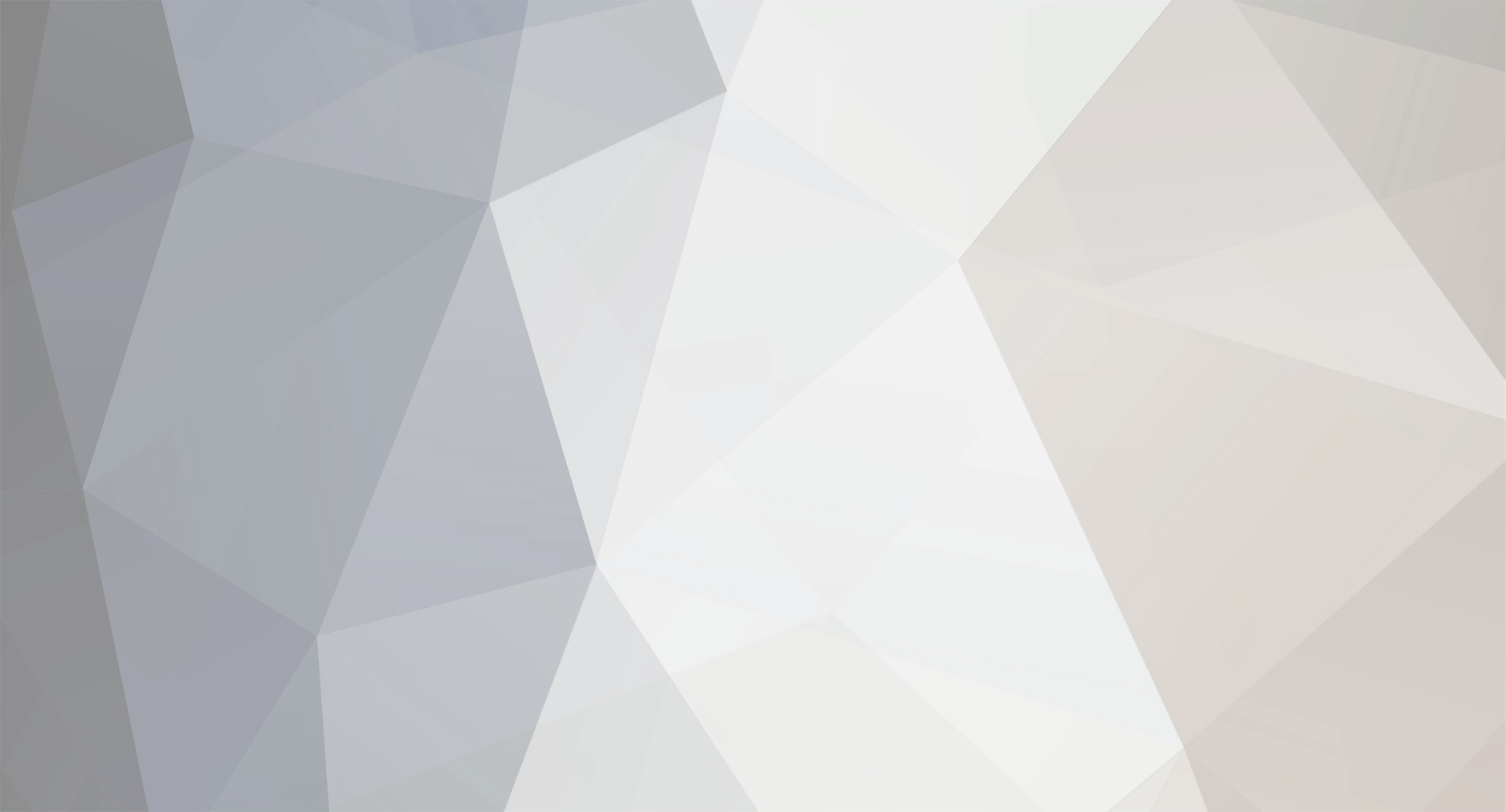
HenryRichard
Members-
Posts
42 -
Joined
-
Last visited
Everything posted by HenryRichard
-
[1.8]Make a block react on Redstone input
HenryRichard replied to ItsAMysteriousYT's topic in Modder Support
This works for me: public void onBlockAdded(World worldIn, BlockPos pos, IBlockState state) { super.onBlockAdded(worldIn, pos, state); if(worldIn.isBlockPowered(pos)) { //Do stuff } else { //Do other stuff } } /** * Called when a neighboring block changes. */ public void onNeighborBlockChange(World worldIn, BlockPos pos, IBlockState state, Block neighborBlock) { if(worldIn.isBlockPowered(pos)) { //Do stuff } else { //Do other stuff } } -
If it's loaded I have no idea. Sorry! EDIT: Wait - shouldn't the Blocks in Blocks.stone.getDefaultState() be capatalized?
-
It's trying to replace the block at 0, 4, 0 - if that's not loaded, it won't work. Also, what do I know, but I'd use !event.work.isRemote() instead of event.side.isServer().
-
[1.7.10] Missing Resources for my domain
HenryRichard replied to Dragonmasterk's topic in Modder Support
Your assets should use "textures" not "texture." -
I don't know exactly how, but it seems your quotation marks around your texture path are... different. I'm not sure why, but if you change it it should fix it. Use this: { "parent":"builtin/generated", "textures": { "layer0":"modid:items/test" }, "display": { "thirdperson": { "rotation": [ -90, 0, 0 ], "translation": [ 0, 1, -3 ], "scale": [ 0.55, 0.55, 0.55 ] }, "firstperson": { "rotation": [ 0, -135, 25 ], "translation": [ 0, 4, 2 ], "scale": [ 1.7, 1.7, 1.7 ] } } }
-
I'm trying to make a block that changes light level based on it's metadata. I've got it to kind-sorta work, but not very well - it only works if I use /setblock. This is the code: package com.henrich.epicwasteoftime.blocks; import com.henrich.epicwasteoftime.EWOTCreativeTabs; import net.minecraft.block.Block; import net.minecraft.block.material.Material; import net.minecraft.block.properties.IProperty; import net.minecraft.block.properties.PropertyBool; import net.minecraft.block.state.BlockState; import net.minecraft.block.state.IBlockState; import net.minecraft.creativetab.CreativeTabs; import net.minecraft.util.BlockPos; import net.minecraft.world.IBlockAccess; import net.minecraft.world.World; public class PinkiteBlock extends Block { public static final PropertyBool POWERED = PropertyBool.create("powered"); protected static final int onLight = (int)(15.0F * 1f); protected static final int offLight = (int)(15.0F * 0f); public PinkiteBlock(String unlocalizedName, Material material) { super(material); this.setUnlocalizedName(unlocalizedName); this.setDefaultState(this.blockState.getBaseState().withProperty(POWERED, false)); this.setCreativeTab(EWOTCreativeTabs.ewotBlocks); } public PinkiteBlock(String unlocalizedName) { this(unlocalizedName, Material.rock); } @Override protected BlockState createBlockState() { return new BlockState(this, new IProperty[] { POWERED }); } @Override public IBlockState getStateFromMeta(int meta) { switch(meta) { case 0: return getDefaultState().withProperty(POWERED, false); case 1: return getDefaultState().withProperty(POWERED, true); default: return getDefaultState().withProperty(POWERED, false); } } @Override public int getMetaFromState(IBlockState state) { return state == this.getDefaultState() ? 0 : 1; } @Override /** * Get a light value for the block at the specified coordinates, normal ranges are between 0 and 15 * * @param world The current world * @param pos Block position in world * @return The light value */ public int getLightValue(IBlockAccess world, BlockPos pos) { Block block = world.getBlockState(pos).getBlock(); if (block != this) { return block.getLightValue(world, pos); } return world.getBlockState(pos) == this.getDefaultState() ? offLight : onLight; } public void onBlockAdded(World worldIn, BlockPos pos, IBlockState state) { super.onBlockAdded(worldIn, pos, state); worldIn.setBlockState(pos, this.blockState.getBaseState().withProperty(POWERED, worldIn.isBlockPowered(pos))); worldIn.markBlockForUpdate(pos); } /** * Called when a neighboring block changes. */ public void onNeighborBlockChange(World worldIn, BlockPos pos, IBlockState state, Block neighborBlock) { worldIn.setBlockState(pos, this.blockState.getBaseState().withProperty(POWERED, worldIn.isBlockPowered(pos))); worldIn.markBlockForUpdate(pos); } }
-
Try re-saving the item textures. That worked for me.
-
[1.7.10]Custom shovel with potion effects
HenryRichard replied to theOriginalByte's topic in Modder Support
Don't use speeds of haste higher than about 3, it messes up the swing animation. -
[1.7.10]Custom texture mapping for ModelRender
HenryRichard replied to yo4you's topic in Modder Support
Couldn't you use more than one texture? I don't do much entity rendering, but I know with blocks you can do that. If not can't you just make the texture bigger? If all else fails you can make 6 individual cuboids that have a thickness of 0 with a different texture for each arranged in a cube (I did a bad job of explaining it, but I hope you get the idea). -
[1.8] Can you help me understand Y in chunks
HenryRichard replied to wmcritter's topic in Modder Support
Chunks are 16x256x16 (xyz). They are saved to disk in 16x16x16 sections so that empty areas don't take up space, but they're generated 16x256x16 (still xyz). -
It seems kinda dumb that I have to do that; but I didn't make the language, I just (try to learn how to) use it. Thanks, it's working fine now. For anyone who's interested, here's the code I used in CropsBlock: package com.henrich.epicwasteoftime.blocks; import java.util.ArrayList; import java.util.List; import java.util.Random; import com.henrich.epicwasteoftime.EWOTCreativeTabs; import com.henrich.epicwasteoftime.init.EWOTItems; import com.henrich.epicwasteoftime.init.EWOTPlants; import net.minecraft.block.Block; import net.minecraft.block.BlockBush; import net.minecraft.block.BlockCrops; import net.minecraft.block.IGrowable; import net.minecraft.block.material.Material; import net.minecraft.block.properties.IProperty; import net.minecraft.block.properties.PropertyInteger; import net.minecraft.block.state.BlockState; import net.minecraft.block.state.IBlockState; import net.minecraft.creativetab.CreativeTabs; import net.minecraft.init.Blocks; import net.minecraft.init.Items; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.util.BlockPos; import net.minecraft.world.IBlockAccess; import net.minecraft.world.World; import net.minecraftforge.common.EnumPlantType; public class CropsBlock extends BlockCrops { public final int minCrop; public final int maxCrop; public CropsBlock(String unlocalizedName, int minCrop, int maxCrop) { super(); this.setUnlocalizedName(unlocalizedName); this.minCrop = minCrop; this.maxCrop = maxCrop; this.setDefaultState(getDefaultState().withProperty(AGE, 0)); } @Override protected Item getSeed() { return null; } @Override protected Item getCrop() { return null; } @Override public EnumPlantType getPlantType(net.minecraft.world.IBlockAccess world, BlockPos pos) { return EnumPlantType.Crop; } public static class Beetroot extends CropsBlock { public Beetroot(String unlocalizedName) { super(unlocalizedName, 1, 3); } @Override protected Item getSeed() { return EWOTPlants.beetrootSeeds; } @Override protected Item getCrop() { return EWOTItems.beetroot; } @Override public List<ItemStack> getDrops(IBlockAccess world, BlockPos pos, IBlockState state, int fortune) { List<ItemStack> ret = new ArrayList<ItemStack>(); Random rand = world instanceof World ? ((World)world).rand : RANDOM; int age = ((Integer)state.getValue(AGE)).intValue(); if (age >= 7) { int k = 3 + fortune; for (int i = 0; i < 3 + fortune; ++i) { if (rand.nextInt(15) <= age) { ret.add(new ItemStack(this.getSeed(), 1, 0)); } } int j = age == 7 ? (this.minCrop >= this.maxCrop ? this.minCrop : this.minCrop + rand.nextInt(this.maxCrop - this.minCrop + 1)) : 0; for (int i = 0; i < j; ++i) ret.add(new ItemStack(this.getCrop(), 1, 0)); } return ret; } } } ...And I used this to register it (before I registered the seeds) beetrootCrop = new CropsBlock.Beetroot("crop_beetroot"); GameRegistry.registerBlock(beetrootCrop, "crop_beetroot");
-
I'm trying to make a crop, but I'm having trouble with the order I instantiate things in. When I register the seeds before the block, I get a NullPointerException when I use the seeds (I understand why, so you don't need to explain it). But if I register the seeds after the block, the block doesn't drop any seeds! The block drops seeds just fine if I tell it to drop Items.wheat_seeds, and it drops EWOTItems.beetroot too, just none of the seeds that will actually grow the plant. Here's my code: package com.henrich.epicwasteoftime.init; import net.minecraft.block.Block; import net.minecraft.init.Blocks; import net.minecraft.init.Items; import net.minecraft.item.Item; import net.minecraftforge.fml.common.registry.GameRegistry; import com.henrich.epicwasteoftime.blocks.CropsBlock; import com.henrich.epicwasteoftime.items.SeedItem; public class EWOTPlants { public static Item beetrootSeeds; public static Block beetrootCrop; public static void createPlants () { beetrootCrop = new CropsBlock("crop_beetroot", beetrootSeeds, EWOTItems.beetroot); beetrootSeeds = new SeedItem("beetroot_seeds", beetrootCrop, Blocks.farmland); GameRegistry.registerBlock(beetrootCrop, "crop_beetroot"); GameRegistry.registerItem(beetrootSeeds, "beetroot_seeds"); } } package com.henrich.epicwasteoftime.items; import com.henrich.epicwasteoftime.EWOTCreativeTabs; import net.minecraft.block.Block; import net.minecraft.creativetab.CreativeTabs; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.init.Blocks; import net.minecraft.item.Item; import net.minecraft.item.ItemSeeds; import net.minecraft.item.ItemStack; import net.minecraft.util.BlockPos; import net.minecraft.util.EnumFacing; import net.minecraft.world.World; import net.minecraftforge.common.EnumPlantType; public class SeedItem extends ItemSeeds { private final Block crops; public SeedItem(String unlocalizedName, Block crops, Block soil) { super(crops, soil); this.crops = crops; this.setUnlocalizedName(unlocalizedName); this.setCreativeTab(EWOTCreativeTabs.ewotItems); } @Override public net.minecraftforge.common.EnumPlantType getPlantType(net.minecraft.world.IBlockAccess world, BlockPos pos) { return EnumPlantType.Crop; } } package com.henrich.epicwasteoftime.blocks; import java.util.List; import java.util.Random; import com.henrich.epicwasteoftime.EWOTCreativeTabs; import net.minecraft.block.Block; import net.minecraft.block.BlockBush; import net.minecraft.block.BlockCrops; import net.minecraft.block.IGrowable; import net.minecraft.block.material.Material; import net.minecraft.block.properties.IProperty; import net.minecraft.block.properties.PropertyInteger; import net.minecraft.block.state.BlockState; import net.minecraft.block.state.IBlockState; import net.minecraft.creativetab.CreativeTabs; import net.minecraft.init.Blocks; import net.minecraft.init.Items; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.util.BlockPos; import net.minecraft.world.World; public class CropsBlock extends BlockCrops { public final Item seed; public final Item crop; public final int minCrop; public final int maxCrop; public CropsBlock(String unlocalizedName, Item seed, Item crop, int minCrop, int maxCrop) { super(); this.setUnlocalizedName(unlocalizedName); this.seed = seed; this.crop = crop; this.minCrop = minCrop; this.maxCrop = maxCrop; this.setDefaultState(getDefaultState().withProperty(AGE, 0)); } public CropsBlock(String unlocalizedName, Item seed, Item crop) { this(unlocalizedName, seed, crop, 1, 1); } @Override protected Item getSeed() { return seed; } @Override protected Item getCrop() { return crop; } @Override public net.minecraftforge.common.EnumPlantType getPlantType(net.minecraft.world.IBlockAccess world, BlockPos pos) { return net.minecraftforge.common.EnumPlantType.Crop; } } (EWOTPlants.createPlants() is called in the preInit of my CommonProxy)
-
While I don't know how to fix this, I'm pretty sure that that's not how you use hitEntity. I believe it's used more like this: @Override public boolean hitEntity(ItemStack item, EntityLivingBase target, EntityLivingBase player) { target.worldObj.addWeatherEffect(new EntityLightningBolt(target.worldObj, target.posX, target.posY, target.posZ)); item.damageItem(1, player); return true; } (this code is for a lightning sword, but I think you get the idea)
-
I discovered that my problem was that I forgot to actually set iblockstate1 to equal the new value. The new code is this: package com.henrich.epicwasteoftime.blocks; import net.minecraft.block.Block; import net.minecraft.block.state.IBlockState; import net.minecraft.entity.Entity; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.item.ItemBlock; import net.minecraft.item.ItemStack; import net.minecraft.util.BlockPos; import net.minecraft.util.EnumFacing; import net.minecraft.world.World; public class ItemPillarBlock extends ItemBlock { public ItemPillarBlock(Block block) { super(block); if (!(block instanceof IMetaBlockName)) { throw new IllegalArgumentException(String.format("The given Block %s is not an instance of ISpecialBlockName!", block.getUnlocalizedName())); } this.setMaxDamage(0); } /** * Called when a Block is right-clicked with this Item * * @param pos The block being right-clicked * @param side The side being right-clicked */ public boolean onItemUse(ItemStack stack, EntityPlayer playerIn, World worldIn, BlockPos pos, EnumFacing side, float hitX, float hitY, float hitZ) { IBlockState iblockstate = worldIn.getBlockState(pos); Block block = iblockstate.getBlock(); if (!block.isReplaceable(worldIn, pos)) { pos = pos.offset(side); } if (stack.stackSize == 0) { return false; } else if (!playerIn.canPlayerEdit(pos, side, stack)) { return false; } else if (pos.getY() == 255 && this.block.getMaterial().isSolid()) { return false; } else if (worldIn.canBlockBePlaced(this.block, pos, false, side, (Entity)null, stack)) { int i = this.getMetadata(stack.getMetadata()); IBlockState iblockstate1 = this.block.onBlockPlaced(worldIn, pos, side, hitX, hitY, hitZ, i, playerIn); switch(side) { case UP: case DOWN: iblockstate1=iblockstate1.withProperty(PillarBlock.ORIENTATION, PillarBlock.EnumOrientation.Y); break; case NORTH: case SOUTH: iblockstate1=iblockstate1.withProperty(PillarBlock.ORIENTATION, PillarBlock.EnumOrientation.Z); break; case EAST: case WEST: iblockstate1=iblockstate1.withProperty(PillarBlock.ORIENTATION, PillarBlock.EnumOrientation.X); break; default: break; } if (placeBlockAt(stack, playerIn, worldIn, pos, side, hitX, hitY, hitZ, iblockstate1)) { worldIn.playSoundEffect((double)((float)pos.getX() + 0.5F), (double)((float)pos.getY() + 0.5F), (double)((float)pos.getZ() + 0.5F), this.block.stepSound.getPlaceSound(), (this.block.stepSound.getVolume() + 1.0F) / 2.0F, this.block.stepSound.getFrequency() * 0.8F); --stack.stackSize; } return true; } else { return false; } } @Override public String getUnlocalizedName(ItemStack stack) { return super.getUnlocalizedName(stack); } } I should probably try to make a tutorial for this so that no one else has the same problem. I'm trying to make a pillar block that behaves like the existing log blocks. At the moment the only non-working thing seems to be the ItemBlock, because when I use /setblock nether_tree_wood <0, 1, 2> (where nether_tree_wood is the ID of the block and <0, 1, 2> are the possible metadatas) they get placed just fine with the proper rotations. This is the ItemBlock code I'm using: (code) If you think you need the rest of the code for this block, it's available on request. I just don't think it's necessary, though I could very well be wrong.
-
EDIT: It turns out I was dumb and forgot to set the item to use my class - it was using the default sword class. Hello, I'm trying to make a sword that launches the user and the target into the air. I successfully made an item like this in 1.6.4 sometime last year, but the code is no longer working for me. The sword itself loads in the game fine, the launching part just doesn't work. This is the code I'm using: @Override public boolean hitEntity(ItemStack stack, EntityLivingBase target, EntityLivingBase attacker) { target.setVelocity(0, 1, 0); attacker.setVelocity(0, 1, 0); stack.damageItem(1, attacker); return true; }