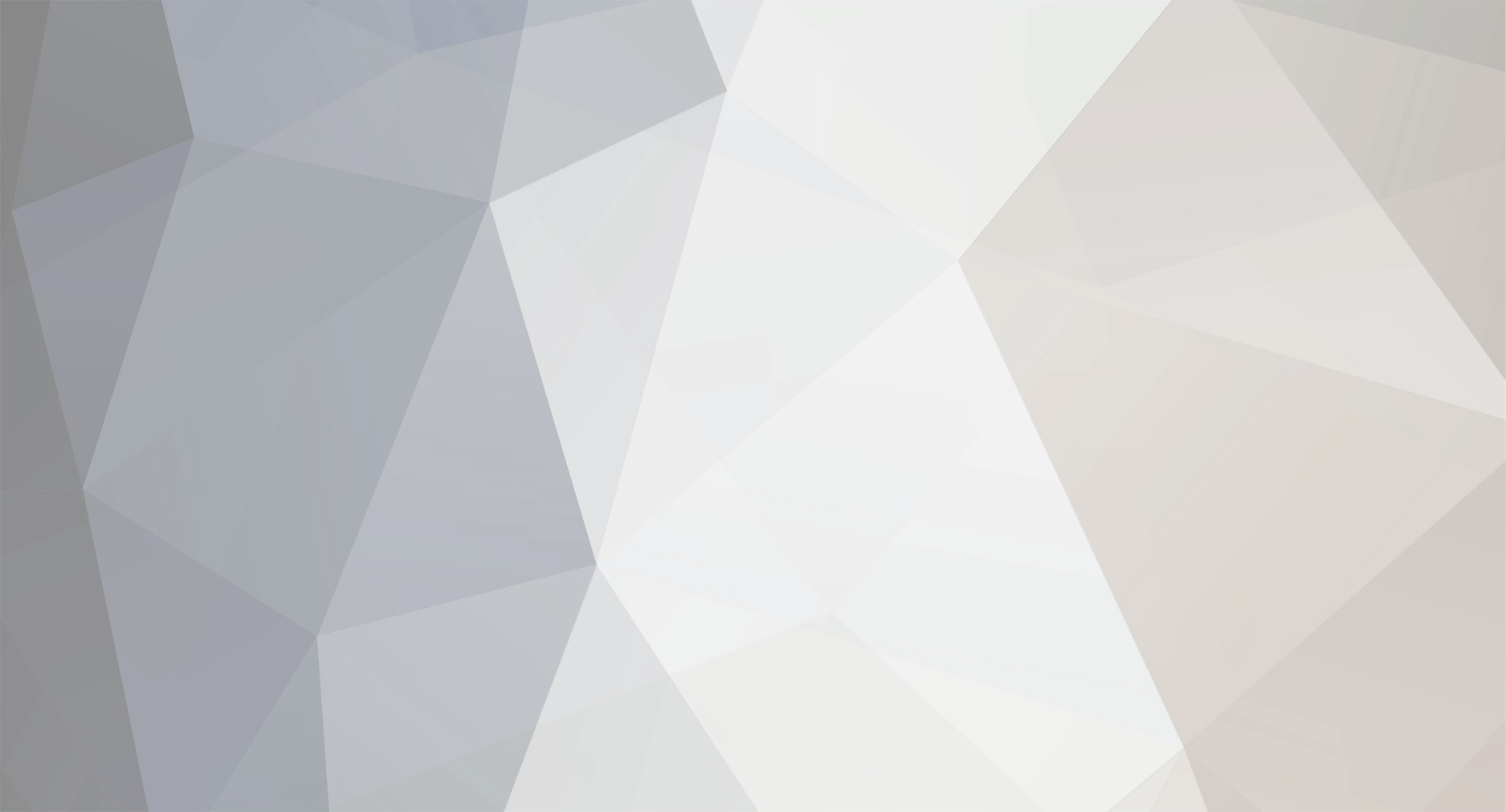
EverythingGames
Forge Modder-
Posts
388 -
Joined
-
Last visited
Everything posted by EverythingGames
-
I've decided that there would be an easier way to do this, I don't know if you necessarily need ISmartItemModel, though. I'm pretty sure you can render two models together. I'm thinking that the class should implement IPerspectiveAwareModel and contain two IBakedModels in the constructor, one being the base model and the other being the model you want to merge with the base model. To merge them, you create a new arraylist containing both the baked quads for the base model and the model you want to merge with then return them in the getGeneralQuads() method. For some reason, that theory doesn't seem to work, it only renders the base model fine, not the model I want to merge with it. If anyone can tell me why, here is my code: IPerspectiveAwareModel Class public class ItemModelPerspective implements IPerspectiveAwareModel { //The model resource locations for the two models (no specific reason to be in this class, but hey) public static final ModelResourceLocation BASEMODEL = new ModelResourceLocation(Main.ID + ":" + "gun", "inventory"); public static final ModelResourceLocation MODELTOMERGE = new ModelResourceLocation(Main.ID + ":" + "modelarm", "inventory"); //The two models - one as a base, the other to merge with it private final IBakedModel baseModel; private final IBakedModel modelToMerge; public ItemModelPerspective(IBakedModel baseModel, IBakedModel modelToMerge) { this.baseModel = baseModel; this.modelToMerge = modelToMerge; } @Override public List getFaceQuads(EnumFacing enumFacing) { return this.baseModel.getFaceQuads(enumFacing); } @Override public List getGeneralQuads() //Combine the general quads in a list and return as one { List<BakedQuad> combinedQuads = new ArrayList(this.baseModel.getGeneralQuads()); combinedQuads.addAll(this.modelToMerge.getGeneralQuads()); return combinedQuads; } @Override public boolean isAmbientOcclusion() { return this.baseModel.isAmbientOcclusion(); } @Override public boolean isGui3d() { return this.baseModel.isGui3d(); } @Override public boolean isBuiltInRenderer() { return this.baseModel.isBuiltInRenderer(); } @Override public TextureAtlasSprite getTexture() { return this.baseModel.getTexture(); } @Override public ItemCameraTransforms getItemCameraTransforms() { return this.baseModel.getItemCameraTransforms(); } @Override public Pair<IBakedModel, Matrix4f> handlePerspective(TransformType transformType) { switch(transformType) { case FIRST_PERSON: return Pair.of(this, ForgeHooksClient.getMatrix(this.baseModel.getItemCameraTransforms().firstPerson)); case THIRD_PERSON: return Pair.of(this, ForgeHooksClient.getMatrix(this.baseModel.getItemCameraTransforms().thirdPerson)); case GUI: return Pair.of(this, ForgeHooksClient.getMatrix(this.baseModel.getItemCameraTransforms().gui)); case HEAD: return Pair.of(this, ForgeHooksClient.getMatrix(this.baseModel.getItemCameraTransforms().head)); default: return null; } } } ModelBakeEvent Class public class ItemModelBake { @SubscribeEvent public void modelBakeEvent(ModelBakeEvent event) { Object baseModel = event.modelRegistry.getObject(ItemModelPerspective.BASEMODEL); Object modelToMerge = event.modelRegistry.getObject(ItemModelPerspective.MODELTOMERGE); if(baseModel instanceof IBakedModel && modelToMerge instanceof IBakedModel) { IBakedModel newBaseModel = (IBakedModel) baseModel; IBakedModel newModelToMerge = (IBakedModel) modelToMerge; ItemModelPerspective finalModel = new ItemModelPerspective(newBaseModel, newModelToMerge); event.modelRegistry.putObject(ItemModelPerspective.BASEMODEL, finalModel); } } } EDIT: The code works completely, renders both the models perfecly other than the weird rotation it has on it. Can someone explain why the new, odd rotation is added to the item model? Thanks.
-
Ahh, your right. I didn't previously check the instanceof for IBakedModel, which caused a ClassCastException. I managed to work with what you were saying (returning the Pair) of IBakedModel and Matrix4f. This code works, however, the model is oddly rotated in a weird way. Here is the code used: @Override public Pair<IBakedModel, Matrix4f> handlePerspective(TransformType transformType) { switch(transformType) { case FIRST_PERSON: return Pair.of(this, ForgeHooksClient.getMatrix(this.baseModel.getItemCameraTransforms().firstPerson)); case THIRD_PERSON: return Pair.of(this, ForgeHooksClient.getMatrix(this.baseModel.getItemCameraTransforms().thirdPerson)); case GUI: return Pair.of(this, ForgeHooksClient.getMatrix(this.baseModel.getItemCameraTransforms().gui)); case HEAD: return Pair.of(this, ForgeHooksClient.getMatrix(this.baseModel.getItemCameraTransforms().head)); default: return null; } }
-
[1.7.10]Looking for tutorials on several subjects of java
EverythingGames replied to Vulpixy's topic in Modder Support
Hi, look into IExtendedEntityProperties for saving data to an entity (the player in your case). You will need packets to sync values between the server and client, take a look at the SimpleNetworkWrapper packet system. You will also need events, (I.E PlayerJoinWorldEvent, to get when each player joins the world - prompt them to pick a class). For the player class, use GUI's - packets may be needed for this as well. Some links for tutorials on these: Events http://jabelarminecraft.blogspot.com/p/minecraft-forge-172-event-handling.html IEEP https://github.com/coolAlias/Tutorial-Demo/blob/master/src/main/java/tutorial/entity/ExtendedPlayer.java SNW http://www.minecraftforge.net/forum/index.php/topic,20135.0.html GUI http://jabelarminecraft.blogspot.com/p/minecraft-modding-configuration-guis.html Hope that helps. -
Isn't that the same logic used in MBE14 ItemModelFlexibleCamera? (I looked at this considering it implemented IPerspectiveAwareModel): @Override public Pair<IBakedModel, Matrix4f> handlePerspective(ItemCameraTransforms.TransformType cameraTransformType) { IBakedModel baseModel = getIBakedModel(); return ((IPerspectiveAwareModel) baseModel).handlePerspective(cameraTransformType); } } I appreciate your help TGG.
-
I'm getting a crash from handlePerspective. And what exactly am I supposed to do within the switch block to change the model? Here's the code: @Override public Pair<IBakedModel, Matrix4f> handlePerspective(TransformType cameraTransformType) { switch(cameraTransformType) { case GUI : //do stuff break; case FIRST_PERSON : //do stuff break; case THIRD_PERSON : //do stuff break; default : //do nothing break; } return ((IPerspectiveAwareModel) this.model).handlePerspective(cameraTransformType); }
-
Thanks for the help, TGG! I'm learning from MBE15 SmartItemChessboardModel I believe it is. Creating the baked quads looks very confusing - is it possible to recreate a 4x4x12 (Length, Width, Height) box (from a model in java) by creating baked quads? Let's say I created those baked quads - would I be able to render them when the player is in first person and de-render them when in third person? I am asking because ISmartItemModel does not include a handlePerspectiveMethod as does IPerspectiveAwareModel (I've seen where both interfaces are implemented). Thanks again.
-
Hi, I want to be able to render two models together as one based on first person, third person, etc. I know IPerspectiveAwareModel can take care of the camera transformations, but I don't know how I would render another model with my main model based on those camera transformations. What is ISmartItemModel and IFlexibleBakedModel used for - and why is a great number of things deprecated in IPerspectiveAwareModel? Thanks in advance!
-
Hi, Why does the model not rotate with the player? I did exactly as the vanilla code does (called all the functions it does with exact replicated code). It renders fine but it does not rotate properly with the player. Any solutions to this? Also, is the RenderHandEvent broken? It gets called when in third person - we already have the RenderPlayerEvent for that. Anyway, here's the working rendering code: @SideOnly(Side.CLIENT) public class RenderEntityPlayer { @SubscribeEvent public void renderHandEvent(RenderHandEvent event) { this.renderArmInFirstPerson(event.partialTicks); } public void renderArmInFirstPerson(float floatOne) { try { EntityPlayerSP entityplayersp = Minecraft.getMinecraft().thePlayer; ItemRenderer itemrenderer = Minecraft.getMinecraft().entityRenderer.itemRenderer; Field fi1 = ItemRenderer.class.getDeclaredField("equippedProgress"); Field fi2 = ItemRenderer.class.getDeclaredField("prevEquippedProgress"); fi1.setAccessible(true); fi2.setAccessible(true); float equippedProgress = fi1.getFloat(itemrenderer); float prevEquippedProgress = fi2.getFloat(itemrenderer); float f1 = 1.0F - (prevEquippedProgress + (equippedProgress - prevEquippedProgress) * floatOne); float f2 = entityplayersp.getSwingProgress(floatOne); float f3 = entityplayersp.prevRotationPitch + (entityplayersp.rotationPitch - entityplayersp.prevRotationPitch) * floatOne; float f4 = entityplayersp.prevRotationYaw + (entityplayersp.rotationYaw - entityplayersp.prevRotationYaw) * floatOne; this.functionOne(f3, f4); this.functionTwo(entityplayersp); this.functionThree(entityplayersp, floatOne); GlStateManager.enableRescaleNormal(); GlStateManager.pushMatrix(); if(!entityplayersp.isInvisible()) { this.renderArm(entityplayersp, f1, f2); } GlStateManager.popMatrix(); GlStateManager.disableRescaleNormal(); RenderHelper.disableStandardItemLighting(); } catch(Exception exception) { exception.printStackTrace(); } } public void functionOne(float floatOne, float floatTwo) { GlStateManager.pushMatrix(); GlStateManager.rotate(floatOne, 1.0F, 0.0F, 0.0F); GlStateManager.rotate(floatTwo, 0.0F, 1.0F, 0.0F); RenderHelper.enableStandardItemLighting(); GlStateManager.popMatrix(); } public void functionTwo(AbstractClientPlayer abstractClientPlayer) { int i = Minecraft.getMinecraft().theWorld.getCombinedLight(new BlockPos(abstractClientPlayer.posX, abstractClientPlayer.posY + (double)abstractClientPlayer.getEyeHeight(), abstractClientPlayer.posZ), 0); float f1 = (float)(i & 65535); float f2 = (float)(i >> 16); OpenGlHelper.setLightmapTextureCoords(OpenGlHelper.lightmapTexUnit, f1, f2); } public void functionThree(EntityPlayerSP entityplayersp, float floatOne) { float f1 = entityplayersp.prevRenderArmPitch + (entityplayersp.renderArmPitch - entityplayersp.prevRenderArmPitch) * floatOne; float f2 = entityplayersp.prevRenderArmYaw + (entityplayersp.renderArmYaw - entityplayersp.prevRenderArmYaw) * floatOne; GlStateManager.rotate((entityplayersp.rotationPitch - f1) * 0.1F, 1.0F, 0.0F, 0.0F); GlStateManager.rotate((entityplayersp.rotationYaw - f2) * 0.1F, 0.0F, 1.0F, 0.0F); } public void renderArm(AbstractClientPlayer abstractClientPlayer, float floatOne, float floatTwo) { float f1 = -0.3F * MathHelper.sin(MathHelper.sqrt_float(floatTwo) * (float) Math.PI); float f2 = 0.4F * MathHelper.sin(MathHelper.sqrt_float(floatTwo) * (float) Math.PI * 2.0F); float f3 = -0.4F * MathHelper.sin(floatTwo * (float) Math.PI); float f4 = MathHelper.sin(floatTwo * floatTwo * (float) Math.PI); float f5 = MathHelper.sin(MathHelper.sqrt_float(floatTwo) * (float) Math.PI); Minecraft.getMinecraft().getTextureManager().bindTexture(abstractClientPlayer.getLocationSkin()); RenderPlayer renderplayer = (RenderPlayer) Minecraft.getMinecraft().getRenderManager().getEntityRenderObject(Minecraft.getMinecraft().thePlayer); renderplayer.func_177138_b(abstractClientPlayer); GlStateManager.translate(f1, f2, f3); GlStateManager.translate(0.64000005F, -0.6F, -0.71999997F); GlStateManager.translate(0.0F, floatOne * -0.6F, 0.0F); GlStateManager.rotate(45.0F, 0.0F, 1.0F, 0.0F); GlStateManager.rotate(f5 * 70.0F, 0.0F, 1.0F, 0.0F); GlStateManager.rotate(f4 * -20.0F, 0.0F, 0.0F, 1.0F); GlStateManager.translate(-1.0F, 3.6F, 3.5F); GlStateManager.rotate(120.0F, 0.0F, 0.0F, 1.0F); GlStateManager.rotate(200.0F, 1.0F, 0.0F, 0.0F); GlStateManager.rotate(-135.0F, 0.0F, 1.0F, 0.0F); GlStateManager.scale(1.0F, 1.0F, 1.0F); GlStateManager.translate(5.6F, 0.0F, 0.0F); } }
-
Hi Have you tried EnumChatFormatting? You said you needed color / italics / bold right? Look at this: ((EntityPlayer) entity).addChatComponentMessage(new ChatComponentTranslation(EnumChatFormatting.RED + "event.slimerain.end")) *May not be 100% accurate code*
-
[Solved][1.7.10] Problem with rendering shadows
EverythingGames replied to wesserboy's topic in Modder Support
Just a thought - GL11 might help out. Look at its various rendering methods. -
Sounds like getPlayerEntityByName should be your best bet. Store the name passed in the GUI and pass it in that method. Read and write the list with the qualified waypoints somehow and send that to the PlayerEntity. That should be solid.
-
Hi You are opening your GUI on both the client and server. Only the client needs to know about this: if(world.isRemote())
-
Take a look at how private messaging works in the vanilla chat. Data is sent between two players based on a player name the sender specified. Basing it off this, you can send this information about the waypoints to the targeted client utilizing packets containing your data. If I'm not mistaken, everything should be client side (sending to client, GUI, etc.).
-
[Solved][1.7.10] Problem with rendering shadows
EverythingGames replied to wesserboy's topic in Modder Support
Hi What happens when you do not use these methods: tessellator.setColorOpaque_F(1F, 1F, 1F); tessellator.setBrightness(block.getMixedBrightnessForBlock(world, x, y, z)); I'm not good at rendering, but there seems to be either something your setting that is causing this, or lightning that needs to be updated when changing the block model frequently. -
Hi Override the isFullCube() boolean in your block class and return false.
-
Hi Try looking at a tutorial on the SimpleNetworkWrapper (SNW). It has various methods such as sending to a client in your situation.
-
Hi In my gun mod, I created a new Entity inheriting from EntityThrowable and I spawn it accordingly. Damage is taken care in that class, try looking at the snowball code when it hits a Blaze adding the damage.
-
Hi I believe its EnumChatColor and stack.stackDisplayName (may not be correctly quoted from code).
-
Hi You need to specify "inventory" in your ModelResourceLocation. This might do it right here: Minecraft.getMinecraft().getRenderItem().getItemModelMesher().register(item, 0, new ModelResourceLocation("test:test", "inventory"));
-
[1.8] Techne Model Not Corresponding To Player
EverythingGames replied to EverythingGames's topic in Modder Support
Sorry, the images aren't doing what I want them to do. Basically the model pieces are bunching up together into 1 piece. The armor stand's rotation points aren't the same either. -
[1.7.10] How to detect when Right Click is not pressed.
EverythingGames replied to noahc3's topic in Modder Support
Try using mouse events. -
[1.8] Techne Model Not Corresponding To Player
EverythingGames replied to EverythingGames's topic in Modder Support
If I set all the rotation offset points to the same area, my model renders oddly. Besides, if I did that I would just be able to call GL.glRotated to rotate the whole model and would achieve the same thing. The armor stand needs to rotate its specific parts and I need to rotate the thing as a whole with the player. -
[1.8] Techne Model Not Corresponding To Player
EverythingGames replied to EverythingGames's topic in Modder Support
Ahh I see what you mean. I just centered them to their own shape. Let me go change them to the same point (middle of the model). -
[1.8] Techne Model Not Corresponding To Player
EverythingGames replied to EverythingGames's topic in Modder Support
Not working. This just makes the individual model parts spin on its axis / rotation points. I need something that will turn the whole model based on player rotation yaw. @Override public void setRotationAngles(float par1Float, float par2Float, float par3Float, float par4Float, float par5Float, float par6Float, Entity par7Entity) { if (par7Entity instanceof EntityPlayer) { EntityPlayer entityplayer = (EntityPlayer) par7Entity; this.baseOne.rotateAngleY = 0.017453292F * entityplayer.rotationYaw; this.baseTwo.rotateAngleY = 0.017453292F * entityplayer.rotationYaw; this.sideOne.rotateAngleY = 0.017453292F * entityplayer.rotationYaw; this.sideTwo.rotateAngleY = 0.017453292F * entityplayer.rotationYaw; this.pouchOne.rotateAngleY = 0.017453292F * entityplayer.rotationYaw; this.pouchTwo.rotateAngleY = 0.017453292F * entityplayer.rotationYaw; } } -
[1.8] Techne Model Not Corresponding To Player
EverythingGames replied to EverythingGames's topic in Modder Support
I tried all the values individually in that line by plugging in Minecraft.getMinecraft().thePlayer.rotationYaw. None of those seemed to do anything.