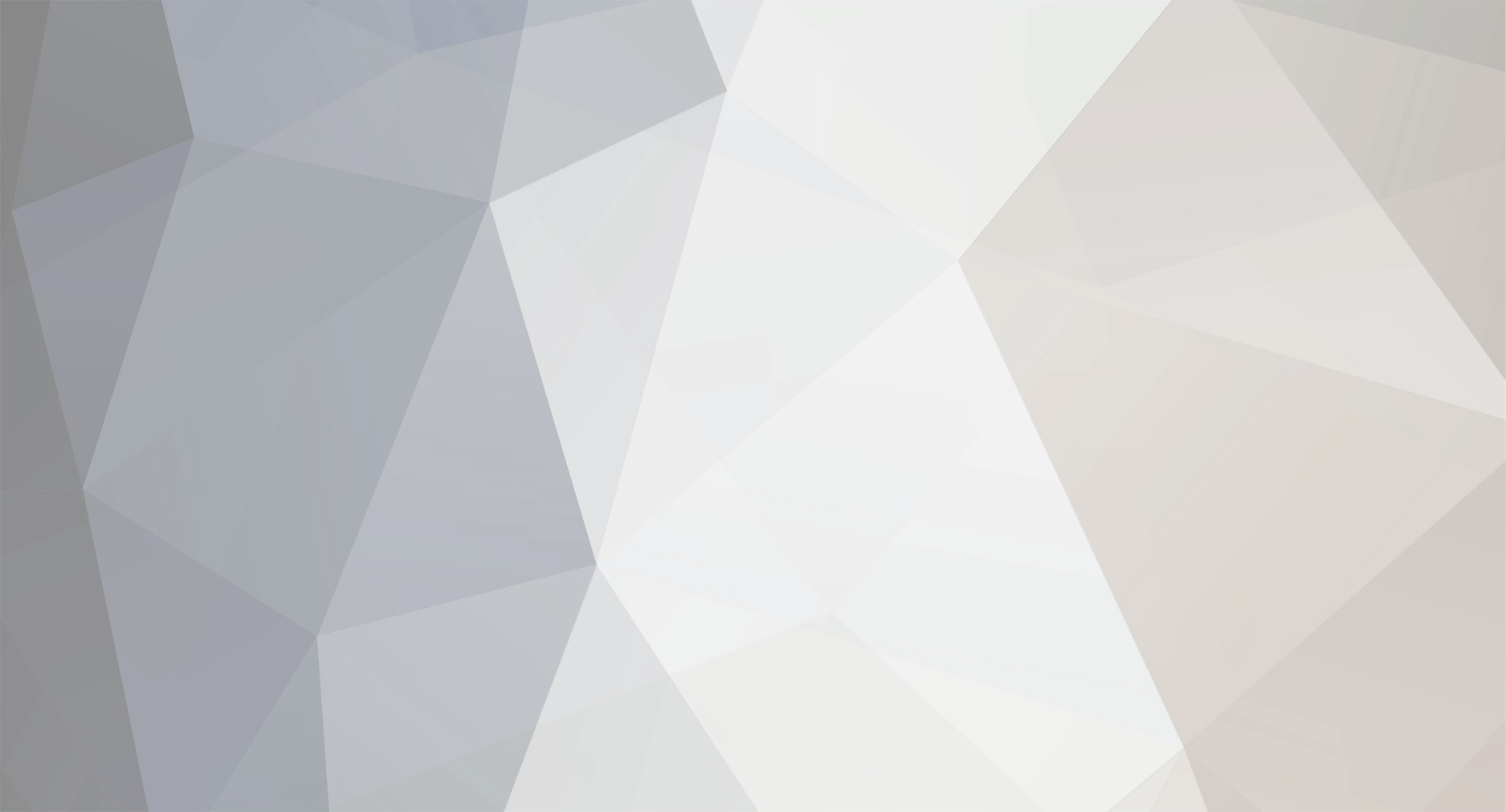
EverythingGames
Forge Modder-
Posts
388 -
Joined
-
Last visited
Everything posted by EverythingGames
-
Then create a random object and use the nextInt() method. The random code should not be difficult.
-
C'mon man, I haven't used that event because my mod doesn't involve what your doing, but I'm guessing the list within the event - what other list is there?
-
Hi, that random generated int looks confusing, the event code looks correct (at a quick glance). Here is an cleaner alternative which will convert a double to an int, while giving you a value 0-3 inclusive (1 or 2): @SubscribeEvent public void livingDropsEvent(LivingDropsEvent event) { Item item = YOURITEM; int random = (int) Math.random * 3; if(event.entityLiving instanceof EntitySquid) { event.entityLiving.dropItem(item, random); } }
-
[1.8][SOLVED] question about @SubscribeEvent
EverythingGames replied to Chewiie88's topic in Modder Support
Hi, it depends on what your doing, I believe the way it works is - when you register your events you can have multiple client side events in the same class and register them through your client proxy and vice versa with server side events (I may be wrong on registering them in sperate classes based on server / client side, but I do know for a fact you can use multiple events in same class). It would be redundant if you used multiple events that are exaclty the same with different method names, however. Hope that helps, here's a piece of info on events including a almost full, if not full, kist of events: http://jabelarminecraft.blogspot.com/p/minecraft-forge-172-event-handling.html -
An easy way of doing this - make two models - one the blade, the other the handle. Create a class implementing ISmartItemModel containg two IBakedModel fields and initialize them in the constructor. In every method not including getFaceQuads(), getGeneralQuads(), and handleItemState(), return this.FIRSTBAKEDMODELFIELDNAME.similarMethodName(). In your getFace / getGeneral Quads methods, you should create an array of quads for the first bakedmodel (sword blade) and the secoond bakedmodel (sword handle) and combine them - finally, return them in that method. Here is a useful example (Sorry for the crazy indentations, I tried to fix most): public class SmartSwordModel implements ISmartItemModel { private final IBakedModel blade; private final IBakedModel handle; public SmartItemModel(IBakedModel blade, IBakedModel handle) { this.blade = blade; this.handle = handle; } @Override public List getFaceQuads(EnumFacing enumFacing) { List<BakedQuad> bladeQuads= new ArrayList<BakedQuad>(this.blade.getFaceQuads(enumFacing)); List<BakedQuad> handleQuads = new ArrayList<BakedQuad>(this.handle.getFaceQuads(enumFacing)); return //Combine the two quad arraylists and return as one; make sure your models are in the correct pos } @Override public List getGeneralQuads() { List<BakedQuad> bladeQuads= new ArrayList<BakedQuad>(this.blade.getGeneralQuads()); List<BakedQuad> handleQuads= new ArrayList<BakedQuad>(this.handle.getGeneralQuads()); return //Combine the two quad arraylists and return as one; make sure your models are in the correct pos } @Override public boolean isAmbientOcclusion() { return this.blade.isAmbientOcclusion(); } @Override public boolean isGui3d() { return this.blade.isGui3d(); } @Override public boolean isBuiltInRenderer() { return this.blade.isBuiltInRenderer(); } @Override public TextureAtlasSprite getTexture() { return this.blade.getTexture(); } @Override public ItemCameraTransforms getItemCameraTransforms() { return this.blade.getItemCameraTransforms(); } @Override public IBakedModel handleItemState(ItemStack stack) { return this; // Return "this" - this is an instanceof IBakedModel - you can return different models here based //on ItemStack information such as NBT } }
-
That is because you are extending BlockFurnace. Don't do that. You are also copying all the code from BlockFurnace and overriding it in your campfire block class. Don't do that. What you need to be doing is creating your own block class and code your own custom furnace from the ground up - there has to be work done if you want something done correctly and efficiently.
-
Hi, as the the title says, I'd like to be able to modify the texture of a BakedQuad from a model. Is this possible with ISmartItemModel? Can I replace the whole model's texture? I am asking because I am doing some work with ISmartItemModel and I need to be able to modify a model's texture (the model contains only one BakedQuad, as it is a replica of the vanilla player arm). Any help is much appreciated, if you can explain if this is possible and which class to use, I'd much appreciate it. Thanks.
-
Hi, I'm pretty sure you can use ISmartBlockModel for this to return different IBakedModels based on NBT. I'm assuming this is correct considering you can do this with items. For example, one block model has the stone texture and another has the grass. When you send your command, have the player hold the block you want to change and convert that into an itemstack. Then, save NBT to it saying to render whatever block you typed in your command - test if the NBT boolean is true, and if it is return the new model in handleBlockState(), I believe it's called (may be named differently). This way, the new models you returned will contain the different textures. Heck, for your ModelResourceLocations just use the vanilla .JSON block models. This is completely untested, I've only used ISmartItemModel and I am yet to use ISmartBlockModel (some names may be incorrect), but in theory this should work as it does in items.
-
Hi, this is quite tricky as the vanilla code causes the hand in first person to move downward out of visible range (or it de-renders completely). You have a few options: 1.) Use the RenderHandEvent to render a new hand in it's place depending on if you are holding an item. 2.) Try to figure a way through the RenderPlayerAPI - not recommended 3.) Use the new modeling system to render the arm and bind the skin to the BakedQuad (arm). (Basically the arm is a part of the item) Here is what I have done for a sword rendering a player arm with it: First, I made an item (for example a steel sword) through .JSON - [MODEL 1] Second, I made the same exact item model through .JSON except this model has the arm holding it (keep in mind this arm is a part of the model) - [MODEL 2] Considering above, MODEL 1 is used for third person / gui rendering. MODEL 2 is used for first person rendering (because MODEL 2 has the player arm in it). The way I done this was creating a class with sub-classes within itself - one wrapper class implementing ISmartItemModel, the other wrapper class implementing IPerspectiveAwareModel. ISmartItemModel customizes the model based on NBT data, the IPerspectiveAwareModel customizes the model based on camera transforms (First Person, Third Person, GUI, Head, None). Basically, in IPerspectiveAwareModel, you return a list of faceQuads and generalQuads for what you want to render. In ISmartItemModel, you return an IBakedModel - I return different models based on NBT. Here is a topic of myself solving the similar issue: http://www.minecraftforge.net/forum/index.php/topic,32309.0.html
-
Thanks, TGG. If you figure out why the matricies are messed up and you know how to fix it, I would greatly appreciate it if you could show me how to fix it - refactoring model transforms in .JSON code is a pain when you've already done it beforehand and IPerspective messes it up. If it turns out to be a bug, maybe you could see to it getting fixed by Forge by reporting the bug - its really making the modeling system more work than it needs to be. Again, thanks!
-
Just like @Ernio said, bake models for each provided texture. Create a wrapper class implementing ISmartItemModel and return different lists of baked quads in the getFaceQuads() and getGeneralQuads() methods. To do this with your item NBT, you need to provide various conditions in the handleItemState(ItemStack itemStack) method. I've been doing work similar to this, it is extremely hard! Be ready to code for a while, but with a little effort, it can be done. EDIT: Changing BakedQuads / drawing new ones is going to be a whole lot harder, I recommend using a .JSON model creator and create all the pieces, then place them into code, bake them, and then return whichever model piece you want to be rendered based on NBT information - to me, this seems easier but do as best pleases yourself. Great work there, @Ernio!
-
Well what do you know, a few good hours of coding paid off. I got the model to do exaclty what I want. TGG, I did something very similar to your suggestion by using ISmartItemModel and customizing the model base on the handleItemState() method (by NBT, of course). I set NBT in pickup / drop entityitem methods to control entity item rendering. In the SmartItemModel class, I returned "this" if the NBT boolean was true, else I returned an IPerspectiveAwareModel to hande drawing of the new model. The odd rotation from earlier doesn't effect the entityitem model, because it being an ISmartItemModel - though it still effects the first person / IPerspectiveAwareModel due to the Matrix4f - maybe you could ask RainWarrior why and see if he has a better way of grabbing the model's transform matricies. Anyway, thanks for all the help TGG, hope this topic helps others as well!
-
Ah, true on that one, yet the class is made for creating many objects because I need to wrap a lot of models. Anyway, you are exacly right on the entity item having no transform, this can be proven with this method I wrote earlier to see if I could change the perspective, thinking that TransofrmTyoe.NONE is the entity item transform - which it isn't: @Override public List getGeneralQuads() { List<BakedQuad> base = new ArrayList<BakedQuad>(this.baseModel.getGeneralQuads()); List<BakedQuad> merge = new ArrayList<BakedQuad>(this.mergeModel.getGeneralQuads()); List<BakedQuad> combined = new ArrayList<BakedQuad>(); combined.addAll(base); combined.addAll(merge); switch(this.transform) { case FIRST_PERSON : return combined; case THIRD_PERSON : return base; case GUI : return base; case HEAD : return base; case NONE : return base; default : return base; } } The odd thing is, the default .JSON model used for the item (not wrapped model - without IPerspectiveAwareModel) is the baseModel E.G. the model not merged with the other model / the third person model is the default model. For some reason when the entity item enters the world, it gets the current transform which leads me to another question - I thought the generalQuads method pre-determined the transform to get ready for rendering, so it looks like when you throw the item down it already wants to use the transfom type first person. Is there any event that can set the model or any other suggestions? TGG I'll be taking a look at your suggestions in the meantime. Cheers!
-
That worked, TGG! Thanks! I can't believe all the times I tried to check the transform type I never thought of saving it in a global field like that. There came another problem - when is this ever going to end, haha - the entity item (when you drop the item on the ground) uses the first person model instead of the wanted third person model. That should be the exact last problem, thanks.
-
[1.8] Potion Effect rendering names and pictures
EverythingGames replied to Ytt's topic in Modder Support
Can you further describe the problem and/or include a photo? Do you mean that the string and the icon is not matching up based on the screen width / height? If so, use ScaledResolution (I'm pretty sure you must use this rather than just finding the center of the screen and drawing from there, but I could be wrong): @SubscribeEvent public void renderOverlay(RenderGameOverlayEvent.Post event) { ScaledResolution scaledresolution = new ScaledResolution(mc, mc.displayWidth, mc.displayHeight); int posX = scaledresolution.getScaledWidth() / 2 + SOME INTEGER; //Get the center of the screen width and add from there int posY = scaledresolution.getScaledHeight() / 2 + SOME INTEGER; //Get the center of the screen height and add from there this.drawString(mc.fontRendererObj, "SOME STRING", posX, posY, 0xFFFFFF); //Render as you please } Make sure you use the post subclass for RenderGameOverlayEvent to avoid rendering HUD issues: @SubscribeEvent public void renderOverlay(RenderGameOverlayEvent.Post event) { //Check element type, if cancelable, etc. } -
I appreciate your reply, TGG. So should I just null check each perspective to see if the model returns a first person, third person, etc. perspective, like this?: if(this.baseModel.getItemCameraTransforms().firstPerson != null) combined.addAll(this.mergeModel.getGeneralQuads()); Is there a proper way of checking these? The odd rotation caused by the changed matrix can be fixed by going into the .JSON code - sadly that makes the model incompatible with your great and so very useful ItemCameraTransform tool. Thanks. EDIT: Above code doesn't work, still renders both models. BTW, does the camera transform "NONE" refer to the entity item when it is on the ground? I'd like to know this because I need to return the third person model model for the entity item as well. Thanks!
-
This works, it may be very incorrect, but it works. The only thing wrong with it is that you can see the extra model when in your survival inventory (when you can see the player - the 2x2 crafting grid with your player next to it): @Override public List getFaceQuads(EnumFacing enumFacing) { List<BakedQuad> combined = new ArrayList<BakedQuad>(this.baseModel.getFaceQuads(enumFacing)); if(Minecraft.getMinecraft().gameSettings.thirdPersonView == 0) combined.addAll(this.mergeModel.getFaceQuads(enumFacing)); return combined; } @Override public List getGeneralQuads() { List<BakedQuad> combined = new ArrayList<BakedQuad>(this.baseModel.getGeneralQuads()); if(Minecraft.getMinecraft().gameSettings.thirdPersonView == 0) combined.addAll(this.mergeModel.getGeneralQuads()); return combined; } EDIT: Just wanted to clarify, the obove code was tested in a class implementing ISmartItemModel only, an earlier post with code contains the IPerspectiveAwareModel code. The IPerspectiveAwareModel class's handlePerspectiveMethod() changes the rotation of the model for some reason, while ISmartItemModel does not (obviously because of Matrix4f).
-
Minecraft Forge Modding 1.8 Problem - Textures Mix
EverythingGames replied to Mr.sKrabs's topic in Modder Support
@OP if you can't figure it out from there...try harder. Ernio has given you ALL the code. What part was so hard? -
Minecraft Forge Modding 1.8 Problem - Textures Mix
EverythingGames replied to Mr.sKrabs's topic in Modder Support
What your doing is a very basic way of registering and rendering items. Personally, I store items in a list and iterate through them using Java 8's new lambda expressions (I.e forEach) to register and render. Point is, there are many ways to register / render items, just a tip. The registerRender(Item item) just saves time by shortening code, while the registerRender() method contains all the lines of code that call registerRender(Item item) with the item you want to render as the parameter. Hope that was a better explanation than my last post. -
Minecraft Forge Modding 1.8 Problem - Textures Mix
EverythingGames replied to Mr.sKrabs's topic in Modder Support
In your registerRender(Item item) method, it should only contain one line of code registering the item parameter not specific items you made. What do you think you have the item parameter for? This is really basic Java man. -
Minecraft Forge Modding 1.8 Problem - Textures Mix
EverythingGames replied to Mr.sKrabs's topic in Modder Support
Hi, your registerRender() method logic is messed up. Your registering specific items in there when you should use your Item parameter. That's the whole point of having a parameter in a method - you call that method with whatever item you want to render. Call it in your registerRenders method. Incorrect Java. -
That's fine TGG, it can be fixed with some simple tweaking in the JSON file - that seems to solve the problem. Can you rather show me how to return a list of baked quads vs. another list of baked quads based on the camera transform? I'm confused on if I should do this in the getGeneralQuads() method or somehow handle it in the handlePerspectiveMethod (tell the model which quads to use there somehow). If you can, tell me what method to do this in and what I should call as a boolean to test if it's first person, third, etc. If I can get that working, we should be good to go! Thanks.