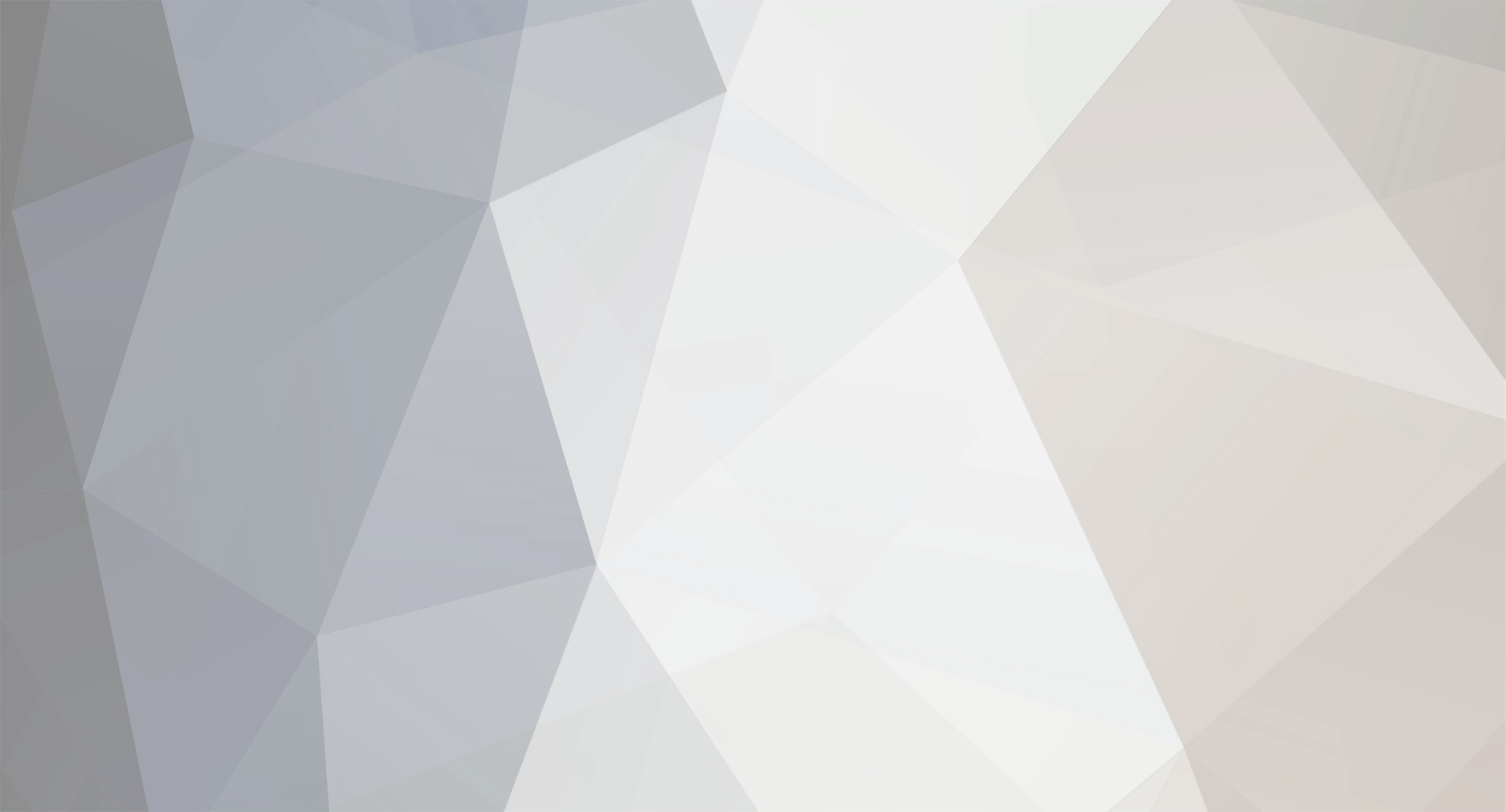
kevinmd88
Members-
Posts
23 -
Joined
-
Last visited
Converted
-
Gender
Male
kevinmd88's Achievements

Tree Puncher (2/8)
0
Reputation
-
Forge 1.14.3 : Is it considered stable?
kevinmd88 replied to kevinmd88's topic in General Discussion
Thank you LexManos - I'm setting up my IDE as we speak! -
Hi all, I haven't been keeping up with Minecraft in the last month or two (real life stuff going on) and I'm delighted to see there's now a 1.13 version on the Files page and even some 1.14 and 1.14.3 releases! Kudos to all the devs, I'm well aware of the painstaking process they've been through there. I'm not here asking for a release date (I read the blue-stripe announcement ), but I wanted to ask, especially any modders who've worked with it already, if the 1.14.3 release (or anything beyond 1.12.2) is considered stable at this time? I'm eager to upgrade and experiment but I also understand things take time and work and am always willing to wait patiently with or without a release date. Just wanted to ask before I started looking at the API and trying to do any modding. Hope everyone understands my intent here! Thank you!
-
To a developer, this thread feels like you're in a software company and you have two dozen managers staring over your shoulder with every key you type wondering if you're done yet. For developers that don't even get paid for what they're doing, this would probably make me want to make one final Delete keypress on the entire project. I know the OP's intentions were good and not the same as the WHY ISNT IT DONE YET WAAAHHH sludge that others have spewed around, but I think this should still be locked due to the way it's continuing after an answer was given. I feel horrible for everyone who got stuck with this project while people nag them this way.
-
Literally read the announcement on the big blue banner at the top of every forum page
-
Anyone else getting a MissingRequiredOptionsException when they attempt to launch the beta? Just wondering if I messed it up. (Edit: FYI I do know it's a beta so issues are expected, I'm interested in beta testing as a developer myself. Just wanted to ask about this one in particular because I haven't found a way around it and it prevents any of it from launching. Hope I'm not crossing any lines in asking here.)
-
[1.12.2] Prevent sand/gravel blocks from falling
kevinmd88 replied to kevinmd88's topic in Modder Support
By replacing them with my own versions, would other recipes that depend on the vanilla versions still function? -
Extreme LAG on Minecraft multiplayer
kevinmd88 replied to Sandrituky's topic in Support & Bug Reports
Also, JourneyMap can implicate extreme lag spikes: Especially if your server is fresh, and even moreso if being hosted from an underpowered desktop PC (relatively speaking by server hardware standards). So, another few steps you could try would be Crowfooted's and InThayne's commented suggestions in that above Reddit thread, regarding checking with Shift+F3 to see if anything's chewing CPU on your client side, and regarding the JRE garbage collection parameter (try that one on both your client and server startup commands). -
Extreme LAG on Minecraft multiplayer
kevinmd88 replied to Sandrituky's topic in Support & Bug Reports
Sorry, I apparently hit Control-Enter which in my browser posted my message prematurely. -
Extreme LAG on Minecraft multiplayer
kevinmd88 replied to Sandrituky's topic in Support & Bug Reports
[00:06:14] [Thread-22/WARN] [THAUMCRAFT]: AURAS TAKING 1320 ms LONGER THAN NORMAL IN DIM 0 [00:06:16] [Server thread/INFO] [STDOUT]: [thut.api.terrain.TerrainSegment:refresh:504]: subBiome refresh took 0.1984083137 [00:06:39] [Server thread/WARN] [net.minecraft.server.MinecraftServer]: Can't keep up! Did the system time change, or is the server overloaded? Running 18045ms behind, skipping 360 tick(s) Those two lines are the only ones reported in the server log after you're detected connecting to it. That is a pretty big tick delay, and Thaumcraft also appears to be the only one in your list with "BETA" in its filename. In any case, beta versions can be unstable (that's why they're beta). I would start by attempting to remove this mod from your installation (and any dependents) and if that doesn't help try other mods one by one until you can find the problem. -
Oh, I see. List<EntityPlayer> entityList = worldIn.getEntitiesWithinAABB(EntityPlayer.class, boundingbox); No need to get the class from an object, when it's available as a static field that doesn't require instantiation. I suppose in this particular case it still technically works since playerIn is available, though it's like taking one step backward to take two forward.
-
It is the code I'm using... Can you clarify what is wrong with it? If I'm doing it the wrong way, I'd like to correct it too
-
@diesieben07 All it took was adding .getClass() to it
-
Perhaps you could use LivingEntityUseItemEvent.Start to store the type of food being eaten somewhere, and then upon LivingEntityUseItemEvent.Finish reference that stored data to determine what they ate. A HashMap<EntityPlayer, ItemStack> might work, otherwise maybe just write a simple NBT string for the player? Something like this, this code probably won't work as-is but might get you started... private Map<EntityPlayer, ItemStack> currentFood = new HashMap<EntityPlayer, ItemStack>(); @SubscribeEvent public void onFoodBeingEaten(LivingEntityUseItemEvent.Start event) { if (event.getEntity() instanceof EntityPlayer) { EntityPlayer player = (EntityPlayer)event.getEntity(); currentFood.put(player, event.getItem()); } } @SubscribeEvent public void onFoodEaten(LivingEntityUseItemEvent.Finish event) { if (event.getEntity() instanceof EntityPlayer) { EntityPlayer player = (EntityPlayer)event.getEntity(); if (currentFood.containsKey(player)) { ItemStack food = currentFood.remove(player); // Do stuff based on food here } } }
-
I'm trying to determine a way to stop blocks like sand and gravel from falling when there's nothing supportive beneath them. I found this topic in my searches: However unfortunately it isn't solved there. I'm not aware of such an event I can subscribe to... Is there an effective way in Forge to handle this? If it helps as to why I'm trying to accomplish this... I'm attempting to port over the CA-Teleport and CA-Static Bukkit plugins that Rumsey wrote for the CraftingAzeroth project over to Forge. I have finished coding for CA-Teleport (though I have not yet tested it), and my question here relates to how to adapt the plugin's onBlockPhysics handler in the Bukkit implementation.