre-init event (FMLCommonSetupEvent). http://jabelarminecraft.blogspot.com/p/minecraft-modding-custom-dimension.html
-
I have created a block which has a facing property and a boolean property. Both properties work, and the state change gets applied to the model, but only when using rotation. The facing-property changes the y-rotation, as it should. To debug, I made the boolean-property change the x-rotation, which also worked. But Actually the boolean property should add a submodel to the model, but that doesn't work. The blockstate gets loaded correctly (no purple-black checkerboards), but when I set a block with the boolean property enabled, I don't see any submodel being added. Here is my blockstate json: { "forge_marker": 1, "defaults": { "model": "sortingstorage:storagecell" }, "variants": { "facing": { "north": {}, "south": { "y": 180 }, "west": { "y": 270 }, "east": { "y": 90 } }, "drive_top": { "true": { "submodel": { "top_drive": { "model": "sortingstorage:storagecell_drive_top" } } }, "false": {} } } }
-
So I came up with following Idea: WorldServer world = (WorldServer) event.getWorld(); Field pcm = WorldServer.class.getDeclaredField("playerChunkMap"); pcm.setAccessible(true); PlayerChunkMap map = (PlayerChunkMap) pcm.get(world); List<EntityPlayerMP> players = world.getPlayers(EntityPlayerMP.class, (p) -> true); ImmutableSetMultimap<ChunkPos, ForgeChunkManager.Ticket> chunks = ForgeChunkManager.getPersistentChunksFor(world); for(ChunkPos pos : chunks.keySet()) { for(EntityPlayerMP player : players) { map.getEntry(pos.x, pos.z).addPlayer(player); } } Basically I would add the player to every entry corresponding to the persistent chunks in the ForgeChunkManager. I'm not quite sure about where exactly it would make most sense to put this code.
-
I am trying to access my TileEntity from another place (I know the coordinates of the TileEntity which I want to access). When I call World.getTileEntity, it returns null. I know that the TileEntity is loaded because I'm printing it's coordinates in the update function. I'm getting the TileEntity on client side using World.getTileEntity but the update function is printing in the server thread only. I suspect that the TileEntity is loaded server-side only, and not on client side. I am using ForgeChunkManager to keep the chunk loaded. How can I keep the chunk loaded on client-side aswell?
-
[SOLVED] How can I render a TESR without lighting?
deerangle replied to deerangle's topic in Modder Support
I didn't know about the order, but that fixed it. Thank you for your help! -
[SOLVED] How can I render a TESR without lighting?
deerangle replied to deerangle's topic in Modder Support
Would a DynamicTexture really work? I'm rendering the color buffer attachment of a framebuffer, which is a GL texture... Also, I need to access values from my TileEntity when rendering, hence I'm using a TESR. Could you please elaborate on how one would achieve this?: I have tried it with this, but now the quad is appearing completely black: Tessellator tessellator = Tessellator.getInstance(); BufferBuilder bufferbuilder = tessellator.getBuffer(); bufferbuilder.begin(7, DefaultVertexFormats.POSITION_TEX_LMAP_COLOR); bufferbuilder.pos(15 * px - 0.001, 1 - px + 0.01, px - 0.01).tex(0.0D, 1.0D).color(1, 1, 1, 1f).lightmap(255, 255).endVertex(); bufferbuilder.pos(15 * px - 0.001, px - 0.01, px - 0.01).tex(0.0D, 0.0D).color(1, 1, 1, 1f).lightmap(255, 255).endVertex(); bufferbuilder.pos(15 * px - 0.001, px - 0.01, 1 - px + 0.01).tex(1.0D, 0.0D).color(1, 1, 1, 1f).lightmap(255, 255).endVertex(); bufferbuilder.pos(15 * px - 0.001, 1 - px + 0.01, 1 - px + 0.01).tex(1.0D, 1.0D).color(1, 1, 1, 1f).lightmap(255, 255).endVertex(); tessellator.draw(); -
I need to render a quad in my TESR without lighting. It should always have full brightness (because it is a screen which "emits light"). How could that be achieved? I've tried using GlStateManager.disableLighting() but that didn't work. I also tried GL11.glDisable(GL11.GL_LIGHTING) without success. My current rendering code: GlStateManager.pushMatrix(); GlStateManager.disableLighting(); GlStateManager.enableRescaleNormal(); RenderHelper.disableStandardItemLighting(); GL11.glTranslated(x, y, z); GlStateManager.bindTexture(cam.getTexture()); Tessellator tessellator = Tessellator.getInstance(); BufferBuilder bufferbuilder = tessellator.getBuffer(); bufferbuilder.begin(7, DefaultVertexFormats.POSITION_TEX); bufferbuilder.pos(15 * px - 0.001, 1 - px + 0.01, px - 0.01).tex(0.0D, 1.0D).endVertex(); bufferbuilder.pos(15 * px - 0.001, px - 0.01, px - 0.01).tex(0.0D, 0.0D).endVertex(); bufferbuilder.pos(15 * px - 0.001, px - 0.01, 1 - px + 0.01).tex(1.0D, 0.0D).endVertex(); bufferbuilder.pos(15 * px - 0.001, 1 - px + 0.01, 1 - px + 0.01).tex(1.0D, 1.0D).endVertex(); tessellator.draw(); GlStateManager.bindTexture(0); GL11.glTranslated(-x, -y, -z); RenderHelper.enableStandardItemLighting(); GlStateManager.disableRescaleNormal(); GlStateManager.enableLighting(); GlStateManager.popMatrix();
-
[1.12.2] Rendering with Transparency Turns White
deerangle replied to DavidM's topic in Modder Support
I believe this has to do with default lighting. Try putting GlStateManager.disableLighting(); before rendering and GlStateManager.enableLighting(); after rendering. Might also have to do with blending (See GlStateManager) -
Solved it! Under IntelliJ settings I went to Build, Execution, Deployment > Build Tools > Gradle > Runner and disabled the Delegate IDE build/run actions to gradle option. Now it's working like it did before
-
So I just found that my older projects (though using the same forge version) compiled my code and copied my resources to the classes/ directory. Now it generates my class files in build/classes/ and copies the resources to build/resources/. I don't see any kind of configuration for the build target location in the build.gradle file though. Can anyone tell me how I can fix this, and possibly even tell me where this error originates from?
-
Seems like the decompiled source code either doesn't exist or is not properly loaded into eclipse. When setting up your project, did you use setupDevWorkspace or setupDecompWorkspace? If you didn't use the latter, you are going to need to do that in order to get the sources displayed.
-
Uploaded it to GitHub here. Through further debugging I found that the root location from where my the resource domain is loading the resources is at ./build/classes/main instead of ./build/resources for whatever reason. Manually moving my resources into the correct directory works, but is not a solution to the core problem at hand.
-
Hello forge development team, I have an idea about forge optimization
deerangle replied to JackMeds's topic in Suggestions
Why don't you do a pull request to github here? -
I've set up my development environment with IntelliJ using Gradle and I followed this tutorial to create the project. It starts without any errors, but somehow none of my resources are being loaded into the game. I'm very sure this has nothing to do with misspelled resource locations. I ran my project in debug mode to find out that the Minecraft resource manager didn't even load my resource domain into the game (SimpleReloadableResourceManager#domainResourceManagers doesn't contain my resource domain). This is my resources directory: $ find . . ./mcmod.info ./assets ./assets/examplemod ./assets/examplemod/test ./assets/examplemod/test/test.txt ./pack.mcmeta And this code is supposed to load the resource (called directly from the FMLInitializationEvent) : ResourceLocation testLoc = new ResourceLocation(MODID, "test/test.txt"); InputStreamReader reader = new InputStreamReader(Minecraft.getMinecraft().getResourceManager().getResource(testLoc).getInputStream()); BufferedReader r = new BufferedReader(reader); System.out.println(r.readLine()); The full error trace: net.minecraftforge.fml.common.LoaderExceptionModCrash: Caught exception from Example Mod (examplemod) Caused by: java.io.FileNotFoundException: examplemod:test/test.txt at net.minecraft.client.resources.SimpleReloadableResourceManager.getResource(SimpleReloadableResourceManager.java:69) at com.example.examplemod.ExampleMod.init(ExampleMod.java:36) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at net.minecraftforge.fml.common.FMLModContainer.handleModStateEvent(FMLModContainer.java:626) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at com.google.common.eventbus.Subscriber.invokeSubscriberMethod(Subscriber.java:91) at com.google.common.eventbus.Subscriber$SynchronizedSubscriber.invokeSubscriberMethod(Subscriber.java:150) at com.google.common.eventbus.Subscriber$1.run(Subscriber.java:76) at com.google.common.util.concurrent.MoreExecutors$DirectExecutor.execute(MoreExecutors.java:399) at com.google.common.eventbus.Subscriber.dispatchEvent(Subscriber.java:71) at com.google.common.eventbus.Dispatcher$PerThreadQueuedDispatcher.dispatch(Dispatcher.java:116) at com.google.common.eventbus.EventBus.post(EventBus.java:217) at net.minecraftforge.fml.common.LoadController.sendEventToModContainer(LoadController.java:219) at net.minecraftforge.fml.common.LoadController.propogateStateMessage(LoadController.java:197) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at com.google.common.eventbus.Subscriber.invokeSubscriberMethod(Subscriber.java:91) at com.google.common.eventbus.Subscriber$SynchronizedSubscriber.invokeSubscriberMethod(Subscriber.java:150) at com.google.common.eventbus.Subscriber$1.run(Subscriber.java:76) at com.google.common.util.concurrent.MoreExecutors$DirectExecutor.execute(MoreExecutors.java:399) at com.google.common.eventbus.Subscriber.dispatchEvent(Subscriber.java:71) at com.google.common.eventbus.Dispatcher$PerThreadQueuedDispatcher.dispatch(Dispatcher.java:116) at com.google.common.eventbus.EventBus.post(EventBus.java:217) at net.minecraftforge.fml.common.LoadController.distributeStateMessage(LoadController.java:136) at net.minecraftforge.fml.common.Loader.initializeMods(Loader.java:744) at net.minecraftforge.fml.client.FMLClientHandler.finishMinecraftLoading(FMLClientHandler.java:336) at net.minecraft.client.Minecraft.init(Minecraft.java:582) at net.minecraft.client.Minecraft.run(Minecraft.java:422) at net.minecraft.client.main.Main.main(Main.java:118) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) at GradleStart.main(GradleStart.java:25) I strongy suspect that something with my project setup is faulty, since this project is not much more than the default examplemod that comes with the MDK. PS: just noticed this line in the logs, but I don't know what exactly it means: [11:42:54] [Client thread/INFO] [minecraft/SimpleReloadableResourceManager]: Reloading ResourceManager: Default, FMLFileResourcePack:Forge Mod Loader, FMLFileResourcePack:Minecraft Forge, FMLFileResourcePack:Example Mod
-
Where the event is called really depends on the Event and where it is being invoked.
-
Did you run gradle from intellij or from terminal?
-
This is because opengl coordinate space defines the coordinate (x0, y0, z0) as the camera position. In order to have the bounding box displayed around the entity, you would have to translate (and probably also rotate) the bounding box to the entity position. You could check out the code used in minecraft debug mode (Pressing F3+B).
-
The old ISidedInventory interface supports the getSlotsForFace method, but as of newer forge versions one should use Capabilites. How would I implement this using Capabilities? I know that I can control if I want to return a Capability using the EnumFacing attribute of the getCapability function, but how can I make each side of the block correspond to certain slots just like SidedInventory does?